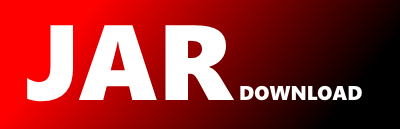
com.amazonaws.services.kendra.model.ServiceNowKnowledgeArticleConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides the configuration information for crawling knowledge articles in the ServiceNow site.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ServiceNowKnowledgeArticleConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* TRUE
to index attachments to knowledge articles.
*
*/
private Boolean crawlAttachments;
/**
*
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that match
* the patterns are included in the index. Items that don't match the patterns are excluded from the index. If an
* item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item isn't
* included in the index.
*
*/
private java.util.List includeAttachmentFilePatterns;
/**
*
* A list of regular expression patterns applied to exclude certain knowledge article attachments. Attachments that
* match the patterns are excluded from the index. Items that don't match the patterns are included in the index. If
* an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item
* isn't included in the index.
*
*/
private java.util.List excludeAttachmentFilePatterns;
/**
*
* The name of the ServiceNow field that is mapped to the index document contents field in the Amazon Kendra index.
*
*/
private String documentDataFieldName;
/**
*
* The name of the ServiceNow field that is mapped to the index document title field.
*
*/
private String documentTitleFieldName;
/**
*
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom fields,
* use the UpdateIndex
API before you map to ServiceNow fields. For more information, see Mapping data source fields. The
* ServiceNow data source field names must exist in your ServiceNow custom metadata.
*
*/
private java.util.List fieldMappings;
/**
*
* A query that selects the knowledge articles to index. The query can return articles from multiple knowledge
* bases, and the knowledge bases can be public or private.
*
*
* The query string must be one generated by the ServiceNow console. For more information, see Specifying documents to index with a
* query.
*
*/
private String filterQuery;
/**
*
* TRUE
to index attachments to knowledge articles.
*
*
* @param crawlAttachments
* TRUE
to index attachments to knowledge articles.
*/
public void setCrawlAttachments(Boolean crawlAttachments) {
this.crawlAttachments = crawlAttachments;
}
/**
*
* TRUE
to index attachments to knowledge articles.
*
*
* @return TRUE
to index attachments to knowledge articles.
*/
public Boolean getCrawlAttachments() {
return this.crawlAttachments;
}
/**
*
* TRUE
to index attachments to knowledge articles.
*
*
* @param crawlAttachments
* TRUE
to index attachments to knowledge articles.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withCrawlAttachments(Boolean crawlAttachments) {
setCrawlAttachments(crawlAttachments);
return this;
}
/**
*
* TRUE
to index attachments to knowledge articles.
*
*
* @return TRUE
to index attachments to knowledge articles.
*/
public Boolean isCrawlAttachments() {
return this.crawlAttachments;
}
/**
*
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that match
* the patterns are included in the index. Items that don't match the patterns are excluded from the index. If an
* item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item isn't
* included in the index.
*
*
* @return A list of regular expression patterns applied to include knowledge article attachments. Attachments that
* match the patterns are included in the index. Items that don't match the patterns are excluded from the
* index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the item isn't included in the index.
*/
public java.util.List getIncludeAttachmentFilePatterns() {
return includeAttachmentFilePatterns;
}
/**
*
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that match
* the patterns are included in the index. Items that don't match the patterns are excluded from the index. If an
* item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item isn't
* included in the index.
*
*
* @param includeAttachmentFilePatterns
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that
* match the patterns are included in the index. Items that don't match the patterns are excluded from the
* index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the item isn't included in the index.
*/
public void setIncludeAttachmentFilePatterns(java.util.Collection includeAttachmentFilePatterns) {
if (includeAttachmentFilePatterns == null) {
this.includeAttachmentFilePatterns = null;
return;
}
this.includeAttachmentFilePatterns = new java.util.ArrayList(includeAttachmentFilePatterns);
}
/**
*
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that match
* the patterns are included in the index. Items that don't match the patterns are excluded from the index. If an
* item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item isn't
* included in the index.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIncludeAttachmentFilePatterns(java.util.Collection)} or
* {@link #withIncludeAttachmentFilePatterns(java.util.Collection)} if you want to override the existing values.
*
*
* @param includeAttachmentFilePatterns
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that
* match the patterns are included in the index. Items that don't match the patterns are excluded from the
* index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the item isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withIncludeAttachmentFilePatterns(String... includeAttachmentFilePatterns) {
if (this.includeAttachmentFilePatterns == null) {
setIncludeAttachmentFilePatterns(new java.util.ArrayList(includeAttachmentFilePatterns.length));
}
for (String ele : includeAttachmentFilePatterns) {
this.includeAttachmentFilePatterns.add(ele);
}
return this;
}
/**
*
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that match
* the patterns are included in the index. Items that don't match the patterns are excluded from the index. If an
* item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item isn't
* included in the index.
