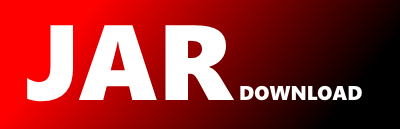
com.amazonaws.services.kendra.AWSkendraAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra;
import javax.annotation.Generated;
import com.amazonaws.services.kendra.model.*;
/**
* Interface for accessing kendra asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.kendra.AbstractAWSkendraAsync} instead.
*
*
*
* Amazon Kendra is a service for indexing large document sets.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSkendraAsync extends AWSkendra {
/**
*
* Grants users or groups in your IAM Identity Center identity source access to your Amazon Kendra experience. You
* can create an Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, see Building a search
* experience with no code.
*
*
* @param associateEntitiesToExperienceRequest
* @return A Java Future containing the result of the AssociateEntitiesToExperience operation returned by the
* service.
* @sample AWSkendraAsync.AssociateEntitiesToExperience
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEntitiesToExperienceAsync(
AssociateEntitiesToExperienceRequest associateEntitiesToExperienceRequest);
/**
*
* Grants users or groups in your IAM Identity Center identity source access to your Amazon Kendra experience. You
* can create an Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, see Building a search
* experience with no code.
*
*
* @param associateEntitiesToExperienceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateEntitiesToExperience operation returned by the
* service.
* @sample AWSkendraAsyncHandler.AssociateEntitiesToExperience
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEntitiesToExperienceAsync(
AssociateEntitiesToExperienceRequest associateEntitiesToExperienceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Defines the specific permissions of users or groups in your IAM Identity Center identity source with access to
* your Amazon Kendra experience. You can create an Amazon Kendra experience such as a search application. For more
* information on creating a search application experience, see Building a search
* experience with no code.
*
*
* @param associatePersonasToEntitiesRequest
* @return A Java Future containing the result of the AssociatePersonasToEntities operation returned by the service.
* @sample AWSkendraAsync.AssociatePersonasToEntities
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePersonasToEntitiesAsync(
AssociatePersonasToEntitiesRequest associatePersonasToEntitiesRequest);
/**
*
* Defines the specific permissions of users or groups in your IAM Identity Center identity source with access to
* your Amazon Kendra experience. You can create an Amazon Kendra experience such as a search application. For more
* information on creating a search application experience, see Building a search
* experience with no code.
*
*
* @param associatePersonasToEntitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociatePersonasToEntities operation returned by the service.
* @sample AWSkendraAsyncHandler.AssociatePersonasToEntities
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePersonasToEntitiesAsync(
AssociatePersonasToEntitiesRequest associatePersonasToEntitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more documents from an index. The documents must have been added with the
* BatchPutDocument
API.
*
*
* The documents are deleted asynchronously. You can see the progress of the deletion by using Amazon Web Services
* CloudWatch. Any error messages related to the processing of the batch are sent to your Amazon Web Services
* CloudWatch log. You can also use the BatchGetDocumentStatus
API to monitor the progress of deleting
* your documents.
*
*
* Deleting documents from an index using BatchDeleteDocument
could take up to an hour or more,
* depending on the number of documents you want to delete.
*
*
* @param batchDeleteDocumentRequest
* @return A Java Future containing the result of the BatchDeleteDocument operation returned by the service.
* @sample AWSkendraAsync.BatchDeleteDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchDeleteDocumentAsync(BatchDeleteDocumentRequest batchDeleteDocumentRequest);
/**
*
* Removes one or more documents from an index. The documents must have been added with the
* BatchPutDocument
API.
*
*
* The documents are deleted asynchronously. You can see the progress of the deletion by using Amazon Web Services
* CloudWatch. Any error messages related to the processing of the batch are sent to your Amazon Web Services
* CloudWatch log. You can also use the BatchGetDocumentStatus
API to monitor the progress of deleting
* your documents.
*
*
* Deleting documents from an index using BatchDeleteDocument
could take up to an hour or more,
* depending on the number of documents you want to delete.
*
*
* @param batchDeleteDocumentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteDocument operation returned by the service.
* @sample AWSkendraAsyncHandler.BatchDeleteDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchDeleteDocumentAsync(BatchDeleteDocumentRequest batchDeleteDocumentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more sets of featured results. Features results are placed above all other results for certain
* queries. If there's an exact match of a query, then one or more specific documents are featured in the search
* results.
*
*
* @param batchDeleteFeaturedResultsSetRequest
* @return A Java Future containing the result of the BatchDeleteFeaturedResultsSet operation returned by the
* service.
* @sample AWSkendraAsync.BatchDeleteFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteFeaturedResultsSetAsync(
BatchDeleteFeaturedResultsSetRequest batchDeleteFeaturedResultsSetRequest);
/**
*
* Removes one or more sets of featured results. Features results are placed above all other results for certain
* queries. If there's an exact match of a query, then one or more specific documents are featured in the search
* results.
*
*
* @param batchDeleteFeaturedResultsSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteFeaturedResultsSet operation returned by the
* service.
* @sample AWSkendraAsyncHandler.BatchDeleteFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteFeaturedResultsSetAsync(
BatchDeleteFeaturedResultsSetRequest batchDeleteFeaturedResultsSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the indexing status for one or more documents submitted with the BatchPutDocument API.
*
*
* When you use the BatchPutDocument
API, documents are indexed asynchronously. You can use the
* BatchGetDocumentStatus
API to get the current status of a list of documents so that you can
* determine if they have been successfully indexed.
*
*
* You can also use the BatchGetDocumentStatus
API to check the status of the BatchDeleteDocument API.
* When a document is deleted from the index, Amazon Kendra returns NOT_FOUND
as the status.
*
*
* @param batchGetDocumentStatusRequest
* @return A Java Future containing the result of the BatchGetDocumentStatus operation returned by the service.
* @sample AWSkendraAsync.BatchGetDocumentStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchGetDocumentStatusAsync(BatchGetDocumentStatusRequest batchGetDocumentStatusRequest);
/**
*
* Returns the indexing status for one or more documents submitted with the BatchPutDocument API.
*
*
* When you use the BatchPutDocument
API, documents are indexed asynchronously. You can use the
* BatchGetDocumentStatus
API to get the current status of a list of documents so that you can
* determine if they have been successfully indexed.
*
*
* You can also use the BatchGetDocumentStatus
API to check the status of the BatchDeleteDocument API.
* When a document is deleted from the index, Amazon Kendra returns NOT_FOUND
as the status.
*
*
* @param batchGetDocumentStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetDocumentStatus operation returned by the service.
* @sample AWSkendraAsyncHandler.BatchGetDocumentStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchGetDocumentStatusAsync(BatchGetDocumentStatusRequest batchGetDocumentStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more documents to an index.
*
*
* The BatchPutDocument
API enables you to ingest inline documents or a set of documents stored in an
* Amazon S3 bucket. Use this API to ingest your text and unstructured text into an index, add custom attributes to
* the documents, and to attach an access control list to the documents added to the index.
*
*
* The documents are indexed asynchronously. You can see the progress of the batch using Amazon Web Services
* CloudWatch. Any error messages related to processing the batch are sent to your Amazon Web Services CloudWatch
* log. You can also use the BatchGetDocumentStatus
API to monitor the progress of indexing your
* documents.
*
*
* For an example of ingesting inline documents using Python and Java SDKs, see Adding files directly to an
* index.
*
*
* @param batchPutDocumentRequest
* @return A Java Future containing the result of the BatchPutDocument operation returned by the service.
* @sample AWSkendraAsync.BatchPutDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchPutDocumentAsync(BatchPutDocumentRequest batchPutDocumentRequest);
/**
*
* Adds one or more documents to an index.
*
*
* The BatchPutDocument
API enables you to ingest inline documents or a set of documents stored in an
* Amazon S3 bucket. Use this API to ingest your text and unstructured text into an index, add custom attributes to
* the documents, and to attach an access control list to the documents added to the index.
*
*
* The documents are indexed asynchronously. You can see the progress of the batch using Amazon Web Services
* CloudWatch. Any error messages related to processing the batch are sent to your Amazon Web Services CloudWatch
* log. You can also use the BatchGetDocumentStatus
API to monitor the progress of indexing your
* documents.
*
*
* For an example of ingesting inline documents using Python and Java SDKs, see Adding files directly to an
* index.
*
*
* @param batchPutDocumentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchPutDocument operation returned by the service.
