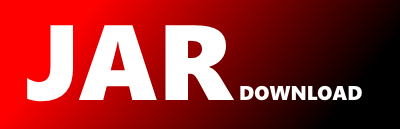
com.amazonaws.services.iotwireless.model.CdmaObj Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotwireless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotwireless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* CDMA (Code-division multiple access) object.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CdmaObj implements Serializable, Cloneable, StructuredPojo {
/**
*
* CDMA system ID (SID).
*
*/
private Integer systemId;
/**
*
* CDMA network ID (NID).
*
*/
private Integer networkId;
/**
*
* CDMA base station ID (BSID).
*
*/
private Integer baseStationId;
/**
*
* CDMA registration zone (RZ).
*
*/
private Integer registrationZone;
/**
*
* CDMA local identification (local ID) parameters.
*
*/
private CdmaLocalId cdmaLocalId;
/**
*
* Transmit power level of the pilot signal, measured in dBm (decibel-milliwatts).
*
*/
private Integer pilotPower;
/**
*
* CDMA base station latitude in degrees.
*
*/
private Float baseLat;
/**
*
* CDMA base station longitude in degrees.
*
*/
private Float baseLng;
/**
*
* CDMA network measurement reports.
*
*/
private java.util.List cdmaNmr;
/**
*
* CDMA system ID (SID).
*
*
* @param systemId
* CDMA system ID (SID).
*/
public void setSystemId(Integer systemId) {
this.systemId = systemId;
}
/**
*
* CDMA system ID (SID).
*
*
* @return CDMA system ID (SID).
*/
public Integer getSystemId() {
return this.systemId;
}
/**
*
* CDMA system ID (SID).
*
*
* @param systemId
* CDMA system ID (SID).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withSystemId(Integer systemId) {
setSystemId(systemId);
return this;
}
/**
*
* CDMA network ID (NID).
*
*
* @param networkId
* CDMA network ID (NID).
*/
public void setNetworkId(Integer networkId) {
this.networkId = networkId;
}
/**
*
* CDMA network ID (NID).
*
*
* @return CDMA network ID (NID).
*/
public Integer getNetworkId() {
return this.networkId;
}
/**
*
* CDMA network ID (NID).
*
*
* @param networkId
* CDMA network ID (NID).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withNetworkId(Integer networkId) {
setNetworkId(networkId);
return this;
}
/**
*
* CDMA base station ID (BSID).
*
*
* @param baseStationId
* CDMA base station ID (BSID).
*/
public void setBaseStationId(Integer baseStationId) {
this.baseStationId = baseStationId;
}
/**
*
* CDMA base station ID (BSID).
*
*
* @return CDMA base station ID (BSID).
*/
public Integer getBaseStationId() {
return this.baseStationId;
}
/**
*
* CDMA base station ID (BSID).
*
*
* @param baseStationId
* CDMA base station ID (BSID).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withBaseStationId(Integer baseStationId) {
setBaseStationId(baseStationId);
return this;
}
/**
*
* CDMA registration zone (RZ).
*
*
* @param registrationZone
* CDMA registration zone (RZ).
*/
public void setRegistrationZone(Integer registrationZone) {
this.registrationZone = registrationZone;
}
/**
*
* CDMA registration zone (RZ).
*
*
* @return CDMA registration zone (RZ).
*/
public Integer getRegistrationZone() {
return this.registrationZone;
}
/**
*
* CDMA registration zone (RZ).
*
*
* @param registrationZone
* CDMA registration zone (RZ).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withRegistrationZone(Integer registrationZone) {
setRegistrationZone(registrationZone);
return this;
}
/**
*
* CDMA local identification (local ID) parameters.
*
*
* @param cdmaLocalId
* CDMA local identification (local ID) parameters.
*/
public void setCdmaLocalId(CdmaLocalId cdmaLocalId) {
this.cdmaLocalId = cdmaLocalId;
}
/**
*
* CDMA local identification (local ID) parameters.
