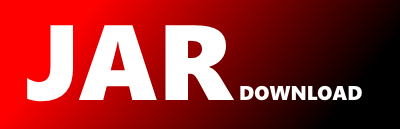
com.amazonaws.services.iotwireless.AWSIoTWirelessClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotwireless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotwireless;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.iotwireless.AWSIoTWirelessClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.iotwireless.model.*;
import com.amazonaws.services.iotwireless.model.transform.*;
/**
* Client for accessing AWS IoT Wireless. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* AWS IoT Wireless provides bi-directional communication between internet-connected wireless devices and the AWS Cloud.
* To onboard both LoRaWAN and Sidewalk devices to AWS IoT, use the IoT Wireless API. These wireless devices use the Low
* Power Wide Area Networking (LPWAN) communication protocol to communicate with AWS IoT.
*
*
* Using the API, you can perform create, read, update, and delete operations for your wireless devices, gateways,
* destinations, and profiles. After onboarding your devices, you can use the API operations to set log levels and
* monitor your devices with CloudWatch.
*
*
* You can also use the API operations to create multicast groups and schedule a multicast session for sending a
* downlink message to devices in the group. By using Firmware Updates Over-The-Air (FUOTA) API operations, you can
* create a FUOTA task and schedule a session to update the firmware of individual devices or an entire group of devices
* in a multicast group.
*
*
* To connect to the AWS IoT Wireless Service, use the Service endpoints as described in IoT Wireless Service
* endpoints in the AWS General Reference.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSIoTWirelessClient extends AmazonWebServiceClient implements AWSIoTWireless {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSIoTWireless.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "iotwireless";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.iotwireless.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.iotwireless.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.iotwireless.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.iotwireless.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyTagsException").withExceptionUnmarshaller(
com.amazonaws.services.iotwireless.model.transform.TooManyTagsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.iotwireless.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.iotwireless.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.iotwireless.model.AWSIoTWirelessException.class));
public static AWSIoTWirelessClientBuilder builder() {
return AWSIoTWirelessClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS IoT Wireless using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSIoTWirelessClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS IoT Wireless using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSIoTWirelessClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("api.iotwireless.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/iotwireless/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/iotwireless/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates a partner account with your AWS account.
*
*
* @param associateAwsAccountWithPartnerAccountRequest
* @return Result of the AssociateAwsAccountWithPartnerAccount operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.AssociateAwsAccountWithPartnerAccount
* @see AWS API Documentation
*/
@Override
public AssociateAwsAccountWithPartnerAccountResult associateAwsAccountWithPartnerAccount(AssociateAwsAccountWithPartnerAccountRequest request) {
request = beforeClientExecution(request);
return executeAssociateAwsAccountWithPartnerAccount(request);
}
@SdkInternalApi
final AssociateAwsAccountWithPartnerAccountResult executeAssociateAwsAccountWithPartnerAccount(
AssociateAwsAccountWithPartnerAccountRequest associateAwsAccountWithPartnerAccountRequest) {
ExecutionContext executionContext = createExecutionContext(associateAwsAccountWithPartnerAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateAwsAccountWithPartnerAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateAwsAccountWithPartnerAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateAwsAccountWithPartnerAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateAwsAccountWithPartnerAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associate a multicast group with a FUOTA task.
*
*
* @param associateMulticastGroupWithFuotaTaskRequest
* @return Result of the AssociateMulticastGroupWithFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateMulticastGroupWithFuotaTask
* @see AWS API Documentation
*/
@Override
public AssociateMulticastGroupWithFuotaTaskResult associateMulticastGroupWithFuotaTask(AssociateMulticastGroupWithFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeAssociateMulticastGroupWithFuotaTask(request);
}
@SdkInternalApi
final AssociateMulticastGroupWithFuotaTaskResult executeAssociateMulticastGroupWithFuotaTask(
AssociateMulticastGroupWithFuotaTaskRequest associateMulticastGroupWithFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(associateMulticastGroupWithFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateMulticastGroupWithFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateMulticastGroupWithFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateMulticastGroupWithFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateMulticastGroupWithFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associate a wireless device with a FUOTA task.
*
*
* @param associateWirelessDeviceWithFuotaTaskRequest
* @return Result of the AssociateWirelessDeviceWithFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessDeviceWithFuotaTask
* @see AWS API Documentation
*/
@Override
public AssociateWirelessDeviceWithFuotaTaskResult associateWirelessDeviceWithFuotaTask(AssociateWirelessDeviceWithFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeAssociateWirelessDeviceWithFuotaTask(request);
}
@SdkInternalApi
final AssociateWirelessDeviceWithFuotaTaskResult executeAssociateWirelessDeviceWithFuotaTask(
AssociateWirelessDeviceWithFuotaTaskRequest associateWirelessDeviceWithFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(associateWirelessDeviceWithFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateWirelessDeviceWithFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateWirelessDeviceWithFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateWirelessDeviceWithFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateWirelessDeviceWithFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a wireless device with a multicast group.
*
*
* @param associateWirelessDeviceWithMulticastGroupRequest
* @return Result of the AssociateWirelessDeviceWithMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessDeviceWithMulticastGroup
* @see AWS API Documentation
*/
@Override
public AssociateWirelessDeviceWithMulticastGroupResult associateWirelessDeviceWithMulticastGroup(AssociateWirelessDeviceWithMulticastGroupRequest request) {
request = beforeClientExecution(request);
return executeAssociateWirelessDeviceWithMulticastGroup(request);
}
@SdkInternalApi
final AssociateWirelessDeviceWithMulticastGroupResult executeAssociateWirelessDeviceWithMulticastGroup(
AssociateWirelessDeviceWithMulticastGroupRequest associateWirelessDeviceWithMulticastGroupRequest) {
ExecutionContext executionContext = createExecutionContext(associateWirelessDeviceWithMulticastGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateWirelessDeviceWithMulticastGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateWirelessDeviceWithMulticastGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateWirelessDeviceWithMulticastGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateWirelessDeviceWithMulticastGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a wireless device with a thing.
*
*
* @param associateWirelessDeviceWithThingRequest
* @return Result of the AssociateWirelessDeviceWithThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessDeviceWithThing
* @see AWS API Documentation
*/
@Override
public AssociateWirelessDeviceWithThingResult associateWirelessDeviceWithThing(AssociateWirelessDeviceWithThingRequest request) {
request = beforeClientExecution(request);
return executeAssociateWirelessDeviceWithThing(request);
}
@SdkInternalApi
final AssociateWirelessDeviceWithThingResult executeAssociateWirelessDeviceWithThing(
AssociateWirelessDeviceWithThingRequest associateWirelessDeviceWithThingRequest) {
ExecutionContext executionContext = createExecutionContext(associateWirelessDeviceWithThingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateWirelessDeviceWithThingRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateWirelessDeviceWithThingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateWirelessDeviceWithThing");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateWirelessDeviceWithThingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a wireless gateway with a certificate.
*
*
* @param associateWirelessGatewayWithCertificateRequest
* @return Result of the AssociateWirelessGatewayWithCertificate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.AssociateWirelessGatewayWithCertificate
* @see AWS API Documentation
*/
@Override
public AssociateWirelessGatewayWithCertificateResult associateWirelessGatewayWithCertificate(AssociateWirelessGatewayWithCertificateRequest request) {
request = beforeClientExecution(request);
return executeAssociateWirelessGatewayWithCertificate(request);
}
@SdkInternalApi
final AssociateWirelessGatewayWithCertificateResult executeAssociateWirelessGatewayWithCertificate(
AssociateWirelessGatewayWithCertificateRequest associateWirelessGatewayWithCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(associateWirelessGatewayWithCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateWirelessGatewayWithCertificateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateWirelessGatewayWithCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateWirelessGatewayWithCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateWirelessGatewayWithCertificateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a wireless gateway with a thing.
