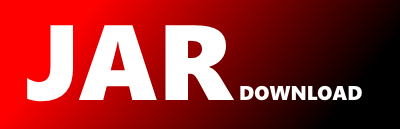
com.amazonaws.services.kms.AWSKMSAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-kms Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.kms;
import com.amazonaws.services.kms.model.*;
import com.amazonaws.annotation.ThreadSafe;
/**
* Interface for accessing KMS asynchronously. Each asynchronous method will
* return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* AWS Key Management Service
*
* AWS Key Management Service (AWS KMS) is an encryption and key management web
* service. This guide describes the AWS KMS operations that you can call
* programmatically. For general information about AWS KMS, see the AWS Key
* Management Service Developer Guide.
*
*
*
* AWS provides SDKs that consist of libraries and sample code for various
* programming languages and platforms (Java, Ruby, .Net, iOS, Android, etc.).
* The SDKs provide a convenient way to create programmatic access to AWS KMS
* and other AWS services. For example, the SDKs take care of tasks such as
* signing requests (see below), managing errors, and retrying requests
* automatically. For more information about the AWS SDKs, including how to
* download and install them, see Tools
* for Amazon Web Services.
*
*
*
* We recommend that you use the AWS SDKs to make programmatic API calls to AWS
* KMS.
*
*
* Clients must support TLS (Transport Layer Security) 1.0. We recommend TLS
* 1.2. Clients must also support cipher suites with Perfect Forward Secrecy
* (PFS) such as Ephemeral Diffie-Hellman (DHE) or Elliptic Curve Ephemeral
* Diffie-Hellman (ECDHE). Most modern systems such as Java 7 and later support
* these modes.
*
*
* Signing Requests
*
*
* Requests must be signed by using an access key ID and a secret access key. We
* strongly recommend that you do not use your AWS account (root) access
* key ID and secret key for everyday work with AWS KMS. Instead, use the access
* key ID and secret access key for an IAM user, or you can use the AWS Security
* Token Service to generate temporary security credentials that you can use to
* sign requests.
*
*
* All AWS KMS operations require Signature Version 4.
*
*
* Logging API Requests
*
*
* AWS KMS supports AWS CloudTrail, a service that logs AWS API calls and
* related events for your AWS account and delivers them to an Amazon S3 bucket
* that you specify. By using the information collected by CloudTrail, you can
* determine what requests were made to AWS KMS, who made the request, when it
* was made, and so on. To learn more about CloudTrail, including how to turn it
* on and find your log files, see the AWS
* CloudTrail User Guide.
*
*
* Additional Resources
*
*
* For more information about credentials and request signing, see the
* following:
*
*
* -
*
* AWS Security Credentials - This topic provides general information about
* the types of credentials used for accessing AWS.
*
*
* -
*
* Temporary Security Credentials - This section of the IAM User
* Guide describes how to create and use temporary security credentials.
*
*
* -
*
* Signature Version 4 Signing Process - This set of topics walks you
* through the process of signing a request using an access key ID and a secret
* access key.
*
*
*
*
* Commonly Used APIs
*
*
* Of the APIs discussed in this guide, the following will prove the most useful
* for most applications. You will likely perform actions other than these, such
* as creating keys and assigning policies, by using the console.
*
*
* -
*
* Encrypt
*
*
* -
*
* Decrypt
*
*
* -
*
* GenerateDataKey
*
*
* -
*
*
*
*/
@ThreadSafe
public class AWSKMSAsyncClient extends AWSKMSClient implements AWSKMSAsync {
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
private final java.util.concurrent.ExecutorService executorService;
/**
* Constructs a new asynchronous client to invoke service methods on KMS. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSKMSAsyncClient() {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to KMS (ex: proxy settings, retry counts, etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSKMSAsyncClient(
com.amazonaws.ClientConfiguration clientConfiguration) {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain(),
clientConfiguration, java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS
* using the specified AWS account credentials.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSKMSAsyncClient(com.amazonaws.auth.AWSCredentials awsCredentials) {
this(awsCredentials, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS
* using the specified AWS account credentials and executor service. Default
* client settings will be used.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSKMSAsyncClient(com.amazonaws.auth.AWSCredentials awsCredentials,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentials, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS
* using the specified AWS account credentials, executor service, and client
* configuration options.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSKMSAsyncClient(com.amazonaws.auth.AWSCredentials awsCredentials,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS
* using the specified AWS account credentials provider. Default client
* settings will be used.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSKMSAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS
* using the provided AWS account credentials provider and client
* configuration options.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSKMSAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration,
java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS
* using the specified AWS account credentials provider and executor
* service. Default client settings will be used.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSKMSAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentialsProvider, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on KMS
* using the specified AWS account credentials provider, executor service,
* and client configuration options.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSKMSAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this client to execute async
* requests.
