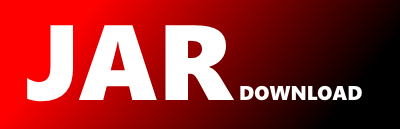
com.amazonaws.services.keyspaces.model.UpdateTableRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-keyspaces Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.keyspaces.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateTableRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the keyspace the specified table is stored in.
*
*/
private String keyspaceName;
/**
*
* The name of the table.
*
*/
private String tableName;
/**
*
* For each column to be added to the specified table:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*/
private java.util.List addColumns;
/**
*
* Modifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*/
private CapacitySpecification capacitySpecification;
/**
*
* Modifies the encryption settings of the table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*/
private EncryptionSpecification encryptionSpecification;
/**
*
* Modifies the pointInTimeRecovery
settings of the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*/
private PointInTimeRecovery pointInTimeRecovery;
/**
*
* Modifies Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*/
private TimeToLive ttl;
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*/
private Integer defaultTimeToLive;
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*/
private ClientSideTimestamps clientSideTimestamps;
/**
*
* The optional auto scaling settings to update for a table in provisioned capacity mode. Specifies if the service
* can manage throughput capacity of a provisioned table automatically on your behalf. Amazon Keyspaces auto scaling
* helps you provision throughput capacity for variable workloads efficiently by increasing and decreasing your
* table's read and write capacity automatically in response to application traffic.
*
*
* If auto scaling is already enabled for the table, you can use UpdateTable
to update the minimum and
* maximum values or the auto scaling policy settings independently.
*
*
* For more information, see Managing throughput capacity
* automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer Guide.
*
*/
private AutoScalingSpecification autoScalingSpecification;
/**
*
* The Region specific settings of a multi-Regional table.
*
*/
private java.util.List replicaSpecifications;
/**
*
* The name of the keyspace the specified table is stored in.
*
*
* @param keyspaceName
* The name of the keyspace the specified table is stored in.
*/
public void setKeyspaceName(String keyspaceName) {
this.keyspaceName = keyspaceName;
}
/**
*
* The name of the keyspace the specified table is stored in.
*
*
* @return The name of the keyspace the specified table is stored in.
*/
public String getKeyspaceName() {
return this.keyspaceName;
}
/**
*
* The name of the keyspace the specified table is stored in.
*
*
* @param keyspaceName
* The name of the keyspace the specified table is stored in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withKeyspaceName(String keyspaceName) {
setKeyspaceName(keyspaceName);
return this;
}
/**
*
* The name of the table.
*
*
* @param tableName
* The name of the table.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
*
* The name of the table.
*
*
* @return The name of the table.
*/
public String getTableName() {
return this.tableName;
}
/**
*
* The name of the table.
*
*
* @param tableName
* The name of the table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withTableName(String tableName) {
setTableName(tableName);
return this;
}
/**
*
* For each column to be added to the specified table:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* @return For each column to be added to the specified table:
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data
* types in the Amazon Keyspaces Developer Guide.
*
*
*/
public java.util.List getAddColumns() {
return addColumns;
}
/**
*
* For each column to be added to the specified table:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* @param addColumns
* For each column to be added to the specified table:
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data
* types in the Amazon Keyspaces Developer Guide.
*
*
*/
public void setAddColumns(java.util.Collection addColumns) {
if (addColumns == null) {
this.addColumns = null;
return;
}
this.addColumns = new java.util.ArrayList(addColumns);
}
/**
*
* For each column to be added to the specified table:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAddColumns(java.util.Collection)} or {@link #withAddColumns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param addColumns
* For each column to be added to the specified table:
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data
* types in the Amazon Keyspaces Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withAddColumns(ColumnDefinition... addColumns) {
if (this.addColumns == null) {
setAddColumns(new java.util.ArrayList(addColumns.length));
}
for (ColumnDefinition ele : addColumns) {
this.addColumns.add(ele);
}
return this;
}
/**
*
* For each column to be added to the specified table:
*
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data types in
* the Amazon Keyspaces Developer Guide.
*
*
*
*
* @param addColumns
* For each column to be added to the specified table:
*
* -
*
* name
- The name of the column.
