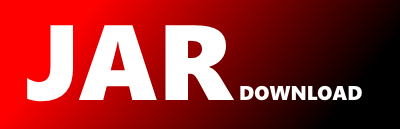
com.amazonaws.services.keyspaces.model.RestoreTableRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-keyspaces Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.keyspaces.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RestoreTableRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The keyspace name of the source table.
*
*/
private String sourceKeyspaceName;
/**
*
* The name of the source table.
*
*/
private String sourceTableName;
/**
*
* The name of the target keyspace.
*
*/
private String targetKeyspaceName;
/**
*
* The name of the target table.
*
*/
private String targetTableName;
/**
*
* The restore timestamp in ISO 8601 format.
*
*/
private java.util.Date restoreTimestamp;
/**
*
* Specifies the read/write throughput capacity mode for the target table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*/
private CapacitySpecification capacitySpecificationOverride;
/**
*
* Specifies the encryption settings for the target table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*/
private EncryptionSpecification encryptionSpecificationOverride;
/**
*
* Specifies the pointInTimeRecovery
settings for the target table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*/
private PointInTimeRecovery pointInTimeRecoveryOverride;
/**
*
* A list of key-value pair tags to be attached to the restored table.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*/
private java.util.List tagsOverride;
/**
*
* The keyspace name of the source table.
*
*
* @param sourceKeyspaceName
* The keyspace name of the source table.
*/
public void setSourceKeyspaceName(String sourceKeyspaceName) {
this.sourceKeyspaceName = sourceKeyspaceName;
}
/**
*
* The keyspace name of the source table.
*
*
* @return The keyspace name of the source table.
*/
public String getSourceKeyspaceName() {
return this.sourceKeyspaceName;
}
/**
*
* The keyspace name of the source table.
*
*
* @param sourceKeyspaceName
* The keyspace name of the source table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withSourceKeyspaceName(String sourceKeyspaceName) {
setSourceKeyspaceName(sourceKeyspaceName);
return this;
}
/**
*
* The name of the source table.
*
*
* @param sourceTableName
* The name of the source table.
*/
public void setSourceTableName(String sourceTableName) {
this.sourceTableName = sourceTableName;
}
/**
*
* The name of the source table.
*
*
* @return The name of the source table.
*/
public String getSourceTableName() {
return this.sourceTableName;
}
/**
*
* The name of the source table.
*
*
* @param sourceTableName
* The name of the source table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withSourceTableName(String sourceTableName) {
setSourceTableName(sourceTableName);
return this;
}
/**
*
* The name of the target keyspace.
*
*
* @param targetKeyspaceName
* The name of the target keyspace.
*/
public void setTargetKeyspaceName(String targetKeyspaceName) {
this.targetKeyspaceName = targetKeyspaceName;
}
/**
*
* The name of the target keyspace.
*
*
* @return The name of the target keyspace.
*/
public String getTargetKeyspaceName() {
return this.targetKeyspaceName;
}
/**
*
* The name of the target keyspace.
*
*
* @param targetKeyspaceName
* The name of the target keyspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withTargetKeyspaceName(String targetKeyspaceName) {
setTargetKeyspaceName(targetKeyspaceName);
return this;
}
/**
*
* The name of the target table.
*
*
* @param targetTableName
* The name of the target table.
*/
public void setTargetTableName(String targetTableName) {
this.targetTableName = targetTableName;
}
/**
*
* The name of the target table.
*
*
* @return The name of the target table.
*/
public String getTargetTableName() {
return this.targetTableName;
}
/**
*
* The name of the target table.
*
*
* @param targetTableName
* The name of the target table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withTargetTableName(String targetTableName) {
setTargetTableName(targetTableName);
return this;
}
/**
*
* The restore timestamp in ISO 8601 format.
*
*
* @param restoreTimestamp
* The restore timestamp in ISO 8601 format.
*/
public void setRestoreTimestamp(java.util.Date restoreTimestamp) {
this.restoreTimestamp = restoreTimestamp;
}
/**
*
* The restore timestamp in ISO 8601 format.
*
*
* @return The restore timestamp in ISO 8601 format.
*/
public java.util.Date getRestoreTimestamp() {
return this.restoreTimestamp;
}
/**
*
* The restore timestamp in ISO 8601 format.