*
*
* @param associateWirelessGatewayWithThingRequest
* @return Result of the AssociateWirelessGatewayWithThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.AssociateWirelessGatewayWithThing
* @see AWS API Documentation
*/
@Override
public AssociateWirelessGatewayWithThingResult associateWirelessGatewayWithThing(AssociateWirelessGatewayWithThingRequest request) {
request = beforeClientExecution(request);
return executeAssociateWirelessGatewayWithThing(request);
}
@SdkInternalApi
final AssociateWirelessGatewayWithThingResult executeAssociateWirelessGatewayWithThing(
AssociateWirelessGatewayWithThingRequest associateWirelessGatewayWithThingRequest) {
ExecutionContext executionContext = createExecutionContext(associateWirelessGatewayWithThingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateWirelessGatewayWithThingRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateWirelessGatewayWithThingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateWirelessGatewayWithThing");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateWirelessGatewayWithThingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels an existing multicast group session.
*
*
* @param cancelMulticastGroupSessionRequest
* @return Result of the CancelMulticastGroupSession operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CancelMulticastGroupSession
* @see AWS API Documentation
*/
@Override
public CancelMulticastGroupSessionResult cancelMulticastGroupSession(CancelMulticastGroupSessionRequest request) {
request = beforeClientExecution(request);
return executeCancelMulticastGroupSession(request);
}
@SdkInternalApi
final CancelMulticastGroupSessionResult executeCancelMulticastGroupSession(CancelMulticastGroupSessionRequest cancelMulticastGroupSessionRequest) {
ExecutionContext executionContext = createExecutionContext(cancelMulticastGroupSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelMulticastGroupSessionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(cancelMulticastGroupSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelMulticastGroupSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CancelMulticastGroupSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new destination that maps a device message to an AWS IoT rule.
*
*
* @param createDestinationRequest
* @return Result of the CreateDestination operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateDestination
* @see AWS
* API Documentation
*/
@Override
public CreateDestinationResult createDestination(CreateDestinationRequest request) {
request = beforeClientExecution(request);
return executeCreateDestination(request);
}
@SdkInternalApi
final CreateDestinationResult executeCreateDestination(CreateDestinationRequest createDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(createDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDestinationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new device profile.
*
*
* @param createDeviceProfileRequest
* @return Result of the CreateDeviceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateDeviceProfile
* @see AWS API Documentation
*/
@Override
public CreateDeviceProfileResult createDeviceProfile(CreateDeviceProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateDeviceProfile(request);
}
@SdkInternalApi
final CreateDeviceProfileResult executeCreateDeviceProfile(CreateDeviceProfileRequest createDeviceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createDeviceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDeviceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDeviceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDeviceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDeviceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a FUOTA task.
*
*
* @param createFuotaTaskRequest
* @return Result of the CreateFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateFuotaTask
* @see AWS
* API Documentation
*/
@Override
public CreateFuotaTaskResult createFuotaTask(CreateFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeCreateFuotaTask(request);
}
@SdkInternalApi
final CreateFuotaTaskResult executeCreateFuotaTask(CreateFuotaTaskRequest createFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(createFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a multicast group.
*
*
* @param createMulticastGroupRequest
* @return Result of the CreateMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateMulticastGroup
* @see AWS API Documentation
*/
@Override
public CreateMulticastGroupResult createMulticastGroup(CreateMulticastGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateMulticastGroup(request);
}
@SdkInternalApi
final CreateMulticastGroupResult executeCreateMulticastGroup(CreateMulticastGroupRequest createMulticastGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createMulticastGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMulticastGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMulticastGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMulticastGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMulticastGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new network analyzer configuration.
*
*
* @param createNetworkAnalyzerConfigurationRequest
* @return Result of the CreateNetworkAnalyzerConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateNetworkAnalyzerConfiguration
* @see AWS API Documentation
*/
@Override
public CreateNetworkAnalyzerConfigurationResult createNetworkAnalyzerConfiguration(CreateNetworkAnalyzerConfigurationRequest request) {
request = beforeClientExecution(request);
return executeCreateNetworkAnalyzerConfiguration(request);
}
@SdkInternalApi
final CreateNetworkAnalyzerConfigurationResult executeCreateNetworkAnalyzerConfiguration(
CreateNetworkAnalyzerConfigurationRequest createNetworkAnalyzerConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createNetworkAnalyzerConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateNetworkAnalyzerConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createNetworkAnalyzerConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateNetworkAnalyzerConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateNetworkAnalyzerConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new service profile.
*
*
* @param createServiceProfileRequest
* @return Result of the CreateServiceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateServiceProfile
* @see AWS API Documentation
*/
@Override
public CreateServiceProfileResult createServiceProfile(CreateServiceProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateServiceProfile(request);
}
@SdkInternalApi
final CreateServiceProfileResult executeCreateServiceProfile(CreateServiceProfileRequest createServiceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createServiceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateServiceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createServiceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateServiceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateServiceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provisions a wireless device.
*
*
* @param createWirelessDeviceRequest
* @return Result of the CreateWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessDevice
* @see AWS API Documentation
*/
@Override
public CreateWirelessDeviceResult createWirelessDevice(CreateWirelessDeviceRequest request) {
request = beforeClientExecution(request);
return executeCreateWirelessDevice(request);
}
@SdkInternalApi
final CreateWirelessDeviceResult executeCreateWirelessDevice(CreateWirelessDeviceRequest createWirelessDeviceRequest) {
ExecutionContext executionContext = createExecutionContext(createWirelessDeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWirelessDeviceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createWirelessDeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWirelessDevice");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateWirelessDeviceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provisions a wireless gateway.
*
*
*
* When provisioning a wireless gateway, you might run into duplication errors for the following reasons.
*
*
* -
*
* If you specify a GatewayEui
value that already exists.
*
*
* -
*
* If you used a ClientRequestToken
with the same parameters within the last 10 minutes.
*
*
*
*
* To avoid this error, make sure that you use unique identifiers and parameters for each request within the
* specified time period.
*
*
*
* @param createWirelessGatewayRequest
* @return Result of the CreateWirelessGateway operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessGateway
* @see AWS API Documentation
*/
@Override
public CreateWirelessGatewayResult createWirelessGateway(CreateWirelessGatewayRequest request) {
request = beforeClientExecution(request);
return executeCreateWirelessGateway(request);
}
@SdkInternalApi
final CreateWirelessGatewayResult executeCreateWirelessGateway(CreateWirelessGatewayRequest createWirelessGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(createWirelessGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWirelessGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createWirelessGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWirelessGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateWirelessGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a task for a wireless gateway.
*
*
* @param createWirelessGatewayTaskRequest
* @return Result of the CreateWirelessGatewayTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessGatewayTask
* @see AWS API Documentation
*/
@Override
public CreateWirelessGatewayTaskResult createWirelessGatewayTask(CreateWirelessGatewayTaskRequest request) {
request = beforeClientExecution(request);
return executeCreateWirelessGatewayTask(request);
}
@SdkInternalApi
final CreateWirelessGatewayTaskResult executeCreateWirelessGatewayTask(CreateWirelessGatewayTaskRequest createWirelessGatewayTaskRequest) {
ExecutionContext executionContext = createExecutionContext(createWirelessGatewayTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWirelessGatewayTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createWirelessGatewayTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWirelessGatewayTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateWirelessGatewayTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a gateway task definition.
*
*
* @param createWirelessGatewayTaskDefinitionRequest
* @return Result of the CreateWirelessGatewayTaskDefinition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.CreateWirelessGatewayTaskDefinition
* @see AWS API Documentation
*/
@Override
public CreateWirelessGatewayTaskDefinitionResult createWirelessGatewayTaskDefinition(CreateWirelessGatewayTaskDefinitionRequest request) {
request = beforeClientExecution(request);
return executeCreateWirelessGatewayTaskDefinition(request);
}
@SdkInternalApi
final CreateWirelessGatewayTaskDefinitionResult executeCreateWirelessGatewayTaskDefinition(
CreateWirelessGatewayTaskDefinitionRequest createWirelessGatewayTaskDefinitionRequest) {
ExecutionContext executionContext = createExecutionContext(createWirelessGatewayTaskDefinitionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWirelessGatewayTaskDefinitionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createWirelessGatewayTaskDefinitionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWirelessGatewayTaskDefinition");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateWirelessGatewayTaskDefinitionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a destination.
