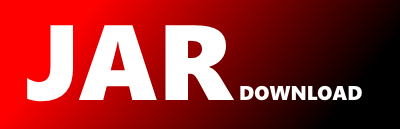
com.amazonaws.services.keyspaces.model.CreateTableRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-keyspaces Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.keyspaces.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateTableRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the keyspace that the table is going to be created in.
*
*/
private String keyspaceName;
/**
*
* The name of the table.
*
*/
private String tableName;
/**
*
* The schemaDefinition
consists of the following parameters.
*
*
* For each column to be created:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* The primary key of the table consists of the following columns:
*
*
* -
*
* partitionKeys
- The partition key can be a single column, or it can be a compound value composed of
* two or more columns. The partition key portion of the primary key is required and determines how Amazon Keyspaces
* stores your data.
*
*
* -
*
* name
- The name of each partition key column.
*
*
* -
*
* clusteringKeys
- The optional clustering column portion of your primary key determines how the data
* is clustered and sorted within each partition.
*
*
* -
*
* name
- The name of the clustering column.
*
*
* -
*
* orderBy
- Sets the ascendant (ASC
) or descendant (DESC
) order modifier.
*
*
* To define a column as static use staticColumns
- Static columns store values that are shared by all
* rows in the same partition:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type.
*
*
*
*/
private SchemaDefinition schemaDefinition;
/**
*
* This parameter allows to enter a description of the table.
*
*/
private Comment comment;
/**
*
* Specifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*/
private CapacitySpecification capacitySpecification;
/**
*
* Specifies how the encryption key for encryption at rest is managed for the table. You can choose one of the
* following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*/
private EncryptionSpecification encryptionSpecification;
/**
*
* Specifies if pointInTimeRecovery
is enabled or disabled for the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*/
private PointInTimeRecovery pointInTimeRecovery;
/**
*
* Enables Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*/
private TimeToLive ttl;
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*/
private Integer defaultTimeToLive;
/**
*
* A list of key-value pair tags to be attached to the resource.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*/
private java.util.List tags;
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*/
private ClientSideTimestamps clientSideTimestamps;
/**
*
* The optional auto scaling settings for a table in provisioned capacity mode. Specifies if the service can manage
* throughput capacity automatically on your behalf.
*
*
* Auto scaling helps you provision throughput capacity for variable workloads efficiently by increasing and
* decreasing your table's read and write capacity automatically in response to application traffic. For more
* information, see Managing
* throughput capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer
* Guide.
*
*
* By default, auto scaling is disabled for a table.
*
*/
private AutoScalingSpecification autoScalingSpecification;
/**
*
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings overwrite the
* general settings of the table for the specified Region.
*
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity differently
* for each Region's replica. The write capacity, however, remains synchronized between all replicas to ensure that
* there's enough capacity to replicate writes across all Regions. To define the read capacity for a table replica
* in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
*
*/
private java.util.List replicaSpecifications;
/**
*
* The name of the keyspace that the table is going to be created in.
*
*
* @param keyspaceName
* The name of the keyspace that the table is going to be created in.
*/
public void setKeyspaceName(String keyspaceName) {
this.keyspaceName = keyspaceName;
}
/**
*
* The name of the keyspace that the table is going to be created in.
*
*
* @return The name of the keyspace that the table is going to be created in.
*/
public String getKeyspaceName() {
return this.keyspaceName;
}
/**
*
* The name of the keyspace that the table is going to be created in.
*
*
* @param keyspaceName
* The name of the keyspace that the table is going to be created in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withKeyspaceName(String keyspaceName) {
setKeyspaceName(keyspaceName);
return this;
}
/**
*
* The name of the table.
*
*
* @param tableName
* The name of the table.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
*
* The name of the table.
*
*
* @return The name of the table.
*/
public String getTableName() {
return this.tableName;
}
/**
*
* The name of the table.
*
*
* @param tableName
* The name of the table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withTableName(String tableName) {
setTableName(tableName);
return this;
}
/**
*
* The schemaDefinition
consists of the following parameters.
