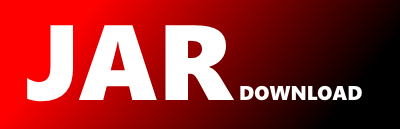
com.amazonaws.services.kendra.model.QueryResultItem Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A single query result.
*
*
* A query result contains information about a document returned by the query. This includes the original location of
* the document, a list of attributes assigned to the document, and relevant text from the document that satisfies the
* query.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class QueryResultItem implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique identifier for the query result item id (Id
) and the query result item document id (
* DocumentId
) combined. The value of this field changes with every request, even when you have the
* same documents.
*
*/
private String id;
/**
*
* The type of document within the response. For example, a response could include a question-answer that's relevant
* to the query.
*
*/
private String type;
/**
*
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned in
* TableExcerpt
. If it's a text answer, a text excerpt is returned in DocumentExcerpt
.
*
*/
private String format;
/**
*
* One or more additional fields/attributes associated with the query result.
*
*/
private java.util.List additionalAttributes;
/**
*
* The identifier for the document.
*
*/
private String documentId;
/**
*
* The title of the document. Contains the text of the title and information for highlighting the relevant terms in
* the title.
*
*/
private TextWithHighlights documentTitle;
/**
*
* An extract of the text in the document. Contains information about highlighting the relevant terms in the
* excerpt.
*
*/
private TextWithHighlights documentExcerpt;
/**
*
* The URI of the original location of the document.
*
*/
private String documentURI;
/**
*
* An array of document fields/attributes assigned to a document in the search results. For example, the document
* author (_author
) or the source URI (_source_uri
) of the document.
*
*/
private java.util.List documentAttributes;
/**
*
* Indicates the confidence level of Amazon Kendra providing a relevant result for the query. Each result is placed
* into a bin that indicates the confidence, VERY_HIGH
, HIGH
, MEDIUM
and
* LOW
. You can use the score to determine if a response meets the confidence needed for your
* application.
*
*
* The field is only set to LOW
when the Type
field is set to DOCUMENT
and
* Amazon Kendra is not confident that the result is relevant to the query.
*
*/
private ScoreAttributes scoreAttributes;
/**
*
* A token that identifies a particular result from a particular query. Use this token to provide click-through
* feedback for the result. For more information, see Submitting feedback.
*
*/
private String feedbackToken;
/**
*
* An excerpt from a table within a document.
*
*/
private TableExcerpt tableExcerpt;
/**
*
* Provides details about a collapsed group of search results.
*
*/
private CollapsedResultDetail collapsedResultDetail;
/**
*
* The unique identifier for the query result item id (Id
) and the query result item document id (
* DocumentId
) combined. The value of this field changes with every request, even when you have the
* same documents.
*
*
* @param id
* The unique identifier for the query result item id (Id
) and the query result item document id
* (DocumentId
) combined. The value of this field changes with every request, even when you have
* the same documents.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique identifier for the query result item id (Id
) and the query result item document id (
* DocumentId
) combined. The value of this field changes with every request, even when you have the
* same documents.
*
*
* @return The unique identifier for the query result item id (Id
) and the query result item document
* id (DocumentId
) combined. The value of this field changes with every request, even when you
* have the same documents.
*/
public String getId() {
return this.id;
}
/**
*
* The unique identifier for the query result item id (Id
) and the query result item document id (
* DocumentId
) combined. The value of this field changes with every request, even when you have the
* same documents.
*
*
* @param id
* The unique identifier for the query result item id (Id
) and the query result item document id
* (DocumentId
) combined. The value of this field changes with every request, even when you have
* the same documents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withId(String id) {
setId(id);
return this;
}
/**
*
* The type of document within the response. For example, a response could include a question-answer that's relevant
* to the query.
*
*
* @param type
* The type of document within the response. For example, a response could include a question-answer that's
* relevant to the query.
* @see QueryResultType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of document within the response. For example, a response could include a question-answer that's relevant
* to the query.
