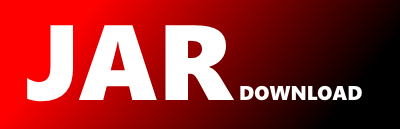
com.amazonaws.services.ivs.model.Channel Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ivs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Object specifying a channel.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Channel implements Serializable, Cloneable, StructuredPojo {
/**
*
* Channel ARN.
*
*/
private String arn;
/**
*
* Whether the channel is private (enabled for playback authorization). Default: false
.
*
*/
private Boolean authorized;
/**
*
* Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming software.
*
*/
private String ingestEndpoint;
/**
*
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the Amazon IVS
* console, LOW
and NORMAL
correspond to Ultra-low and Standard, respectively.)
*
*/
private String latencyMode;
/**
*
* Channel name.
*
*/
private String name;
/**
*
* Channel playback URL.
*
*/
private String playbackUrl;
/**
*
* Recording-configuration ARN. A value other than an empty string indicates that recording is enabled. Default: ""
* (empty string, recording is disabled).
*
*/
private String recordingConfigurationArn;
/**
*
* Array of 1-50 maps, each of the form string:string (key:value)
.
*
*/
private java.util.Map tags;
/**
*
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable resolution or
* bitrate, the stream probably will disconnect immediately. Default: STANDARD
. Valid values:
*
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give viewers
* the best experience for their devices and network conditions. Resolution can be up to 1080p and bitrate can be up
* to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is
* limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
*
*/
private String type;
/**
*
* Channel ARN.
*
*
* @param arn
* Channel ARN.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* Channel ARN.
*
*
* @return Channel ARN.
*/
public String getArn() {
return this.arn;
}
/**
*
* Channel ARN.
*
*
* @param arn
* Channel ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* Whether the channel is private (enabled for playback authorization). Default: false
.
*
*
* @param authorized
* Whether the channel is private (enabled for playback authorization). Default: false
.
*/
public void setAuthorized(Boolean authorized) {
this.authorized = authorized;
}
/**
*
* Whether the channel is private (enabled for playback authorization). Default: false
.
*
*
* @return Whether the channel is private (enabled for playback authorization). Default: false
.
*/
public Boolean getAuthorized() {
return this.authorized;
}
/**
*
* Whether the channel is private (enabled for playback authorization). Default: false
.
*
*
* @param authorized
* Whether the channel is private (enabled for playback authorization). Default: false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel withAuthorized(Boolean authorized) {
setAuthorized(authorized);
return this;
}
/**
*
* Whether the channel is private (enabled for playback authorization). Default: false
.
*
*
* @return Whether the channel is private (enabled for playback authorization). Default: false
.
*/
public Boolean isAuthorized() {
return this.authorized;
}
/**
*
* Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming software.
*
*
* @param ingestEndpoint
* Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming
* software.
*/
public void setIngestEndpoint(String ingestEndpoint) {
this.ingestEndpoint = ingestEndpoint;
}
/**
*
* Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming software.
*
*
* @return Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming
* software.
*/
public String getIngestEndpoint() {
return this.ingestEndpoint;
}
/**
*
* Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming software.
*
*
* @param ingestEndpoint
* Channel ingest endpoint, part of the definition of an ingest server, used when you set up streaming
* software.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel withIngestEndpoint(String ingestEndpoint) {
setIngestEndpoint(ingestEndpoint);
return this;
}
/**
*
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the Amazon IVS
* console, LOW
and NORMAL
correspond to Ultra-low and Standard, respectively.)
*
*
* @param latencyMode
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the
* Amazon IVS console, LOW
and NORMAL
correspond to Ultra-low and Standard,
* respectively.)
* @see ChannelLatencyMode
*/
public void setLatencyMode(String latencyMode) {
this.latencyMode = latencyMode;
}
/**
*
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the Amazon IVS
* console, LOW
and NORMAL
correspond to Ultra-low and Standard, respectively.)
*
*
* @return Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the
* Amazon IVS console, LOW
and NORMAL
correspond to Ultra-low and Standard,
* respectively.)
* @see ChannelLatencyMode
*/
public String getLatencyMode() {
return this.latencyMode;
}
/**
*
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the Amazon IVS
* console, LOW
and NORMAL
correspond to Ultra-low and Standard, respectively.)