* @sample AWSkendraAsyncHandler.BatchPutDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchPutDocumentAsync(BatchPutDocumentRequest batchPutDocumentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Clears existing query suggestions from an index.
*
*
* This deletes existing suggestions only, not the queries in the query log. After you clear suggestions, Amazon
* Kendra learns new suggestions based on new queries added to the query log from the time you cleared suggestions.
* If you do not see any new suggestions, then please allow Amazon Kendra to collect enough queries to learn new
* suggestions.
*
*
* ClearQuerySuggestions
is currently not supported in the Amazon Web Services GovCloud (US-West)
* region.
*
*
* @param clearQuerySuggestionsRequest
* @return A Java Future containing the result of the ClearQuerySuggestions operation returned by the service.
* @sample AWSkendraAsync.ClearQuerySuggestions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future clearQuerySuggestionsAsync(ClearQuerySuggestionsRequest clearQuerySuggestionsRequest);
/**
*
* Clears existing query suggestions from an index.
*
*
* This deletes existing suggestions only, not the queries in the query log. After you clear suggestions, Amazon
* Kendra learns new suggestions based on new queries added to the query log from the time you cleared suggestions.
* If you do not see any new suggestions, then please allow Amazon Kendra to collect enough queries to learn new
* suggestions.
*
*
* ClearQuerySuggestions
is currently not supported in the Amazon Web Services GovCloud (US-West)
* region.
*
*
* @param clearQuerySuggestionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ClearQuerySuggestions operation returned by the service.
* @sample AWSkendraAsyncHandler.ClearQuerySuggestions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future clearQuerySuggestionsAsync(ClearQuerySuggestionsRequest clearQuerySuggestionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an access configuration for your documents. This includes user and group access information for your
* documents. This is useful for user context filtering, where search results are filtered based on the user or
* their group access to documents.
*
*
* You can use this to re-configure your existing document level access control without indexing all of your
* documents again. For example, your index contains top-secret company documents that only certain employees or
* users should access. One of these users leaves the company or switches to a team that should be blocked from
* accessing top-secret documents. The user still has access to top-secret documents because the user had access
* when your documents were previously indexed. You can create a specific access control configuration for the user
* with deny access. You can later update the access control configuration to allow access if the user returns to
* the company and re-joins the 'top-secret' team. You can re-configure access control for your documents as
* circumstances change.
*
*
* To apply your access control configuration to certain documents, you call the BatchPutDocument API with the
* AccessControlConfigurationId
included in the Document object. If you use an S3
* bucket as a data source, you update the .metadata.json
with the
* AccessControlConfigurationId
and synchronize your data source. Amazon Kendra currently only supports
* access control configuration for S3 data sources and documents indexed using the BatchPutDocument
* API.
*
*
* @param createAccessControlConfigurationRequest
* @return A Java Future containing the result of the CreateAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsync.CreateAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createAccessControlConfigurationAsync(
CreateAccessControlConfigurationRequest createAccessControlConfigurationRequest);
/**
*
* Creates an access configuration for your documents. This includes user and group access information for your
* documents. This is useful for user context filtering, where search results are filtered based on the user or
* their group access to documents.
*
*
* You can use this to re-configure your existing document level access control without indexing all of your
* documents again. For example, your index contains top-secret company documents that only certain employees or
* users should access. One of these users leaves the company or switches to a team that should be blocked from
* accessing top-secret documents. The user still has access to top-secret documents because the user had access
* when your documents were previously indexed. You can create a specific access control configuration for the user
* with deny access. You can later update the access control configuration to allow access if the user returns to
* the company and re-joins the 'top-secret' team. You can re-configure access control for your documents as
* circumstances change.
*
*
* To apply your access control configuration to certain documents, you call the BatchPutDocument API with the
* AccessControlConfigurationId
included in the Document object. If you use an S3
* bucket as a data source, you update the .metadata.json
with the
* AccessControlConfigurationId
and synchronize your data source. Amazon Kendra currently only supports
* access control configuration for S3 data sources and documents indexed using the BatchPutDocument
* API.
*
*
* @param createAccessControlConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsyncHandler.CreateAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createAccessControlConfigurationAsync(
CreateAccessControlConfigurationRequest createAccessControlConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a data source connector that you want to use with an Amazon Kendra index.
*
*
* You specify a name, data source connector type and description for your data source. You also specify
* configuration information for the data source connector.
*
*
* CreateDataSource
is a synchronous operation. The operation returns 200 if the data source was
* successfully created. Otherwise, an exception is raised.
*
*
* For an example of creating an index and data source using the Python SDK, see Getting started with Python SDK. For an
* example of creating an index and data source using the Java SDK, see Getting started with Java SDK.
*
*
* @param createDataSourceRequest
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AWSkendraAsync.CreateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates a data source connector that you want to use with an Amazon Kendra index.
*
*
* You specify a name, data source connector type and description for your data source. You also specify
* configuration information for the data source connector.
*
*
* CreateDataSource
is a synchronous operation. The operation returns 200 if the data source was
* successfully created. Otherwise, an exception is raised.
*
*
* For an example of creating an index and data source using the Python SDK, see Getting started with Python SDK. For an
* example of creating an index and data source using the Java SDK, see Getting started with Java SDK.
*
*
* @param createDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AWSkendraAsyncHandler.CreateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, including using the Python and Java SDKs, see Building a search
* experience with no code.
*
*
* @param createExperienceRequest
* @return A Java Future containing the result of the CreateExperience operation returned by the service.
* @sample AWSkendraAsync.CreateExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createExperienceAsync(CreateExperienceRequest createExperienceRequest);
/**
*
* Creates an Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, including using the Python and Java SDKs, see Building a search
* experience with no code.
*
*
* @param createExperienceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateExperience operation returned by the service.
* @sample AWSkendraAsyncHandler.CreateExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createExperienceAsync(CreateExperienceRequest createExperienceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a set of frequently ask questions (FAQs) using a specified FAQ file stored in an Amazon S3 bucket.
*
*
* Adding FAQs to an index is an asynchronous operation.
*
*
* For an example of adding an FAQ to an index using Python and Java SDKs, see Using your FAQ file.
*
*
* @param createFaqRequest
* @return A Java Future containing the result of the CreateFaq operation returned by the service.
* @sample AWSkendraAsync.CreateFaq
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFaqAsync(CreateFaqRequest createFaqRequest);
/**
*
* Creates a set of frequently ask questions (FAQs) using a specified FAQ file stored in an Amazon S3 bucket.
*
*
* Adding FAQs to an index is an asynchronous operation.
*
*
* For an example of adding an FAQ to an index using Python and Java SDKs, see Using your FAQ file.
*
*
* @param createFaqRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFaq operation returned by the service.
* @sample AWSkendraAsyncHandler.CreateFaq
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFaqAsync(CreateFaqRequest createFaqRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a set of featured results to display at the top of the search results page. Featured results are placed
* above all other results for certain queries. You map specific queries to specific documents for featuring in the
* results. If a query contains an exact match, then one or more specific documents are featured in the search
* results.
*
*
* You can create up to 50 sets of featured results per index. You can request to increase this limit by contacting
* Support.
*
*
* @param createFeaturedResultsSetRequest
* @return A Java Future containing the result of the CreateFeaturedResultsSet operation returned by the service.
* @sample AWSkendraAsync.CreateFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createFeaturedResultsSetAsync(CreateFeaturedResultsSetRequest createFeaturedResultsSetRequest);
/**
*
* Creates a set of featured results to display at the top of the search results page. Featured results are placed
* above all other results for certain queries. You map specific queries to specific documents for featuring in the
* results. If a query contains an exact match, then one or more specific documents are featured in the search
* results.
*
*
* You can create up to 50 sets of featured results per index. You can request to increase this limit by contacting
* Support.
*
*
* @param createFeaturedResultsSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFeaturedResultsSet operation returned by the service.
* @sample AWSkendraAsyncHandler.CreateFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createFeaturedResultsSetAsync(CreateFeaturedResultsSetRequest createFeaturedResultsSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Kendra index. Index creation is an asynchronous API. To determine if index creation has
* completed, check the Status
field returned from a call to DescribeIndex
. The
* Status
field is set to ACTIVE
when the index is ready to use.
*
*
* Once the index is active, you can index your documents using the BatchPutDocument
API or using one
* of the supported data sources.
*
*
* For an example of creating an index and data source using the Python SDK, see Getting started with Python SDK. For an
* example of creating an index and data source using the Java SDK, see Getting started with Java SDK.
