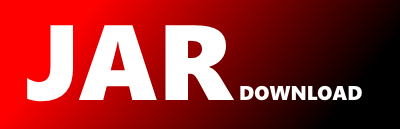
com.amazonaws.services.kendra.model.ServiceNowConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kendra Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kendra.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides the configuration information to connect to ServiceNow as your data source.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ServiceNowConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ServiceNow instance that the data source connects to. The host endpoint should look like the following:
* {instance}.service-now.com.
*
*/
private String hostUrl;
/**
*
* The Amazon Resource Name (ARN) of the Secrets Manager secret that contains the user name and password required to
* connect to the ServiceNow instance. You can also provide OAuth authentication credentials of user name, password,
* client ID, and client secret. For more information, see Using a ServiceNow data
* source.
*
*/
private String secretArn;
/**
*
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
*
*/
private String serviceNowBuildVersion;
/**
*
* Configuration information for crawling knowledge articles in the ServiceNow site.
*
*/
private ServiceNowKnowledgeArticleConfiguration knowledgeArticleConfiguration;
/**
*
* Configuration information for crawling service catalogs in the ServiceNow site.
*
*/
private ServiceNowServiceCatalogConfiguration serviceCatalogConfiguration;
/**
*
* The type of authentication used to connect to the ServiceNow instance. If you choose HTTP_BASIC
,
* Amazon Kendra is authenticated using the user name and password provided in the Secrets Manager secret in the
* SecretArn
field. If you choose OAUTH2
, Amazon Kendra is authenticated using the
* credentials of client ID, client secret, user name and password.
*
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
*
*/
private String authenticationType;
/**
*
* The ServiceNow instance that the data source connects to. The host endpoint should look like the following:
* {instance}.service-now.com.
*
*
* @param hostUrl
* The ServiceNow instance that the data source connects to. The host endpoint should look like the
* following: {instance}.service-now.com.
*/
public void setHostUrl(String hostUrl) {
this.hostUrl = hostUrl;
}
/**
*
* The ServiceNow instance that the data source connects to. The host endpoint should look like the following:
* {instance}.service-now.com.
*
*
* @return The ServiceNow instance that the data source connects to. The host endpoint should look like the
* following: {instance}.service-now.com.
*/
public String getHostUrl() {
return this.hostUrl;
}
/**
*
* The ServiceNow instance that the data source connects to. The host endpoint should look like the following:
* {instance}.service-now.com.
*
*
* @param hostUrl
* The ServiceNow instance that the data source connects to. The host endpoint should look like the
* following: {instance}.service-now.com.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowConfiguration withHostUrl(String hostUrl) {
setHostUrl(hostUrl);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Secrets Manager secret that contains the user name and password required to
* connect to the ServiceNow instance. You can also provide OAuth authentication credentials of user name, password,
* client ID, and client secret. For more information, see Using a ServiceNow data
* source.
*
*
* @param secretArn
* The Amazon Resource Name (ARN) of the Secrets Manager secret that contains the user name and password
* required to connect to the ServiceNow instance. You can also provide OAuth authentication credentials of
* user name, password, client ID, and client secret. For more information, see Using a ServiceNow data
* source.
*/
public void setSecretArn(String secretArn) {
this.secretArn = secretArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Secrets Manager secret that contains the user name and password required to
* connect to the ServiceNow instance. You can also provide OAuth authentication credentials of user name, password,
* client ID, and client secret. For more information, see Using a ServiceNow data
* source.
*
*
* @return The Amazon Resource Name (ARN) of the Secrets Manager secret that contains the user name and password
* required to connect to the ServiceNow instance. You can also provide OAuth authentication credentials of
* user name, password, client ID, and client secret. For more information, see Using a ServiceNow data
* source.
*/
public String getSecretArn() {
return this.secretArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Secrets Manager secret that contains the user name and password required to
* connect to the ServiceNow instance. You can also provide OAuth authentication credentials of user name, password,
* client ID, and client secret. For more information, see Using a ServiceNow data
* source.
*
*
* @param secretArn
* The Amazon Resource Name (ARN) of the Secrets Manager secret that contains the user name and password
* required to connect to the ServiceNow instance. You can also provide OAuth authentication credentials of
* user name, password, client ID, and client secret. For more information, see Using a ServiceNow data
* source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowConfiguration withSecretArn(String secretArn) {
setSecretArn(secretArn);
return this;
}
/**
*
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
*
*
* @param serviceNowBuildVersion
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
* @see ServiceNowBuildVersionType
*/
public void setServiceNowBuildVersion(String serviceNowBuildVersion) {
this.serviceNowBuildVersion = serviceNowBuildVersion;
}
/**
*
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
*
*
* @return The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
* @see ServiceNowBuildVersionType
*/
public String getServiceNowBuildVersion() {
return this.serviceNowBuildVersion;
}
/**
*
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
*
*
* @param serviceNowBuildVersion
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNowBuildVersionType
*/
public ServiceNowConfiguration withServiceNowBuildVersion(String serviceNowBuildVersion) {
setServiceNowBuildVersion(serviceNowBuildVersion);
return this;
}
/**
*
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
*
*
* @param serviceNowBuildVersion
* The identifier of the release that the ServiceNow host is running. If the host is not running the
* LONDON
release, use OTHERS
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNowBuildVersionType
*/
public ServiceNowConfiguration withServiceNowBuildVersion(ServiceNowBuildVersionType serviceNowBuildVersion) {
this.serviceNowBuildVersion = serviceNowBuildVersion.toString();
return this;
}
/**
*
* Configuration information for crawling knowledge articles in the ServiceNow site.
