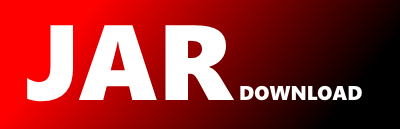
com.amazonaws.services.kendra.model.ConfluenceConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides the configuration information to connect to Confluence as your data source.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ConfluenceConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The URL of your Confluence instance. Use the full URL of the server. For example,
* https://server.example.com:port/. You can also use an IP address, for example,
* https://192.168.1.113/.
*
*/
private String serverUrl;
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the user name and password required to
* connect to the Confluence instance. If you use Confluence Cloud, you use a generated API token as the password.
*
*
* You can also provide authentication credentials in the form of a personal access token. For more information, see
* Using a Confluence data
* source.
*
*/
private String secretArn;
/**
*
* The version or the type of Confluence installation to connect to.
*
*/
private String version;
/**
*
* Configuration information for indexing Confluence spaces.
*
*/
private ConfluenceSpaceConfiguration spaceConfiguration;
/**
*
* Configuration information for indexing Confluence pages.
*
*/
private ConfluencePageConfiguration pageConfiguration;
/**
*
* Configuration information for indexing Confluence blogs.
*
*/
private ConfluenceBlogConfiguration blogConfiguration;
/**
*
* Configuration information for indexing attachments to Confluence blogs and pages.
*
*/
private ConfluenceAttachmentConfiguration attachmentConfiguration;
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Confluence. For more
* information, see Configuring a
* VPC.
*
*/
private DataSourceVpcConfiguration vpcConfiguration;
/**
*
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the patterns
* is excluded from the index. If content matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the content isn't included in the index.
*
*/
private java.util.List inclusionPatterns;
/**
*
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the exclusion
* pattern takes precedence and the content isn't included in the index.
*
*/
private java.util.List exclusionPatterns;
/**
*
* Configuration information to connect to your Confluence URL instance via a web proxy. You can use this option for
* Confluence Server.
*
*
* You must provide the website host name and port number. For example, the host name of
* https://a.example.com/page1.html is "a.example.com" and the port is 443, the standard port for HTTPS.
*
*
* Web proxy credentials are optional and you can use them to connect to a web proxy server that requires basic
* authentication of user name and password. To store web proxy credentials, you use a secret in Secrets Manager.
*
*
* It is recommended that you follow best security practices when configuring your web proxy. This includes setting
* up throttling, setting up logging and monitoring, and applying security patches on a regular basis. If you use
* your web proxy with multiple data sources, sync jobs that occur at the same time could strain the load on your
* proxy. It is recommended you prepare your proxy beforehand for any security and load requirements.
*
*/
private ProxyConfiguration proxyConfiguration;
/**
*
* Whether you want to connect to Confluence using basic authentication of user name and password, or a personal
* access token. You can use a personal access token for Confluence Server.
*
*/
private String authenticationType;
/**
*
* The URL of your Confluence instance. Use the full URL of the server. For example,
* https://server.example.com:port/. You can also use an IP address, for example,
* https://192.168.1.113/.
*
*
* @param serverUrl
* The URL of your Confluence instance. Use the full URL of the server. For example,
* https://server.example.com:port/. You can also use an IP address, for example,
* https://192.168.1.113/.
*/
public void setServerUrl(String serverUrl) {
this.serverUrl = serverUrl;
}
/**
*
* The URL of your Confluence instance. Use the full URL of the server. For example,
* https://server.example.com:port/. You can also use an IP address, for example,
* https://192.168.1.113/.
*
*
* @return The URL of your Confluence instance. Use the full URL of the server. For example,
* https://server.example.com:port/. You can also use an IP address, for example,
* https://192.168.1.113/.
*/
public String getServerUrl() {
return this.serverUrl;
}
/**
*
* The URL of your Confluence instance. Use the full URL of the server. For example,
* https://server.example.com:port/. You can also use an IP address, for example,
* https://192.168.1.113/.
*
*
* @param serverUrl
* The URL of your Confluence instance. Use the full URL of the server. For example,
* https://server.example.com:port/. You can also use an IP address, for example,
* https://192.168.1.113/.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withServerUrl(String serverUrl) {
setServerUrl(serverUrl);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the user name and password required to
* connect to the Confluence instance. If you use Confluence Cloud, you use a generated API token as the password.
*
*
* You can also provide authentication credentials in the form of a personal access token. For more information, see
* Using a Confluence data
* source.