*
*
* @param createIndexRequest
* @return A Java Future containing the result of the CreateIndex operation returned by the service.
* @sample AWSkendraAsync.CreateIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIndexAsync(CreateIndexRequest createIndexRequest);
/**
*
* Creates an Amazon Kendra index. Index creation is an asynchronous API. To determine if index creation has
* completed, check the Status
field returned from a call to DescribeIndex
. The
* Status
field is set to ACTIVE
when the index is ready to use.
*
*
* Once the index is active, you can index your documents using the BatchPutDocument
API or using one
* of the supported data sources.
*
*
* For an example of creating an index and data source using the Python SDK, see Getting started with Python SDK. For an
* example of creating an index and data source using the Java SDK, see Getting started with Java SDK.
*
*
* @param createIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIndex operation returned by the service.
* @sample AWSkendraAsyncHandler.CreateIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIndexAsync(CreateIndexRequest createIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a block list to exlcude certain queries from suggestions.
*
*
* Any query that contains words or phrases specified in the block list is blocked or filtered out from being shown
* as a suggestion.
*
*
* You need to provide the file location of your block list text file in your S3 bucket. In your text file, enter
* each block word or phrase on a separate line.
*
*
* For information on the current quota limits for block lists, see Quotas for Amazon Kendra.
*
*
* CreateQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* For an example of creating a block list for query suggestions using the Python SDK, see Query
* suggestions block list.
*
*
* @param createQuerySuggestionsBlockListRequest
* @return A Java Future containing the result of the CreateQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsync.CreateQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future createQuerySuggestionsBlockListAsync(
CreateQuerySuggestionsBlockListRequest createQuerySuggestionsBlockListRequest);
/**
*
* Creates a block list to exlcude certain queries from suggestions.
*
*
* Any query that contains words or phrases specified in the block list is blocked or filtered out from being shown
* as a suggestion.
*
*
* You need to provide the file location of your block list text file in your S3 bucket. In your text file, enter
* each block word or phrase on a separate line.
*
*
* For information on the current quota limits for block lists, see Quotas for Amazon Kendra.
*
*
* CreateQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* For an example of creating a block list for query suggestions using the Python SDK, see Query
* suggestions block list.
*
*
* @param createQuerySuggestionsBlockListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsyncHandler.CreateQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future createQuerySuggestionsBlockListAsync(
CreateQuerySuggestionsBlockListRequest createQuerySuggestionsBlockListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a thesaurus for an index. The thesaurus contains a list of synonyms in Solr format.
*
*
* For an example of adding a thesaurus file to an index, see Adding custom
* synonyms to an index.
*
*
* @param createThesaurusRequest
* @return A Java Future containing the result of the CreateThesaurus operation returned by the service.
* @sample AWSkendraAsync.CreateThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createThesaurusAsync(CreateThesaurusRequest createThesaurusRequest);
/**
*
* Creates a thesaurus for an index. The thesaurus contains a list of synonyms in Solr format.
*
*
* For an example of adding a thesaurus file to an index, see Adding custom
* synonyms to an index.
*
*
* @param createThesaurusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateThesaurus operation returned by the service.
* @sample AWSkendraAsyncHandler.CreateThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createThesaurusAsync(CreateThesaurusRequest createThesaurusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an access control configuration that you created for your documents in an index. This includes user and
* group access information for your documents. This is useful for user context filtering, where search results are
* filtered based on the user or their group access to documents.
*
*
* @param deleteAccessControlConfigurationRequest
* @return A Java Future containing the result of the DeleteAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsync.DeleteAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccessControlConfigurationAsync(
DeleteAccessControlConfigurationRequest deleteAccessControlConfigurationRequest);
/**
*
* Deletes an access control configuration that you created for your documents in an index. This includes user and
* group access information for your documents. This is useful for user context filtering, where search results are
* filtered based on the user or their group access to documents.
*
*
* @param deleteAccessControlConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsyncHandler.DeleteAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccessControlConfigurationAsync(
DeleteAccessControlConfigurationRequest deleteAccessControlConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Kendra data source connector. An exception is not thrown if the data source is already being
* deleted. While the data source is being deleted, the Status
field returned by a call to the
* DescribeDataSource
API is set to DELETING
. For more information, see Deleting Data Sources.
*
*
* Deleting an entire data source or re-syncing your index after deleting specific documents from a data source
* could take up to an hour or more, depending on the number of documents you want to delete.
*
*
* @param deleteDataSourceRequest
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AWSkendraAsync.DeleteDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes an Amazon Kendra data source connector. An exception is not thrown if the data source is already being
* deleted. While the data source is being deleted, the Status
field returned by a call to the
* DescribeDataSource
API is set to DELETING
. For more information, see Deleting Data Sources.
*
*
* Deleting an entire data source or re-syncing your index after deleting specific documents from a data source
* could take up to an hour or more, depending on the number of documents you want to delete.
*
*
* @param deleteDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AWSkendraAsyncHandler.DeleteDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes your Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, see Building a search
* experience with no code.
*
*
* @param deleteExperienceRequest
* @return A Java Future containing the result of the DeleteExperience operation returned by the service.
* @sample AWSkendraAsync.DeleteExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteExperienceAsync(DeleteExperienceRequest deleteExperienceRequest);
/**
*
* Deletes your Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, see Building a search
* experience with no code.
*
*
* @param deleteExperienceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteExperience operation returned by the service.
* @sample AWSkendraAsyncHandler.DeleteExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteExperienceAsync(DeleteExperienceRequest deleteExperienceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an FAQ from an index.
*
*
* @param deleteFaqRequest
* @return A Java Future containing the result of the DeleteFaq operation returned by the service.
* @sample AWSkendraAsync.DeleteFaq
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFaqAsync(DeleteFaqRequest deleteFaqRequest);
/**
*
* Removes an FAQ from an index.
*
*
* @param deleteFaqRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFaq operation returned by the service.
* @sample AWSkendraAsyncHandler.DeleteFaq
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFaqAsync(DeleteFaqRequest deleteFaqRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Kendra index. An exception is not thrown if the index is already being deleted. While the index
* is being deleted, the Status
field returned by a call to the DescribeIndex
API is set
* to DELETING
.
*
*
* @param deleteIndexRequest
* @return A Java Future containing the result of the DeleteIndex operation returned by the service.
* @sample AWSkendraAsync.DeleteIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIndexAsync(DeleteIndexRequest deleteIndexRequest);
/**
*
* Deletes an Amazon Kendra index. An exception is not thrown if the index is already being deleted. While the index
* is being deleted, the Status
field returned by a call to the DescribeIndex
API is set
* to DELETING
.
*
*
* @param deleteIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIndex operation returned by the service.
* @sample AWSkendraAsyncHandler.DeleteIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIndexAsync(DeleteIndexRequest deleteIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a group so that all users and sub groups that belong to the group can no longer access documents only
* available to that group.
*
*
* For example, after deleting the group "Summer Interns", all interns who belonged to that group no longer see
* intern-only documents in their search results.
*
*
* If you want to delete or replace users or sub groups of a group, you need to use the
* PutPrincipalMapping
operation. For example, if a user in the group "Engineering" leaves the
* engineering team and another user takes their place, you provide an updated list of users or sub groups that
* belong to the "Engineering" group when calling PutPrincipalMapping
. You can update your internal
* list of users or sub groups and input this list when calling PutPrincipalMapping
.
*
*
* DeletePrincipalMapping
is currently not supported in the Amazon Web Services GovCloud (US-West)
* region.
*
*
* @param deletePrincipalMappingRequest
* @return A Java Future containing the result of the DeletePrincipalMapping operation returned by the service.
* @sample AWSkendraAsync.DeletePrincipalMapping
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePrincipalMappingAsync(DeletePrincipalMappingRequest deletePrincipalMappingRequest);
/**
*
* Deletes a group so that all users and sub groups that belong to the group can no longer access documents only
* available to that group.
*
*
* For example, after deleting the group "Summer Interns", all interns who belonged to that group no longer see
* intern-only documents in their search results.
*
*
* If you want to delete or replace users or sub groups of a group, you need to use the
* PutPrincipalMapping
operation. For example, if a user in the group "Engineering" leaves the
* engineering team and another user takes their place, you provide an updated list of users or sub groups that
* belong to the "Engineering" group when calling PutPrincipalMapping
. You can update your internal
* list of users or sub groups and input this list when calling PutPrincipalMapping
.