*
*
* For each column to be created:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* The primary key of the table consists of the following columns:
*
*
* -
*
* partitionKeys
- The partition key can be a single column, or it can be a compound value composed of
* two or more columns. The partition key portion of the primary key is required and determines how Amazon Keyspaces
* stores your data.
*
*
* -
*
* name
- The name of each partition key column.
*
*
* -
*
* clusteringKeys
- The optional clustering column portion of your primary key determines how the data
* is clustered and sorted within each partition.
*
*
* -
*
* name
- The name of the clustering column.
*
*
* -
*
* orderBy
- Sets the ascendant (ASC
) or descendant (DESC
) order modifier.
*
*
* To define a column as static use staticColumns
- Static columns store values that are shared by all
* rows in the same partition:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type.
*
*
*
*
* @param schemaDefinition
* The schemaDefinition
consists of the following parameters.
*
* For each column to be created:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data
* types in the Amazon Keyspaces Developer Guide.
*
*
*
*
* The primary key of the table consists of the following columns:
*
*
* -
*
* partitionKeys
- The partition key can be a single column, or it can be a compound value
* composed of two or more columns. The partition key portion of the primary key is required and determines
* how Amazon Keyspaces stores your data.
*
*
* -
*
* name
- The name of each partition key column.
*
*
* -
*
* clusteringKeys
- The optional clustering column portion of your primary key determines how
* the data is clustered and sorted within each partition.
*
*
* -
*
* name
- The name of the clustering column.
*
*
* -
*
* orderBy
- Sets the ascendant (ASC
) or descendant (DESC
) order
* modifier.
*
*
* To define a column as static use staticColumns
- Static columns store values that are shared
* by all rows in the same partition:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type.
*
*
*/
public void setSchemaDefinition(SchemaDefinition schemaDefinition) {
this.schemaDefinition = schemaDefinition;
}
/**
*
* The schemaDefinition
consists of the following parameters.
*
*
* For each column to be created:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* The primary key of the table consists of the following columns:
*
*
* -
*
* partitionKeys
- The partition key can be a single column, or it can be a compound value composed of
* two or more columns. The partition key portion of the primary key is required and determines how Amazon Keyspaces
* stores your data.
*
*
* -
*
* name
- The name of each partition key column.
*
*
* -
*
* clusteringKeys
- The optional clustering column portion of your primary key determines how the data
* is clustered and sorted within each partition.
*
*
* -
*
* name
- The name of the clustering column.
*
*
* -
*
* orderBy
- Sets the ascendant (ASC
) or descendant (DESC
) order modifier.
*
*
* To define a column as static use staticColumns
- Static columns store values that are shared by all
* rows in the same partition:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type.
*
*
*
*
* @return The schemaDefinition
consists of the following parameters.
*
* For each column to be created:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data
* types in the Amazon Keyspaces Developer Guide.
*
*
*
*
* The primary key of the table consists of the following columns:
*
*
* -
*
* partitionKeys
- The partition key can be a single column, or it can be a compound value
* composed of two or more columns. The partition key portion of the primary key is required and determines
* how Amazon Keyspaces stores your data.
*
*
* -
*
* name
- The name of each partition key column.
*
*
* -
*
* clusteringKeys
- The optional clustering column portion of your primary key determines how
* the data is clustered and sorted within each partition.
*
*
* -
*
* name
- The name of the clustering column.
*
*
* -
*
* orderBy
- Sets the ascendant (ASC
) or descendant (DESC
) order
* modifier.
*
*
* To define a column as static use staticColumns
- Static columns store values that are shared
* by all rows in the same partition:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type.
*
*
*/
public SchemaDefinition getSchemaDefinition() {
return this.schemaDefinition;
}
/**
*
* The schemaDefinition
consists of the following parameters.
*
*
* For each column to be created:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* The primary key of the table consists of the following columns:
*
*
* -
*
* partitionKeys
- The partition key can be a single column, or it can be a compound value composed of
* two or more columns. The partition key portion of the primary key is required and determines how Amazon Keyspaces
* stores your data.
