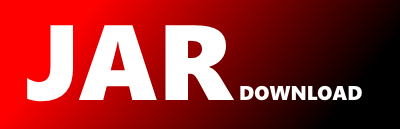
com.amazonaws.services.lambda.AWSLambdaClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lambda Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.lambda;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.services.lambda.model.*;
import com.amazonaws.services.lambda.model.transform.*;
/**
* Client for accessing AWS Lambda. All service calls made using this client are
* blocking, and will not return until the service call completes.
*
* AWS Lambda
*
* Overview
*
*
* This is the AWS Lambda API Reference. The AWS Lambda Developer Guide
* provides additional information. For the service overview, go to What is AWS
* Lambda, and for information about how the service works, go to AWS Lambda: How it Works in the AWS Lambda Developer Guide.
*
*/
@ThreadSafe
public class AWSLambdaClient extends AmazonWebServiceClient implements
AWSLambda {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSLambda.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "lambda";
/** The region metadata service name for computing region endpoints. */
private static final String DEFAULT_ENDPOINT_PREFIX = "lambda";
/**
* Client configuration factory providing ClientConfigurations tailored to
* this client
*/
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final SdkJsonProtocolFactory protocolFactory = new SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("ResourceConflictException")
.withModeledClass(
com.amazonaws.services.lambda.model.ResourceConflictException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"CodeStorageExceededException")
.withModeledClass(
com.amazonaws.services.lambda.model.CodeStorageExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("ServiceException")
.withModeledClass(
com.amazonaws.services.lambda.model.ServiceException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("RequestTooLargeException")
.withModeledClass(
com.amazonaws.services.lambda.model.RequestTooLargeException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"InvalidSecurityGroupIDException")
.withModeledClass(
com.amazonaws.services.lambda.model.InvalidSecurityGroupIDException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"InvalidParameterValueException")
.withModeledClass(
com.amazonaws.services.lambda.model.InvalidParameterValueException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("EC2AccessDeniedException")
.withModeledClass(
com.amazonaws.services.lambda.model.EC2AccessDeniedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"PolicyLengthExceededException")
.withModeledClass(
com.amazonaws.services.lambda.model.PolicyLengthExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("InvalidSubnetIDException")
.withModeledClass(
com.amazonaws.services.lambda.model.InvalidSubnetIDException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("ENILimitReachedException")
.withModeledClass(
com.amazonaws.services.lambda.model.ENILimitReachedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("EC2ThrottledException")
.withModeledClass(
com.amazonaws.services.lambda.model.EC2ThrottledException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("EC2UnexpectedException")
.withModeledClass(
com.amazonaws.services.lambda.model.EC2UnexpectedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"SubnetIPAddressLimitReachedException")
.withModeledClass(
com.amazonaws.services.lambda.model.SubnetIPAddressLimitReachedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"UnsupportedMediaTypeException")
.withModeledClass(
com.amazonaws.services.lambda.model.UnsupportedMediaTypeException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"InvalidRequestContentException")
.withModeledClass(
com.amazonaws.services.lambda.model.InvalidRequestContentException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("ResourceNotFoundException")
.withModeledClass(
com.amazonaws.services.lambda.model.ResourceNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("TooManyRequestsException")
.withModeledClass(
com.amazonaws.services.lambda.model.TooManyRequestsException.class)));
/**
* Constructs a new client to invoke service methods on AWS Lambda. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSLambdaClient() {
this(new DefaultAWSCredentialsProviderChain(), configFactory
.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Lambda. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Lambda (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSLambdaClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on AWS Lambda using the
* specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
*/
public AWSLambdaClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Lambda using the
* specified AWS account credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Lambda (ex: proxy settings, retry counts, etc.).
*/
public AWSLambdaClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(
awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on AWS Lambda using the
* specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
*/
public AWSLambdaClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Lambda using the
* specified AWS account credentials provider and client configuration
* options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Lambda (ex: proxy settings, retry counts, etc.).
