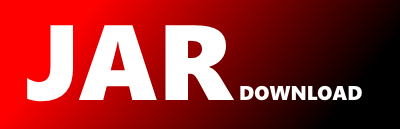
com.amazonaws.services.ivsrealtime.model.Video Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ivsrealtime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ivsrealtime.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Settings for video.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Video implements Serializable, Cloneable, StructuredPojo {
/**
*
* Bitrate for generated output, in bps. Default: 2500000.
*
*/
private Integer bitrate;
/**
*
* Video frame rate, in fps. Default: 30.
*
*/
private Float framerate;
/**
*
* Video-resolution height. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 720.
*
*/
private Integer height;
/**
*
* Video-resolution width. Note that the maximum value is determined by width
times height
* , such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 1280.
*
*/
private Integer width;
/**
*
* Bitrate for generated output, in bps. Default: 2500000.
*
*
* @param bitrate
* Bitrate for generated output, in bps. Default: 2500000.
*/
public void setBitrate(Integer bitrate) {
this.bitrate = bitrate;
}
/**
*
* Bitrate for generated output, in bps. Default: 2500000.
*
*
* @return Bitrate for generated output, in bps. Default: 2500000.
*/
public Integer getBitrate() {
return this.bitrate;
}
/**
*
* Bitrate for generated output, in bps. Default: 2500000.
*
*
* @param bitrate
* Bitrate for generated output, in bps. Default: 2500000.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Video withBitrate(Integer bitrate) {
setBitrate(bitrate);
return this;
}
/**
*
* Video frame rate, in fps. Default: 30.
*
*
* @param framerate
* Video frame rate, in fps. Default: 30.
*/
public void setFramerate(Float framerate) {
this.framerate = framerate;
}
/**
*
* Video frame rate, in fps. Default: 30.
*
*
* @return Video frame rate, in fps. Default: 30.
*/
public Float getFramerate() {
return this.framerate;
}
/**
*
* Video frame rate, in fps. Default: 30.
*
*
* @param framerate
* Video frame rate, in fps. Default: 30.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Video withFramerate(Float framerate) {
setFramerate(framerate);
return this;
}
/**
*
* Video-resolution height. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 720.
*
*
* @param height
* Video-resolution height. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 720.
*/
public void setHeight(Integer height) {
this.height = height;
}
/**
*
* Video-resolution height. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 720.
*
*
* @return Video-resolution height. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default:
* 720.
*/
public Integer getHeight() {
return this.height;
}
/**
*
* Video-resolution height. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 720.
*
*
* @param height
* Video-resolution height. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 720.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Video withHeight(Integer height) {
setHeight(height);
return this;
}
/**
*
* Video-resolution width. Note that the maximum value is determined by width
times height
* , such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 1280.
*
*
* @param width
* Video-resolution width. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default:
* 1280.
*/
public void setWidth(Integer width) {
this.width = width;
}
/**
*
* Video-resolution width. Note that the maximum value is determined by width
times height
* , such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 1280.
*
*
* @return Video-resolution width. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default:
* 1280.
*/
public Integer getWidth() {
return this.width;
}
/**
*
* Video-resolution width. Note that the maximum value is determined by width
times height
* , such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default: 1280.
*
*
* @param width
* Video-resolution width. Note that the maximum value is determined by width
times
* height
, such that the maximum total pixels is 2073600 (1920x1080 or 1080x1920). Default:
* 1280.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Video withWidth(Integer width) {
setWidth(width);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBitrate() != null)
sb.append("Bitrate: ").append(getBitrate()).append(",");
if (getFramerate() != null)
sb.append("Framerate: ").append(getFramerate()).append(",");
if (getHeight() != null)
sb.append("Height: ").append(getHeight()).append(",");
if (getWidth() != null)
sb.append("Width: ").append(getWidth());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Video == false)
return false;
Video other = (Video) obj;
if (other.getBitrate() == null ^ this.getBitrate() == null)
return false;
if (other.getBitrate() != null && other.getBitrate().equals(this.getBitrate()) == false)
return false;
if (other.getFramerate() == null ^ this.getFramerate() == null)
return false;
if (other.getFramerate() != null && other.getFramerate().equals(this.getFramerate()) == false)
return false;
if (other.getHeight() == null ^ this.getHeight() == null)
return false;
if (other.getHeight() != null && other.getHeight().equals(this.getHeight()) == false)
return false;
if (other.getWidth() == null ^ this.getWidth() == null)
return false;
if (other.getWidth() != null && other.getWidth().equals(this.getWidth()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBitrate() == null) ? 0 : getBitrate().hashCode());
hashCode = prime * hashCode + ((getFramerate() == null) ? 0 : getFramerate().hashCode());
hashCode = prime * hashCode + ((getHeight() == null) ? 0 : getHeight().hashCode());
hashCode = prime * hashCode + ((getWidth() == null) ? 0 : getWidth().hashCode());
return hashCode;
}
@Override
public Video clone() {
try {
return (Video) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ivsrealtime.model.transform.VideoMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}