*
*
* @param secretArn
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the user name and password
* required to connect to the Confluence instance. If you use Confluence Cloud, you use a generated API token
* as the password.
*
* You can also provide authentication credentials in the form of a personal access token. For more
* information, see Using
* a Confluence data source.
*/
public void setSecretArn(String secretArn) {
this.secretArn = secretArn;
}
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the user name and password required to
* connect to the Confluence instance. If you use Confluence Cloud, you use a generated API token as the password.
*
*
* You can also provide authentication credentials in the form of a personal access token. For more information, see
* Using a Confluence data
* source.
*
*
* @return The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the user name and password
* required to connect to the Confluence instance. If you use Confluence Cloud, you use a generated API
* token as the password.
*
* You can also provide authentication credentials in the form of a personal access token. For more
* information, see Using
* a Confluence data source.
*/
public String getSecretArn() {
return this.secretArn;
}
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the user name and password required to
* connect to the Confluence instance. If you use Confluence Cloud, you use a generated API token as the password.
*
*
* You can also provide authentication credentials in the form of a personal access token. For more information, see
* Using a Confluence data
* source.
*
*
* @param secretArn
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the user name and password
* required to connect to the Confluence instance. If you use Confluence Cloud, you use a generated API token
* as the password.
*
* You can also provide authentication credentials in the form of a personal access token. For more
* information, see Using
* a Confluence data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withSecretArn(String secretArn) {
setSecretArn(secretArn);
return this;
}
/**
*
* The version or the type of Confluence installation to connect to.
*
*
* @param version
* The version or the type of Confluence installation to connect to.
* @see ConfluenceVersion
*/
public void setVersion(String version) {
this.version = version;
}
/**
*
* The version or the type of Confluence installation to connect to.
*
*
* @return The version or the type of Confluence installation to connect to.
* @see ConfluenceVersion
*/
public String getVersion() {
return this.version;
}
/**
*
* The version or the type of Confluence installation to connect to.
*
*
* @param version
* The version or the type of Confluence installation to connect to.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfluenceVersion
*/
public ConfluenceConfiguration withVersion(String version) {
setVersion(version);
return this;
}
/**
*
* The version or the type of Confluence installation to connect to.
*
*
* @param version
* The version or the type of Confluence installation to connect to.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfluenceVersion
*/
public ConfluenceConfiguration withVersion(ConfluenceVersion version) {
this.version = version.toString();
return this;
}
/**
*
* Configuration information for indexing Confluence spaces.
*
*
* @param spaceConfiguration
* Configuration information for indexing Confluence spaces.
*/
public void setSpaceConfiguration(ConfluenceSpaceConfiguration spaceConfiguration) {
this.spaceConfiguration = spaceConfiguration;
}
/**
*
* Configuration information for indexing Confluence spaces.
*
*
* @return Configuration information for indexing Confluence spaces.
*/
public ConfluenceSpaceConfiguration getSpaceConfiguration() {
return this.spaceConfiguration;
}
/**
*
* Configuration information for indexing Confluence spaces.
*
*
* @param spaceConfiguration
* Configuration information for indexing Confluence spaces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withSpaceConfiguration(ConfluenceSpaceConfiguration spaceConfiguration) {
setSpaceConfiguration(spaceConfiguration);
return this;
}
/**
*
* Configuration information for indexing Confluence pages.
*
*
* @param pageConfiguration
* Configuration information for indexing Confluence pages.
*/
public void setPageConfiguration(ConfluencePageConfiguration pageConfiguration) {
this.pageConfiguration = pageConfiguration;
}
/**
*
* Configuration information for indexing Confluence pages.
*
*
* @return Configuration information for indexing Confluence pages.
*/
public ConfluencePageConfiguration getPageConfiguration() {
return this.pageConfiguration;
}
/**
*
* Configuration information for indexing Confluence pages.
*
*
* @param pageConfiguration
* Configuration information for indexing Confluence pages.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withPageConfiguration(ConfluencePageConfiguration pageConfiguration) {
setPageConfiguration(pageConfiguration);
return this;
}
/**
*
* Configuration information for indexing Confluence blogs.
*
*
* @param blogConfiguration
* Configuration information for indexing Confluence blogs.
*/
public void setBlogConfiguration(ConfluenceBlogConfiguration blogConfiguration) {
this.blogConfiguration = blogConfiguration;
}
/**
*
* Configuration information for indexing Confluence blogs.
*
*
* @return Configuration information for indexing Confluence blogs.