*
*
* -
*
* name
- The name of each partition key column.
*
*
* -
*
* clusteringKeys
- The optional clustering column portion of your primary key determines how the data
* is clustered and sorted within each partition.
*
*
* -
*
* name
- The name of the clustering column.
*
*
* -
*
* orderBy
- Sets the ascendant (ASC
) or descendant (DESC
) order modifier.
*
*
* To define a column as static use staticColumns
- Static columns store values that are shared by all
* rows in the same partition:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type.
*
*
*
*
* @param schemaDefinition
* The schemaDefinition
consists of the following parameters.
*
* For each column to be created:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data
* types in the Amazon Keyspaces Developer Guide.
*
*
*
*
* The primary key of the table consists of the following columns:
*
*
* -
*
* partitionKeys
- The partition key can be a single column, or it can be a compound value
* composed of two or more columns. The partition key portion of the primary key is required and determines
* how Amazon Keyspaces stores your data.
*
*
* -
*
* name
- The name of each partition key column.
*
*
* -
*
* clusteringKeys
- The optional clustering column portion of your primary key determines how
* the data is clustered and sorted within each partition.
*
*
* -
*
* name
- The name of the clustering column.
*
*
* -
*
* orderBy
- Sets the ascendant (ASC
) or descendant (DESC
) order
* modifier.
*
*
* To define a column as static use staticColumns
- Static columns store values that are shared
* by all rows in the same partition:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withSchemaDefinition(SchemaDefinition schemaDefinition) {
setSchemaDefinition(schemaDefinition);
return this;
}
/**
*
* This parameter allows to enter a description of the table.
*
*
* @param comment
* This parameter allows to enter a description of the table.
*/
public void setComment(Comment comment) {
this.comment = comment;
}
/**
*
* This parameter allows to enter a description of the table.
*
*
* @return This parameter allows to enter a description of the table.
*/
public Comment getComment() {
return this.comment;
}
/**
*
* This parameter allows to enter a description of the table.
*
*
* @param comment
* This parameter allows to enter a description of the table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withComment(Comment comment) {
setComment(comment);
return this;
}
/**
*
* Specifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @param capacitySpecification
* Specifies the read/write throughput capacity mode for the table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
*/
public void setCapacitySpecification(CapacitySpecification capacitySpecification) {
this.capacitySpecification = capacitySpecification;
}
/**
*
* Specifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @return Specifies the read/write throughput capacity mode for the table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
*/
public CapacitySpecification getCapacitySpecification() {
return this.capacitySpecification;
}
/**
*
* Specifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @param capacitySpecification
* Specifies the read/write throughput capacity mode for the table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withCapacitySpecification(CapacitySpecification capacitySpecification) {
setCapacitySpecification(capacitySpecification);
return this;
}
/**
*
* Specifies how the encryption key for encryption at rest is managed for the table. You can choose one of the
* following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @param encryptionSpecification
* Specifies how the encryption key for encryption at rest is managed for the table. You can choose one of
* the following KMS key (KMS key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource
* Name (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
*/
public void setEncryptionSpecification(EncryptionSpecification encryptionSpecification) {
this.encryptionSpecification = encryptionSpecification;
}
/**
*
* Specifies how the encryption key for encryption at rest is managed for the table. You can choose one of the
* following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @return Specifies how the encryption key for encryption at rest is managed for the table. You can choose one of
* the following KMS key (KMS key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned,
* and managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon
* Resource Name (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
*/
public EncryptionSpecification getEncryptionSpecification() {
return this.encryptionSpecification;
}
/**
*
* Specifies how the encryption key for encryption at rest is managed for the table. You can choose one of the
* following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @param encryptionSpecification
* Specifies how the encryption key for encryption at rest is managed for the table. You can choose one of
* the following KMS key (KMS key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource
* Name (ARN) format as input.
