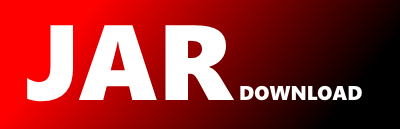
com.amazonaws.services.iotwireless.AWSIoTWireless Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotwireless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotwireless;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.iotwireless.model.*;
/**
* Interface for accessing AWS IoT Wireless.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.iotwireless.AbstractAWSIoTWireless} instead.
*
*
*
* AWS IoT Wireless provides bi-directional communication between internet-connected wireless devices and the AWS Cloud.
* To onboard both LoRaWAN and Sidewalk devices to AWS IoT, use the IoT Wireless API. These wireless devices use the Low
* Power Wide Area Networking (LPWAN) communication protocol to communicate with AWS IoT.
*
*
* Using the API, you can perform create, read, update, and delete operations for your wireless devices, gateways,
* destinations, and profiles. After onboarding your devices, you can use the API operations to set log levels and
* monitor your devices with CloudWatch.
*
*
* You can also use the API operations to create multicast groups and schedule a multicast session for sending a
* downlink message to devices in the group. By using Firmware Updates Over-The-Air (FUOTA) API operations, you can
* create a FUOTA task and schedule a session to update the firmware of individual devices or an entire group of devices
* in a multicast group.
*
*
* To connect to the AWS IoT Wireless Service, use the Service endpoints as described in IoT Wireless Service
* endpoints in the AWS General Reference.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSIoTWireless {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "api.iotwireless";
/**
*
* Associates a partner account with your AWS account.
*
*
* @param associateAwsAccountWithPartnerAccountRequest
* @return Result of the AssociateAwsAccountWithPartnerAccount operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.AssociateAwsAccountWithPartnerAccount
* @see AWS API Documentation
*/
AssociateAwsAccountWithPartnerAccountResult associateAwsAccountWithPartnerAccount(
AssociateAwsAccountWithPartnerAccountRequest associateAwsAccountWithPartnerAccountRequest);
/**
*
* Associate a multicast group with a FUOTA task.
*
*
* @param associateMulticastGroupWithFuotaTaskRequest
* @return Result of the AssociateMulticastGroupWithFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateMulticastGroupWithFuotaTask
* @see AWS API Documentation
*/
AssociateMulticastGroupWithFuotaTaskResult associateMulticastGroupWithFuotaTask(
AssociateMulticastGroupWithFuotaTaskRequest associateMulticastGroupWithFuotaTaskRequest);
/**
*
* Associate a wireless device with a FUOTA task.
*
*
* @param associateWirelessDeviceWithFuotaTaskRequest
* @return Result of the AssociateWirelessDeviceWithFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessDeviceWithFuotaTask
* @see AWS API Documentation
*/
AssociateWirelessDeviceWithFuotaTaskResult associateWirelessDeviceWithFuotaTask(
AssociateWirelessDeviceWithFuotaTaskRequest associateWirelessDeviceWithFuotaTaskRequest);
/**
*
* Associates a wireless device with a multicast group.
*
*
* @param associateWirelessDeviceWithMulticastGroupRequest
* @return Result of the AssociateWirelessDeviceWithMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessDeviceWithMulticastGroup
* @see AWS API Documentation
*/
AssociateWirelessDeviceWithMulticastGroupResult associateWirelessDeviceWithMulticastGroup(
AssociateWirelessDeviceWithMulticastGroupRequest associateWirelessDeviceWithMulticastGroupRequest);
/**
*
* Associates a wireless device with a thing.
*
*
* @param associateWirelessDeviceWithThingRequest
* @return Result of the AssociateWirelessDeviceWithThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessDeviceWithThing
* @see AWS API Documentation
*/
AssociateWirelessDeviceWithThingResult associateWirelessDeviceWithThing(AssociateWirelessDeviceWithThingRequest associateWirelessDeviceWithThingRequest);
/**
*
* Associates a wireless gateway with a certificate.
*
*
* @param associateWirelessGatewayWithCertificateRequest
* @return Result of the AssociateWirelessGatewayWithCertificate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.AssociateWirelessGatewayWithCertificate
* @see AWS API Documentation
*/
AssociateWirelessGatewayWithCertificateResult associateWirelessGatewayWithCertificate(
AssociateWirelessGatewayWithCertificateRequest associateWirelessGatewayWithCertificateRequest);
/**
*
* Associates a wireless gateway with a thing.
*
*
* @param associateWirelessGatewayWithThingRequest
* @return Result of the AssociateWirelessGatewayWithThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessGatewayWithThing
* @see AWS API Documentation
*/
AssociateWirelessGatewayWithThingResult associateWirelessGatewayWithThing(AssociateWirelessGatewayWithThingRequest associateWirelessGatewayWithThingRequest);
/**
*
* Cancels an existing multicast group session.
*
*
* @param cancelMulticastGroupSessionRequest
* @return Result of the CancelMulticastGroupSession operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CancelMulticastGroupSession
* @see AWS API Documentation
*/
CancelMulticastGroupSessionResult cancelMulticastGroupSession(CancelMulticastGroupSessionRequest cancelMulticastGroupSessionRequest);
/**
*
* Creates a new destination that maps a device message to an AWS IoT rule.
*
*
* @param createDestinationRequest
* @return Result of the CreateDestination operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateDestination
* @see AWS
* API Documentation
*/
CreateDestinationResult createDestination(CreateDestinationRequest createDestinationRequest);
/**
*
* Creates a new device profile.
*
*
* @param createDeviceProfileRequest
* @return Result of the CreateDeviceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateDeviceProfile
* @see AWS API Documentation
*/
CreateDeviceProfileResult createDeviceProfile(CreateDeviceProfileRequest createDeviceProfileRequest);
/**
*
* Creates a FUOTA task.