*/
public AWSLambdaClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on AWS Lambda using the
* specified AWS account credentials provider, client configuration options,
* and request metric collector.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Lambda (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
*/
public AWSLambdaClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(DEFAULT_ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("lambda.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s
.addAll(chainFactory
.newRequestHandlerChain("/com/amazonaws/services/lambda/request.handlers"));
requestHandler2s
.addAll(chainFactory
.newRequestHandler2Chain("/com/amazonaws/services/lambda/request.handler2s"));
}
/**
*
* Adds a permission to the resource policy associated with the specified
* AWS Lambda function. You use resource policies to grant permissions to
* event sources that use push model. In a push model, event
* sources (such as Amazon S3 and custom applications) invoke your Lambda
* function. Each permission you add to the resource policy allows an event
* source, permission to invoke the Lambda function.
*
*
* For information about the push model, see AWS Lambda: How it Works.
*
*
* If you are using versioning, the permissions you add are specific to the
* Lambda function version or alias you specify in the
* AddPermission
request via the Qualifier
* parameter. For more information about versioning, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:AddPermission
action.
*
*
* @param addPermissionRequest
* @return Result of the AddPermission operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws ResourceConflictException
* The resource already exists.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws PolicyLengthExceededException
* Lambda function access policy is limited to 20 KB.
* @throws TooManyRequestsException
* @sample AWSLambda.AddPermission
*/
@Override
public AddPermissionResult addPermission(
AddPermissionRequest addPermissionRequest) {
ExecutionContext executionContext = createExecutionContext(addPermissionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddPermissionRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(addPermissionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new AddPermissionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an alias that points to the specified Lambda function version.
* For more information, see Introduction to AWS Lambda Aliases.
*
* Alias names are unique for a given function.
*
* This requires permission for the lambda:CreateAlias action.
*
*
* @param createAliasRequest
* @return Result of the CreateAlias operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws ResourceConflictException
* The resource already exists.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.CreateAlias
*/
@Override
public CreateAliasResult createAlias(CreateAliasRequest createAliasRequest) {
ExecutionContext executionContext = createExecutionContext(createAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAliasRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(createAliasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateAliasResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Identifies a stream as an event source for a Lambda function. It can be
* either an Amazon Kinesis stream or an Amazon DynamoDB stream. AWS Lambda
* invokes the specified function when records are posted to the stream.
*
*
* This association between a stream source and a Lambda function is called
* the event source mapping.
*
* This event source mapping is relevant only in the AWS Lambda
* pull model, where AWS Lambda invokes the function. For more information,
* go to AWS Lambda: How it Works in the AWS Lambda Developer
* Guide.
*
* You provide mapping information (for example, which stream to read from
* and which Lambda function to invoke) in the request body.
*
*
* Each event source, such as an Amazon Kinesis or a DynamoDB stream, can be
* associated with multiple AWS Lambda function. A given Lambda function can
* be associated with multiple AWS event sources.
*
*
*
* If you are using versioning, you can specify a specific function version
* or an alias via the function name parameter. For more information about
* versioning, see AWS
* Lambda Function Versioning and Aliases.
*
*
*
* This operation requires permission for the
* lambda:CreateEventSourceMapping
action.
*
*
* @param createEventSourceMappingRequest
* @return Result of the CreateEventSourceMapping operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws ResourceConflictException
* The resource already exists.
* @throws TooManyRequestsException
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @sample AWSLambda.CreateEventSourceMapping
*/
@Override
public CreateEventSourceMappingResult createEventSourceMapping(
CreateEventSourceMappingRequest createEventSourceMappingRequest) {
ExecutionContext executionContext = createExecutionContext(createEventSourceMappingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEventSourceMappingRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(createEventSourceMappingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateEventSourceMappingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Lambda function. The function metadata is created from the
* request parameters, and the code for the function is provided by a .zip
* file in the request body. If the function name already exists, the
* operation will fail. Note that the function name is case-sensitive.
*
*
* If you are using versioning, you can also publish a version of the Lambda
* function you are creating using the Publish
parameter. For
* more information about versioning, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:CreateFunction
action.
*
*
* @param createFunctionRequest
* @return Result of the CreateFunction operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws ResourceConflictException
* The resource already exists.
* @throws TooManyRequestsException
* @throws CodeStorageExceededException
* You have exceeded your maximum total code size per account.
* Limits
* @sample AWSLambda.CreateFunction
*/
@Override
public CreateFunctionResult createFunction(
CreateFunctionRequest createFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(createFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFunctionRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(createFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Lambda function alias. For more information, see
* Introduction to AWS Lambda Aliases.