*
*
* DeletePrincipalMapping
is currently not supported in the Amazon Web Services GovCloud (US-West)
* region.
*
*
* @param deletePrincipalMappingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePrincipalMapping operation returned by the service.
* @sample AWSkendraAsyncHandler.DeletePrincipalMapping
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deletePrincipalMappingAsync(DeletePrincipalMappingRequest deletePrincipalMappingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a block list used for query suggestions for an index.
*
*
* A deleted block list might not take effect right away. Amazon Kendra needs to refresh the entire suggestions list
* to add back the queries that were previously blocked.
*
*
* DeleteQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param deleteQuerySuggestionsBlockListRequest
* @return A Java Future containing the result of the DeleteQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsync.DeleteQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteQuerySuggestionsBlockListAsync(
DeleteQuerySuggestionsBlockListRequest deleteQuerySuggestionsBlockListRequest);
/**
*
* Deletes a block list used for query suggestions for an index.
*
*
* A deleted block list might not take effect right away. Amazon Kendra needs to refresh the entire suggestions list
* to add back the queries that were previously blocked.
*
*
* DeleteQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param deleteQuerySuggestionsBlockListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsyncHandler.DeleteQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteQuerySuggestionsBlockListAsync(
DeleteQuerySuggestionsBlockListRequest deleteQuerySuggestionsBlockListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Kendra thesaurus.
*
*
* @param deleteThesaurusRequest
* @return A Java Future containing the result of the DeleteThesaurus operation returned by the service.
* @sample AWSkendraAsync.DeleteThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteThesaurusAsync(DeleteThesaurusRequest deleteThesaurusRequest);
/**
*
* Deletes an Amazon Kendra thesaurus.
*
*
* @param deleteThesaurusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteThesaurus operation returned by the service.
* @sample AWSkendraAsyncHandler.DeleteThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteThesaurusAsync(DeleteThesaurusRequest deleteThesaurusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an access control configuration that you created for your documents in an index. This
* includes user and group access information for your documents. This is useful for user context filtering, where
* search results are filtered based on the user or their group access to documents.
*
*
* @param describeAccessControlConfigurationRequest
* @return A Java Future containing the result of the DescribeAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsync.DescribeAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccessControlConfigurationAsync(
DescribeAccessControlConfigurationRequest describeAccessControlConfigurationRequest);
/**
*
* Gets information about an access control configuration that you created for your documents in an index. This
* includes user and group access information for your documents. This is useful for user context filtering, where
* search results are filtered based on the user or their group access to documents.
*
*
* @param describeAccessControlConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsyncHandler.DescribeAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccessControlConfigurationAsync(
DescribeAccessControlConfigurationRequest describeAccessControlConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an Amazon Kendra data source connector.
*
*
* @param describeDataSourceRequest
* @return A Java Future containing the result of the DescribeDataSource operation returned by the service.
* @sample AWSkendraAsync.DescribeDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataSourceAsync(DescribeDataSourceRequest describeDataSourceRequest);
/**
*
* Gets information about an Amazon Kendra data source connector.
*
*
* @param describeDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSource operation returned by the service.
* @sample AWSkendraAsyncHandler.DescribeDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataSourceAsync(DescribeDataSourceRequest describeDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about your Amazon Kendra experience such as a search application. For more information on
* creating a search application experience, see Building a search
* experience with no code.
*
*
* @param describeExperienceRequest
* @return A Java Future containing the result of the DescribeExperience operation returned by the service.
* @sample AWSkendraAsync.DescribeExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeExperienceAsync(DescribeExperienceRequest describeExperienceRequest);
/**
*
* Gets information about your Amazon Kendra experience such as a search application. For more information on
* creating a search application experience, see Building a search
* experience with no code.
*
*
* @param describeExperienceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeExperience operation returned by the service.
* @sample AWSkendraAsyncHandler.DescribeExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeExperienceAsync(DescribeExperienceRequest describeExperienceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an FAQ list.
*
*
* @param describeFaqRequest
* @return A Java Future containing the result of the DescribeFaq operation returned by the service.
* @sample AWSkendraAsync.DescribeFaq
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFaqAsync(DescribeFaqRequest describeFaqRequest);
/**
*
* Gets information about an FAQ list.
*
*
* @param describeFaqRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFaq operation returned by the service.
* @sample AWSkendraAsyncHandler.DescribeFaq
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFaqAsync(DescribeFaqRequest describeFaqRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a set of featured results. Features results are placed above all other results for certain
* queries. If there's an exact match of a query, then one or more specific documents are featured in the search
* results.
*
*
* @param describeFeaturedResultsSetRequest
* @return A Java Future containing the result of the DescribeFeaturedResultsSet operation returned by the service.
* @sample AWSkendraAsync.DescribeFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFeaturedResultsSetAsync(
DescribeFeaturedResultsSetRequest describeFeaturedResultsSetRequest);
/**
*
* Gets information about a set of featured results. Features results are placed above all other results for certain
* queries. If there's an exact match of a query, then one or more specific documents are featured in the search
* results.
*
*
* @param describeFeaturedResultsSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFeaturedResultsSet operation returned by the service.
* @sample AWSkendraAsyncHandler.DescribeFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFeaturedResultsSetAsync(
DescribeFeaturedResultsSetRequest describeFeaturedResultsSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an Amazon Kendra index.
*
*
* @param describeIndexRequest
* @return A Java Future containing the result of the DescribeIndex operation returned by the service.
* @sample AWSkendraAsync.DescribeIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeIndexAsync(DescribeIndexRequest describeIndexRequest);
/**
*
* Gets information about an Amazon Kendra index.
*
*
* @param describeIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIndex operation returned by the service.
* @sample AWSkendraAsyncHandler.DescribeIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeIndexAsync(DescribeIndexRequest describeIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the processing of PUT
and DELETE
actions for mapping users to their groups.
* This includes information on the status of actions currently processing or yet to be processed, when actions were
* last updated, when actions were received by Amazon Kendra, the latest action that should process and apply after
* other actions, and useful error messages if an action could not be processed.
*
*
* DescribePrincipalMapping
is currently not supported in the Amazon Web Services GovCloud (US-West)
* region.
*
*
* @param describePrincipalMappingRequest
* @return A Java Future containing the result of the DescribePrincipalMapping operation returned by the service.
* @sample AWSkendraAsync.DescribePrincipalMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future describePrincipalMappingAsync(DescribePrincipalMappingRequest describePrincipalMappingRequest);
/**
*
* Describes the processing of PUT
and DELETE
actions for mapping users to their groups.
* This includes information on the status of actions currently processing or yet to be processed, when actions were
* last updated, when actions were received by Amazon Kendra, the latest action that should process and apply after
* other actions, and useful error messages if an action could not be processed.
*
*
* DescribePrincipalMapping
is currently not supported in the Amazon Web Services GovCloud (US-West)
* region.
*
*
* @param describePrincipalMappingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePrincipalMapping operation returned by the service.
* @sample AWSkendraAsyncHandler.DescribePrincipalMapping
* @see AWS API Documentation
*/
java.util.concurrent.Future describePrincipalMappingAsync(DescribePrincipalMappingRequest describePrincipalMappingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about a block list used for query suggestions for an index.
*
*
* This is used to check the current settings that are applied to a block list.
*
*
* DescribeQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param describeQuerySuggestionsBlockListRequest
* @return A Java Future containing the result of the DescribeQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsync.DescribeQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future describeQuerySuggestionsBlockListAsync(
DescribeQuerySuggestionsBlockListRequest describeQuerySuggestionsBlockListRequest);
/**
*
* Gets information about a block list used for query suggestions for an index.
*
*
* This is used to check the current settings that are applied to a block list.
*
*
* DescribeQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param describeQuerySuggestionsBlockListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsyncHandler.DescribeQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future describeQuerySuggestionsBlockListAsync(
DescribeQuerySuggestionsBlockListRequest describeQuerySuggestionsBlockListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information on the settings of query suggestions for an index.
*
*
* This is used to check the current settings applied to query suggestions.
*
*
* DescribeQuerySuggestionsConfig
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param describeQuerySuggestionsConfigRequest
* @return A Java Future containing the result of the DescribeQuerySuggestionsConfig operation returned by the
* service.
* @sample AWSkendraAsync.DescribeQuerySuggestionsConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future describeQuerySuggestionsConfigAsync(
DescribeQuerySuggestionsConfigRequest describeQuerySuggestionsConfigRequest);
/**
*
* Gets information on the settings of query suggestions for an index.