*/
public ConfluenceBlogConfiguration getBlogConfiguration() {
return this.blogConfiguration;
}
/**
*
* Configuration information for indexing Confluence blogs.
*
*
* @param blogConfiguration
* Configuration information for indexing Confluence blogs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withBlogConfiguration(ConfluenceBlogConfiguration blogConfiguration) {
setBlogConfiguration(blogConfiguration);
return this;
}
/**
*
* Configuration information for indexing attachments to Confluence blogs and pages.
*
*
* @param attachmentConfiguration
* Configuration information for indexing attachments to Confluence blogs and pages.
*/
public void setAttachmentConfiguration(ConfluenceAttachmentConfiguration attachmentConfiguration) {
this.attachmentConfiguration = attachmentConfiguration;
}
/**
*
* Configuration information for indexing attachments to Confluence blogs and pages.
*
*
* @return Configuration information for indexing attachments to Confluence blogs and pages.
*/
public ConfluenceAttachmentConfiguration getAttachmentConfiguration() {
return this.attachmentConfiguration;
}
/**
*
* Configuration information for indexing attachments to Confluence blogs and pages.
*
*
* @param attachmentConfiguration
* Configuration information for indexing attachments to Confluence blogs and pages.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withAttachmentConfiguration(ConfluenceAttachmentConfiguration attachmentConfiguration) {
setAttachmentConfiguration(attachmentConfiguration);
return this;
}
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Confluence. For more
* information, see Configuring a
* VPC.
*
*
* @param vpcConfiguration
* Configuration information for an Amazon Virtual Private Cloud to connect to your Confluence. For more
* information, see Configuring
* a VPC.
*/
public void setVpcConfiguration(DataSourceVpcConfiguration vpcConfiguration) {
this.vpcConfiguration = vpcConfiguration;
}
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Confluence. For more
* information, see Configuring a
* VPC.
*
*
* @return Configuration information for an Amazon Virtual Private Cloud to connect to your Confluence. For more
* information, see Configuring a VPC.
*/
public DataSourceVpcConfiguration getVpcConfiguration() {
return this.vpcConfiguration;
}
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Confluence. For more
* information, see Configuring a
* VPC.
*
*
* @param vpcConfiguration
* Configuration information for an Amazon Virtual Private Cloud to connect to your Confluence. For more
* information, see Configuring
* a VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withVpcConfiguration(DataSourceVpcConfiguration vpcConfiguration) {
setVpcConfiguration(vpcConfiguration);
return this;
}
/**
*
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the patterns
* is excluded from the index. If content matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the content isn't included in the index.
*
*
* @return A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in
* your Confluence. Content that matches the patterns are included in the index. Content that doesn't match
* the patterns is excluded from the index. If content matches both an inclusion and exclusion pattern, the
* exclusion pattern takes precedence and the content isn't included in the index.
*/
public java.util.List getInclusionPatterns() {
return inclusionPatterns;
}
/**
*
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the patterns
* is excluded from the index. If content matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the content isn't included in the index.
*
*
* @param inclusionPatterns
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the
* patterns is excluded from the index. If content matches both an inclusion and exclusion pattern, the
* exclusion pattern takes precedence and the content isn't included in the index.
*/
public void setInclusionPatterns(java.util.Collection inclusionPatterns) {
if (inclusionPatterns == null) {
this.inclusionPatterns = null;
return;
}
this.inclusionPatterns = new java.util.ArrayList(inclusionPatterns);
}
/**
*
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the patterns
* is excluded from the index. If content matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the content isn't included in the index.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInclusionPatterns(java.util.Collection)} or {@link #withInclusionPatterns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param inclusionPatterns
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the
* patterns is excluded from the index. If content matches both an inclusion and exclusion pattern, the
* exclusion pattern takes precedence and the content isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withInclusionPatterns(String... inclusionPatterns) {
if (this.inclusionPatterns == null) {
setInclusionPatterns(new java.util.ArrayList(inclusionPatterns.length));
}
for (String ele : inclusionPatterns) {
this.inclusionPatterns.add(ele);
}
return this;
}
/**
*
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the patterns
* is excluded from the index. If content matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the content isn't included in the index.
*
*
* @param inclusionPatterns
* A list of regular expression patterns to include certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are included in the index. Content that doesn't match the
* patterns is excluded from the index. If content matches both an inclusion and exclusion pattern, the
* exclusion pattern takes precedence and the content isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withInclusionPatterns(java.util.Collection inclusionPatterns) {
setInclusionPatterns(inclusionPatterns);
return this;
}
/**
*
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the exclusion
* pattern takes precedence and the content isn't included in the index.
