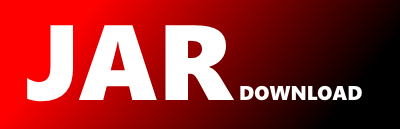
com.amazonaws.services.kendra.model.FsxConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides the configuration information to connect to Amazon FSx as your data source.
*
*
*
* Amazon Kendra now supports an upgraded Amazon FSx Windows connector.
*
*
* You must now use the TemplateConfiguration
* object instead of the FsxConfiguration
object to configure your connector.
*
*
* Connectors configured using the older console and API architecture will continue to function as configured. However,
* you won't be able to edit or update them. If you want to edit or update your connector configuration, you must create
* a new connector.
*
*
* We recommended migrating your connector workflow to the upgraded version. Support for connectors configured using the
* older architecture is scheduled to end by June 2024.
*
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FsxConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The identifier of the Amazon FSx file system.
*
*
* You can find your file system ID on the file system dashboard in the Amazon FSx console. For information on how
* to create a file system in Amazon FSx console, using Windows File Server as an example, see Amazon FSx Getting started
* guide.
*
*/
private String fileSystemId;
/**
*
* The Amazon FSx file system type. Windows is currently the only supported type.
*
*/
private String fileSystemType;
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Amazon FSx. Your Amazon FSx
* instance must reside inside your VPC.
*
*/
private DataSourceVpcConfiguration vpcConfiguration;
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the key-value pairs required to connect
* to your Amazon FSx file system. Windows is currently the only supported type. The secret must contain a JSON
* structure with the following keys:
*
*
* -
*
* username—The Active Directory user name, along with the Domain Name System (DNS) domain name. For example,
* [email protected]. The Active Directory user account must have read and mounting access to the Amazon
* FSx file system for Windows.
*
*
* -
*
* password—The password of the Active Directory user account with read and mounting access to the Amazon FSx
* Windows file system.
*
*
*
*/
private String secretArn;
/**
*
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that match
* the patterns are included in the index. Files that don't match the patterns are excluded from the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*/
private java.util.List inclusionPatterns;
/**
*
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that match
* the patterns are excluded from the index. Files that don't match the patterns are included in the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*/
private java.util.List exclusionPatterns;
/**
*
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or field
* names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
API before
* you map to Amazon FSx fields. For more information, see Mapping data source fields. The Amazon
* FSx data source field names must exist in your Amazon FSx custom metadata.
*
*/
private java.util.List fieldMappings;
/**
*
* The identifier of the Amazon FSx file system.
*
*
* You can find your file system ID on the file system dashboard in the Amazon FSx console. For information on how
* to create a file system in Amazon FSx console, using Windows File Server as an example, see Amazon FSx Getting started
* guide.
*
*
* @param fileSystemId
* The identifier of the Amazon FSx file system.
*
* You can find your file system ID on the file system dashboard in the Amazon FSx console. For information
* on how to create a file system in Amazon FSx console, using Windows File Server as an example, see Amazon FSx Getting
* started guide.
*/
public void setFileSystemId(String fileSystemId) {
this.fileSystemId = fileSystemId;
}
/**
*
* The identifier of the Amazon FSx file system.
*
*
* You can find your file system ID on the file system dashboard in the Amazon FSx console. For information on how
* to create a file system in Amazon FSx console, using Windows File Server as an example, see Amazon FSx Getting started
* guide.
*
*
* @return The identifier of the Amazon FSx file system.
*
* You can find your file system ID on the file system dashboard in the Amazon FSx console. For information
* on how to create a file system in Amazon FSx console, using Windows File Server as an example, see Amazon FSx Getting
* started guide.
*/
public String getFileSystemId() {
return this.fileSystemId;
}
/**
*
* The identifier of the Amazon FSx file system.
*
*
* You can find your file system ID on the file system dashboard in the Amazon FSx console. For information on how
* to create a file system in Amazon FSx console, using Windows File Server as an example, see Amazon FSx Getting started
* guide.
