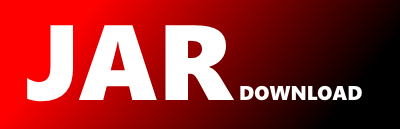
com.amazonaws.services.kinesisanalyticsv2.model.UpdateApplicationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kinesisanalyticsv2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.kinesisanalyticsv2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateApplicationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the application to update.
*
*/
private String applicationName;
/**
*
* The current application version ID. You must provide the CurrentApplicationVersionId
or the
* ConditionalToken
.You can retrieve the application version ID using DescribeApplication. For
* better concurrency support, use the ConditionalToken
parameter instead of
* CurrentApplicationVersionId
.
*
*/
private Long currentApplicationVersionId;
/**
*
* Describes application configuration updates.
*
*/
private ApplicationConfigurationUpdate applicationConfigurationUpdate;
/**
*
* Describes updates to the service execution role.
*
*/
private String serviceExecutionRoleUpdate;
/**
*
* Describes updates to the application's starting parameters.
*
*/
private RunConfigurationUpdate runConfigurationUpdate;
/**
*
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch logging
* options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
*
*/
private java.util.List cloudWatchLoggingOptionUpdates;
/**
*
* A value you use to implement strong concurrency for application updates. You must provide the
* CurrentApplicationVersionId
or the ConditionalToken
. You get the application's current
* ConditionalToken
using DescribeApplication. For better concurrency support, use the
* ConditionalToken
parameter instead of CurrentApplicationVersionId
.
*
*/
private String conditionalToken;
/**
*
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues you must:
*
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
*
*/
private String runtimeEnvironmentUpdate;
/**
*
* The name of the application to update.
*
*
* @param applicationName
* The name of the application to update.
*/
public void setApplicationName(String applicationName) {
this.applicationName = applicationName;
}
/**
*
* The name of the application to update.
*
*
* @return The name of the application to update.
*/
public String getApplicationName() {
return this.applicationName;
}
/**
*
* The name of the application to update.
*
*
* @param applicationName
* The name of the application to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withApplicationName(String applicationName) {
setApplicationName(applicationName);
return this;
}
/**
*
* The current application version ID. You must provide the CurrentApplicationVersionId
or the
* ConditionalToken
.You can retrieve the application version ID using DescribeApplication. For
* better concurrency support, use the ConditionalToken
parameter instead of
* CurrentApplicationVersionId
.
*
*
* @param currentApplicationVersionId
* The current application version ID. You must provide the CurrentApplicationVersionId
or the
* ConditionalToken
.You can retrieve the application version ID using
* DescribeApplication. For better concurrency support, use the ConditionalToken
* parameter instead of CurrentApplicationVersionId
.
*/
public void setCurrentApplicationVersionId(Long currentApplicationVersionId) {
this.currentApplicationVersionId = currentApplicationVersionId;
}
/**
*
* The current application version ID. You must provide the CurrentApplicationVersionId
or the
* ConditionalToken
.You can retrieve the application version ID using DescribeApplication. For
* better concurrency support, use the ConditionalToken
parameter instead of
* CurrentApplicationVersionId
.
*
*
* @return The current application version ID. You must provide the CurrentApplicationVersionId
or the
* ConditionalToken
.You can retrieve the application version ID using
* DescribeApplication. For better concurrency support, use the ConditionalToken
* parameter instead of CurrentApplicationVersionId
.
*/
public Long getCurrentApplicationVersionId() {
return this.currentApplicationVersionId;
}
/**
*
* The current application version ID. You must provide the CurrentApplicationVersionId
or the
* ConditionalToken
.You can retrieve the application version ID using DescribeApplication. For
* better concurrency support, use the ConditionalToken
parameter instead of
* CurrentApplicationVersionId
.
*
*
* @param currentApplicationVersionId
* The current application version ID. You must provide the CurrentApplicationVersionId
or the
* ConditionalToken
.You can retrieve the application version ID using
* DescribeApplication. For better concurrency support, use the ConditionalToken
* parameter instead of CurrentApplicationVersionId
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withCurrentApplicationVersionId(Long currentApplicationVersionId) {
setCurrentApplicationVersionId(currentApplicationVersionId);
return this;
}
/**
*
* Describes application configuration updates.
*
*
* @param applicationConfigurationUpdate
* Describes application configuration updates.
