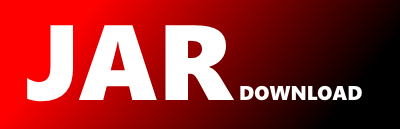
com.amazonaws.services.ivschat.AmazonivschatAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ivschat Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ivschat;
import javax.annotation.Generated;
import com.amazonaws.services.ivschat.model.*;
import com.amazonaws.client.AwsAsyncClientParams;
import com.amazonaws.annotation.ThreadSafe;
import java.util.concurrent.ExecutorService;
/**
* Client for accessing ivschat asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
*
* Introduction
*
*
* The Amazon IVS Chat control-plane API enables you to create and manage Amazon IVS Chat resources. You also need to
* integrate with the
* Amazon IVS Chat Messaging API, to enable users to interact with chat rooms in real time.
*
*
* The API is an AWS regional service. For a list of supported regions and Amazon IVS Chat HTTPS service endpoints, see
* the Amazon IVS Chat information on the Amazon IVS
* page in the AWS General Reference.
*
*
* This document describes HTTP operations. There is a separate messaging API for managing Chat resources; see
* the Amazon IVS Chat
* Messaging API Reference.
*
*
* Notes on terminology:
*
*
* -
*
* You create service applications using the Amazon IVS Chat API. We refer to these as applications.
*
*
* -
*
* You create front-end client applications (browser and Android/iOS apps) using the Amazon IVS Chat Messaging API. We
* refer to these as clients.
*
*
*
*
* Resources
*
*
* The following resources are part of Amazon IVS Chat:
*
*
* -
*
* LoggingConfiguration — A configuration that allows customers to store and record sent messages in a chat room.
* See the Logging Configuration endpoints for more information.
*
*
* -
*
* Room — The central Amazon IVS Chat resource through which clients connect to and exchange chat messages. See
* the Room endpoints for more information.
*
*
*
*
* Tagging
*
*
* A tag is a metadata label that you assign to an AWS resource. A tag comprises a key and a value,
* both set by you. For example, you might set a tag as topic:nature
to label a particular video category.
* See Tagging AWS Resources for more
* information, including restrictions that apply to tags and "Tag naming limits and requirements"; Amazon IVS Chat has
* no service-specific constraints beyond what is documented there.
*
*
* Tags can help you identify and organize your AWS resources. For example, you can use the same tag for different
* resources to indicate that they are related. You can also use tags to manage access (see Access Tags).
*
*
* The Amazon IVS Chat API has these tag-related endpoints: TagResource, UntagResource, and
* ListTagsForResource. The following resource supports tagging: Room.
*
*
* At most 50 tags can be applied to a resource.
*
*
* API Access Security
*
*
* Your Amazon IVS Chat applications (service applications and clients) must be authenticated and authorized to access
* Amazon IVS Chat resources. Note the differences between these concepts:
*
*
* -
*
* Authentication is about verifying identity. Requests to the Amazon IVS Chat API must be signed to verify your
* identity.
*
*
* -
*
* Authorization is about granting permissions. Your IAM roles need to have permissions for Amazon IVS Chat API
* requests.
*
*
*
*
* Users (viewers) connect to a room using secure access tokens that you create using the CreateChatToken
* endpoint through the AWS SDK. You call CreateChatToken for every user’s chat session, passing identity and
* authorization information about the user.
*
*
* Signing API Requests
*
*
* HTTP API requests must be signed with an AWS SigV4 signature using your AWS security credentials. The AWS Command
* Line Interface (CLI) and the AWS SDKs take care of signing the underlying API calls for you. However, if your
* application calls the Amazon IVS Chat HTTP API directly, it’s your responsibility to sign the requests.
*
*
* You generate a signature using valid AWS credentials for an IAM role that has permission to perform the requested
* action. For example, DeleteMessage requests must be made using an IAM role that has the
* ivschat:DeleteMessage
permission.