*
*
* @param fileSystemId
* The identifier of the Amazon FSx file system.
*
* You can find your file system ID on the file system dashboard in the Amazon FSx console. For information
* on how to create a file system in Amazon FSx console, using Windows File Server as an example, see Amazon FSx Getting
* started guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withFileSystemId(String fileSystemId) {
setFileSystemId(fileSystemId);
return this;
}
/**
*
* The Amazon FSx file system type. Windows is currently the only supported type.
*
*
* @param fileSystemType
* The Amazon FSx file system type. Windows is currently the only supported type.
* @see FsxFileSystemType
*/
public void setFileSystemType(String fileSystemType) {
this.fileSystemType = fileSystemType;
}
/**
*
* The Amazon FSx file system type. Windows is currently the only supported type.
*
*
* @return The Amazon FSx file system type. Windows is currently the only supported type.
* @see FsxFileSystemType
*/
public String getFileSystemType() {
return this.fileSystemType;
}
/**
*
* The Amazon FSx file system type. Windows is currently the only supported type.
*
*
* @param fileSystemType
* The Amazon FSx file system type. Windows is currently the only supported type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FsxFileSystemType
*/
public FsxConfiguration withFileSystemType(String fileSystemType) {
setFileSystemType(fileSystemType);
return this;
}
/**
*
* The Amazon FSx file system type. Windows is currently the only supported type.
*
*
* @param fileSystemType
* The Amazon FSx file system type. Windows is currently the only supported type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FsxFileSystemType
*/
public FsxConfiguration withFileSystemType(FsxFileSystemType fileSystemType) {
this.fileSystemType = fileSystemType.toString();
return this;
}
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Amazon FSx. Your Amazon FSx
* instance must reside inside your VPC.
*
*
* @param vpcConfiguration
* Configuration information for an Amazon Virtual Private Cloud to connect to your Amazon FSx. Your Amazon
* FSx instance must reside inside your VPC.
*/
public void setVpcConfiguration(DataSourceVpcConfiguration vpcConfiguration) {
this.vpcConfiguration = vpcConfiguration;
}
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Amazon FSx. Your Amazon FSx
* instance must reside inside your VPC.
*
*
* @return Configuration information for an Amazon Virtual Private Cloud to connect to your Amazon FSx. Your Amazon
* FSx instance must reside inside your VPC.
*/
public DataSourceVpcConfiguration getVpcConfiguration() {
return this.vpcConfiguration;
}
/**
*
* Configuration information for an Amazon Virtual Private Cloud to connect to your Amazon FSx. Your Amazon FSx
* instance must reside inside your VPC.
*
*
* @param vpcConfiguration
* Configuration information for an Amazon Virtual Private Cloud to connect to your Amazon FSx. Your Amazon
* FSx instance must reside inside your VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withVpcConfiguration(DataSourceVpcConfiguration vpcConfiguration) {
setVpcConfiguration(vpcConfiguration);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the key-value pairs required to connect
* to your Amazon FSx file system. Windows is currently the only supported type. The secret must contain a JSON
* structure with the following keys:
*
*
* -
*
* username—The Active Directory user name, along with the Domain Name System (DNS) domain name. For example,
* [email protected]. The Active Directory user account must have read and mounting access to the Amazon
* FSx file system for Windows.
*
*
* -
*
* password—The password of the Active Directory user account with read and mounting access to the Amazon FSx
* Windows file system.
*
*
*
*
* @param secretArn
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the key-value pairs required to
* connect to your Amazon FSx file system. Windows is currently the only supported type. The secret must
* contain a JSON structure with the following keys:
*
* -
*
* username—The Active Directory user name, along with the Domain Name System (DNS) domain name. For example,
* [email protected]. The Active Directory user account must have read and mounting access to the
* Amazon FSx file system for Windows.
*
*
* -
*
* password—The password of the Active Directory user account with read and mounting access to the Amazon FSx
* Windows file system.