*
*
* @return CDMA local identification (local ID) parameters.
*/
public CdmaLocalId getCdmaLocalId() {
return this.cdmaLocalId;
}
/**
*
* CDMA local identification (local ID) parameters.
*
*
* @param cdmaLocalId
* CDMA local identification (local ID) parameters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withCdmaLocalId(CdmaLocalId cdmaLocalId) {
setCdmaLocalId(cdmaLocalId);
return this;
}
/**
*
* Transmit power level of the pilot signal, measured in dBm (decibel-milliwatts).
*
*
* @param pilotPower
* Transmit power level of the pilot signal, measured in dBm (decibel-milliwatts).
*/
public void setPilotPower(Integer pilotPower) {
this.pilotPower = pilotPower;
}
/**
*
* Transmit power level of the pilot signal, measured in dBm (decibel-milliwatts).
*
*
* @return Transmit power level of the pilot signal, measured in dBm (decibel-milliwatts).
*/
public Integer getPilotPower() {
return this.pilotPower;
}
/**
*
* Transmit power level of the pilot signal, measured in dBm (decibel-milliwatts).
*
*
* @param pilotPower
* Transmit power level of the pilot signal, measured in dBm (decibel-milliwatts).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withPilotPower(Integer pilotPower) {
setPilotPower(pilotPower);
return this;
}
/**
*
* CDMA base station latitude in degrees.
*
*
* @param baseLat
* CDMA base station latitude in degrees.
*/
public void setBaseLat(Float baseLat) {
this.baseLat = baseLat;
}
/**
*
* CDMA base station latitude in degrees.
*
*
* @return CDMA base station latitude in degrees.
*/
public Float getBaseLat() {
return this.baseLat;
}
/**
*
* CDMA base station latitude in degrees.
*
*
* @param baseLat
* CDMA base station latitude in degrees.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withBaseLat(Float baseLat) {
setBaseLat(baseLat);
return this;
}
/**
*
* CDMA base station longitude in degrees.
*
*
* @param baseLng
* CDMA base station longitude in degrees.
*/
public void setBaseLng(Float baseLng) {
this.baseLng = baseLng;
}
/**
*
* CDMA base station longitude in degrees.
*
*
* @return CDMA base station longitude in degrees.
*/
public Float getBaseLng() {
return this.baseLng;
}
/**
*
* CDMA base station longitude in degrees.
*
*
* @param baseLng
* CDMA base station longitude in degrees.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withBaseLng(Float baseLng) {
setBaseLng(baseLng);
return this;
}
/**
*
* CDMA network measurement reports.
*
*
* @return CDMA network measurement reports.
*/
public java.util.List getCdmaNmr() {
return cdmaNmr;
}
/**
*
* CDMA network measurement reports.
*
*
* @param cdmaNmr
* CDMA network measurement reports.
*/
public void setCdmaNmr(java.util.Collection cdmaNmr) {
if (cdmaNmr == null) {
this.cdmaNmr = null;
return;
}
this.cdmaNmr = new java.util.ArrayList(cdmaNmr);
}
/**
*
* CDMA network measurement reports.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCdmaNmr(java.util.Collection)} or {@link #withCdmaNmr(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param cdmaNmr
* CDMA network measurement reports.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withCdmaNmr(CdmaNmrObj... cdmaNmr) {
if (this.cdmaNmr == null) {
setCdmaNmr(new java.util.ArrayList(cdmaNmr.length));
}
for (CdmaNmrObj ele : cdmaNmr) {
this.cdmaNmr.add(ele);
}
return this;
}
/**
*
* CDMA network measurement reports.
