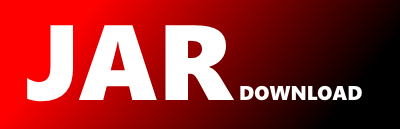
com.amazonaws.services.kendra.AWSkendraClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.kendra.AWSkendraClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.kendra.model.*;
import com.amazonaws.services.kendra.model.transform.*;
/**
* Client for accessing kendra. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
*
* Amazon Kendra is a service for indexing large document sets.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSkendraClient extends AmazonWebServiceClient implements AWSkendra {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSkendra.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "kendra";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceInUseException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ResourceInUseExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ResourceUnavailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceAlreadyExistException").withExceptionUnmarshaller(
com.amazonaws.services.kendra.model.transform.ResourceAlreadyExistExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.kendra.model.AWSkendraException.class));
public static AWSkendraClientBuilder builder() {
return AWSkendraClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on kendra using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSkendraClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on kendra using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSkendraClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("kendra.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/kendra/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/kendra/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Removes one or more documents from an index. The documents must have been added with the BatchPutDocument
* operation.
*
*
* The documents are deleted asynchronously. You can see the progress of the deletion by using AWS CloudWatch. Any
* error messages releated to the processing of the batch are sent to you CloudWatch log.
*
*
* @param batchDeleteDocumentRequest
* @return Result of the BatchDeleteDocument operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.BatchDeleteDocument
* @see AWS API
* Documentation
*/
@Override
public BatchDeleteDocumentResult batchDeleteDocument(BatchDeleteDocumentRequest request) {
request = beforeClientExecution(request);
return executeBatchDeleteDocument(request);
}
@SdkInternalApi
final BatchDeleteDocumentResult executeBatchDeleteDocument(BatchDeleteDocumentRequest batchDeleteDocumentRequest) {
ExecutionContext executionContext = createExecutionContext(batchDeleteDocumentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDeleteDocumentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchDeleteDocumentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDeleteDocument");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new BatchDeleteDocumentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds one or more documents to an index.
*
*
* The BatchPutDocument
operation enables you to ingest inline documents or a set of documents stored
* in an Amazon S3 bucket. Use this operation to ingest your text and unstructured text into an index, add custom
* attributes to the documents, and to attach an access control list to the documents added to the index.
*
*
* The documents are indexed asynchronously. You can see the progress of the batch using AWS CloudWatch. Any error
* messages related to processing the batch are sent to your AWS CloudWatch log.
*
*
* @param batchPutDocumentRequest
* @return Result of the BatchPutDocument operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws ServiceQuotaExceededException
* @throws InternalServerException
* @sample AWSkendra.BatchPutDocument
* @see AWS API
* Documentation
*/
@Override
public BatchPutDocumentResult batchPutDocument(BatchPutDocumentRequest request) {
request = beforeClientExecution(request);
return executeBatchPutDocument(request);
}
@SdkInternalApi
final BatchPutDocumentResult executeBatchPutDocument(BatchPutDocumentRequest batchPutDocumentRequest) {
ExecutionContext executionContext = createExecutionContext(batchPutDocumentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchPutDocumentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchPutDocumentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchPutDocument");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new BatchPutDocumentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a data source that you use to with an Amazon Kendra index.
*
*
* You specify a name, connector type and description for your data source. You can choose between an S3 connector,
* a SharePoint Online connector, and a database connector.
*
*
* You also specify configuration information such as document metadata (author, source URI, and so on) and user
* context information.
*
*
* CreateDataSource
is a synchronous operation. The operation returns 200 if the data source was
* successfully created. Otherwise, an exception is raised.
*
*
* @param createDataSourceRequest
* @return Result of the CreateDataSource operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ResourceAlreadyExistException
* @throws ServiceQuotaExceededException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.CreateDataSource
* @see AWS API
* Documentation
*/
@Override
public CreateDataSourceResult createDataSource(CreateDataSourceRequest request) {
request = beforeClientExecution(request);
return executeCreateDataSource(request);
}
@SdkInternalApi
final CreateDataSourceResult executeCreateDataSource(CreateDataSourceRequest createDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(createDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an new set of frequently asked question (FAQ) questions and answers.