*
*
*/
public void setSecretArn(String secretArn) {
this.secretArn = secretArn;
}
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the key-value pairs required to connect
* to your Amazon FSx file system. Windows is currently the only supported type. The secret must contain a JSON
* structure with the following keys:
*
*
* -
*
* username—The Active Directory user name, along with the Domain Name System (DNS) domain name. For example,
* [email protected]. The Active Directory user account must have read and mounting access to the Amazon
* FSx file system for Windows.
*
*
* -
*
* password—The password of the Active Directory user account with read and mounting access to the Amazon FSx
* Windows file system.
*
*
*
*
* @return The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the key-value pairs required to
* connect to your Amazon FSx file system. Windows is currently the only supported type. The secret must
* contain a JSON structure with the following keys:
*
* -
*
* username—The Active Directory user name, along with the Domain Name System (DNS) domain name. For
* example, [email protected]. The Active Directory user account must have read and mounting
* access to the Amazon FSx file system for Windows.
*
*
* -
*
* password—The password of the Active Directory user account with read and mounting access to the Amazon
* FSx Windows file system.
*
*
*/
public String getSecretArn() {
return this.secretArn;
}
/**
*
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the key-value pairs required to connect
* to your Amazon FSx file system. Windows is currently the only supported type. The secret must contain a JSON
* structure with the following keys:
*
*
* -
*
* username—The Active Directory user name, along with the Domain Name System (DNS) domain name. For example,
* [email protected]. The Active Directory user account must have read and mounting access to the Amazon
* FSx file system for Windows.
*
*
* -
*
* password—The password of the Active Directory user account with read and mounting access to the Amazon FSx
* Windows file system.
*
*
*
*
* @param secretArn
* The Amazon Resource Name (ARN) of an Secrets Manager secret that contains the key-value pairs required to
* connect to your Amazon FSx file system. Windows is currently the only supported type. The secret must
* contain a JSON structure with the following keys:
*
* -
*
* username—The Active Directory user name, along with the Domain Name System (DNS) domain name. For example,
* [email protected]. The Active Directory user account must have read and mounting access to the
* Amazon FSx file system for Windows.
*
*
* -
*
* password—The password of the Active Directory user account with read and mounting access to the Amazon FSx
* Windows file system.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withSecretArn(String secretArn) {
setSecretArn(secretArn);
return this;
}
/**
*
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that match
* the patterns are included in the index. Files that don't match the patterns are excluded from the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* @return A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that
* match the patterns are included in the index. Files that don't match the patterns are excluded from the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
*/
public java.util.List getInclusionPatterns() {
return inclusionPatterns;
}
/**
*
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that match
* the patterns are included in the index. Files that don't match the patterns are excluded from the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* @param inclusionPatterns
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that
* match the patterns are included in the index. Files that don't match the patterns are excluded from the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
*/
public void setInclusionPatterns(java.util.Collection inclusionPatterns) {
if (inclusionPatterns == null) {
this.inclusionPatterns = null;
return;
}
this.inclusionPatterns = new java.util.ArrayList(inclusionPatterns);
}
/**
*
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that match
* the patterns are included in the index. Files that don't match the patterns are excluded from the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInclusionPatterns(java.util.Collection)} or {@link #withInclusionPatterns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param inclusionPatterns
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that
* match the patterns are included in the index. Files that don't match the patterns are excluded from the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withInclusionPatterns(String... inclusionPatterns) {
if (this.inclusionPatterns == null) {
setInclusionPatterns(new java.util.ArrayList(inclusionPatterns.length));
}
for (String ele : inclusionPatterns) {
this.inclusionPatterns.add(ele);
}
return this;
}
/**
*
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that match
* the patterns are included in the index. Files that don't match the patterns are excluded from the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* @param inclusionPatterns
* A list of regular expression patterns to include certain files in your Amazon FSx file system. Files that
* match the patterns are included in the index. Files that don't match the patterns are excluded from the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withInclusionPatterns(java.util.Collection inclusionPatterns) {
setInclusionPatterns(inclusionPatterns);
return this;
}
/**
*
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that match
