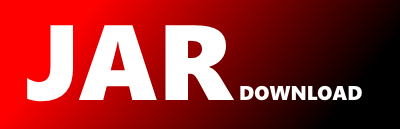
com.amazonaws.services.iottwinmaker.AWSIoTTwinMakerClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iottwinmaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iottwinmaker;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.iottwinmaker.AWSIoTTwinMakerClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.iottwinmaker.model.*;
import com.amazonaws.services.iottwinmaker.model.transform.*;
/**
* Client for accessing AWS IoT TwinMaker. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* IoT TwinMaker is a service with which you can build operational digital twins of physical systems. IoT TwinMaker
* overlays measurements and analysis from real-world sensors, cameras, and enterprise applications so you can create
* data visualizations to monitor your physical factory, building, or industrial plant. You can use this real-world data
* to monitor operations and diagnose and repair errors.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSIoTTwinMakerClient extends AmazonWebServiceClient implements AWSIoTTwinMaker {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSIoTTwinMaker.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "iottwinmaker";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConnectorFailureException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.ConnectorFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyTagsException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.TooManyTagsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConnectorTimeoutException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.ConnectorTimeoutExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("QueryTimeoutException").withExceptionUnmarshaller(
com.amazonaws.services.iottwinmaker.model.transform.QueryTimeoutExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.iottwinmaker.model.AWSIoTTwinMakerException.class));
public static AWSIoTTwinMakerClientBuilder builder() {
return AWSIoTTwinMakerClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS IoT TwinMaker using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSIoTTwinMakerClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS IoT TwinMaker using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSIoTTwinMakerClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("iottwinmaker.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/iottwinmaker/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/iottwinmaker/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Sets values for multiple time series properties.
*
*
* @param batchPutPropertyValuesRequest
* @return Result of the BatchPutPropertyValues operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.BatchPutPropertyValues
* @see AWS API Documentation
*/
@Override
public BatchPutPropertyValuesResult batchPutPropertyValues(BatchPutPropertyValuesRequest request) {
request = beforeClientExecution(request);
return executeBatchPutPropertyValues(request);
}
@SdkInternalApi
final BatchPutPropertyValuesResult executeBatchPutPropertyValues(BatchPutPropertyValuesRequest batchPutPropertyValuesRequest) {
ExecutionContext executionContext = createExecutionContext(batchPutPropertyValuesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchPutPropertyValuesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchPutPropertyValuesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchPutPropertyValues");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "data.";
String resolvedHostPrefix = String.format("data.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchPutPropertyValuesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels the metadata transfer job.
*
*
* @param cancelMetadataTransferJobRequest
* @return Result of the CancelMetadataTransferJob operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @sample AWSIoTTwinMaker.CancelMetadataTransferJob
* @see AWS API Documentation
*/
@Override
public CancelMetadataTransferJobResult cancelMetadataTransferJob(CancelMetadataTransferJobRequest request) {
request = beforeClientExecution(request);
return executeCancelMetadataTransferJob(request);
}
@SdkInternalApi
final CancelMetadataTransferJobResult executeCancelMetadataTransferJob(CancelMetadataTransferJobRequest cancelMetadataTransferJobRequest) {
ExecutionContext executionContext = createExecutionContext(cancelMetadataTransferJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelMetadataTransferJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(cancelMetadataTransferJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelMetadataTransferJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CancelMetadataTransferJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a component type.
*
*
* @param createComponentTypeRequest
* @return Result of the CreateComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateComponentType
* @see AWS API Documentation
*/
@Override
public CreateComponentTypeResult createComponentType(CreateComponentTypeRequest request) {
request = beforeClientExecution(request);
return executeCreateComponentType(request);
}
@SdkInternalApi
final CreateComponentTypeResult executeCreateComponentType(CreateComponentTypeRequest createComponentTypeRequest) {
ExecutionContext executionContext = createExecutionContext(createComponentTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateComponentTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createComponentTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateComponentType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateComponentTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an entity.