*
*
* @param createFuotaTaskRequest
* @return Result of the CreateFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateFuotaTask
* @see AWS
* API Documentation
*/
CreateFuotaTaskResult createFuotaTask(CreateFuotaTaskRequest createFuotaTaskRequest);
/**
*
* Creates a multicast group.
*
*
* @param createMulticastGroupRequest
* @return Result of the CreateMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateMulticastGroup
* @see AWS API Documentation
*/
CreateMulticastGroupResult createMulticastGroup(CreateMulticastGroupRequest createMulticastGroupRequest);
/**
*
* Creates a new network analyzer configuration.
*
*
* @param createNetworkAnalyzerConfigurationRequest
* @return Result of the CreateNetworkAnalyzerConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateNetworkAnalyzerConfiguration
* @see AWS API Documentation
*/
CreateNetworkAnalyzerConfigurationResult createNetworkAnalyzerConfiguration(
CreateNetworkAnalyzerConfigurationRequest createNetworkAnalyzerConfigurationRequest);
/**
*
* Creates a new service profile.
*
*
* @param createServiceProfileRequest
* @return Result of the CreateServiceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateServiceProfile
* @see AWS API Documentation
*/
CreateServiceProfileResult createServiceProfile(CreateServiceProfileRequest createServiceProfileRequest);
/**
*
* Provisions a wireless device.
*
*
* @param createWirelessDeviceRequest
* @return Result of the CreateWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessDevice
* @see AWS API Documentation
*/
CreateWirelessDeviceResult createWirelessDevice(CreateWirelessDeviceRequest createWirelessDeviceRequest);
/**
*
* Provisions a wireless gateway.
*
*
*
* When provisioning a wireless gateway, you might run into duplication errors for the following reasons.
*
*
* -
*
* If you specify a GatewayEui
value that already exists.
*
*
* -
*
* If you used a ClientRequestToken
with the same parameters within the last 10 minutes.
*
*
*
*
* To avoid this error, make sure that you use unique identifiers and parameters for each request within the
* specified time period.
*
*
*
* @param createWirelessGatewayRequest
* @return Result of the CreateWirelessGateway operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessGateway
* @see AWS API Documentation
*/
CreateWirelessGatewayResult createWirelessGateway(CreateWirelessGatewayRequest createWirelessGatewayRequest);
/**
*
* Creates a task for a wireless gateway.
*
*
* @param createWirelessGatewayTaskRequest
* @return Result of the CreateWirelessGatewayTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessGatewayTask
* @see AWS API Documentation
*/
CreateWirelessGatewayTaskResult createWirelessGatewayTask(CreateWirelessGatewayTaskRequest createWirelessGatewayTaskRequest);
/**
*
* Creates a gateway task definition.
*
*
* @param createWirelessGatewayTaskDefinitionRequest
* @return Result of the CreateWirelessGatewayTaskDefinition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessGatewayTaskDefinition
* @see AWS API Documentation
*/
CreateWirelessGatewayTaskDefinitionResult createWirelessGatewayTaskDefinition(
CreateWirelessGatewayTaskDefinitionRequest createWirelessGatewayTaskDefinitionRequest);
/**
*
* Deletes a destination.
*
*
* @param deleteDestinationRequest
* @return Result of the DeleteDestination operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteDestination
* @see AWS
* API Documentation
*/
DeleteDestinationResult deleteDestination(DeleteDestinationRequest deleteDestinationRequest);
/**
*
* Deletes a device profile.
*
*
* @param deleteDeviceProfileRequest
* @return Result of the DeleteDeviceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteDeviceProfile
* @see AWS API Documentation
*/
DeleteDeviceProfileResult deleteDeviceProfile(DeleteDeviceProfileRequest deleteDeviceProfileRequest);
/**
*
* Deletes a FUOTA task.
*
*
* @param deleteFuotaTaskRequest
* @return Result of the DeleteFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteFuotaTask
* @see AWS
* API Documentation
*/
DeleteFuotaTaskResult deleteFuotaTask(DeleteFuotaTaskRequest deleteFuotaTaskRequest);
/**
*
* Deletes a multicast group if it is not in use by a fuota task.
*
*
* @param deleteMulticastGroupRequest
* @return Result of the DeleteMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteMulticastGroup
* @see AWS API Documentation
*/
DeleteMulticastGroupResult deleteMulticastGroup(DeleteMulticastGroupRequest deleteMulticastGroupRequest);
/**
*
* Deletes a network analyzer configuration.
*
*
* @param deleteNetworkAnalyzerConfigurationRequest
* @return Result of the DeleteNetworkAnalyzerConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteNetworkAnalyzerConfiguration
* @see AWS API Documentation
*/
DeleteNetworkAnalyzerConfigurationResult deleteNetworkAnalyzerConfiguration(
DeleteNetworkAnalyzerConfigurationRequest deleteNetworkAnalyzerConfigurationRequest);
/**
*
* Remove queued messages from the downlink queue.
*
*
* @param deleteQueuedMessagesRequest
* @return Result of the DeleteQueuedMessages operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.DeleteQueuedMessages
* @see AWS API Documentation
*/
DeleteQueuedMessagesResult deleteQueuedMessages(DeleteQueuedMessagesRequest deleteQueuedMessagesRequest);
/**
*
* Deletes a service profile.
*
*
* @param deleteServiceProfileRequest
* @return Result of the DeleteServiceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteServiceProfile
* @see AWS API Documentation
*/
DeleteServiceProfileResult deleteServiceProfile(DeleteServiceProfileRequest deleteServiceProfileRequest);
/**
*
* Deletes a wireless device.
