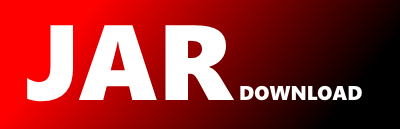
com.amazonaws.services.kinesis.model.StreamDescription Maven / Gradle / Ivy
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.kinesis.model;
import java.io.Serializable;
/**
*
* Represents the output for DescribeStream.
*
*/
public class StreamDescription implements Serializable, Cloneable {
/**
*
* The name of the stream being described.
*
*/
private String streamName;
/**
*
* The Amazon Resource Name (ARN) for the stream being described.
*
*/
private String streamARN;
/**
*
* The current status of the stream being described. The stream status is
* one of the following states:
*
*
* CREATING
- The stream is being created. Amazon Kinesis
* immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The specified
* stream is in the DELETING
state until Amazon Kinesis
* completes the deletion.
* ACTIVE
- The stream exists and is ready for read and
* write operations or deletion. You should perform read and write
* operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged or
* split. Read and write operations continue to work while the stream is in
* the UPDATING
state.
*
*/
private String streamStatus;
/**
*
* The shards that comprise the stream.
*
*/
private com.amazonaws.internal.SdkInternalList shards;
/**
*
* If set to true
, more shards in the stream are available to
* describe.
*
*/
private Boolean hasMoreShards;
/**
*
* The current retention period, in hours.
*
*/
private Integer retentionPeriodHours;
/**
*
* Represents the current enhanced monitoring settings of the stream.
*
*/
private com.amazonaws.internal.SdkInternalList enhancedMonitoring;
/**
*
* The name of the stream being described.
*
*
* @param streamName
* The name of the stream being described.
*/
public void setStreamName(String streamName) {
this.streamName = streamName;
}
/**
*
* The name of the stream being described.
*
*
* @return The name of the stream being described.
*/
public String getStreamName() {
return this.streamName;
}
/**
*
* The name of the stream being described.
*
*
* @param streamName
* The name of the stream being described.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withStreamName(String streamName) {
setStreamName(streamName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the stream being described.
*
*
* @param streamARN
* The Amazon Resource Name (ARN) for the stream being described.
*/
public void setStreamARN(String streamARN) {
this.streamARN = streamARN;
}
/**
*
* The Amazon Resource Name (ARN) for the stream being described.
*
*
* @return The Amazon Resource Name (ARN) for the stream being described.
*/
public String getStreamARN() {
return this.streamARN;
}
/**
*
* The Amazon Resource Name (ARN) for the stream being described.
*
*
* @param streamARN
* The Amazon Resource Name (ARN) for the stream being described.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withStreamARN(String streamARN) {
setStreamARN(streamARN);
return this;
}
/**
*
* The current status of the stream being described. The stream status is
* one of the following states:
*
*
* CREATING
- The stream is being created. Amazon Kinesis
* immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The specified
* stream is in the DELETING
state until Amazon Kinesis
* completes the deletion.
* ACTIVE
- The stream exists and is ready for read and
* write operations or deletion. You should perform read and write
* operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged or
* split. Read and write operations continue to work while the stream is in
* the UPDATING
state.
*
*
* @param streamStatus
* The current status of the stream being described. The stream
* status is one of the following states:
*
* CREATING
- The stream is being created. Amazon
* Kinesis immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The
* specified stream is in the DELETING
state until
* Amazon Kinesis completes the deletion.
* ACTIVE
- The stream exists and is ready for read
* and write operations or deletion. You should perform read and
* write operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged
* or split. Read and write operations continue to work while the
* stream is in the UPDATING
state.
* @see StreamStatus
*/
public void setStreamStatus(String streamStatus) {
this.streamStatus = streamStatus;
}
/**
*
* The current status of the stream being described. The stream status is
* one of the following states:
*
*
* CREATING
- The stream is being created. Amazon Kinesis
* immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The specified
* stream is in the DELETING
state until Amazon Kinesis
* completes the deletion.