*/
public void setApplicationConfigurationUpdate(ApplicationConfigurationUpdate applicationConfigurationUpdate) {
this.applicationConfigurationUpdate = applicationConfigurationUpdate;
}
/**
*
* Describes application configuration updates.
*
*
* @return Describes application configuration updates.
*/
public ApplicationConfigurationUpdate getApplicationConfigurationUpdate() {
return this.applicationConfigurationUpdate;
}
/**
*
* Describes application configuration updates.
*
*
* @param applicationConfigurationUpdate
* Describes application configuration updates.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withApplicationConfigurationUpdate(ApplicationConfigurationUpdate applicationConfigurationUpdate) {
setApplicationConfigurationUpdate(applicationConfigurationUpdate);
return this;
}
/**
*
* Describes updates to the service execution role.
*
*
* @param serviceExecutionRoleUpdate
* Describes updates to the service execution role.
*/
public void setServiceExecutionRoleUpdate(String serviceExecutionRoleUpdate) {
this.serviceExecutionRoleUpdate = serviceExecutionRoleUpdate;
}
/**
*
* Describes updates to the service execution role.
*
*
* @return Describes updates to the service execution role.
*/
public String getServiceExecutionRoleUpdate() {
return this.serviceExecutionRoleUpdate;
}
/**
*
* Describes updates to the service execution role.
*
*
* @param serviceExecutionRoleUpdate
* Describes updates to the service execution role.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withServiceExecutionRoleUpdate(String serviceExecutionRoleUpdate) {
setServiceExecutionRoleUpdate(serviceExecutionRoleUpdate);
return this;
}
/**
*
* Describes updates to the application's starting parameters.
*
*
* @param runConfigurationUpdate
* Describes updates to the application's starting parameters.
*/
public void setRunConfigurationUpdate(RunConfigurationUpdate runConfigurationUpdate) {
this.runConfigurationUpdate = runConfigurationUpdate;
}
/**
*
* Describes updates to the application's starting parameters.
*
*
* @return Describes updates to the application's starting parameters.
*/
public RunConfigurationUpdate getRunConfigurationUpdate() {
return this.runConfigurationUpdate;
}
/**
*
* Describes updates to the application's starting parameters.
*
*
* @param runConfigurationUpdate
* Describes updates to the application's starting parameters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withRunConfigurationUpdate(RunConfigurationUpdate runConfigurationUpdate) {
setRunConfigurationUpdate(runConfigurationUpdate);
return this;
}
/**
*
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch logging
* options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
*
*
* @return Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch
* logging options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
*/
public java.util.List getCloudWatchLoggingOptionUpdates() {
return cloudWatchLoggingOptionUpdates;
}
/**
*
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch logging
* options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
*
*
* @param cloudWatchLoggingOptionUpdates
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch
* logging options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
*/
public void setCloudWatchLoggingOptionUpdates(java.util.Collection cloudWatchLoggingOptionUpdates) {
if (cloudWatchLoggingOptionUpdates == null) {
this.cloudWatchLoggingOptionUpdates = null;
return;
}
this.cloudWatchLoggingOptionUpdates = new java.util.ArrayList(cloudWatchLoggingOptionUpdates);
}
/**
*
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch logging
* options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCloudWatchLoggingOptionUpdates(java.util.Collection)} or
* {@link #withCloudWatchLoggingOptionUpdates(java.util.Collection)} if you want to override the existing values.
*
*
* @param cloudWatchLoggingOptionUpdates
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch
* logging options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withCloudWatchLoggingOptionUpdates(CloudWatchLoggingOptionUpdate... cloudWatchLoggingOptionUpdates) {
if (this.cloudWatchLoggingOptionUpdates == null) {
setCloudWatchLoggingOptionUpdates(new java.util.ArrayList(cloudWatchLoggingOptionUpdates.length));
}
for (CloudWatchLoggingOptionUpdate ele : cloudWatchLoggingOptionUpdates) {
this.cloudWatchLoggingOptionUpdates.add(ele);
}
return this;
}
/**
*
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch logging
* options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
*
*
* @param cloudWatchLoggingOptionUpdates
* Describes application Amazon CloudWatch logging option updates. You can only update existing CloudWatch
* logging options with this action. To add a new CloudWatch logging option, use
* AddApplicationCloudWatchLoggingOption.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withCloudWatchLoggingOptionUpdates(java.util.Collection cloudWatchLoggingOptionUpdates) {
setCloudWatchLoggingOptionUpdates(cloudWatchLoggingOptionUpdates);
return this;
}
/**
*
* A value you use to implement strong concurrency for application updates. You must provide the
* CurrentApplicationVersionId
or the ConditionalToken
. You get the application's current
* ConditionalToken
using DescribeApplication. For better concurrency support, use the
* ConditionalToken
parameter instead of CurrentApplicationVersionId
.
