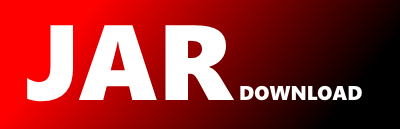
com.amazonaws.services.kendra.model.Relevance Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information for manually tuning the relevance of a field in a search. When a query includes terms that match
* the field, the results are given a boost in the response based on these tuning parameters.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Relevance implements Serializable, Cloneable, StructuredPojo {
/**
*
* Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can only set
* the Freshness
field on one DATE
type field. Only applies to DATE
fields.
*
*/
private Boolean freshness;
/**
*
* The relative importance of the field in the search. Larger numbers provide more of a boost than smaller numbers.
*
*/
private Integer importance;
/**
*
* Specifies the time period that the boost applies to. For example, to make the boost apply to documents with the
* field value within the last month, you would use "2628000s". Once the field value is beyond the specified range,
* the effect of the boost drops off. The higher the importance, the faster the effect drops off. If you don't
* specify a value, the default is 3 months. The value of the field is a numeric string followed by the character
* "s", for example "86400s" for one day, or "604800s" for one week.
*
*
* Only applies to DATE
fields.
*
*/
private String duration;
/**
*
* Determines how values should be interpreted.
*
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example, in a
* task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
*
*/
private String rankOrder;
/**
*
* A list of values that should be given a different boost when they appear in the result list. For example, if you
* are boosting a field called "department," query terms that match the department field are boosted in the result.
* However, you can add entries from the department field to boost documents with those values higher.
*
*
* For example, you can add entries to the map with names of departments. If you add "HR",5 and "Legal",3 those
* departments are given special attention when they appear in the metadata of a document. When those terms appear
* they are given the specified importance instead of the regular importance for the boost.
*
*/
private java.util.Map valueImportanceMap;
/**
*
* Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can only set
* the Freshness
field on one DATE
type field. Only applies to DATE
fields.
*
*
* @param freshness
* Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can
* only set the Freshness
field on one DATE
type field. Only applies to
* DATE
fields.
*/
public void setFreshness(Boolean freshness) {
this.freshness = freshness;
}
/**
*
* Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can only set
* the Freshness
field on one DATE
type field. Only applies to DATE
fields.
*
*
* @return Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can
* only set the Freshness
field on one DATE
type field. Only applies to
* DATE
fields.
*/
public Boolean getFreshness() {
return this.freshness;
}
/**
*
* Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can only set
* the Freshness
field on one DATE
type field. Only applies to DATE
fields.
*
*
* @param freshness
* Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can
* only set the Freshness
field on one DATE
type field. Only applies to
* DATE
fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Relevance withFreshness(Boolean freshness) {
setFreshness(freshness);
return this;
}
/**
*
* Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can only set
* the Freshness
field on one DATE
type field. Only applies to DATE
fields.
*
*
* @return Indicates that this field determines how "fresh" a document is. For example, if document 1 was created on
* November 5, and document 2 was created on October 31, document 1 is "fresher" than document 2. You can
* only set the Freshness
field on one DATE
type field. Only applies to
* DATE
fields.
*/
public Boolean isFreshness() {
return this.freshness;
}
/**
*
* The relative importance of the field in the search. Larger numbers provide more of a boost than smaller numbers.
*
*
* @param importance
* The relative importance of the field in the search. Larger numbers provide more of a boost than smaller
* numbers.
*/
public void setImportance(Integer importance) {
this.importance = importance;
}
/**
*
* The relative importance of the field in the search. Larger numbers provide more of a boost than smaller numbers.
*
*
* @return The relative importance of the field in the search. Larger numbers provide more of a boost than smaller
* numbers.
*/
public Integer getImportance() {
return this.importance;
}
/**
*
* The relative importance of the field in the search. Larger numbers provide more of a boost than smaller numbers.
*
*
* @param importance
* The relative importance of the field in the search. Larger numbers provide more of a boost than smaller
* numbers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Relevance withImportance(Integer importance) {
setImportance(importance);
return this;
}
/**
*
* Specifies the time period that the boost applies to. For example, to make the boost apply to documents with the
* field value within the last month, you would use "2628000s". Once the field value is beyond the specified range,
* the effect of the boost drops off. The higher the importance, the faster the effect drops off. If you don't
* specify a value, the default is 3 months. The value of the field is a numeric string followed by the character
* "s", for example "86400s" for one day, or "604800s" for one week.
*
*
* Only applies to DATE
fields.
*
*
* @param duration
* Specifies the time period that the boost applies to. For example, to make the boost apply to documents
* with the field value within the last month, you would use "2628000s". Once the field value is beyond the
* specified range, the effect of the boost drops off. The higher the importance, the faster the effect drops
* off. If you don't specify a value, the default is 3 months. The value of the field is a numeric string
* followed by the character "s", for example "86400s" for one day, or "604800s" for one week.