*
*
* @param createEntityRequest
* @return Result of the CreateEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateEntity
* @see AWS API
* Documentation
*/
@Override
public CreateEntityResult createEntity(CreateEntityRequest request) {
request = beforeClientExecution(request);
return executeCreateEntity(request);
}
@SdkInternalApi
final CreateEntityResult executeCreateEntity(CreateEntityRequest createEntityRequest) {
ExecutionContext executionContext = createExecutionContext(createEntityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEntityRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createEntityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEntity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateEntityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new metadata transfer job.
*
*
* @param createMetadataTransferJobRequest
* @return Result of the CreateMetadataTransferJob operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateMetadataTransferJob
* @see AWS API Documentation
*/
@Override
public CreateMetadataTransferJobResult createMetadataTransferJob(CreateMetadataTransferJobRequest request) {
request = beforeClientExecution(request);
return executeCreateMetadataTransferJob(request);
}
@SdkInternalApi
final CreateMetadataTransferJobResult executeCreateMetadataTransferJob(CreateMetadataTransferJobRequest createMetadataTransferJobRequest) {
ExecutionContext executionContext = createExecutionContext(createMetadataTransferJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMetadataTransferJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMetadataTransferJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMetadataTransferJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMetadataTransferJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a scene.
*
*
* @param createSceneRequest
* @return Result of the CreateScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateScene
* @see AWS API
* Documentation
*/
@Override
public CreateSceneResult createScene(CreateSceneRequest request) {
request = beforeClientExecution(request);
return executeCreateScene(request);
}
@SdkInternalApi
final CreateSceneResult executeCreateScene(CreateSceneRequest createSceneRequest) {
ExecutionContext executionContext = createExecutionContext(createSceneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSceneRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSceneRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateScene");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSceneResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action creates a SyncJob.
*
*
* @param createSyncJobRequest
* @return Result of the CreateSyncJob operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateSyncJob
* @see AWS API
* Documentation
*/
@Override
public CreateSyncJobResult createSyncJob(CreateSyncJobRequest request) {
request = beforeClientExecution(request);
return executeCreateSyncJob(request);
}
@SdkInternalApi
final CreateSyncJobResult executeCreateSyncJob(CreateSyncJobRequest createSyncJobRequest) {
ExecutionContext executionContext = createExecutionContext(createSyncJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSyncJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSyncJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSyncJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSyncJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a workplace.
*
*
* @param createWorkspaceRequest
* @return Result of the CreateWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.CreateWorkspace
* @see AWS
* API Documentation
*/
@Override
public CreateWorkspaceResult createWorkspace(CreateWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeCreateWorkspace(request);
}
@SdkInternalApi
final CreateWorkspaceResult executeCreateWorkspace(CreateWorkspaceRequest createWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(createWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a component type.
*
*
* @param deleteComponentTypeRequest
* @return Result of the DeleteComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.DeleteComponentType
* @see AWS API Documentation
*/
@Override
public DeleteComponentTypeResult deleteComponentType(DeleteComponentTypeRequest request) {
request = beforeClientExecution(request);
return executeDeleteComponentType(request);
}
@SdkInternalApi
final DeleteComponentTypeResult executeDeleteComponentType(DeleteComponentTypeRequest deleteComponentTypeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteComponentTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteComponentTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteComponentTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteComponentType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteComponentTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an entity.
*
*
* @param deleteEntityRequest
* @return Result of the DeleteEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.DeleteEntity
* @see AWS API
* Documentation
*/
@Override
public DeleteEntityResult deleteEntity(DeleteEntityRequest request) {
request = beforeClientExecution(request);
return executeDeleteEntity(request);
}
@SdkInternalApi
final DeleteEntityResult executeDeleteEntity(DeleteEntityRequest deleteEntityRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEntityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEntityRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteEntityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEntity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteEntityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a scene.
*
*
* @param deleteSceneRequest
* @return Result of the DeleteScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.DeleteScene
* @see AWS API
* Documentation
*/
@Override
public DeleteSceneResult deleteScene(DeleteSceneRequest request) {
request = beforeClientExecution(request);
return executeDeleteScene(request);
}
@SdkInternalApi
final DeleteSceneResult executeDeleteScene(DeleteSceneRequest deleteSceneRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSceneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSceneRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSceneRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteScene");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSceneResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete the SyncJob.