*
*
* @return The type of document within the response. For example, a response could include a question-answer that's
* relevant to the query.
* @see QueryResultType
*/
public String getType() {
return this.type;
}
/**
*
* The type of document within the response. For example, a response could include a question-answer that's relevant
* to the query.
*
*
* @param type
* The type of document within the response. For example, a response could include a question-answer that's
* relevant to the query.
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryResultType
*/
public QueryResultItem withType(String type) {
setType(type);
return this;
}
/**
*
* The type of document within the response. For example, a response could include a question-answer that's relevant
* to the query.
*
*
* @param type
* The type of document within the response. For example, a response could include a question-answer that's
* relevant to the query.
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryResultType
*/
public QueryResultItem withType(QueryResultType type) {
this.type = type.toString();
return this;
}
/**
*
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned in
* TableExcerpt
. If it's a text answer, a text excerpt is returned in DocumentExcerpt
.
*
*
* @param format
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned
* in TableExcerpt
. If it's a text answer, a text excerpt is returned in
* DocumentExcerpt
.
* @see QueryResultFormat
*/
public void setFormat(String format) {
this.format = format;
}
/**
*
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned in
* TableExcerpt
. If it's a text answer, a text excerpt is returned in DocumentExcerpt
.
*
*
* @return If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is
* returned in TableExcerpt
. If it's a text answer, a text excerpt is returned in
* DocumentExcerpt
.
* @see QueryResultFormat
*/
public String getFormat() {
return this.format;
}
/**
*
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned in
* TableExcerpt
. If it's a text answer, a text excerpt is returned in DocumentExcerpt
.
*
*
* @param format
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned
* in TableExcerpt
. If it's a text answer, a text excerpt is returned in
* DocumentExcerpt
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryResultFormat
*/
public QueryResultItem withFormat(String format) {
setFormat(format);
return this;
}
/**
*
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned in
* TableExcerpt
. If it's a text answer, a text excerpt is returned in DocumentExcerpt
.
*
*
* @param format
* If the Type
of document within the response is ANSWER
, then it is either a
* TABLE
answer or TEXT
answer. If it's a table answer, a table excerpt is returned
* in TableExcerpt
. If it's a text answer, a text excerpt is returned in
* DocumentExcerpt
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryResultFormat
*/
public QueryResultItem withFormat(QueryResultFormat format) {
this.format = format.toString();
return this;
}
/**
*
* One or more additional fields/attributes associated with the query result.
*
*
* @return One or more additional fields/attributes associated with the query result.
*/
public java.util.List getAdditionalAttributes() {
return additionalAttributes;
}
/**
*
* One or more additional fields/attributes associated with the query result.
*
*
* @param additionalAttributes
* One or more additional fields/attributes associated with the query result.
*/
public void setAdditionalAttributes(java.util.Collection additionalAttributes) {
if (additionalAttributes == null) {
this.additionalAttributes = null;
return;
}
this.additionalAttributes = new java.util.ArrayList(additionalAttributes);
}
/**
*
* One or more additional fields/attributes associated with the query result.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalAttributes(java.util.Collection)} or {@link #withAdditionalAttributes(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param additionalAttributes
* One or more additional fields/attributes associated with the query result.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withAdditionalAttributes(AdditionalResultAttribute... additionalAttributes) {
if (this.additionalAttributes == null) {
setAdditionalAttributes(new java.util.ArrayList(additionalAttributes.length));
}
for (AdditionalResultAttribute ele : additionalAttributes) {
this.additionalAttributes.add(ele);
}
return this;
}
/**
*
* One or more additional fields/attributes associated with the query result.
*
*
* @param additionalAttributes
* One or more additional fields/attributes associated with the query result.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withAdditionalAttributes(java.util.Collection additionalAttributes) {
setAdditionalAttributes(additionalAttributes);
return this;
}
/**
*
* The identifier for the document.
*
*
* @param documentId
* The identifier for the document.