*
*
*
*
* The default is type:AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withEncryptionSpecification(EncryptionSpecification encryptionSpecification) {
setEncryptionSpecification(encryptionSpecification);
return this;
}
/**
*
* Specifies if pointInTimeRecovery
is enabled or disabled for the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @param pointInTimeRecovery
* Specifies if pointInTimeRecovery
is enabled or disabled for the table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
*/
public void setPointInTimeRecovery(PointInTimeRecovery pointInTimeRecovery) {
this.pointInTimeRecovery = pointInTimeRecovery;
}
/**
*
* Specifies if pointInTimeRecovery
is enabled or disabled for the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @return Specifies if pointInTimeRecovery
is enabled or disabled for the table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
*/
public PointInTimeRecovery getPointInTimeRecovery() {
return this.pointInTimeRecovery;
}
/**
*
* Specifies if pointInTimeRecovery
is enabled or disabled for the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @param pointInTimeRecovery
* Specifies if pointInTimeRecovery
is enabled or disabled for the table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withPointInTimeRecovery(PointInTimeRecovery pointInTimeRecovery) {
setPointInTimeRecovery(pointInTimeRecovery);
return this;
}
/**
*
* Enables Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*
* @param ttl
* Enables Time to Live custom settings for the table. The options are:
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for
* the table.
*
*
* For more information, see Expiring data by using Amazon
* Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*/
public void setTtl(TimeToLive ttl) {
this.ttl = ttl;
}
/**
*
* Enables Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*
* @return Enables Time to Live custom settings for the table. The options are:
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for
* the table.
*
*
* For more information, see Expiring data by using Amazon
* Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*/
public TimeToLive getTtl() {
return this.ttl;
}
/**
*
* Enables Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*
* @param ttl
* Enables Time to Live custom settings for the table. The options are:
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for
* the table.
*
*
* For more information, see Expiring data by using Amazon
* Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withTtl(TimeToLive ttl) {
setTtl(ttl);
return this;
}
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*
* @param defaultTimeToLive
* The default Time to Live setting in seconds for the table.
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*/
public void setDefaultTimeToLive(Integer defaultTimeToLive) {
this.defaultTimeToLive = defaultTimeToLive;
}
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*
* @return The default Time to Live setting in seconds for the table.
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*/
public Integer getDefaultTimeToLive() {
return this.defaultTimeToLive;
}
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*
* @param defaultTimeToLive
* The default Time to Live setting in seconds for the table.
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withDefaultTimeToLive(Integer defaultTimeToLive) {
setDefaultTimeToLive(defaultTimeToLive);
return this;
}
/**
*
* A list of key-value pair tags to be attached to the resource.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* @return A list of key-value pair tags to be attached to the resource.
*
* For more information, see Adding tags and
* labels to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of key-value pair tags to be attached to the resource.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* @param tags
* A list of key-value pair tags to be attached to the resource.
*
* For more information, see Adding tags and labels
* to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of key-value pair tags to be attached to the resource.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of key-value pair tags to be attached to the resource.
*
* For more information, see Adding tags and labels
* to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of key-value pair tags to be attached to the resource.
*
*
* For more information, see Adding tags and labels to
* Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
*
*
* @param tags
* A list of key-value pair tags to be attached to the resource.
*
* For more information, see Adding tags and labels
* to Amazon Keyspaces resources in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*
* @param clientSideTimestamps
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable
* client-side timestamps with the following option:
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*/
public void setClientSideTimestamps(ClientSideTimestamps clientSideTimestamps) {
this.clientSideTimestamps = clientSideTimestamps;
}
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*
* @return Enables client-side timestamps for the table. By default, the setting is disabled. You can enable
* client-side timestamps with the following option:
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*/
public ClientSideTimestamps getClientSideTimestamps() {
return this.clientSideTimestamps;
}
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*
* @param clientSideTimestamps
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable
* client-side timestamps with the following option:
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withClientSideTimestamps(ClientSideTimestamps clientSideTimestamps) {
setClientSideTimestamps(clientSideTimestamps);
return this;
}
/**
*
* The optional auto scaling settings for a table in provisioned capacity mode. Specifies if the service can manage
* throughput capacity automatically on your behalf.