*
*
* @param restoreTimestamp
* The restore timestamp in ISO 8601 format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withRestoreTimestamp(java.util.Date restoreTimestamp) {
setRestoreTimestamp(restoreTimestamp);
return this;
}
/**
*
* Specifies the read/write throughput capacity mode for the target table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @param capacitySpecificationOverride
* Specifies the read/write throughput capacity mode for the target table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
*/
public void setCapacitySpecificationOverride(CapacitySpecification capacitySpecificationOverride) {
this.capacitySpecificationOverride = capacitySpecificationOverride;
}
/**
*
* Specifies the read/write throughput capacity mode for the target table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @return Specifies the read/write throughput capacity mode for the target table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
*/
public CapacitySpecification getCapacitySpecificationOverride() {
return this.capacitySpecificationOverride;
}
/**
*
* Specifies the read/write throughput capacity mode for the target table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @param capacitySpecificationOverride
* Specifies the read/write throughput capacity mode for the target table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withCapacitySpecificationOverride(CapacitySpecification capacitySpecificationOverride) {
setCapacitySpecificationOverride(capacitySpecificationOverride);
return this;
}
/**
*
* Specifies the encryption settings for the target table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @param encryptionSpecificationOverride
* Specifies the encryption settings for the target table. You can choose one of the following KMS key (KMS
* key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource
* Name (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
*/
public void setEncryptionSpecificationOverride(EncryptionSpecification encryptionSpecificationOverride) {
this.encryptionSpecificationOverride = encryptionSpecificationOverride;
}
/**
*
* Specifies the encryption settings for the target table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @return Specifies the encryption settings for the target table. You can choose one of the following KMS key (KMS
* key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned,
* and managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon
* Resource Name (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
*/
public EncryptionSpecification getEncryptionSpecificationOverride() {
return this.encryptionSpecificationOverride;
}
/**
*
* Specifies the encryption settings for the target table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @param encryptionSpecificationOverride
* Specifies the encryption settings for the target table. You can choose one of the following KMS key (KMS
* key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource
* Name (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withEncryptionSpecificationOverride(EncryptionSpecification encryptionSpecificationOverride) {
setEncryptionSpecificationOverride(encryptionSpecificationOverride);
return this;
}
/**
*
* Specifies the pointInTimeRecovery
settings for the target table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @param pointInTimeRecoveryOverride
* Specifies the pointInTimeRecovery
settings for the target table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
*/
public void setPointInTimeRecoveryOverride(PointInTimeRecovery pointInTimeRecoveryOverride) {
this.pointInTimeRecoveryOverride = pointInTimeRecoveryOverride;
}
/**
*
* Specifies the pointInTimeRecovery
settings for the target table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @return Specifies the pointInTimeRecovery
settings for the target table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
*/
public PointInTimeRecovery getPointInTimeRecoveryOverride() {
return this.pointInTimeRecoveryOverride;
}
/**
*
* Specifies the pointInTimeRecovery
settings for the target table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @param pointInTimeRecoveryOverride
* Specifies the pointInTimeRecovery
settings for the target table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withPointInTimeRecoveryOverride(PointInTimeRecovery pointInTimeRecoveryOverride) {
setPointInTimeRecoveryOverride(pointInTimeRecoveryOverride);
return this;
}
/**
*
* A list of key-value pair tags to be attached to the restored table.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* @return A list of key-value pair tags to be attached to the restored table.
*
* For more information, see Adding tags and
* labels to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*/
public java.util.List getTagsOverride() {
return tagsOverride;
}
/**
*
* A list of key-value pair tags to be attached to the restored table.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* @param tagsOverride
* A list of key-value pair tags to be attached to the restored table.
*
* For more information, see Adding tags and labels
* to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*/
public void setTagsOverride(java.util.Collection tagsOverride) {
if (tagsOverride == null) {
this.tagsOverride = null;
return;
}
this.tagsOverride = new java.util.ArrayList(tagsOverride);
}
/**
*
* A list of key-value pair tags to be attached to the restored table.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagsOverride(java.util.Collection)} or {@link #withTagsOverride(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param tagsOverride
* A list of key-value pair tags to be attached to the restored table.
*
* For more information, see Adding tags and labels
* to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withTagsOverride(Tag... tagsOverride) {
if (this.tagsOverride == null) {
setTagsOverride(new java.util.ArrayList(tagsOverride.length));
}
for (Tag ele : tagsOverride) {
this.tagsOverride.add(ele);
}
return this;
}
/**
*
* A list of key-value pair tags to be attached to the restored table.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* @param tagsOverride
* A list of key-value pair tags to be attached to the restored table.