*/
public void setDocumentId(String documentId) {
this.documentId = documentId;
}
/**
*
* The identifier for the document.
*
*
* @return The identifier for the document.
*/
public String getDocumentId() {
return this.documentId;
}
/**
*
* The identifier for the document.
*
*
* @param documentId
* The identifier for the document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withDocumentId(String documentId) {
setDocumentId(documentId);
return this;
}
/**
*
* The title of the document. Contains the text of the title and information for highlighting the relevant terms in
* the title.
*
*
* @param documentTitle
* The title of the document. Contains the text of the title and information for highlighting the relevant
* terms in the title.
*/
public void setDocumentTitle(TextWithHighlights documentTitle) {
this.documentTitle = documentTitle;
}
/**
*
* The title of the document. Contains the text of the title and information for highlighting the relevant terms in
* the title.
*
*
* @return The title of the document. Contains the text of the title and information for highlighting the relevant
* terms in the title.
*/
public TextWithHighlights getDocumentTitle() {
return this.documentTitle;
}
/**
*
* The title of the document. Contains the text of the title and information for highlighting the relevant terms in
* the title.
*
*
* @param documentTitle
* The title of the document. Contains the text of the title and information for highlighting the relevant
* terms in the title.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withDocumentTitle(TextWithHighlights documentTitle) {
setDocumentTitle(documentTitle);
return this;
}
/**
*
* An extract of the text in the document. Contains information about highlighting the relevant terms in the
* excerpt.
*
*
* @param documentExcerpt
* An extract of the text in the document. Contains information about highlighting the relevant terms in the
* excerpt.
*/
public void setDocumentExcerpt(TextWithHighlights documentExcerpt) {
this.documentExcerpt = documentExcerpt;
}
/**
*
* An extract of the text in the document. Contains information about highlighting the relevant terms in the
* excerpt.
*
*
* @return An extract of the text in the document. Contains information about highlighting the relevant terms in the
* excerpt.
*/
public TextWithHighlights getDocumentExcerpt() {
return this.documentExcerpt;
}
/**
*
* An extract of the text in the document. Contains information about highlighting the relevant terms in the
* excerpt.
*
*
* @param documentExcerpt
* An extract of the text in the document. Contains information about highlighting the relevant terms in the
* excerpt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withDocumentExcerpt(TextWithHighlights documentExcerpt) {
setDocumentExcerpt(documentExcerpt);
return this;
}
/**
*
* The URI of the original location of the document.
*
*
* @param documentURI
* The URI of the original location of the document.
*/
public void setDocumentURI(String documentURI) {
this.documentURI = documentURI;
}
/**
*
* The URI of the original location of the document.
*
*
* @return The URI of the original location of the document.
*/
public String getDocumentURI() {
return this.documentURI;
}
/**
*
* The URI of the original location of the document.
*
*
* @param documentURI
* The URI of the original location of the document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withDocumentURI(String documentURI) {
setDocumentURI(documentURI);
return this;
}
/**
*
* An array of document fields/attributes assigned to a document in the search results. For example, the document
* author (_author
) or the source URI (_source_uri
) of the document.
*
*
* @return An array of document fields/attributes assigned to a document in the search results. For example, the
* document author (_author
) or the source URI (_source_uri
) of the document.
*/
public java.util.List getDocumentAttributes() {
return documentAttributes;
}
/**
*
* An array of document fields/attributes assigned to a document in the search results. For example, the document
* author (_author
) or the source URI (_source_uri
) of the document.
*
*
* @param documentAttributes
* An array of document fields/attributes assigned to a document in the search results. For example, the
* document author (_author
) or the source URI (_source_uri
) of the document.