* the patterns are excluded from the index. Files that don't match the patterns are included in the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* @return A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that
* match the patterns are excluded from the index. Files that don't match the patterns are included in the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
*/
public java.util.List getExclusionPatterns() {
return exclusionPatterns;
}
/**
*
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that match
* the patterns are excluded from the index. Files that don't match the patterns are included in the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* @param exclusionPatterns
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that
* match the patterns are excluded from the index. Files that don't match the patterns are included in the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
*/
public void setExclusionPatterns(java.util.Collection exclusionPatterns) {
if (exclusionPatterns == null) {
this.exclusionPatterns = null;
return;
}
this.exclusionPatterns = new java.util.ArrayList(exclusionPatterns);
}
/**
*
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that match
* the patterns are excluded from the index. Files that don't match the patterns are included in the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExclusionPatterns(java.util.Collection)} or {@link #withExclusionPatterns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param exclusionPatterns
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that
* match the patterns are excluded from the index. Files that don't match the patterns are included in the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withExclusionPatterns(String... exclusionPatterns) {
if (this.exclusionPatterns == null) {
setExclusionPatterns(new java.util.ArrayList(exclusionPatterns.length));
}
for (String ele : exclusionPatterns) {
this.exclusionPatterns.add(ele);
}
return this;
}
/**
*
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that match
* the patterns are excluded from the index. Files that don't match the patterns are included in the index. If a
* file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the file isn't
* included in the index.
*
*
* @param exclusionPatterns
* A list of regular expression patterns to exclude certain files in your Amazon FSx file system. Files that
* match the patterns are excluded from the index. Files that don't match the patterns are included in the
* index. If a file matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the file isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withExclusionPatterns(java.util.Collection exclusionPatterns) {
setExclusionPatterns(exclusionPatterns);
return this;
}
/**
*
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or field
* names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
API before
* you map to Amazon FSx fields. For more information, see Mapping data source fields. The Amazon
* FSx data source field names must exist in your Amazon FSx custom metadata.
*
*
* @return A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes
* or field names to Amazon Kendra index field names. To create custom fields, use the
* UpdateIndex
API before you map to Amazon FSx fields. For more information, see Mapping data source fields.
* The Amazon FSx data source field names must exist in your Amazon FSx custom metadata.
*/
public java.util.List getFieldMappings() {
return fieldMappings;
}
/**
*
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or field
* names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
API before
* you map to Amazon FSx fields. For more information, see Mapping data source fields. The Amazon
* FSx data source field names must exist in your Amazon FSx custom metadata.
*
*
* @param fieldMappings
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or
* field names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
* API before you map to Amazon FSx fields. For more information, see Mapping data source fields. The
* Amazon FSx data source field names must exist in your Amazon FSx custom metadata.
*/
public void setFieldMappings(java.util.Collection fieldMappings) {
if (fieldMappings == null) {
this.fieldMappings = null;
return;
}
this.fieldMappings = new java.util.ArrayList(fieldMappings);
}
/**
*
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or field
* names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
API before
* you map to Amazon FSx fields. For more information, see Mapping data source fields. The Amazon
* FSx data source field names must exist in your Amazon FSx custom metadata.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFieldMappings(java.util.Collection)} or {@link #withFieldMappings(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param fieldMappings
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or
* field names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
* API before you map to Amazon FSx fields. For more information, see Mapping data source fields. The
* Amazon FSx data source field names must exist in your Amazon FSx custom metadata.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withFieldMappings(DataSourceToIndexFieldMapping... fieldMappings) {
if (this.fieldMappings == null) {
setFieldMappings(new java.util.ArrayList(fieldMappings.length));
}
for (DataSourceToIndexFieldMapping ele : fieldMappings) {
this.fieldMappings.add(ele);
}
return this;
}
/**
*
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or field
* names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
API before
* you map to Amazon FSx fields. For more information, see Mapping data source fields. The Amazon
* FSx data source field names must exist in your Amazon FSx custom metadata.
