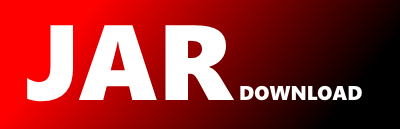
com.amazonaws.services.kafkaconnect.AWSKafkaConnectAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kafkaconnect Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kafkaconnect;
import javax.annotation.Generated;
import com.amazonaws.services.kafkaconnect.model.*;
/**
* Interface for accessing Kafka Connect asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.kafkaconnect.AbstractAWSKafkaConnectAsync} instead.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSKafkaConnectAsync extends AWSKafkaConnect {
/**
*
* Creates a connector using the specified properties.
*
*
* @param createConnectorRequest
* @return A Java Future containing the result of the CreateConnector operation returned by the service.
* @sample AWSKafkaConnectAsync.CreateConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createConnectorAsync(CreateConnectorRequest createConnectorRequest);
/**
*
* Creates a connector using the specified properties.
*
*
* @param createConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConnector operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.CreateConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createConnectorAsync(CreateConnectorRequest createConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom plugin using the specified properties.
*
*
* @param createCustomPluginRequest
* @return A Java Future containing the result of the CreateCustomPlugin operation returned by the service.
* @sample AWSKafkaConnectAsync.CreateCustomPlugin
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomPluginAsync(CreateCustomPluginRequest createCustomPluginRequest);
/**
*
* Creates a custom plugin using the specified properties.
*
*
* @param createCustomPluginRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomPlugin operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.CreateCustomPlugin
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomPluginAsync(CreateCustomPluginRequest createCustomPluginRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a worker configuration using the specified properties.
*
*
* @param createWorkerConfigurationRequest
* @return A Java Future containing the result of the CreateWorkerConfiguration operation returned by the service.
* @sample AWSKafkaConnectAsync.CreateWorkerConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createWorkerConfigurationAsync(
CreateWorkerConfigurationRequest createWorkerConfigurationRequest);
/**
*
* Creates a worker configuration using the specified properties.
*
*
* @param createWorkerConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorkerConfiguration operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.CreateWorkerConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createWorkerConfigurationAsync(
CreateWorkerConfigurationRequest createWorkerConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified connector.
*
*
* @param deleteConnectorRequest
* @return A Java Future containing the result of the DeleteConnector operation returned by the service.
* @sample AWSKafkaConnectAsync.DeleteConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteConnectorAsync(DeleteConnectorRequest deleteConnectorRequest);
/**
*
* Deletes the specified connector.
*
*
* @param deleteConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConnector operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.DeleteConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteConnectorAsync(DeleteConnectorRequest deleteConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom plugin.
*
*
* @param deleteCustomPluginRequest
* @return A Java Future containing the result of the DeleteCustomPlugin operation returned by the service.
* @sample AWSKafkaConnectAsync.DeleteCustomPlugin
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomPluginAsync(DeleteCustomPluginRequest deleteCustomPluginRequest);
/**
*
* Deletes a custom plugin.
*
*
* @param deleteCustomPluginRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomPlugin operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.DeleteCustomPlugin
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomPluginAsync(DeleteCustomPluginRequest deleteCustomPluginRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified worker configuration.
*
*
* @param deleteWorkerConfigurationRequest
* @return A Java Future containing the result of the DeleteWorkerConfiguration operation returned by the service.
* @sample AWSKafkaConnectAsync.DeleteWorkerConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteWorkerConfigurationAsync(
DeleteWorkerConfigurationRequest deleteWorkerConfigurationRequest);
/**
*
* Deletes the specified worker configuration.
*
*
* @param deleteWorkerConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorkerConfiguration operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.DeleteWorkerConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteWorkerConfigurationAsync(
DeleteWorkerConfigurationRequest deleteWorkerConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns summary information about the connector.
*
*
* @param describeConnectorRequest
* @return A Java Future containing the result of the DescribeConnector operation returned by the service.
