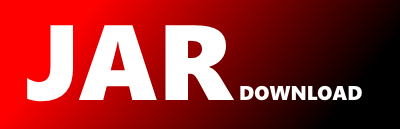
com.amazonaws.services.lambda.model.CreateFunctionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lambda Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.lambda.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*/
public class CreateFunctionRequest extends AmazonWebServiceRequest implements
Serializable, Cloneable {
/**
*
* The name you want to assign to the function you are uploading. The
* function names appear in the console and are returned in the
* ListFunctions API. Function names are used to specify functions to
* other AWS Lambda APIs, such as Invoke.
*
*/
private String functionName;
/**
*
* The runtime environment for the Lambda function you are uploading.
*
*/
private String runtime;
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Lambda assumes when
* it executes your function to access any other Amazon Web Services (AWS)
* resources. For more information, see AWS Lambda: How it Works.
*
*/
private String role;
/**
*
* The function within your code that Lambda calls to begin execution. For
* Node.js, it is the module-name.export value in your
* function. For Java, it can be package.class-name::handler
or
* package.class-name
. For more information, see Lambda Function Handler (Java).
*
*/
private String handler;
/**
*
* The code for the Lambda function.
*
*/
private FunctionCode code;
/**
*
* A short, user-defined function description. Lambda does not use this
* value. Assign a meaningful description as you see fit.
*
*/
private String description;
/**
*
* The function execution time at which Lambda should terminate the
* function. Because the execution time has cost implications, we recommend
* you set this value based on your expected execution time. The default is
* 3 seconds.
*
*/
private Integer timeout;
/**
*
* The amount of memory, in MB, your Lambda function is given. Lambda uses
* this memory size to infer the amount of CPU and memory allocated to your
* function. Your function use-case determines your CPU and memory
* requirements. For example, a database operation might need less memory
* compared to an image processing function. The default value is 128 MB.
* The value must be a multiple of 64 MB.
*
*/
private Integer memorySize;
/**
*
* This boolean parameter can be used to request AWS Lambda to create the
* Lambda function and publish a version as an atomic operation.
*
*/
private Boolean publish;
/**
*
* If your Lambda function accesses resources in a VPC, you provide this
* parameter identifying the list of security group IDs and subnet IDs.
* These must belong to the same VPC. You must provide at least one security
* group and one subnet ID.
*
*/
private VpcConfig vpcConfig;
/**
*
* The name you want to assign to the function you are uploading. The
* function names appear in the console and are returned in the
* ListFunctions API. Function names are used to specify functions to
* other AWS Lambda APIs, such as Invoke.
*
*
* @param functionName
* The name you want to assign to the function you are uploading. The
* function names appear in the console and are returned in the
* ListFunctions API. Function names are used to specify
* functions to other AWS Lambda APIs, such as Invoke.
*/
public void setFunctionName(String functionName) {
this.functionName = functionName;
}
/**
*
* The name you want to assign to the function you are uploading. The
* function names appear in the console and are returned in the
* ListFunctions API. Function names are used to specify functions to
* other AWS Lambda APIs, such as Invoke.
*
*
* @return The name you want to assign to the function you are uploading.
* The function names appear in the console and are returned in the
* ListFunctions API. Function names are used to specify
* functions to other AWS Lambda APIs, such as Invoke.
*/
public String getFunctionName() {
return this.functionName;
}
/**
*
* The name you want to assign to the function you are uploading. The
* function names appear in the console and are returned in the
* ListFunctions API. Function names are used to specify functions to
* other AWS Lambda APIs, such as Invoke.
*
*
* @param functionName
* The name you want to assign to the function you are uploading. The
* function names appear in the console and are returned in the
* ListFunctions API. Function names are used to specify
* functions to other AWS Lambda APIs, such as Invoke.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withFunctionName(String functionName) {
setFunctionName(functionName);
return this;
}
/**
*
* The runtime environment for the Lambda function you are uploading.
*
*
* @param runtime
* The runtime environment for the Lambda function you are uploading.
* @see Runtime
*/
public void setRuntime(String runtime) {
this.runtime = runtime;
}
/**
*
* The runtime environment for the Lambda function you are uploading.
*
*
* @return The runtime environment for the Lambda function you are
* uploading.
* @see Runtime
*/
public String getRuntime() {
return this.runtime;
}
/**
*
* The runtime environment for the Lambda function you are uploading.
*
*
* @param runtime
* The runtime environment for the Lambda function you are uploading.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see Runtime
*/
public CreateFunctionRequest withRuntime(String runtime) {
setRuntime(runtime);
return this;
}
/**
*
* The runtime environment for the Lambda function you are uploading.
*
*
* @param runtime
* The runtime environment for the Lambda function you are uploading.