*
*
* @param fieldMappings
* A list of DataSourceToIndexFieldMapping
objects that map Amazon FSx data source attributes or
* field names to Amazon Kendra index field names. To create custom fields, use the UpdateIndex
* API before you map to Amazon FSx fields. For more information, see Mapping data source fields. The
* Amazon FSx data source field names must exist in your Amazon FSx custom metadata.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FsxConfiguration withFieldMappings(java.util.Collection fieldMappings) {
setFieldMappings(fieldMappings);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFileSystemId() != null)
sb.append("FileSystemId: ").append(getFileSystemId()).append(",");
if (getFileSystemType() != null)
sb.append("FileSystemType: ").append(getFileSystemType()).append(",");
if (getVpcConfiguration() != null)
sb.append("VpcConfiguration: ").append(getVpcConfiguration()).append(",");
if (getSecretArn() != null)
sb.append("SecretArn: ").append(getSecretArn()).append(",");
if (getInclusionPatterns() != null)
sb.append("InclusionPatterns: ").append(getInclusionPatterns()).append(",");
if (getExclusionPatterns() != null)
sb.append("ExclusionPatterns: ").append(getExclusionPatterns()).append(",");
if (getFieldMappings() != null)
sb.append("FieldMappings: ").append(getFieldMappings());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FsxConfiguration == false)
return false;
FsxConfiguration other = (FsxConfiguration) obj;
if (other.getFileSystemId() == null ^ this.getFileSystemId() == null)
return false;
if (other.getFileSystemId() != null && other.getFileSystemId().equals(this.getFileSystemId()) == false)
return false;
if (other.getFileSystemType() == null ^ this.getFileSystemType() == null)
return false;
if (other.getFileSystemType() != null && other.getFileSystemType().equals(this.getFileSystemType()) == false)
return false;
if (other.getVpcConfiguration() == null ^ this.getVpcConfiguration() == null)
return false;
if (other.getVpcConfiguration() != null && other.getVpcConfiguration().equals(this.getVpcConfiguration()) == false)
return false;
if (other.getSecretArn() == null ^ this.getSecretArn() == null)
return false;
if (other.getSecretArn() != null && other.getSecretArn().equals(this.getSecretArn()) == false)
return false;
if (other.getInclusionPatterns() == null ^ this.getInclusionPatterns() == null)
return false;
if (other.getInclusionPatterns() != null && other.getInclusionPatterns().equals(this.getInclusionPatterns()) == false)
return false;
if (other.getExclusionPatterns() == null ^ this.getExclusionPatterns() == null)
return false;
if (other.getExclusionPatterns() != null && other.getExclusionPatterns().equals(this.getExclusionPatterns()) == false)
return false;
if (other.getFieldMappings() == null ^ this.getFieldMappings() == null)
return false;
if (other.getFieldMappings() != null && other.getFieldMappings().equals(this.getFieldMappings()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFileSystemId() == null) ? 0 : getFileSystemId().hashCode());
hashCode = prime * hashCode + ((getFileSystemType() == null) ? 0 : getFileSystemType().hashCode());
hashCode = prime * hashCode + ((getVpcConfiguration() == null) ? 0 : getVpcConfiguration().hashCode());
hashCode = prime * hashCode + ((getSecretArn() == null) ? 0 : getSecretArn().hashCode());
hashCode = prime * hashCode + ((getInclusionPatterns() == null) ? 0 : getInclusionPatterns().hashCode());
hashCode = prime * hashCode + ((getExclusionPatterns() == null) ? 0 : getExclusionPatterns().hashCode());
hashCode = prime * hashCode + ((getFieldMappings() == null) ? 0 : getFieldMappings().hashCode());
return hashCode;
}
@Override
public FsxConfiguration clone() {
try {
return (FsxConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.FsxConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}