* ACTIVE
- The stream exists and is ready for read and
* write operations or deletion. You should perform read and write
* operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged or
* split. Read and write operations continue to work while the stream is in
* the UPDATING
state.
*
*
* @return The current status of the stream being described. The stream
* status is one of the following states:
*
* CREATING
- The stream is being created. Amazon
* Kinesis immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The
* specified stream is in the DELETING
state until
* Amazon Kinesis completes the deletion.
* ACTIVE
- The stream exists and is ready for read
* and write operations or deletion. You should perform read and
* write operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged
* or split. Read and write operations continue to work while the
* stream is in the UPDATING
state.
* @see StreamStatus
*/
public String getStreamStatus() {
return this.streamStatus;
}
/**
*
* The current status of the stream being described. The stream status is
* one of the following states:
*
*
* CREATING
- The stream is being created. Amazon Kinesis
* immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The specified
* stream is in the DELETING
state until Amazon Kinesis
* completes the deletion.
* ACTIVE
- The stream exists and is ready for read and
* write operations or deletion. You should perform read and write
* operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged or
* split. Read and write operations continue to work while the stream is in
* the UPDATING
state.
*
*
* @param streamStatus
* The current status of the stream being described. The stream
* status is one of the following states:
*
* CREATING
- The stream is being created. Amazon
* Kinesis immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The
* specified stream is in the DELETING
state until
* Amazon Kinesis completes the deletion.
* ACTIVE
- The stream exists and is ready for read
* and write operations or deletion. You should perform read and
* write operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged
* or split. Read and write operations continue to work while the
* stream is in the UPDATING
state.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see StreamStatus
*/
public StreamDescription withStreamStatus(String streamStatus) {
setStreamStatus(streamStatus);
return this;
}
/**
*
* The current status of the stream being described. The stream status is
* one of the following states:
*
*
* CREATING
- The stream is being created. Amazon Kinesis
* immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The specified
* stream is in the DELETING
state until Amazon Kinesis
* completes the deletion.
* ACTIVE
- The stream exists and is ready for read and
* write operations or deletion. You should perform read and write
* operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged or
* split. Read and write operations continue to work while the stream is in
* the UPDATING
state.
*
*
* @param streamStatus
* The current status of the stream being described. The stream
* status is one of the following states:
*
* CREATING
- The stream is being created. Amazon
* Kinesis immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The
* specified stream is in the DELETING
state until
* Amazon Kinesis completes the deletion.
* ACTIVE
- The stream exists and is ready for read
* and write operations or deletion. You should perform read and
* write operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged
* or split. Read and write operations continue to work while the
* stream is in the UPDATING
state.
* @see StreamStatus
*/
public void setStreamStatus(StreamStatus streamStatus) {
this.streamStatus = streamStatus.toString();
}
/**
*
* The current status of the stream being described. The stream status is
* one of the following states:
*
*
* CREATING
- The stream is being created. Amazon Kinesis
* immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The specified
* stream is in the DELETING
state until Amazon Kinesis
* completes the deletion.
* ACTIVE
- The stream exists and is ready for read and
* write operations or deletion. You should perform read and write
* operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged or
* split. Read and write operations continue to work while the stream is in
* the UPDATING
state.
*
*
* @param streamStatus
* The current status of the stream being described. The stream
* status is one of the following states:
*
* CREATING
- The stream is being created. Amazon
* Kinesis immediately returns and sets StreamStatus
to
* CREATING
.
* DELETING
- The stream is being deleted. The
* specified stream is in the DELETING
state until
* Amazon Kinesis completes the deletion.
* ACTIVE
- The stream exists and is ready for read
* and write operations or deletion. You should perform read and
* write operations only on an ACTIVE
stream.
* UPDATING
- Shards in the stream are being merged
* or split. Read and write operations continue to work while the
* stream is in the UPDATING
state.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see StreamStatus
*/
public StreamDescription withStreamStatus(StreamStatus streamStatus) {
setStreamStatus(streamStatus);
return this;
}
/**
*
* The shards that comprise the stream.