*
*
* This requires permission for the lambda:DeleteAlias action.
*
*
* @param deleteAliasRequest
* @return Result of the DeleteAlias operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.DeleteAlias
*/
@Override
public DeleteAliasResult deleteAlias(DeleteAliasRequest deleteAliasRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAliasRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(deleteAliasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteAliasResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes an event source mapping. This means AWS Lambda will no longer
* invoke the function for events in the associated source.
*
*
* This operation requires permission for the
* lambda:DeleteEventSourceMapping
action.
*
*
* @param deleteEventSourceMappingRequest
* @return Result of the DeleteEventSourceMapping operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.DeleteEventSourceMapping
*/
@Override
public DeleteEventSourceMappingResult deleteEventSourceMapping(
DeleteEventSourceMappingRequest deleteEventSourceMappingRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventSourceMappingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEventSourceMappingRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(deleteEventSourceMappingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteEventSourceMappingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Lambda function code and configuration.
*
*
* If you are using the versioning feature and you don't specify a function
* version in your DeleteFunction
request, AWS Lambda will
* delete the function, including all its versions, and any aliases pointing
* to the function versions. To delete a specific function version, you must
* provide the function version via the Qualifier
parameter.
* For information about function versioning, see AWS
* Lambda Function Versioning and Aliases.
*
*
* When you delete a function the associated resource policy is also
* deleted. You will need to delete the event source mappings explicitly.
*
*
* This operation requires permission for the
* lambda:DeleteFunction
action.
*
*
* @param deleteFunctionRequest
* @return Result of the DeleteFunction operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws TooManyRequestsException
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws ResourceConflictException
* The resource already exists.
* @sample AWSLambda.DeleteFunction
*/
@Override
public DeleteFunctionResult deleteFunction(
DeleteFunctionRequest deleteFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFunctionRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(deleteFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the specified alias information such as the alias ARN,
* description, and function version it is pointing to. For more
* information, see Introduction to AWS Lambda Aliases.
*
*
* This requires permission for the lambda:GetAlias
action.
*
*
* @param getAliasRequest
* @return Result of the GetAlias operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.GetAlias
*/
@Override
public GetAliasResult getAlias(GetAliasRequest getAliasRequest) {
ExecutionContext executionContext = createExecutionContext(getAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAliasRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(getAliasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetAliasResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns configuration information for the specified event source mapping
* (see CreateEventSourceMapping).
*
*
* This operation requires permission for the
* lambda:GetEventSourceMapping
action.
*
*
* @param getEventSourceMappingRequest
* @return Result of the GetEventSourceMapping operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.GetEventSourceMapping
*/
@Override
public GetEventSourceMappingResult getEventSourceMapping(
GetEventSourceMappingRequest getEventSourceMappingRequest) {
ExecutionContext executionContext = createExecutionContext(getEventSourceMappingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetEventSourceMappingRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(getEventSourceMappingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetEventSourceMappingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the configuration information of the Lambda function and a
* presigned URL link to the .zip file you uploaded with
* CreateFunction so you can download the .zip file. Note that the
* URL is valid for up to 10 minutes. The configuration information is the
* same information you provided as parameters when uploading the function.
*
*
* Using the optional Qualifier
parameter, you can specify a
* specific function version for which you want this information. If you
* don't specify this parameter, the API uses unqualified function ARN which
* return information about the $LATEST
version of the Lambda
* function. For more information, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:GetFunction
action.
*
*
* @param getFunctionRequest
* @return Result of the GetFunction operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws TooManyRequestsException
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @sample AWSLambda.GetFunction
*/
@Override
public GetFunctionResult getFunction(GetFunctionRequest getFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(getFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFunctionRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(getFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the configuration information of the Lambda function. This the
* same information you provided as parameters when uploading the function
* by using CreateFunction.
*
*
* If you are using the versioning feature, you can retrieve this
* information for a specific function version by using the optional
* Qualifier
parameter and specifying the function version or
* alias that points to it. If you don't provide it, the API returns
* information about the $LATEST version of the function. For more
* information about versioning, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:GetFunctionConfiguration
operation.