*
*
* Auto scaling helps you provision throughput capacity for variable workloads efficiently by increasing and
* decreasing your table's read and write capacity automatically in response to application traffic. For more
* information, see Managing
* throughput capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer
* Guide.
*
*
* By default, auto scaling is disabled for a table.
*
*
* @param autoScalingSpecification
* The optional auto scaling settings for a table in provisioned capacity mode. Specifies if the service can
* manage throughput capacity automatically on your behalf.
*
* Auto scaling helps you provision throughput capacity for variable workloads efficiently by increasing and
* decreasing your table's read and write capacity automatically in response to application traffic. For more
* information, see Managing
* throughput capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces
* Developer Guide.
*
*
* By default, auto scaling is disabled for a table.
*/
public void setAutoScalingSpecification(AutoScalingSpecification autoScalingSpecification) {
this.autoScalingSpecification = autoScalingSpecification;
}
/**
*
* The optional auto scaling settings for a table in provisioned capacity mode. Specifies if the service can manage
* throughput capacity automatically on your behalf.
*
*
* Auto scaling helps you provision throughput capacity for variable workloads efficiently by increasing and
* decreasing your table's read and write capacity automatically in response to application traffic. For more
* information, see Managing
* throughput capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer
* Guide.
*
*
* By default, auto scaling is disabled for a table.
*
*
* @return The optional auto scaling settings for a table in provisioned capacity mode. Specifies if the service can
* manage throughput capacity automatically on your behalf.
*
* Auto scaling helps you provision throughput capacity for variable workloads efficiently by increasing and
* decreasing your table's read and write capacity automatically in response to application traffic. For
* more information, see Managing throughput
* capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer
* Guide.
*
*
* By default, auto scaling is disabled for a table.
*/
public AutoScalingSpecification getAutoScalingSpecification() {
return this.autoScalingSpecification;
}
/**
*
* The optional auto scaling settings for a table in provisioned capacity mode. Specifies if the service can manage
* throughput capacity automatically on your behalf.
*
*
* Auto scaling helps you provision throughput capacity for variable workloads efficiently by increasing and
* decreasing your table's read and write capacity automatically in response to application traffic. For more
* information, see Managing
* throughput capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer
* Guide.
*
*
* By default, auto scaling is disabled for a table.
*
*
* @param autoScalingSpecification
* The optional auto scaling settings for a table in provisioned capacity mode. Specifies if the service can
* manage throughput capacity automatically on your behalf.
*
* Auto scaling helps you provision throughput capacity for variable workloads efficiently by increasing and
* decreasing your table's read and write capacity automatically in response to application traffic. For more
* information, see Managing
* throughput capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces
* Developer Guide.
*
*
* By default, auto scaling is disabled for a table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withAutoScalingSpecification(AutoScalingSpecification autoScalingSpecification) {
setAutoScalingSpecification(autoScalingSpecification);
return this;
}
/**
*
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings overwrite the
* general settings of the table for the specified Region.
*
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity differently
* for each Region's replica. The write capacity, however, remains synchronized between all replicas to ensure that
* there's enough capacity to replicate writes across all Regions. To define the read capacity for a table replica
* in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
*
*
* @return The optional Amazon Web Services Region specific settings of a multi-Region table. These settings
* overwrite the general settings of the table for the specified Region.
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity
* differently for each Region's replica. The write capacity, however, remains synchronized between all
* replicas to ensure that there's enough capacity to replicate writes across all Regions. To define the
* read capacity for a table replica in a specific Region, you can do so by configuring the following
* parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
*/
public java.util.List getReplicaSpecifications() {
return replicaSpecifications;
}
/**
*
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings overwrite the
* general settings of the table for the specified Region.