* @see Runtime
*/
public void setRuntime(Runtime runtime) {
this.runtime = runtime.toString();
}
/**
*
* The runtime environment for the Lambda function you are uploading.
*
*
* @param runtime
* The runtime environment for the Lambda function you are uploading.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see Runtime
*/
public CreateFunctionRequest withRuntime(Runtime runtime) {
setRuntime(runtime);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Lambda assumes when
* it executes your function to access any other Amazon Web Services (AWS)
* resources. For more information, see AWS Lambda: How it Works.
*
*
* @param role
* The Amazon Resource Name (ARN) of the IAM role that Lambda assumes
* when it executes your function to access any other Amazon Web
* Services (AWS) resources. For more information, see AWS Lambda: How it Works.
*/
public void setRole(String role) {
this.role = role;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Lambda assumes when
* it executes your function to access any other Amazon Web Services (AWS)
* resources. For more information, see AWS Lambda: How it Works.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role that Lambda
* assumes when it executes your function to access any other Amazon
* Web Services (AWS) resources. For more information, see AWS Lambda: How it Works.
*/
public String getRole() {
return this.role;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that Lambda assumes when
* it executes your function to access any other Amazon Web Services (AWS)
* resources. For more information, see AWS Lambda: How it Works.
*
*
* @param role
* The Amazon Resource Name (ARN) of the IAM role that Lambda assumes
* when it executes your function to access any other Amazon Web
* Services (AWS) resources. For more information, see AWS Lambda: How it Works.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withRole(String role) {
setRole(role);
return this;
}
/**
*
* The function within your code that Lambda calls to begin execution. For
* Node.js, it is the module-name.export value in your
* function. For Java, it can be package.class-name::handler
or
* package.class-name
. For more information, see Lambda Function Handler (Java).
*
*
* @param handler
* The function within your code that Lambda calls to begin
* execution. For Node.js, it is the module-name.export
* value in your function. For Java, it can be
* package.class-name::handler
or
* package.class-name
. For more information, see Lambda Function Handler (Java).
*/
public void setHandler(String handler) {
this.handler = handler;
}
/**
*
* The function within your code that Lambda calls to begin execution. For
* Node.js, it is the module-name.export value in your
* function. For Java, it can be package.class-name::handler
or
* package.class-name
. For more information, see Lambda Function Handler (Java).
*
*
* @return The function within your code that Lambda calls to begin
* execution. For Node.js, it is the
* module-name.export value in your function. For
* Java, it can be package.class-name::handler
or
* package.class-name
. For more information, see Lambda Function Handler (Java).
*/
public String getHandler() {
return this.handler;
}
/**
*
* The function within your code that Lambda calls to begin execution. For
* Node.js, it is the module-name.export value in your
* function. For Java, it can be package.class-name::handler
or
* package.class-name
. For more information, see Lambda Function Handler (Java).
*
*
* @param handler
* The function within your code that Lambda calls to begin
* execution. For Node.js, it is the module-name.export
* value in your function. For Java, it can be
* package.class-name::handler
or
* package.class-name
. For more information, see Lambda Function Handler (Java).
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withHandler(String handler) {
setHandler(handler);
return this;
}
/**
*
* The code for the Lambda function.
*
*
* @param code
* The code for the Lambda function.
*/
public void setCode(FunctionCode code) {
this.code = code;
}
/**
*
* The code for the Lambda function.
*
*
* @return The code for the Lambda function.
*/
public FunctionCode getCode() {
return this.code;
}
/**
*
* The code for the Lambda function.
*
*
* @param code
* The code for the Lambda function.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withCode(FunctionCode code) {
setCode(code);
return this;
}
/**
*
* A short, user-defined function description. Lambda does not use this
* value. Assign a meaningful description as you see fit.
*
*
* @param description
* A short, user-defined function description. Lambda does not use
* this value. Assign a meaningful description as you see fit.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A short, user-defined function description. Lambda does not use this
* value. Assign a meaningful description as you see fit.
*
*
* @return A short, user-defined function description. Lambda does not use
* this value. Assign a meaningful description as you see fit.
*/
public String getDescription() {
return this.description;
}
/**
*
* A short, user-defined function description. Lambda does not use this
* value. Assign a meaningful description as you see fit.
*
*
* @param description
* A short, user-defined function description. Lambda does not use
* this value. Assign a meaningful description as you see fit.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The function execution time at which Lambda should terminate the
* function. Because the execution time has cost implications, we recommend
* you set this value based on your expected execution time. The default is
* 3 seconds.
*
*
* @param timeout
* The function execution time at which Lambda should terminate the
* function. Because the execution time has cost implications, we
* recommend you set this value based on your expected execution
* time. The default is 3 seconds.