*
*
* @param createFaqRequest
* @return Result of the CreateFaq operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws ServiceQuotaExceededException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.CreateFaq
* @see AWS API
* Documentation
*/
@Override
public CreateFaqResult createFaq(CreateFaqRequest request) {
request = beforeClientExecution(request);
return executeCreateFaq(request);
}
@SdkInternalApi
final CreateFaqResult executeCreateFaq(CreateFaqRequest createFaqRequest) {
ExecutionContext executionContext = createExecutionContext(createFaqRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFaqRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFaqRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFaq");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFaqResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Kendra index. Index creation is an asynchronous operation. To determine if index creation
* has completed, check the Status
field returned from a call to . The Status
field is set
* to ACTIVE
when the index is ready to use.
*
*
* Once the index is active you can index your documents using the operation or using one of the supported data
* sources.
*
*
* @param createIndexRequest
* @return Result of the CreateIndex operation returned by the service.
* @throws ValidationException
* @throws ResourceAlreadyExistException
* @throws ServiceQuotaExceededException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.CreateIndex
* @see AWS API
* Documentation
*/
@Override
public CreateIndexResult createIndex(CreateIndexRequest request) {
request = beforeClientExecution(request);
return executeCreateIndex(request);
}
@SdkInternalApi
final CreateIndexResult executeCreateIndex(CreateIndexRequest createIndexRequest) {
ExecutionContext executionContext = createExecutionContext(createIndexRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateIndexRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createIndexRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateIndex");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateIndexResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes an FAQ from an index.
*
*
* @param deleteFaqRequest
* @return Result of the DeleteFaq operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.DeleteFaq
* @see AWS API
* Documentation
*/
@Override
public DeleteFaqResult deleteFaq(DeleteFaqRequest request) {
request = beforeClientExecution(request);
return executeDeleteFaq(request);
}
@SdkInternalApi
final DeleteFaqResult executeDeleteFaq(DeleteFaqRequest deleteFaqRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFaqRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFaqRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFaqRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFaq");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFaqResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing Amazon Kendra index. An exception is not thrown if the index is already being deleted. While
* the index is being deleted, the Status
field returned by a call to the DescribeIndex
* operation is set to DELETING
.
*
*
* @param deleteIndexRequest
* @return Result of the DeleteIndex operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.DeleteIndex
* @see AWS API
* Documentation
*/
@Override
public DeleteIndexResult deleteIndex(DeleteIndexRequest request) {
request = beforeClientExecution(request);
return executeDeleteIndex(request);
}
@SdkInternalApi
final DeleteIndexResult executeDeleteIndex(DeleteIndexRequest deleteIndexRequest) {
ExecutionContext executionContext = createExecutionContext(deleteIndexRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteIndexRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteIndexRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteIndex");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteIndexResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a Amazon Kendra data source.
*
*
* @param describeDataSourceRequest
* @return Result of the DescribeDataSource operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.DescribeDataSource
* @see AWS API
* Documentation
*/
@Override
public DescribeDataSourceResult describeDataSource(DescribeDataSourceRequest request) {
request = beforeClientExecution(request);
return executeDescribeDataSource(request);
}
@SdkInternalApi
final DescribeDataSourceResult executeDescribeDataSource(DescribeDataSourceRequest describeDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(describeDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about an FAQ list.
*
*
* @param describeFaqRequest
* @return Result of the DescribeFaq operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.DescribeFaq
* @see AWS API
* Documentation
*/
@Override
public DescribeFaqResult describeFaq(DescribeFaqRequest request) {
request = beforeClientExecution(request);
return executeDescribeFaq(request);
}
@SdkInternalApi
final DescribeFaqResult executeDescribeFaq(DescribeFaqRequest describeFaqRequest) {
ExecutionContext executionContext = createExecutionContext(describeFaqRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFaqRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFaqRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFaq");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFaqResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an existing Amazon Kendra index
*
*
* @param describeIndexRequest
* @return Result of the DescribeIndex operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.DescribeIndex
* @see AWS API
* Documentation
*/
@Override
public DescribeIndexResult describeIndex(DescribeIndexRequest request) {
request = beforeClientExecution(request);
return executeDescribeIndex(request);
}
@SdkInternalApi
final DescribeIndexResult executeDescribeIndex(DescribeIndexRequest describeIndexRequest) {
ExecutionContext executionContext = createExecutionContext(describeIndexRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeIndexRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeIndexRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeIndex");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeIndexResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets statistics about synchronizing Amazon Kendra with a data source.