*
* Only applies to DATE
fields.
*/
public void setDuration(String duration) {
this.duration = duration;
}
/**
*
* Specifies the time period that the boost applies to. For example, to make the boost apply to documents with the
* field value within the last month, you would use "2628000s". Once the field value is beyond the specified range,
* the effect of the boost drops off. The higher the importance, the faster the effect drops off. If you don't
* specify a value, the default is 3 months. The value of the field is a numeric string followed by the character
* "s", for example "86400s" for one day, or "604800s" for one week.
*
*
* Only applies to DATE
fields.
*
*
* @return Specifies the time period that the boost applies to. For example, to make the boost apply to documents
* with the field value within the last month, you would use "2628000s". Once the field value is beyond the
* specified range, the effect of the boost drops off. The higher the importance, the faster the effect
* drops off. If you don't specify a value, the default is 3 months. The value of the field is a numeric
* string followed by the character "s", for example "86400s" for one day, or "604800s" for one week.
*
* Only applies to DATE
fields.
*/
public String getDuration() {
return this.duration;
}
/**
*
* Specifies the time period that the boost applies to. For example, to make the boost apply to documents with the
* field value within the last month, you would use "2628000s". Once the field value is beyond the specified range,
* the effect of the boost drops off. The higher the importance, the faster the effect drops off. If you don't
* specify a value, the default is 3 months. The value of the field is a numeric string followed by the character
* "s", for example "86400s" for one day, or "604800s" for one week.
*
*
* Only applies to DATE
fields.
*
*
* @param duration
* Specifies the time period that the boost applies to. For example, to make the boost apply to documents
* with the field value within the last month, you would use "2628000s". Once the field value is beyond the
* specified range, the effect of the boost drops off. The higher the importance, the faster the effect drops
* off. If you don't specify a value, the default is 3 months. The value of the field is a numeric string
* followed by the character "s", for example "86400s" for one day, or "604800s" for one week.
*
* Only applies to DATE
fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Relevance withDuration(String duration) {
setDuration(duration);
return this;
}
/**
*
* Determines how values should be interpreted.
*
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example, in a
* task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
*
*
* @param rankOrder
* Determines how values should be interpreted.
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example,
* in a task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
* @see Order
*/
public void setRankOrder(String rankOrder) {
this.rankOrder = rankOrder;
}
/**
*
* Determines how values should be interpreted.
*
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example, in a
* task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
*
*
* @return Determines how values should be interpreted.
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example,
* a document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example,
* in a task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
* @see Order
*/
public String getRankOrder() {
return this.rankOrder;
}
/**
*
* Determines how values should be interpreted.
*
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example, in a
* task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
*
*
* @param rankOrder
* Determines how values should be interpreted.
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example,
* in a task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Order
*/
public Relevance withRankOrder(String rankOrder) {
setRankOrder(rankOrder);
return this;
}
/**
*
* Determines how values should be interpreted.
*
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example, in a
* task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
*
*
* @param rankOrder
* Determines how values should be interpreted.
*
* When the RankOrder
field is ASCENDING
, higher numbers are better. For example, a
* document with a rating score of 10 is higher ranking than a document with a rating score of 1.
*
*
* When the RankOrder
field is DESCENDING
, lower numbers are better. For example,
* in a task tracking application, a priority 1 task is more important than a priority 5 task.
*
*
* Only applies to LONG
and DOUBLE
fields.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Order
*/
public Relevance withRankOrder(Order rankOrder) {
this.rankOrder = rankOrder.toString();
return this;
}
/**
*
* A list of values that should be given a different boost when they appear in the result list. For example, if you
* are boosting a field called "department," query terms that match the department field are boosted in the result.
* However, you can add entries from the department field to boost documents with those values higher.
*
*
* For example, you can add entries to the map with names of departments. If you add "HR",5 and "Legal",3 those
* departments are given special attention when they appear in the metadata of a document. When those terms appear
* they are given the specified importance instead of the regular importance for the boost.
*
*
* @return A list of values that should be given a different boost when they appear in the result list. For example,
* if you are boosting a field called "department," query terms that match the department field are boosted
* in the result. However, you can add entries from the department field to boost documents with those
* values higher.
*
* For example, you can add entries to the map with names of departments. If you add "HR",5 and "Legal",3
* those departments are given special attention when they appear in the metadata of a document. When those
* terms appear they are given the specified importance instead of the regular importance for the boost.
*/
public java.util.Map getValueImportanceMap() {
return valueImportanceMap;
}
/**
*
* A list of values that should be given a different boost when they appear in the result list. For example, if you
* are boosting a field called "department," query terms that match the department field are boosted in the result.