*
*
* @param latencyMode
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the
* Amazon IVS console, LOW
and NORMAL
correspond to Ultra-low and Standard,
* respectively.)
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChannelLatencyMode
*/
public Channel withLatencyMode(String latencyMode) {
setLatencyMode(latencyMode);
return this;
}
/**
*
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the Amazon IVS
* console, LOW
and NORMAL
correspond to Ultra-low and Standard, respectively.)
*
*
* @param latencyMode
* Channel latency mode. Use NORMAL
to broadcast and deliver live video up to Full HD. Use
* LOW
for near-real-time interaction with viewers. Default: LOW
. (Note: In the
* Amazon IVS console, LOW
and NORMAL
correspond to Ultra-low and Standard,
* respectively.)
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChannelLatencyMode
*/
public Channel withLatencyMode(ChannelLatencyMode latencyMode) {
this.latencyMode = latencyMode.toString();
return this;
}
/**
*
* Channel name.
*
*
* @param name
* Channel name.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* Channel name.
*
*
* @return Channel name.
*/
public String getName() {
return this.name;
}
/**
*
* Channel name.
*
*
* @param name
* Channel name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel withName(String name) {
setName(name);
return this;
}
/**
*
* Channel playback URL.
*
*
* @param playbackUrl
* Channel playback URL.
*/
public void setPlaybackUrl(String playbackUrl) {
this.playbackUrl = playbackUrl;
}
/**
*
* Channel playback URL.
*
*
* @return Channel playback URL.
*/
public String getPlaybackUrl() {
return this.playbackUrl;
}
/**
*
* Channel playback URL.
*
*
* @param playbackUrl
* Channel playback URL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel withPlaybackUrl(String playbackUrl) {
setPlaybackUrl(playbackUrl);
return this;
}
/**
*
* Recording-configuration ARN. A value other than an empty string indicates that recording is enabled. Default: ""
* (empty string, recording is disabled).
*
*
* @param recordingConfigurationArn
* Recording-configuration ARN. A value other than an empty string indicates that recording is enabled.
* Default: "" (empty string, recording is disabled).
*/
public void setRecordingConfigurationArn(String recordingConfigurationArn) {
this.recordingConfigurationArn = recordingConfigurationArn;
}
/**
*
* Recording-configuration ARN. A value other than an empty string indicates that recording is enabled. Default: ""
* (empty string, recording is disabled).
*
*
* @return Recording-configuration ARN. A value other than an empty string indicates that recording is enabled.
* Default: "" (empty string, recording is disabled).
*/
public String getRecordingConfigurationArn() {
return this.recordingConfigurationArn;
}
/**
*
* Recording-configuration ARN. A value other than an empty string indicates that recording is enabled. Default: ""
* (empty string, recording is disabled).
*
*
* @param recordingConfigurationArn
* Recording-configuration ARN. A value other than an empty string indicates that recording is enabled.
* Default: "" (empty string, recording is disabled).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel withRecordingConfigurationArn(String recordingConfigurationArn) {
setRecordingConfigurationArn(recordingConfigurationArn);
return this;
}
/**
*
* Array of 1-50 maps, each of the form string:string (key:value)
.
*
*
* @return Array of 1-50 maps, each of the form string:string (key:value)
.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* Array of 1-50 maps, each of the form string:string (key:value)
.
*
*
* @param tags
* Array of 1-50 maps, each of the form string:string (key:value)
.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* Array of 1-50 maps, each of the form string:string (key:value)
.