*
*
* @param deleteDestinationRequest
* @return Result of the DeleteDestination operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteDestination
* @see AWS
* API Documentation
*/
@Override
public DeleteDestinationResult deleteDestination(DeleteDestinationRequest request) {
request = beforeClientExecution(request);
return executeDeleteDestination(request);
}
@SdkInternalApi
final DeleteDestinationResult executeDeleteDestination(DeleteDestinationRequest deleteDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDestinationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a device profile.
*
*
* @param deleteDeviceProfileRequest
* @return Result of the DeleteDeviceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteDeviceProfile
* @see AWS API Documentation
*/
@Override
public DeleteDeviceProfileResult deleteDeviceProfile(DeleteDeviceProfileRequest request) {
request = beforeClientExecution(request);
return executeDeleteDeviceProfile(request);
}
@SdkInternalApi
final DeleteDeviceProfileResult executeDeleteDeviceProfile(DeleteDeviceProfileRequest deleteDeviceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDeviceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDeviceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDeviceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDeviceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDeviceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a FUOTA task.
*
*
* @param deleteFuotaTaskRequest
* @return Result of the DeleteFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteFuotaTask
* @see AWS
* API Documentation
*/
@Override
public DeleteFuotaTaskResult deleteFuotaTask(DeleteFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeDeleteFuotaTask(request);
}
@SdkInternalApi
final DeleteFuotaTaskResult executeDeleteFuotaTask(DeleteFuotaTaskRequest deleteFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a multicast group if it is not in use by a fuota task.
*
*
* @param deleteMulticastGroupRequest
* @return Result of the DeleteMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteMulticastGroup
* @see AWS API Documentation
*/
@Override
public DeleteMulticastGroupResult deleteMulticastGroup(DeleteMulticastGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteMulticastGroup(request);
}
@SdkInternalApi
final DeleteMulticastGroupResult executeDeleteMulticastGroup(DeleteMulticastGroupRequest deleteMulticastGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMulticastGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMulticastGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMulticastGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMulticastGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMulticastGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a network analyzer configuration.
*
*
* @param deleteNetworkAnalyzerConfigurationRequest
* @return Result of the DeleteNetworkAnalyzerConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteNetworkAnalyzerConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteNetworkAnalyzerConfigurationResult deleteNetworkAnalyzerConfiguration(DeleteNetworkAnalyzerConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteNetworkAnalyzerConfiguration(request);
}
@SdkInternalApi
final DeleteNetworkAnalyzerConfigurationResult executeDeleteNetworkAnalyzerConfiguration(
DeleteNetworkAnalyzerConfigurationRequest deleteNetworkAnalyzerConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteNetworkAnalyzerConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteNetworkAnalyzerConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteNetworkAnalyzerConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteNetworkAnalyzerConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteNetworkAnalyzerConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove queued messages from the downlink queue.
*
*
* @param deleteQueuedMessagesRequest
* @return Result of the DeleteQueuedMessages operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.DeleteQueuedMessages
* @see AWS API Documentation
*/
@Override
public DeleteQueuedMessagesResult deleteQueuedMessages(DeleteQueuedMessagesRequest request) {
request = beforeClientExecution(request);
return executeDeleteQueuedMessages(request);
}
@SdkInternalApi
final DeleteQueuedMessagesResult executeDeleteQueuedMessages(DeleteQueuedMessagesRequest deleteQueuedMessagesRequest) {
ExecutionContext executionContext = createExecutionContext(deleteQueuedMessagesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteQueuedMessagesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteQueuedMessagesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteQueuedMessages");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteQueuedMessagesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a service profile.
*
*
* @param deleteServiceProfileRequest
* @return Result of the DeleteServiceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteServiceProfile
* @see AWS API Documentation
*/
@Override
public DeleteServiceProfileResult deleteServiceProfile(DeleteServiceProfileRequest request) {
request = beforeClientExecution(request);
return executeDeleteServiceProfile(request);
}
@SdkInternalApi
final DeleteServiceProfileResult executeDeleteServiceProfile(DeleteServiceProfileRequest deleteServiceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteServiceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteServiceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteServiceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteServiceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteServiceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a wireless device.
*
*
* @param deleteWirelessDeviceRequest
* @return Result of the DeleteWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessDevice
* @see AWS API Documentation
*/
@Override
public DeleteWirelessDeviceResult deleteWirelessDevice(DeleteWirelessDeviceRequest request) {
request = beforeClientExecution(request);
return executeDeleteWirelessDevice(request);
}
@SdkInternalApi
final DeleteWirelessDeviceResult executeDeleteWirelessDevice(DeleteWirelessDeviceRequest deleteWirelessDeviceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWirelessDeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWirelessDeviceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteWirelessDeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWirelessDevice");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteWirelessDeviceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete an import task.
*
*
* @param deleteWirelessDeviceImportTaskRequest
* @return Result of the DeleteWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessDeviceImportTask
* @see AWS API Documentation
*/
@Override
public DeleteWirelessDeviceImportTaskResult deleteWirelessDeviceImportTask(DeleteWirelessDeviceImportTaskRequest request) {
request = beforeClientExecution(request);
return executeDeleteWirelessDeviceImportTask(request);
}
@SdkInternalApi
final DeleteWirelessDeviceImportTaskResult executeDeleteWirelessDeviceImportTask(DeleteWirelessDeviceImportTaskRequest deleteWirelessDeviceImportTaskRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWirelessDeviceImportTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWirelessDeviceImportTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteWirelessDeviceImportTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWirelessDeviceImportTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteWirelessDeviceImportTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a wireless gateway.
*
*
*
* When deleting a wireless gateway, you might run into duplication errors for the following reasons.
*
*
* -
*
* If you specify a GatewayEui
value that already exists.
*
*
* -
*
* If you used a ClientRequestToken
with the same parameters within the last 10 minutes.
*
*
*
*
* To avoid this error, make sure that you use unique identifiers and parameters for each request within the
* specified time period.
*
*
*
* @param deleteWirelessGatewayRequest
* @return Result of the DeleteWirelessGateway operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessGateway
* @see AWS API Documentation
*/
@Override
public DeleteWirelessGatewayResult deleteWirelessGateway(DeleteWirelessGatewayRequest request) {
request = beforeClientExecution(request);
return executeDeleteWirelessGateway(request);
}
@SdkInternalApi
final DeleteWirelessGatewayResult executeDeleteWirelessGateway(DeleteWirelessGatewayRequest deleteWirelessGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWirelessGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWirelessGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteWirelessGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWirelessGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteWirelessGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a wireless gateway task.
*
*
* @param deleteWirelessGatewayTaskRequest
* @return Result of the DeleteWirelessGatewayTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessGatewayTask
* @see AWS API Documentation
*/
@Override
public DeleteWirelessGatewayTaskResult deleteWirelessGatewayTask(DeleteWirelessGatewayTaskRequest request) {
request = beforeClientExecution(request);
return executeDeleteWirelessGatewayTask(request);
}
@SdkInternalApi
final DeleteWirelessGatewayTaskResult executeDeleteWirelessGatewayTask(DeleteWirelessGatewayTaskRequest deleteWirelessGatewayTaskRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWirelessGatewayTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWirelessGatewayTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteWirelessGatewayTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWirelessGatewayTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteWirelessGatewayTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a wireless gateway task definition. Deleting this task definition does not affect tasks that are
* currently in progress.
