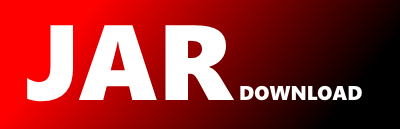
com.amazonaws.services.kendra.model.GroupMembers Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A list of users or sub groups that belong to a group. This is useful for user context filtering, where search results
* are filtered based on the user or their group access to documents.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GroupMembers implements Serializable, Cloneable, StructuredPojo {
/**
*
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
*
*/
private java.util.List memberGroups;
/**
*
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
*
*/
private java.util.List memberUsers;
/**
*
* If you have more than 1000 users and/or sub groups for a single group, you need to provide the path to the S3
* file that lists your users and sub groups for a group. Your sub groups can contain more than 1000 users, but the
* list of sub groups that belong to a group (and/or users) must be no more than 1000.
*
*
* You can download this example S3
* file that uses the correct format for listing group members. Note, dataSourceId
is optional. The
* value of type
for a group is always GROUP
and for a user it is always USER
* .
*
*/
private S3Path s3PathforGroupMembers;
/**
*
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
*
*
* @return A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
*/
public java.util.List getMemberGroups() {
return memberGroups;
}
/**
*
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
*
*
* @param memberGroups
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
*/
public void setMemberGroups(java.util.Collection memberGroups) {
if (memberGroups == null) {
this.memberGroups = null;
return;
}
this.memberGroups = new java.util.ArrayList(memberGroups);
}
/**
*
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMemberGroups(java.util.Collection)} or {@link #withMemberGroups(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param memberGroups
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GroupMembers withMemberGroups(MemberGroup... memberGroups) {
if (this.memberGroups == null) {
setMemberGroups(new java.util.ArrayList(memberGroups.length));
}
for (MemberGroup ele : memberGroups) {
this.memberGroups.add(ele);
}
return this;
}
/**
*
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
*
*
* @param memberGroups
* A list of sub groups that belong to a group. For example, the sub groups "Research", "Engineering", and
* "Sales and Marketing" all belong to the group "Company".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GroupMembers withMemberGroups(java.util.Collection memberGroups) {
setMemberGroups(memberGroups);
return this;
}
/**
*
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
*
*
* @return A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
*/
public java.util.List getMemberUsers() {
return memberUsers;
}
/**
*
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
*
*
* @param memberUsers
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
*/
public void setMemberUsers(java.util.Collection memberUsers) {
if (memberUsers == null) {
this.memberUsers = null;
return;
}
this.memberUsers = new java.util.ArrayList(memberUsers);
}
/**
*
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMemberUsers(java.util.Collection)} or {@link #withMemberUsers(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param memberUsers
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GroupMembers withMemberUsers(MemberUser... memberUsers) {
if (this.memberUsers == null) {
setMemberUsers(new java.util.ArrayList(memberUsers.length));
}
for (MemberUser ele : memberUsers) {
this.memberUsers.add(ele);
}
return this;
}
/**
*
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
*
*
* @param memberUsers
* A list of users that belong to a group. For example, a list of interns all belong to the "Interns" group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GroupMembers withMemberUsers(java.util.Collection memberUsers) {
setMemberUsers(memberUsers);
return this;
}
/**
*
* If you have more than 1000 users and/or sub groups for a single group, you need to provide the path to the S3
* file that lists your users and sub groups for a group. Your sub groups can contain more than 1000 users, but the
* list of sub groups that belong to a group (and/or users) must be no more than 1000.
*
*
* You can download this example S3
* file that uses the correct format for listing group members. Note, dataSourceId
is optional. The
* value of type
for a group is always GROUP
and for a user it is always USER
* .
*
*
* @param s3PathforGroupMembers
* If you have more than 1000 users and/or sub groups for a single group, you need to provide the path to the
* S3 file that lists your users and sub groups for a group. Your sub groups can contain more than 1000
* users, but the list of sub groups that belong to a group (and/or users) must be no more than 1000.