*
*
* @param getFunctionConfigurationRequest
* @return Result of the GetFunctionConfiguration operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws TooManyRequestsException
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @sample AWSLambda.GetFunctionConfiguration
*/
@Override
public GetFunctionConfigurationResult getFunctionConfiguration(
GetFunctionConfigurationRequest getFunctionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getFunctionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFunctionConfigurationRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(getFunctionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetFunctionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the resource policy associated with the specified Lambda
* function.
*
*
* If you are using the versioning feature, you can get the resource policy
* associated with the specific Lambda function version or alias by
* specifying the version or alias name using the Qualifier
* parameter. For more information about versioning, see AWS
* Lambda Function Versioning and Aliases.
*
*
* For information about adding permissions, see AddPermission.
*
*
* You need permission for the lambda:GetPolicy action.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws TooManyRequestsException
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @sample AWSLambda.GetPolicy
*/
@Override
public GetPolicyResult getPolicy(GetPolicyRequest getPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPolicyRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(getPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Invokes a specific Lambda function.
*
*
* If you are using the versioning feature, you can invoke the specific
* function version by providing function version or alias name that is
* pointing to the function version using the Qualifier
* parameter in the request. If you don't provide the Qualifier
* parameter, the $LATEST
version of the Lambda function is
* invoked. For information about the versioning feature, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:InvokeFunction
action.
*
*
* @param invokeRequest
* @return Result of the Invoke operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidRequestContentException
* The request body could not be parsed as JSON.
* @throws RequestTooLargeException
* The request payload exceeded the Invoke
request body
* JSON input limit. For more information, see Limits.
* @throws UnsupportedMediaTypeException
* The content type of the Invoke
request body is not
* JSON.
* @throws TooManyRequestsException
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws EC2UnexpectedException
* AWS Lambda received an unexpected EC2 client exception while
* setting up for the Lambda function.
* @throws SubnetIPAddressLimitReachedException
* AWS Lambda was not able to set up VPC access for the Lambda
* function because one or more configured subnets has no available
* IP addresses.
* @throws ENILimitReachedException
* AWS Lambda was not able to create an Elastic Network Interface
* (ENI) in the VPC, specified as part of Lambda function
* configuration, because the limit for network interfaces has been
* reached.
* @throws EC2ThrottledException
* AWS Lambda was throttled by Amazon EC2 during Lambda function
* initiatization using the execution role provided for the Lambda
* function.
* @throws EC2AccessDeniedException
* @throws InvalidSubnetIDException
* The Subnet ID provided in the Lambda function VPC configuration
* is invalid.
* @throws InvalidSecurityGroupIDException
* The Security Group ID provided in the Lambda function VPC
* configuration is invalid.
* @sample AWSLambda.Invoke
*/
@Override
public InvokeResult invoke(InvokeRequest invokeRequest) {
ExecutionContext executionContext = createExecutionContext(invokeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new InvokeRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(invokeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(false)
.withHasStreamingSuccessResponse(false),
new InvokeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* This API is deprecated. We recommend you use
* Invoke
API (see Invoke).
*
* Submits an invocation request to AWS Lambda. Upon receiving the request,
* Lambda executes the specified function asynchronously. To see the logs
* generated by the Lambda function execution, see the CloudWatch Logs
* console.
*
*
* This operation requires permission for the
* lambda:InvokeFunction
action.
*
*
* @param invokeAsyncRequest
* @return Result of the InvokeAsync operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidRequestContentException
* The request body could not be parsed as JSON.
* @sample AWSLambda.InvokeAsync
*/
@Override
@Deprecated
public InvokeAsyncResult invokeAsync(InvokeAsyncRequest invokeAsyncRequest) {
ExecutionContext executionContext = createExecutionContext(invokeAsyncRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new InvokeAsyncRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(invokeAsyncRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new InvokeAsyncResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns list of aliases created for a Lambda function. For each alias,
* the response includes information such as the alias ARN, description,
* alias name, and the function version to which it points. For more
* information, see Introduction to AWS Lambda Aliases.
*
*
* This requires permission for the lambda:ListAliases action.
*
*
* @param listAliasesRequest
* @return Result of the ListAliases operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.ListAliases
*/
@Override
public ListAliasesResult listAliases(ListAliasesRequest listAliasesRequest) {
ExecutionContext executionContext = createExecutionContext(listAliasesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAliasesRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(listAliasesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListAliasesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of event source mappings you created using the
* CreateEventSourceMapping
(see
* CreateEventSourceMapping).