*
*
* This is used to check the current settings applied to query suggestions.
*
*
* DescribeQuerySuggestionsConfig
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param describeQuerySuggestionsConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeQuerySuggestionsConfig operation returned by the
* service.
* @sample AWSkendraAsyncHandler.DescribeQuerySuggestionsConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future describeQuerySuggestionsConfigAsync(
DescribeQuerySuggestionsConfigRequest describeQuerySuggestionsConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about an Amazon Kendra thesaurus.
*
*
* @param describeThesaurusRequest
* @return A Java Future containing the result of the DescribeThesaurus operation returned by the service.
* @sample AWSkendraAsync.DescribeThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeThesaurusAsync(DescribeThesaurusRequest describeThesaurusRequest);
/**
*
* Gets information about an Amazon Kendra thesaurus.
*
*
* @param describeThesaurusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeThesaurus operation returned by the service.
* @sample AWSkendraAsyncHandler.DescribeThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeThesaurusAsync(DescribeThesaurusRequest describeThesaurusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Prevents users or groups in your IAM Identity Center identity source from accessing your Amazon Kendra
* experience. You can create an Amazon Kendra experience such as a search application. For more information on
* creating a search application experience, see Building a search
* experience with no code.
*
*
* @param disassociateEntitiesFromExperienceRequest
* @return A Java Future containing the result of the DisassociateEntitiesFromExperience operation returned by the
* service.
* @sample AWSkendraAsync.DisassociateEntitiesFromExperience
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateEntitiesFromExperienceAsync(
DisassociateEntitiesFromExperienceRequest disassociateEntitiesFromExperienceRequest);
/**
*
* Prevents users or groups in your IAM Identity Center identity source from accessing your Amazon Kendra
* experience. You can create an Amazon Kendra experience such as a search application. For more information on
* creating a search application experience, see Building a search
* experience with no code.
*
*
* @param disassociateEntitiesFromExperienceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateEntitiesFromExperience operation returned by the
* service.
* @sample AWSkendraAsyncHandler.DisassociateEntitiesFromExperience
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateEntitiesFromExperienceAsync(
DisassociateEntitiesFromExperienceRequest disassociateEntitiesFromExperienceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specific permissions of users or groups in your IAM Identity Center identity source with access to
* your Amazon Kendra experience. You can create an Amazon Kendra experience such as a search application. For more
* information on creating a search application experience, see Building a search
* experience with no code.
*
*
* @param disassociatePersonasFromEntitiesRequest
* @return A Java Future containing the result of the DisassociatePersonasFromEntities operation returned by the
* service.
* @sample AWSkendraAsync.DisassociatePersonasFromEntities
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePersonasFromEntitiesAsync(
DisassociatePersonasFromEntitiesRequest disassociatePersonasFromEntitiesRequest);
/**
*
* Removes the specific permissions of users or groups in your IAM Identity Center identity source with access to
* your Amazon Kendra experience. You can create an Amazon Kendra experience such as a search application. For more
* information on creating a search application experience, see Building a search
* experience with no code.
*
*
* @param disassociatePersonasFromEntitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociatePersonasFromEntities operation returned by the
* service.
* @sample AWSkendraAsyncHandler.DisassociatePersonasFromEntities
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePersonasFromEntitiesAsync(
DisassociatePersonasFromEntitiesRequest disassociatePersonasFromEntitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Fetches the queries that are suggested to your users.
*
*
* GetQuerySuggestions
is currently not supported in the Amazon Web Services GovCloud (US-West) region.
*
*
* @param getQuerySuggestionsRequest
* @return A Java Future containing the result of the GetQuerySuggestions operation returned by the service.
* @sample AWSkendraAsync.GetQuerySuggestions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQuerySuggestionsAsync(GetQuerySuggestionsRequest getQuerySuggestionsRequest);
/**
*
* Fetches the queries that are suggested to your users.
*
*
* GetQuerySuggestions
is currently not supported in the Amazon Web Services GovCloud (US-West) region.
*
*
* @param getQuerySuggestionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQuerySuggestions operation returned by the service.
* @sample AWSkendraAsyncHandler.GetQuerySuggestions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQuerySuggestionsAsync(GetQuerySuggestionsRequest getQuerySuggestionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves search metrics data. The data provides a snapshot of how your users interact with your search
* application and how effective the application is.
*
*
* @param getSnapshotsRequest
* @return A Java Future containing the result of the GetSnapshots operation returned by the service.
* @sample AWSkendraAsync.GetSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSnapshotsAsync(GetSnapshotsRequest getSnapshotsRequest);
/**
*
* Retrieves search metrics data. The data provides a snapshot of how your users interact with your search
* application and how effective the application is.
*
*
* @param getSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSnapshots operation returned by the service.
* @sample AWSkendraAsyncHandler.GetSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSnapshotsAsync(GetSnapshotsRequest getSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists one or more access control configurations for an index. This includes user and group access information for
* your documents. This is useful for user context filtering, where search results are filtered based on the user or
* their group access to documents.
*
*
* @param listAccessControlConfigurationsRequest
* @return A Java Future containing the result of the ListAccessControlConfigurations operation returned by the
* service.
* @sample AWSkendraAsync.ListAccessControlConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAccessControlConfigurationsAsync(
ListAccessControlConfigurationsRequest listAccessControlConfigurationsRequest);
/**
*
* Lists one or more access control configurations for an index. This includes user and group access information for
* your documents. This is useful for user context filtering, where search results are filtered based on the user or
* their group access to documents.
*
*
* @param listAccessControlConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAccessControlConfigurations operation returned by the
* service.
* @sample AWSkendraAsyncHandler.ListAccessControlConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAccessControlConfigurationsAsync(
ListAccessControlConfigurationsRequest listAccessControlConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets statistics about synchronizing a data source connector.
*
*
* @param listDataSourceSyncJobsRequest
* @return A Java Future containing the result of the ListDataSourceSyncJobs operation returned by the service.
* @sample AWSkendraAsync.ListDataSourceSyncJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDataSourceSyncJobsAsync(ListDataSourceSyncJobsRequest listDataSourceSyncJobsRequest);
/**
*
* Gets statistics about synchronizing a data source connector.
*
*
* @param listDataSourceSyncJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSourceSyncJobs operation returned by the service.
* @sample AWSkendraAsyncHandler.ListDataSourceSyncJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDataSourceSyncJobsAsync(ListDataSourceSyncJobsRequest listDataSourceSyncJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the data source connectors that you have created.
*
*
* @param listDataSourcesRequest
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AWSkendraAsync.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* Lists the data source connectors that you have created.
*
*
* @param listDataSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AWSkendraAsyncHandler.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists specific permissions of users and groups with access to your Amazon Kendra experience.
*
*
* @param listEntityPersonasRequest
* @return A Java Future containing the result of the ListEntityPersonas operation returned by the service.
* @sample AWSkendraAsync.ListEntityPersonas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEntityPersonasAsync(ListEntityPersonasRequest listEntityPersonasRequest);
/**
*
* Lists specific permissions of users and groups with access to your Amazon Kendra experience.
*
*
* @param listEntityPersonasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEntityPersonas operation returned by the service.
* @sample AWSkendraAsyncHandler.ListEntityPersonas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEntityPersonasAsync(ListEntityPersonasRequest listEntityPersonasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists users or groups in your IAM Identity Center identity source that are granted access to your Amazon Kendra
* experience. You can create an Amazon Kendra experience such as a search application. For more information on
* creating a search application experience, see Building a search
* experience with no code.
*
*
* @param listExperienceEntitiesRequest
* @return A Java Future containing the result of the ListExperienceEntities operation returned by the service.
* @sample AWSkendraAsync.ListExperienceEntities
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listExperienceEntitiesAsync(ListExperienceEntitiesRequest listExperienceEntitiesRequest);
/**
*
* Lists users or groups in your IAM Identity Center identity source that are granted access to your Amazon Kendra
* experience. You can create an Amazon Kendra experience such as a search application. For more information on
* creating a search application experience, see Building a search
* experience with no code.
*
*
* @param listExperienceEntitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListExperienceEntities operation returned by the service.