*
*
* @return The shards that comprise the stream.
*/
public java.util.List getShards() {
if (shards == null) {
shards = new com.amazonaws.internal.SdkInternalList();
}
return shards;
}
/**
*
* The shards that comprise the stream.
*
*
* @param shards
* The shards that comprise the stream.
*/
public void setShards(java.util.Collection shards) {
if (shards == null) {
this.shards = null;
return;
}
this.shards = new com.amazonaws.internal.SdkInternalList(shards);
}
/**
*
* The shards that comprise the stream.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setShards(java.util.Collection)} or
* {@link #withShards(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param shards
* The shards that comprise the stream.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withShards(Shard... shards) {
if (this.shards == null) {
setShards(new com.amazonaws.internal.SdkInternalList(
shards.length));
}
for (Shard ele : shards) {
this.shards.add(ele);
}
return this;
}
/**
*
* The shards that comprise the stream.
*
*
* @param shards
* The shards that comprise the stream.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withShards(java.util.Collection shards) {
setShards(shards);
return this;
}
/**
*
* If set to true
, more shards in the stream are available to
* describe.
*
*
* @param hasMoreShards
* If set to true
, more shards in the stream are
* available to describe.
*/
public void setHasMoreShards(Boolean hasMoreShards) {
this.hasMoreShards = hasMoreShards;
}
/**
*
* If set to true
, more shards in the stream are available to
* describe.
*
*
* @return If set to true
, more shards in the stream are
* available to describe.
*/
public Boolean getHasMoreShards() {
return this.hasMoreShards;
}
/**
*
* If set to true
, more shards in the stream are available to
* describe.
*
*
* @param hasMoreShards
* If set to true
, more shards in the stream are
* available to describe.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withHasMoreShards(Boolean hasMoreShards) {
setHasMoreShards(hasMoreShards);
return this;
}
/**
*
* If set to true
, more shards in the stream are available to
* describe.
*
*
* @return If set to true
, more shards in the stream are
* available to describe.
*/
public Boolean isHasMoreShards() {
return this.hasMoreShards;
}
/**
*
* The current retention period, in hours.
*
*
* @param retentionPeriodHours
* The current retention period, in hours.
*/
public void setRetentionPeriodHours(Integer retentionPeriodHours) {
this.retentionPeriodHours = retentionPeriodHours;
}
/**
*
* The current retention period, in hours.
*
*
* @return The current retention period, in hours.
*/
public Integer getRetentionPeriodHours() {
return this.retentionPeriodHours;
}
/**
*
* The current retention period, in hours.
*
*
* @param retentionPeriodHours
* The current retention period, in hours.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withRetentionPeriodHours(
Integer retentionPeriodHours) {
setRetentionPeriodHours(retentionPeriodHours);
return this;
}
/**
*
* Represents the current enhanced monitoring settings of the stream.
*
*
* @return Represents the current enhanced monitoring settings of the
* stream.
*/
public java.util.List getEnhancedMonitoring() {
if (enhancedMonitoring == null) {
enhancedMonitoring = new com.amazonaws.internal.SdkInternalList();
}
return enhancedMonitoring;
}
/**
*
* Represents the current enhanced monitoring settings of the stream.
*
*
* @param enhancedMonitoring
* Represents the current enhanced monitoring settings of the stream.
*/
public void setEnhancedMonitoring(
java.util.Collection enhancedMonitoring) {
if (enhancedMonitoring == null) {
this.enhancedMonitoring = null;
return;
}
this.enhancedMonitoring = new com.amazonaws.internal.SdkInternalList(
enhancedMonitoring);
}
/**
*
* Represents the current enhanced monitoring settings of the stream.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setEnhancedMonitoring(java.util.Collection)} or
* {@link #withEnhancedMonitoring(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param enhancedMonitoring
* Represents the current enhanced monitoring settings of the stream.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withEnhancedMonitoring(
EnhancedMetrics... enhancedMonitoring) {
if (this.enhancedMonitoring == null) {
setEnhancedMonitoring(new com.amazonaws.internal.SdkInternalList(
enhancedMonitoring.length));
}
for (EnhancedMetrics ele : enhancedMonitoring) {
this.enhancedMonitoring.add(ele);
}
return this;
}
/**
*
* Represents the current enhanced monitoring settings of the stream.