*
*
* @return A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in
* your Confluence. Content that matches the patterns are excluded from the index. Content that doesn't
* match the patterns is included in the index. If content matches both an inclusion and exclusion pattern,
* the exclusion pattern takes precedence and the content isn't included in the index.
*/
public java.util.List getExclusionPatterns() {
return exclusionPatterns;
}
/**
*
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the exclusion
* pattern takes precedence and the content isn't included in the index.
*
*
* @param exclusionPatterns
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the
* exclusion pattern takes precedence and the content isn't included in the index.
*/
public void setExclusionPatterns(java.util.Collection exclusionPatterns) {
if (exclusionPatterns == null) {
this.exclusionPatterns = null;
return;
}
this.exclusionPatterns = new java.util.ArrayList(exclusionPatterns);
}
/**
*
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the exclusion
* pattern takes precedence and the content isn't included in the index.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExclusionPatterns(java.util.Collection)} or {@link #withExclusionPatterns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param exclusionPatterns
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the
* exclusion pattern takes precedence and the content isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withExclusionPatterns(String... exclusionPatterns) {
if (this.exclusionPatterns == null) {
setExclusionPatterns(new java.util.ArrayList(exclusionPatterns.length));
}
for (String ele : exclusionPatterns) {
this.exclusionPatterns.add(ele);
}
return this;
}
/**
*
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the exclusion
* pattern takes precedence and the content isn't included in the index.
*
*
* @param exclusionPatterns
* A list of regular expression patterns to exclude certain blog posts, pages, spaces, or attachments in your
* Confluence. Content that matches the patterns are excluded from the index. Content that doesn't match the
* patterns is included in the index. If content matches both an inclusion and exclusion pattern, the
* exclusion pattern takes precedence and the content isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withExclusionPatterns(java.util.Collection exclusionPatterns) {
setExclusionPatterns(exclusionPatterns);
return this;
}
/**
*
* Configuration information to connect to your Confluence URL instance via a web proxy. You can use this option for
* Confluence Server.
*
*
* You must provide the website host name and port number. For example, the host name of
* https://a.example.com/page1.html is "a.example.com" and the port is 443, the standard port for HTTPS.
*
*
* Web proxy credentials are optional and you can use them to connect to a web proxy server that requires basic
* authentication of user name and password. To store web proxy credentials, you use a secret in Secrets Manager.
*
*
* It is recommended that you follow best security practices when configuring your web proxy. This includes setting
* up throttling, setting up logging and monitoring, and applying security patches on a regular basis. If you use
* your web proxy with multiple data sources, sync jobs that occur at the same time could strain the load on your
* proxy. It is recommended you prepare your proxy beforehand for any security and load requirements.
*
*
* @param proxyConfiguration
* Configuration information to connect to your Confluence URL instance via a web proxy. You can use this
* option for Confluence Server.
*
* You must provide the website host name and port number. For example, the host name of
* https://a.example.com/page1.html is "a.example.com" and the port is 443, the standard port for
* HTTPS.
*
*
* Web proxy credentials are optional and you can use them to connect to a web proxy server that requires
* basic authentication of user name and password. To store web proxy credentials, you use a secret in
* Secrets Manager.
*
*
* It is recommended that you follow best security practices when configuring your web proxy. This includes
* setting up throttling, setting up logging and monitoring, and applying security patches on a regular
* basis. If you use your web proxy with multiple data sources, sync jobs that occur at the same time could
* strain the load on your proxy. It is recommended you prepare your proxy beforehand for any security and
* load requirements.
*/
public void setProxyConfiguration(ProxyConfiguration proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration;
}
/**
*
* Configuration information to connect to your Confluence URL instance via a web proxy. You can use this option for
* Confluence Server.
*
*
* You must provide the website host name and port number. For example, the host name of
* https://a.example.com/page1.html is "a.example.com" and the port is 443, the standard port for HTTPS.
*
*
* Web proxy credentials are optional and you can use them to connect to a web proxy server that requires basic
* authentication of user name and password. To store web proxy credentials, you use a secret in Secrets Manager.
*
*
* It is recommended that you follow best security practices when configuring your web proxy. This includes setting
* up throttling, setting up logging and monitoring, and applying security patches on a regular basis. If you use
* your web proxy with multiple data sources, sync jobs that occur at the same time could strain the load on your
* proxy. It is recommended you prepare your proxy beforehand for any security and load requirements.