*
*
* @param cdmaNmr
* CDMA network measurement reports.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CdmaObj withCdmaNmr(java.util.Collection cdmaNmr) {
setCdmaNmr(cdmaNmr);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSystemId() != null)
sb.append("SystemId: ").append(getSystemId()).append(",");
if (getNetworkId() != null)
sb.append("NetworkId: ").append(getNetworkId()).append(",");
if (getBaseStationId() != null)
sb.append("BaseStationId: ").append(getBaseStationId()).append(",");
if (getRegistrationZone() != null)
sb.append("RegistrationZone: ").append(getRegistrationZone()).append(",");
if (getCdmaLocalId() != null)
sb.append("CdmaLocalId: ").append(getCdmaLocalId()).append(",");
if (getPilotPower() != null)
sb.append("PilotPower: ").append(getPilotPower()).append(",");
if (getBaseLat() != null)
sb.append("BaseLat: ").append(getBaseLat()).append(",");
if (getBaseLng() != null)
sb.append("BaseLng: ").append(getBaseLng()).append(",");
if (getCdmaNmr() != null)
sb.append("CdmaNmr: ").append(getCdmaNmr());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CdmaObj == false)
return false;
CdmaObj other = (CdmaObj) obj;
if (other.getSystemId() == null ^ this.getSystemId() == null)
return false;
if (other.getSystemId() != null && other.getSystemId().equals(this.getSystemId()) == false)
return false;
if (other.getNetworkId() == null ^ this.getNetworkId() == null)
return false;
if (other.getNetworkId() != null && other.getNetworkId().equals(this.getNetworkId()) == false)
return false;
if (other.getBaseStationId() == null ^ this.getBaseStationId() == null)
return false;
if (other.getBaseStationId() != null && other.getBaseStationId().equals(this.getBaseStationId()) == false)
return false;
if (other.getRegistrationZone() == null ^ this.getRegistrationZone() == null)
return false;
if (other.getRegistrationZone() != null && other.getRegistrationZone().equals(this.getRegistrationZone()) == false)
return false;
if (other.getCdmaLocalId() == null ^ this.getCdmaLocalId() == null)
return false;
if (other.getCdmaLocalId() != null && other.getCdmaLocalId().equals(this.getCdmaLocalId()) == false)
return false;
if (other.getPilotPower() == null ^ this.getPilotPower() == null)
return false;
if (other.getPilotPower() != null && other.getPilotPower().equals(this.getPilotPower()) == false)
return false;
if (other.getBaseLat() == null ^ this.getBaseLat() == null)
return false;
if (other.getBaseLat() != null && other.getBaseLat().equals(this.getBaseLat()) == false)
return false;
if (other.getBaseLng() == null ^ this.getBaseLng() == null)
return false;
if (other.getBaseLng() != null && other.getBaseLng().equals(this.getBaseLng()) == false)
return false;
if (other.getCdmaNmr() == null ^ this.getCdmaNmr() == null)
return false;
if (other.getCdmaNmr() != null && other.getCdmaNmr().equals(this.getCdmaNmr()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSystemId() == null) ? 0 : getSystemId().hashCode());
hashCode = prime * hashCode + ((getNetworkId() == null) ? 0 : getNetworkId().hashCode());
hashCode = prime * hashCode + ((getBaseStationId() == null) ? 0 : getBaseStationId().hashCode());
hashCode = prime * hashCode + ((getRegistrationZone() == null) ? 0 : getRegistrationZone().hashCode());
hashCode = prime * hashCode + ((getCdmaLocalId() == null) ? 0 : getCdmaLocalId().hashCode());
hashCode = prime * hashCode + ((getPilotPower() == null) ? 0 : getPilotPower().hashCode());
hashCode = prime * hashCode + ((getBaseLat() == null) ? 0 : getBaseLat().hashCode());
hashCode = prime * hashCode + ((getBaseLng() == null) ? 0 : getBaseLng().hashCode());
hashCode = prime * hashCode + ((getCdmaNmr() == null) ? 0 : getCdmaNmr().hashCode());
return hashCode;
}
@Override
public CdmaObj clone() {
try {
return (CdmaObj) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.iotwireless.model.transform.CdmaObjMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}