*
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity differently
* for each Region's replica. The write capacity, however, remains synchronized between all replicas to ensure that
* there's enough capacity to replicate writes across all Regions. To define the read capacity for a table replica
* in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
*
*
* @param replicaSpecifications
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings
* overwrite the general settings of the table for the specified Region.
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity
* differently for each Region's replica. The write capacity, however, remains synchronized between all
* replicas to ensure that there's enough capacity to replicate writes across all Regions. To define the read
* capacity for a table replica in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
*/
public void setReplicaSpecifications(java.util.Collection replicaSpecifications) {
if (replicaSpecifications == null) {
this.replicaSpecifications = null;
return;
}
this.replicaSpecifications = new java.util.ArrayList(replicaSpecifications);
}
/**
*
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings overwrite the
* general settings of the table for the specified Region.
*
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity differently
* for each Region's replica. The write capacity, however, remains synchronized between all replicas to ensure that
* there's enough capacity to replicate writes across all Regions. To define the read capacity for a table replica
* in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReplicaSpecifications(java.util.Collection)} or
* {@link #withReplicaSpecifications(java.util.Collection)} if you want to override the existing values.
*
*
* @param replicaSpecifications
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings
* overwrite the general settings of the table for the specified Region.
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity
* differently for each Region's replica. The write capacity, however, remains synchronized between all
* replicas to ensure that there's enough capacity to replicate writes across all Regions. To define the read
* capacity for a table replica in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withReplicaSpecifications(ReplicaSpecification... replicaSpecifications) {
if (this.replicaSpecifications == null) {
setReplicaSpecifications(new java.util.ArrayList(replicaSpecifications.length));
}
for (ReplicaSpecification ele : replicaSpecifications) {
this.replicaSpecifications.add(ele);
}
return this;
}
/**
*
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings overwrite the
* general settings of the table for the specified Region.
*
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity differently
* for each Region's replica. The write capacity, however, remains synchronized between all replicas to ensure that
* there's enough capacity to replicate writes across all Regions. To define the read capacity for a table replica
* in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
*
*
* @param replicaSpecifications
* The optional Amazon Web Services Region specific settings of a multi-Region table. These settings
* overwrite the general settings of the table for the specified Region.
*
* For a multi-Region table in provisioned capacity mode, you can configure the table's read capacity
* differently for each Region's replica. The write capacity, however, remains synchronized between all
* replicas to ensure that there's enough capacity to replicate writes across all Regions. To define the read
* capacity for a table replica in a specific Region, you can do so by configuring the following parameters.
*
*
* -
*
* region
: The Region where these settings are applied. (Required)
*
*
* -
*
* readCapacityUnits
: The provisioned read capacity units. (Optional)
*
*
* -
*
* readCapacityAutoScaling
: The read capacity auto scaling settings for the table. (Optional)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTableRequest withReplicaSpecifications(java.util.Collection replicaSpecifications) {
setReplicaSpecifications(replicaSpecifications);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getKeyspaceName() != null)
sb.append("KeyspaceName: ").append(getKeyspaceName()).append(",");
if (getTableName() != null)
sb.append("TableName: ").append(getTableName()).append(",");
if (getSchemaDefinition() != null)
sb.append("SchemaDefinition: ").append(getSchemaDefinition()).append(",");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getCapacitySpecification() != null)
sb.append("CapacitySpecification: ").append(getCapacitySpecification()).append(",");