*
*
* For more information:
*
*
* -
*
* Authentication and generating signatures — See Authenticating Requests
* (Amazon Web Services Signature Version 4) in the Amazon Web Services General Reference.
*
*
* -
*
* Managing Amazon IVS permissions — See Identity and Access Management on the
* Security page of the Amazon IVS User Guide.
*
*
*
*
* Amazon Resource Names (ARNs)
*
*
* ARNs uniquely identify AWS resources. An ARN is required when you need to specify a resource unambiguously across all
* of AWS, such as in IAM policies and API calls. For more information, see Amazon Resource Names in the
* AWS General Reference.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonivschatAsyncClient extends AmazonivschatClient implements AmazonivschatAsync {
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
private final java.util.concurrent.ExecutorService executorService;
public static AmazonivschatAsyncClientBuilder asyncBuilder() {
return AmazonivschatAsyncClientBuilder.standard();
}
/**
* Constructs a new asynchronous client to invoke service methods on ivschat using the specified parameters.
*
* @param asyncClientParams
* Object providing client parameters.
*/
AmazonivschatAsyncClient(AwsAsyncClientParams asyncClientParams) {
this(asyncClientParams, false);
}
/**
* Constructs a new asynchronous client to invoke service methods on ivschat using the specified parameters.
*
* @param asyncClientParams
* Object providing client parameters.
* @param endpointDiscoveryEnabled
* true will enable endpoint discovery if the service supports it.
*/
AmazonivschatAsyncClient(AwsAsyncClientParams asyncClientParams, boolean endpointDiscoveryEnabled) {
super(asyncClientParams, endpointDiscoveryEnabled);
this.executorService = asyncClientParams.getExecutor();
}
/**
* Returns the executor service used by this client to execute async requests.
*
* @return The executor service used by this client to execute async requests.
*/
public ExecutorService getExecutorService() {
return executorService;
}
@Override
public java.util.concurrent.Future createChatTokenAsync(CreateChatTokenRequest request) {
return createChatTokenAsync(request, null);
}
@Override
public java.util.concurrent.Future createChatTokenAsync(final CreateChatTokenRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final CreateChatTokenRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public CreateChatTokenResult call() throws Exception {
CreateChatTokenResult result = null;
try {
result = executeCreateChatToken(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createLoggingConfigurationAsync(CreateLoggingConfigurationRequest request) {
return createLoggingConfigurationAsync(request, null);
}
@Override
public java.util.concurrent.Future createLoggingConfigurationAsync(final CreateLoggingConfigurationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final CreateLoggingConfigurationRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public CreateLoggingConfigurationResult call() throws Exception {
CreateLoggingConfigurationResult result = null;
try {
result = executeCreateLoggingConfiguration(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createRoomAsync(CreateRoomRequest request) {
return createRoomAsync(request, null);
}
@Override
public java.util.concurrent.Future createRoomAsync(final CreateRoomRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final CreateRoomRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public CreateRoomResult call() throws Exception {
CreateRoomResult result = null;
try {
result = executeCreateRoom(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteLoggingConfigurationAsync(DeleteLoggingConfigurationRequest request) {
return deleteLoggingConfigurationAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteLoggingConfigurationAsync(final DeleteLoggingConfigurationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DeleteLoggingConfigurationRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DeleteLoggingConfigurationResult call() throws Exception {
DeleteLoggingConfigurationResult result = null;
try {
result = executeDeleteLoggingConfiguration(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteMessageAsync(DeleteMessageRequest request) {
return deleteMessageAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteMessageAsync(final DeleteMessageRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DeleteMessageRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DeleteMessageResult call() throws Exception {
DeleteMessageResult result = null;
try {
result = executeDeleteMessage(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteRoomAsync(DeleteRoomRequest request) {
return deleteRoomAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteRoomAsync(final DeleteRoomRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DeleteRoomRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DeleteRoomResult call() throws Exception {
DeleteRoomResult result = null;
try {
result = executeDeleteRoom(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future disconnectUserAsync(DisconnectUserRequest request) {
return disconnectUserAsync(request, null);
}
@Override
public java.util.concurrent.Future disconnectUserAsync(final DisconnectUserRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DisconnectUserRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DisconnectUserResult call() throws Exception {
DisconnectUserResult result = null;
try {