*
* For more information, see Adding tags and labels
* to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RestoreTableRequest withTagsOverride(java.util.Collection tagsOverride) {
setTagsOverride(tagsOverride);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSourceKeyspaceName() != null)
sb.append("SourceKeyspaceName: ").append(getSourceKeyspaceName()).append(",");
if (getSourceTableName() != null)
sb.append("SourceTableName: ").append(getSourceTableName()).append(",");
if (getTargetKeyspaceName() != null)
sb.append("TargetKeyspaceName: ").append(getTargetKeyspaceName()).append(",");
if (getTargetTableName() != null)
sb.append("TargetTableName: ").append(getTargetTableName()).append(",");
if (getRestoreTimestamp() != null)
sb.append("RestoreTimestamp: ").append(getRestoreTimestamp()).append(",");
if (getCapacitySpecificationOverride() != null)
sb.append("CapacitySpecificationOverride: ").append(getCapacitySpecificationOverride()).append(",");
if (getEncryptionSpecificationOverride() != null)
sb.append("EncryptionSpecificationOverride: ").append(getEncryptionSpecificationOverride()).append(",");
if (getPointInTimeRecoveryOverride() != null)
sb.append("PointInTimeRecoveryOverride: ").append(getPointInTimeRecoveryOverride()).append(",");
if (getTagsOverride() != null)
sb.append("TagsOverride: ").append(getTagsOverride());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RestoreTableRequest == false)
return false;
RestoreTableRequest other = (RestoreTableRequest) obj;
if (other.getSourceKeyspaceName() == null ^ this.getSourceKeyspaceName() == null)
return false;
if (other.getSourceKeyspaceName() != null && other.getSourceKeyspaceName().equals(this.getSourceKeyspaceName()) == false)
return false;
if (other.getSourceTableName() == null ^ this.getSourceTableName() == null)
return false;
if (other.getSourceTableName() != null && other.getSourceTableName().equals(this.getSourceTableName()) == false)
return false;
if (other.getTargetKeyspaceName() == null ^ this.getTargetKeyspaceName() == null)
return false;
if (other.getTargetKeyspaceName() != null && other.getTargetKeyspaceName().equals(this.getTargetKeyspaceName()) == false)
return false;
if (other.getTargetTableName() == null ^ this.getTargetTableName() == null)
return false;
if (other.getTargetTableName() != null && other.getTargetTableName().equals(this.getTargetTableName()) == false)
return false;
if (other.getRestoreTimestamp() == null ^ this.getRestoreTimestamp() == null)
return false;
if (other.getRestoreTimestamp() != null && other.getRestoreTimestamp().equals(this.getRestoreTimestamp()) == false)
return false;
if (other.getCapacitySpecificationOverride() == null ^ this.getCapacitySpecificationOverride() == null)
return false;
if (other.getCapacitySpecificationOverride() != null
&& other.getCapacitySpecificationOverride().equals(this.getCapacitySpecificationOverride()) == false)
return false;
if (other.getEncryptionSpecificationOverride() == null ^ this.getEncryptionSpecificationOverride() == null)
return false;
if (other.getEncryptionSpecificationOverride() != null
&& other.getEncryptionSpecificationOverride().equals(this.getEncryptionSpecificationOverride()) == false)
return false;
if (other.getPointInTimeRecoveryOverride() == null ^ this.getPointInTimeRecoveryOverride() == null)
return false;
if (other.getPointInTimeRecoveryOverride() != null && other.getPointInTimeRecoveryOverride().equals(this.getPointInTimeRecoveryOverride()) == false)
return false;
if (other.getTagsOverride() == null ^ this.getTagsOverride() == null)
return false;
if (other.getTagsOverride() != null && other.getTagsOverride().equals(this.getTagsOverride()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSourceKeyspaceName() == null) ? 0 : getSourceKeyspaceName().hashCode());
hashCode = prime * hashCode + ((getSourceTableName() == null) ? 0 : getSourceTableName().hashCode());
hashCode = prime * hashCode + ((getTargetKeyspaceName() == null) ? 0 : getTargetKeyspaceName().hashCode());
hashCode = prime * hashCode + ((getTargetTableName() == null) ? 0 : getTargetTableName().hashCode());
hashCode = prime * hashCode + ((getRestoreTimestamp() == null) ? 0 : getRestoreTimestamp().hashCode());
hashCode = prime * hashCode + ((getCapacitySpecificationOverride() == null) ? 0 : getCapacitySpecificationOverride().hashCode());
hashCode = prime * hashCode + ((getEncryptionSpecificationOverride() == null) ? 0 : getEncryptionSpecificationOverride().hashCode());
hashCode = prime * hashCode + ((getPointInTimeRecoveryOverride() == null) ? 0 : getPointInTimeRecoveryOverride().hashCode());
hashCode = prime * hashCode + ((getTagsOverride() == null) ? 0 : getTagsOverride().hashCode());
return hashCode;
}
@Override
public RestoreTableRequest clone() {
return (RestoreTableRequest) super.clone();
}
}