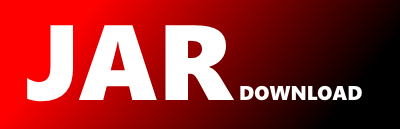
com.amazonaws.services.ivsrealtime.AmazonIVSRealTimeAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ivsrealtime;
import javax.annotation.Generated;
import com.amazonaws.services.ivsrealtime.model.*;
/**
* Interface for accessing ivsrealtime asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.ivsrealtime.AbstractAmazonIVSRealTimeAsync} instead.
*
*
*
* Introduction
*
*
* The Amazon Interactive Video Service (IVS) real-time API is REST compatible, using a standard HTTP API and an AWS
* EventBridge event stream for responses. JSON is used for both requests and responses, including errors.
*
*
* Terminology:
*
*
* -
*
* A stage is a virtual space where participants can exchange video in real time.
*
*
* -
*
* A participant token is a token that authenticates a participant when they join a stage.
*
*
* -
*
* A participant object represents participants (people) in the stage and contains information about them. When a
* token is created, it includes a participant ID; when a participant uses that token to join a stage, the participant
* is associated with that participant ID. There is a 1:1 mapping between participant tokens and participants.
*
*
* -
*
* Server-side composition: The composition process composites participants of a stage into a single video and
* forwards it to a set of outputs (e.g., IVS channels). Composition endpoints support this process.
*
*
* -
*
* Server-side composition: A composition controls the look of the outputs, including how participants are
* positioned in the video.
*
*
*
*
* Resources
*
*
* The following resources contain information about your IVS live stream (see Getting Started with Amazon IVS
* Real-Time Streaming):
*
*
* -
*
* Stage — A stage is a virtual space where participants can exchange video in real time.
*
*
*
*
* Tagging
*
*
* A tag is a metadata label that you assign to an AWS resource. A tag comprises a key and a value,
* both set by you. For example, you might set a tag as topic:nature
to label a particular video category.
* See Tagging AWS Resources for more
* information, including restrictions that apply to tags and "Tag naming limits and requirements"; Amazon IVS stages
* has no service-specific constraints beyond what is documented there.
*
*
* Tags can help you identify and organize your AWS resources. For example, you can use the same tag for different
* resources to indicate that they are related. You can also use tags to manage access (see Access Tags).
*
*
* The Amazon IVS real-time API has these tag-related endpoints: TagResource, UntagResource, and
* ListTagsForResource. The following resource supports tagging: Stage.
*
*
* At most 50 tags can be applied to a resource.
*
*
* Stages Endpoints
*
*
* -
*
* CreateParticipantToken — Creates an additional token for a specified stage. This can be done after stage
* creation or when tokens expire.
*
*
* -
*
* CreateStage — Creates a new stage (and optionally participant tokens).
*
*
* -
*
* DeleteStage — Shuts down and deletes the specified stage (disconnecting all participants).
*
*
* -
*
* DisconnectParticipant — Disconnects a specified participant and revokes the participant permanently from a
* specified stage.
*
*
* -
*
* GetParticipant — Gets information about the specified participant token.
*
*
* -
*
* GetStage — Gets information for the specified stage.
*
*
* -
*
* GetStageSession — Gets information for the specified stage session.
*
*
* -
*
* ListParticipantEvents — Lists events for a specified participant that occurred during a specified stage
* session.
*
*
* -
*
* ListParticipants — Lists all participants in a specified stage session.
*
*
* -
*
* ListStages — Gets summary information about all stages in your account, in the AWS region where the API
* request is processed.
*
*
* -
*
* ListStageSessions — Gets all sessions for a specified stage.
*
*
* -
*
* UpdateStage — Updates a stage’s configuration.
*
*
*
*
* Composition Endpoints
*
*
* -
*
* GetComposition — Gets information about the specified Composition resource.
*
*
* -
*
* ListCompositions — Gets summary information about all Compositions in your account, in the AWS region where
* the API request is processed.
*
*
* -
*
* StartComposition — Starts a Composition from a stage based on the configuration provided in the request.
*
*
* -
*
* StopComposition — Stops and deletes a Composition resource. Any broadcast from the Composition resource is
* stopped.
