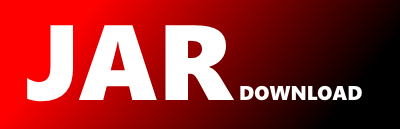
com.amazonaws.services.iotwireless.model.LoRaWANServiceProfile Maven / Gradle / Ivy
Show all versions of aws-java-sdk-iotwireless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.iotwireless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* LoRaWANServiceProfile object.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class LoRaWANServiceProfile implements Serializable, Cloneable, StructuredPojo {
/**
*
* The AddGWMetaData value.
*
*/
private Boolean addGwMetadata;
/**
*
* The DrMin value.
*
*/
private Integer drMin;
/**
*
* The DrMax value.
*
*/
private Integer drMax;
/**
*
* The PRAllowed value that describes whether passive roaming is allowed.
*
*/
private Boolean prAllowed;
/**
*
* The RAAllowed value that describes whether roaming activation is allowed.
*
*/
private Boolean raAllowed;
/**
*
* The AddGWMetaData value.
*
*
* @param addGwMetadata
* The AddGWMetaData value.
*/
public void setAddGwMetadata(Boolean addGwMetadata) {
this.addGwMetadata = addGwMetadata;
}
/**
*
* The AddGWMetaData value.
*
*
* @return The AddGWMetaData value.
*/
public Boolean getAddGwMetadata() {
return this.addGwMetadata;
}
/**
*
* The AddGWMetaData value.
*
*
* @param addGwMetadata
* The AddGWMetaData value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LoRaWANServiceProfile withAddGwMetadata(Boolean addGwMetadata) {
setAddGwMetadata(addGwMetadata);
return this;
}
/**
*
* The AddGWMetaData value.
*
*
* @return The AddGWMetaData value.
*/
public Boolean isAddGwMetadata() {
return this.addGwMetadata;
}
/**
*
* The DrMin value.
*
*
* @param drMin
* The DrMin value.
*/
public void setDrMin(Integer drMin) {
this.drMin = drMin;
}
/**
*
* The DrMin value.
*
*
* @return The DrMin value.
*/
public Integer getDrMin() {
return this.drMin;
}
/**
*
* The DrMin value.
*
*
* @param drMin
* The DrMin value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LoRaWANServiceProfile withDrMin(Integer drMin) {
setDrMin(drMin);
return this;
}
/**
*
* The DrMax value.
*
*
* @param drMax
* The DrMax value.
*/
public void setDrMax(Integer drMax) {
this.drMax = drMax;
}
/**
*
* The DrMax value.
*
*
* @return The DrMax value.
*/
public Integer getDrMax() {
return this.drMax;
}
/**
*
* The DrMax value.
*
*
* @param drMax
* The DrMax value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LoRaWANServiceProfile withDrMax(Integer drMax) {
setDrMax(drMax);
return this;
}
/**
*
* The PRAllowed value that describes whether passive roaming is allowed.
*
*
* @param prAllowed
* The PRAllowed value that describes whether passive roaming is allowed.
*/
public void setPrAllowed(Boolean prAllowed) {
this.prAllowed = prAllowed;
}
/**
*
* The PRAllowed value that describes whether passive roaming is allowed.
*
*
* @return The PRAllowed value that describes whether passive roaming is allowed.
*/
public Boolean getPrAllowed() {
return this.prAllowed;
}
/**
*
* The PRAllowed value that describes whether passive roaming is allowed.
*
*
* @param prAllowed
* The PRAllowed value that describes whether passive roaming is allowed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LoRaWANServiceProfile withPrAllowed(Boolean prAllowed) {
setPrAllowed(prAllowed);
return this;
}
/**
*
* The PRAllowed value that describes whether passive roaming is allowed.
*
*
* @return The PRAllowed value that describes whether passive roaming is allowed.
*/
public Boolean isPrAllowed() {
return this.prAllowed;
}
/**
*
* The RAAllowed value that describes whether roaming activation is allowed.
*
*
* @param raAllowed
* The RAAllowed value that describes whether roaming activation is allowed.
*/
public void setRaAllowed(Boolean raAllowed) {
this.raAllowed = raAllowed;
}
/**
*
* The RAAllowed value that describes whether roaming activation is allowed.
*
*
* @return The RAAllowed value that describes whether roaming activation is allowed.
*/
public Boolean getRaAllowed() {
return this.raAllowed;
}
/**
*
* The RAAllowed value that describes whether roaming activation is allowed.
*
*
* @param raAllowed
* The RAAllowed value that describes whether roaming activation is allowed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LoRaWANServiceProfile withRaAllowed(Boolean raAllowed) {
setRaAllowed(raAllowed);
return this;
}
/**
*
* The RAAllowed value that describes whether roaming activation is allowed.
*
*
* @return The RAAllowed value that describes whether roaming activation is allowed.
*/
public Boolean isRaAllowed() {
return this.raAllowed;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAddGwMetadata() != null)
sb.append("AddGwMetadata: ").append(getAddGwMetadata()).append(",");
if (getDrMin() != null)
sb.append("DrMin: ").append(getDrMin()).append(",");
if (getDrMax() != null)
sb.append("DrMax: ").append(getDrMax()).append(",");
if (getPrAllowed() != null)
sb.append("PrAllowed: ").append(getPrAllowed()).append(",");
if (getRaAllowed() != null)
sb.append("RaAllowed: ").append(getRaAllowed());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof LoRaWANServiceProfile == false)
return false;
LoRaWANServiceProfile other = (LoRaWANServiceProfile) obj;
if (other.getAddGwMetadata() == null ^ this.getAddGwMetadata() == null)
return false;
if (other.getAddGwMetadata() != null && other.getAddGwMetadata().equals(this.getAddGwMetadata()) == false)
return false;
if (other.getDrMin() == null ^ this.getDrMin() == null)
return false;
if (other.getDrMin() != null && other.getDrMin().equals(this.getDrMin()) == false)
return false;
if (other.getDrMax() == null ^ this.getDrMax() == null)
return false;
if (other.getDrMax() != null && other.getDrMax().equals(this.getDrMax()) == false)
return false;
if (other.getPrAllowed() == null ^ this.getPrAllowed() == null)
return false;
if (other.getPrAllowed() != null && other.getPrAllowed().equals(this.getPrAllowed()) == false)
return false;
if (other.getRaAllowed() == null ^ this.getRaAllowed() == null)
return false;
if (other.getRaAllowed() != null && other.getRaAllowed().equals(this.getRaAllowed()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAddGwMetadata() == null) ? 0 : getAddGwMetadata().hashCode());
hashCode = prime * hashCode + ((getDrMin() == null) ? 0 : getDrMin().hashCode());
hashCode = prime * hashCode + ((getDrMax() == null) ? 0 : getDrMax().hashCode());
hashCode = prime * hashCode + ((getPrAllowed() == null) ? 0 : getPrAllowed().hashCode());
hashCode = prime * hashCode + ((getRaAllowed() == null) ? 0 : getRaAllowed().hashCode());
return hashCode;
}
@Override
public LoRaWANServiceProfile clone() {
try {
return (LoRaWANServiceProfile) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.iotwireless.model.transform.LoRaWANServiceProfileMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}