*
*
* @param deleteSyncJobRequest
* @return Result of the DeleteSyncJob operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.DeleteSyncJob
* @see AWS API
* Documentation
*/
@Override
public DeleteSyncJobResult deleteSyncJob(DeleteSyncJobRequest request) {
request = beforeClientExecution(request);
return executeDeleteSyncJob(request);
}
@SdkInternalApi
final DeleteSyncJobResult executeDeleteSyncJob(DeleteSyncJobRequest deleteSyncJobRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSyncJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSyncJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSyncJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSyncJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSyncJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a workspace.
*
*
* @param deleteWorkspaceRequest
* @return Result of the DeleteWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.DeleteWorkspace
* @see AWS
* API Documentation
*/
@Override
public DeleteWorkspaceResult deleteWorkspace(DeleteWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeDeleteWorkspace(request);
}
@SdkInternalApi
final DeleteWorkspaceResult executeDeleteWorkspace(DeleteWorkspaceRequest deleteWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Run queries to access information from your knowledge graph of entities within individual workspaces.
*
*
*
* The ExecuteQuery action only works with Amazon Web Services Java
* SDK2. ExecuteQuery will not work with any Amazon Web Services Java SDK version < 2.x.
*
*
*
* @param executeQueryRequest
* @return Result of the ExecuteQuery operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws QueryTimeoutException
* The query timeout exception.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.ExecuteQuery
* @see AWS API
* Documentation
*/
@Override
public ExecuteQueryResult executeQuery(ExecuteQueryRequest request) {
request = beforeClientExecution(request);
return executeExecuteQuery(request);
}
@SdkInternalApi
final ExecuteQueryResult executeExecuteQuery(ExecuteQueryRequest executeQueryRequest) {
ExecutionContext executionContext = createExecutionContext(executeQueryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ExecuteQueryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(executeQueryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ExecuteQuery");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ExecuteQueryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about a component type.
*
*
* @param getComponentTypeRequest
* @return Result of the GetComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.GetComponentType
* @see AWS
* API Documentation
*/
@Override
public GetComponentTypeResult getComponentType(GetComponentTypeRequest request) {
request = beforeClientExecution(request);
return executeGetComponentType(request);
}
@SdkInternalApi
final GetComponentTypeResult executeGetComponentType(GetComponentTypeRequest getComponentTypeRequest) {
ExecutionContext executionContext = createExecutionContext(getComponentTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetComponentTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getComponentTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetComponentType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetComponentTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about an entity.
*
*
* @param getEntityRequest
* @return Result of the GetEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.GetEntity
* @see AWS API
* Documentation
*/
@Override
public GetEntityResult getEntity(GetEntityRequest request) {
request = beforeClientExecution(request);
return executeGetEntity(request);
}
@SdkInternalApi
final GetEntityResult executeGetEntity(GetEntityRequest getEntityRequest) {
ExecutionContext executionContext = createExecutionContext(getEntityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEntityRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getEntityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEntity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetEntityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a nmetadata transfer job.
*
*
* @param getMetadataTransferJobRequest
* @return Result of the GetMetadataTransferJob operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.GetMetadataTransferJob
* @see AWS API Documentation
*/
@Override
public GetMetadataTransferJobResult getMetadataTransferJob(GetMetadataTransferJobRequest request) {
request = beforeClientExecution(request);
return executeGetMetadataTransferJob(request);
}
@SdkInternalApi
final GetMetadataTransferJobResult executeGetMetadataTransferJob(GetMetadataTransferJobRequest getMetadataTransferJobRequest) {
ExecutionContext executionContext = createExecutionContext(getMetadataTransferJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMetadataTransferJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMetadataTransferJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMetadataTransferJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetMetadataTransferJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the pricing plan.
*
*
* @param getPricingPlanRequest
* @return Result of the GetPricingPlan operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.GetPricingPlan
* @see AWS
* API Documentation
*/
@Override
public GetPricingPlanResult getPricingPlan(GetPricingPlanRequest request) {
request = beforeClientExecution(request);
return executeGetPricingPlan(request);
}
@SdkInternalApi
final GetPricingPlanResult executeGetPricingPlan(GetPricingPlanRequest getPricingPlanRequest) {
ExecutionContext executionContext = createExecutionContext(getPricingPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPricingPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPricingPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPricingPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetPricingPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the property values for a component, component type, entity, or workspace.