*
*
* @param conditionalToken
* A value you use to implement strong concurrency for application updates. You must provide the
* CurrentApplicationVersionId
or the ConditionalToken
. You get the application's
* current ConditionalToken
using DescribeApplication. For better concurrency support,
* use the ConditionalToken
parameter instead of CurrentApplicationVersionId
.
*/
public void setConditionalToken(String conditionalToken) {
this.conditionalToken = conditionalToken;
}
/**
*
* A value you use to implement strong concurrency for application updates. You must provide the
* CurrentApplicationVersionId
or the ConditionalToken
. You get the application's current
* ConditionalToken
using DescribeApplication. For better concurrency support, use the
* ConditionalToken
parameter instead of CurrentApplicationVersionId
.
*
*
* @return A value you use to implement strong concurrency for application updates. You must provide the
* CurrentApplicationVersionId
or the ConditionalToken
. You get the application's
* current ConditionalToken
using DescribeApplication. For better concurrency support,
* use the ConditionalToken
parameter instead of CurrentApplicationVersionId
.
*/
public String getConditionalToken() {
return this.conditionalToken;
}
/**
*
* A value you use to implement strong concurrency for application updates. You must provide the
* CurrentApplicationVersionId
or the ConditionalToken
. You get the application's current
* ConditionalToken
using DescribeApplication. For better concurrency support, use the
* ConditionalToken
parameter instead of CurrentApplicationVersionId
.
*
*
* @param conditionalToken
* A value you use to implement strong concurrency for application updates. You must provide the
* CurrentApplicationVersionId
or the ConditionalToken
. You get the application's
* current ConditionalToken
using DescribeApplication. For better concurrency support,
* use the ConditionalToken
parameter instead of CurrentApplicationVersionId
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateApplicationRequest withConditionalToken(String conditionalToken) {
setConditionalToken(conditionalToken);
return this;
}
/**
*
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues you must:
*
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
*
*
* @param runtimeEnvironmentUpdate
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues
* you must:
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
* @see RuntimeEnvironment
*/
public void setRuntimeEnvironmentUpdate(String runtimeEnvironmentUpdate) {
this.runtimeEnvironmentUpdate = runtimeEnvironmentUpdate;
}
/**
*
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues you must:
*
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
*
*
* @return Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues
* you must:
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
* @see RuntimeEnvironment
*/
public String getRuntimeEnvironmentUpdate() {
return this.runtimeEnvironmentUpdate;
}
/**
*
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues you must:
*
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
*
*
* @param runtimeEnvironmentUpdate
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues
* you must:
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuntimeEnvironment
*/
public UpdateApplicationRequest withRuntimeEnvironmentUpdate(String runtimeEnvironmentUpdate) {
setRuntimeEnvironmentUpdate(runtimeEnvironmentUpdate);
return this;
}
/**
*
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues you must:
*
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
*
*
* @param runtimeEnvironmentUpdate
* Updates the Managed Service for Apache Flink runtime environment used to run your code. To avoid issues
* you must:
*
* -
*
* Ensure your new jar and dependencies are compatible with the new runtime selected.