*
*
* @param deleteWirelessDeviceRequest
* @return Result of the DeleteWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessDevice
* @see AWS API Documentation
*/
DeleteWirelessDeviceResult deleteWirelessDevice(DeleteWirelessDeviceRequest deleteWirelessDeviceRequest);
/**
*
* Delete an import task.
*
*
* @param deleteWirelessDeviceImportTaskRequest
* @return Result of the DeleteWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessDeviceImportTask
* @see AWS API Documentation
*/
DeleteWirelessDeviceImportTaskResult deleteWirelessDeviceImportTask(DeleteWirelessDeviceImportTaskRequest deleteWirelessDeviceImportTaskRequest);
/**
*
* Deletes a wireless gateway.
*
*
*
* When deleting a wireless gateway, you might run into duplication errors for the following reasons.
*
*
* -
*
* If you specify a GatewayEui
value that already exists.
*
*
* -
*
* If you used a ClientRequestToken
with the same parameters within the last 10 minutes.
*
*
*
*
* To avoid this error, make sure that you use unique identifiers and parameters for each request within the
* specified time period.
*
*
*
* @param deleteWirelessGatewayRequest
* @return Result of the DeleteWirelessGateway operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessGateway
* @see AWS API Documentation
*/
DeleteWirelessGatewayResult deleteWirelessGateway(DeleteWirelessGatewayRequest deleteWirelessGatewayRequest);
/**
*
* Deletes a wireless gateway task.
*
*
* @param deleteWirelessGatewayTaskRequest
* @return Result of the DeleteWirelessGatewayTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessGatewayTask
* @see AWS API Documentation
*/
DeleteWirelessGatewayTaskResult deleteWirelessGatewayTask(DeleteWirelessGatewayTaskRequest deleteWirelessGatewayTaskRequest);
/**
*
* Deletes a wireless gateway task definition. Deleting this task definition does not affect tasks that are
* currently in progress.
*
*
* @param deleteWirelessGatewayTaskDefinitionRequest
* @return Result of the DeleteWirelessGatewayTaskDefinition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessGatewayTaskDefinition
* @see AWS API Documentation
*/
DeleteWirelessGatewayTaskDefinitionResult deleteWirelessGatewayTaskDefinition(
DeleteWirelessGatewayTaskDefinitionRequest deleteWirelessGatewayTaskDefinitionRequest);
/**
*
* Deregister a wireless device from AWS IoT Wireless.
*
*
* @param deregisterWirelessDeviceRequest
* @return Result of the DeregisterWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeregisterWirelessDevice
* @see AWS API Documentation
*/
DeregisterWirelessDeviceResult deregisterWirelessDevice(DeregisterWirelessDeviceRequest deregisterWirelessDeviceRequest);
/**
*
* Disassociates your AWS account from a partner account. If PartnerAccountId
and
* PartnerType
are null
, disassociates your AWS account from all partner accounts.
*
*
* @param disassociateAwsAccountFromPartnerAccountRequest
* @return Result of the DisassociateAwsAccountFromPartnerAccount operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DisassociateAwsAccountFromPartnerAccount
* @see AWS API Documentation
*/
DisassociateAwsAccountFromPartnerAccountResult disassociateAwsAccountFromPartnerAccount(
DisassociateAwsAccountFromPartnerAccountRequest disassociateAwsAccountFromPartnerAccountRequest);
/**
*
* Disassociates a multicast group from a fuota task.
*
*
* @param disassociateMulticastGroupFromFuotaTaskRequest
* @return Result of the DisassociateMulticastGroupFromFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateMulticastGroupFromFuotaTask
* @see AWS API Documentation
*/
DisassociateMulticastGroupFromFuotaTaskResult disassociateMulticastGroupFromFuotaTask(
DisassociateMulticastGroupFromFuotaTaskRequest disassociateMulticastGroupFromFuotaTaskRequest);
/**
*
* Disassociates a wireless device from a FUOTA task.
*
*
* @param disassociateWirelessDeviceFromFuotaTaskRequest
* @return Result of the DisassociateWirelessDeviceFromFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessDeviceFromFuotaTask
* @see AWS API Documentation
*/
DisassociateWirelessDeviceFromFuotaTaskResult disassociateWirelessDeviceFromFuotaTask(
DisassociateWirelessDeviceFromFuotaTaskRequest disassociateWirelessDeviceFromFuotaTaskRequest);
/**
*
* Disassociates a wireless device from a multicast group.
*
*
* @param disassociateWirelessDeviceFromMulticastGroupRequest
* @return Result of the DisassociateWirelessDeviceFromMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessDeviceFromMulticastGroup
* @see AWS API Documentation
*/
DisassociateWirelessDeviceFromMulticastGroupResult disassociateWirelessDeviceFromMulticastGroup(
DisassociateWirelessDeviceFromMulticastGroupRequest disassociateWirelessDeviceFromMulticastGroupRequest);
/**
*
* Disassociates a wireless device from its currently associated thing.
*
*
* @param disassociateWirelessDeviceFromThingRequest
* @return Result of the DisassociateWirelessDeviceFromThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessDeviceFromThing
* @see AWS API Documentation
*/
DisassociateWirelessDeviceFromThingResult disassociateWirelessDeviceFromThing(
DisassociateWirelessDeviceFromThingRequest disassociateWirelessDeviceFromThingRequest);
/**
*
* Disassociates a wireless gateway from its currently associated certificate.
*
*
* @param disassociateWirelessGatewayFromCertificateRequest
* @return Result of the DisassociateWirelessGatewayFromCertificate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DisassociateWirelessGatewayFromCertificate
* @see AWS API Documentation
*/
DisassociateWirelessGatewayFromCertificateResult disassociateWirelessGatewayFromCertificate(
DisassociateWirelessGatewayFromCertificateRequest disassociateWirelessGatewayFromCertificateRequest);
/**
*
* Disassociates a wireless gateway from its currently associated thing.