*
*
* -
*
* type
- An Amazon Keyspaces data type. For more information, see Data
* types in the Amazon Keyspaces Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withAddColumns(java.util.Collection addColumns) {
setAddColumns(addColumns);
return this;
}
/**
*
* Modifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @param capacitySpecification
* Modifies the read/write throughput capacity mode for the table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
*/
public void setCapacitySpecification(CapacitySpecification capacitySpecification) {
this.capacitySpecification = capacitySpecification;
}
/**
*
* Modifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @return Modifies the read/write throughput capacity mode for the table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
*/
public CapacitySpecification getCapacitySpecification() {
return this.capacitySpecification;
}
/**
*
* Modifies the read/write throughput capacity mode for the table. The options are:
*
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires readCapacityUnits
and
* writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write capacity
* modes in the Amazon Keyspaces Developer Guide.
*
*
* @param capacitySpecification
* Modifies the read/write throughput capacity mode for the table. The options are:
*
* -
*
* throughputMode:PAY_PER_REQUEST
and
*
*
* -
*
* throughputMode:PROVISIONED
- Provisioned capacity mode requires
* readCapacityUnits
and writeCapacityUnits
as input.
*
*
*
*
* The default is throughput_mode:PAY_PER_REQUEST
.
*
*
* For more information, see Read/write
* capacity modes in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withCapacitySpecification(CapacitySpecification capacitySpecification) {
setCapacitySpecification(capacitySpecification);
return this;
}
/**
*
* Modifies the encryption settings of the table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @param encryptionSpecification
* Modifies the encryption settings of the table. You can choose one of the following KMS key (KMS key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource
* Name (ARN) format as input.
*
*
*
*
* The default is AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
*/
public void setEncryptionSpecification(EncryptionSpecification encryptionSpecification) {
this.encryptionSpecification = encryptionSpecification;
}
/**
*
* Modifies the encryption settings of the table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @return Modifies the encryption settings of the table. You can choose one of the following KMS key (KMS key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned,
* and managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon
* Resource Name (ARN) format as input.
*
*
*
*
* The default is AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
*/
public EncryptionSpecification getEncryptionSpecification() {
return this.encryptionSpecification;
}
/**
*
* Modifies the encryption settings of the table. You can choose one of the following KMS key (KMS key):
*
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource Name
* (ARN) format as input.
*
*
*
*
* The default is AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest in the
* Amazon Keyspaces Developer Guide.
*
*
* @param encryptionSpecification
* Modifies the encryption settings of the table. You can choose one of the following KMS key (KMS key):
*
* -
*
* type:AWS_OWNED_KMS_KEY
- This key is owned by Amazon Keyspaces.
*
*
* -
*
* type:CUSTOMER_MANAGED_KMS_KEY
- This key is stored in your account and is created, owned, and
* managed by you. This option requires the kms_key_identifier
of the KMS key in Amazon Resource
* Name (ARN) format as input.
*
*
*
*
* The default is AWS_OWNED_KMS_KEY
.
*
*
* For more information, see Encryption at rest
* in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withEncryptionSpecification(EncryptionSpecification encryptionSpecification) {
setEncryptionSpecification(encryptionSpecification);
return this;
}
/**
*
* Modifies the pointInTimeRecovery
settings of the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @param pointInTimeRecovery
* Modifies the pointInTimeRecovery
settings of the table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
*/
public void setPointInTimeRecovery(PointInTimeRecovery pointInTimeRecovery) {
this.pointInTimeRecovery = pointInTimeRecovery;
}
/**
*
* Modifies the pointInTimeRecovery
settings of the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @return Modifies the pointInTimeRecovery
settings of the table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
*/
public PointInTimeRecovery getPointInTimeRecovery() {
return this.pointInTimeRecovery;
}
/**
*
* Modifies the pointInTimeRecovery
settings of the table. The options are:
*
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time recovery
* in the Amazon Keyspaces Developer Guide.
*
*
* @param pointInTimeRecovery
* Modifies the pointInTimeRecovery
settings of the table. The options are:
*
* -
*
* status=ENABLED
*
*
* -
*
* status=DISABLED
*
*
*
*
* If it's not specified, the default is status=DISABLED
.
*
*
* For more information, see Point-in-time
* recovery in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withPointInTimeRecovery(PointInTimeRecovery pointInTimeRecovery) {
setPointInTimeRecovery(pointInTimeRecovery);
return this;
}
/**
*
* Modifies Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*
* @param ttl
* Modifies Time to Live custom settings for the table. The options are:
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for
* the table.
