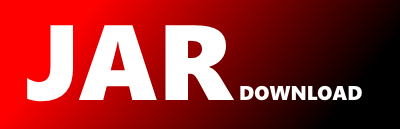
com.amazonaws.services.ivsrealtime.model.ParticipantSummary Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ivsrealtime.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Summary object describing a participant that has joined a stage.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ParticipantSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* ISO 8601 timestamp (returned as a string) when the participant first joined the stage session.
*
*/
private java.util.Date firstJoinTime;
/**
*
* Unique identifier for this participant, assigned by IVS.
*
*/
private String participantId;
/**
*
* Whether the participant ever published to the stage session.
*
*/
private Boolean published;
/**
*
* Whether the participant is connected to or disconnected from the stage.
*
*/
private String state;
/**
*
* Customer-assigned name to help identify the token; this can be used to link a participant to a user in the
* customer’s own systems. This can be any UTF-8 encoded text. This field is exposed to all stage participants
* and should not be used for personally identifying, confidential, or sensitive information.
*
*/
private String userId;
/**
*
* ISO 8601 timestamp (returned as a string) when the participant first joined the stage session.
*
*
* @param firstJoinTime
* ISO 8601 timestamp (returned as a string) when the participant first joined the stage session.
*/
public void setFirstJoinTime(java.util.Date firstJoinTime) {
this.firstJoinTime = firstJoinTime;
}
/**
*
* ISO 8601 timestamp (returned as a string) when the participant first joined the stage session.
*
*
* @return ISO 8601 timestamp (returned as a string) when the participant first joined the stage session.
*/
public java.util.Date getFirstJoinTime() {
return this.firstJoinTime;
}
/**
*
* ISO 8601 timestamp (returned as a string) when the participant first joined the stage session.
*
*
* @param firstJoinTime
* ISO 8601 timestamp (returned as a string) when the participant first joined the stage session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParticipantSummary withFirstJoinTime(java.util.Date firstJoinTime) {
setFirstJoinTime(firstJoinTime);
return this;
}
/**
*
* Unique identifier for this participant, assigned by IVS.
*
*
* @param participantId
* Unique identifier for this participant, assigned by IVS.
*/
public void setParticipantId(String participantId) {
this.participantId = participantId;
}
/**
*
* Unique identifier for this participant, assigned by IVS.
*
*
* @return Unique identifier for this participant, assigned by IVS.
*/
public String getParticipantId() {
return this.participantId;
}
/**
*
* Unique identifier for this participant, assigned by IVS.
*
*
* @param participantId
* Unique identifier for this participant, assigned by IVS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParticipantSummary withParticipantId(String participantId) {
setParticipantId(participantId);
return this;
}
/**
*
* Whether the participant ever published to the stage session.
*
*
* @param published
* Whether the participant ever published to the stage session.
*/
public void setPublished(Boolean published) {
this.published = published;
}
/**
*
* Whether the participant ever published to the stage session.
*
*
* @return Whether the participant ever published to the stage session.
*/
public Boolean getPublished() {
return this.published;
}
/**
*
* Whether the participant ever published to the stage session.
*
*
* @param published
* Whether the participant ever published to the stage session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParticipantSummary withPublished(Boolean published) {
setPublished(published);
return this;
}
/**
*
* Whether the participant ever published to the stage session.
*
*
* @return Whether the participant ever published to the stage session.
*/
public Boolean isPublished() {
return this.published;
}
/**
*
* Whether the participant is connected to or disconnected from the stage.
*
*
* @param state
* Whether the participant is connected to or disconnected from the stage.
* @see ParticipantState
*/
public void setState(String state) {
this.state = state;
}
/**
*
* Whether the participant is connected to or disconnected from the stage.
*
*
* @return Whether the participant is connected to or disconnected from the stage.
* @see ParticipantState
*/
public String getState() {
return this.state;
}
/**
*
* Whether the participant is connected to or disconnected from the stage.