* @sample AWSKafkaConnectAsync.DescribeConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeConnectorAsync(DescribeConnectorRequest describeConnectorRequest);
/**
*
* Returns summary information about the connector.
*
*
* @param describeConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConnector operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.DescribeConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeConnectorAsync(DescribeConnectorRequest describeConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A summary description of the custom plugin.
*
*
* @param describeCustomPluginRequest
* @return A Java Future containing the result of the DescribeCustomPlugin operation returned by the service.
* @sample AWSKafkaConnectAsync.DescribeCustomPlugin
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomPluginAsync(DescribeCustomPluginRequest describeCustomPluginRequest);
/**
*
* A summary description of the custom plugin.
*
*
* @param describeCustomPluginRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCustomPlugin operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.DescribeCustomPlugin
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomPluginAsync(DescribeCustomPluginRequest describeCustomPluginRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a worker configuration.
*
*
* @param describeWorkerConfigurationRequest
* @return A Java Future containing the result of the DescribeWorkerConfiguration operation returned by the service.
* @sample AWSKafkaConnectAsync.DescribeWorkerConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkerConfigurationAsync(
DescribeWorkerConfigurationRequest describeWorkerConfigurationRequest);
/**
*
* Returns information about a worker configuration.
*
*
* @param describeWorkerConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkerConfiguration operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.DescribeWorkerConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkerConfigurationAsync(
DescribeWorkerConfigurationRequest describeWorkerConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the connectors in this account and Region. The list is limited to connectors whose name
* starts with the specified prefix. The response also includes a description of each of the listed connectors.
*
*
* @param listConnectorsRequest
* @return A Java Future containing the result of the ListConnectors operation returned by the service.
* @sample AWSKafkaConnectAsync.ListConnectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listConnectorsAsync(ListConnectorsRequest listConnectorsRequest);
/**
*
* Returns a list of all the connectors in this account and Region. The list is limited to connectors whose name
* starts with the specified prefix. The response also includes a description of each of the listed connectors.
*
*
* @param listConnectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConnectors operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.ListConnectors
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listConnectorsAsync(ListConnectorsRequest listConnectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all of the custom plugins in this account and Region.
*
*
* @param listCustomPluginsRequest
* @return A Java Future containing the result of the ListCustomPlugins operation returned by the service.
* @sample AWSKafkaConnectAsync.ListCustomPlugins
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCustomPluginsAsync(ListCustomPluginsRequest listCustomPluginsRequest);
/**
*
* Returns a list of all of the custom plugins in this account and Region.
*
*
* @param listCustomPluginsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCustomPlugins operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.ListCustomPlugins
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listCustomPluginsAsync(ListCustomPluginsRequest listCustomPluginsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the tags attached to the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSKafkaConnectAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all the tags attached to the specified resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all of the worker configurations in this account and Region.
*
*
* @param listWorkerConfigurationsRequest
* @return A Java Future containing the result of the ListWorkerConfigurations operation returned by the service.
* @sample AWSKafkaConnectAsync.ListWorkerConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkerConfigurationsAsync(ListWorkerConfigurationsRequest listWorkerConfigurationsRequest);
/**
*
* Returns a list of all of the worker configurations in this account and Region.
*
*
* @param listWorkerConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorkerConfigurations operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.ListWorkerConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkerConfigurationsAsync(ListWorkerConfigurationsRequest listWorkerConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSKafkaConnectAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Attaches tags to the specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSKafkaConnectAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified connector.
*
*
* @param updateConnectorRequest
* @return A Java Future containing the result of the UpdateConnector operation returned by the service.
* @sample AWSKafkaConnectAsync.UpdateConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateConnectorAsync(UpdateConnectorRequest updateConnectorRequest);
/**
*
* Updates the specified connector.
*
*
* @param updateConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateConnector operation returned by the service.
* @sample AWSKafkaConnectAsyncHandler.UpdateConnector
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateConnectorAsync(UpdateConnectorRequest updateConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}