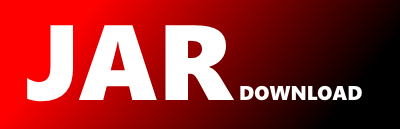
com.amazonaws.services.kafkaconnect.model.CreateConnectorRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kafkaconnect Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kafkaconnect.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateConnectorRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Information about the capacity allocated to the connector. Exactly one of the two properties must be specified.
*
*/
private Capacity capacity;
/**
*
* A map of keys to values that represent the configuration for the connector.
*
*/
private java.util.Map connectorConfiguration;
/**
*
* A summary description of the connector.
*
*/
private String connectorDescription;
/**
*
* The name of the connector.
*
*/
private String connectorName;
/**
*
* Specifies which Apache Kafka cluster to connect to.
*
*/
private KafkaCluster kafkaCluster;
/**
*
* Details of the client authentication used by the Apache Kafka cluster.
*
*/
private KafkaClusterClientAuthentication kafkaClusterClientAuthentication;
/**
*
* Details of encryption in transit to the Apache Kafka cluster.
*
*/
private KafkaClusterEncryptionInTransit kafkaClusterEncryptionInTransit;
/**
*
* The version of Kafka Connect. It has to be compatible with both the Apache Kafka cluster's version and the
* plugins.
*
*/
private String kafkaConnectVersion;
/**
*
* Details about log delivery.
*
*/
private LogDelivery logDelivery;
/**
*
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one plugin
* for your connector, you can create a single custom plugin using a ZIP file that bundles multiple plugins
* together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
*
*/
private java.util.List plugins;
/**
*
* The Amazon Resource Name (ARN) of the IAM role used by the connector to access the Amazon Web Services resources
* that it needs. The types of resources depends on the logic of the connector. For example, a connector that has
* Amazon S3 as a destination must have permissions that allow it to write to the S3 destination bucket.
*
*/
private String serviceExecutionRoleArn;
/**
*
* The tags you want to attach to the connector.
*
*/
private java.util.Map tags;
/**
*
* Specifies which worker configuration to use with the connector.
*
*/
private WorkerConfiguration workerConfiguration;
/**
*
* Information about the capacity allocated to the connector. Exactly one of the two properties must be specified.
*
*
* @param capacity
* Information about the capacity allocated to the connector. Exactly one of the two properties must be
* specified.
*/
public void setCapacity(Capacity capacity) {
this.capacity = capacity;
}
/**
*
* Information about the capacity allocated to the connector. Exactly one of the two properties must be specified.
*
*
* @return Information about the capacity allocated to the connector. Exactly one of the two properties must be
* specified.
*/
public Capacity getCapacity() {
return this.capacity;
}
/**
*
* Information about the capacity allocated to the connector. Exactly one of the two properties must be specified.
*
*
* @param capacity
* Information about the capacity allocated to the connector. Exactly one of the two properties must be
* specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withCapacity(Capacity capacity) {
setCapacity(capacity);
return this;
}
/**
*
* A map of keys to values that represent the configuration for the connector.
*
*
* @return A map of keys to values that represent the configuration for the connector.
*/
public java.util.Map getConnectorConfiguration() {
return connectorConfiguration;
}
/**
*
* A map of keys to values that represent the configuration for the connector.
*
*
* @param connectorConfiguration
* A map of keys to values that represent the configuration for the connector.
*/
public void setConnectorConfiguration(java.util.Map connectorConfiguration) {
this.connectorConfiguration = connectorConfiguration;
}
/**
*
* A map of keys to values that represent the configuration for the connector.
*
*
* @param connectorConfiguration
* A map of keys to values that represent the configuration for the connector.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withConnectorConfiguration(java.util.Map connectorConfiguration) {
setConnectorConfiguration(connectorConfiguration);
return this;
}
/**
* Add a single ConnectorConfiguration entry
*
* @see CreateConnectorRequest#withConnectorConfiguration
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest addConnectorConfigurationEntry(String key, String value) {
if (null == this.connectorConfiguration) {
this.connectorConfiguration = new java.util.HashMap();
}
if (this.connectorConfiguration.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.connectorConfiguration.put(key, value);
return this;
}
/**
* Removes all the entries added into ConnectorConfiguration.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest clearConnectorConfigurationEntries() {
this.connectorConfiguration = null;
return this;
}
/**
*
* A summary description of the connector.