result = executeDisconnectUser(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getLoggingConfigurationAsync(GetLoggingConfigurationRequest request) {
return getLoggingConfigurationAsync(request, null);
}
@Override
public java.util.concurrent.Future getLoggingConfigurationAsync(final GetLoggingConfigurationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final GetLoggingConfigurationRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public GetLoggingConfigurationResult call() throws Exception {
GetLoggingConfigurationResult result = null;
try {
result = executeGetLoggingConfiguration(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getRoomAsync(GetRoomRequest request) {
return getRoomAsync(request, null);
}
@Override
public java.util.concurrent.Future getRoomAsync(final GetRoomRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final GetRoomRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public GetRoomResult call() throws Exception {
GetRoomResult result = null;
try {
result = executeGetRoom(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listLoggingConfigurationsAsync(ListLoggingConfigurationsRequest request) {
return listLoggingConfigurationsAsync(request, null);
}
@Override
public java.util.concurrent.Future listLoggingConfigurationsAsync(final ListLoggingConfigurationsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final ListLoggingConfigurationsRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public ListLoggingConfigurationsResult call() throws Exception {
ListLoggingConfigurationsResult result = null;
try {
result = executeListLoggingConfigurations(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listRoomsAsync(ListRoomsRequest request) {
return listRoomsAsync(request, null);
}
@Override
public java.util.concurrent.Future listRoomsAsync(final ListRoomsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final ListRoomsRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public ListRoomsResult call() throws Exception {
ListRoomsResult result = null;
try {
result = executeListRooms(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest request) {
return listTagsForResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future listTagsForResourceAsync(final ListTagsForResourceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final ListTagsForResourceRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public ListTagsForResourceResult call() throws Exception {
ListTagsForResourceResult result = null;
try {
result = executeListTagsForResource(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future sendEventAsync(SendEventRequest request) {
return sendEventAsync(request, null);
}
@Override
public java.util.concurrent.Future sendEventAsync(final SendEventRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final SendEventRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public SendEventResult call() throws Exception {
SendEventResult result = null;
try {
result = executeSendEvent(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future tagResourceAsync(TagResourceRequest request) {
return tagResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future tagResourceAsync(final TagResourceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final TagResourceRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public TagResourceResult call() throws Exception {
TagResourceResult result = null;
try {
result = executeTagResource(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future untagResourceAsync(UntagResourceRequest request) {
return untagResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future untagResourceAsync(final UntagResourceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final UntagResourceRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public UntagResourceResult call() throws Exception {
UntagResourceResult result = null;
try {
result = executeUntagResource(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateLoggingConfigurationAsync(UpdateLoggingConfigurationRequest request) {
return updateLoggingConfigurationAsync(request, null);
}
@Override
public java.util.concurrent.Future updateLoggingConfigurationAsync(final UpdateLoggingConfigurationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final UpdateLoggingConfigurationRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public UpdateLoggingConfigurationResult call() throws Exception {
UpdateLoggingConfigurationResult result = null;
try {
result = executeUpdateLoggingConfiguration(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateRoomAsync(UpdateRoomRequest request) {
return updateRoomAsync(request, null);
}
@Override
public java.util.concurrent.Future updateRoomAsync(final UpdateRoomRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final UpdateRoomRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public UpdateRoomResult call() throws Exception {
UpdateRoomResult result = null;
try {
result = executeUpdateRoom(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
/**
* Shuts down the client, releasing all managed resources. This includes forcibly terminating all pending
* asynchronous service calls. Clients who wish to give pending asynchronous service calls time to complete should
* call {@code getExecutorService().shutdown()} followed by {@code getExecutorService().awaitTermination()} prior to
* calling this method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
}