*
*
* @return Configuration information to connect to your Confluence URL instance via a web proxy. You can use this
* option for Confluence Server.
*
* You must provide the website host name and port number. For example, the host name of
* https://a.example.com/page1.html is "a.example.com" and the port is 443, the standard port for
* HTTPS.
*
*
* Web proxy credentials are optional and you can use them to connect to a web proxy server that requires
* basic authentication of user name and password. To store web proxy credentials, you use a secret in
* Secrets Manager.
*
*
* It is recommended that you follow best security practices when configuring your web proxy. This includes
* setting up throttling, setting up logging and monitoring, and applying security patches on a regular
* basis. If you use your web proxy with multiple data sources, sync jobs that occur at the same time could
* strain the load on your proxy. It is recommended you prepare your proxy beforehand for any security and
* load requirements.
*/
public ProxyConfiguration getProxyConfiguration() {
return this.proxyConfiguration;
}
/**
*
* Configuration information to connect to your Confluence URL instance via a web proxy. You can use this option for
* Confluence Server.
*
*
* You must provide the website host name and port number. For example, the host name of
* https://a.example.com/page1.html is "a.example.com" and the port is 443, the standard port for HTTPS.
*
*
* Web proxy credentials are optional and you can use them to connect to a web proxy server that requires basic
* authentication of user name and password. To store web proxy credentials, you use a secret in Secrets Manager.
*
*
* It is recommended that you follow best security practices when configuring your web proxy. This includes setting
* up throttling, setting up logging and monitoring, and applying security patches on a regular basis. If you use
* your web proxy with multiple data sources, sync jobs that occur at the same time could strain the load on your
* proxy. It is recommended you prepare your proxy beforehand for any security and load requirements.
*
*
* @param proxyConfiguration
* Configuration information to connect to your Confluence URL instance via a web proxy. You can use this
* option for Confluence Server.
*
* You must provide the website host name and port number. For example, the host name of
* https://a.example.com/page1.html is "a.example.com" and the port is 443, the standard port for
* HTTPS.
*
*
* Web proxy credentials are optional and you can use them to connect to a web proxy server that requires
* basic authentication of user name and password. To store web proxy credentials, you use a secret in
* Secrets Manager.
*
*
* It is recommended that you follow best security practices when configuring your web proxy. This includes
* setting up throttling, setting up logging and monitoring, and applying security patches on a regular
* basis. If you use your web proxy with multiple data sources, sync jobs that occur at the same time could
* strain the load on your proxy. It is recommended you prepare your proxy beforehand for any security and
* load requirements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfluenceConfiguration withProxyConfiguration(ProxyConfiguration proxyConfiguration) {
setProxyConfiguration(proxyConfiguration);
return this;
}
/**
*
* Whether you want to connect to Confluence using basic authentication of user name and password, or a personal
* access token. You can use a personal access token for Confluence Server.
*
*
* @param authenticationType
* Whether you want to connect to Confluence using basic authentication of user name and password, or a
* personal access token. You can use a personal access token for Confluence Server.
* @see ConfluenceAuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* Whether you want to connect to Confluence using basic authentication of user name and password, or a personal
* access token. You can use a personal access token for Confluence Server.
*
*
* @return Whether you want to connect to Confluence using basic authentication of user name and password, or a
* personal access token. You can use a personal access token for Confluence Server.
* @see ConfluenceAuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* Whether you want to connect to Confluence using basic authentication of user name and password, or a personal
* access token. You can use a personal access token for Confluence Server.
*
*
* @param authenticationType
* Whether you want to connect to Confluence using basic authentication of user name and password, or a
* personal access token. You can use a personal access token for Confluence Server.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfluenceAuthenticationType
*/
public ConfluenceConfiguration withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* Whether you want to connect to Confluence using basic authentication of user name and password, or a personal
* access token. You can use a personal access token for Confluence Server.
