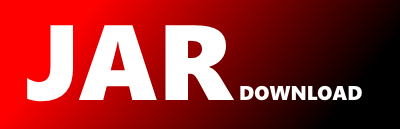
com.amazonaws.services.lambda.model.InvokeRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lambda Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.lambda.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*/
public class InvokeRequest extends AmazonWebServiceRequest implements
Serializable, Cloneable {
/**
*
* The Lambda function name.
*
*
* You can specify a function name (for example, Thumbnail
) or
* you can specify Amazon Resource Name (ARN) of the function (for example,
* arn:aws:lambda:us-west-2:account-id:function:ThumbNail
). AWS
* Lambda also allows you to specify a partial ARN (for example,
* account-id:Thumbnail
). Note that the length constraint
* applies only to the ARN. If you specify only the function name, it is
* limited to 64 character in length.
*
*/
private String functionName;
/**
*
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally request
* asynchronous execution by specifying Event
as the
* InvocationType
. You can also use this parameter to request
* AWS Lambda to not execute the function but do some verification, such as
* if the caller is authorized to invoke the function and if the inputs are
* valid. You request this by specifying DryRun
as the
* InvocationType
. This is useful in a cross-account scenario
* when you want to verify access to a function without running it.
*
*/
private String invocationType;
/**
*
* You can set this optional parameter to Tail
in the request
* only if you specify the InvocationType
parameter with value
* RequestResponse
. In this case, AWS Lambda returns the
* base64-encoded last 4 KB of log data produced by your Lambda function in
* the x-amz-log-results
header.
*
*/
private String logType;
/**
*
* Using the ClientContext
you can pass client-specific
* information to the Lambda function you are invoking. You can then process
* the client information in your Lambda function as you choose through the
* context variable. For an example of a ClientContext
JSON,
* see PutEvents in the Amazon Mobile Analytics API Reference and User
* Guide.
*
*
* The ClientContext JSON must be base64-encoded.
*
*/
private String clientContext;
/**
*
* JSON that you want to provide to your Lambda function as input.
*
*/
private java.nio.ByteBuffer payload;
/**
*
* You can use this optional parameter to specify a Lambda function version
* or alias name. If you specify a function version, the API uses the
* qualified function ARN to invoke a specific Lambda function. If you
* specify an alias name, the API uses the alias ARN to invoke the Lambda
* function version to which the alias points.
*
*
* If you don't provide this parameter, then the API uses unqualified
* function ARN which results in invocation of the $LATEST
* version.
*
*/
private String qualifier;
/**
*
* The Lambda function name.
*
*
* You can specify a function name (for example, Thumbnail
) or
* you can specify Amazon Resource Name (ARN) of the function (for example,
* arn:aws:lambda:us-west-2:account-id:function:ThumbNail
). AWS
* Lambda also allows you to specify a partial ARN (for example,
* account-id:Thumbnail
). Note that the length constraint
* applies only to the ARN. If you specify only the function name, it is
* limited to 64 character in length.
*
*
* @param functionName
* The Lambda function name.
*
* You can specify a function name (for example,
* Thumbnail
) or you can specify Amazon Resource Name
* (ARN) of the function (for example,
* arn:aws:lambda:us-west-2:account-id:function:ThumbNail
* ). AWS Lambda also allows you to specify a partial ARN (for
* example, account-id:Thumbnail
). Note that the length
* constraint applies only to the ARN. If you specify only the
* function name, it is limited to 64 character in length.
*/
public void setFunctionName(String functionName) {
this.functionName = functionName;
}
/**
*
* The Lambda function name.
*
*
* You can specify a function name (for example, Thumbnail
) or
* you can specify Amazon Resource Name (ARN) of the function (for example,
* arn:aws:lambda:us-west-2:account-id:function:ThumbNail
). AWS
* Lambda also allows you to specify a partial ARN (for example,
* account-id:Thumbnail
). Note that the length constraint
* applies only to the ARN. If you specify only the function name, it is
* limited to 64 character in length.
*
*
* @return The Lambda function name.
*
* You can specify a function name (for example,
* Thumbnail
) or you can specify Amazon Resource Name
* (ARN) of the function (for example,
* arn:aws:lambda:us-west-2:account-id:function:ThumbNail
* ). AWS Lambda also allows you to specify a partial ARN (for
* example, account-id:Thumbnail
). Note that the length
* constraint applies only to the ARN. If you specify only the
* function name, it is limited to 64 character in length.
