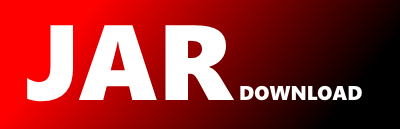
com.amazonaws.services.kendra.model.GetSnapshotsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetSnapshotsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The identifier of the index to get search metrics data.
*
*/
private String indexId;
/**
*
* The time interval or time window to get search metrics data. The time interval uses the time zone of your index.
* You can view data in the following time windows:
*
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the following
* Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day before
* the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the last day
* of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month and
* ending on last day of the month.
*
*
*
*/
private String interval;
/**
*
* The metric you want to retrieve. You can specify only one metric per call.
*
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
*
*/
private String metricType;
/**
*
* If the previous response was incomplete (because there is more data to retrieve), Amazon Kendra returns a
* pagination token in the response. You can use this pagination token to retrieve the next set of search metrics
* data.
*
*/
private String nextToken;
/**
*
* The maximum number of returned data for the metric.
*
*/
private Integer maxResults;
/**
*
* The identifier of the index to get search metrics data.
*
*
* @param indexId
* The identifier of the index to get search metrics data.
*/
public void setIndexId(String indexId) {
this.indexId = indexId;
}
/**
*
* The identifier of the index to get search metrics data.
*
*
* @return The identifier of the index to get search metrics data.
*/
public String getIndexId() {
return this.indexId;
}
/**
*
* The identifier of the index to get search metrics data.
*
*
* @param indexId
* The identifier of the index to get search metrics data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSnapshotsRequest withIndexId(String indexId) {
setIndexId(indexId);
return this;
}
/**
*
* The time interval or time window to get search metrics data. The time interval uses the time zone of your index.
* You can view data in the following time windows:
*
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the following
* Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day before
* the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the last day
* of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month and
* ending on last day of the month.
*
*
*
*
* @param interval
* The time interval or time window to get search metrics data. The time interval uses the time zone of your
* index. You can view data in the following time windows:
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current
* date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the
* following Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day
* before the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the
* last day of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month
* and ending on last day of the month.
*
*
* @see Interval
*/
public void setInterval(String interval) {
this.interval = interval;
}
/**
*
* The time interval or time window to get search metrics data. The time interval uses the time zone of your index.
* You can view data in the following time windows:
*
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the following
* Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day before
* the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the last day
* of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month and
* ending on last day of the month.
*
*
*
*
* @return The time interval or time window to get search metrics data. The time interval uses the time zone of your
* index. You can view data in the following time windows:
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current
* date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following
* Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the
* following Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day
* before the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the
* last day of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month
* and ending on last day of the month.
*
*
* @see Interval
*/
public String getInterval() {
return this.interval;
}
/**
*
* The time interval or time window to get search metrics data. The time interval uses the time zone of your index.
* You can view data in the following time windows:
*
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the following
* Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day before
* the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the last day
* of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month and
* ending on last day of the month.
*
*
*
*
* @param interval
* The time interval or time window to get search metrics data. The time interval uses the time zone of your
* index. You can view data in the following time windows:
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current
* date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the
* following Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day
* before the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the
* last day of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month
* and ending on last day of the month.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Interval
*/
public GetSnapshotsRequest withInterval(String interval) {
setInterval(interval);
return this;
}
/**
*
* The time interval or time window to get search metrics data. The time interval uses the time zone of your index.
* You can view data in the following time windows:
*
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the following
* Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day before
* the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the last day
* of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month and
* ending on last day of the month.
*
*
*
*
* @param interval
* The time interval or time window to get search metrics data. The time interval uses the time zone of your
* index. You can view data in the following time windows:
*
* -
*
* THIS_WEEK
: The current week, starting on the Sunday and ending on the day before the current
* date.
*
*
* -
*
* ONE_WEEK_AGO
: The previous week, starting on the Sunday and ending on the following Saturday.
*
*
* -
*
* TWO_WEEKS_AGO
: The week before the previous week, starting on the Sunday and ending on the
* following Saturday.
*
*
* -
*
* THIS_MONTH
: The current month, starting on the first day of the month and ending on the day
* before the current date.
*
*
* -
*
* ONE_MONTH_AGO
: The previous month, starting on the first day of the month and ending on the
* last day of the month.