*
*
* @param enhancedMonitoring
* Represents the current enhanced monitoring settings of the stream.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StreamDescription withEnhancedMonitoring(
java.util.Collection enhancedMonitoring) {
setEnhancedMonitoring(enhancedMonitoring);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStreamName() != null)
sb.append("StreamName: " + getStreamName() + ",");
if (getStreamARN() != null)
sb.append("StreamARN: " + getStreamARN() + ",");
if (getStreamStatus() != null)
sb.append("StreamStatus: " + getStreamStatus() + ",");
if (getShards() != null)
sb.append("Shards: " + getShards() + ",");
if (getHasMoreShards() != null)
sb.append("HasMoreShards: " + getHasMoreShards() + ",");
if (getRetentionPeriodHours() != null)
sb.append("RetentionPeriodHours: " + getRetentionPeriodHours()
+ ",");
if (getEnhancedMonitoring() != null)
sb.append("EnhancedMonitoring: " + getEnhancedMonitoring());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StreamDescription == false)
return false;
StreamDescription other = (StreamDescription) obj;
if (other.getStreamName() == null ^ this.getStreamName() == null)
return false;
if (other.getStreamName() != null
&& other.getStreamName().equals(this.getStreamName()) == false)
return false;
if (other.getStreamARN() == null ^ this.getStreamARN() == null)
return false;
if (other.getStreamARN() != null
&& other.getStreamARN().equals(this.getStreamARN()) == false)
return false;
if (other.getStreamStatus() == null ^ this.getStreamStatus() == null)
return false;
if (other.getStreamStatus() != null
&& other.getStreamStatus().equals(this.getStreamStatus()) == false)
return false;
if (other.getShards() == null ^ this.getShards() == null)
return false;
if (other.getShards() != null
&& other.getShards().equals(this.getShards()) == false)
return false;
if (other.getHasMoreShards() == null ^ this.getHasMoreShards() == null)
return false;
if (other.getHasMoreShards() != null
&& other.getHasMoreShards().equals(this.getHasMoreShards()) == false)
return false;
if (other.getRetentionPeriodHours() == null
^ this.getRetentionPeriodHours() == null)
return false;
if (other.getRetentionPeriodHours() != null
&& other.getRetentionPeriodHours().equals(
this.getRetentionPeriodHours()) == false)
return false;
if (other.getEnhancedMonitoring() == null
^ this.getEnhancedMonitoring() == null)
return false;
if (other.getEnhancedMonitoring() != null
&& other.getEnhancedMonitoring().equals(
this.getEnhancedMonitoring()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getStreamName() == null) ? 0 : getStreamName().hashCode());
hashCode = prime * hashCode
+ ((getStreamARN() == null) ? 0 : getStreamARN().hashCode());
hashCode = prime
* hashCode
+ ((getStreamStatus() == null) ? 0 : getStreamStatus()
.hashCode());
hashCode = prime * hashCode
+ ((getShards() == null) ? 0 : getShards().hashCode());
hashCode = prime
* hashCode
+ ((getHasMoreShards() == null) ? 0 : getHasMoreShards()
.hashCode());
hashCode = prime
* hashCode
+ ((getRetentionPeriodHours() == null) ? 0
: getRetentionPeriodHours().hashCode());
hashCode = prime
* hashCode
+ ((getEnhancedMonitoring() == null) ? 0
: getEnhancedMonitoring().hashCode());
return hashCode;
}
@Override
public StreamDescription clone() {
try {
return (StreamDescription) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}