* @sample AWSkendraAsyncHandler.ListExperienceEntities
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listExperienceEntitiesAsync(ListExperienceEntitiesRequest listExperienceEntitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists one or more Amazon Kendra experiences. You can create an Amazon Kendra experience such as a search
* application. For more information on creating a search application experience, see Building a search
* experience with no code.
*
*
* @param listExperiencesRequest
* @return A Java Future containing the result of the ListExperiences operation returned by the service.
* @sample AWSkendraAsync.ListExperiences
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExperiencesAsync(ListExperiencesRequest listExperiencesRequest);
/**
*
* Lists one or more Amazon Kendra experiences. You can create an Amazon Kendra experience such as a search
* application. For more information on creating a search application experience, see Building a search
* experience with no code.
*
*
* @param listExperiencesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListExperiences operation returned by the service.
* @sample AWSkendraAsyncHandler.ListExperiences
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExperiencesAsync(ListExperiencesRequest listExperiencesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of FAQ lists associated with an index.
*
*
* @param listFaqsRequest
* @return A Java Future containing the result of the ListFaqs operation returned by the service.
* @sample AWSkendraAsync.ListFaqs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFaqsAsync(ListFaqsRequest listFaqsRequest);
/**
*
* Gets a list of FAQ lists associated with an index.
*
*
* @param listFaqsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFaqs operation returned by the service.
* @sample AWSkendraAsyncHandler.ListFaqs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFaqsAsync(ListFaqsRequest listFaqsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all your sets of featured results for a given index. Features results are placed above all other results
* for certain queries. If there's an exact match of a query, then one or more specific documents are featured in
* the search results.
*
*
* @param listFeaturedResultsSetsRequest
* @return A Java Future containing the result of the ListFeaturedResultsSets operation returned by the service.
* @sample AWSkendraAsync.ListFeaturedResultsSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFeaturedResultsSetsAsync(ListFeaturedResultsSetsRequest listFeaturedResultsSetsRequest);
/**
*
* Lists all your sets of featured results for a given index. Features results are placed above all other results
* for certain queries. If there's an exact match of a query, then one or more specific documents are featured in
* the search results.
*
*
* @param listFeaturedResultsSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFeaturedResultsSets operation returned by the service.
* @sample AWSkendraAsyncHandler.ListFeaturedResultsSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFeaturedResultsSetsAsync(ListFeaturedResultsSetsRequest listFeaturedResultsSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of groups that are mapped to users before a given ordering or timestamp identifier.
*
*
* ListGroupsOlderThanOrderingId
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param listGroupsOlderThanOrderingIdRequest
* @return A Java Future containing the result of the ListGroupsOlderThanOrderingId operation returned by the
* service.
* @sample AWSkendraAsync.ListGroupsOlderThanOrderingId
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroupsOlderThanOrderingIdAsync(
ListGroupsOlderThanOrderingIdRequest listGroupsOlderThanOrderingIdRequest);
/**
*
* Provides a list of groups that are mapped to users before a given ordering or timestamp identifier.
*
*
* ListGroupsOlderThanOrderingId
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param listGroupsOlderThanOrderingIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroupsOlderThanOrderingId operation returned by the
* service.
* @sample AWSkendraAsyncHandler.ListGroupsOlderThanOrderingId
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroupsOlderThanOrderingIdAsync(
ListGroupsOlderThanOrderingIdRequest listGroupsOlderThanOrderingIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Amazon Kendra indexes that you created.
*
*
* @param listIndicesRequest
* @return A Java Future containing the result of the ListIndices operation returned by the service.
* @sample AWSkendraAsync.ListIndices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIndicesAsync(ListIndicesRequest listIndicesRequest);
/**
*
* Lists the Amazon Kendra indexes that you created.
*
*
* @param listIndicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIndices operation returned by the service.
* @sample AWSkendraAsyncHandler.ListIndices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIndicesAsync(ListIndicesRequest listIndicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the block lists used for query suggestions for an index.
*
*
* For information on the current quota limits for block lists, see Quotas for Amazon Kendra.
*
*
* ListQuerySuggestionsBlockLists
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param listQuerySuggestionsBlockListsRequest
* @return A Java Future containing the result of the ListQuerySuggestionsBlockLists operation returned by the
* service.
* @sample AWSkendraAsync.ListQuerySuggestionsBlockLists
* @see AWS API Documentation
*/
java.util.concurrent.Future listQuerySuggestionsBlockListsAsync(
ListQuerySuggestionsBlockListsRequest listQuerySuggestionsBlockListsRequest);
/**
*
* Lists the block lists used for query suggestions for an index.
*
*
* For information on the current quota limits for block lists, see Quotas for Amazon Kendra.
*
*
* ListQuerySuggestionsBlockLists
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param listQuerySuggestionsBlockListsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListQuerySuggestionsBlockLists operation returned by the
* service.
* @sample AWSkendraAsyncHandler.ListQuerySuggestionsBlockLists
* @see AWS API Documentation
*/
java.util.concurrent.Future listQuerySuggestionsBlockListsAsync(
ListQuerySuggestionsBlockListsRequest listQuerySuggestionsBlockListsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of tags associated with a specified resource. Indexes, FAQs, and data sources can have tags
* associated with them.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSkendraAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Gets a list of tags associated with a specified resource. Indexes, FAQs, and data sources can have tags
* associated with them.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSkendraAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the thesauri for an index.
*
*
* @param listThesauriRequest
* @return A Java Future containing the result of the ListThesauri operation returned by the service.
* @sample AWSkendraAsync.ListThesauri
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listThesauriAsync(ListThesauriRequest listThesauriRequest);
/**
*
* Lists the thesauri for an index.
*
*
* @param listThesauriRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListThesauri operation returned by the service.
* @sample AWSkendraAsyncHandler.ListThesauri
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listThesauriAsync(ListThesauriRequest listThesauriRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Maps users to their groups so that you only need to provide the user ID when you issue the query.
*
*
* You can also map sub groups to groups. For example, the group "Company Intellectual Property Teams" includes sub
* groups "Research" and "Engineering". These sub groups include their own list of users or people who work in these
* teams. Only users who work in research and engineering, and therefore belong in the intellectual property group,
* can see top-secret company documents in their search results.
*
*
* This is useful for user context filtering, where search results are filtered based on the user or their group
* access to documents. For more information, see Filtering on user context.
*
*
* If more than five PUT
actions for a group are currently processing, a validation exception is
* thrown.
*
*
* @param putPrincipalMappingRequest
* @return A Java Future containing the result of the PutPrincipalMapping operation returned by the service.
* @sample AWSkendraAsync.PutPrincipalMapping
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putPrincipalMappingAsync(PutPrincipalMappingRequest putPrincipalMappingRequest);
/**
*
* Maps users to their groups so that you only need to provide the user ID when you issue the query.
*
*
* You can also map sub groups to groups. For example, the group "Company Intellectual Property Teams" includes sub
* groups "Research" and "Engineering". These sub groups include their own list of users or people who work in these
* teams. Only users who work in research and engineering, and therefore belong in the intellectual property group,
* can see top-secret company documents in their search results.
*
*
* This is useful for user context filtering, where search results are filtered based on the user or their group
* access to documents. For more information, see Filtering on user context.
*
*
* If more than five PUT
actions for a group are currently processing, a validation exception is
* thrown.
*
*
* @param putPrincipalMappingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutPrincipalMapping operation returned by the service.
* @sample AWSkendraAsyncHandler.PutPrincipalMapping
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putPrincipalMappingAsync(PutPrincipalMappingRequest putPrincipalMappingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches an index given an input query.
*
*
*
* If you are working with large language models (LLMs) or implementing retrieval augmented generation (RAG)
* systems, you can use Amazon Kendra's Retrieve API, which can
* return longer semantically relevant passages. We recommend using the Retrieve
API instead of filing
* a service limit increase to increase the Query
API document excerpt length.
*
*
*
* You can configure boosting or relevance tuning at the query level to override boosting at the index level, filter
* based on document fields/attributes and faceted search, and filter based on the user or their group access to
* documents. You can also include certain fields in the response that might provide useful additional information.
*
*
* A query response contains three types of results.
*
*
* -
*
* Relevant suggested answers. The answers can be either a text excerpt or table excerpt. The answer can be
* highlighted in the excerpt.
*
*
* -
*
* Matching FAQs or questions-answer from your FAQ file.
*
*
* -
*
* Relevant documents. This result type includes an excerpt of the document with the document title. The searched
* terms can be highlighted in the excerpt.