*
*
* You must specify a value for either componentName
, componentTypeId
,
* entityId
, or workspaceId
.
*
*
* @param getPropertyValueRequest
* @return Result of the GetPropertyValue operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ConnectorFailureException
* The connector failed.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConnectorTimeoutException
* The connector timed out.
* @sample AWSIoTTwinMaker.GetPropertyValue
* @see AWS
* API Documentation
*/
@Override
public GetPropertyValueResult getPropertyValue(GetPropertyValueRequest request) {
request = beforeClientExecution(request);
return executeGetPropertyValue(request);
}
@SdkInternalApi
final GetPropertyValueResult executeGetPropertyValue(GetPropertyValueRequest getPropertyValueRequest) {
ExecutionContext executionContext = createExecutionContext(getPropertyValueRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPropertyValueRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPropertyValueRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPropertyValue");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "data.";
String resolvedHostPrefix = String.format("data.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetPropertyValueResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the history of a time series property value for a component, component type, entity,
* or workspace.
*
*
* You must specify a value for workspaceId
. For entity-specific queries, specify values for
* componentName
and entityId
. For cross-entity quries, specify a value for
* componentTypeId
.
*
*
* @param getPropertyValueHistoryRequest
* @return Result of the GetPropertyValueHistory operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ConnectorFailureException
* The connector failed.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConnectorTimeoutException
* The connector timed out.
* @sample AWSIoTTwinMaker.GetPropertyValueHistory
* @see AWS API Documentation
*/
@Override
public GetPropertyValueHistoryResult getPropertyValueHistory(GetPropertyValueHistoryRequest request) {
request = beforeClientExecution(request);
return executeGetPropertyValueHistory(request);
}
@SdkInternalApi
final GetPropertyValueHistoryResult executeGetPropertyValueHistory(GetPropertyValueHistoryRequest getPropertyValueHistoryRequest) {
ExecutionContext executionContext = createExecutionContext(getPropertyValueHistoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPropertyValueHistoryRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getPropertyValueHistoryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPropertyValueHistory");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "data.";
String resolvedHostPrefix = String.format("data.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetPropertyValueHistoryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about a scene.
*
*
* @param getSceneRequest
* @return Result of the GetScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.GetScene
* @see AWS API
* Documentation
*/
@Override
public GetSceneResult getScene(GetSceneRequest request) {
request = beforeClientExecution(request);
return executeGetScene(request);
}
@SdkInternalApi
final GetSceneResult executeGetScene(GetSceneRequest getSceneRequest) {
ExecutionContext executionContext = createExecutionContext(getSceneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSceneRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSceneRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetScene");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSceneResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the SyncJob.
*
*
* @param getSyncJobRequest
* @return Result of the GetSyncJob operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.GetSyncJob
* @see AWS API
* Documentation
*/
@Override
public GetSyncJobResult getSyncJob(GetSyncJobRequest request) {
request = beforeClientExecution(request);
return executeGetSyncJob(request);
}
@SdkInternalApi
final GetSyncJobResult executeGetSyncJob(GetSyncJobRequest getSyncJobRequest) {
ExecutionContext executionContext = createExecutionContext(getSyncJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSyncJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSyncJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSyncJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSyncJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about a workspace.
*
*
* @param getWorkspaceRequest
* @return Result of the GetWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.GetWorkspace
* @see AWS API
* Documentation
*/
@Override
public GetWorkspaceResult getWorkspace(GetWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeGetWorkspace(request);
}
@SdkInternalApi
final GetWorkspaceResult executeGetWorkspace(GetWorkspaceRequest getWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(getWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all component types in a workspace.
*
*
* @param listComponentTypesRequest
* @return Result of the ListComponentTypes operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.ListComponentTypes
* @see AWS API Documentation
*/
@Override
public ListComponentTypesResult listComponentTypes(ListComponentTypesRequest request) {
request = beforeClientExecution(request);
return executeListComponentTypes(request);
}
@SdkInternalApi
final ListComponentTypesResult executeListComponentTypes(ListComponentTypesRequest listComponentTypesRequest) {
ExecutionContext executionContext = createExecutionContext(listComponentTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListComponentTypesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listComponentTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListComponentTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListComponentTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This API lists the components of an entity.