*
*
*
*
* EncoderConfiguration Endpoints
*
*
* -
*
* CreateEncoderConfiguration — Creates an EncoderConfiguration object.
*
*
* -
*
* DeleteEncoderConfiguration — Deletes an EncoderConfiguration resource. Ensures that no Compositions are using
* this template; otherwise, returns an error.
*
*
* -
*
* GetEncoderConfiguration — Gets information about the specified EncoderConfiguration resource.
*
*
* -
*
* ListEncoderConfigurations — Gets summary information about all EncoderConfigurations in your account, in the
* AWS region where the API request is processed.
*
*
*
*
* StorageConfiguration Endpoints
*
*
* -
*
* CreateStorageConfiguration — Creates a new storage configuration, used to enable recording to Amazon S3.
*
*
* -
*
* DeleteStorageConfiguration — Deletes the storage configuration for the specified ARN.
*
*
* -
*
* GetStorageConfiguration — Gets the storage configuration for the specified ARN.
*
*
* -
*
* ListStorageConfigurations — Gets summary information about all storage configurations in your account, in the
* AWS region where the API request is processed.
*
*
*
*
* Tags Endpoints
*
*
* -
*
* ListTagsForResource — Gets information about AWS tags for the specified ARN.
*
*
* -
*
* TagResource — Adds or updates tags for the AWS resource with the specified ARN.
*
*
* -
*
* UntagResource — Removes tags from the resource with the specified ARN.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonIVSRealTimeAsync extends AmazonIVSRealTime {
/**
*
* Creates an EncoderConfiguration object.
*
*
* @param createEncoderConfigurationRequest
* @return A Java Future containing the result of the CreateEncoderConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsync.CreateEncoderConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createEncoderConfigurationAsync(
CreateEncoderConfigurationRequest createEncoderConfigurationRequest);
/**
*
* Creates an EncoderConfiguration object.
*
*
* @param createEncoderConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEncoderConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.CreateEncoderConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createEncoderConfigurationAsync(
CreateEncoderConfigurationRequest createEncoderConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an additional token for a specified stage. This can be done after stage creation or when tokens expire.
* Tokens always are scoped to the stage for which they are created.
*
*
* Encryption keys are owned by Amazon IVS and never used directly by your application.
*
*
* @param createParticipantTokenRequest
* @return A Java Future containing the result of the CreateParticipantToken operation returned by the service.
* @sample AmazonIVSRealTimeAsync.CreateParticipantToken
* @see AWS API Documentation
*/
java.util.concurrent.Future createParticipantTokenAsync(CreateParticipantTokenRequest createParticipantTokenRequest);
/**
*
* Creates an additional token for a specified stage. This can be done after stage creation or when tokens expire.
* Tokens always are scoped to the stage for which they are created.
*
*
* Encryption keys are owned by Amazon IVS and never used directly by your application.
*
*
* @param createParticipantTokenRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateParticipantToken operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.CreateParticipantToken
* @see AWS API Documentation
*/
java.util.concurrent.Future createParticipantTokenAsync(CreateParticipantTokenRequest createParticipantTokenRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new stage (and optionally participant tokens).
*
*
* @param createStageRequest
* @return A Java Future containing the result of the CreateStage operation returned by the service.
* @sample AmazonIVSRealTimeAsync.CreateStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStageAsync(CreateStageRequest createStageRequest);
/**
*
* Creates a new stage (and optionally participant tokens).
*
*
* @param createStageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStage operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.CreateStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStageAsync(CreateStageRequest createStageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new storage configuration, used to enable recording to Amazon S3. When a StorageConfiguration is
* created, IVS will modify the S3 bucketPolicy of the provided bucket. This will ensure that IVS has sufficient
* permissions to write content to the provided bucket.
*
*
* @param createStorageConfigurationRequest
* @return A Java Future containing the result of the CreateStorageConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsync.CreateStorageConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createStorageConfigurationAsync(
CreateStorageConfigurationRequest createStorageConfigurationRequest);
/**
*
* Creates a new storage configuration, used to enable recording to Amazon S3. When a StorageConfiguration is
* created, IVS will modify the S3 bucketPolicy of the provided bucket. This will ensure that IVS has sufficient
* permissions to write content to the provided bucket.