*
*
* @param listDataSourceSyncJobsRequest
* @return Result of the ListDataSourceSyncJobs operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws ConflictException
* @throws InternalServerException
* @sample AWSkendra.ListDataSourceSyncJobs
* @see AWS
* API Documentation
*/
@Override
public ListDataSourceSyncJobsResult listDataSourceSyncJobs(ListDataSourceSyncJobsRequest request) {
request = beforeClientExecution(request);
return executeListDataSourceSyncJobs(request);
}
@SdkInternalApi
final ListDataSourceSyncJobsResult executeListDataSourceSyncJobs(ListDataSourceSyncJobsRequest listDataSourceSyncJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listDataSourceSyncJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataSourceSyncJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDataSourceSyncJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataSourceSyncJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListDataSourceSyncJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the data sources that you have created.
*
*
* @param listDataSourcesRequest
* @return Result of the ListDataSources operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws AccessDeniedException
* @throws ThrottlingException
* @throws InternalServerException
* @sample AWSkendra.ListDataSources
* @see AWS API
* Documentation
*/
@Override
public ListDataSourcesResult listDataSources(ListDataSourcesRequest request) {
request = beforeClientExecution(request);
return executeListDataSources(request);
}
@SdkInternalApi
final ListDataSourcesResult executeListDataSources(ListDataSourcesRequest listDataSourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listDataSourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDataSourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDataSourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDataSources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDataSourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of FAQ lists associated with an index.
*
*
* @param listFaqsRequest
* @return Result of the ListFaqs operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.ListFaqs
* @see AWS API
* Documentation
*/
@Override
public ListFaqsResult listFaqs(ListFaqsRequest request) {
request = beforeClientExecution(request);
return executeListFaqs(request);
}
@SdkInternalApi
final ListFaqsResult executeListFaqs(ListFaqsRequest listFaqsRequest) {
ExecutionContext executionContext = createExecutionContext(listFaqsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFaqsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFaqsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFaqs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFaqsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the Amazon Kendra indexes that you have created.
*
*
* @param listIndicesRequest
* @return Result of the ListIndices operation returned by the service.
* @throws ValidationException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.ListIndices
* @see AWS API
* Documentation
*/
@Override
public ListIndicesResult listIndices(ListIndicesRequest request) {
request = beforeClientExecution(request);
return executeListIndices(request);
}
@SdkInternalApi
final ListIndicesResult executeListIndices(ListIndicesRequest listIndicesRequest) {
ExecutionContext executionContext = createExecutionContext(listIndicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListIndicesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listIndicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListIndices");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListIndicesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Searches an active index. Use this API to search your documents using query. The Query
operation
* enables to do faceted search and to filter results based on document attributes.
*
*
* It also enables you to provide user context that Amazon Kendra uses to enforce document access control in the
* search results.
*
*
* Amazon Kendra searches your index for text content and question and answer (FAQ) content. By default the response
* contains three types of results.
*
*
* -
*
* Relevant passages
*
*
* -
*
* Matching FAQs
*
*
* -
*
* Relevant documents
*
*
*
*
* You can specify that the query return only one type of result using the QueryResultTypeConfig
* parameter.
*
*
* @param queryRequest
* @return Result of the Query operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.Query
* @see AWS API
* Documentation
*/
@Override
public QueryResult query(QueryRequest request) {
request = beforeClientExecution(request);
return executeQuery(request);
}
@SdkInternalApi
final QueryResult executeQuery(QueryRequest queryRequest) {
ExecutionContext executionContext = createExecutionContext(queryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new QueryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(queryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "Query");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new QueryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a synchronization job for a data source. If a synchronization job is already in progress, Amazon Kendra
* returns a ResourceInUseException
exception.