*
* You can download this example S3 file that
* uses the correct format for listing group members. Note, dataSourceId
is optional. The value
* of type
for a group is always GROUP
and for a user it is always
* USER
.
*/
public void setS3PathforGroupMembers(S3Path s3PathforGroupMembers) {
this.s3PathforGroupMembers = s3PathforGroupMembers;
}
/**
*
* If you have more than 1000 users and/or sub groups for a single group, you need to provide the path to the S3
* file that lists your users and sub groups for a group. Your sub groups can contain more than 1000 users, but the
* list of sub groups that belong to a group (and/or users) must be no more than 1000.
*
*
* You can download this example S3
* file that uses the correct format for listing group members. Note, dataSourceId
is optional. The
* value of type
for a group is always GROUP
and for a user it is always USER
* .
*
*
* @return If you have more than 1000 users and/or sub groups for a single group, you need to provide the path to
* the S3 file that lists your users and sub groups for a group. Your sub groups can contain more than 1000
* users, but the list of sub groups that belong to a group (and/or users) must be no more than 1000.
*
* You can download this example S3 file that
* uses the correct format for listing group members. Note, dataSourceId
is optional. The value
* of type
for a group is always GROUP
and for a user it is always
* USER
.
*/
public S3Path getS3PathforGroupMembers() {
return this.s3PathforGroupMembers;
}
/**
*
* If you have more than 1000 users and/or sub groups for a single group, you need to provide the path to the S3
* file that lists your users and sub groups for a group. Your sub groups can contain more than 1000 users, but the
* list of sub groups that belong to a group (and/or users) must be no more than 1000.
*
*
* You can download this example S3
* file that uses the correct format for listing group members. Note, dataSourceId
is optional. The
* value of type
for a group is always GROUP
and for a user it is always USER
* .
*
*
* @param s3PathforGroupMembers
* If you have more than 1000 users and/or sub groups for a single group, you need to provide the path to the
* S3 file that lists your users and sub groups for a group. Your sub groups can contain more than 1000
* users, but the list of sub groups that belong to a group (and/or users) must be no more than 1000.
*
* You can download this example S3 file that
* uses the correct format for listing group members. Note, dataSourceId
is optional. The value
* of type
for a group is always GROUP
and for a user it is always
* USER
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GroupMembers withS3PathforGroupMembers(S3Path s3PathforGroupMembers) {
setS3PathforGroupMembers(s3PathforGroupMembers);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMemberGroups() != null)
sb.append("MemberGroups: ").append(getMemberGroups()).append(",");
if (getMemberUsers() != null)
sb.append("MemberUsers: ").append(getMemberUsers()).append(",");
if (getS3PathforGroupMembers() != null)
sb.append("S3PathforGroupMembers: ").append(getS3PathforGroupMembers());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GroupMembers == false)
return false;
GroupMembers other = (GroupMembers) obj;
if (other.getMemberGroups() == null ^ this.getMemberGroups() == null)
return false;
if (other.getMemberGroups() != null && other.getMemberGroups().equals(this.getMemberGroups()) == false)
return false;
if (other.getMemberUsers() == null ^ this.getMemberUsers() == null)
return false;
if (other.getMemberUsers() != null && other.getMemberUsers().equals(this.getMemberUsers()) == false)
return false;
if (other.getS3PathforGroupMembers() == null ^ this.getS3PathforGroupMembers() == null)
return false;
if (other.getS3PathforGroupMembers() != null && other.getS3PathforGroupMembers().equals(this.getS3PathforGroupMembers()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMemberGroups() == null) ? 0 : getMemberGroups().hashCode());
hashCode = prime * hashCode + ((getMemberUsers() == null) ? 0 : getMemberUsers().hashCode());
hashCode = prime * hashCode + ((getS3PathforGroupMembers() == null) ? 0 : getS3PathforGroupMembers().hashCode());
return hashCode;
}
@Override
public GroupMembers clone() {
try {
return (GroupMembers) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.GroupMembersMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}