*
* @return The executor service used by this client to execute async
* requests.
*/
public java.util.concurrent.ExecutorService getExecutorService() {
return executorService;
}
@Override
public java.util.concurrent.Future cancelKeyDeletionAsync(
CancelKeyDeletionRequest request) {
return cancelKeyDeletionAsync(request, null);
}
@Override
public java.util.concurrent.Future cancelKeyDeletionAsync(
final CancelKeyDeletionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CancelKeyDeletionResult call() throws Exception {
CancelKeyDeletionResult result;
try {
result = cancelKeyDeletion(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createAliasAsync(
CreateAliasRequest request) {
return createAliasAsync(request, null);
}
@Override
public java.util.concurrent.Future createAliasAsync(
final CreateAliasRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateAliasResult call() throws Exception {
CreateAliasResult result;
try {
result = createAlias(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createGrantAsync(
CreateGrantRequest request) {
return createGrantAsync(request, null);
}
@Override
public java.util.concurrent.Future createGrantAsync(
final CreateGrantRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateGrantResult call() throws Exception {
CreateGrantResult result;
try {
result = createGrant(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createKeyAsync(
CreateKeyRequest request) {
return createKeyAsync(request, null);
}
@Override
public java.util.concurrent.Future createKeyAsync(
final CreateKeyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateKeyResult call() throws Exception {
CreateKeyResult result;
try {
result = createKey(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the CreateKey operation.
*
* @see #createKeyAsync(CreateKeyRequest)
*/
@Override
public java.util.concurrent.Future createKeyAsync() {
return createKeyAsync(new CreateKeyRequest());
}
/**
* Simplified method form for invoking the CreateKey operation with an
* AsyncHandler.
*
* @see #createKeyAsync(CreateKeyRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future createKeyAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return createKeyAsync(new CreateKeyRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future decryptAsync(
DecryptRequest request) {
return decryptAsync(request, null);
}
@Override
public java.util.concurrent.Future decryptAsync(
final DecryptRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DecryptResult call() throws Exception {
DecryptResult result;
try {
result = decrypt(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteAliasAsync(
DeleteAliasRequest request) {
return deleteAliasAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteAliasAsync(
final DeleteAliasRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeleteAliasResult call() throws Exception {
DeleteAliasResult result;
try {
result = deleteAlias(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeKeyAsync(
DescribeKeyRequest request) {
return describeKeyAsync(request, null);
}
@Override
public java.util.concurrent.Future describeKeyAsync(
final DescribeKeyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DescribeKeyResult call() throws Exception {
DescribeKeyResult result;
try {
result = describeKey(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future disableKeyAsync(
DisableKeyRequest request) {
return disableKeyAsync(request, null);
}
@Override
public java.util.concurrent.Future disableKeyAsync(
final DisableKeyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DisableKeyResult call() throws Exception {
DisableKeyResult result;
try {
result = disableKey(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future disableKeyRotationAsync(
DisableKeyRotationRequest request) {
return disableKeyRotationAsync(request, null);
}
@Override
public java.util.concurrent.Future disableKeyRotationAsync(
final DisableKeyRotationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DisableKeyRotationResult call() throws Exception {
DisableKeyRotationResult result;
try {
result = disableKeyRotation(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future enableKeyAsync(
EnableKeyRequest request) {
return enableKeyAsync(request, null);
}
@Override
public java.util.concurrent.Future enableKeyAsync(
final EnableKeyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public EnableKeyResult call() throws Exception {
EnableKeyResult result;
try {
result = enableKey(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future enableKeyRotationAsync(
EnableKeyRotationRequest request) {
return enableKeyRotationAsync(request, null);
}
@Override
public java.util.concurrent.Future enableKeyRotationAsync(
final EnableKeyRotationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public EnableKeyRotationResult call() throws Exception {
EnableKeyRotationResult result;
try {
result = enableKeyRotation(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future encryptAsync(
EncryptRequest request) {
return encryptAsync(request, null);
}
@Override