*/
public String getFunctionName() {
return this.functionName;
}
/**
*
* The Lambda function name.
*
*
* You can specify a function name (for example, Thumbnail
) or
* you can specify Amazon Resource Name (ARN) of the function (for example,
* arn:aws:lambda:us-west-2:account-id:function:ThumbNail
). AWS
* Lambda also allows you to specify a partial ARN (for example,
* account-id:Thumbnail
). Note that the length constraint
* applies only to the ARN. If you specify only the function name, it is
* limited to 64 character in length.
*
*
* @param functionName
* The Lambda function name.
*
* You can specify a function name (for example,
* Thumbnail
) or you can specify Amazon Resource Name
* (ARN) of the function (for example,
* arn:aws:lambda:us-west-2:account-id:function:ThumbNail
* ). AWS Lambda also allows you to specify a partial ARN (for
* example, account-id:Thumbnail
). Note that the length
* constraint applies only to the ARN. If you specify only the
* function name, it is limited to 64 character in length.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public InvokeRequest withFunctionName(String functionName) {
setFunctionName(functionName);
return this;
}
/**
*
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally request
* asynchronous execution by specifying Event
as the
* InvocationType
. You can also use this parameter to request
* AWS Lambda to not execute the function but do some verification, such as
* if the caller is authorized to invoke the function and if the inputs are
* valid. You request this by specifying DryRun
as the
* InvocationType
. This is useful in a cross-account scenario
* when you want to verify access to a function without running it.
*
*
* @param invocationType
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally
* request asynchronous execution by specifying Event
as
* the InvocationType
. You can also use this parameter
* to request AWS Lambda to not execute the function but do some
* verification, such as if the caller is authorized to invoke the
* function and if the inputs are valid. You request this by
* specifying DryRun
as the InvocationType
.
* This is useful in a cross-account scenario when you want to verify
* access to a function without running it.
* @see InvocationType
*/
public void setInvocationType(String invocationType) {
this.invocationType = invocationType;
}
/**
*
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally request
* asynchronous execution by specifying Event
as the
* InvocationType
. You can also use this parameter to request
* AWS Lambda to not execute the function but do some verification, such as
* if the caller is authorized to invoke the function and if the inputs are
* valid. You request this by specifying DryRun
as the
* InvocationType
. This is useful in a cross-account scenario
* when you want to verify access to a function without running it.
*
*
* @return By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally
* request asynchronous execution by specifying Event
* as the InvocationType
. You can also use this
* parameter to request AWS Lambda to not execute the function but
* do some verification, such as if the caller is authorized to
* invoke the function and if the inputs are valid. You request this
* by specifying DryRun
as the
* InvocationType
. This is useful in a cross-account
* scenario when you want to verify access to a function without
* running it.
* @see InvocationType
*/
public String getInvocationType() {
return this.invocationType;
}
/**
*
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally request
* asynchronous execution by specifying Event
as the
* InvocationType
. You can also use this parameter to request
* AWS Lambda to not execute the function but do some verification, such as
* if the caller is authorized to invoke the function and if the inputs are
* valid. You request this by specifying DryRun
as the
* InvocationType
. This is useful in a cross-account scenario
* when you want to verify access to a function without running it.
*
*
* @param invocationType
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally
* request asynchronous execution by specifying Event
as
* the InvocationType
. You can also use this parameter
* to request AWS Lambda to not execute the function but do some
* verification, such as if the caller is authorized to invoke the
* function and if the inputs are valid. You request this by
* specifying DryRun
as the InvocationType
.
* This is useful in a cross-account scenario when you want to verify
* access to a function without running it.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see InvocationType
*/
public InvokeRequest withInvocationType(String invocationType) {
setInvocationType(invocationType);
return this;
}
/**
*
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally request
* asynchronous execution by specifying Event
as the
* InvocationType
. You can also use this parameter to request
* AWS Lambda to not execute the function but do some verification, such as
* if the caller is authorized to invoke the function and if the inputs are
* valid. You request this by specifying DryRun
as the
* InvocationType
. This is useful in a cross-account scenario
* when you want to verify access to a function without running it.