*
*
* @param connectorDescription
* A summary description of the connector.
*/
public void setConnectorDescription(String connectorDescription) {
this.connectorDescription = connectorDescription;
}
/**
*
* A summary description of the connector.
*
*
* @return A summary description of the connector.
*/
public String getConnectorDescription() {
return this.connectorDescription;
}
/**
*
* A summary description of the connector.
*
*
* @param connectorDescription
* A summary description of the connector.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withConnectorDescription(String connectorDescription) {
setConnectorDescription(connectorDescription);
return this;
}
/**
*
* The name of the connector.
*
*
* @param connectorName
* The name of the connector.
*/
public void setConnectorName(String connectorName) {
this.connectorName = connectorName;
}
/**
*
* The name of the connector.
*
*
* @return The name of the connector.
*/
public String getConnectorName() {
return this.connectorName;
}
/**
*
* The name of the connector.
*
*
* @param connectorName
* The name of the connector.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withConnectorName(String connectorName) {
setConnectorName(connectorName);
return this;
}
/**
*
* Specifies which Apache Kafka cluster to connect to.
*
*
* @param kafkaCluster
* Specifies which Apache Kafka cluster to connect to.
*/
public void setKafkaCluster(KafkaCluster kafkaCluster) {
this.kafkaCluster = kafkaCluster;
}
/**
*
* Specifies which Apache Kafka cluster to connect to.
*
*
* @return Specifies which Apache Kafka cluster to connect to.
*/
public KafkaCluster getKafkaCluster() {
return this.kafkaCluster;
}
/**
*
* Specifies which Apache Kafka cluster to connect to.
*
*
* @param kafkaCluster
* Specifies which Apache Kafka cluster to connect to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withKafkaCluster(KafkaCluster kafkaCluster) {
setKafkaCluster(kafkaCluster);
return this;
}
/**
*
* Details of the client authentication used by the Apache Kafka cluster.
*
*
* @param kafkaClusterClientAuthentication
* Details of the client authentication used by the Apache Kafka cluster.
*/
public void setKafkaClusterClientAuthentication(KafkaClusterClientAuthentication kafkaClusterClientAuthentication) {
this.kafkaClusterClientAuthentication = kafkaClusterClientAuthentication;
}
/**
*
* Details of the client authentication used by the Apache Kafka cluster.
*
*
* @return Details of the client authentication used by the Apache Kafka cluster.
*/
public KafkaClusterClientAuthentication getKafkaClusterClientAuthentication() {
return this.kafkaClusterClientAuthentication;
}
/**
*
* Details of the client authentication used by the Apache Kafka cluster.
*
*
* @param kafkaClusterClientAuthentication
* Details of the client authentication used by the Apache Kafka cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withKafkaClusterClientAuthentication(KafkaClusterClientAuthentication kafkaClusterClientAuthentication) {
setKafkaClusterClientAuthentication(kafkaClusterClientAuthentication);
return this;
}
/**
*
* Details of encryption in transit to the Apache Kafka cluster.
*
*
* @param kafkaClusterEncryptionInTransit
* Details of encryption in transit to the Apache Kafka cluster.
*/
public void setKafkaClusterEncryptionInTransit(KafkaClusterEncryptionInTransit kafkaClusterEncryptionInTransit) {
this.kafkaClusterEncryptionInTransit = kafkaClusterEncryptionInTransit;
}
/**
*
* Details of encryption in transit to the Apache Kafka cluster.
*
*
* @return Details of encryption in transit to the Apache Kafka cluster.
*/
public KafkaClusterEncryptionInTransit getKafkaClusterEncryptionInTransit() {
return this.kafkaClusterEncryptionInTransit;
}
/**
*
* Details of encryption in transit to the Apache Kafka cluster.
*
*
* @param kafkaClusterEncryptionInTransit
* Details of encryption in transit to the Apache Kafka cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withKafkaClusterEncryptionInTransit(KafkaClusterEncryptionInTransit kafkaClusterEncryptionInTransit) {
setKafkaClusterEncryptionInTransit(kafkaClusterEncryptionInTransit);
return this;
}
/**
*
* The version of Kafka Connect. It has to be compatible with both the Apache Kafka cluster's version and the
* plugins.