*
*
* @param includeAttachmentFilePatterns
* A list of regular expression patterns applied to include knowledge article attachments. Attachments that
* match the patterns are included in the index. Items that don't match the patterns are excluded from the
* index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence
* and the item isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withIncludeAttachmentFilePatterns(java.util.Collection includeAttachmentFilePatterns) {
setIncludeAttachmentFilePatterns(includeAttachmentFilePatterns);
return this;
}
/**
*
* A list of regular expression patterns applied to exclude certain knowledge article attachments. Attachments that
* match the patterns are excluded from the index. Items that don't match the patterns are included in the index. If
* an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item
* isn't included in the index.
*
*
* @return A list of regular expression patterns applied to exclude certain knowledge article attachments.
* Attachments that match the patterns are excluded from the index. Items that don't match the patterns are
* included in the index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the item isn't included in the index.
*/
public java.util.List getExcludeAttachmentFilePatterns() {
return excludeAttachmentFilePatterns;
}
/**
*
* A list of regular expression patterns applied to exclude certain knowledge article attachments. Attachments that
* match the patterns are excluded from the index. Items that don't match the patterns are included in the index. If
* an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item
* isn't included in the index.
*
*
* @param excludeAttachmentFilePatterns
* A list of regular expression patterns applied to exclude certain knowledge article attachments.
* Attachments that match the patterns are excluded from the index. Items that don't match the patterns are
* included in the index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the item isn't included in the index.
*/
public void setExcludeAttachmentFilePatterns(java.util.Collection excludeAttachmentFilePatterns) {
if (excludeAttachmentFilePatterns == null) {
this.excludeAttachmentFilePatterns = null;
return;
}
this.excludeAttachmentFilePatterns = new java.util.ArrayList(excludeAttachmentFilePatterns);
}
/**
*
* A list of regular expression patterns applied to exclude certain knowledge article attachments. Attachments that
* match the patterns are excluded from the index. Items that don't match the patterns are included in the index. If
* an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item
* isn't included in the index.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExcludeAttachmentFilePatterns(java.util.Collection)} or
* {@link #withExcludeAttachmentFilePatterns(java.util.Collection)} if you want to override the existing values.
*
*
* @param excludeAttachmentFilePatterns
* A list of regular expression patterns applied to exclude certain knowledge article attachments.
* Attachments that match the patterns are excluded from the index. Items that don't match the patterns are
* included in the index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the item isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withExcludeAttachmentFilePatterns(String... excludeAttachmentFilePatterns) {
if (this.excludeAttachmentFilePatterns == null) {
setExcludeAttachmentFilePatterns(new java.util.ArrayList(excludeAttachmentFilePatterns.length));
}
for (String ele : excludeAttachmentFilePatterns) {
this.excludeAttachmentFilePatterns.add(ele);
}
return this;
}
/**
*
* A list of regular expression patterns applied to exclude certain knowledge article attachments. Attachments that
* match the patterns are excluded from the index. Items that don't match the patterns are included in the index. If
* an item matches both an inclusion and exclusion pattern, the exclusion pattern takes precedence and the item
* isn't included in the index.
*
*
* @param excludeAttachmentFilePatterns
* A list of regular expression patterns applied to exclude certain knowledge article attachments.
* Attachments that match the patterns are excluded from the index. Items that don't match the patterns are
* included in the index. If an item matches both an inclusion and exclusion pattern, the exclusion pattern
* takes precedence and the item isn't included in the index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withExcludeAttachmentFilePatterns(java.util.Collection excludeAttachmentFilePatterns) {
setExcludeAttachmentFilePatterns(excludeAttachmentFilePatterns);
return this;
}
/**
*
* The name of the ServiceNow field that is mapped to the index document contents field in the Amazon Kendra index.
*
*
* @param documentDataFieldName
* The name of the ServiceNow field that is mapped to the index document contents field in the Amazon Kendra
* index.
*/
public void setDocumentDataFieldName(String documentDataFieldName) {
this.documentDataFieldName = documentDataFieldName;
}
/**
*
* The name of the ServiceNow field that is mapped to the index document contents field in the Amazon Kendra index.
*
*
* @return The name of the ServiceNow field that is mapped to the index document contents field in the Amazon Kendra
* index.
*/
public String getDocumentDataFieldName() {
return this.documentDataFieldName;
}
/**
*
* The name of the ServiceNow field that is mapped to the index document contents field in the Amazon Kendra index.
*
*
* @param documentDataFieldName
* The name of the ServiceNow field that is mapped to the index document contents field in the Amazon Kendra
* index.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withDocumentDataFieldName(String documentDataFieldName) {
setDocumentDataFieldName(documentDataFieldName);
return this;
}
/**
*
* The name of the ServiceNow field that is mapped to the index document title field.