*
*
* @param invocationType
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally
* request asynchronous execution by specifying Event
as
* the InvocationType
. You can also use this parameter
* to request AWS Lambda to not execute the function but do some
* verification, such as if the caller is authorized to invoke the
* function and if the inputs are valid. You request this by
* specifying DryRun
as the InvocationType
.
* This is useful in a cross-account scenario when you want to verify
* access to a function without running it.
* @see InvocationType
*/
public void setInvocationType(InvocationType invocationType) {
this.invocationType = invocationType.toString();
}
/**
*
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally request
* asynchronous execution by specifying Event
as the
* InvocationType
. You can also use this parameter to request
* AWS Lambda to not execute the function but do some verification, such as
* if the caller is authorized to invoke the function and if the inputs are
* valid. You request this by specifying DryRun
as the
* InvocationType
. This is useful in a cross-account scenario
* when you want to verify access to a function without running it.
*
*
* @param invocationType
* By default, the Invoke
API assumes
* RequestResponse
invocation type. You can optionally
* request asynchronous execution by specifying Event
as
* the InvocationType
. You can also use this parameter
* to request AWS Lambda to not execute the function but do some
* verification, such as if the caller is authorized to invoke the
* function and if the inputs are valid. You request this by
* specifying DryRun
as the InvocationType
.
* This is useful in a cross-account scenario when you want to verify
* access to a function without running it.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see InvocationType
*/
public InvokeRequest withInvocationType(InvocationType invocationType) {
setInvocationType(invocationType);
return this;
}
/**
*
* You can set this optional parameter to Tail
in the request
* only if you specify the InvocationType
parameter with value
* RequestResponse
. In this case, AWS Lambda returns the
* base64-encoded last 4 KB of log data produced by your Lambda function in
* the x-amz-log-results
header.
*
*
* @param logType
* You can set this optional parameter to Tail
in the
* request only if you specify the InvocationType
* parameter with value RequestResponse
. In this case,
* AWS Lambda returns the base64-encoded last 4 KB of log data
* produced by your Lambda function in the
* x-amz-log-results
header.
* @see LogType
*/
public void setLogType(String logType) {
this.logType = logType;
}
/**
*
* You can set this optional parameter to Tail
in the request
* only if you specify the InvocationType
parameter with value
* RequestResponse
. In this case, AWS Lambda returns the
* base64-encoded last 4 KB of log data produced by your Lambda function in
* the x-amz-log-results
header.
*
*
* @return You can set this optional parameter to Tail
in the
* request only if you specify the InvocationType
* parameter with value RequestResponse
. In this case,
* AWS Lambda returns the base64-encoded last 4 KB of log data
* produced by your Lambda function in the
* x-amz-log-results
header.
* @see LogType
*/
public String getLogType() {
return this.logType;
}
/**
*
* You can set this optional parameter to Tail
in the request
* only if you specify the InvocationType
parameter with value
* RequestResponse
. In this case, AWS Lambda returns the
* base64-encoded last 4 KB of log data produced by your Lambda function in
* the x-amz-log-results
header.
*
*
* @param logType
* You can set this optional parameter to Tail
in the
* request only if you specify the InvocationType
* parameter with value RequestResponse
. In this case,
* AWS Lambda returns the base64-encoded last 4 KB of log data
* produced by your Lambda function in the
* x-amz-log-results
header.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see LogType
*/
public InvokeRequest withLogType(String logType) {
setLogType(logType);
return this;
}
/**
*
* You can set this optional parameter to Tail
in the request
* only if you specify the InvocationType
parameter with value
* RequestResponse
. In this case, AWS Lambda returns the
* base64-encoded last 4 KB of log data produced by your Lambda function in
* the x-amz-log-results
header.
*
*
* @param logType
* You can set this optional parameter to Tail
in the
* request only if you specify the InvocationType
* parameter with value RequestResponse
. In this case,
* AWS Lambda returns the base64-encoded last 4 KB of log data
* produced by your Lambda function in the
* x-amz-log-results
header.
* @see LogType
*/
public void setLogType(LogType logType) {
this.logType = logType.toString();
}
/**
*
* You can set this optional parameter to Tail
in the request
* only if you specify the InvocationType
parameter with value
* RequestResponse
. In this case, AWS Lambda returns the
* base64-encoded last 4 KB of log data produced by your Lambda function in
* the x-amz-log-results
header.