*
*
* For more information, see Expiring data by using Amazon
* Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*/
public void setTtl(TimeToLive ttl) {
this.ttl = ttl;
}
/**
*
* Modifies Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*
* @return Modifies Time to Live custom settings for the table. The options are:
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for
* the table.
*
*
* For more information, see Expiring data by using Amazon
* Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*/
public TimeToLive getTtl() {
return this.ttl;
}
/**
*
* Modifies Time to Live custom settings for the table. The options are:
*
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for the
* table.
*
*
* For more information, see Expiring data
* by using Amazon Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
*
*
* @param ttl
* Modifies Time to Live custom settings for the table. The options are:
*
* -
*
* status:enabled
*
*
* -
*
* status:disabled
*
*
*
*
* The default is status:disabled
. After ttl
is enabled, you can't disable it for
* the table.
*
*
* For more information, see Expiring data by using Amazon
* Keyspaces Time to Live (TTL) in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withTtl(TimeToLive ttl) {
setTtl(ttl);
return this;
}
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*
* @param defaultTimeToLive
* The default Time to Live setting in seconds for the table.
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*/
public void setDefaultTimeToLive(Integer defaultTimeToLive) {
this.defaultTimeToLive = defaultTimeToLive;
}
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*
* @return The default Time to Live setting in seconds for the table.
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*/
public Integer getDefaultTimeToLive() {
return this.defaultTimeToLive;
}
/**
*
* The default Time to Live setting in seconds for the table.
*
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
*
*
* @param defaultTimeToLive
* The default Time to Live setting in seconds for the table.
*
* For more information, see Setting the default TTL value for a table in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withDefaultTimeToLive(Integer defaultTimeToLive) {
setDefaultTimeToLive(defaultTimeToLive);
return this;
}
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*
* @param clientSideTimestamps
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable
* client-side timestamps with the following option:
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*/
public void setClientSideTimestamps(ClientSideTimestamps clientSideTimestamps) {
this.clientSideTimestamps = clientSideTimestamps;
}
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*
* @return Enables client-side timestamps for the table. By default, the setting is disabled. You can enable
* client-side timestamps with the following option:
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*/
public ClientSideTimestamps getClientSideTimestamps() {
return this.clientSideTimestamps;
}
/**
*
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable client-side
* timestamps with the following option:
*
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
*
*
* @param clientSideTimestamps
* Enables client-side timestamps for the table. By default, the setting is disabled. You can enable
* client-side timestamps with the following option:
*
* -
*
* status: "enabled"
*
*
*
*
* Once client-side timestamps are enabled for a table, this setting cannot be disabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withClientSideTimestamps(ClientSideTimestamps clientSideTimestamps) {
setClientSideTimestamps(clientSideTimestamps);
return this;
}
/**
*
* The optional auto scaling settings to update for a table in provisioned capacity mode. Specifies if the service
* can manage throughput capacity of a provisioned table automatically on your behalf. Amazon Keyspaces auto scaling
* helps you provision throughput capacity for variable workloads efficiently by increasing and decreasing your
* table's read and write capacity automatically in response to application traffic.
*
*
* If auto scaling is already enabled for the table, you can use UpdateTable
to update the minimum and
* maximum values or the auto scaling policy settings independently.
*
*
* For more information, see Managing throughput capacity
* automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer Guide.
*
*
* @param autoScalingSpecification
* The optional auto scaling settings to update for a table in provisioned capacity mode. Specifies if the
* service can manage throughput capacity of a provisioned table automatically on your behalf. Amazon
* Keyspaces auto scaling helps you provision throughput capacity for variable workloads efficiently by
* increasing and decreasing your table's read and write capacity automatically in response to application
* traffic.
*
* If auto scaling is already enabled for the table, you can use UpdateTable
to update the
* minimum and maximum values or the auto scaling policy settings independently.
*
*
* For more information, see Managing throughput capacity
* automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer Guide.
*/
public void setAutoScalingSpecification(AutoScalingSpecification autoScalingSpecification) {
this.autoScalingSpecification = autoScalingSpecification;
}
/**
*
* The optional auto scaling settings to update for a table in provisioned capacity mode. Specifies if the service
* can manage throughput capacity of a provisioned table automatically on your behalf. Amazon Keyspaces auto scaling
* helps you provision throughput capacity for variable workloads efficiently by increasing and decreasing your
* table's read and write capacity automatically in response to application traffic.
*
*
* If auto scaling is already enabled for the table, you can use UpdateTable
to update the minimum and
* maximum values or the auto scaling policy settings independently.