*
*
*
*
* You can specify that the query return only one type of result using the QueryResultTypeFilter
* parameter. Each query returns the 100 most relevant results. If you filter result type to only question-answers,
* a maximum of four results are returned. If you filter result type to only answers, a maximum of three results are
* returned.
*
*
* @param queryRequest
* @return A Java Future containing the result of the Query operation returned by the service.
* @sample AWSkendraAsync.Query
* @see AWS API
* Documentation
*/
java.util.concurrent.Future queryAsync(QueryRequest queryRequest);
/**
*
* Searches an index given an input query.
*
*
*
* If you are working with large language models (LLMs) or implementing retrieval augmented generation (RAG)
* systems, you can use Amazon Kendra's Retrieve API, which can
* return longer semantically relevant passages. We recommend using the Retrieve
API instead of filing
* a service limit increase to increase the Query
API document excerpt length.
*
*
*
* You can configure boosting or relevance tuning at the query level to override boosting at the index level, filter
* based on document fields/attributes and faceted search, and filter based on the user or their group access to
* documents. You can also include certain fields in the response that might provide useful additional information.
*
*
* A query response contains three types of results.
*
*
* -
*
* Relevant suggested answers. The answers can be either a text excerpt or table excerpt. The answer can be
* highlighted in the excerpt.
*
*
* -
*
* Matching FAQs or questions-answer from your FAQ file.
*
*
* -
*
* Relevant documents. This result type includes an excerpt of the document with the document title. The searched
* terms can be highlighted in the excerpt.
*
*
*
*
* You can specify that the query return only one type of result using the QueryResultTypeFilter
* parameter. Each query returns the 100 most relevant results. If you filter result type to only question-answers,
* a maximum of four results are returned. If you filter result type to only answers, a maximum of three results are
* returned.
*
*
* @param queryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the Query operation returned by the service.
* @sample AWSkendraAsyncHandler.Query
* @see AWS API
* Documentation
*/
java.util.concurrent.Future queryAsync(QueryRequest queryRequest, com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves relevant passages or text excerpts given an input query.
*
*
* This API is similar to the Query API. However, by default,
* the Query
API only returns excerpt passages of up to 100 token words. With the Retrieve
* API, you can retrieve longer passages of up to 200 token words and up to 100 semantically relevant passages. This
* doesn't include question-answer or FAQ type responses from your index. The passages are text excerpts that can be
* semantically extracted from multiple documents and multiple parts of the same document. If in extreme cases your
* documents produce zero passages using the Retrieve
API, you can alternatively use the
* Query
API and its types of responses.
*
*
* You can also do the following:
*
*
* -
*
* Override boosting at the index level
*
*
* -
*
* Filter based on document fields or attributes
*
*
* -
*
* Filter based on the user or their group access to documents
*
*
* -
*
* View the confidence score bucket for a retrieved passage result. The confidence bucket provides a relative
* ranking that indicates how confident Amazon Kendra is that the response is relevant to the query.
*
*
*
* Confidence score buckets are currently available only for English.
*
*
*
*
* You can also include certain fields in the response that might provide useful additional information.
*
*
* The Retrieve
API shares the number of query capacity
* units that you set for your index. For more information on what's included in a single capacity unit and the
* default base capacity for an index, see Adjusting capacity.
*
*
* @param retrieveRequest
* @return A Java Future containing the result of the Retrieve operation returned by the service.
* @sample AWSkendraAsync.Retrieve
* @see AWS API
* Documentation
*/
java.util.concurrent.Future retrieveAsync(RetrieveRequest retrieveRequest);
/**
*
* Retrieves relevant passages or text excerpts given an input query.
*
*
* This API is similar to the Query API. However, by default,
* the Query
API only returns excerpt passages of up to 100 token words. With the Retrieve
* API, you can retrieve longer passages of up to 200 token words and up to 100 semantically relevant passages. This
* doesn't include question-answer or FAQ type responses from your index. The passages are text excerpts that can be
* semantically extracted from multiple documents and multiple parts of the same document. If in extreme cases your
* documents produce zero passages using the Retrieve
API, you can alternatively use the
* Query
API and its types of responses.
*
*
* You can also do the following:
*
*
* -
*
* Override boosting at the index level
*
*
* -
*
* Filter based on document fields or attributes
*
*
* -
*
* Filter based on the user or their group access to documents
*
*
* -
*
* View the confidence score bucket for a retrieved passage result. The confidence bucket provides a relative
* ranking that indicates how confident Amazon Kendra is that the response is relevant to the query.
*
*
*
* Confidence score buckets are currently available only for English.
*
*
*
*
* You can also include certain fields in the response that might provide useful additional information.
*
*
* The Retrieve
API shares the number of query capacity
* units that you set for your index. For more information on what's included in a single capacity unit and the
* default base capacity for an index, see Adjusting capacity.
*
*
* @param retrieveRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the Retrieve operation returned by the service.
* @sample AWSkendraAsyncHandler.Retrieve
* @see AWS API
* Documentation
*/
java.util.concurrent.Future retrieveAsync(RetrieveRequest retrieveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a synchronization job for a data source connector. If a synchronization job is already in progress, Amazon
* Kendra returns a ResourceInUseException
exception.
*
*
* Re-syncing your data source with your index after modifying, adding, or deleting documents from your data source
* respository could take up to an hour or more, depending on the number of documents to sync.
*
*
* @param startDataSourceSyncJobRequest
* @return A Java Future containing the result of the StartDataSourceSyncJob operation returned by the service.
* @sample AWSkendraAsync.StartDataSourceSyncJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startDataSourceSyncJobAsync(StartDataSourceSyncJobRequest startDataSourceSyncJobRequest);
/**
*
* Starts a synchronization job for a data source connector. If a synchronization job is already in progress, Amazon
* Kendra returns a ResourceInUseException
exception.
*
*
* Re-syncing your data source with your index after modifying, adding, or deleting documents from your data source
* respository could take up to an hour or more, depending on the number of documents to sync.
*
*
* @param startDataSourceSyncJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartDataSourceSyncJob operation returned by the service.
* @sample AWSkendraAsyncHandler.StartDataSourceSyncJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startDataSourceSyncJobAsync(StartDataSourceSyncJobRequest startDataSourceSyncJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a synchronization job that is currently running. You can't stop a scheduled synchronization job.
*
*
* @param stopDataSourceSyncJobRequest
* @return A Java Future containing the result of the StopDataSourceSyncJob operation returned by the service.
* @sample AWSkendraAsync.StopDataSourceSyncJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopDataSourceSyncJobAsync(StopDataSourceSyncJobRequest stopDataSourceSyncJobRequest);
/**
*
* Stops a synchronization job that is currently running. You can't stop a scheduled synchronization job.
*
*
* @param stopDataSourceSyncJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopDataSourceSyncJob operation returned by the service.
* @sample AWSkendraAsyncHandler.StopDataSourceSyncJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopDataSourceSyncJobAsync(StopDataSourceSyncJobRequest stopDataSourceSyncJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables you to provide feedback to Amazon Kendra to improve the performance of your index.
*
*
* SubmitFeedback
is currently not supported in the Amazon Web Services GovCloud (US-West) region.
*
*
* @param submitFeedbackRequest
* @return A Java Future containing the result of the SubmitFeedback operation returned by the service.
* @sample AWSkendraAsync.SubmitFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future submitFeedbackAsync(SubmitFeedbackRequest submitFeedbackRequest);
/**
*
* Enables you to provide feedback to Amazon Kendra to improve the performance of your index.
*
*
* SubmitFeedback
is currently not supported in the Amazon Web Services GovCloud (US-West) region.
*
*
* @param submitFeedbackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SubmitFeedback operation returned by the service.
* @sample AWSkendraAsyncHandler.SubmitFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future submitFeedbackAsync(SubmitFeedbackRequest submitFeedbackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified tag to the specified index, FAQ, or data source resource. If the tag already exists, the
* existing value is replaced with the new value.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSkendraAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds the specified tag to the specified index, FAQ, or data source resource. If the tag already exists, the
* existing value is replaced with the new value.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSkendraAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a tag from an index, FAQ, or a data source.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSkendraAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes a tag from an index, FAQ, or a data source.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSkendraAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an access control configuration for your documents in an index. This includes user and group access
* information for your documents. This is useful for user context filtering, where search results are filtered
* based on the user or their group access to documents.