*/
public void setDocumentAttributes(java.util.Collection documentAttributes) {
if (documentAttributes == null) {
this.documentAttributes = null;
return;
}
this.documentAttributes = new java.util.ArrayList(documentAttributes);
}
/**
*
* An array of document fields/attributes assigned to a document in the search results. For example, the document
* author (_author
) or the source URI (_source_uri
) of the document.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDocumentAttributes(java.util.Collection)} or {@link #withDocumentAttributes(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param documentAttributes
* An array of document fields/attributes assigned to a document in the search results. For example, the
* document author (_author
) or the source URI (_source_uri
) of the document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withDocumentAttributes(DocumentAttribute... documentAttributes) {
if (this.documentAttributes == null) {
setDocumentAttributes(new java.util.ArrayList(documentAttributes.length));
}
for (DocumentAttribute ele : documentAttributes) {
this.documentAttributes.add(ele);
}
return this;
}
/**
*
* An array of document fields/attributes assigned to a document in the search results. For example, the document
* author (_author
) or the source URI (_source_uri
) of the document.
*
*
* @param documentAttributes
* An array of document fields/attributes assigned to a document in the search results. For example, the
* document author (_author
) or the source URI (_source_uri
) of the document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withDocumentAttributes(java.util.Collection documentAttributes) {
setDocumentAttributes(documentAttributes);
return this;
}
/**
*
* Indicates the confidence level of Amazon Kendra providing a relevant result for the query. Each result is placed
* into a bin that indicates the confidence, VERY_HIGH
, HIGH
, MEDIUM
and
* LOW
. You can use the score to determine if a response meets the confidence needed for your
* application.
*
*
* The field is only set to LOW
when the Type
field is set to DOCUMENT
and
* Amazon Kendra is not confident that the result is relevant to the query.
*
*
* @param scoreAttributes
* Indicates the confidence level of Amazon Kendra providing a relevant result for the query. Each result is
* placed into a bin that indicates the confidence, VERY_HIGH
, HIGH
,
* MEDIUM
and LOW
. You can use the score to determine if a response meets the
* confidence needed for your application.
*
* The field is only set to LOW
when the Type
field is set to DOCUMENT
* and Amazon Kendra is not confident that the result is relevant to the query.
*/
public void setScoreAttributes(ScoreAttributes scoreAttributes) {
this.scoreAttributes = scoreAttributes;
}
/**
*
* Indicates the confidence level of Amazon Kendra providing a relevant result for the query. Each result is placed
* into a bin that indicates the confidence, VERY_HIGH
, HIGH
, MEDIUM
and
* LOW
. You can use the score to determine if a response meets the confidence needed for your
* application.
*
*
* The field is only set to LOW
when the Type
field is set to DOCUMENT
and
* Amazon Kendra is not confident that the result is relevant to the query.
*
*
* @return Indicates the confidence level of Amazon Kendra providing a relevant result for the query. Each result is
* placed into a bin that indicates the confidence, VERY_HIGH
, HIGH
,
* MEDIUM
and LOW
. You can use the score to determine if a response meets the
* confidence needed for your application.
*
* The field is only set to LOW
when the Type
field is set to
* DOCUMENT
and Amazon Kendra is not confident that the result is relevant to the query.
*/
public ScoreAttributes getScoreAttributes() {
return this.scoreAttributes;
}
/**
*
* Indicates the confidence level of Amazon Kendra providing a relevant result for the query. Each result is placed
* into a bin that indicates the confidence, VERY_HIGH
, HIGH
, MEDIUM
and
* LOW
. You can use the score to determine if a response meets the confidence needed for your
* application.
*
*
* The field is only set to LOW
when the Type
field is set to DOCUMENT
and
* Amazon Kendra is not confident that the result is relevant to the query.
*
*
* @param scoreAttributes
* Indicates the confidence level of Amazon Kendra providing a relevant result for the query. Each result is
* placed into a bin that indicates the confidence, VERY_HIGH
, HIGH
,
* MEDIUM
and LOW
. You can use the score to determine if a response meets the
* confidence needed for your application.
*
* The field is only set to LOW
when the Type
field is set to DOCUMENT
* and Amazon Kendra is not confident that the result is relevant to the query.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withScoreAttributes(ScoreAttributes scoreAttributes) {
setScoreAttributes(scoreAttributes);
return this;
}
/**
*
* A token that identifies a particular result from a particular query. Use this token to provide click-through
* feedback for the result. For more information, see Submitting feedback.