*
*
* @param disassociateWirelessGatewayFromThingRequest
* @return Result of the DisassociateWirelessGatewayFromThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessGatewayFromThing
* @see AWS API Documentation
*/
DisassociateWirelessGatewayFromThingResult disassociateWirelessGatewayFromThing(
DisassociateWirelessGatewayFromThingRequest disassociateWirelessGatewayFromThingRequest);
/**
*
* Gets information about a destination.
*
*
* @param getDestinationRequest
* @return Result of the GetDestination operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetDestination
* @see AWS API
* Documentation
*/
GetDestinationResult getDestination(GetDestinationRequest getDestinationRequest);
/**
*
* Gets information about a device profile.
*
*
* @param getDeviceProfileRequest
* @return Result of the GetDeviceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetDeviceProfile
* @see AWS
* API Documentation
*/
GetDeviceProfileResult getDeviceProfile(GetDeviceProfileRequest getDeviceProfileRequest);
/**
*
* Get the event configuration based on resource types.
*
*
* @param getEventConfigurationByResourceTypesRequest
* @return Result of the GetEventConfigurationByResourceTypes operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetEventConfigurationByResourceTypes
* @see AWS API Documentation
*/
GetEventConfigurationByResourceTypesResult getEventConfigurationByResourceTypes(
GetEventConfigurationByResourceTypesRequest getEventConfigurationByResourceTypesRequest);
/**
*
* Gets information about a FUOTA task.
*
*
* @param getFuotaTaskRequest
* @return Result of the GetFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetFuotaTask
* @see AWS API
* Documentation
*/
GetFuotaTaskResult getFuotaTask(GetFuotaTaskRequest getFuotaTaskRequest);
/**
*
* Returns current default log levels or log levels by resource types. Based on resource types, log levels can be
* for wireless device log options or wireless gateway log options.
*
*
* @param getLogLevelsByResourceTypesRequest
* @return Result of the GetLogLevelsByResourceTypes operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.GetLogLevelsByResourceTypes
* @see AWS API Documentation
*/
GetLogLevelsByResourceTypesResult getLogLevelsByResourceTypes(GetLogLevelsByResourceTypesRequest getLogLevelsByResourceTypesRequest);
/**
*
* Get the metric configuration status for this AWS account.
*
*
* @param getMetricConfigurationRequest
* @return Result of the GetMetricConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMetricConfiguration
* @see AWS API Documentation
*/
GetMetricConfigurationResult getMetricConfiguration(GetMetricConfigurationRequest getMetricConfigurationRequest);
/**
*
* Get the summary metrics for this AWS account.
*
*
* @param getMetricsRequest
* @return Result of the GetMetrics operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMetrics
* @see AWS API
* Documentation
*/
GetMetricsResult getMetrics(GetMetricsRequest getMetricsRequest);
/**
*
* Gets information about a multicast group.
*
*
* @param getMulticastGroupRequest
* @return Result of the GetMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMulticastGroup
* @see AWS
* API Documentation
*/
GetMulticastGroupResult getMulticastGroup(GetMulticastGroupRequest getMulticastGroupRequest);
/**
*
* Gets information about a multicast group session.
*
*
* @param getMulticastGroupSessionRequest
* @return Result of the GetMulticastGroupSession operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMulticastGroupSession
* @see AWS API Documentation
*/
GetMulticastGroupSessionResult getMulticastGroupSession(GetMulticastGroupSessionRequest getMulticastGroupSessionRequest);
/**
*
* Get network analyzer configuration.
*
*
* @param getNetworkAnalyzerConfigurationRequest
* @return Result of the GetNetworkAnalyzerConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetNetworkAnalyzerConfiguration
* @see AWS API Documentation
*/
GetNetworkAnalyzerConfigurationResult getNetworkAnalyzerConfiguration(GetNetworkAnalyzerConfigurationRequest getNetworkAnalyzerConfigurationRequest);
/**
*
* Gets information about a partner account. If PartnerAccountId
and PartnerType
are
* null
, returns all partner accounts.
*
*
* @param getPartnerAccountRequest
* @return Result of the GetPartnerAccount operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetPartnerAccount
* @see AWS
* API Documentation
*/
GetPartnerAccountResult getPartnerAccount(GetPartnerAccountRequest getPartnerAccountRequest);
/**
*
* Get the position information for a given resource.
*
*
*
* This action is no longer supported. Calls to retrieve the position information should use the GetResourcePosition API operation instead.
*
*
*
* @param getPositionRequest
* @return Result of the GetPosition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetPosition
* @see AWS API
* Documentation
*/
@Deprecated
GetPositionResult getPosition(GetPositionRequest getPositionRequest);
/**
*
* Get position configuration for a given resource.
*
*
*
* This action is no longer supported. Calls to retrieve the position configuration should use the GetResourcePosition API operation instead.
*
*
*
* @param getPositionConfigurationRequest
* @return Result of the GetPositionConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetPositionConfiguration
* @see AWS API Documentation
*/
@Deprecated
GetPositionConfigurationResult getPositionConfiguration(GetPositionConfigurationRequest getPositionConfigurationRequest);
/**
*
* Get estimated position information as a payload in GeoJSON format. The payload measurement data is resolved using
* solvers that are provided by third-party vendors.
*
*
* @param getPositionEstimateRequest
* @return Result of the GetPositionEstimate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetPositionEstimate
* @see AWS API Documentation
*/
GetPositionEstimateResult getPositionEstimate(GetPositionEstimateRequest getPositionEstimateRequest);
/**
*
* Get the event configuration for a particular resource identifier.