*
*
* @param listComponentsRequest
* @return Result of the ListComponents operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.ListComponents
* @see AWS
* API Documentation
*/
@Override
public ListComponentsResult listComponents(ListComponentsRequest request) {
request = beforeClientExecution(request);
return executeListComponents(request);
}
@SdkInternalApi
final ListComponentsResult executeListComponents(ListComponentsRequest listComponentsRequest) {
ExecutionContext executionContext = createExecutionContext(listComponentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListComponentsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listComponentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListComponents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListComponentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all entities in a workspace.
*
*
* @param listEntitiesRequest
* @return Result of the ListEntities operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.ListEntities
* @see AWS API
* Documentation
*/
@Override
public ListEntitiesResult listEntities(ListEntitiesRequest request) {
request = beforeClientExecution(request);
return executeListEntities(request);
}
@SdkInternalApi
final ListEntitiesResult executeListEntities(ListEntitiesRequest listEntitiesRequest) {
ExecutionContext executionContext = createExecutionContext(listEntitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEntitiesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listEntitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEntities");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListEntitiesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the metadata transfer jobs.
*
*
* @param listMetadataTransferJobsRequest
* @return Result of the ListMetadataTransferJobs operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.ListMetadataTransferJobs
* @see AWS API Documentation
*/
@Override
public ListMetadataTransferJobsResult listMetadataTransferJobs(ListMetadataTransferJobsRequest request) {
request = beforeClientExecution(request);
return executeListMetadataTransferJobs(request);
}
@SdkInternalApi
final ListMetadataTransferJobsResult executeListMetadataTransferJobs(ListMetadataTransferJobsRequest listMetadataTransferJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listMetadataTransferJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMetadataTransferJobsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listMetadataTransferJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMetadataTransferJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListMetadataTransferJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This API lists the properties of a component.
*
*
* @param listPropertiesRequest
* @return Result of the ListProperties operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.ListProperties
* @see AWS
* API Documentation
*/
@Override
public ListPropertiesResult listProperties(ListPropertiesRequest request) {
request = beforeClientExecution(request);
return executeListProperties(request);
}
@SdkInternalApi
final ListPropertiesResult executeListProperties(ListPropertiesRequest listPropertiesRequest) {
ExecutionContext executionContext = createExecutionContext(listPropertiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPropertiesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPropertiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListProperties");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPropertiesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all scenes in a workspace.
*
*
* @param listScenesRequest
* @return Result of the ListScenes operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.ListScenes
* @see AWS API
* Documentation
*/
@Override
public ListScenesResult listScenes(ListScenesRequest request) {
request = beforeClientExecution(request);
return executeListScenes(request);
}
@SdkInternalApi
final ListScenesResult executeListScenes(ListScenesRequest listScenesRequest) {
ExecutionContext executionContext = createExecutionContext(listScenesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListScenesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listScenesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListScenes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListScenesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all SyncJobs.
*
*
* @param listSyncJobsRequest
* @return Result of the ListSyncJobs operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.ListSyncJobs
* @see AWS API
* Documentation
*/
@Override
public ListSyncJobsResult listSyncJobs(ListSyncJobsRequest request) {
request = beforeClientExecution(request);
return executeListSyncJobs(request);
}
@SdkInternalApi
final ListSyncJobsResult executeListSyncJobs(ListSyncJobsRequest listSyncJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listSyncJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSyncJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSyncJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSyncJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSyncJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the sync resources.
*
*
* @param listSyncResourcesRequest
* @return Result of the ListSyncResources operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.ListSyncResources
* @see AWS
* API Documentation
*/
@Override
public ListSyncResourcesResult listSyncResources(ListSyncResourcesRequest request) {
request = beforeClientExecution(request);
return executeListSyncResources(request);
}
@SdkInternalApi
final ListSyncResourcesResult executeListSyncResources(ListSyncResourcesRequest listSyncResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listSyncResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSyncResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSyncResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSyncResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSyncResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all tags associated with a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @sample AWSIoTTwinMaker.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about workspaces in the current account.