*
*
* @param kafkaConnectVersion
* The version of Kafka Connect. It has to be compatible with both the Apache Kafka cluster's version and the
* plugins.
*/
public void setKafkaConnectVersion(String kafkaConnectVersion) {
this.kafkaConnectVersion = kafkaConnectVersion;
}
/**
*
* The version of Kafka Connect. It has to be compatible with both the Apache Kafka cluster's version and the
* plugins.
*
*
* @return The version of Kafka Connect. It has to be compatible with both the Apache Kafka cluster's version and
* the plugins.
*/
public String getKafkaConnectVersion() {
return this.kafkaConnectVersion;
}
/**
*
* The version of Kafka Connect. It has to be compatible with both the Apache Kafka cluster's version and the
* plugins.
*
*
* @param kafkaConnectVersion
* The version of Kafka Connect. It has to be compatible with both the Apache Kafka cluster's version and the
* plugins.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withKafkaConnectVersion(String kafkaConnectVersion) {
setKafkaConnectVersion(kafkaConnectVersion);
return this;
}
/**
*
* Details about log delivery.
*
*
* @param logDelivery
* Details about log delivery.
*/
public void setLogDelivery(LogDelivery logDelivery) {
this.logDelivery = logDelivery;
}
/**
*
* Details about log delivery.
*
*
* @return Details about log delivery.
*/
public LogDelivery getLogDelivery() {
return this.logDelivery;
}
/**
*
* Details about log delivery.
*
*
* @param logDelivery
* Details about log delivery.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withLogDelivery(LogDelivery logDelivery) {
setLogDelivery(logDelivery);
return this;
}
/**
*
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one plugin
* for your connector, you can create a single custom plugin using a ZIP file that bundles multiple plugins
* together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
*
*
* @return
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one
* plugin for your connector, you can create a single custom plugin using a ZIP file that bundles multiple
* plugins together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
*/
public java.util.List getPlugins() {
return plugins;
}
/**
*
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one plugin
* for your connector, you can create a single custom plugin using a ZIP file that bundles multiple plugins
* together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
*
*
* @param plugins
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one
* plugin for your connector, you can create a single custom plugin using a ZIP file that bundles multiple
* plugins together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
*/
public void setPlugins(java.util.Collection plugins) {
if (plugins == null) {
this.plugins = null;
return;
}
this.plugins = new java.util.ArrayList(plugins);
}
/**
*
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one plugin
* for your connector, you can create a single custom plugin using a ZIP file that bundles multiple plugins
* together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlugins(java.util.Collection)} or {@link #withPlugins(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param plugins
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one
* plugin for your connector, you can create a single custom plugin using a ZIP file that bundles multiple
* plugins together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withPlugins(Plugin... plugins) {
if (this.plugins == null) {
setPlugins(new java.util.ArrayList(plugins.length));
}
for (Plugin ele : plugins) {
this.plugins.add(ele);
}
return this;
}
/**
*
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one plugin
* for your connector, you can create a single custom plugin using a ZIP file that bundles multiple plugins
* together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
*
*
* @param plugins
*
* Amazon MSK Connect does not currently support specifying multiple plugins as a list. To use more than one
* plugin for your connector, you can create a single custom plugin using a ZIP file that bundles multiple
* plugins together.
*
*
*
* Specifies which plugin to use for the connector. You must specify a single-element list containing one
* customPlugin
object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withPlugins(java.util.Collection plugins) {
setPlugins(plugins);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used by the connector to access the Amazon Web Services resources
* that it needs. The types of resources depends on the logic of the connector. For example, a connector that has
* Amazon S3 as a destination must have permissions that allow it to write to the S3 destination bucket.
*
*
* @param serviceExecutionRoleArn
* The Amazon Resource Name (ARN) of the IAM role used by the connector to access the Amazon Web Services
* resources that it needs. The types of resources depends on the logic of the connector. For example, a
* connector that has Amazon S3 as a destination must have permissions that allow it to write to the S3
* destination bucket.