*
*
* @param deleteWirelessGatewayTaskDefinitionRequest
* @return Result of the DeleteWirelessGatewayTaskDefinition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeleteWirelessGatewayTaskDefinition
* @see AWS API Documentation
*/
@Override
public DeleteWirelessGatewayTaskDefinitionResult deleteWirelessGatewayTaskDefinition(DeleteWirelessGatewayTaskDefinitionRequest request) {
request = beforeClientExecution(request);
return executeDeleteWirelessGatewayTaskDefinition(request);
}
@SdkInternalApi
final DeleteWirelessGatewayTaskDefinitionResult executeDeleteWirelessGatewayTaskDefinition(
DeleteWirelessGatewayTaskDefinitionRequest deleteWirelessGatewayTaskDefinitionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWirelessGatewayTaskDefinitionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWirelessGatewayTaskDefinitionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteWirelessGatewayTaskDefinitionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWirelessGatewayTaskDefinition");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteWirelessGatewayTaskDefinitionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deregister a wireless device from AWS IoT Wireless.
*
*
* @param deregisterWirelessDeviceRequest
* @return Result of the DeregisterWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DeregisterWirelessDevice
* @see AWS API Documentation
*/
@Override
public DeregisterWirelessDeviceResult deregisterWirelessDevice(DeregisterWirelessDeviceRequest request) {
request = beforeClientExecution(request);
return executeDeregisterWirelessDevice(request);
}
@SdkInternalApi
final DeregisterWirelessDeviceResult executeDeregisterWirelessDevice(DeregisterWirelessDeviceRequest deregisterWirelessDeviceRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterWirelessDeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterWirelessDeviceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deregisterWirelessDeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeregisterWirelessDevice");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeregisterWirelessDeviceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates your AWS account from a partner account. If PartnerAccountId
and
* PartnerType
are null
, disassociates your AWS account from all partner accounts.
*
*
* @param disassociateAwsAccountFromPartnerAccountRequest
* @return Result of the DisassociateAwsAccountFromPartnerAccount operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DisassociateAwsAccountFromPartnerAccount
* @see AWS API Documentation
*/
@Override
public DisassociateAwsAccountFromPartnerAccountResult disassociateAwsAccountFromPartnerAccount(DisassociateAwsAccountFromPartnerAccountRequest request) {
request = beforeClientExecution(request);
return executeDisassociateAwsAccountFromPartnerAccount(request);
}
@SdkInternalApi
final DisassociateAwsAccountFromPartnerAccountResult executeDisassociateAwsAccountFromPartnerAccount(
DisassociateAwsAccountFromPartnerAccountRequest disassociateAwsAccountFromPartnerAccountRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateAwsAccountFromPartnerAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateAwsAccountFromPartnerAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateAwsAccountFromPartnerAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateAwsAccountFromPartnerAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateAwsAccountFromPartnerAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a multicast group from a fuota task.
*
*
* @param disassociateMulticastGroupFromFuotaTaskRequest
* @return Result of the DisassociateMulticastGroupFromFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateMulticastGroupFromFuotaTask
* @see AWS API Documentation
*/
@Override
public DisassociateMulticastGroupFromFuotaTaskResult disassociateMulticastGroupFromFuotaTask(DisassociateMulticastGroupFromFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeDisassociateMulticastGroupFromFuotaTask(request);
}
@SdkInternalApi
final DisassociateMulticastGroupFromFuotaTaskResult executeDisassociateMulticastGroupFromFuotaTask(
DisassociateMulticastGroupFromFuotaTaskRequest disassociateMulticastGroupFromFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateMulticastGroupFromFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateMulticastGroupFromFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateMulticastGroupFromFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateMulticastGroupFromFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateMulticastGroupFromFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a wireless device from a FUOTA task.
*
*
* @param disassociateWirelessDeviceFromFuotaTaskRequest
* @return Result of the DisassociateWirelessDeviceFromFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessDeviceFromFuotaTask
* @see AWS API Documentation
*/
@Override
public DisassociateWirelessDeviceFromFuotaTaskResult disassociateWirelessDeviceFromFuotaTask(DisassociateWirelessDeviceFromFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeDisassociateWirelessDeviceFromFuotaTask(request);
}
@SdkInternalApi
final DisassociateWirelessDeviceFromFuotaTaskResult executeDisassociateWirelessDeviceFromFuotaTask(
DisassociateWirelessDeviceFromFuotaTaskRequest disassociateWirelessDeviceFromFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateWirelessDeviceFromFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateWirelessDeviceFromFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateWirelessDeviceFromFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateWirelessDeviceFromFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateWirelessDeviceFromFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a wireless device from a multicast group.
*
*
* @param disassociateWirelessDeviceFromMulticastGroupRequest
* @return Result of the DisassociateWirelessDeviceFromMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessDeviceFromMulticastGroup
* @see AWS API Documentation
*/
@Override
public DisassociateWirelessDeviceFromMulticastGroupResult disassociateWirelessDeviceFromMulticastGroup(
DisassociateWirelessDeviceFromMulticastGroupRequest request) {
request = beforeClientExecution(request);
return executeDisassociateWirelessDeviceFromMulticastGroup(request);
}
@SdkInternalApi
final DisassociateWirelessDeviceFromMulticastGroupResult executeDisassociateWirelessDeviceFromMulticastGroup(
DisassociateWirelessDeviceFromMulticastGroupRequest disassociateWirelessDeviceFromMulticastGroupRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateWirelessDeviceFromMulticastGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateWirelessDeviceFromMulticastGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateWirelessDeviceFromMulticastGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateWirelessDeviceFromMulticastGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateWirelessDeviceFromMulticastGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a wireless device from its currently associated thing.
*
*
* @param disassociateWirelessDeviceFromThingRequest
* @return Result of the DisassociateWirelessDeviceFromThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessDeviceFromThing
* @see AWS API Documentation
*/
@Override
public DisassociateWirelessDeviceFromThingResult disassociateWirelessDeviceFromThing(DisassociateWirelessDeviceFromThingRequest request) {
request = beforeClientExecution(request);
return executeDisassociateWirelessDeviceFromThing(request);
}
@SdkInternalApi
final DisassociateWirelessDeviceFromThingResult executeDisassociateWirelessDeviceFromThing(
DisassociateWirelessDeviceFromThingRequest disassociateWirelessDeviceFromThingRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateWirelessDeviceFromThingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateWirelessDeviceFromThingRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateWirelessDeviceFromThingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateWirelessDeviceFromThing");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateWirelessDeviceFromThingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a wireless gateway from its currently associated certificate.
*
*
* @param disassociateWirelessGatewayFromCertificateRequest
* @return Result of the DisassociateWirelessGatewayFromCertificate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.DisassociateWirelessGatewayFromCertificate
* @see AWS API Documentation
*/
@Override
public DisassociateWirelessGatewayFromCertificateResult disassociateWirelessGatewayFromCertificate(DisassociateWirelessGatewayFromCertificateRequest request) {
request = beforeClientExecution(request);
return executeDisassociateWirelessGatewayFromCertificate(request);
}
@SdkInternalApi
final DisassociateWirelessGatewayFromCertificateResult executeDisassociateWirelessGatewayFromCertificate(
DisassociateWirelessGatewayFromCertificateRequest disassociateWirelessGatewayFromCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateWirelessGatewayFromCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateWirelessGatewayFromCertificateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateWirelessGatewayFromCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateWirelessGatewayFromCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateWirelessGatewayFromCertificateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a wireless gateway from its currently associated thing.
*
*
* @param disassociateWirelessGatewayFromThingRequest
* @return Result of the DisassociateWirelessGatewayFromThing operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.DisassociateWirelessGatewayFromThing
* @see AWS API Documentation
*/
@Override
public DisassociateWirelessGatewayFromThingResult disassociateWirelessGatewayFromThing(DisassociateWirelessGatewayFromThingRequest request) {
request = beforeClientExecution(request);
return executeDisassociateWirelessGatewayFromThing(request);
}
@SdkInternalApi
final DisassociateWirelessGatewayFromThingResult executeDisassociateWirelessGatewayFromThing(
DisassociateWirelessGatewayFromThingRequest disassociateWirelessGatewayFromThingRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateWirelessGatewayFromThingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateWirelessGatewayFromThingRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateWirelessGatewayFromThingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateWirelessGatewayFromThing");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateWirelessGatewayFromThingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a destination.