*/
public void setTimeout(Integer timeout) {
this.timeout = timeout;
}
/**
*
* The function execution time at which Lambda should terminate the
* function. Because the execution time has cost implications, we recommend
* you set this value based on your expected execution time. The default is
* 3 seconds.
*
*
* @return The function execution time at which Lambda should terminate the
* function. Because the execution time has cost implications, we
* recommend you set this value based on your expected execution
* time. The default is 3 seconds.
*/
public Integer getTimeout() {
return this.timeout;
}
/**
*
* The function execution time at which Lambda should terminate the
* function. Because the execution time has cost implications, we recommend
* you set this value based on your expected execution time. The default is
* 3 seconds.
*
*
* @param timeout
* The function execution time at which Lambda should terminate the
* function. Because the execution time has cost implications, we
* recommend you set this value based on your expected execution
* time. The default is 3 seconds.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withTimeout(Integer timeout) {
setTimeout(timeout);
return this;
}
/**
*
* The amount of memory, in MB, your Lambda function is given. Lambda uses
* this memory size to infer the amount of CPU and memory allocated to your
* function. Your function use-case determines your CPU and memory
* requirements. For example, a database operation might need less memory
* compared to an image processing function. The default value is 128 MB.
* The value must be a multiple of 64 MB.
*
*
* @param memorySize
* The amount of memory, in MB, your Lambda function is given. Lambda
* uses this memory size to infer the amount of CPU and memory
* allocated to your function. Your function use-case determines your
* CPU and memory requirements. For example, a database operation
* might need less memory compared to an image processing function.
* The default value is 128 MB. The value must be a multiple of 64
* MB.
*/
public void setMemorySize(Integer memorySize) {
this.memorySize = memorySize;
}
/**
*
* The amount of memory, in MB, your Lambda function is given. Lambda uses
* this memory size to infer the amount of CPU and memory allocated to your
* function. Your function use-case determines your CPU and memory
* requirements. For example, a database operation might need less memory
* compared to an image processing function. The default value is 128 MB.
* The value must be a multiple of 64 MB.
*
*
* @return The amount of memory, in MB, your Lambda function is given.
* Lambda uses this memory size to infer the amount of CPU and
* memory allocated to your function. Your function use-case
* determines your CPU and memory requirements. For example, a
* database operation might need less memory compared to an image
* processing function. The default value is 128 MB. The value must
* be a multiple of 64 MB.
*/
public Integer getMemorySize() {
return this.memorySize;
}
/**
*
* The amount of memory, in MB, your Lambda function is given. Lambda uses
* this memory size to infer the amount of CPU and memory allocated to your
* function. Your function use-case determines your CPU and memory
* requirements. For example, a database operation might need less memory
* compared to an image processing function. The default value is 128 MB.
* The value must be a multiple of 64 MB.
*
*
* @param memorySize
* The amount of memory, in MB, your Lambda function is given. Lambda
* uses this memory size to infer the amount of CPU and memory
* allocated to your function. Your function use-case determines your
* CPU and memory requirements. For example, a database operation
* might need less memory compared to an image processing function.
* The default value is 128 MB. The value must be a multiple of 64
* MB.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withMemorySize(Integer memorySize) {
setMemorySize(memorySize);
return this;
}
/**
*
* This boolean parameter can be used to request AWS Lambda to create the
* Lambda function and publish a version as an atomic operation.
*
*
* @param publish
* This boolean parameter can be used to request AWS Lambda to create
* the Lambda function and publish a version as an atomic operation.
*/
public void setPublish(Boolean publish) {
this.publish = publish;
}
/**
*
* This boolean parameter can be used to request AWS Lambda to create the
* Lambda function and publish a version as an atomic operation.
*
*
* @return This boolean parameter can be used to request AWS Lambda to
* create the Lambda function and publish a version as an atomic
* operation.
*/
public Boolean getPublish() {
return this.publish;
}
/**
*
* This boolean parameter can be used to request AWS Lambda to create the
* Lambda function and publish a version as an atomic operation.
*
*
* @param publish
* This boolean parameter can be used to request AWS Lambda to create
* the Lambda function and publish a version as an atomic operation.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withPublish(Boolean publish) {
setPublish(publish);
return this;
}
/**
*
* This boolean parameter can be used to request AWS Lambda to create the
* Lambda function and publish a version as an atomic operation.
*
*
* @return This boolean parameter can be used to request AWS Lambda to
* create the Lambda function and publish a version as an atomic
* operation.
*/
public Boolean isPublish() {
return this.publish;
}
/**
*
* If your Lambda function accesses resources in a VPC, you provide this
* parameter identifying the list of security group IDs and subnet IDs.