*
*
* @param getResourceEventConfigurationRequest
* @return Result of the GetResourceEventConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetResourceEventConfiguration
* @see AWS API Documentation
*/
GetResourceEventConfigurationResult getResourceEventConfiguration(GetResourceEventConfigurationRequest getResourceEventConfigurationRequest);
/**
*
* Fetches the log-level override, if any, for a given resource-ID and resource-type. It can be used for a wireless
* device or a wireless gateway.
*
*
* @param getResourceLogLevelRequest
* @return Result of the GetResourceLogLevel operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.GetResourceLogLevel
* @see AWS API Documentation
*/
GetResourceLogLevelResult getResourceLogLevel(GetResourceLogLevelRequest getResourceLogLevelRequest);
/**
*
* Get the position information for a given wireless device or a wireless gateway resource. The position information
* uses the World Geodetic System (WGS84).
*
*
* @param getResourcePositionRequest
* @return Result of the GetResourcePosition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetResourcePosition
* @see AWS API Documentation
*/
GetResourcePositionResult getResourcePosition(GetResourcePositionRequest getResourcePositionRequest);
/**
*
* Gets the account-specific endpoint for Configuration and Update Server (CUPS) protocol or LoRaWAN Network Server
* (LNS) connections.
*
*
* @param getServiceEndpointRequest
* @return Result of the GetServiceEndpoint operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetServiceEndpoint
* @see AWS
* API Documentation
*/
GetServiceEndpointResult getServiceEndpoint(GetServiceEndpointRequest getServiceEndpointRequest);
/**
*
* Gets information about a service profile.
*
*
* @param getServiceProfileRequest
* @return Result of the GetServiceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetServiceProfile
* @see AWS
* API Documentation
*/
GetServiceProfileResult getServiceProfile(GetServiceProfileRequest getServiceProfileRequest);
/**
*
* Gets information about a wireless device.
*
*
* @param getWirelessDeviceRequest
* @return Result of the GetWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessDevice
* @see AWS
* API Documentation
*/
GetWirelessDeviceResult getWirelessDevice(GetWirelessDeviceRequest getWirelessDeviceRequest);
/**
*
* Get information about an import task and count of device onboarding summary information for the import task.
*
*
* @param getWirelessDeviceImportTaskRequest
* @return Result of the GetWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessDeviceImportTask
* @see AWS API Documentation
*/
GetWirelessDeviceImportTaskResult getWirelessDeviceImportTask(GetWirelessDeviceImportTaskRequest getWirelessDeviceImportTaskRequest);
/**
*
* Gets operating information about a wireless device.
*
*
* @param getWirelessDeviceStatisticsRequest
* @return Result of the GetWirelessDeviceStatistics operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessDeviceStatistics
* @see AWS API Documentation
*/
GetWirelessDeviceStatisticsResult getWirelessDeviceStatistics(GetWirelessDeviceStatisticsRequest getWirelessDeviceStatisticsRequest);
/**
*
* Gets information about a wireless gateway.
*
*
* @param getWirelessGatewayRequest
* @return Result of the GetWirelessGateway operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGateway
* @see AWS
* API Documentation
*/
GetWirelessGatewayResult getWirelessGateway(GetWirelessGatewayRequest getWirelessGatewayRequest);
/**
*
* Gets the ID of the certificate that is currently associated with a wireless gateway.
*
*
* @param getWirelessGatewayCertificateRequest
* @return Result of the GetWirelessGatewayCertificate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayCertificate
* @see AWS API Documentation
*/
GetWirelessGatewayCertificateResult getWirelessGatewayCertificate(GetWirelessGatewayCertificateRequest getWirelessGatewayCertificateRequest);
/**
*
* Gets the firmware version and other information about a wireless gateway.
*
*
* @param getWirelessGatewayFirmwareInformationRequest
* @return Result of the GetWirelessGatewayFirmwareInformation operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayFirmwareInformation
* @see AWS API Documentation
*/
GetWirelessGatewayFirmwareInformationResult getWirelessGatewayFirmwareInformation(
GetWirelessGatewayFirmwareInformationRequest getWirelessGatewayFirmwareInformationRequest);
/**
*
* Gets operating information about a wireless gateway.
*
*
* @param getWirelessGatewayStatisticsRequest
* @return Result of the GetWirelessGatewayStatistics operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayStatistics
* @see AWS API Documentation
*/
GetWirelessGatewayStatisticsResult getWirelessGatewayStatistics(GetWirelessGatewayStatisticsRequest getWirelessGatewayStatisticsRequest);
/**
*
* Gets information about a wireless gateway task.
*
*
* @param getWirelessGatewayTaskRequest
* @return Result of the GetWirelessGatewayTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayTask
* @see AWS API Documentation
*/
GetWirelessGatewayTaskResult getWirelessGatewayTask(GetWirelessGatewayTaskRequest getWirelessGatewayTaskRequest);
/**
*
* Gets information about a wireless gateway task definition.
*
*
* @param getWirelessGatewayTaskDefinitionRequest
* @return Result of the GetWirelessGatewayTaskDefinition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayTaskDefinition
* @see AWS API Documentation
*/
GetWirelessGatewayTaskDefinitionResult getWirelessGatewayTaskDefinition(GetWirelessGatewayTaskDefinitionRequest getWirelessGatewayTaskDefinitionRequest);
/**
*
* Lists the destinations registered to your AWS account.
*
*
* @param listDestinationsRequest
* @return Result of the ListDestinations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListDestinations
* @see AWS
* API Documentation
*/
ListDestinationsResult listDestinations(ListDestinationsRequest listDestinationsRequest);
/**
*
* Lists the device profiles registered to your AWS account.