*
*
* @param state
* Whether the participant is connected to or disconnected from the stage.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParticipantState
*/
public ParticipantSummary withState(String state) {
setState(state);
return this;
}
/**
*
* Whether the participant is connected to or disconnected from the stage.
*
*
* @param state
* Whether the participant is connected to or disconnected from the stage.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ParticipantState
*/
public ParticipantSummary withState(ParticipantState state) {
this.state = state.toString();
return this;
}
/**
*
* Customer-assigned name to help identify the token; this can be used to link a participant to a user in the
* customer’s own systems. This can be any UTF-8 encoded text. This field is exposed to all stage participants
* and should not be used for personally identifying, confidential, or sensitive information.
*
*
* @param userId
* Customer-assigned name to help identify the token; this can be used to link a participant to a user in the
* customer’s own systems. This can be any UTF-8 encoded text. This field is exposed to all stage
* participants and should not be used for personally identifying, confidential, or sensitive
* information.
*/
public void setUserId(String userId) {
this.userId = userId;
}
/**
*
* Customer-assigned name to help identify the token; this can be used to link a participant to a user in the
* customer’s own systems. This can be any UTF-8 encoded text. This field is exposed to all stage participants
* and should not be used for personally identifying, confidential, or sensitive information.
*
*
* @return Customer-assigned name to help identify the token; this can be used to link a participant to a user in
* the customer’s own systems. This can be any UTF-8 encoded text. This field is exposed to all stage
* participants and should not be used for personally identifying, confidential, or sensitive
* information.
*/
public String getUserId() {
return this.userId;
}
/**
*
* Customer-assigned name to help identify the token; this can be used to link a participant to a user in the
* customer’s own systems. This can be any UTF-8 encoded text. This field is exposed to all stage participants
* and should not be used for personally identifying, confidential, or sensitive information.
*
*
* @param userId
* Customer-assigned name to help identify the token; this can be used to link a participant to a user in the
* customer’s own systems. This can be any UTF-8 encoded text. This field is exposed to all stage
* participants and should not be used for personally identifying, confidential, or sensitive
* information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ParticipantSummary withUserId(String userId) {
setUserId(userId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFirstJoinTime() != null)
sb.append("FirstJoinTime: ").append(getFirstJoinTime()).append(",");
if (getParticipantId() != null)
sb.append("ParticipantId: ").append(getParticipantId()).append(",");
if (getPublished() != null)
sb.append("Published: ").append(getPublished()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getUserId() != null)
sb.append("UserId: ").append(getUserId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ParticipantSummary == false)
return false;
ParticipantSummary other = (ParticipantSummary) obj;
if (other.getFirstJoinTime() == null ^ this.getFirstJoinTime() == null)
return false;
if (other.getFirstJoinTime() != null && other.getFirstJoinTime().equals(this.getFirstJoinTime()) == false)
return false;
if (other.getParticipantId() == null ^ this.getParticipantId() == null)
return false;
if (other.getParticipantId() != null && other.getParticipantId().equals(this.getParticipantId()) == false)
return false;
if (other.getPublished() == null ^ this.getPublished() == null)
return false;
if (other.getPublished() != null && other.getPublished().equals(this.getPublished()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getUserId() == null ^ this.getUserId() == null)
return false;
if (other.getUserId() != null && other.getUserId().equals(this.getUserId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFirstJoinTime() == null) ? 0 : getFirstJoinTime().hashCode());
hashCode = prime * hashCode + ((getParticipantId() == null) ? 0 : getParticipantId().hashCode());
hashCode = prime * hashCode + ((getPublished() == null) ? 0 : getPublished().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getUserId() == null) ? 0 : getUserId().hashCode());
return hashCode;
}
@Override
public ParticipantSummary clone() {
try {
return (ParticipantSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ivsrealtime.model.transform.ParticipantSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}