*
*
* @param documentTitleFieldName
* The name of the ServiceNow field that is mapped to the index document title field.
*/
public void setDocumentTitleFieldName(String documentTitleFieldName) {
this.documentTitleFieldName = documentTitleFieldName;
}
/**
*
* The name of the ServiceNow field that is mapped to the index document title field.
*
*
* @return The name of the ServiceNow field that is mapped to the index document title field.
*/
public String getDocumentTitleFieldName() {
return this.documentTitleFieldName;
}
/**
*
* The name of the ServiceNow field that is mapped to the index document title field.
*
*
* @param documentTitleFieldName
* The name of the ServiceNow field that is mapped to the index document title field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withDocumentTitleFieldName(String documentTitleFieldName) {
setDocumentTitleFieldName(documentTitleFieldName);
return this;
}
/**
*
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom fields,
* use the UpdateIndex
API before you map to ServiceNow fields. For more information, see Mapping data source fields. The
* ServiceNow data source field names must exist in your ServiceNow custom metadata.
*
*
* @return Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom
* fields, use the UpdateIndex
API before you map to ServiceNow fields. For more information,
* see Mapping data source
* fields. The ServiceNow data source field names must exist in your ServiceNow custom metadata.
*/
public java.util.List getFieldMappings() {
return fieldMappings;
}
/**
*
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom fields,
* use the UpdateIndex
API before you map to ServiceNow fields. For more information, see Mapping data source fields. The
* ServiceNow data source field names must exist in your ServiceNow custom metadata.
*
*
* @param fieldMappings
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom
* fields, use the UpdateIndex
API before you map to ServiceNow fields. For more information,
* see Mapping data source
* fields. The ServiceNow data source field names must exist in your ServiceNow custom metadata.
*/
public void setFieldMappings(java.util.Collection fieldMappings) {
if (fieldMappings == null) {
this.fieldMappings = null;
return;
}
this.fieldMappings = new java.util.ArrayList(fieldMappings);
}
/**
*
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom fields,
* use the UpdateIndex
API before you map to ServiceNow fields. For more information, see Mapping data source fields. The
* ServiceNow data source field names must exist in your ServiceNow custom metadata.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFieldMappings(java.util.Collection)} or {@link #withFieldMappings(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param fieldMappings
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom
* fields, use the UpdateIndex
API before you map to ServiceNow fields. For more information,
* see Mapping data source
* fields. The ServiceNow data source field names must exist in your ServiceNow custom metadata.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withFieldMappings(DataSourceToIndexFieldMapping... fieldMappings) {
if (this.fieldMappings == null) {
setFieldMappings(new java.util.ArrayList(fieldMappings.length));
}
for (DataSourceToIndexFieldMapping ele : fieldMappings) {
this.fieldMappings.add(ele);
}
return this;
}
/**
*
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom fields,
* use the UpdateIndex
API before you map to ServiceNow fields. For more information, see Mapping data source fields. The
* ServiceNow data source field names must exist in your ServiceNow custom metadata.
*
*
* @param fieldMappings
* Maps attributes or field names of knoweldge articles to Amazon Kendra index field names. To create custom
* fields, use the UpdateIndex
API before you map to ServiceNow fields. For more information,
* see Mapping data source
* fields. The ServiceNow data source field names must exist in your ServiceNow custom metadata.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withFieldMappings(java.util.Collection fieldMappings) {
setFieldMappings(fieldMappings);
return this;
}
/**
*
* A query that selects the knowledge articles to index. The query can return articles from multiple knowledge
* bases, and the knowledge bases can be public or private.
*
*
* The query string must be one generated by the ServiceNow console. For more information, see Specifying documents to index with a
* query.
*
*
* @param filterQuery
* A query that selects the knowledge articles to index. The query can return articles from multiple
* knowledge bases, and the knowledge bases can be public or private.
*
* The query string must be one generated by the ServiceNow console. For more information, see Specifying documents to index
* with a query.
*/
public void setFilterQuery(String filterQuery) {
this.filterQuery = filterQuery;
}
/**
*
* A query that selects the knowledge articles to index. The query can return articles from multiple knowledge
* bases, and the knowledge bases can be public or private.
*
*
* The query string must be one generated by the ServiceNow console. For more information, see Specifying documents to index with a
* query.
*
*
* @return A query that selects the knowledge articles to index. The query can return articles from multiple
* knowledge bases, and the knowledge bases can be public or private.
*
* The query string must be one generated by the ServiceNow console. For more information, see Specifying documents to index
* with a query.