*
*
* @param listDeviceProfilesRequest
* @return Result of the ListDeviceProfiles operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListDeviceProfiles
* @see AWS
* API Documentation
*/
ListDeviceProfilesResult listDeviceProfiles(ListDeviceProfilesRequest listDeviceProfilesRequest);
/**
*
* List the Sidewalk devices in an import task and their onboarding status.
*
*
* @param listDevicesForWirelessDeviceImportTaskRequest
* @return Result of the ListDevicesForWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListDevicesForWirelessDeviceImportTask
* @see AWS API Documentation
*/
ListDevicesForWirelessDeviceImportTaskResult listDevicesForWirelessDeviceImportTask(
ListDevicesForWirelessDeviceImportTaskRequest listDevicesForWirelessDeviceImportTaskRequest);
/**
*
* List event configurations where at least one event topic has been enabled.
*
*
* @param listEventConfigurationsRequest
* @return Result of the ListEventConfigurations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.ListEventConfigurations
* @see AWS API Documentation
*/
ListEventConfigurationsResult listEventConfigurations(ListEventConfigurationsRequest listEventConfigurationsRequest);
/**
*
* Lists the FUOTA tasks registered to your AWS account.
*
*
* @param listFuotaTasksRequest
* @return Result of the ListFuotaTasks operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListFuotaTasks
* @see AWS API
* Documentation
*/
ListFuotaTasksResult listFuotaTasks(ListFuotaTasksRequest listFuotaTasksRequest);
/**
*
* Lists the multicast groups registered to your AWS account.
*
*
* @param listMulticastGroupsRequest
* @return Result of the ListMulticastGroups operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListMulticastGroups
* @see AWS API Documentation
*/
ListMulticastGroupsResult listMulticastGroups(ListMulticastGroupsRequest listMulticastGroupsRequest);
/**
*
* List all multicast groups associated with a fuota task.
*
*
* @param listMulticastGroupsByFuotaTaskRequest
* @return Result of the ListMulticastGroupsByFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListMulticastGroupsByFuotaTask
* @see AWS API Documentation
*/
ListMulticastGroupsByFuotaTaskResult listMulticastGroupsByFuotaTask(ListMulticastGroupsByFuotaTaskRequest listMulticastGroupsByFuotaTaskRequest);
/**
*
* Lists the network analyzer configurations.
*
*
* @param listNetworkAnalyzerConfigurationsRequest
* @return Result of the ListNetworkAnalyzerConfigurations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListNetworkAnalyzerConfigurations
* @see AWS API Documentation
*/
ListNetworkAnalyzerConfigurationsResult listNetworkAnalyzerConfigurations(ListNetworkAnalyzerConfigurationsRequest listNetworkAnalyzerConfigurationsRequest);
/**
*
* Lists the partner accounts associated with your AWS account.
*
*
* @param listPartnerAccountsRequest
* @return Result of the ListPartnerAccounts operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListPartnerAccounts
* @see AWS API Documentation
*/
ListPartnerAccountsResult listPartnerAccounts(ListPartnerAccountsRequest listPartnerAccountsRequest);
/**
*
* List position configurations for a given resource, such as positioning solvers.
*
*
*
* This action is no longer supported. Calls to retrieve position information should use the GetResourcePosition API operation instead.
*
*
*
* @param listPositionConfigurationsRequest
* @return Result of the ListPositionConfigurations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.ListPositionConfigurations
* @see AWS API Documentation
*/
@Deprecated
ListPositionConfigurationsResult listPositionConfigurations(ListPositionConfigurationsRequest listPositionConfigurationsRequest);
/**
*
* List queued messages in the downlink queue.
*
*
* @param listQueuedMessagesRequest
* @return Result of the ListQueuedMessages operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.ListQueuedMessages
* @see AWS
* API Documentation
*/
ListQueuedMessagesResult listQueuedMessages(ListQueuedMessagesRequest listQueuedMessagesRequest);
/**
*
* Lists the service profiles registered to your AWS account.
*
*
* @param listServiceProfilesRequest
* @return Result of the ListServiceProfiles operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListServiceProfiles
* @see AWS API Documentation
*/
ListServiceProfilesResult listServiceProfiles(ListServiceProfilesRequest listServiceProfilesRequest);
/**
*
* Lists the tags (metadata) you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List wireless devices that have been added to an import task.
*
*
* @param listWirelessDeviceImportTasksRequest
* @return Result of the ListWirelessDeviceImportTasks operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListWirelessDeviceImportTasks
* @see AWS API Documentation
*/
ListWirelessDeviceImportTasksResult listWirelessDeviceImportTasks(ListWirelessDeviceImportTasksRequest listWirelessDeviceImportTasksRequest);
/**
*
* Lists the wireless devices registered to your AWS account.
*
*
* @param listWirelessDevicesRequest
* @return Result of the ListWirelessDevices operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.ListWirelessDevices
* @see AWS API Documentation
*/
ListWirelessDevicesResult listWirelessDevices(ListWirelessDevicesRequest listWirelessDevicesRequest);
/**
*
* List the wireless gateway tasks definitions registered to your AWS account.
*
*
* @param listWirelessGatewayTaskDefinitionsRequest
* @return Result of the ListWirelessGatewayTaskDefinitions operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListWirelessGatewayTaskDefinitions
* @see AWS API Documentation
*/
ListWirelessGatewayTaskDefinitionsResult listWirelessGatewayTaskDefinitions(
ListWirelessGatewayTaskDefinitionsRequest listWirelessGatewayTaskDefinitionsRequest);
/**
*
* Lists the wireless gateways registered to your AWS account.