* These must belong to the same VPC. You must provide at least one security
* group and one subnet ID.
*
*
* @param vpcConfig
* If your Lambda function accesses resources in a VPC, you provide
* this parameter identifying the list of security group IDs and
* subnet IDs. These must belong to the same VPC. You must provide at
* least one security group and one subnet ID.
*/
public void setVpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* If your Lambda function accesses resources in a VPC, you provide this
* parameter identifying the list of security group IDs and subnet IDs.
* These must belong to the same VPC. You must provide at least one security
* group and one subnet ID.
*
*
* @return If your Lambda function accesses resources in a VPC, you provide
* this parameter identifying the list of security group IDs and
* subnet IDs. These must belong to the same VPC. You must provide
* at least one security group and one subnet ID.
*/
public VpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* If your Lambda function accesses resources in a VPC, you provide this
* parameter identifying the list of security group IDs and subnet IDs.
* These must belong to the same VPC. You must provide at least one security
* group and one subnet ID.
*
*
* @param vpcConfig
* If your Lambda function accesses resources in a VPC, you provide
* this parameter identifying the list of security group IDs and
* subnet IDs. These must belong to the same VPC. You must provide at
* least one security group and one subnet ID.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateFunctionRequest withVpcConfig(VpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFunctionName() != null)
sb.append("FunctionName: " + getFunctionName() + ",");
if (getRuntime() != null)
sb.append("Runtime: " + getRuntime() + ",");
if (getRole() != null)
sb.append("Role: " + getRole() + ",");
if (getHandler() != null)
sb.append("Handler: " + getHandler() + ",");
if (getCode() != null)
sb.append("Code: " + getCode() + ",");
if (getDescription() != null)
sb.append("Description: " + getDescription() + ",");
if (getTimeout() != null)
sb.append("Timeout: " + getTimeout() + ",");
if (getMemorySize() != null)
sb.append("MemorySize: " + getMemorySize() + ",");
if (getPublish() != null)
sb.append("Publish: " + getPublish() + ",");
if (getVpcConfig() != null)
sb.append("VpcConfig: " + getVpcConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateFunctionRequest == false)
return false;
CreateFunctionRequest other = (CreateFunctionRequest) obj;
if (other.getFunctionName() == null ^ this.getFunctionName() == null)
return false;
if (other.getFunctionName() != null
&& other.getFunctionName().equals(this.getFunctionName()) == false)
return false;
if (other.getRuntime() == null ^ this.getRuntime() == null)
return false;
if (other.getRuntime() != null
&& other.getRuntime().equals(this.getRuntime()) == false)
return false;
if (other.getRole() == null ^ this.getRole() == null)
return false;
if (other.getRole() != null
&& other.getRole().equals(this.getRole()) == false)
return false;
if (other.getHandler() == null ^ this.getHandler() == null)
return false;
if (other.getHandler() != null
&& other.getHandler().equals(this.getHandler()) == false)
return false;
if (other.getCode() == null ^ this.getCode() == null)
return false;
if (other.getCode() != null
&& other.getCode().equals(this.getCode()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null
&& other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getTimeout() == null ^ this.getTimeout() == null)
return false;
if (other.getTimeout() != null
&& other.getTimeout().equals(this.getTimeout()) == false)
return false;
if (other.getMemorySize() == null ^ this.getMemorySize() == null)
return false;
if (other.getMemorySize() != null
&& other.getMemorySize().equals(this.getMemorySize()) == false)
return false;
if (other.getPublish() == null ^ this.getPublish() == null)
return false;
if (other.getPublish() != null
&& other.getPublish().equals(this.getPublish()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null
&& other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getFunctionName() == null) ? 0 : getFunctionName()
.hashCode());
hashCode = prime * hashCode
+ ((getRuntime() == null) ? 0 : getRuntime().hashCode());
hashCode = prime * hashCode
+ ((getRole() == null) ? 0 : getRole().hashCode());
hashCode = prime * hashCode
+ ((getHandler() == null) ? 0 : getHandler().hashCode());
hashCode = prime * hashCode
+ ((getCode() == null) ? 0 : getCode().hashCode());
hashCode = prime
* hashCode
+ ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode
+ ((getTimeout() == null) ? 0 : getTimeout().hashCode());
hashCode = prime * hashCode
+ ((getMemorySize() == null) ? 0 : getMemorySize().hashCode());
hashCode = prime * hashCode
+ ((getPublish() == null) ? 0 : getPublish().hashCode());
hashCode = prime * hashCode
+ ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
return hashCode;
}
@Override
public CreateFunctionRequest clone() {
return (CreateFunctionRequest) super.clone();
}
}