*
*
* -
*
* TWO_MONTHS_AGO
: The month before the previous month, starting on the first day of the month
* and ending on last day of the month.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Interval
*/
public GetSnapshotsRequest withInterval(Interval interval) {
this.interval = interval.toString();
return this;
}
/**
*
* The metric you want to retrieve. You can specify only one metric per call.
*
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
*
*
* @param metricType
* The metric you want to retrieve. You can specify only one metric per call.
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
* @see MetricType
*/
public void setMetricType(String metricType) {
this.metricType = metricType;
}
/**
*
* The metric you want to retrieve. You can specify only one metric per call.
*
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
*
*
* @return The metric you want to retrieve. You can specify only one metric per call.
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
* @see MetricType
*/
public String getMetricType() {
return this.metricType;
}
/**
*
* The metric you want to retrieve. You can specify only one metric per call.
*
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
*
*
* @param metricType
* The metric you want to retrieve. You can specify only one metric per call.
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MetricType
*/
public GetSnapshotsRequest withMetricType(String metricType) {
setMetricType(metricType);
return this;
}
/**
*
* The metric you want to retrieve. You can specify only one metric per call.
*
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
*
*
* @param metricType
* The metric you want to retrieve. You can specify only one metric per call.
*
* For more information about the metrics you can view, see Gaining insights with search
* analytics.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MetricType
*/
public GetSnapshotsRequest withMetricType(MetricType metricType) {
this.metricType = metricType.toString();
return this;
}
/**
*
* If the previous response was incomplete (because there is more data to retrieve), Amazon Kendra returns a
* pagination token in the response. You can use this pagination token to retrieve the next set of search metrics
* data.
*
*
* @param nextToken
* If the previous response was incomplete (because there is more data to retrieve), Amazon Kendra returns a
* pagination token in the response. You can use this pagination token to retrieve the next set of search
* metrics data.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* If the previous response was incomplete (because there is more data to retrieve), Amazon Kendra returns a
* pagination token in the response. You can use this pagination token to retrieve the next set of search metrics
* data.
*
*
* @return If the previous response was incomplete (because there is more data to retrieve), Amazon Kendra returns a
* pagination token in the response. You can use this pagination token to retrieve the next set of search
* metrics data.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* If the previous response was incomplete (because there is more data to retrieve), Amazon Kendra returns a
* pagination token in the response. You can use this pagination token to retrieve the next set of search metrics
* data.
*
*
* @param nextToken
* If the previous response was incomplete (because there is more data to retrieve), Amazon Kendra returns a
* pagination token in the response. You can use this pagination token to retrieve the next set of search
* metrics data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSnapshotsRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* The maximum number of returned data for the metric.
*
*
* @param maxResults
* The maximum number of returned data for the metric.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of returned data for the metric.
*
*
* @return The maximum number of returned data for the metric.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of returned data for the metric.
*
*
* @param maxResults
* The maximum number of returned data for the metric.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSnapshotsRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getIndexId() != null)
sb.append("IndexId: ").append(getIndexId()).append(",");
if (getInterval() != null)
sb.append("Interval: ").append(getInterval()).append(",");
if (getMetricType() != null)
sb.append("MetricType: ").append(getMetricType()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetSnapshotsRequest == false)
return false;
GetSnapshotsRequest other = (GetSnapshotsRequest) obj;
if (other.getIndexId() == null ^ this.getIndexId() == null)
return false;
if (other.getIndexId() != null && other.getIndexId().equals(this.getIndexId()) == false)
return false;
if (other.getInterval() == null ^ this.getInterval() == null)
return false;
if (other.getInterval() != null && other.getInterval().equals(this.getInterval()) == false)
return false;
if (other.getMetricType() == null ^ this.getMetricType() == null)
return false;
if (other.getMetricType() != null && other.getMetricType().equals(this.getMetricType()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getIndexId() == null) ? 0 : getIndexId().hashCode());
hashCode = prime * hashCode + ((getInterval() == null) ? 0 : getInterval().hashCode());
hashCode = prime * hashCode + ((getMetricType() == null) ? 0 : getMetricType().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
return hashCode;
}
@Override
public GetSnapshotsRequest clone() {
return (GetSnapshotsRequest) super.clone();
}
}