*
*
* @param listWirelessGatewaysRequest
* @return Result of the ListWirelessGateways operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.ListWirelessGateways
* @see AWS API Documentation
*/
ListWirelessGatewaysResult listWirelessGateways(ListWirelessGatewaysRequest listWirelessGatewaysRequest);
/**
*
* Put position configuration for a given resource.
*
*
*
* This action is no longer supported. Calls to update the position configuration should use the UpdateResourcePosition API operation instead.
*
*
*
* @param putPositionConfigurationRequest
* @return Result of the PutPositionConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.PutPositionConfiguration
* @see AWS API Documentation
*/
@Deprecated
PutPositionConfigurationResult putPositionConfiguration(PutPositionConfigurationRequest putPositionConfigurationRequest);
/**
*
* Sets the log-level override for a resource-ID and resource-type. This option can be specified for a wireless
* gateway or a wireless device. A limit of 200 log level override can be set per account.
*
*
* @param putResourceLogLevelRequest
* @return Result of the PutResourceLogLevel operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.PutResourceLogLevel
* @see AWS API Documentation
*/
PutResourceLogLevelResult putResourceLogLevel(PutResourceLogLevelRequest putResourceLogLevelRequest);
/**
*
* Removes the log-level overrides for all resources; both wireless devices and wireless gateways.
*
*
* @param resetAllResourceLogLevelsRequest
* @return Result of the ResetAllResourceLogLevels operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.ResetAllResourceLogLevels
* @see AWS API Documentation
*/
ResetAllResourceLogLevelsResult resetAllResourceLogLevels(ResetAllResourceLogLevelsRequest resetAllResourceLogLevelsRequest);
/**
*
* Removes the log-level override, if any, for a specific resource-ID and resource-type. It can be used for a
* wireless device or a wireless gateway.
*
*
* @param resetResourceLogLevelRequest
* @return Result of the ResetResourceLogLevel operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.ResetResourceLogLevel
* @see AWS API Documentation
*/
ResetResourceLogLevelResult resetResourceLogLevel(ResetResourceLogLevelRequest resetResourceLogLevelRequest);
/**
*
* Sends the specified data to a multicast group.
*
*
* @param sendDataToMulticastGroupRequest
* @return Result of the SendDataToMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.SendDataToMulticastGroup
* @see AWS API Documentation
*/
SendDataToMulticastGroupResult sendDataToMulticastGroup(SendDataToMulticastGroupRequest sendDataToMulticastGroupRequest);
/**
*
* Sends a decrypted application data frame to a device.
*
*
* @param sendDataToWirelessDeviceRequest
* @return Result of the SendDataToWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.SendDataToWirelessDevice
* @see AWS API Documentation
*/
SendDataToWirelessDeviceResult sendDataToWirelessDevice(SendDataToWirelessDeviceRequest sendDataToWirelessDeviceRequest);
/**
*
* Starts a bulk association of all qualifying wireless devices with a multicast group.
*
*
* @param startBulkAssociateWirelessDeviceWithMulticastGroupRequest
* @return Result of the StartBulkAssociateWirelessDeviceWithMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.StartBulkAssociateWirelessDeviceWithMulticastGroup
* @see AWS API Documentation
*/
StartBulkAssociateWirelessDeviceWithMulticastGroupResult startBulkAssociateWirelessDeviceWithMulticastGroup(
StartBulkAssociateWirelessDeviceWithMulticastGroupRequest startBulkAssociateWirelessDeviceWithMulticastGroupRequest);
/**
*
* Starts a bulk disassociatin of all qualifying wireless devices from a multicast group.
*
*
* @param startBulkDisassociateWirelessDeviceFromMulticastGroupRequest
* @return Result of the StartBulkDisassociateWirelessDeviceFromMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.StartBulkDisassociateWirelessDeviceFromMulticastGroup
* @see AWS API Documentation
*/
StartBulkDisassociateWirelessDeviceFromMulticastGroupResult startBulkDisassociateWirelessDeviceFromMulticastGroup(
StartBulkDisassociateWirelessDeviceFromMulticastGroupRequest startBulkDisassociateWirelessDeviceFromMulticastGroupRequest);
/**
*
* Starts a FUOTA task.
*
*
* @param startFuotaTaskRequest
* @return Result of the StartFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.StartFuotaTask
* @see AWS API
* Documentation
*/
StartFuotaTaskResult startFuotaTask(StartFuotaTaskRequest startFuotaTaskRequest);
/**
*
* Starts a multicast group session.
*
*
* @param startMulticastGroupSessionRequest
* @return Result of the StartMulticastGroupSession operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.StartMulticastGroupSession
* @see AWS API Documentation
*/
StartMulticastGroupSessionResult startMulticastGroupSession(StartMulticastGroupSessionRequest startMulticastGroupSessionRequest);
/**
*
* Start import task for a single wireless device.
*
*
* @param startSingleWirelessDeviceImportTaskRequest
* @return Result of the StartSingleWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.StartSingleWirelessDeviceImportTask
* @see AWS API Documentation
*/
StartSingleWirelessDeviceImportTaskResult startSingleWirelessDeviceImportTask(
StartSingleWirelessDeviceImportTaskRequest startSingleWirelessDeviceImportTaskRequest);
/**
*
* Start import task for provisioning Sidewalk devices in bulk using an S3 CSV file.
*
*
* @param startWirelessDeviceImportTaskRequest
* @return Result of the StartWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.StartWirelessDeviceImportTask
* @see AWS API Documentation
*/
StartWirelessDeviceImportTaskResult startWirelessDeviceImportTask(StartWirelessDeviceImportTaskRequest startWirelessDeviceImportTaskRequest);
/**
*
* Adds a tag to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws TooManyTagsException
* The request was denied because the resource can't have any more tags.
* @sample AWSIoTWireless.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Simulates a provisioned device by sending an uplink data payload of Hello
.