*
*
* For more information, see Managing throughput capacity
* automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer Guide.
*
*
* @return The optional auto scaling settings to update for a table in provisioned capacity mode. Specifies if the
* service can manage throughput capacity of a provisioned table automatically on your behalf. Amazon
* Keyspaces auto scaling helps you provision throughput capacity for variable workloads efficiently by
* increasing and decreasing your table's read and write capacity automatically in response to application
* traffic.
*
* If auto scaling is already enabled for the table, you can use UpdateTable
to update the
* minimum and maximum values or the auto scaling policy settings independently.
*
*
* For more information, see Managing throughput
* capacity automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer
* Guide.
*/
public AutoScalingSpecification getAutoScalingSpecification() {
return this.autoScalingSpecification;
}
/**
*
* The optional auto scaling settings to update for a table in provisioned capacity mode. Specifies if the service
* can manage throughput capacity of a provisioned table automatically on your behalf. Amazon Keyspaces auto scaling
* helps you provision throughput capacity for variable workloads efficiently by increasing and decreasing your
* table's read and write capacity automatically in response to application traffic.
*
*
* If auto scaling is already enabled for the table, you can use UpdateTable
to update the minimum and
* maximum values or the auto scaling policy settings independently.
*
*
* For more information, see Managing throughput capacity
* automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer Guide.
*
*
* @param autoScalingSpecification
* The optional auto scaling settings to update for a table in provisioned capacity mode. Specifies if the
* service can manage throughput capacity of a provisioned table automatically on your behalf. Amazon
* Keyspaces auto scaling helps you provision throughput capacity for variable workloads efficiently by
* increasing and decreasing your table's read and write capacity automatically in response to application
* traffic.
*
* If auto scaling is already enabled for the table, you can use UpdateTable
to update the
* minimum and maximum values or the auto scaling policy settings independently.
*
*
* For more information, see Managing throughput capacity
* automatically with Amazon Keyspaces auto scaling in the Amazon Keyspaces Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withAutoScalingSpecification(AutoScalingSpecification autoScalingSpecification) {
setAutoScalingSpecification(autoScalingSpecification);
return this;
}
/**
*
* The Region specific settings of a multi-Regional table.
*
*
* @return The Region specific settings of a multi-Regional table.
*/
public java.util.List getReplicaSpecifications() {
return replicaSpecifications;
}
/**
*
* The Region specific settings of a multi-Regional table.
*
*
* @param replicaSpecifications
* The Region specific settings of a multi-Regional table.
*/
public void setReplicaSpecifications(java.util.Collection replicaSpecifications) {
if (replicaSpecifications == null) {
this.replicaSpecifications = null;
return;
}
this.replicaSpecifications = new java.util.ArrayList(replicaSpecifications);
}
/**
*
* The Region specific settings of a multi-Regional table.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReplicaSpecifications(java.util.Collection)} or
* {@link #withReplicaSpecifications(java.util.Collection)} if you want to override the existing values.
*
*
* @param replicaSpecifications
* The Region specific settings of a multi-Regional table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withReplicaSpecifications(ReplicaSpecification... replicaSpecifications) {
if (this.replicaSpecifications == null) {
setReplicaSpecifications(new java.util.ArrayList(replicaSpecifications.length));
}
for (ReplicaSpecification ele : replicaSpecifications) {
this.replicaSpecifications.add(ele);
}
return this;
}
/**
*
* The Region specific settings of a multi-Regional table.
