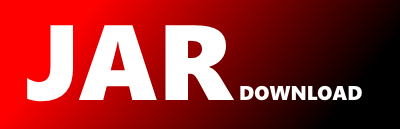
com.amazonaws.services.kendra.model.SalesforceChatterFeedConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configuration information for syncing a Salesforce chatter feed. The contents of the object comes from the
* Salesforce FeedItem table.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SalesforceChatterFeedConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the column in the Salesforce FeedItem table that contains the content to index. Typically this is the
* Body
column.
*
*/
private String documentDataFieldName;
/**
*
* The name of the column in the Salesforce FeedItem table that contains the title of the document. This is
* typically the Title
column.
*
*/
private String documentTitleFieldName;
/**
*
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
*
*/
private java.util.List fieldMappings;
/**
*
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
only
* documents from users who have an active account are indexed. When you specify STANDARD_USER
only
* documents for Salesforce standard users are documented. You can specify both.
*
*/
private java.util.List includeFilterTypes;
/**
*
* The name of the column in the Salesforce FeedItem table that contains the content to index. Typically this is the
* Body
column.
*
*
* @param documentDataFieldName
* The name of the column in the Salesforce FeedItem table that contains the content to index. Typically this
* is the Body
column.
*/
public void setDocumentDataFieldName(String documentDataFieldName) {
this.documentDataFieldName = documentDataFieldName;
}
/**
*
* The name of the column in the Salesforce FeedItem table that contains the content to index. Typically this is the
* Body
column.
*
*
* @return The name of the column in the Salesforce FeedItem table that contains the content to index. Typically
* this is the Body
column.
*/
public String getDocumentDataFieldName() {
return this.documentDataFieldName;
}
/**
*
* The name of the column in the Salesforce FeedItem table that contains the content to index. Typically this is the
* Body
column.
*
*
* @param documentDataFieldName
* The name of the column in the Salesforce FeedItem table that contains the content to index. Typically this
* is the Body
column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SalesforceChatterFeedConfiguration withDocumentDataFieldName(String documentDataFieldName) {
setDocumentDataFieldName(documentDataFieldName);
return this;
}
/**
*
* The name of the column in the Salesforce FeedItem table that contains the title of the document. This is
* typically the Title
column.
*
*
* @param documentTitleFieldName
* The name of the column in the Salesforce FeedItem table that contains the title of the document. This is
* typically the Title
column.
*/
public void setDocumentTitleFieldName(String documentTitleFieldName) {
this.documentTitleFieldName = documentTitleFieldName;
}
/**
*
* The name of the column in the Salesforce FeedItem table that contains the title of the document. This is
* typically the Title
column.
*
*
* @return The name of the column in the Salesforce FeedItem table that contains the title of the document. This is
* typically the Title
column.
*/
public String getDocumentTitleFieldName() {
return this.documentTitleFieldName;
}
/**
*
* The name of the column in the Salesforce FeedItem table that contains the title of the document. This is
* typically the Title
column.
*
*
* @param documentTitleFieldName
* The name of the column in the Salesforce FeedItem table that contains the title of the document. This is
* typically the Title
column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SalesforceChatterFeedConfiguration withDocumentTitleFieldName(String documentTitleFieldName) {
setDocumentTitleFieldName(documentTitleFieldName);
return this;
}
/**
*
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
*
*
* @return Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
*/
public java.util.List getFieldMappings() {
return fieldMappings;
}
/**
*
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
*
*
* @param fieldMappings
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
*/
public void setFieldMappings(java.util.Collection fieldMappings) {
if (fieldMappings == null) {
this.fieldMappings = null;
return;
}
this.fieldMappings = new java.util.ArrayList(fieldMappings);
}
/**
*
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFieldMappings(java.util.Collection)} or {@link #withFieldMappings(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param fieldMappings
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SalesforceChatterFeedConfiguration withFieldMappings(DataSourceToIndexFieldMapping... fieldMappings) {
if (this.fieldMappings == null) {
setFieldMappings(new java.util.ArrayList(fieldMappings.length));
}
for (DataSourceToIndexFieldMapping ele : fieldMappings) {
this.fieldMappings.add(ele);
}
return this;
}
/**
*
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
*
*
* @param fieldMappings
* Maps fields from a Salesforce chatter feed into Amazon Kendra index fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SalesforceChatterFeedConfiguration withFieldMappings(java.util.Collection fieldMappings) {
setFieldMappings(fieldMappings);
return this;
}
/**
*
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
only
* documents from users who have an active account are indexed. When you specify STANDARD_USER
only
* documents for Salesforce standard users are documented. You can specify both.
*
*
* @return Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
* only documents from users who have an active account are indexed. When you specify
* STANDARD_USER
only documents for Salesforce standard users are documented. You can specify
* both.
* @see SalesforceChatterFeedIncludeFilterType
*/
public java.util.List getIncludeFilterTypes() {
return includeFilterTypes;
}
/**
*
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
only
* documents from users who have an active account are indexed. When you specify STANDARD_USER
only
* documents for Salesforce standard users are documented. You can specify both.
*
*
* @param includeFilterTypes
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
* only documents from users who have an active account are indexed. When you specify
* STANDARD_USER
only documents for Salesforce standard users are documented. You can specify
* both.
