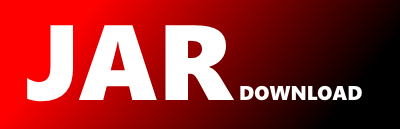
com.amazonaws.services.kendra.model.DocumentAttributeValueCountPair Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides the count of documents that match a particular document attribute or field when doing a faceted search.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DocumentAttributeValueCountPair implements Serializable, Cloneable, StructuredPojo {
/**
*
* The value of the attribute/field. For example, "HR".
*
*/
private DocumentAttributeValue documentAttributeValue;
/**
*
* The number of documents in the response that have the attribute/field value for the key.
*
*/
private Integer count;
/**
*
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
contains
* the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In addition, the
* document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for documents assigned
* to "Engineering". You can display nested facets in the search results so that documents can be searched not only
* by department but also by a sub department within a department. The counts for documents that belong to
* "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
*
*/
private java.util.List facetResults;
/**
*
* The value of the attribute/field. For example, "HR".
*
*
* @param documentAttributeValue
* The value of the attribute/field. For example, "HR".
*/
public void setDocumentAttributeValue(DocumentAttributeValue documentAttributeValue) {
this.documentAttributeValue = documentAttributeValue;
}
/**
*
* The value of the attribute/field. For example, "HR".
*
*
* @return The value of the attribute/field. For example, "HR".
*/
public DocumentAttributeValue getDocumentAttributeValue() {
return this.documentAttributeValue;
}
/**
*
* The value of the attribute/field. For example, "HR".
*
*
* @param documentAttributeValue
* The value of the attribute/field. For example, "HR".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DocumentAttributeValueCountPair withDocumentAttributeValue(DocumentAttributeValue documentAttributeValue) {
setDocumentAttributeValue(documentAttributeValue);
return this;
}
/**
*
* The number of documents in the response that have the attribute/field value for the key.
*
*
* @param count
* The number of documents in the response that have the attribute/field value for the key.
*/
public void setCount(Integer count) {
this.count = count;
}
/**
*
* The number of documents in the response that have the attribute/field value for the key.
*
*
* @return The number of documents in the response that have the attribute/field value for the key.
*/
public Integer getCount() {
return this.count;
}
/**
*
* The number of documents in the response that have the attribute/field value for the key.
*
*
* @param count
* The number of documents in the response that have the attribute/field value for the key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DocumentAttributeValueCountPair withCount(Integer count) {
setCount(count);
return this;
}
/**
*
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
contains
* the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In addition, the
* document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for documents assigned
* to "Engineering". You can display nested facets in the search results so that documents can be searched not only
* by department but also by a sub department within a department. The counts for documents that belong to
* "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
*
*
* @return Contains the results of a document attribute/field that is a nested facet. A FacetResult
* contains the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In
* addition, the document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend"
* for documents assigned to "Engineering". You can display nested facets in the search results so that
* documents can be searched not only by department but also by a sub department within a department. The
* counts for documents that belong to "Frontend" and "Backend" within "Engineering" are returned for a
* query.
*
*
*/
public java.util.List getFacetResults() {
return facetResults;
}
/**
*
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
contains
* the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In addition, the
* document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for documents assigned
* to "Engineering". You can display nested facets in the search results so that documents can be searched not only
* by department but also by a sub department within a department. The counts for documents that belong to
* "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
*
*
* @param facetResults
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
* contains the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In
* addition, the document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for
* documents assigned to "Engineering". You can display nested facets in the search results so that documents
* can be searched not only by department but also by a sub department within a department. The counts for
* documents that belong to "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
*/
public void setFacetResults(java.util.Collection facetResults) {
if (facetResults == null) {
this.facetResults = null;
return;
}
this.facetResults = new java.util.ArrayList(facetResults);
}
/**
*
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
contains
* the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In addition, the
* document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for documents assigned
* to "Engineering". You can display nested facets in the search results so that documents can be searched not only
* by department but also by a sub department within a department. The counts for documents that belong to
* "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFacetResults(java.util.Collection)} or {@link #withFacetResults(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param facetResults
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
* contains the counts for each facet nested within a facet.
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In
* addition, the document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for
* documents assigned to "Engineering". You can display nested facets in the search results so that documents
* can be searched not only by department but also by a sub department within a department. The counts for
* documents that belong to "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DocumentAttributeValueCountPair withFacetResults(FacetResult... facetResults) {
if (this.facetResults == null) {
setFacetResults(new java.util.ArrayList(facetResults.length));
}
for (FacetResult ele : facetResults) {
this.facetResults.add(ele);
}
return this;
}
/**
*
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
contains
* the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In addition, the
* document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for documents assigned
* to "Engineering". You can display nested facets in the search results so that documents can be searched not only
* by department but also by a sub department within a department. The counts for documents that belong to
* "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
*
*
* @param facetResults
* Contains the results of a document attribute/field that is a nested facet. A FacetResult
* contains the counts for each facet nested within a facet.
*
*
* For example, the document attribute or facet "Department" includes a value called "Engineering". In
* addition, the document attribute or facet "SubDepartment" includes the values "Frontend" and "Backend" for
* documents assigned to "Engineering". You can display nested facets in the search results so that documents
* can be searched not only by department but also by a sub department within a department. The counts for
* documents that belong to "Frontend" and "Backend" within "Engineering" are returned for a query.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DocumentAttributeValueCountPair withFacetResults(java.util.Collection facetResults) {
setFacetResults(facetResults);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDocumentAttributeValue() != null)
sb.append("DocumentAttributeValue: ").append(getDocumentAttributeValue()).append(",");
if (getCount() != null)
sb.append("Count: ").append(getCount()).append(",");
if (getFacetResults() != null)
sb.append("FacetResults: ").append(getFacetResults());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DocumentAttributeValueCountPair == false)
return false;
DocumentAttributeValueCountPair other = (DocumentAttributeValueCountPair) obj;
if (other.getDocumentAttributeValue() == null ^ this.getDocumentAttributeValue() == null)
return false;
if (other.getDocumentAttributeValue() != null && other.getDocumentAttributeValue().equals(this.getDocumentAttributeValue()) == false)
return false;
if (other.getCount() == null ^ this.getCount() == null)
return false;
if (other.getCount() != null && other.getCount().equals(this.getCount()) == false)
return false;
if (other.getFacetResults() == null ^ this.getFacetResults() == null)
return false;
if (other.getFacetResults() != null && other.getFacetResults().equals(this.getFacetResults()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDocumentAttributeValue() == null) ? 0 : getDocumentAttributeValue().hashCode());
hashCode = prime * hashCode + ((getCount() == null) ? 0 : getCount().hashCode());
hashCode = prime * hashCode + ((getFacetResults() == null) ? 0 : getFacetResults().hashCode());
return hashCode;
}
@Override
public DocumentAttributeValueCountPair clone() {
try {
return (DocumentAttributeValueCountPair) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.DocumentAttributeValueCountPairMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}