*
*
* @param createStorageConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStorageConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.CreateStorageConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createStorageConfigurationAsync(
CreateStorageConfigurationRequest createStorageConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an EncoderConfiguration resource. Ensures that no Compositions are using this template; otherwise,
* returns an error.
*
*
* @param deleteEncoderConfigurationRequest
* @return A Java Future containing the result of the DeleteEncoderConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsync.DeleteEncoderConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEncoderConfigurationAsync(
DeleteEncoderConfigurationRequest deleteEncoderConfigurationRequest);
/**
*
* Deletes an EncoderConfiguration resource. Ensures that no Compositions are using this template; otherwise,
* returns an error.
*
*
* @param deleteEncoderConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEncoderConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.DeleteEncoderConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEncoderConfigurationAsync(
DeleteEncoderConfigurationRequest deleteEncoderConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Shuts down and deletes the specified stage (disconnecting all participants).
*
*
* @param deleteStageRequest
* @return A Java Future containing the result of the DeleteStage operation returned by the service.
* @sample AmazonIVSRealTimeAsync.DeleteStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStageAsync(DeleteStageRequest deleteStageRequest);
/**
*
* Shuts down and deletes the specified stage (disconnecting all participants).
*
*
* @param deleteStageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStage operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.DeleteStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStageAsync(DeleteStageRequest deleteStageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the storage configuration for the specified ARN.
*
*
* If you try to delete a storage configuration that is used by a Composition, you will get an error (409
* ConflictException). To avoid this, for all Compositions that reference the storage configuration, first use
* StopComposition and wait for it to complete, then use DeleteStorageConfiguration.
*
*
* @param deleteStorageConfigurationRequest
* @return A Java Future containing the result of the DeleteStorageConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsync.DeleteStorageConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStorageConfigurationAsync(
DeleteStorageConfigurationRequest deleteStorageConfigurationRequest);
/**
*
* Deletes the storage configuration for the specified ARN.
*
*
* If you try to delete a storage configuration that is used by a Composition, you will get an error (409
* ConflictException). To avoid this, for all Compositions that reference the storage configuration, first use
* StopComposition and wait for it to complete, then use DeleteStorageConfiguration.
*
*
* @param deleteStorageConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStorageConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.DeleteStorageConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStorageConfigurationAsync(
DeleteStorageConfigurationRequest deleteStorageConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disconnects a specified participant and revokes the participant permanently from a specified stage.
*
*
* @param disconnectParticipantRequest
* @return A Java Future containing the result of the DisconnectParticipant operation returned by the service.
* @sample AmazonIVSRealTimeAsync.DisconnectParticipant
* @see AWS API Documentation
*/
java.util.concurrent.Future disconnectParticipantAsync(DisconnectParticipantRequest disconnectParticipantRequest);
/**
*
* Disconnects a specified participant and revokes the participant permanently from a specified stage.
*
*
* @param disconnectParticipantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisconnectParticipant operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.DisconnectParticipant
* @see AWS API Documentation
*/
java.util.concurrent.Future disconnectParticipantAsync(DisconnectParticipantRequest disconnectParticipantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get information about the specified Composition resource.
*
*
* @param getCompositionRequest
* @return A Java Future containing the result of the GetComposition operation returned by the service.
* @sample AmazonIVSRealTimeAsync.GetComposition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getCompositionAsync(GetCompositionRequest getCompositionRequest);
/**
*
* Get information about the specified Composition resource.
*
*
* @param getCompositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetComposition operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.GetComposition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getCompositionAsync(GetCompositionRequest getCompositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified EncoderConfiguration resource.
*
*
* @param getEncoderConfigurationRequest
* @return A Java Future containing the result of the GetEncoderConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsync.GetEncoderConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getEncoderConfigurationAsync(GetEncoderConfigurationRequest getEncoderConfigurationRequest);
/**
*
* Gets information about the specified EncoderConfiguration resource.
*
*
* @param getEncoderConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEncoderConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.GetEncoderConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getEncoderConfigurationAsync(GetEncoderConfigurationRequest getEncoderConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the specified participant token.