*
*
* @param listWorkspacesRequest
* @return Result of the ListWorkspaces operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.ListWorkspaces
* @see AWS
* API Documentation
*/
@Override
public ListWorkspacesResult listWorkspaces(ListWorkspacesRequest request) {
request = beforeClientExecution(request);
return executeListWorkspaces(request);
}
@SdkInternalApi
final ListWorkspacesResult executeListWorkspaces(ListWorkspacesRequest listWorkspacesRequest) {
ExecutionContext executionContext = createExecutionContext(listWorkspacesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWorkspacesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listWorkspacesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListWorkspaces");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListWorkspacesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws TooManyTagsException
* The number of tags exceeds the limit.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @sample AWSIoTTwinMaker.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @sample AWSIoTTwinMaker.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates information in a component type.
*
*
* @param updateComponentTypeRequest
* @return Result of the UpdateComponentType operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.UpdateComponentType
* @see AWS API Documentation
*/
@Override
public UpdateComponentTypeResult updateComponentType(UpdateComponentTypeRequest request) {
request = beforeClientExecution(request);
return executeUpdateComponentType(request);
}
@SdkInternalApi
final UpdateComponentTypeResult executeUpdateComponentType(UpdateComponentTypeRequest updateComponentTypeRequest) {
ExecutionContext executionContext = createExecutionContext(updateComponentTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateComponentTypeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateComponentTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateComponentType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateComponentTypeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an entity.
*
*
* @param updateEntityRequest
* @return Result of the UpdateEntity operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ConflictException
* A conflict occurred.
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.UpdateEntity
* @see AWS API
* Documentation
*/
@Override
public UpdateEntityResult updateEntity(UpdateEntityRequest request) {
request = beforeClientExecution(request);
return executeUpdateEntity(request);
}
@SdkInternalApi
final UpdateEntityResult executeUpdateEntity(UpdateEntityRequest updateEntityRequest) {
ExecutionContext executionContext = createExecutionContext(updateEntityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateEntityRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateEntityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateEntity");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateEntityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Update the pricing plan.
*
*
* @param updatePricingPlanRequest
* @return Result of the UpdatePricingPlan operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.UpdatePricingPlan
* @see AWS
* API Documentation
*/
@Override
public UpdatePricingPlanResult updatePricingPlan(UpdatePricingPlanRequest request) {
request = beforeClientExecution(request);
return executeUpdatePricingPlan(request);
}
@SdkInternalApi
final UpdatePricingPlanResult executeUpdatePricingPlan(UpdatePricingPlanRequest updatePricingPlanRequest) {
ExecutionContext executionContext = createExecutionContext(updatePricingPlanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdatePricingPlanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updatePricingPlanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdatePricingPlan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdatePricingPlanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a scene.
*
*
* @param updateSceneRequest
* @return Result of the UpdateScene operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @sample AWSIoTTwinMaker.UpdateScene
* @see AWS API
* Documentation
*/
@Override
public UpdateSceneResult updateScene(UpdateSceneRequest request) {
request = beforeClientExecution(request);
return executeUpdateScene(request);
}
@SdkInternalApi
final UpdateSceneResult executeUpdateScene(UpdateSceneRequest updateSceneRequest) {
ExecutionContext executionContext = createExecutionContext(updateSceneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSceneRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateSceneRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateScene");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateSceneResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a workspace.
*
*
* @param updateWorkspaceRequest
* @return Result of the UpdateWorkspace operation returned by the service.
* @throws InternalServerException
* An unexpected error has occurred.
* @throws AccessDeniedException
* Access is denied.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The rate exceeds the limit.
* @throws ValidationException
* Failed
* @throws ServiceQuotaExceededException
* The service quota was exceeded.
* @sample AWSIoTTwinMaker.UpdateWorkspace
* @see AWS
* API Documentation
*/
@Override
public UpdateWorkspaceResult updateWorkspace(UpdateWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeUpdateWorkspace(request);
}
@SdkInternalApi
final UpdateWorkspaceResult executeUpdateWorkspace(UpdateWorkspaceRequest updateWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(updateWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoTTwinMaker");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "api.";
String resolvedHostPrefix = String.format("api.");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}