*
*
* @param startDataSourceSyncJobRequest
* @return Result of the StartDataSourceSyncJob operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws ResourceInUseException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws ConflictException
* @throws InternalServerException
* @sample AWSkendra.StartDataSourceSyncJob
* @see AWS
* API Documentation
*/
@Override
public StartDataSourceSyncJobResult startDataSourceSyncJob(StartDataSourceSyncJobRequest request) {
request = beforeClientExecution(request);
return executeStartDataSourceSyncJob(request);
}
@SdkInternalApi
final StartDataSourceSyncJobResult executeStartDataSourceSyncJob(StartDataSourceSyncJobRequest startDataSourceSyncJobRequest) {
ExecutionContext executionContext = createExecutionContext(startDataSourceSyncJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartDataSourceSyncJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startDataSourceSyncJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartDataSourceSyncJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartDataSourceSyncJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a running synchronization job. You can't stop a scheduled synchronization job.
*
*
* @param stopDataSourceSyncJobRequest
* @return Result of the StopDataSourceSyncJob operation returned by the service.
* @throws ValidationException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.StopDataSourceSyncJob
* @see AWS
* API Documentation
*/
@Override
public StopDataSourceSyncJobResult stopDataSourceSyncJob(StopDataSourceSyncJobRequest request) {
request = beforeClientExecution(request);
return executeStopDataSourceSyncJob(request);
}
@SdkInternalApi
final StopDataSourceSyncJobResult executeStopDataSourceSyncJob(StopDataSourceSyncJobRequest stopDataSourceSyncJobRequest) {
ExecutionContext executionContext = createExecutionContext(stopDataSourceSyncJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopDataSourceSyncJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopDataSourceSyncJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopDataSourceSyncJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopDataSourceSyncJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables you to provide feedback to Amazon Kendra to improve the performance of the service.
*
*
* @param submitFeedbackRequest
* @return Result of the SubmitFeedback operation returned by the service.
* @throws ValidationException
* @throws ResourceUnavailableException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.SubmitFeedback
* @see AWS API
* Documentation
*/
@Override
public SubmitFeedbackResult submitFeedback(SubmitFeedbackRequest request) {
request = beforeClientExecution(request);
return executeSubmitFeedback(request);
}
@SdkInternalApi
final SubmitFeedbackResult executeSubmitFeedback(SubmitFeedbackRequest submitFeedbackRequest) {
ExecutionContext executionContext = createExecutionContext(submitFeedbackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SubmitFeedbackRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(submitFeedbackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SubmitFeedback");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SubmitFeedbackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing Amazon Kendra data source.
*
*
* @param updateDataSourceRequest
* @return Result of the UpdateDataSource operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.UpdateDataSource
* @see AWS API
* Documentation
*/
@Override
public UpdateDataSourceResult updateDataSource(UpdateDataSourceRequest request) {
request = beforeClientExecution(request);
return executeUpdateDataSource(request);
}
@SdkInternalApi
final UpdateDataSourceResult executeUpdateDataSource(UpdateDataSourceRequest updateDataSourceRequest) {
ExecutionContext executionContext = createExecutionContext(updateDataSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDataSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDataSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDataSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDataSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing Amazon Kendra index.
*
*
* @param updateIndexRequest
* @return Result of the UpdateIndex operation returned by the service.
* @throws ValidationException
* @throws ConflictException
* @throws ResourceNotFoundException
* @throws ThrottlingException
* @throws AccessDeniedException
* @throws InternalServerException
* @sample AWSkendra.UpdateIndex
* @see AWS API
* Documentation
*/
@Override
public UpdateIndexResult updateIndex(UpdateIndexRequest request) {
request = beforeClientExecution(request);
return executeUpdateIndex(request);
}
@SdkInternalApi
final UpdateIndexResult executeUpdateIndex(UpdateIndexRequest updateIndexRequest) {
ExecutionContext executionContext = createExecutionContext(updateIndexRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateIndexRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateIndexRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "kendra");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateIndex");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateIndexResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
}