*
*
* @param getDestinationRequest
* @return Result of the GetDestination operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetDestination
* @see AWS API
* Documentation
*/
@Override
public GetDestinationResult getDestination(GetDestinationRequest request) {
request = beforeClientExecution(request);
return executeGetDestination(request);
}
@SdkInternalApi
final GetDestinationResult executeGetDestination(GetDestinationRequest getDestinationRequest) {
ExecutionContext executionContext = createExecutionContext(getDestinationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDestinationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDestinationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDestination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDestinationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a device profile.
*
*
* @param getDeviceProfileRequest
* @return Result of the GetDeviceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetDeviceProfile
* @see AWS
* API Documentation
*/
@Override
public GetDeviceProfileResult getDeviceProfile(GetDeviceProfileRequest request) {
request = beforeClientExecution(request);
return executeGetDeviceProfile(request);
}
@SdkInternalApi
final GetDeviceProfileResult executeGetDeviceProfile(GetDeviceProfileRequest getDeviceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getDeviceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDeviceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDeviceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDeviceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetDeviceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the event configuration based on resource types.
*
*
* @param getEventConfigurationByResourceTypesRequest
* @return Result of the GetEventConfigurationByResourceTypes operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetEventConfigurationByResourceTypes
* @see AWS API Documentation
*/
@Override
public GetEventConfigurationByResourceTypesResult getEventConfigurationByResourceTypes(GetEventConfigurationByResourceTypesRequest request) {
request = beforeClientExecution(request);
return executeGetEventConfigurationByResourceTypes(request);
}
@SdkInternalApi
final GetEventConfigurationByResourceTypesResult executeGetEventConfigurationByResourceTypes(
GetEventConfigurationByResourceTypesRequest getEventConfigurationByResourceTypesRequest) {
ExecutionContext executionContext = createExecutionContext(getEventConfigurationByResourceTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEventConfigurationByResourceTypesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getEventConfigurationByResourceTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetEventConfigurationByResourceTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetEventConfigurationByResourceTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a FUOTA task.
*
*
* @param getFuotaTaskRequest
* @return Result of the GetFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetFuotaTask
* @see AWS API
* Documentation
*/
@Override
public GetFuotaTaskResult getFuotaTask(GetFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeGetFuotaTask(request);
}
@SdkInternalApi
final GetFuotaTaskResult executeGetFuotaTask(GetFuotaTaskRequest getFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(getFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns current default log levels or log levels by resource types. Based on resource types, log levels can be
* for wireless device log options or wireless gateway log options.
*
*
* @param getLogLevelsByResourceTypesRequest
* @return Result of the GetLogLevelsByResourceTypes operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.GetLogLevelsByResourceTypes
* @see AWS API Documentation
*/
@Override
public GetLogLevelsByResourceTypesResult getLogLevelsByResourceTypes(GetLogLevelsByResourceTypesRequest request) {
request = beforeClientExecution(request);
return executeGetLogLevelsByResourceTypes(request);
}
@SdkInternalApi
final GetLogLevelsByResourceTypesResult executeGetLogLevelsByResourceTypes(GetLogLevelsByResourceTypesRequest getLogLevelsByResourceTypesRequest) {
ExecutionContext executionContext = createExecutionContext(getLogLevelsByResourceTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetLogLevelsByResourceTypesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getLogLevelsByResourceTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetLogLevelsByResourceTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetLogLevelsByResourceTypesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the metric configuration status for this AWS account.
*
*
* @param getMetricConfigurationRequest
* @return Result of the GetMetricConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMetricConfiguration
* @see AWS API Documentation
*/
@Override
public GetMetricConfigurationResult getMetricConfiguration(GetMetricConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetMetricConfiguration(request);
}
@SdkInternalApi
final GetMetricConfigurationResult executeGetMetricConfiguration(GetMetricConfigurationRequest getMetricConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getMetricConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMetricConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMetricConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMetricConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetMetricConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the summary metrics for this AWS account.
*
*
* @param getMetricsRequest
* @return Result of the GetMetrics operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMetrics
* @see AWS API
* Documentation
*/
@Override
public GetMetricsResult getMetrics(GetMetricsRequest request) {
request = beforeClientExecution(request);
return executeGetMetrics(request);
}
@SdkInternalApi
final GetMetricsResult executeGetMetrics(GetMetricsRequest getMetricsRequest) {
ExecutionContext executionContext = createExecutionContext(getMetricsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMetricsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMetricsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMetrics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMetricsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a multicast group.
*
*
* @param getMulticastGroupRequest
* @return Result of the GetMulticastGroup operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMulticastGroup
* @see AWS
* API Documentation
*/
@Override
public GetMulticastGroupResult getMulticastGroup(GetMulticastGroupRequest request) {
request = beforeClientExecution(request);
return executeGetMulticastGroup(request);
}
@SdkInternalApi
final GetMulticastGroupResult executeGetMulticastGroup(GetMulticastGroupRequest getMulticastGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getMulticastGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMulticastGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMulticastGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMulticastGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMulticastGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a multicast group session.
*
*
* @param getMulticastGroupSessionRequest
* @return Result of the GetMulticastGroupSession operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetMulticastGroupSession
* @see AWS API Documentation
*/
@Override
public GetMulticastGroupSessionResult getMulticastGroupSession(GetMulticastGroupSessionRequest request) {
request = beforeClientExecution(request);
return executeGetMulticastGroupSession(request);
}
@SdkInternalApi
final GetMulticastGroupSessionResult executeGetMulticastGroupSession(GetMulticastGroupSessionRequest getMulticastGroupSessionRequest) {
ExecutionContext executionContext = createExecutionContext(getMulticastGroupSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMulticastGroupSessionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getMulticastGroupSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMulticastGroupSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetMulticastGroupSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get network analyzer configuration.
*
*
* @param getNetworkAnalyzerConfigurationRequest
* @return Result of the GetNetworkAnalyzerConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetNetworkAnalyzerConfiguration
* @see AWS API Documentation
*/
@Override
public GetNetworkAnalyzerConfigurationResult getNetworkAnalyzerConfiguration(GetNetworkAnalyzerConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetNetworkAnalyzerConfiguration(request);
}
@SdkInternalApi
final GetNetworkAnalyzerConfigurationResult executeGetNetworkAnalyzerConfiguration(
GetNetworkAnalyzerConfigurationRequest getNetworkAnalyzerConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getNetworkAnalyzerConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetNetworkAnalyzerConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getNetworkAnalyzerConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetNetworkAnalyzerConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetNetworkAnalyzerConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a partner account. If PartnerAccountId
and PartnerType
are
* null
, returns all partner accounts.
*
*
* @param getPartnerAccountRequest
* @return Result of the GetPartnerAccount operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetPartnerAccount
* @see AWS
* API Documentation
*/
@Override
public GetPartnerAccountResult getPartnerAccount(GetPartnerAccountRequest request) {
request = beforeClientExecution(request);
return executeGetPartnerAccount(request);
}
@SdkInternalApi
final GetPartnerAccountResult executeGetPartnerAccount(GetPartnerAccountRequest getPartnerAccountRequest) {
ExecutionContext executionContext = createExecutionContext(getPartnerAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPartnerAccountRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPartnerAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPartnerAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetPartnerAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the position information for a given resource.
*
*
*
* This action is no longer supported. Calls to retrieve the position information should use the GetResourcePosition API operation instead.
*
*
*
* @param getPositionRequest
* @return Result of the GetPosition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetPosition
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public GetPositionResult getPosition(GetPositionRequest request) {
request = beforeClientExecution(request);
return executeGetPosition(request);
}
@SdkInternalApi
final GetPositionResult executeGetPosition(GetPositionRequest getPositionRequest) {
ExecutionContext executionContext = createExecutionContext(getPositionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPositionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPositionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPosition");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetPositionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get position configuration for a given resource.
