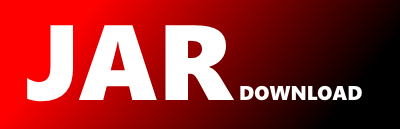
com.amazonaws.services.lambda.AWSLambdaAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lambda Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.lambda;
import com.amazonaws.services.lambda.model.*;
import com.amazonaws.annotation.ThreadSafe;
/**
* Interface for accessing AWS Lambda asynchronously. Each asynchronous method
* will return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* AWS Lambda
*
* Overview
*
*
* This is the AWS Lambda API Reference. The AWS Lambda Developer Guide
* provides additional information. For the service overview, go to What is AWS
* Lambda, and for information about how the service works, go to AWS Lambda: How it Works in the AWS Lambda Developer Guide.
*
*/
@ThreadSafe
public class AWSLambdaAsyncClient extends AWSLambdaClient implements
AWSLambdaAsync {
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
private final java.util.concurrent.ExecutorService executorService;
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda. A credentials provider chain will be used that searches for
* credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSLambdaAsyncClient() {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda. A credentials provider chain will be used that searches for
* credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Lambda (ex: proxy settings, retry counts, etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSLambdaAsyncClient(
com.amazonaws.ClientConfiguration clientConfiguration) {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain(),
clientConfiguration, java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda using the specified AWS account credentials.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSLambdaAsyncClient(com.amazonaws.auth.AWSCredentials awsCredentials) {
this(awsCredentials, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda using the specified AWS account credentials and executor service.
* Default client settings will be used.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSLambdaAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentials, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda using the specified AWS account credentials, executor service, and
* client configuration options.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSLambdaAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda using the specified AWS account credentials provider. Default
* client settings will be used.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSLambdaAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda using the provided AWS account credentials provider and client
* configuration options.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AWSLambdaAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration,
java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda using the specified AWS account credentials provider and executor
* service. Default client settings will be used.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSLambdaAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentialsProvider, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* Lambda using the specified AWS account credentials provider, executor
* service, and client configuration options.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AWSLambdaAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this client to execute async
* requests.
*
* @return The executor service used by this client to execute async
* requests.
*/
public java.util.concurrent.ExecutorService getExecutorService() {
return executorService;
}
@Override
public java.util.concurrent.Future addPermissionAsync(
AddPermissionRequest request) {
return addPermissionAsync(request, null);
}
@Override
public java.util.concurrent.Future addPermissionAsync(
final AddPermissionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public AddPermissionResult call() throws Exception {
AddPermissionResult result;
try {
result = addPermission(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createAliasAsync(
CreateAliasRequest request) {
return createAliasAsync(request, null);
}
@Override
public java.util.concurrent.Future createAliasAsync(
final CreateAliasRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateAliasResult call() throws Exception {
CreateAliasResult result;
try {
result = createAlias(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createEventSourceMappingAsync(
CreateEventSourceMappingRequest request) {
return createEventSourceMappingAsync(request, null);
}
@Override
public java.util.concurrent.Future createEventSourceMappingAsync(
final CreateEventSourceMappingRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateEventSourceMappingResult call()
throws Exception {
CreateEventSourceMappingResult result;
try {
result = createEventSourceMapping(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createFunctionAsync(
CreateFunctionRequest request) {
return createFunctionAsync(request, null);
}
@Override
public java.util.concurrent.Future createFunctionAsync(
final CreateFunctionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateFunctionResult call() throws Exception {
CreateFunctionResult result;
try {
result = createFunction(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteAliasAsync(
DeleteAliasRequest request) {
return deleteAliasAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteAliasAsync(
final DeleteAliasRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeleteAliasResult call() throws Exception {
DeleteAliasResult result;
try {
result = deleteAlias(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteEventSourceMappingAsync(
DeleteEventSourceMappingRequest request) {
return deleteEventSourceMappingAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteEventSourceMappingAsync(
final DeleteEventSourceMappingRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeleteEventSourceMappingResult call()
throws Exception {
DeleteEventSourceMappingResult result;
try {
result = deleteEventSourceMapping(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteFunctionAsync(
DeleteFunctionRequest request) {
return deleteFunctionAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteFunctionAsync(
final DeleteFunctionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DeleteFunctionResult call() throws Exception {
DeleteFunctionResult result;
try {
result = deleteFunction(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getAliasAsync(
GetAliasRequest request) {
return getAliasAsync(request, null);
}
@Override
public java.util.concurrent.Future getAliasAsync(
final GetAliasRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetAliasResult call() throws Exception {
GetAliasResult result;
try {
result = getAlias(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getEventSourceMappingAsync(
GetEventSourceMappingRequest request) {
return getEventSourceMappingAsync(request, null);
}
@Override
public java.util.concurrent.Future getEventSourceMappingAsync(
final GetEventSourceMappingRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetEventSourceMappingResult call() throws Exception {
GetEventSourceMappingResult result;
try {
result = getEventSourceMapping(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getFunctionAsync(
GetFunctionRequest request) {
return getFunctionAsync(request, null);
}
@Override
public java.util.concurrent.Future getFunctionAsync(
final GetFunctionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetFunctionResult call() throws Exception {
GetFunctionResult result;
try {
result = getFunction(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getFunctionConfigurationAsync(
GetFunctionConfigurationRequest request) {
return getFunctionConfigurationAsync(request, null);
}
@Override
public java.util.concurrent.Future getFunctionConfigurationAsync(
final GetFunctionConfigurationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetFunctionConfigurationResult call()
throws Exception {
GetFunctionConfigurationResult result;
try {
result = getFunctionConfiguration(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getPolicyAsync(
GetPolicyRequest request) {
return getPolicyAsync(request, null);
}
@Override
public java.util.concurrent.Future getPolicyAsync(
final GetPolicyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetPolicyResult call() throws Exception {
GetPolicyResult result;
try {
result = getPolicy(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future invokeAsync(
InvokeRequest request) {
return invokeAsync(request, null);
}
@Override
public java.util.concurrent.Future invokeAsync(
final InvokeRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public InvokeResult call() throws Exception {
InvokeResult result;
try {
result = invoke(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
@Deprecated
public java.util.concurrent.Future invokeAsyncAsync(
InvokeAsyncRequest request) {
return invokeAsyncAsync(request, null);
}
@Override
@Deprecated
public java.util.concurrent.Future invokeAsyncAsync(
final InvokeAsyncRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public InvokeAsyncResult call() throws Exception {
InvokeAsyncResult result;
try {
result = invokeAsync(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listAliasesAsync(
ListAliasesRequest request) {
return listAliasesAsync(request, null);
}
@Override
public java.util.concurrent.Future listAliasesAsync(
final ListAliasesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListAliasesResult call() throws Exception {
ListAliasesResult result;
try {
result = listAliases(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listEventSourceMappingsAsync(
ListEventSourceMappingsRequest request) {
return listEventSourceMappingsAsync(request, null);
}
@Override
public java.util.concurrent.Future listEventSourceMappingsAsync(
final ListEventSourceMappingsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListEventSourceMappingsResult call()
throws Exception {
ListEventSourceMappingsResult result;
try {
result = listEventSourceMappings(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListEventSourceMappings
* operation.
*
* @see #listEventSourceMappingsAsync(ListEventSourceMappingsRequest)
*/
@Override
public java.util.concurrent.Future listEventSourceMappingsAsync() {
return listEventSourceMappingsAsync(new ListEventSourceMappingsRequest());
}
/**
* Simplified method form for invoking the ListEventSourceMappings operation
* with an AsyncHandler.
*
* @see #listEventSourceMappingsAsync(ListEventSourceMappingsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listEventSourceMappingsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listEventSourceMappingsAsync(
new ListEventSourceMappingsRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future listFunctionsAsync(
ListFunctionsRequest request) {
return listFunctionsAsync(request, null);
}
@Override
public java.util.concurrent.Future listFunctionsAsync(
final ListFunctionsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListFunctionsResult call() throws Exception {
ListFunctionsResult result;
try {
result = listFunctions(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListFunctions operation.
*
* @see #listFunctionsAsync(ListFunctionsRequest)
*/
@Override
public java.util.concurrent.Future listFunctionsAsync() {
return listFunctionsAsync(new ListFunctionsRequest());
}
/**
* Simplified method form for invoking the ListFunctions operation with an
* AsyncHandler.
*
* @see #listFunctionsAsync(ListFunctionsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listFunctionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listFunctionsAsync(new ListFunctionsRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future listVersionsByFunctionAsync(
ListVersionsByFunctionRequest request) {
return listVersionsByFunctionAsync(request, null);
}
@Override
public java.util.concurrent.Future listVersionsByFunctionAsync(
final ListVersionsByFunctionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListVersionsByFunctionResult call() throws Exception {
ListVersionsByFunctionResult result;
try {
result = listVersionsByFunction(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future publishVersionAsync(
PublishVersionRequest request) {
return publishVersionAsync(request, null);
}
@Override
public java.util.concurrent.Future publishVersionAsync(
final PublishVersionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public PublishVersionResult call() throws Exception {
PublishVersionResult result;
try {
result = publishVersion(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future removePermissionAsync(
RemovePermissionRequest request) {
return removePermissionAsync(request, null);
}
@Override
public java.util.concurrent.Future removePermissionAsync(
final RemovePermissionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public RemovePermissionResult call() throws Exception {
RemovePermissionResult result;
try {
result = removePermission(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateAliasAsync(
UpdateAliasRequest request) {
return updateAliasAsync(request, null);
}
@Override
public java.util.concurrent.Future updateAliasAsync(
final UpdateAliasRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateAliasResult call() throws Exception {
UpdateAliasResult result;
try {
result = updateAlias(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateEventSourceMappingAsync(
UpdateEventSourceMappingRequest request) {
return updateEventSourceMappingAsync(request, null);
}
@Override
public java.util.concurrent.Future updateEventSourceMappingAsync(
final UpdateEventSourceMappingRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateEventSourceMappingResult call()
throws Exception {
UpdateEventSourceMappingResult result;
try {
result = updateEventSourceMapping(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateFunctionCodeAsync(
UpdateFunctionCodeRequest request) {
return updateFunctionCodeAsync(request, null);
}
@Override
public java.util.concurrent.Future updateFunctionCodeAsync(
final UpdateFunctionCodeRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateFunctionCodeResult call() throws Exception {
UpdateFunctionCodeResult result;
try {
result = updateFunctionCode(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateFunctionConfigurationAsync(
UpdateFunctionConfigurationRequest request) {
return updateFunctionConfigurationAsync(request, null);
}
@Override
public java.util.concurrent.Future updateFunctionConfigurationAsync(
final UpdateFunctionConfigurationRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateFunctionConfigurationResult call()
throws Exception {
UpdateFunctionConfigurationResult result;
try {
result = updateFunctionConfiguration(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call {@code getExecutorService().shutdown()} followed by
* {@code getExecutorService().awaitTermination()} prior to calling this
* method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
}