*
*
* @param tags
* Array of 1-50 maps, each of the form string:string (key:value)
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see Channel#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public Channel addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Channel clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable resolution or
* bitrate, the stream probably will disconnect immediately. Default: STANDARD
. Valid values:
*
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give viewers
* the best experience for their devices and network conditions. Resolution can be up to 1080p and bitrate can be up
* to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is
* limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
*
*
* @param type
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable
* resolution or bitrate, the stream probably will disconnect immediately. Default: STANDARD
* . Valid values:
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give
* viewers the best experience for their devices and network conditions. Resolution can be up to 1080p and
* bitrate can be up to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio
* is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice
* is limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
* @see ChannelType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable resolution or
* bitrate, the stream probably will disconnect immediately. Default: STANDARD
. Valid values:
*
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give viewers
* the best experience for their devices and network conditions. Resolution can be up to 1080p and bitrate can be up
* to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is
* limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
*
*
* @return Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable
* resolution or bitrate, the stream probably will disconnect immediately. Default:
* STANDARD
. Valid values:
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give
* viewers the best experience for their devices and network conditions. Resolution can be up to 1080p and
* bitrate can be up to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio
* is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice
* is limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
* @see ChannelType
*/
public String getType() {
return this.type;
}
/**
*
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable resolution or
* bitrate, the stream probably will disconnect immediately. Default: STANDARD
. Valid values:
*
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give viewers
* the best experience for their devices and network conditions. Resolution can be up to 1080p and bitrate can be up
* to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is
* limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
*
*
* @param type
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable
* resolution or bitrate, the stream probably will disconnect immediately. Default: STANDARD
* . Valid values:
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give
* viewers the best experience for their devices and network conditions. Resolution can be up to 1080p and
* bitrate can be up to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio
* is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice
* is limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChannelType
*/
public Channel withType(String type) {
setType(type);
return this;
}
/**
*
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable resolution or
* bitrate, the stream probably will disconnect immediately. Default: STANDARD
. Valid values:
*
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give viewers
* the best experience for their devices and network conditions. Resolution can be up to 1080p and bitrate can be up
* to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice is
* limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
*
*
* @param type
* Channel type, which determines the allowable resolution and bitrate. If you exceed the allowable
* resolution or bitrate, the stream probably will disconnect immediately. Default: STANDARD
* . Valid values:
*
* -
*
* STANDARD
: Multiple qualities are generated from the original input, to automatically give
* viewers the best experience for their devices and network conditions. Resolution can be up to 1080p and
* bitrate can be up to 8.5 Mbps. Audio is transcoded only for renditions 360p and below; above that, audio
* is passed through.
*
*
* -
*
* BASIC
: Amazon IVS delivers the original input to viewers. The viewer’s video-quality choice
* is limited to the original input. Resolution can be up to 480p and bitrate can be up to 1.5 Mbps.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChannelType
*/
public Channel withType(ChannelType type) {
this.type = type.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getAuthorized() != null)
sb.append("Authorized: ").append(getAuthorized()).append(",");
if (getIngestEndpoint() != null)
sb.append("IngestEndpoint: ").append(getIngestEndpoint()).append(",");
if (getLatencyMode() != null)
sb.append("LatencyMode: ").append(getLatencyMode()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getPlaybackUrl() != null)
sb.append("PlaybackUrl: ").append(getPlaybackUrl()).append(",");
if (getRecordingConfigurationArn() != null)
sb.append("RecordingConfigurationArn: ").append(getRecordingConfigurationArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Channel == false)
return false;
Channel other = (Channel) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getAuthorized() == null ^ this.getAuthorized() == null)
return false;
if (other.getAuthorized() != null && other.getAuthorized().equals(this.getAuthorized()) == false)
return false;
if (other.getIngestEndpoint() == null ^ this.getIngestEndpoint() == null)
return false;
if (other.getIngestEndpoint() != null && other.getIngestEndpoint().equals(this.getIngestEndpoint()) == false)
return false;
if (other.getLatencyMode() == null ^ this.getLatencyMode() == null)
return false;
if (other.getLatencyMode() != null && other.getLatencyMode().equals(this.getLatencyMode()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getPlaybackUrl() == null ^ this.getPlaybackUrl() == null)
return false;
if (other.getPlaybackUrl() != null && other.getPlaybackUrl().equals(this.getPlaybackUrl()) == false)
return false;
if (other.getRecordingConfigurationArn() == null ^ this.getRecordingConfigurationArn() == null)
return false;
if (other.getRecordingConfigurationArn() != null && other.getRecordingConfigurationArn().equals(this.getRecordingConfigurationArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getAuthorized() == null) ? 0 : getAuthorized().hashCode());
hashCode = prime * hashCode + ((getIngestEndpoint() == null) ? 0 : getIngestEndpoint().hashCode());
hashCode = prime * hashCode + ((getLatencyMode() == null) ? 0 : getLatencyMode().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getPlaybackUrl() == null) ? 0 : getPlaybackUrl().hashCode());
hashCode = prime * hashCode + ((getRecordingConfigurationArn() == null) ? 0 : getRecordingConfigurationArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
return hashCode;
}
@Override
public Channel clone() {
try {
return (Channel) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ivs.model.transform.ChannelMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}