*
*
*
* This action is no longer supported. Calls to retrieve the position configuration should use the GetResourcePosition API operation instead.
*
*
*
* @param getPositionConfigurationRequest
* @return Result of the GetPositionConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetPositionConfiguration
* @see AWS API Documentation
*/
@Override
@Deprecated
public GetPositionConfigurationResult getPositionConfiguration(GetPositionConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetPositionConfiguration(request);
}
@SdkInternalApi
final GetPositionConfigurationResult executeGetPositionConfiguration(GetPositionConfigurationRequest getPositionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getPositionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPositionConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getPositionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPositionConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetPositionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get estimated position information as a payload in GeoJSON format. The payload measurement data is resolved using
* solvers that are provided by third-party vendors.
*
*
* @param getPositionEstimateRequest
* @return Result of the GetPositionEstimate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetPositionEstimate
* @see AWS API Documentation
*/
@Override
public GetPositionEstimateResult getPositionEstimate(GetPositionEstimateRequest request) {
request = beforeClientExecution(request);
return executeGetPositionEstimate(request);
}
@SdkInternalApi
final GetPositionEstimateResult executeGetPositionEstimate(GetPositionEstimateRequest getPositionEstimateRequest) {
ExecutionContext executionContext = createExecutionContext(getPositionEstimateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPositionEstimateRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPositionEstimateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPositionEstimate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(false), new GetPositionEstimateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the event configuration for a particular resource identifier.
*
*
* @param getResourceEventConfigurationRequest
* @return Result of the GetResourceEventConfiguration operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetResourceEventConfiguration
* @see AWS API Documentation
*/
@Override
public GetResourceEventConfigurationResult getResourceEventConfiguration(GetResourceEventConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetResourceEventConfiguration(request);
}
@SdkInternalApi
final GetResourceEventConfigurationResult executeGetResourceEventConfiguration(GetResourceEventConfigurationRequest getResourceEventConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getResourceEventConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourceEventConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getResourceEventConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourceEventConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetResourceEventConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Fetches the log-level override, if any, for a given resource-ID and resource-type. It can be used for a wireless
* device or a wireless gateway.
*
*
* @param getResourceLogLevelRequest
* @return Result of the GetResourceLogLevel operation returned by the service.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws ValidationException
* The input did not meet the specified constraints.
* @sample AWSIoTWireless.GetResourceLogLevel
* @see AWS API Documentation
*/
@Override
public GetResourceLogLevelResult getResourceLogLevel(GetResourceLogLevelRequest request) {
request = beforeClientExecution(request);
return executeGetResourceLogLevel(request);
}
@SdkInternalApi
final GetResourceLogLevelResult executeGetResourceLogLevel(GetResourceLogLevelRequest getResourceLogLevelRequest) {
ExecutionContext executionContext = createExecutionContext(getResourceLogLevelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourceLogLevelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResourceLogLevelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourceLogLevel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetResourceLogLevelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get the position information for a given wireless device or a wireless gateway resource. The position information
* uses the World Geodetic System (WGS84).
*
*
* @param getResourcePositionRequest
* @return Result of the GetResourcePosition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.GetResourcePosition
* @see AWS API Documentation
*/
@Override
public GetResourcePositionResult getResourcePosition(GetResourcePositionRequest request) {
request = beforeClientExecution(request);
return executeGetResourcePosition(request);
}
@SdkInternalApi
final GetResourcePositionResult executeGetResourcePosition(GetResourcePositionRequest getResourcePositionRequest) {
ExecutionContext executionContext = createExecutionContext(getResourcePositionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourcePositionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResourcePositionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourcePosition");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(false), new GetResourcePositionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the account-specific endpoint for Configuration and Update Server (CUPS) protocol or LoRaWAN Network Server
* (LNS) connections.
*
*
* @param getServiceEndpointRequest
* @return Result of the GetServiceEndpoint operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetServiceEndpoint
* @see AWS
* API Documentation
*/
@Override
public GetServiceEndpointResult getServiceEndpoint(GetServiceEndpointRequest request) {
request = beforeClientExecution(request);
return executeGetServiceEndpoint(request);
}
@SdkInternalApi
final GetServiceEndpointResult executeGetServiceEndpoint(GetServiceEndpointRequest getServiceEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(getServiceEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetServiceEndpointRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getServiceEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetServiceEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetServiceEndpointResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a service profile.
*
*
* @param getServiceProfileRequest
* @return Result of the GetServiceProfile operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetServiceProfile
* @see AWS
* API Documentation
*/
@Override
public GetServiceProfileResult getServiceProfile(GetServiceProfileRequest request) {
request = beforeClientExecution(request);
return executeGetServiceProfile(request);
}
@SdkInternalApi
final GetServiceProfileResult executeGetServiceProfile(GetServiceProfileRequest getServiceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getServiceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetServiceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getServiceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetServiceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetServiceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a wireless device.
*
*
* @param getWirelessDeviceRequest
* @return Result of the GetWirelessDevice operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessDevice
* @see AWS
* API Documentation
*/
@Override
public GetWirelessDeviceResult getWirelessDevice(GetWirelessDeviceRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessDevice(request);
}
@SdkInternalApi
final GetWirelessDeviceResult executeGetWirelessDevice(GetWirelessDeviceRequest getWirelessDeviceRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessDeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessDeviceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getWirelessDeviceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessDevice");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetWirelessDeviceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get information about an import task and count of device onboarding summary information for the import task.
*
*
* @param getWirelessDeviceImportTaskRequest
* @return Result of the GetWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessDeviceImportTask
* @see AWS API Documentation
*/
@Override
public GetWirelessDeviceImportTaskResult getWirelessDeviceImportTask(GetWirelessDeviceImportTaskRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessDeviceImportTask(request);
}
@SdkInternalApi
final GetWirelessDeviceImportTaskResult executeGetWirelessDeviceImportTask(GetWirelessDeviceImportTaskRequest getWirelessDeviceImportTaskRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessDeviceImportTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessDeviceImportTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getWirelessDeviceImportTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessDeviceImportTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetWirelessDeviceImportTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets operating information about a wireless device.
*
*
* @param getWirelessDeviceStatisticsRequest
* @return Result of the GetWirelessDeviceStatistics operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessDeviceStatistics
* @see AWS API Documentation
*/
@Override
public GetWirelessDeviceStatisticsResult getWirelessDeviceStatistics(GetWirelessDeviceStatisticsRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessDeviceStatistics(request);
}
@SdkInternalApi
final GetWirelessDeviceStatisticsResult executeGetWirelessDeviceStatistics(GetWirelessDeviceStatisticsRequest getWirelessDeviceStatisticsRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessDeviceStatisticsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessDeviceStatisticsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getWirelessDeviceStatisticsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessDeviceStatistics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetWirelessDeviceStatisticsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a wireless gateway.
*
*
* @param getWirelessGatewayRequest
* @return Result of the GetWirelessGateway operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGateway
* @see AWS
* API Documentation
*/
@Override
public GetWirelessGatewayResult getWirelessGateway(GetWirelessGatewayRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessGateway(request);
}
@SdkInternalApi
final GetWirelessGatewayResult executeGetWirelessGateway(GetWirelessGatewayRequest getWirelessGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessGatewayRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getWirelessGatewayRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessGateway");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetWirelessGatewayResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the ID of the certificate that is currently associated with a wireless gateway.
*
*
* @param getWirelessGatewayCertificateRequest
* @return Result of the GetWirelessGatewayCertificate operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayCertificate
* @see AWS API Documentation
*/
@Override
public GetWirelessGatewayCertificateResult getWirelessGatewayCertificate(GetWirelessGatewayCertificateRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessGatewayCertificate(request);
}
@SdkInternalApi
final GetWirelessGatewayCertificateResult executeGetWirelessGatewayCertificate(GetWirelessGatewayCertificateRequest getWirelessGatewayCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessGatewayCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessGatewayCertificateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getWirelessGatewayCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessGatewayCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetWirelessGatewayCertificateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the firmware version and other information about a wireless gateway.