*
*
* @param authenticationType
* Whether you want to connect to Confluence using basic authentication of user name and password, or a
* personal access token. You can use a personal access token for Confluence Server.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfluenceAuthenticationType
*/
public ConfluenceConfiguration withAuthenticationType(ConfluenceAuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServerUrl() != null)
sb.append("ServerUrl: ").append(getServerUrl()).append(",");
if (getSecretArn() != null)
sb.append("SecretArn: ").append(getSecretArn()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion()).append(",");
if (getSpaceConfiguration() != null)
sb.append("SpaceConfiguration: ").append(getSpaceConfiguration()).append(",");
if (getPageConfiguration() != null)
sb.append("PageConfiguration: ").append(getPageConfiguration()).append(",");
if (getBlogConfiguration() != null)
sb.append("BlogConfiguration: ").append(getBlogConfiguration()).append(",");
if (getAttachmentConfiguration() != null)
sb.append("AttachmentConfiguration: ").append(getAttachmentConfiguration()).append(",");
if (getVpcConfiguration() != null)
sb.append("VpcConfiguration: ").append(getVpcConfiguration()).append(",");
if (getInclusionPatterns() != null)
sb.append("InclusionPatterns: ").append(getInclusionPatterns()).append(",");
if (getExclusionPatterns() != null)
sb.append("ExclusionPatterns: ").append(getExclusionPatterns()).append(",");
if (getProxyConfiguration() != null)
sb.append("ProxyConfiguration: ").append(getProxyConfiguration()).append(",");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ConfluenceConfiguration == false)
return false;
ConfluenceConfiguration other = (ConfluenceConfiguration) obj;
if (other.getServerUrl() == null ^ this.getServerUrl() == null)
return false;
if (other.getServerUrl() != null && other.getServerUrl().equals(this.getServerUrl()) == false)
return false;
if (other.getSecretArn() == null ^ this.getSecretArn() == null)
return false;
if (other.getSecretArn() != null && other.getSecretArn().equals(this.getSecretArn()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getSpaceConfiguration() == null ^ this.getSpaceConfiguration() == null)
return false;
if (other.getSpaceConfiguration() != null && other.getSpaceConfiguration().equals(this.getSpaceConfiguration()) == false)
return false;
if (other.getPageConfiguration() == null ^ this.getPageConfiguration() == null)
return false;
if (other.getPageConfiguration() != null && other.getPageConfiguration().equals(this.getPageConfiguration()) == false)
return false;
if (other.getBlogConfiguration() == null ^ this.getBlogConfiguration() == null)
return false;
if (other.getBlogConfiguration() != null && other.getBlogConfiguration().equals(this.getBlogConfiguration()) == false)
return false;
if (other.getAttachmentConfiguration() == null ^ this.getAttachmentConfiguration() == null)
return false;
if (other.getAttachmentConfiguration() != null && other.getAttachmentConfiguration().equals(this.getAttachmentConfiguration()) == false)
return false;
if (other.getVpcConfiguration() == null ^ this.getVpcConfiguration() == null)
return false;
if (other.getVpcConfiguration() != null && other.getVpcConfiguration().equals(this.getVpcConfiguration()) == false)
return false;
if (other.getInclusionPatterns() == null ^ this.getInclusionPatterns() == null)
return false;
if (other.getInclusionPatterns() != null && other.getInclusionPatterns().equals(this.getInclusionPatterns()) == false)
return false;
if (other.getExclusionPatterns() == null ^ this.getExclusionPatterns() == null)
return false;
if (other.getExclusionPatterns() != null && other.getExclusionPatterns().equals(this.getExclusionPatterns()) == false)
return false;
if (other.getProxyConfiguration() == null ^ this.getProxyConfiguration() == null)
return false;
if (other.getProxyConfiguration() != null && other.getProxyConfiguration().equals(this.getProxyConfiguration()) == false)
return false;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServerUrl() == null) ? 0 : getServerUrl().hashCode());
hashCode = prime * hashCode + ((getSecretArn() == null) ? 0 : getSecretArn().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode + ((getSpaceConfiguration() == null) ? 0 : getSpaceConfiguration().hashCode());
hashCode = prime * hashCode + ((getPageConfiguration() == null) ? 0 : getPageConfiguration().hashCode());
hashCode = prime * hashCode + ((getBlogConfiguration() == null) ? 0 : getBlogConfiguration().hashCode());
hashCode = prime * hashCode + ((getAttachmentConfiguration() == null) ? 0 : getAttachmentConfiguration().hashCode());
hashCode = prime * hashCode + ((getVpcConfiguration() == null) ? 0 : getVpcConfiguration().hashCode());
hashCode = prime * hashCode + ((getInclusionPatterns() == null) ? 0 : getInclusionPatterns().hashCode());
hashCode = prime * hashCode + ((getExclusionPatterns() == null) ? 0 : getExclusionPatterns().hashCode());
hashCode = prime * hashCode + ((getProxyConfiguration() == null) ? 0 : getProxyConfiguration().hashCode());
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
return hashCode;
}
@Override
public ConfluenceConfiguration clone() {
try {
return (ConfluenceConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.ConfluenceConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}