*/
public void setServiceExecutionRoleArn(String serviceExecutionRoleArn) {
this.serviceExecutionRoleArn = serviceExecutionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used by the connector to access the Amazon Web Services resources
* that it needs. The types of resources depends on the logic of the connector. For example, a connector that has
* Amazon S3 as a destination must have permissions that allow it to write to the S3 destination bucket.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role used by the connector to access the Amazon Web Services
* resources that it needs. The types of resources depends on the logic of the connector. For example, a
* connector that has Amazon S3 as a destination must have permissions that allow it to write to the S3
* destination bucket.
*/
public String getServiceExecutionRoleArn() {
return this.serviceExecutionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used by the connector to access the Amazon Web Services resources
* that it needs. The types of resources depends on the logic of the connector. For example, a connector that has
* Amazon S3 as a destination must have permissions that allow it to write to the S3 destination bucket.
*
*
* @param serviceExecutionRoleArn
* The Amazon Resource Name (ARN) of the IAM role used by the connector to access the Amazon Web Services
* resources that it needs. The types of resources depends on the logic of the connector. For example, a
* connector that has Amazon S3 as a destination must have permissions that allow it to write to the S3
* destination bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withServiceExecutionRoleArn(String serviceExecutionRoleArn) {
setServiceExecutionRoleArn(serviceExecutionRoleArn);
return this;
}
/**
*
* The tags you want to attach to the connector.
*
*
* @return The tags you want to attach to the connector.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags you want to attach to the connector.
*
*
* @param tags
* The tags you want to attach to the connector.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags you want to attach to the connector.
*
*
* @param tags
* The tags you want to attach to the connector.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateConnectorRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* Specifies which worker configuration to use with the connector.
*
*
* @param workerConfiguration
* Specifies which worker configuration to use with the connector.
*/
public void setWorkerConfiguration(WorkerConfiguration workerConfiguration) {
this.workerConfiguration = workerConfiguration;
}
/**
*
* Specifies which worker configuration to use with the connector.
*
*
* @return Specifies which worker configuration to use with the connector.
*/
public WorkerConfiguration getWorkerConfiguration() {
return this.workerConfiguration;
}
/**
*
* Specifies which worker configuration to use with the connector.
*
*
* @param workerConfiguration
* Specifies which worker configuration to use with the connector.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateConnectorRequest withWorkerConfiguration(WorkerConfiguration workerConfiguration) {
setWorkerConfiguration(workerConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCapacity() != null)
sb.append("Capacity: ").append(getCapacity()).append(",");
if (getConnectorConfiguration() != null)
sb.append("ConnectorConfiguration: ").append("***Sensitive Data Redacted***").append(",");
if (getConnectorDescription() != null)
sb.append("ConnectorDescription: ").append(getConnectorDescription()).append(",");
if (getConnectorName() != null)
sb.append("ConnectorName: ").append(getConnectorName()).append(",");
if (getKafkaCluster() != null)
sb.append("KafkaCluster: ").append(getKafkaCluster()).append(",");
if (getKafkaClusterClientAuthentication() != null)
sb.append("KafkaClusterClientAuthentication: ").append(getKafkaClusterClientAuthentication()).append(",");
if (getKafkaClusterEncryptionInTransit() != null)
sb.append("KafkaClusterEncryptionInTransit: ").append(getKafkaClusterEncryptionInTransit()).append(",");
if (getKafkaConnectVersion() != null)
sb.append("KafkaConnectVersion: ").append(getKafkaConnectVersion()).append(",");
if (getLogDelivery() != null)
sb.append("LogDelivery: ").append(getLogDelivery()).append(",");
if (getPlugins() != null)
sb.append("Plugins: ").append(getPlugins()).append(",");
if (getServiceExecutionRoleArn() != null)