*
*
* @param getWirelessGatewayFirmwareInformationRequest
* @return Result of the GetWirelessGatewayFirmwareInformation operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayFirmwareInformation
* @see AWS API Documentation
*/
@Override
public GetWirelessGatewayFirmwareInformationResult getWirelessGatewayFirmwareInformation(GetWirelessGatewayFirmwareInformationRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessGatewayFirmwareInformation(request);
}
@SdkInternalApi
final GetWirelessGatewayFirmwareInformationResult executeGetWirelessGatewayFirmwareInformation(
GetWirelessGatewayFirmwareInformationRequest getWirelessGatewayFirmwareInformationRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessGatewayFirmwareInformationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessGatewayFirmwareInformationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getWirelessGatewayFirmwareInformationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessGatewayFirmwareInformation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetWirelessGatewayFirmwareInformationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets operating information about a wireless gateway.
*
*
* @param getWirelessGatewayStatisticsRequest
* @return Result of the GetWirelessGatewayStatistics operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayStatistics
* @see AWS API Documentation
*/
@Override
public GetWirelessGatewayStatisticsResult getWirelessGatewayStatistics(GetWirelessGatewayStatisticsRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessGatewayStatistics(request);
}
@SdkInternalApi
final GetWirelessGatewayStatisticsResult executeGetWirelessGatewayStatistics(GetWirelessGatewayStatisticsRequest getWirelessGatewayStatisticsRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessGatewayStatisticsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessGatewayStatisticsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getWirelessGatewayStatisticsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessGatewayStatistics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetWirelessGatewayStatisticsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a wireless gateway task.
*
*
* @param getWirelessGatewayTaskRequest
* @return Result of the GetWirelessGatewayTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayTask
* @see AWS API Documentation
*/
@Override
public GetWirelessGatewayTaskResult getWirelessGatewayTask(GetWirelessGatewayTaskRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessGatewayTask(request);
}
@SdkInternalApi
final GetWirelessGatewayTaskResult executeGetWirelessGatewayTask(GetWirelessGatewayTaskRequest getWirelessGatewayTaskRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessGatewayTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessGatewayTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getWirelessGatewayTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessGatewayTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetWirelessGatewayTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a wireless gateway task definition.
*
*
* @param getWirelessGatewayTaskDefinitionRequest
* @return Result of the GetWirelessGatewayTaskDefinition operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.GetWirelessGatewayTaskDefinition
* @see AWS API Documentation
*/
@Override
public GetWirelessGatewayTaskDefinitionResult getWirelessGatewayTaskDefinition(GetWirelessGatewayTaskDefinitionRequest request) {
request = beforeClientExecution(request);
return executeGetWirelessGatewayTaskDefinition(request);
}
@SdkInternalApi
final GetWirelessGatewayTaskDefinitionResult executeGetWirelessGatewayTaskDefinition(
GetWirelessGatewayTaskDefinitionRequest getWirelessGatewayTaskDefinitionRequest) {
ExecutionContext executionContext = createExecutionContext(getWirelessGatewayTaskDefinitionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWirelessGatewayTaskDefinitionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getWirelessGatewayTaskDefinitionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetWirelessGatewayTaskDefinition");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetWirelessGatewayTaskDefinitionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the destinations registered to your AWS account.
*
*
* @param listDestinationsRequest
* @return Result of the ListDestinations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListDestinations
* @see AWS
* API Documentation
*/
@Override
public ListDestinationsResult listDestinations(ListDestinationsRequest request) {
request = beforeClientExecution(request);
return executeListDestinations(request);
}
@SdkInternalApi
final ListDestinationsResult executeListDestinations(ListDestinationsRequest listDestinationsRequest) {
ExecutionContext executionContext = createExecutionContext(listDestinationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDestinationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDestinationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDestinations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDestinationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the device profiles registered to your AWS account.
*
*
* @param listDeviceProfilesRequest
* @return Result of the ListDeviceProfiles operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListDeviceProfiles
* @see AWS
* API Documentation
*/
@Override
public ListDeviceProfilesResult listDeviceProfiles(ListDeviceProfilesRequest request) {
request = beforeClientExecution(request);
return executeListDeviceProfiles(request);
}
@SdkInternalApi
final ListDeviceProfilesResult executeListDeviceProfiles(ListDeviceProfilesRequest listDeviceProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(listDeviceProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDeviceProfilesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDeviceProfilesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDeviceProfiles");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDeviceProfilesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List the Sidewalk devices in an import task and their onboarding status.
*
*
* @param listDevicesForWirelessDeviceImportTaskRequest
* @return Result of the ListDevicesForWirelessDeviceImportTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListDevicesForWirelessDeviceImportTask
* @see AWS API Documentation
*/
@Override
public ListDevicesForWirelessDeviceImportTaskResult listDevicesForWirelessDeviceImportTask(ListDevicesForWirelessDeviceImportTaskRequest request) {
request = beforeClientExecution(request);
return executeListDevicesForWirelessDeviceImportTask(request);
}
@SdkInternalApi
final ListDevicesForWirelessDeviceImportTaskResult executeListDevicesForWirelessDeviceImportTask(
ListDevicesForWirelessDeviceImportTaskRequest listDevicesForWirelessDeviceImportTaskRequest) {
ExecutionContext executionContext = createExecutionContext(listDevicesForWirelessDeviceImportTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDevicesForWirelessDeviceImportTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listDevicesForWirelessDeviceImportTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDevicesForWirelessDeviceImportTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDevicesForWirelessDeviceImportTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List event configurations where at least one event topic has been enabled.
*
*
* @param listEventConfigurationsRequest
* @return Result of the ListEventConfigurations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.ListEventConfigurations
* @see AWS API Documentation
*/
@Override
public ListEventConfigurationsResult listEventConfigurations(ListEventConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListEventConfigurations(request);
}
@SdkInternalApi
final ListEventConfigurationsResult executeListEventConfigurations(ListEventConfigurationsRequest listEventConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listEventConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEventConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listEventConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListEventConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListEventConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the FUOTA tasks registered to your AWS account.
*
*
* @param listFuotaTasksRequest
* @return Result of the ListFuotaTasks operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListFuotaTasks
* @see AWS API
* Documentation
*/
@Override
public ListFuotaTasksResult listFuotaTasks(ListFuotaTasksRequest request) {
request = beforeClientExecution(request);
return executeListFuotaTasks(request);
}
@SdkInternalApi
final ListFuotaTasksResult executeListFuotaTasks(ListFuotaTasksRequest listFuotaTasksRequest) {
ExecutionContext executionContext = createExecutionContext(listFuotaTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFuotaTasksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFuotaTasksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFuotaTasks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFuotaTasksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the multicast groups registered to your AWS account.
*
*
* @param listMulticastGroupsRequest
* @return Result of the ListMulticastGroups operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListMulticastGroups
* @see AWS API Documentation
*/
@Override
public ListMulticastGroupsResult listMulticastGroups(ListMulticastGroupsRequest request) {
request = beforeClientExecution(request);
return executeListMulticastGroups(request);
}
@SdkInternalApi
final ListMulticastGroupsResult executeListMulticastGroups(ListMulticastGroupsRequest listMulticastGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listMulticastGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMulticastGroupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listMulticastGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMulticastGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListMulticastGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List all multicast groups associated with a fuota task.
*
*
* @param listMulticastGroupsByFuotaTaskRequest
* @return Result of the ListMulticastGroupsByFuotaTask operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListMulticastGroupsByFuotaTask
* @see AWS API Documentation
*/
@Override
public ListMulticastGroupsByFuotaTaskResult listMulticastGroupsByFuotaTask(ListMulticastGroupsByFuotaTaskRequest request) {
request = beforeClientExecution(request);
return executeListMulticastGroupsByFuotaTask(request);
}
@SdkInternalApi
final ListMulticastGroupsByFuotaTaskResult executeListMulticastGroupsByFuotaTask(ListMulticastGroupsByFuotaTaskRequest listMulticastGroupsByFuotaTaskRequest) {
ExecutionContext executionContext = createExecutionContext(listMulticastGroupsByFuotaTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMulticastGroupsByFuotaTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listMulticastGroupsByFuotaTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMulticastGroupsByFuotaTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListMulticastGroupsByFuotaTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the network analyzer configurations.