*
*
* For each mapping, the API returns configuration information. You can
* optionally specify filters to retrieve specific event source mappings.
*
*
* If you are using the versioning feature, you can get list of event source
* mappings for a specific Lambda function version or an alias as described
* in the FunctionName
parameter. For information about the
* versioning feature, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:ListEventSourceMappings
action.
*
*
* @param listEventSourceMappingsRequest
* @return Result of the ListEventSourceMappings operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.ListEventSourceMappings
*/
@Override
public ListEventSourceMappingsResult listEventSourceMappings(
ListEventSourceMappingsRequest listEventSourceMappingsRequest) {
ExecutionContext executionContext = createExecutionContext(listEventSourceMappingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListEventSourceMappingsRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(listEventSourceMappingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListEventSourceMappingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListEventSourceMappingsResult listEventSourceMappings() {
return listEventSourceMappings(new ListEventSourceMappingsRequest());
}
/**
*
* Returns a list of your Lambda functions. For each function, the response
* includes the function configuration information. You must use
* GetFunction to retrieve the code for your function.
*
*
* This operation requires permission for the
* lambda:ListFunctions
action.
*
*
* If you are using versioning feature, the response returns list of $LATEST
* versions of your functions. For information about the versioning feature,
* see AWS
* Lambda Function Versioning and Aliases.
*
*
* @param listFunctionsRequest
* @return Result of the ListFunctions operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws TooManyRequestsException
* @sample AWSLambda.ListFunctions
*/
@Override
public ListFunctionsResult listFunctions(
ListFunctionsRequest listFunctionsRequest) {
ExecutionContext executionContext = createExecutionContext(listFunctionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFunctionsRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(listFunctionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListFunctionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListFunctionsResult listFunctions() {
return listFunctions(new ListFunctionsRequest());
}
/**
*
* List all versions of a function. For information about the versioning
* feature, see AWS
* Lambda Function Versioning and Aliases.
*
*
* @param listVersionsByFunctionRequest
* @return Result of the ListVersionsByFunction operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.ListVersionsByFunction
*/
@Override
public ListVersionsByFunctionResult listVersionsByFunction(
ListVersionsByFunctionRequest listVersionsByFunctionRequest) {
ExecutionContext executionContext = createExecutionContext(listVersionsByFunctionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVersionsByFunctionRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(listVersionsByFunctionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListVersionsByFunctionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Publishes a version of your function from the current snapshot of
* $LATEST. That is, AWS Lambda takes a snapshot of the function code and
* configuration information from $LATEST and publishes a new version. The
* code and configuration cannot be modified after publication. For
* information about the versioning feature, see AWS
* Lambda Function Versioning and Aliases.
*
*
* @param publishVersionRequest
* @return Result of the PublishVersion operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @throws CodeStorageExceededException
* You have exceeded your maximum total code size per account.
* Limits
* @sample AWSLambda.PublishVersion
*/
@Override
public PublishVersionResult publishVersion(
PublishVersionRequest publishVersionRequest) {
ExecutionContext executionContext = createExecutionContext(publishVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PublishVersionRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(publishVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new PublishVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* You can remove individual permissions from an resource policy associated
* with a Lambda function by providing a statement ID that you provided when
* you added the permission.
*
*
* If you are using versioning, the permissions you remove are specific to
* the Lambda function version or alias you specify in the
* AddPermission
request via the Qualifier
* parameter. For more information about versioning, see AWS
* Lambda Function Versioning and Aliases.
*
*
* Note that removal of a permission will cause an active event source to
* lose permission to the function.
*
*
* You need permission for the lambda:RemovePermission
action.
*
*
* @param removePermissionRequest
* @return Result of the RemovePermission operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.RemovePermission
*/
@Override
public RemovePermissionResult removePermission(
RemovePermissionRequest removePermissionRequest) {
ExecutionContext executionContext = createExecutionContext(removePermissionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemovePermissionRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(removePermissionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new RemovePermissionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Using this API you can update the function version to which the alias
* points and the alias description. For more information, see Introduction to AWS Lambda Aliases.
*
*
* This requires permission for the lambda:UpdateAlias action.