*
*
* @param getParticipantRequest
* @return A Java Future containing the result of the GetParticipant operation returned by the service.
* @sample AmazonIVSRealTimeAsync.GetParticipant
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getParticipantAsync(GetParticipantRequest getParticipantRequest);
/**
*
* Gets information about the specified participant token.
*
*
* @param getParticipantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetParticipant operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.GetParticipant
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getParticipantAsync(GetParticipantRequest getParticipantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information for the specified stage.
*
*
* @param getStageRequest
* @return A Java Future containing the result of the GetStage operation returned by the service.
* @sample AmazonIVSRealTimeAsync.GetStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getStageAsync(GetStageRequest getStageRequest);
/**
*
* Gets information for the specified stage.
*
*
* @param getStageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStage operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.GetStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getStageAsync(GetStageRequest getStageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information for the specified stage session.
*
*
* @param getStageSessionRequest
* @return A Java Future containing the result of the GetStageSession operation returned by the service.
* @sample AmazonIVSRealTimeAsync.GetStageSession
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getStageSessionAsync(GetStageSessionRequest getStageSessionRequest);
/**
*
* Gets information for the specified stage session.
*
*
* @param getStageSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStageSession operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.GetStageSession
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getStageSessionAsync(GetStageSessionRequest getStageSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the storage configuration for the specified ARN.
*
*
* @param getStorageConfigurationRequest
* @return A Java Future containing the result of the GetStorageConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsync.GetStorageConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getStorageConfigurationAsync(GetStorageConfigurationRequest getStorageConfigurationRequest);
/**
*
* Gets the storage configuration for the specified ARN.
*
*
* @param getStorageConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStorageConfiguration operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.GetStorageConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getStorageConfigurationAsync(GetStorageConfigurationRequest getStorageConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets summary information about all Compositions in your account, in the AWS region where the API request is
* processed.
*
*
* @param listCompositionsRequest
* @return A Java Future containing the result of the ListCompositions operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListCompositions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCompositionsAsync(ListCompositionsRequest listCompositionsRequest);
/**
*
* Gets summary information about all Compositions in your account, in the AWS region where the API request is
* processed.
*
*
* @param listCompositionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCompositions operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListCompositions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCompositionsAsync(ListCompositionsRequest listCompositionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets summary information about all EncoderConfigurations in your account, in the AWS region where the API request
* is processed.
*
*
* @param listEncoderConfigurationsRequest
* @return A Java Future containing the result of the ListEncoderConfigurations operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListEncoderConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listEncoderConfigurationsAsync(
ListEncoderConfigurationsRequest listEncoderConfigurationsRequest);
/**
*
* Gets summary information about all EncoderConfigurations in your account, in the AWS region where the API request
* is processed.
*
*
* @param listEncoderConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEncoderConfigurations operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListEncoderConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listEncoderConfigurationsAsync(
ListEncoderConfigurationsRequest listEncoderConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists events for a specified participant that occurred during a specified stage session.
*
*
* @param listParticipantEventsRequest
* @return A Java Future containing the result of the ListParticipantEvents operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListParticipantEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future listParticipantEventsAsync(ListParticipantEventsRequest listParticipantEventsRequest);
/**
*
* Lists events for a specified participant that occurred during a specified stage session.
*
*
* @param listParticipantEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListParticipantEvents operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListParticipantEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future listParticipantEventsAsync(ListParticipantEventsRequest listParticipantEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all participants in a specified stage session.
*
*
* @param listParticipantsRequest
* @return A Java Future containing the result of the ListParticipants operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListParticipants
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listParticipantsAsync(ListParticipantsRequest listParticipantsRequest);
/**
*
* Lists all participants in a specified stage session.
*
*
* @param listParticipantsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListParticipants operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListParticipants
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listParticipantsAsync(ListParticipantsRequest listParticipantsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets all sessions for a specified stage.
*
*
* @param listStageSessionsRequest
* @return A Java Future containing the result of the ListStageSessions operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListStageSessions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStageSessionsAsync(ListStageSessionsRequest listStageSessionsRequest);
/**
*
* Gets all sessions for a specified stage.