*
*
* @param listNetworkAnalyzerConfigurationsRequest
* @return Result of the ListNetworkAnalyzerConfigurations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListNetworkAnalyzerConfigurations
* @see AWS API Documentation
*/
@Override
public ListNetworkAnalyzerConfigurationsResult listNetworkAnalyzerConfigurations(ListNetworkAnalyzerConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListNetworkAnalyzerConfigurations(request);
}
@SdkInternalApi
final ListNetworkAnalyzerConfigurationsResult executeListNetworkAnalyzerConfigurations(
ListNetworkAnalyzerConfigurationsRequest listNetworkAnalyzerConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listNetworkAnalyzerConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListNetworkAnalyzerConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listNetworkAnalyzerConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListNetworkAnalyzerConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListNetworkAnalyzerConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the partner accounts associated with your AWS account.
*
*
* @param listPartnerAccountsRequest
* @return Result of the ListPartnerAccounts operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListPartnerAccounts
* @see AWS API Documentation
*/
@Override
public ListPartnerAccountsResult listPartnerAccounts(ListPartnerAccountsRequest request) {
request = beforeClientExecution(request);
return executeListPartnerAccounts(request);
}
@SdkInternalApi
final ListPartnerAccountsResult executeListPartnerAccounts(ListPartnerAccountsRequest listPartnerAccountsRequest) {
ExecutionContext executionContext = createExecutionContext(listPartnerAccountsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPartnerAccountsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPartnerAccountsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPartnerAccounts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPartnerAccountsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List position configurations for a given resource, such as positioning solvers.
*
*
*
* This action is no longer supported. Calls to retrieve position information should use the GetResourcePosition API operation instead.
*
*
*
* @param listPositionConfigurationsRequest
* @return Result of the ListPositionConfigurations operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @sample AWSIoTWireless.ListPositionConfigurations
* @see AWS API Documentation
*/
@Override
@Deprecated
public ListPositionConfigurationsResult listPositionConfigurations(ListPositionConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListPositionConfigurations(request);
}
@SdkInternalApi
final ListPositionConfigurationsResult executeListPositionConfigurations(ListPositionConfigurationsRequest listPositionConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listPositionConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPositionConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listPositionConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPositionConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListPositionConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List queued messages in the downlink queue.
*
*
* @param listQueuedMessagesRequest
* @return Result of the ListQueuedMessages operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.ListQueuedMessages
* @see AWS
* API Documentation
*/
@Override
public ListQueuedMessagesResult listQueuedMessages(ListQueuedMessagesRequest request) {
request = beforeClientExecution(request);
return executeListQueuedMessages(request);
}
@SdkInternalApi
final ListQueuedMessagesResult executeListQueuedMessages(ListQueuedMessagesRequest listQueuedMessagesRequest) {
ExecutionContext executionContext = createExecutionContext(listQueuedMessagesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListQueuedMessagesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listQueuedMessagesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListQueuedMessages");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListQueuedMessagesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the service profiles registered to your AWS account.
*
*
* @param listServiceProfilesRequest
* @return Result of the ListServiceProfiles operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListServiceProfiles
* @see AWS API Documentation
*/
@Override
public ListServiceProfilesResult listServiceProfiles(ListServiceProfilesRequest request) {
request = beforeClientExecution(request);
return executeListServiceProfiles(request);
}
@SdkInternalApi
final ListServiceProfilesResult executeListServiceProfiles(ListServiceProfilesRequest listServiceProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(listServiceProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListServiceProfilesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listServiceProfilesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListServiceProfiles");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListServiceProfilesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags (metadata) you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List wireless devices that have been added to an import task.
*
*
* @param listWirelessDeviceImportTasksRequest
* @return Result of the ListWirelessDeviceImportTasks operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws ResourceNotFoundException
* Resource does not exist.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ConflictException
* Adding, updating, or deleting the resource can cause an inconsistent state.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListWirelessDeviceImportTasks
* @see AWS API Documentation
*/
@Override
public ListWirelessDeviceImportTasksResult listWirelessDeviceImportTasks(ListWirelessDeviceImportTasksRequest request) {
request = beforeClientExecution(request);
return executeListWirelessDeviceImportTasks(request);
}
@SdkInternalApi
final ListWirelessDeviceImportTasksResult executeListWirelessDeviceImportTasks(ListWirelessDeviceImportTasksRequest listWirelessDeviceImportTasksRequest) {
ExecutionContext executionContext = createExecutionContext(listWirelessDeviceImportTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWirelessDeviceImportTasksRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listWirelessDeviceImportTasksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListWirelessDeviceImportTasks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListWirelessDeviceImportTasksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the wireless devices registered to your AWS account.
*
*
* @param listWirelessDevicesRequest
* @return Result of the ListWirelessDevices operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.ListWirelessDevices
* @see AWS API Documentation
*/
@Override
public ListWirelessDevicesResult listWirelessDevices(ListWirelessDevicesRequest request) {
request = beforeClientExecution(request);
return executeListWirelessDevices(request);
}
@SdkInternalApi
final ListWirelessDevicesResult executeListWirelessDevices(ListWirelessDevicesRequest listWirelessDevicesRequest) {
ExecutionContext executionContext = createExecutionContext(listWirelessDevicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWirelessDevicesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listWirelessDevicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListWirelessDevices");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListWirelessDevicesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List the wireless gateway tasks definitions registered to your AWS account.
*
*
* @param listWirelessGatewayTaskDefinitionsRequest
* @return Result of the ListWirelessGatewayTaskDefinitions operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @sample AWSIoTWireless.ListWirelessGatewayTaskDefinitions
* @see AWS API Documentation
*/
@Override
public ListWirelessGatewayTaskDefinitionsResult listWirelessGatewayTaskDefinitions(ListWirelessGatewayTaskDefinitionsRequest request) {
request = beforeClientExecution(request);
return executeListWirelessGatewayTaskDefinitions(request);
}
@SdkInternalApi
final ListWirelessGatewayTaskDefinitionsResult executeListWirelessGatewayTaskDefinitions(
ListWirelessGatewayTaskDefinitionsRequest listWirelessGatewayTaskDefinitionsRequest) {
ExecutionContext executionContext = createExecutionContext(listWirelessGatewayTaskDefinitionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWirelessGatewayTaskDefinitionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listWirelessGatewayTaskDefinitionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListWirelessGatewayTaskDefinitions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListWirelessGatewayTaskDefinitionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the wireless gateways registered to your AWS account.
*
*
* @param listWirelessGatewaysRequest
* @return Result of the ListWirelessGateways operation returned by the service.
* @throws ValidationException
* The input did not meet the specified constraints.
* @throws InternalServerException
* An unexpected error occurred while processing a request.
* @throws ThrottlingException
* The request was denied because it exceeded the allowed API request rate.
* @throws AccessDeniedException
* User does not have permission to perform this action.
* @sample AWSIoTWireless.ListWirelessGateways
* @see AWS API Documentation
*/
@Override
public ListWirelessGatewaysResult listWirelessGateways(ListWirelessGatewaysRequest request) {
request = beforeClientExecution(request);
return executeListWirelessGateways(request);
}
@SdkInternalApi
final ListWirelessGatewaysResult executeListWirelessGateways(ListWirelessGatewaysRequest listWirelessGatewaysRequest) {
ExecutionContext executionContext = createExecutionContext(listWirelessGatewaysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWirelessGatewaysRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listWirelessGatewaysRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "IoT Wireless");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListWirelessGateways");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListWirelessGatewaysResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Put position configuration for a given resource.
*
*
*