if (getEncryptionSpecification() != null)
sb.append("EncryptionSpecification: ").append(getEncryptionSpecification()).append(",");
if (getPointInTimeRecovery() != null)
sb.append("PointInTimeRecovery: ").append(getPointInTimeRecovery()).append(",");
if (getTtl() != null)
sb.append("Ttl: ").append(getTtl()).append(",");
if (getDefaultTimeToLive() != null)
sb.append("DefaultTimeToLive: ").append(getDefaultTimeToLive()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getClientSideTimestamps() != null)
sb.append("ClientSideTimestamps: ").append(getClientSideTimestamps()).append(",");
if (getAutoScalingSpecification() != null)
sb.append("AutoScalingSpecification: ").append(getAutoScalingSpecification()).append(",");
if (getReplicaSpecifications() != null)
sb.append("ReplicaSpecifications: ").append(getReplicaSpecifications());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateTableRequest == false)
return false;
CreateTableRequest other = (CreateTableRequest) obj;
if (other.getKeyspaceName() == null ^ this.getKeyspaceName() == null)
return false;
if (other.getKeyspaceName() != null && other.getKeyspaceName().equals(this.getKeyspaceName()) == false)
return false;
if (other.getTableName() == null ^ this.getTableName() == null)
return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false)
return false;
if (other.getSchemaDefinition() == null ^ this.getSchemaDefinition() == null)
return false;
if (other.getSchemaDefinition() != null && other.getSchemaDefinition().equals(this.getSchemaDefinition()) == false)
return false;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getCapacitySpecification() == null ^ this.getCapacitySpecification() == null)
return false;
if (other.getCapacitySpecification() != null && other.getCapacitySpecification().equals(this.getCapacitySpecification()) == false)
return false;
if (other.getEncryptionSpecification() == null ^ this.getEncryptionSpecification() == null)
return false;
if (other.getEncryptionSpecification() != null && other.getEncryptionSpecification().equals(this.getEncryptionSpecification()) == false)
return false;
if (other.getPointInTimeRecovery() == null ^ this.getPointInTimeRecovery() == null)
return false;
if (other.getPointInTimeRecovery() != null && other.getPointInTimeRecovery().equals(this.getPointInTimeRecovery()) == false)
return false;
if (other.getTtl() == null ^ this.getTtl() == null)
return false;
if (other.getTtl() != null && other.getTtl().equals(this.getTtl()) == false)
return false;
if (other.getDefaultTimeToLive() == null ^ this.getDefaultTimeToLive() == null)
return false;
if (other.getDefaultTimeToLive() != null && other.getDefaultTimeToLive().equals(this.getDefaultTimeToLive()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getClientSideTimestamps() == null ^ this.getClientSideTimestamps() == null)
return false;
if (other.getClientSideTimestamps() != null && other.getClientSideTimestamps().equals(this.getClientSideTimestamps()) == false)
return false;
if (other.getAutoScalingSpecification() == null ^ this.getAutoScalingSpecification() == null)
return false;
if (other.getAutoScalingSpecification() != null && other.getAutoScalingSpecification().equals(this.getAutoScalingSpecification()) == false)
return false;
if (other.getReplicaSpecifications() == null ^ this.getReplicaSpecifications() == null)
return false;
if (other.getReplicaSpecifications() != null && other.getReplicaSpecifications().equals(this.getReplicaSpecifications()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getKeyspaceName() == null) ? 0 : getKeyspaceName().hashCode());
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getSchemaDefinition() == null) ? 0 : getSchemaDefinition().hashCode());
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getCapacitySpecification() == null) ? 0 : getCapacitySpecification().hashCode());
hashCode = prime * hashCode + ((getEncryptionSpecification() == null) ? 0 : getEncryptionSpecification().hashCode());
hashCode = prime * hashCode + ((getPointInTimeRecovery() == null) ? 0 : getPointInTimeRecovery().hashCode());
hashCode = prime * hashCode + ((getTtl() == null) ? 0 : getTtl().hashCode());
hashCode = prime * hashCode + ((getDefaultTimeToLive() == null) ? 0 : getDefaultTimeToLive().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getClientSideTimestamps() == null) ? 0 : getClientSideTimestamps().hashCode());
hashCode = prime * hashCode + ((getAutoScalingSpecification() == null) ? 0 : getAutoScalingSpecification().hashCode());
hashCode = prime * hashCode + ((getReplicaSpecifications() == null) ? 0 : getReplicaSpecifications().hashCode());
return hashCode;
}
@Override
public CreateTableRequest clone() {
return (CreateTableRequest) super.clone();
}
}