*
*
* @param updateAliasRequest
* @return Result of the UpdateAlias operation returned by the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.UpdateAlias
*/
@Override
public UpdateAliasResult updateAlias(UpdateAliasRequest updateAliasRequest) {
ExecutionContext executionContext = createExecutionContext(updateAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAliasRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(updateAliasRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateAliasResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* You can update an event source mapping. This is useful if you want to
* change the parameters of the existing mapping without losing your
* position in the stream. You can change which function will receive the
* stream records, but to change the stream itself, you must create a new
* mapping.
*
*
* If you are using the versioning feature, you can update the event source
* mapping to map to a specific Lambda function version or alias as
* described in the FunctionName
parameter. For information
* about the versioning feature, see AWS
* Lambda Function Versioning and Aliases.
*
*
* If you disable the event source mapping, AWS Lambda stops polling. If you
* enable again, it will resume polling from the time it had stopped
* polling, so you don't lose processing of any records. However, if you
* delete event source mapping and create it again, it will reset.
*
*
* This operation requires permission for the
* lambda:UpdateEventSourceMapping
action.
*
*
* @param updateEventSourceMappingRequest
* @return Result of the UpdateEventSourceMapping operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @throws ResourceConflictException
* The resource already exists.
* @sample AWSLambda.UpdateEventSourceMapping
*/
@Override
public UpdateEventSourceMappingResult updateEventSourceMapping(
UpdateEventSourceMappingRequest updateEventSourceMappingRequest) {
ExecutionContext executionContext = createExecutionContext(updateEventSourceMappingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateEventSourceMappingRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(updateEventSourceMappingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateEventSourceMappingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the code for the specified Lambda function. This operation must
* only be used on an existing Lambda function and cannot be used to update
* the function configuration.
*
*
* If you are using the versioning feature, note this API will always update
* the $LATEST version of your Lambda function. For information about the
* versioning feature, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:UpdateFunctionCode
action.
*
*
* @param updateFunctionCodeRequest
* @return Result of the UpdateFunctionCode operation returned by the
* service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @throws CodeStorageExceededException
* You have exceeded your maximum total code size per account.
* Limits
* @sample AWSLambda.UpdateFunctionCode
*/
@Override
public UpdateFunctionCodeResult updateFunctionCode(
UpdateFunctionCodeRequest updateFunctionCodeRequest) {
ExecutionContext executionContext = createExecutionContext(updateFunctionCodeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFunctionCodeRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(updateFunctionCodeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateFunctionCodeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the configuration parameters for the specified Lambda function by
* using the values provided in the request. You provide only the parameters
* you want to change. This operation must only be used on an existing
* Lambda function and cannot be used to update the function's code.
*
*
* If you are using the versioning feature, note this API will always update
* the $LATEST version of your Lambda function. For information about the
* versioning feature, see AWS
* Lambda Function Versioning and Aliases.
*
*
* This operation requires permission for the
* lambda:UpdateFunctionConfiguration
action.
*
*
* @param updateFunctionConfigurationRequest
* @return Result of the UpdateFunctionConfiguration operation returned by
* the service.
* @throws ServiceException
* The AWS Lambda service encountered an internal error.
* @throws ResourceNotFoundException
* The resource (for example, a Lambda function or access policy
* statement) specified in the request does not exist.
* @throws InvalidParameterValueException
* One of the parameters in the request is invalid. For example, if
* you provided an IAM role for AWS Lambda to assume in the
* CreateFunction
or the
* UpdateFunctionConfiguration
API, that AWS Lambda is
* unable to assume you will get this exception.
* @throws TooManyRequestsException
* @sample AWSLambda.UpdateFunctionConfiguration
*/
@Override
public UpdateFunctionConfigurationResult updateFunctionConfiguration(
UpdateFunctionConfigurationRequest updateFunctionConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateFunctionConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFunctionConfigurationRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(updateFunctionConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateFunctionConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful,
* request, typically used for debugging issues where a service isn't acting
* as expected. This data isn't considered part of the result data returned
* by an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(
AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be
* overriden at the request level.
**/
private Response invoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils
.getCredentialsProvider(request.getOriginalRequest(),
awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any
* credentials set on the client or request will be ignored for this
* operation.
**/
private Response anonymousInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack
* thereof) have been configured in the ExecutionContext beforehand.
**/
private Response doInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory
.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler,
executionContext);
}
}