*
*
* @param replicaSpecifications
* The Region specific settings of a multi-Regional table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTableRequest withReplicaSpecifications(java.util.Collection replicaSpecifications) {
setReplicaSpecifications(replicaSpecifications);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getKeyspaceName() != null)
sb.append("KeyspaceName: ").append(getKeyspaceName()).append(",");
if (getTableName() != null)
sb.append("TableName: ").append(getTableName()).append(",");
if (getAddColumns() != null)
sb.append("AddColumns: ").append(getAddColumns()).append(",");
if (getCapacitySpecification() != null)
sb.append("CapacitySpecification: ").append(getCapacitySpecification()).append(",");
if (getEncryptionSpecification() != null)
sb.append("EncryptionSpecification: ").append(getEncryptionSpecification()).append(",");
if (getPointInTimeRecovery() != null)
sb.append("PointInTimeRecovery: ").append(getPointInTimeRecovery()).append(",");
if (getTtl() != null)
sb.append("Ttl: ").append(getTtl()).append(",");
if (getDefaultTimeToLive() != null)
sb.append("DefaultTimeToLive: ").append(getDefaultTimeToLive()).append(",");
if (getClientSideTimestamps() != null)
sb.append("ClientSideTimestamps: ").append(getClientSideTimestamps()).append(",");
if (getAutoScalingSpecification() != null)
sb.append("AutoScalingSpecification: ").append(getAutoScalingSpecification()).append(",");
if (getReplicaSpecifications() != null)
sb.append("ReplicaSpecifications: ").append(getReplicaSpecifications());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateTableRequest == false)
return false;
UpdateTableRequest other = (UpdateTableRequest) obj;
if (other.getKeyspaceName() == null ^ this.getKeyspaceName() == null)
return false;
if (other.getKeyspaceName() != null && other.getKeyspaceName().equals(this.getKeyspaceName()) == false)
return false;
if (other.getTableName() == null ^ this.getTableName() == null)
return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false)
return false;
if (other.getAddColumns() == null ^ this.getAddColumns() == null)
return false;
if (other.getAddColumns() != null && other.getAddColumns().equals(this.getAddColumns()) == false)
return false;
if (other.getCapacitySpecification() == null ^ this.getCapacitySpecification() == null)
return false;
if (other.getCapacitySpecification() != null && other.getCapacitySpecification().equals(this.getCapacitySpecification()) == false)
return false;
if (other.getEncryptionSpecification() == null ^ this.getEncryptionSpecification() == null)
return false;
if (other.getEncryptionSpecification() != null && other.getEncryptionSpecification().equals(this.getEncryptionSpecification()) == false)
return false;
if (other.getPointInTimeRecovery() == null ^ this.getPointInTimeRecovery() == null)
return false;
if (other.getPointInTimeRecovery() != null && other.getPointInTimeRecovery().equals(this.getPointInTimeRecovery()) == false)
return false;
if (other.getTtl() == null ^ this.getTtl() == null)
return false;
if (other.getTtl() != null && other.getTtl().equals(this.getTtl()) == false)
return false;
if (other.getDefaultTimeToLive() == null ^ this.getDefaultTimeToLive() == null)
return false;
if (other.getDefaultTimeToLive() != null && other.getDefaultTimeToLive().equals(this.getDefaultTimeToLive()) == false)
return false;
if (other.getClientSideTimestamps() == null ^ this.getClientSideTimestamps() == null)
return false;
if (other.getClientSideTimestamps() != null && other.getClientSideTimestamps().equals(this.getClientSideTimestamps()) == false)
return false;
if (other.getAutoScalingSpecification() == null ^ this.getAutoScalingSpecification() == null)
return false;
if (other.getAutoScalingSpecification() != null && other.getAutoScalingSpecification().equals(this.getAutoScalingSpecification()) == false)
return false;
if (other.getReplicaSpecifications() == null ^ this.getReplicaSpecifications() == null)
return false;
if (other.getReplicaSpecifications() != null && other.getReplicaSpecifications().equals(this.getReplicaSpecifications()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getKeyspaceName() == null) ? 0 : getKeyspaceName().hashCode());
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getAddColumns() == null) ? 0 : getAddColumns().hashCode());
hashCode = prime * hashCode + ((getCapacitySpecification() == null) ? 0 : getCapacitySpecification().hashCode());
hashCode = prime * hashCode + ((getEncryptionSpecification() == null) ? 0 : getEncryptionSpecification().hashCode());
hashCode = prime * hashCode + ((getPointInTimeRecovery() == null) ? 0 : getPointInTimeRecovery().hashCode());
hashCode = prime * hashCode + ((getTtl() == null) ? 0 : getTtl().hashCode());
hashCode = prime * hashCode + ((getDefaultTimeToLive() == null) ? 0 : getDefaultTimeToLive().hashCode());
hashCode = prime * hashCode + ((getClientSideTimestamps() == null) ? 0 : getClientSideTimestamps().hashCode());
hashCode = prime * hashCode + ((getAutoScalingSpecification() == null) ? 0 : getAutoScalingSpecification().hashCode());
hashCode = prime * hashCode + ((getReplicaSpecifications() == null) ? 0 : getReplicaSpecifications().hashCode());
return hashCode;
}
@Override
public UpdateTableRequest clone() {
return (UpdateTableRequest) super.clone();
}
}