*
*
* @param feedbackToken
* A token that identifies a particular result from a particular query. Use this token to provide
* click-through feedback for the result. For more information, see Submitting feedback.
*/
public void setFeedbackToken(String feedbackToken) {
this.feedbackToken = feedbackToken;
}
/**
*
* A token that identifies a particular result from a particular query. Use this token to provide click-through
* feedback for the result. For more information, see Submitting feedback.
*
*
* @return A token that identifies a particular result from a particular query. Use this token to provide
* click-through feedback for the result. For more information, see Submitting feedback.
*/
public String getFeedbackToken() {
return this.feedbackToken;
}
/**
*
* A token that identifies a particular result from a particular query. Use this token to provide click-through
* feedback for the result. For more information, see Submitting feedback.
*
*
* @param feedbackToken
* A token that identifies a particular result from a particular query. Use this token to provide
* click-through feedback for the result. For more information, see Submitting feedback.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withFeedbackToken(String feedbackToken) {
setFeedbackToken(feedbackToken);
return this;
}
/**
*
* An excerpt from a table within a document.
*
*
* @param tableExcerpt
* An excerpt from a table within a document.
*/
public void setTableExcerpt(TableExcerpt tableExcerpt) {
this.tableExcerpt = tableExcerpt;
}
/**
*
* An excerpt from a table within a document.
*
*
* @return An excerpt from a table within a document.
*/
public TableExcerpt getTableExcerpt() {
return this.tableExcerpt;
}
/**
*
* An excerpt from a table within a document.
*
*
* @param tableExcerpt
* An excerpt from a table within a document.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withTableExcerpt(TableExcerpt tableExcerpt) {
setTableExcerpt(tableExcerpt);
return this;
}
/**
*
* Provides details about a collapsed group of search results.
*
*
* @param collapsedResultDetail
* Provides details about a collapsed group of search results.
*/
public void setCollapsedResultDetail(CollapsedResultDetail collapsedResultDetail) {
this.collapsedResultDetail = collapsedResultDetail;
}
/**
*
* Provides details about a collapsed group of search results.
*
*
* @return Provides details about a collapsed group of search results.
*/
public CollapsedResultDetail getCollapsedResultDetail() {
return this.collapsedResultDetail;
}
/**
*
* Provides details about a collapsed group of search results.
*
*
* @param collapsedResultDetail
* Provides details about a collapsed group of search results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public QueryResultItem withCollapsedResultDetail(CollapsedResultDetail collapsedResultDetail) {
setCollapsedResultDetail(collapsedResultDetail);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getFormat() != null)
sb.append("Format: ").append(getFormat()).append(",");
if (getAdditionalAttributes() != null)
sb.append("AdditionalAttributes: ").append(getAdditionalAttributes()).append(",");
if (getDocumentId() != null)
sb.append("DocumentId: ").append(getDocumentId()).append(",");
if (getDocumentTitle() != null)
sb.append("DocumentTitle: ").append(getDocumentTitle()).append(",");
if (getDocumentExcerpt() != null)
sb.append("DocumentExcerpt: ").append(getDocumentExcerpt()).append(",");
if (getDocumentURI() != null)
sb.append("DocumentURI: ").append(getDocumentURI()).append(",");
if (getDocumentAttributes() != null)
sb.append("DocumentAttributes: ").append(getDocumentAttributes()).append(",");
if (getScoreAttributes() != null)
sb.append("ScoreAttributes: ").append(getScoreAttributes()).append(",");
if (getFeedbackToken() != null)
sb.append("FeedbackToken: ").append(getFeedbackToken()).append(",");
if (getTableExcerpt() != null)
sb.append("TableExcerpt: ").append(getTableExcerpt()).append(",");