*
*
* @param listStageSessionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStageSessions operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListStageSessions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStageSessionsAsync(ListStageSessionsRequest listStageSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets summary information about all stages in your account, in the AWS region where the API request is processed.
*
*
* @param listStagesRequest
* @return A Java Future containing the result of the ListStages operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListStages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStagesAsync(ListStagesRequest listStagesRequest);
/**
*
* Gets summary information about all stages in your account, in the AWS region where the API request is processed.
*
*
* @param listStagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStages operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListStages
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStagesAsync(ListStagesRequest listStagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets summary information about all storage configurations in your account, in the AWS region where the API
* request is processed.
*
*
* @param listStorageConfigurationsRequest
* @return A Java Future containing the result of the ListStorageConfigurations operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListStorageConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listStorageConfigurationsAsync(
ListStorageConfigurationsRequest listStorageConfigurationsRequest);
/**
*
* Gets summary information about all storage configurations in your account, in the AWS region where the API
* request is processed.
*
*
* @param listStorageConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStorageConfigurations operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListStorageConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listStorageConfigurationsAsync(
ListStorageConfigurationsRequest listStorageConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about AWS tags for the specified ARN.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonIVSRealTimeAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Gets information about AWS tags for the specified ARN.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a Composition from a stage based on the configuration provided in the request.
*
*
* A Composition is an ephemeral resource that exists after this endpoint returns successfully. Composition stops
* and the resource is deleted:
*
*
* -
*
* When StopComposition is called.
*
*
* -
*
* After a 1-minute timeout, when all participants are disconnected from the stage.
*
*
* -
*
* After a 1-minute timeout, if there are no participants in the stage when StartComposition is called.
*
*
* -
*
* When broadcasting to the IVS channel fails and all retries are exhausted.
*
*
* -
*
* When broadcasting is disconnected and all attempts to reconnect are exhausted.
*
*
*
*
* @param startCompositionRequest
* @return A Java Future containing the result of the StartComposition operation returned by the service.
* @sample AmazonIVSRealTimeAsync.StartComposition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startCompositionAsync(StartCompositionRequest startCompositionRequest);
/**
*
* Starts a Composition from a stage based on the configuration provided in the request.
*
*
* A Composition is an ephemeral resource that exists after this endpoint returns successfully. Composition stops
* and the resource is deleted:
*
*
* -
*
* When StopComposition is called.
*
*
* -
*
* After a 1-minute timeout, when all participants are disconnected from the stage.
*
*
* -
*
* After a 1-minute timeout, if there are no participants in the stage when StartComposition is called.
*
*
* -
*
* When broadcasting to the IVS channel fails and all retries are exhausted.
*
*
* -
*
* When broadcasting is disconnected and all attempts to reconnect are exhausted.
*
*
*
*
* @param startCompositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartComposition operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.StartComposition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startCompositionAsync(StartCompositionRequest startCompositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops and deletes a Composition resource. Any broadcast from the Composition resource is stopped.
*
*
* @param stopCompositionRequest
* @return A Java Future containing the result of the StopComposition operation returned by the service.
* @sample AmazonIVSRealTimeAsync.StopComposition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopCompositionAsync(StopCompositionRequest stopCompositionRequest);
/**
*
* Stops and deletes a Composition resource. Any broadcast from the Composition resource is stopped.
*
*
* @param stopCompositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopComposition operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.StopComposition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopCompositionAsync(StopCompositionRequest stopCompositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or updates tags for the AWS resource with the specified ARN.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonIVSRealTimeAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or updates tags for the AWS resource with the specified ARN.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from the resource with the specified ARN.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonIVSRealTimeAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from the resource with the specified ARN.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a stage’s configuration.
*
*
* @param updateStageRequest
* @return A Java Future containing the result of the UpdateStage operation returned by the service.
* @sample AmazonIVSRealTimeAsync.UpdateStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStageAsync(UpdateStageRequest updateStageRequest);
/**
*
* Updates a stage’s configuration.
*
*
* @param updateStageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStage operation returned by the service.
* @sample AmazonIVSRealTimeAsyncHandler.UpdateStage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStageAsync(UpdateStageRequest updateStageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}