*
*
* You can update an access control configuration you created without indexing all of your documents again. For
* example, your index contains top-secret company documents that only certain employees or users should access. You
* created an 'allow' access control configuration for one user who recently joined the 'top-secret' team, switching
* from a team with 'deny' access to top-secret documents. However, the user suddenly returns to their previous team
* and should no longer have access to top secret documents. You can update the access control configuration to
* re-configure access control for your documents as circumstances change.
*
*
* You call the BatchPutDocument API to apply
* the updated access control configuration, with the AccessControlConfigurationId
included in the Document object. If you use an S3
* bucket as a data source, you synchronize your data source to apply the AccessControlConfigurationId
* in the .metadata.json
file. Amazon Kendra currently only supports access control configuration for
* S3 data sources and documents indexed using the BatchPutDocument
API.
*
*
* @param updateAccessControlConfigurationRequest
* @return A Java Future containing the result of the UpdateAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsync.UpdateAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAccessControlConfigurationAsync(
UpdateAccessControlConfigurationRequest updateAccessControlConfigurationRequest);
/**
*
* Updates an access control configuration for your documents in an index. This includes user and group access
* information for your documents. This is useful for user context filtering, where search results are filtered
* based on the user or their group access to documents.
*
*
* You can update an access control configuration you created without indexing all of your documents again. For
* example, your index contains top-secret company documents that only certain employees or users should access. You
* created an 'allow' access control configuration for one user who recently joined the 'top-secret' team, switching
* from a team with 'deny' access to top-secret documents. However, the user suddenly returns to their previous team
* and should no longer have access to top secret documents. You can update the access control configuration to
* re-configure access control for your documents as circumstances change.
*
*
* You call the BatchPutDocument API to apply
* the updated access control configuration, with the AccessControlConfigurationId
included in the Document object. If you use an S3
* bucket as a data source, you synchronize your data source to apply the AccessControlConfigurationId
* in the .metadata.json
file. Amazon Kendra currently only supports access control configuration for
* S3 data sources and documents indexed using the BatchPutDocument
API.
*
*
* @param updateAccessControlConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAccessControlConfiguration operation returned by the
* service.
* @sample AWSkendraAsyncHandler.UpdateAccessControlConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAccessControlConfigurationAsync(
UpdateAccessControlConfigurationRequest updateAccessControlConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an Amazon Kendra data source connector.
*
*
* @param updateDataSourceRequest
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AWSkendraAsync.UpdateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates an Amazon Kendra data source connector.
*
*
* @param updateDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AWSkendraAsyncHandler.UpdateDataSource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates your Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, see Building a search
* experience with no code.
*
*
* @param updateExperienceRequest
* @return A Java Future containing the result of the UpdateExperience operation returned by the service.
* @sample AWSkendraAsync.UpdateExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateExperienceAsync(UpdateExperienceRequest updateExperienceRequest);
/**
*
* Updates your Amazon Kendra experience such as a search application. For more information on creating a search
* application experience, see Building a search
* experience with no code.
*
*
* @param updateExperienceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateExperience operation returned by the service.
* @sample AWSkendraAsyncHandler.UpdateExperience
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateExperienceAsync(UpdateExperienceRequest updateExperienceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a set of featured results. Features results are placed above all other results for certain queries. You
* map specific queries to specific documents for featuring in the results. If a query contains an exact match of a
* query, then one or more specific documents are featured in the search results.
*
*
* @param updateFeaturedResultsSetRequest
* @return A Java Future containing the result of the UpdateFeaturedResultsSet operation returned by the service.
* @sample AWSkendraAsync.UpdateFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFeaturedResultsSetAsync(UpdateFeaturedResultsSetRequest updateFeaturedResultsSetRequest);
/**
*
* Updates a set of featured results. Features results are placed above all other results for certain queries. You
* map specific queries to specific documents for featuring in the results. If a query contains an exact match of a
* query, then one or more specific documents are featured in the search results.
*
*
* @param updateFeaturedResultsSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFeaturedResultsSet operation returned by the service.
* @sample AWSkendraAsyncHandler.UpdateFeaturedResultsSet
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFeaturedResultsSetAsync(UpdateFeaturedResultsSetRequest updateFeaturedResultsSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an Amazon Kendra index.
*
*
* @param updateIndexRequest
* @return A Java Future containing the result of the UpdateIndex operation returned by the service.
* @sample AWSkendraAsync.UpdateIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateIndexAsync(UpdateIndexRequest updateIndexRequest);
/**
*
* Updates an Amazon Kendra index.
*
*
* @param updateIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIndex operation returned by the service.
* @sample AWSkendraAsyncHandler.UpdateIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateIndexAsync(UpdateIndexRequest updateIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a block list used for query suggestions for an index.
*
*
* Updates to a block list might not take effect right away. Amazon Kendra needs to refresh the entire suggestions
* list to apply any updates to the block list. Other changes not related to the block list apply immediately.
*
*
* If a block list is updating, then you need to wait for the first update to finish before submitting another
* update.
*
*
* Amazon Kendra supports partial updates, so you only need to provide the fields you want to update.
*
*
* UpdateQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param updateQuerySuggestionsBlockListRequest
* @return A Java Future containing the result of the UpdateQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsync.UpdateQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future updateQuerySuggestionsBlockListAsync(
UpdateQuerySuggestionsBlockListRequest updateQuerySuggestionsBlockListRequest);
/**
*
* Updates a block list used for query suggestions for an index.
*
*
* Updates to a block list might not take effect right away. Amazon Kendra needs to refresh the entire suggestions
* list to apply any updates to the block list. Other changes not related to the block list apply immediately.
*
*
* If a block list is updating, then you need to wait for the first update to finish before submitting another
* update.
*
*
* Amazon Kendra supports partial updates, so you only need to provide the fields you want to update.
*
*
* UpdateQuerySuggestionsBlockList
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param updateQuerySuggestionsBlockListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateQuerySuggestionsBlockList operation returned by the
* service.
* @sample AWSkendraAsyncHandler.UpdateQuerySuggestionsBlockList
* @see AWS API Documentation
*/
java.util.concurrent.Future updateQuerySuggestionsBlockListAsync(
UpdateQuerySuggestionsBlockListRequest updateQuerySuggestionsBlockListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the settings of query suggestions for an index.
*
*
* Amazon Kendra supports partial updates, so you only need to provide the fields you want to update.
*
*
* If an update is currently processing, you need to wait for the update to finish before making another update.
*
*
* Updates to query suggestions settings might not take effect right away. The time for your updated settings to
* take effect depends on the updates made and the number of search queries in your index.
*
*
* You can still enable/disable query suggestions at any time.
*
*
* UpdateQuerySuggestionsConfig
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param updateQuerySuggestionsConfigRequest
* @return A Java Future containing the result of the UpdateQuerySuggestionsConfig operation returned by the
* service.
* @sample AWSkendraAsync.UpdateQuerySuggestionsConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future updateQuerySuggestionsConfigAsync(
UpdateQuerySuggestionsConfigRequest updateQuerySuggestionsConfigRequest);
/**
*
* Updates the settings of query suggestions for an index.
*
*
* Amazon Kendra supports partial updates, so you only need to provide the fields you want to update.
*
*
* If an update is currently processing, you need to wait for the update to finish before making another update.
*
*
* Updates to query suggestions settings might not take effect right away. The time for your updated settings to
* take effect depends on the updates made and the number of search queries in your index.
*
*
* You can still enable/disable query suggestions at any time.
*
*
* UpdateQuerySuggestionsConfig
is currently not supported in the Amazon Web Services GovCloud
* (US-West) region.
*
*
* @param updateQuerySuggestionsConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateQuerySuggestionsConfig operation returned by the
* service.
* @sample AWSkendraAsyncHandler.UpdateQuerySuggestionsConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future updateQuerySuggestionsConfigAsync(
UpdateQuerySuggestionsConfigRequest updateQuerySuggestionsConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a thesaurus for an index.
*
*
* @param updateThesaurusRequest
* @return A Java Future containing the result of the UpdateThesaurus operation returned by the service.
* @sample AWSkendraAsync.UpdateThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateThesaurusAsync(UpdateThesaurusRequest updateThesaurusRequest);
/**
*
* Updates a thesaurus for an index.
*
*
* @param updateThesaurusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateThesaurus operation returned by the service.
* @sample AWSkendraAsyncHandler.UpdateThesaurus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateThesaurusAsync(UpdateThesaurusRequest updateThesaurusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}