* However, you can add entries from the department field to boost documents with those values higher.
*
*
* For example, you can add entries to the map with names of departments. If you add "HR",5 and "Legal",3 those
* departments are given special attention when they appear in the metadata of a document. When those terms appear
* they are given the specified importance instead of the regular importance for the boost.
*
*
* @param valueImportanceMap
* A list of values that should be given a different boost when they appear in the result list. For example,
* if you are boosting a field called "department," query terms that match the department field are boosted
* in the result. However, you can add entries from the department field to boost documents with those values
* higher.
*
* For example, you can add entries to the map with names of departments. If you add "HR",5 and "Legal",3
* those departments are given special attention when they appear in the metadata of a document. When those
* terms appear they are given the specified importance instead of the regular importance for the boost.
*/
public void setValueImportanceMap(java.util.Map valueImportanceMap) {
this.valueImportanceMap = valueImportanceMap;
}
/**
*
* A list of values that should be given a different boost when they appear in the result list. For example, if you
* are boosting a field called "department," query terms that match the department field are boosted in the result.
* However, you can add entries from the department field to boost documents with those values higher.
*
*
* For example, you can add entries to the map with names of departments. If you add "HR",5 and "Legal",3 those
* departments are given special attention when they appear in the metadata of a document. When those terms appear
* they are given the specified importance instead of the regular importance for the boost.
*
*
* @param valueImportanceMap
* A list of values that should be given a different boost when they appear in the result list. For example,
* if you are boosting a field called "department," query terms that match the department field are boosted
* in the result. However, you can add entries from the department field to boost documents with those values
* higher.
*
* For example, you can add entries to the map with names of departments. If you add "HR",5 and "Legal",3
* those departments are given special attention when they appear in the metadata of a document. When those
* terms appear they are given the specified importance instead of the regular importance for the boost.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Relevance withValueImportanceMap(java.util.Map valueImportanceMap) {
setValueImportanceMap(valueImportanceMap);
return this;
}
/**
* Add a single ValueImportanceMap entry
*
* @see Relevance#withValueImportanceMap
* @returns a reference to this object so that method calls can be chained together.
*/
public Relevance addValueImportanceMapEntry(String key, Integer value) {
if (null == this.valueImportanceMap) {
this.valueImportanceMap = new java.util.HashMap();
}
if (this.valueImportanceMap.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.valueImportanceMap.put(key, value);
return this;
}
/**
* Removes all the entries added into ValueImportanceMap.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Relevance clearValueImportanceMapEntries() {
this.valueImportanceMap = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFreshness() != null)
sb.append("Freshness: ").append(getFreshness()).append(",");
if (getImportance() != null)
sb.append("Importance: ").append(getImportance()).append(",");
if (getDuration() != null)
sb.append("Duration: ").append(getDuration()).append(",");
if (getRankOrder() != null)
sb.append("RankOrder: ").append(getRankOrder()).append(",");
if (getValueImportanceMap() != null)
sb.append("ValueImportanceMap: ").append(getValueImportanceMap());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Relevance == false)
return false;
Relevance other = (Relevance) obj;
if (other.getFreshness() == null ^ this.getFreshness() == null)
return false;
if (other.getFreshness() != null && other.getFreshness().equals(this.getFreshness()) == false)
return false;
if (other.getImportance() == null ^ this.getImportance() == null)
return false;
if (other.getImportance() != null && other.getImportance().equals(this.getImportance()) == false)
return false;
if (other.getDuration() == null ^ this.getDuration() == null)
return false;
if (other.getDuration() != null && other.getDuration().equals(this.getDuration()) == false)
return false;
if (other.getRankOrder() == null ^ this.getRankOrder() == null)
return false;
if (other.getRankOrder() != null && other.getRankOrder().equals(this.getRankOrder()) == false)
return false;
if (other.getValueImportanceMap() == null ^ this.getValueImportanceMap() == null)
return false;
if (other.getValueImportanceMap() != null && other.getValueImportanceMap().equals(this.getValueImportanceMap()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFreshness() == null) ? 0 : getFreshness().hashCode());
hashCode = prime * hashCode + ((getImportance() == null) ? 0 : getImportance().hashCode());
hashCode = prime * hashCode + ((getDuration() == null) ? 0 : getDuration().hashCode());
hashCode = prime * hashCode + ((getRankOrder() == null) ? 0 : getRankOrder().hashCode());
hashCode = prime * hashCode + ((getValueImportanceMap() == null) ? 0 : getValueImportanceMap().hashCode());
return hashCode;
}
@Override
public Relevance clone() {
try {
return (Relevance) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.RelevanceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}