sb.append("ServiceExecutionRoleArn: ").append(getServiceExecutionRoleArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getWorkerConfiguration() != null)
sb.append("WorkerConfiguration: ").append(getWorkerConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateConnectorRequest == false)
return false;
CreateConnectorRequest other = (CreateConnectorRequest) obj;
if (other.getCapacity() == null ^ this.getCapacity() == null)
return false;
if (other.getCapacity() != null && other.getCapacity().equals(this.getCapacity()) == false)
return false;
if (other.getConnectorConfiguration() == null ^ this.getConnectorConfiguration() == null)
return false;
if (other.getConnectorConfiguration() != null && other.getConnectorConfiguration().equals(this.getConnectorConfiguration()) == false)
return false;
if (other.getConnectorDescription() == null ^ this.getConnectorDescription() == null)
return false;
if (other.getConnectorDescription() != null && other.getConnectorDescription().equals(this.getConnectorDescription()) == false)
return false;
if (other.getConnectorName() == null ^ this.getConnectorName() == null)
return false;
if (other.getConnectorName() != null && other.getConnectorName().equals(this.getConnectorName()) == false)
return false;
if (other.getKafkaCluster() == null ^ this.getKafkaCluster() == null)
return false;
if (other.getKafkaCluster() != null && other.getKafkaCluster().equals(this.getKafkaCluster()) == false)
return false;
if (other.getKafkaClusterClientAuthentication() == null ^ this.getKafkaClusterClientAuthentication() == null)
return false;
if (other.getKafkaClusterClientAuthentication() != null
&& other.getKafkaClusterClientAuthentication().equals(this.getKafkaClusterClientAuthentication()) == false)
return false;
if (other.getKafkaClusterEncryptionInTransit() == null ^ this.getKafkaClusterEncryptionInTransit() == null)
return false;
if (other.getKafkaClusterEncryptionInTransit() != null
&& other.getKafkaClusterEncryptionInTransit().equals(this.getKafkaClusterEncryptionInTransit()) == false)
return false;
if (other.getKafkaConnectVersion() == null ^ this.getKafkaConnectVersion() == null)
return false;
if (other.getKafkaConnectVersion() != null && other.getKafkaConnectVersion().equals(this.getKafkaConnectVersion()) == false)
return false;
if (other.getLogDelivery() == null ^ this.getLogDelivery() == null)
return false;
if (other.getLogDelivery() != null && other.getLogDelivery().equals(this.getLogDelivery()) == false)
return false;
if (other.getPlugins() == null ^ this.getPlugins() == null)
return false;
if (other.getPlugins() != null && other.getPlugins().equals(this.getPlugins()) == false)
return false;
if (other.getServiceExecutionRoleArn() == null ^ this.getServiceExecutionRoleArn() == null)
return false;
if (other.getServiceExecutionRoleArn() != null && other.getServiceExecutionRoleArn().equals(this.getServiceExecutionRoleArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getWorkerConfiguration() == null ^ this.getWorkerConfiguration() == null)
return false;
if (other.getWorkerConfiguration() != null && other.getWorkerConfiguration().equals(this.getWorkerConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCapacity() == null) ? 0 : getCapacity().hashCode());
hashCode = prime * hashCode + ((getConnectorConfiguration() == null) ? 0 : getConnectorConfiguration().hashCode());
hashCode = prime * hashCode + ((getConnectorDescription() == null) ? 0 : getConnectorDescription().hashCode());
hashCode = prime * hashCode + ((getConnectorName() == null) ? 0 : getConnectorName().hashCode());
hashCode = prime * hashCode + ((getKafkaCluster() == null) ? 0 : getKafkaCluster().hashCode());
hashCode = prime * hashCode + ((getKafkaClusterClientAuthentication() == null) ? 0 : getKafkaClusterClientAuthentication().hashCode());
hashCode = prime * hashCode + ((getKafkaClusterEncryptionInTransit() == null) ? 0 : getKafkaClusterEncryptionInTransit().hashCode());
hashCode = prime * hashCode + ((getKafkaConnectVersion() == null) ? 0 : getKafkaConnectVersion().hashCode());
hashCode = prime * hashCode + ((getLogDelivery() == null) ? 0 : getLogDelivery().hashCode());
hashCode = prime * hashCode + ((getPlugins() == null) ? 0 : getPlugins().hashCode());
hashCode = prime * hashCode + ((getServiceExecutionRoleArn() == null) ? 0 : getServiceExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getWorkerConfiguration() == null) ? 0 : getWorkerConfiguration().hashCode());
return hashCode;
}
@Override
public CreateConnectorRequest clone() {
return (CreateConnectorRequest) super.clone();
}
}