public java.util.concurrent.Future encryptAsync(
final EncryptRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public EncryptResult call() throws Exception {
EncryptResult result;
try {
result = encrypt(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future generateDataKeyAsync(
GenerateDataKeyRequest request) {
return generateDataKeyAsync(request, null);
}
@Override
public java.util.concurrent.Future generateDataKeyAsync(
final GenerateDataKeyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GenerateDataKeyResult call() throws Exception {
GenerateDataKeyResult result;
try {
result = generateDataKey(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future generateDataKeyWithoutPlaintextAsync(
GenerateDataKeyWithoutPlaintextRequest request) {
return generateDataKeyWithoutPlaintextAsync(request, null);
}
@Override
public java.util.concurrent.Future generateDataKeyWithoutPlaintextAsync(
final GenerateDataKeyWithoutPlaintextRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GenerateDataKeyWithoutPlaintextResult call()
throws Exception {
GenerateDataKeyWithoutPlaintextResult result;
try {
result = generateDataKeyWithoutPlaintext(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future generateRandomAsync(
GenerateRandomRequest request) {
return generateRandomAsync(request, null);
}
@Override
public java.util.concurrent.Future generateRandomAsync(
final GenerateRandomRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GenerateRandomResult call() throws Exception {
GenerateRandomResult result;
try {
result = generateRandom(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the GenerateRandom operation.
*
* @see #generateRandomAsync(GenerateRandomRequest)
*/
@Override
public java.util.concurrent.Future generateRandomAsync() {
return generateRandomAsync(new GenerateRandomRequest());
}
/**
* Simplified method form for invoking the GenerateRandom operation with an
* AsyncHandler.
*
* @see #generateRandomAsync(GenerateRandomRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future generateRandomAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return generateRandomAsync(new GenerateRandomRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future getKeyPolicyAsync(
GetKeyPolicyRequest request) {
return getKeyPolicyAsync(request, null);
}
@Override
public java.util.concurrent.Future getKeyPolicyAsync(
final GetKeyPolicyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetKeyPolicyResult call() throws Exception {
GetKeyPolicyResult result;
try {
result = getKeyPolicy(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getKeyRotationStatusAsync(
GetKeyRotationStatusRequest request) {
return getKeyRotationStatusAsync(request, null);
}
@Override
public java.util.concurrent.Future getKeyRotationStatusAsync(
final GetKeyRotationStatusRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetKeyRotationStatusResult call() throws Exception {
GetKeyRotationStatusResult result;
try {
result = getKeyRotationStatus(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listAliasesAsync(
ListAliasesRequest request) {
return listAliasesAsync(request, null);
}
@Override
public java.util.concurrent.Future listAliasesAsync(
final ListAliasesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListAliasesResult call() throws Exception {
ListAliasesResult result;
try {
result = listAliases(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListAliases operation.
*
* @see #listAliasesAsync(ListAliasesRequest)
*/
@Override
public java.util.concurrent.Future listAliasesAsync() {
return listAliasesAsync(new ListAliasesRequest());
}
/**
* Simplified method form for invoking the ListAliases operation with an
* AsyncHandler.
*
* @see #listAliasesAsync(ListAliasesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listAliasesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listAliasesAsync(new ListAliasesRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future listGrantsAsync(
ListGrantsRequest request) {
return listGrantsAsync(request, null);
}
@Override
public java.util.concurrent.Future listGrantsAsync(
final ListGrantsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListGrantsResult call() throws Exception {
ListGrantsResult result;
try {
result = listGrants(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listKeyPoliciesAsync(
ListKeyPoliciesRequest request) {
return listKeyPoliciesAsync(request, null);
}
@Override
public java.util.concurrent.Future listKeyPoliciesAsync(
final ListKeyPoliciesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListKeyPoliciesResult call() throws Exception {
ListKeyPoliciesResult result;
try {
result = listKeyPolicies(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listKeysAsync(
ListKeysRequest request) {
return listKeysAsync(request, null);
}
@Override
public java.util.concurrent.Future listKeysAsync(
final ListKeysRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListKeysResult call() throws Exception {
ListKeysResult result;
try {
result = listKeys(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListKeys operation.