* @see SalesforceChatterFeedIncludeFilterType
*/
public void setIncludeFilterTypes(java.util.Collection includeFilterTypes) {
if (includeFilterTypes == null) {
this.includeFilterTypes = null;
return;
}
this.includeFilterTypes = new java.util.ArrayList(includeFilterTypes);
}
/**
*
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
only
* documents from users who have an active account are indexed. When you specify STANDARD_USER
only
* documents for Salesforce standard users are documented. You can specify both.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIncludeFilterTypes(java.util.Collection)} or {@link #withIncludeFilterTypes(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param includeFilterTypes
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
* only documents from users who have an active account are indexed. When you specify
* STANDARD_USER
only documents for Salesforce standard users are documented. You can specify
* both.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SalesforceChatterFeedIncludeFilterType
*/
public SalesforceChatterFeedConfiguration withIncludeFilterTypes(String... includeFilterTypes) {
if (this.includeFilterTypes == null) {
setIncludeFilterTypes(new java.util.ArrayList(includeFilterTypes.length));
}
for (String ele : includeFilterTypes) {
this.includeFilterTypes.add(ele);
}
return this;
}
/**
*
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
only
* documents from users who have an active account are indexed. When you specify STANDARD_USER
only
* documents for Salesforce standard users are documented. You can specify both.
*
*
* @param includeFilterTypes
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
* only documents from users who have an active account are indexed. When you specify
* STANDARD_USER
only documents for Salesforce standard users are documented. You can specify
* both.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SalesforceChatterFeedIncludeFilterType
*/
public SalesforceChatterFeedConfiguration withIncludeFilterTypes(java.util.Collection includeFilterTypes) {
setIncludeFilterTypes(includeFilterTypes);
return this;
}
/**
*
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
only
* documents from users who have an active account are indexed. When you specify STANDARD_USER
only
* documents for Salesforce standard users are documented. You can specify both.
*
*
* @param includeFilterTypes
* Filters the documents in the feed based on status of the user. When you specify ACTIVE_USERS
* only documents from users who have an active account are indexed. When you specify
* STANDARD_USER
only documents for Salesforce standard users are documented. You can specify
* both.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SalesforceChatterFeedIncludeFilterType
*/
public SalesforceChatterFeedConfiguration withIncludeFilterTypes(SalesforceChatterFeedIncludeFilterType... includeFilterTypes) {
java.util.ArrayList includeFilterTypesCopy = new java.util.ArrayList(includeFilterTypes.length);
for (SalesforceChatterFeedIncludeFilterType value : includeFilterTypes) {
includeFilterTypesCopy.add(value.toString());
}
if (getIncludeFilterTypes() == null) {
setIncludeFilterTypes(includeFilterTypesCopy);
} else {
getIncludeFilterTypes().addAll(includeFilterTypesCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDocumentDataFieldName() != null)
sb.append("DocumentDataFieldName: ").append(getDocumentDataFieldName()).append(",");
if (getDocumentTitleFieldName() != null)
sb.append("DocumentTitleFieldName: ").append(getDocumentTitleFieldName()).append(",");
if (getFieldMappings() != null)
sb.append("FieldMappings: ").append(getFieldMappings()).append(",");
if (getIncludeFilterTypes() != null)
sb.append("IncludeFilterTypes: ").append(getIncludeFilterTypes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SalesforceChatterFeedConfiguration == false)
return false;
SalesforceChatterFeedConfiguration other = (SalesforceChatterFeedConfiguration) obj;
if (other.getDocumentDataFieldName() == null ^ this.getDocumentDataFieldName() == null)
return false;
if (other.getDocumentDataFieldName() != null && other.getDocumentDataFieldName().equals(this.getDocumentDataFieldName()) == false)
return false;
if (other.getDocumentTitleFieldName() == null ^ this.getDocumentTitleFieldName() == null)
return false;
if (other.getDocumentTitleFieldName() != null && other.getDocumentTitleFieldName().equals(this.getDocumentTitleFieldName()) == false)
return false;
if (other.getFieldMappings() == null ^ this.getFieldMappings() == null)
return false;
if (other.getFieldMappings() != null && other.getFieldMappings().equals(this.getFieldMappings()) == false)
return false;
if (other.getIncludeFilterTypes() == null ^ this.getIncludeFilterTypes() == null)
return false;
if (other.getIncludeFilterTypes() != null && other.getIncludeFilterTypes().equals(this.getIncludeFilterTypes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDocumentDataFieldName() == null) ? 0 : getDocumentDataFieldName().hashCode());
hashCode = prime * hashCode + ((getDocumentTitleFieldName() == null) ? 0 : getDocumentTitleFieldName().hashCode());
hashCode = prime * hashCode + ((getFieldMappings() == null) ? 0 : getFieldMappings().hashCode());
hashCode = prime * hashCode + ((getIncludeFilterTypes() == null) ? 0 : getIncludeFilterTypes().hashCode());
return hashCode;
}
@Override
public SalesforceChatterFeedConfiguration clone() {
try {
return (SalesforceChatterFeedConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.SalesforceChatterFeedConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}