*
*
* @param testWirelessDeviceRequest
* @return Result of the TestWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.TestWirelessDevice
* @see AWS
* API Documentation
*/
TestWirelessDeviceResult testWirelessDevice(TestWirelessDeviceRequest testWirelessDeviceRequest);
/**
*
* Removes one or more tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates properties of a destination.
*
*
* @param updateDestinationRequest
* @return Result of the UpdateDestination operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateDestination
* @see AWS
* API Documentation
*/
UpdateDestinationResult updateDestination(UpdateDestinationRequest updateDestinationRequest);
/**
*
* Update the event configuration based on resource types.
*
*
* @param updateEventConfigurationByResourceTypesRequest
* @return Result of the UpdateEventConfigurationByResourceTypes operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.UpdateEventConfigurationByResourceTypes
* @see AWS API Documentation
*/
UpdateEventConfigurationByResourceTypesResult updateEventConfigurationByResourceTypes(
UpdateEventConfigurationByResourceTypesRequest updateEventConfigurationByResourceTypesRequest);
/**
*
* Updates properties of a FUOTA task.
*
*
* @param updateFuotaTaskRequest
* @return Result of the UpdateFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateFuotaTask
* @see AWS
* API Documentation
*/
UpdateFuotaTaskResult updateFuotaTask(UpdateFuotaTaskRequest updateFuotaTaskRequest);
/**
*
* Set default log level, or log levels by resource types. This can be for wireless device log options or wireless
* gateways log options and is used to control the log messages that'll be displayed in CloudWatch.
*
*
* @param updateLogLevelsByResourceTypesRequest
* @return Result of the UpdateLogLevelsByResourceTypes operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.UpdateLogLevelsByResourceTypes
* @see AWS API Documentation
*/
UpdateLogLevelsByResourceTypesResult updateLogLevelsByResourceTypes(UpdateLogLevelsByResourceTypesRequest updateLogLevelsByResourceTypesRequest);
/**
*
* Update the summary metric configuration.
*
*
* @param updateMetricConfigurationRequest
* @return Result of the UpdateMetricConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateMetricConfiguration
* @see AWS API Documentation
*/
UpdateMetricConfigurationResult updateMetricConfiguration(UpdateMetricConfigurationRequest updateMetricConfigurationRequest);
/**
*
* Updates properties of a multicast group session.
*
*
* @param updateMulticastGroupRequest
* @return Result of the UpdateMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateMulticastGroup
* @see AWS API Documentation
*/
UpdateMulticastGroupResult updateMulticastGroup(UpdateMulticastGroupRequest updateMulticastGroupRequest);
/**
*
* Update network analyzer configuration.
*
*
* @param updateNetworkAnalyzerConfigurationRequest
* @return Result of the UpdateNetworkAnalyzerConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateNetworkAnalyzerConfiguration
* @see AWS API Documentation
*/
UpdateNetworkAnalyzerConfigurationResult updateNetworkAnalyzerConfiguration(
UpdateNetworkAnalyzerConfigurationRequest updateNetworkAnalyzerConfigurationRequest);
/**
*
* Updates properties of a partner account.
*
*
* @param updatePartnerAccountRequest
* @return Result of the UpdatePartnerAccount operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdatePartnerAccount
* @see AWS API Documentation
*/
UpdatePartnerAccountResult updatePartnerAccount(UpdatePartnerAccountRequest updatePartnerAccountRequest);
/**
*
* Update the position information of a resource.
*
*
*
* This action is no longer supported. Calls to update the position information should use the UpdateResourcePosition API operation instead.
*
*
*
* @param updatePositionRequest
* @return Result of the UpdatePosition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.UpdatePosition
* @see AWS API
* Documentation
*/
@Deprecated
UpdatePositionResult updatePosition(UpdatePositionRequest updatePositionRequest);
/**
*
* Update the event configuration for a particular resource identifier.
*
*
* @param updateResourceEventConfigurationRequest
* @return Result of the UpdateResourceEventConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.UpdateResourceEventConfiguration
* @see AWS API Documentation
*/
UpdateResourceEventConfigurationResult updateResourceEventConfiguration(UpdateResourceEventConfigurationRequest updateResourceEventConfigurationRequest);
/**
*
* Update the position information of a given wireless device or a wireless gateway resource. The position
* coordinates are based on the World Geodetic
* System (WGS84).
*
*
* @param updateResourcePositionRequest
* @return Result of the UpdateResourcePosition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.UpdateResourcePosition
* @see AWS API Documentation
*/
UpdateResourcePositionResult updateResourcePosition(UpdateResourcePositionRequest updateResourcePositionRequest);
/**
*
* Updates properties of a wireless device.
*
*
* @param updateWirelessDeviceRequest
* @return Result of the UpdateWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateWirelessDevice
* @see AWS API Documentation
*/
UpdateWirelessDeviceResult updateWirelessDevice(UpdateWirelessDeviceRequest updateWirelessDeviceRequest);
/**
*
* Update an import task to add more devices to the task.
*
*
* @param updateWirelessDeviceImportTaskRequest
* @return Result of the UpdateWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateWirelessDeviceImportTask
* @see AWS API Documentation
*/
UpdateWirelessDeviceImportTaskResult updateWirelessDeviceImportTask(UpdateWirelessDeviceImportTaskRequest updateWirelessDeviceImportTaskRequest);
/**
*
* Updates properties of a wireless gateway.
*
*
* @param updateWirelessGatewayRequest
* @return Result of the UpdateWirelessGateway operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.UpdateWirelessGateway
* @see AWS API Documentation
*/
UpdateWirelessGatewayResult updateWirelessGateway(UpdateWirelessGatewayRequest updateWirelessGatewayRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}