*
* @see #listKeysAsync(ListKeysRequest)
*/
@Override
public java.util.concurrent.Future listKeysAsync() {
return listKeysAsync(new ListKeysRequest());
}
/**
* Simplified method form for invoking the ListKeys operation with an
* AsyncHandler.
*
* @see #listKeysAsync(ListKeysRequest, com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listKeysAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listKeysAsync(new ListKeysRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future listRetirableGrantsAsync(
ListRetirableGrantsRequest request) {
return listRetirableGrantsAsync(request, null);
}
@Override
public java.util.concurrent.Future listRetirableGrantsAsync(
final ListRetirableGrantsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListRetirableGrantsResult call() throws Exception {
ListRetirableGrantsResult result;
try {
result = listRetirableGrants(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future putKeyPolicyAsync(
PutKeyPolicyRequest request) {
return putKeyPolicyAsync(request, null);
}
@Override
public java.util.concurrent.Future putKeyPolicyAsync(
final PutKeyPolicyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public PutKeyPolicyResult call() throws Exception {
PutKeyPolicyResult result;
try {
result = putKeyPolicy(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future reEncryptAsync(
ReEncryptRequest request) {
return reEncryptAsync(request, null);
}
@Override
public java.util.concurrent.Future reEncryptAsync(
final ReEncryptRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ReEncryptResult call() throws Exception {
ReEncryptResult result;
try {
result = reEncrypt(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future retireGrantAsync(
RetireGrantRequest request) {
return retireGrantAsync(request, null);
}
@Override
public java.util.concurrent.Future retireGrantAsync(
final RetireGrantRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public RetireGrantResult call() throws Exception {
RetireGrantResult result;
try {
result = retireGrant(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the RetireGrant operation.
*
* @see #retireGrantAsync(RetireGrantRequest)
*/
@Override
public java.util.concurrent.Future retireGrantAsync() {
return retireGrantAsync(new RetireGrantRequest());
}
/**
* Simplified method form for invoking the RetireGrant operation with an
* AsyncHandler.
*
* @see #retireGrantAsync(RetireGrantRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future retireGrantAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return retireGrantAsync(new RetireGrantRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future revokeGrantAsync(
RevokeGrantRequest request) {
return revokeGrantAsync(request, null);
}
@Override
public java.util.concurrent.Future revokeGrantAsync(
final RevokeGrantRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public RevokeGrantResult call() throws Exception {
RevokeGrantResult result;
try {
result = revokeGrant(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future scheduleKeyDeletionAsync(
ScheduleKeyDeletionRequest request) {
return scheduleKeyDeletionAsync(request, null);
}
@Override
public java.util.concurrent.Future scheduleKeyDeletionAsync(
final ScheduleKeyDeletionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ScheduleKeyDeletionResult call() throws Exception {
ScheduleKeyDeletionResult result;
try {
result = scheduleKeyDeletion(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateAliasAsync(
UpdateAliasRequest request) {
return updateAliasAsync(request, null);
}
@Override
public java.util.concurrent.Future updateAliasAsync(
final UpdateAliasRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateAliasResult call() throws Exception {
UpdateAliasResult result;
try {
result = updateAlias(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateKeyDescriptionAsync(
UpdateKeyDescriptionRequest request) {
return updateKeyDescriptionAsync(request, null);
}
@Override
public java.util.concurrent.Future updateKeyDescriptionAsync(
final UpdateKeyDescriptionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateKeyDescriptionResult call() throws Exception {
UpdateKeyDescriptionResult result;
try {
result = updateKeyDescription(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call {@code getExecutorService().shutdown()} followed by
* {@code getExecutorService().awaitTermination()} prior to calling this
* method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
}