if (getCollapsedResultDetail() != null)
sb.append("CollapsedResultDetail: ").append(getCollapsedResultDetail());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof QueryResultItem == false)
return false;
QueryResultItem other = (QueryResultItem) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getFormat() == null ^ this.getFormat() == null)
return false;
if (other.getFormat() != null && other.getFormat().equals(this.getFormat()) == false)
return false;
if (other.getAdditionalAttributes() == null ^ this.getAdditionalAttributes() == null)
return false;
if (other.getAdditionalAttributes() != null && other.getAdditionalAttributes().equals(this.getAdditionalAttributes()) == false)
return false;
if (other.getDocumentId() == null ^ this.getDocumentId() == null)
return false;
if (other.getDocumentId() != null && other.getDocumentId().equals(this.getDocumentId()) == false)
return false;
if (other.getDocumentTitle() == null ^ this.getDocumentTitle() == null)
return false;
if (other.getDocumentTitle() != null && other.getDocumentTitle().equals(this.getDocumentTitle()) == false)
return false;
if (other.getDocumentExcerpt() == null ^ this.getDocumentExcerpt() == null)
return false;
if (other.getDocumentExcerpt() != null && other.getDocumentExcerpt().equals(this.getDocumentExcerpt()) == false)
return false;
if (other.getDocumentURI() == null ^ this.getDocumentURI() == null)
return false;
if (other.getDocumentURI() != null && other.getDocumentURI().equals(this.getDocumentURI()) == false)
return false;
if (other.getDocumentAttributes() == null ^ this.getDocumentAttributes() == null)
return false;
if (other.getDocumentAttributes() != null && other.getDocumentAttributes().equals(this.getDocumentAttributes()) == false)
return false;
if (other.getScoreAttributes() == null ^ this.getScoreAttributes() == null)
return false;
if (other.getScoreAttributes() != null && other.getScoreAttributes().equals(this.getScoreAttributes()) == false)
return false;
if (other.getFeedbackToken() == null ^ this.getFeedbackToken() == null)
return false;
if (other.getFeedbackToken() != null && other.getFeedbackToken().equals(this.getFeedbackToken()) == false)
return false;
if (other.getTableExcerpt() == null ^ this.getTableExcerpt() == null)
return false;
if (other.getTableExcerpt() != null && other.getTableExcerpt().equals(this.getTableExcerpt()) == false)
return false;
if (other.getCollapsedResultDetail() == null ^ this.getCollapsedResultDetail() == null)
return false;
if (other.getCollapsedResultDetail() != null && other.getCollapsedResultDetail().equals(this.getCollapsedResultDetail()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getFormat() == null) ? 0 : getFormat().hashCode());
hashCode = prime * hashCode + ((getAdditionalAttributes() == null) ? 0 : getAdditionalAttributes().hashCode());
hashCode = prime * hashCode + ((getDocumentId() == null) ? 0 : getDocumentId().hashCode());
hashCode = prime * hashCode + ((getDocumentTitle() == null) ? 0 : getDocumentTitle().hashCode());
hashCode = prime * hashCode + ((getDocumentExcerpt() == null) ? 0 : getDocumentExcerpt().hashCode());
hashCode = prime * hashCode + ((getDocumentURI() == null) ? 0 : getDocumentURI().hashCode());
hashCode = prime * hashCode + ((getDocumentAttributes() == null) ? 0 : getDocumentAttributes().hashCode());
hashCode = prime * hashCode + ((getScoreAttributes() == null) ? 0 : getScoreAttributes().hashCode());
hashCode = prime * hashCode + ((getFeedbackToken() == null) ? 0 : getFeedbackToken().hashCode());
hashCode = prime * hashCode + ((getTableExcerpt() == null) ? 0 : getTableExcerpt().hashCode());
hashCode = prime * hashCode + ((getCollapsedResultDetail() == null) ? 0 : getCollapsedResultDetail().hashCode());
return hashCode;
}
@Override
public QueryResultItem clone() {
try {
return (QueryResultItem) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.QueryResultItemMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}