*
*
* -
*
* Ensure your new code's state is compatible with the snapshot from which your application will start
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RuntimeEnvironment
*/
public UpdateApplicationRequest withRuntimeEnvironmentUpdate(RuntimeEnvironment runtimeEnvironmentUpdate) {
this.runtimeEnvironmentUpdate = runtimeEnvironmentUpdate.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getApplicationName() != null)
sb.append("ApplicationName: ").append(getApplicationName()).append(",");
if (getCurrentApplicationVersionId() != null)
sb.append("CurrentApplicationVersionId: ").append(getCurrentApplicationVersionId()).append(",");
if (getApplicationConfigurationUpdate() != null)
sb.append("ApplicationConfigurationUpdate: ").append(getApplicationConfigurationUpdate()).append(",");
if (getServiceExecutionRoleUpdate() != null)
sb.append("ServiceExecutionRoleUpdate: ").append(getServiceExecutionRoleUpdate()).append(",");
if (getRunConfigurationUpdate() != null)
sb.append("RunConfigurationUpdate: ").append(getRunConfigurationUpdate()).append(",");
if (getCloudWatchLoggingOptionUpdates() != null)
sb.append("CloudWatchLoggingOptionUpdates: ").append(getCloudWatchLoggingOptionUpdates()).append(",");
if (getConditionalToken() != null)
sb.append("ConditionalToken: ").append(getConditionalToken()).append(",");
if (getRuntimeEnvironmentUpdate() != null)
sb.append("RuntimeEnvironmentUpdate: ").append(getRuntimeEnvironmentUpdate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateApplicationRequest == false)
return false;
UpdateApplicationRequest other = (UpdateApplicationRequest) obj;
if (other.getApplicationName() == null ^ this.getApplicationName() == null)
return false;
if (other.getApplicationName() != null && other.getApplicationName().equals(this.getApplicationName()) == false)
return false;
if (other.getCurrentApplicationVersionId() == null ^ this.getCurrentApplicationVersionId() == null)
return false;
if (other.getCurrentApplicationVersionId() != null && other.getCurrentApplicationVersionId().equals(this.getCurrentApplicationVersionId()) == false)
return false;
if (other.getApplicationConfigurationUpdate() == null ^ this.getApplicationConfigurationUpdate() == null)
return false;
if (other.getApplicationConfigurationUpdate() != null
&& other.getApplicationConfigurationUpdate().equals(this.getApplicationConfigurationUpdate()) == false)
return false;
if (other.getServiceExecutionRoleUpdate() == null ^ this.getServiceExecutionRoleUpdate() == null)
return false;
if (other.getServiceExecutionRoleUpdate() != null && other.getServiceExecutionRoleUpdate().equals(this.getServiceExecutionRoleUpdate()) == false)
return false;
if (other.getRunConfigurationUpdate() == null ^ this.getRunConfigurationUpdate() == null)
return false;
if (other.getRunConfigurationUpdate() != null && other.getRunConfigurationUpdate().equals(this.getRunConfigurationUpdate()) == false)
return false;
if (other.getCloudWatchLoggingOptionUpdates() == null ^ this.getCloudWatchLoggingOptionUpdates() == null)
return false;
if (other.getCloudWatchLoggingOptionUpdates() != null
&& other.getCloudWatchLoggingOptionUpdates().equals(this.getCloudWatchLoggingOptionUpdates()) == false)
return false;
if (other.getConditionalToken() == null ^ this.getConditionalToken() == null)
return false;
if (other.getConditionalToken() != null && other.getConditionalToken().equals(this.getConditionalToken()) == false)
return false;
if (other.getRuntimeEnvironmentUpdate() == null ^ this.getRuntimeEnvironmentUpdate() == null)
return false;
if (other.getRuntimeEnvironmentUpdate() != null && other.getRuntimeEnvironmentUpdate().equals(this.getRuntimeEnvironmentUpdate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getApplicationName() == null) ? 0 : getApplicationName().hashCode());
hashCode = prime * hashCode + ((getCurrentApplicationVersionId() == null) ? 0 : getCurrentApplicationVersionId().hashCode());
hashCode = prime * hashCode + ((getApplicationConfigurationUpdate() == null) ? 0 : getApplicationConfigurationUpdate().hashCode());
hashCode = prime * hashCode + ((getServiceExecutionRoleUpdate() == null) ? 0 : getServiceExecutionRoleUpdate().hashCode());
hashCode = prime * hashCode + ((getRunConfigurationUpdate() == null) ? 0 : getRunConfigurationUpdate().hashCode());
hashCode = prime * hashCode + ((getCloudWatchLoggingOptionUpdates() == null) ? 0 : getCloudWatchLoggingOptionUpdates().hashCode());
hashCode = prime * hashCode + ((getConditionalToken() == null) ? 0 : getConditionalToken().hashCode());
hashCode = prime * hashCode + ((getRuntimeEnvironmentUpdate() == null) ? 0 : getRuntimeEnvironmentUpdate().hashCode());
return hashCode;
}
@Override
public UpdateApplicationRequest clone() {
return (UpdateApplicationRequest) super.clone();
}
}