*
*
* @param knowledgeArticleConfiguration
* Configuration information for crawling knowledge articles in the ServiceNow site.
*/
public void setKnowledgeArticleConfiguration(ServiceNowKnowledgeArticleConfiguration knowledgeArticleConfiguration) {
this.knowledgeArticleConfiguration = knowledgeArticleConfiguration;
}
/**
*
* Configuration information for crawling knowledge articles in the ServiceNow site.
*
*
* @return Configuration information for crawling knowledge articles in the ServiceNow site.
*/
public ServiceNowKnowledgeArticleConfiguration getKnowledgeArticleConfiguration() {
return this.knowledgeArticleConfiguration;
}
/**
*
* Configuration information for crawling knowledge articles in the ServiceNow site.
*
*
* @param knowledgeArticleConfiguration
* Configuration information for crawling knowledge articles in the ServiceNow site.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowConfiguration withKnowledgeArticleConfiguration(ServiceNowKnowledgeArticleConfiguration knowledgeArticleConfiguration) {
setKnowledgeArticleConfiguration(knowledgeArticleConfiguration);
return this;
}
/**
*
* Configuration information for crawling service catalogs in the ServiceNow site.
*
*
* @param serviceCatalogConfiguration
* Configuration information for crawling service catalogs in the ServiceNow site.
*/
public void setServiceCatalogConfiguration(ServiceNowServiceCatalogConfiguration serviceCatalogConfiguration) {
this.serviceCatalogConfiguration = serviceCatalogConfiguration;
}
/**
*
* Configuration information for crawling service catalogs in the ServiceNow site.
*
*
* @return Configuration information for crawling service catalogs in the ServiceNow site.
*/
public ServiceNowServiceCatalogConfiguration getServiceCatalogConfiguration() {
return this.serviceCatalogConfiguration;
}
/**
*
* Configuration information for crawling service catalogs in the ServiceNow site.
*
*
* @param serviceCatalogConfiguration
* Configuration information for crawling service catalogs in the ServiceNow site.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceNowConfiguration withServiceCatalogConfiguration(ServiceNowServiceCatalogConfiguration serviceCatalogConfiguration) {
setServiceCatalogConfiguration(serviceCatalogConfiguration);
return this;
}
/**
*
* The type of authentication used to connect to the ServiceNow instance. If you choose HTTP_BASIC
,
* Amazon Kendra is authenticated using the user name and password provided in the Secrets Manager secret in the
* SecretArn
field. If you choose OAUTH2
, Amazon Kendra is authenticated using the
* credentials of client ID, client secret, user name and password.
*
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
*
*
* @param authenticationType
* The type of authentication used to connect to the ServiceNow instance. If you choose
* HTTP_BASIC
, Amazon Kendra is authenticated using the user name and password provided in the
* Secrets Manager secret in the SecretArn
field. If you choose OAUTH2
, Amazon
* Kendra is authenticated using the credentials of client ID, client secret, user name and password.
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
* @see ServiceNowAuthenticationType
*/
public void setAuthenticationType(String authenticationType) {
this.authenticationType = authenticationType;
}
/**
*
* The type of authentication used to connect to the ServiceNow instance. If you choose HTTP_BASIC
,
* Amazon Kendra is authenticated using the user name and password provided in the Secrets Manager secret in the
* SecretArn
field. If you choose OAUTH2
, Amazon Kendra is authenticated using the
* credentials of client ID, client secret, user name and password.
*
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
*
*
* @return The type of authentication used to connect to the ServiceNow instance. If you choose
* HTTP_BASIC
, Amazon Kendra is authenticated using the user name and password provided in the
* Secrets Manager secret in the SecretArn
field. If you choose OAUTH2
, Amazon
* Kendra is authenticated using the credentials of client ID, client secret, user name and password.
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
* @see ServiceNowAuthenticationType
*/
public String getAuthenticationType() {
return this.authenticationType;
}
/**
*
* The type of authentication used to connect to the ServiceNow instance. If you choose HTTP_BASIC
,
* Amazon Kendra is authenticated using the user name and password provided in the Secrets Manager secret in the
* SecretArn
field. If you choose OAUTH2
, Amazon Kendra is authenticated using the
* credentials of client ID, client secret, user name and password.