*/
public String getFilterQuery() {
return this.filterQuery;
}
/**
*
* A query that selects the knowledge articles to index. The query can return articles from multiple knowledge
* bases, and the knowledge bases can be public or private.
*
*
* The query string must be one generated by the ServiceNow console. For more information, see Specifying documents to index with a
* query.
*
*
* @param filterQuery
* A query that selects the knowledge articles to index. The query can return articles from multiple
* knowledge bases, and the knowledge bases can be public or private.
*
* The query string must be one generated by the ServiceNow console. For more information, see Specifying documents to index
* with a query.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowKnowledgeArticleConfiguration withFilterQuery(String filterQuery) {
setFilterQuery(filterQuery);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCrawlAttachments() != null)
sb.append("CrawlAttachments: ").append(getCrawlAttachments()).append(",");
if (getIncludeAttachmentFilePatterns() != null)
sb.append("IncludeAttachmentFilePatterns: ").append(getIncludeAttachmentFilePatterns()).append(",");
if (getExcludeAttachmentFilePatterns() != null)
sb.append("ExcludeAttachmentFilePatterns: ").append(getExcludeAttachmentFilePatterns()).append(",");
if (getDocumentDataFieldName() != null)
sb.append("DocumentDataFieldName: ").append(getDocumentDataFieldName()).append(",");
if (getDocumentTitleFieldName() != null)
sb.append("DocumentTitleFieldName: ").append(getDocumentTitleFieldName()).append(",");
if (getFieldMappings() != null)
sb.append("FieldMappings: ").append(getFieldMappings()).append(",");
if (getFilterQuery() != null)
sb.append("FilterQuery: ").append(getFilterQuery());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ServiceNowKnowledgeArticleConfiguration == false)
return false;
ServiceNowKnowledgeArticleConfiguration other = (ServiceNowKnowledgeArticleConfiguration) obj;
if (other.getCrawlAttachments() == null ^ this.getCrawlAttachments() == null)
return false;
if (other.getCrawlAttachments() != null && other.getCrawlAttachments().equals(this.getCrawlAttachments()) == false)
return false;
if (other.getIncludeAttachmentFilePatterns() == null ^ this.getIncludeAttachmentFilePatterns() == null)
return false;
if (other.getIncludeAttachmentFilePatterns() != null
&& other.getIncludeAttachmentFilePatterns().equals(this.getIncludeAttachmentFilePatterns()) == false)
return false;
if (other.getExcludeAttachmentFilePatterns() == null ^ this.getExcludeAttachmentFilePatterns() == null)
return false;
if (other.getExcludeAttachmentFilePatterns() != null
&& other.getExcludeAttachmentFilePatterns().equals(this.getExcludeAttachmentFilePatterns()) == false)
return false;
if (other.getDocumentDataFieldName() == null ^ this.getDocumentDataFieldName() == null)
return false;
if (other.getDocumentDataFieldName() != null && other.getDocumentDataFieldName().equals(this.getDocumentDataFieldName()) == false)
return false;
if (other.getDocumentTitleFieldName() == null ^ this.getDocumentTitleFieldName() == null)
return false;
if (other.getDocumentTitleFieldName() != null && other.getDocumentTitleFieldName().equals(this.getDocumentTitleFieldName()) == false)
return false;
if (other.getFieldMappings() == null ^ this.getFieldMappings() == null)
return false;
if (other.getFieldMappings() != null && other.getFieldMappings().equals(this.getFieldMappings()) == false)
return false;
if (other.getFilterQuery() == null ^ this.getFilterQuery() == null)
return false;
if (other.getFilterQuery() != null && other.getFilterQuery().equals(this.getFilterQuery()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCrawlAttachments() == null) ? 0 : getCrawlAttachments().hashCode());
hashCode = prime * hashCode + ((getIncludeAttachmentFilePatterns() == null) ? 0 : getIncludeAttachmentFilePatterns().hashCode());
hashCode = prime * hashCode + ((getExcludeAttachmentFilePatterns() == null) ? 0 : getExcludeAttachmentFilePatterns().hashCode());
hashCode = prime * hashCode + ((getDocumentDataFieldName() == null) ? 0 : getDocumentDataFieldName().hashCode());
hashCode = prime * hashCode + ((getDocumentTitleFieldName() == null) ? 0 : getDocumentTitleFieldName().hashCode());
hashCode = prime * hashCode + ((getFieldMappings() == null) ? 0 : getFieldMappings().hashCode());
hashCode = prime * hashCode + ((getFilterQuery() == null) ? 0 : getFilterQuery().hashCode());
return hashCode;
}
@Override
public ServiceNowKnowledgeArticleConfiguration clone() {
try {
return (ServiceNowKnowledgeArticleConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.ServiceNowKnowledgeArticleConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}