*
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
*
*
* @param authenticationType
* The type of authentication used to connect to the ServiceNow instance. If you choose
* HTTP_BASIC
, Amazon Kendra is authenticated using the user name and password provided in the
* Secrets Manager secret in the SecretArn
field. If you choose OAUTH2
, Amazon
* Kendra is authenticated using the credentials of client ID, client secret, user name and password.
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNowAuthenticationType
*/
public ServiceNowConfiguration withAuthenticationType(String authenticationType) {
setAuthenticationType(authenticationType);
return this;
}
/**
*
* The type of authentication used to connect to the ServiceNow instance. If you choose HTTP_BASIC
,
* Amazon Kendra is authenticated using the user name and password provided in the Secrets Manager secret in the
* SecretArn
field. If you choose OAUTH2
, Amazon Kendra is authenticated using the
* credentials of client ID, client secret, user name and password.
*
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
*
*
* @param authenticationType
* The type of authentication used to connect to the ServiceNow instance. If you choose
* HTTP_BASIC
, Amazon Kendra is authenticated using the user name and password provided in the
* Secrets Manager secret in the SecretArn
field. If you choose OAUTH2
, Amazon
* Kendra is authenticated using the credentials of client ID, client secret, user name and password.
*
* When you use OAUTH2
authentication, you must generate a token and a client secret using the
* ServiceNow console. For more information, see Using a ServiceNow data
* source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNowAuthenticationType
*/
public ServiceNowConfiguration withAuthenticationType(ServiceNowAuthenticationType authenticationType) {
this.authenticationType = authenticationType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getHostUrl() != null)
sb.append("HostUrl: ").append(getHostUrl()).append(",");
if (getSecretArn() != null)
sb.append("SecretArn: ").append(getSecretArn()).append(",");
if (getServiceNowBuildVersion() != null)
sb.append("ServiceNowBuildVersion: ").append(getServiceNowBuildVersion()).append(",");
if (getKnowledgeArticleConfiguration() != null)
sb.append("KnowledgeArticleConfiguration: ").append(getKnowledgeArticleConfiguration()).append(",");
if (getServiceCatalogConfiguration() != null)
sb.append("ServiceCatalogConfiguration: ").append(getServiceCatalogConfiguration()).append(",");
if (getAuthenticationType() != null)
sb.append("AuthenticationType: ").append(getAuthenticationType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ServiceNowConfiguration == false)
return false;
ServiceNowConfiguration other = (ServiceNowConfiguration) obj;
if (other.getHostUrl() == null ^ this.getHostUrl() == null)
return false;
if (other.getHostUrl() != null && other.getHostUrl().equals(this.getHostUrl()) == false)
return false;
if (other.getSecretArn() == null ^ this.getSecretArn() == null)
return false;
if (other.getSecretArn() != null && other.getSecretArn().equals(this.getSecretArn()) == false)
return false;
if (other.getServiceNowBuildVersion() == null ^ this.getServiceNowBuildVersion() == null)
return false;
if (other.getServiceNowBuildVersion() != null && other.getServiceNowBuildVersion().equals(this.getServiceNowBuildVersion()) == false)
return false;
if (other.getKnowledgeArticleConfiguration() == null ^ this.getKnowledgeArticleConfiguration() == null)
return false;
if (other.getKnowledgeArticleConfiguration() != null
&& other.getKnowledgeArticleConfiguration().equals(this.getKnowledgeArticleConfiguration()) == false)
return false;
if (other.getServiceCatalogConfiguration() == null ^ this.getServiceCatalogConfiguration() == null)
return false;
if (other.getServiceCatalogConfiguration() != null && other.getServiceCatalogConfiguration().equals(this.getServiceCatalogConfiguration()) == false)
return false;
if (other.getAuthenticationType() == null ^ this.getAuthenticationType() == null)
return false;
if (other.getAuthenticationType() != null && other.getAuthenticationType().equals(this.getAuthenticationType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getHostUrl() == null) ? 0 : getHostUrl().hashCode());
hashCode = prime * hashCode + ((getSecretArn() == null) ? 0 : getSecretArn().hashCode());
hashCode = prime * hashCode + ((getServiceNowBuildVersion() == null) ? 0 : getServiceNowBuildVersion().hashCode());
hashCode = prime * hashCode + ((getKnowledgeArticleConfiguration() == null) ? 0 : getKnowledgeArticleConfiguration().hashCode());
hashCode = prime * hashCode + ((getServiceCatalogConfiguration() == null) ? 0 : getServiceCatalogConfiguration().hashCode());
hashCode = prime * hashCode + ((getAuthenticationType() == null) ? 0 : getAuthenticationType().hashCode());
return hashCode;
}
@Override
public ServiceNowConfiguration clone() {
try {
return (ServiceNowConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.kendra.model.transform.ServiceNowConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}