*
*
* @param logType
* You can set this optional parameter to Tail
in the
* request only if you specify the InvocationType
* parameter with value RequestResponse
. In this case,
* AWS Lambda returns the base64-encoded last 4 KB of log data
* produced by your Lambda function in the
* x-amz-log-results
header.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see LogType
*/
public InvokeRequest withLogType(LogType logType) {
setLogType(logType);
return this;
}
/**
*
* Using the ClientContext
you can pass client-specific
* information to the Lambda function you are invoking. You can then process
* the client information in your Lambda function as you choose through the
* context variable. For an example of a ClientContext
JSON,
* see PutEvents in the Amazon Mobile Analytics API Reference and User
* Guide.
*
*
* The ClientContext JSON must be base64-encoded.
*
*
* @param clientContext
* Using the ClientContext
you can pass client-specific
* information to the Lambda function you are invoking. You can then
* process the client information in your Lambda function as you
* choose through the context variable. For an example of a
* ClientContext
JSON, see PutEvents in the Amazon Mobile Analytics API Reference and
* User Guide.
*
* The ClientContext JSON must be base64-encoded.
*/
public void setClientContext(String clientContext) {
this.clientContext = clientContext;
}
/**
*
* Using the ClientContext
you can pass client-specific
* information to the Lambda function you are invoking. You can then process
* the client information in your Lambda function as you choose through the
* context variable. For an example of a ClientContext
JSON,
* see PutEvents in the Amazon Mobile Analytics API Reference and User
* Guide.
*
*
* The ClientContext JSON must be base64-encoded.
*
*
* @return Using the ClientContext
you can pass client-specific
* information to the Lambda function you are invoking. You can then
* process the client information in your Lambda function as you
* choose through the context variable. For an example of a
* ClientContext
JSON, see PutEvents in the Amazon Mobile Analytics API Reference
* and User Guide.
*
* The ClientContext JSON must be base64-encoded.
*/
public String getClientContext() {
return this.clientContext;
}
/**
*
* Using the ClientContext
you can pass client-specific
* information to the Lambda function you are invoking. You can then process
* the client information in your Lambda function as you choose through the
* context variable. For an example of a ClientContext
JSON,
* see PutEvents in the Amazon Mobile Analytics API Reference and User
* Guide.
*
*
* The ClientContext JSON must be base64-encoded.
*
*
* @param clientContext
* Using the ClientContext
you can pass client-specific
* information to the Lambda function you are invoking. You can then
* process the client information in your Lambda function as you
* choose through the context variable. For an example of a
* ClientContext
JSON, see PutEvents in the Amazon Mobile Analytics API Reference and
* User Guide.
*
* The ClientContext JSON must be base64-encoded.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public InvokeRequest withClientContext(String clientContext) {
setClientContext(clientContext);
return this;
}
/**
*
* JSON that you want to provide to your Lambda function as input.
*
*
* AWS SDK for Java performs a Base64 encoding on this field before sending
* this request to AWS service by default. Users of the SDK should not
* perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the
* content or position of the byte buffer will be seen by all objects that
* have a reference to this object. It is recommended to call
* ByteBuffer.duplicate() or ByteBuffer.asReadOnlyBuffer() before using or
* reading from the buffer. This behavior will be changed in a future major
* version of the SDK.
*
*
* @param payload
* JSON that you want to provide to your Lambda function as input.
*/
public void setPayload(java.nio.ByteBuffer payload) {
this.payload = payload;
}
/**
*
* JSON that you want to provide to your Lambda function as input.
*
*
* {@code ByteBuffer}s are stateful. Calling their {@code get} methods
* changes their {@code position}. We recommend using
* {@link java.nio.ByteBuffer#asReadOnlyBuffer()} to create a read-only view
* of the buffer with an independent {@code position}, and calling
* {@code get} methods on this rather than directly on the returned
* {@code ByteBuffer}. Doing so will ensure that anyone else using the
* {@code ByteBuffer} will not be affected by changes to the {@code position}
* .
*
*
* @return JSON that you want to provide to your Lambda function as input.
*/
public java.nio.ByteBuffer getPayload() {
return this.payload;
}
/**
*
* JSON that you want to provide to your Lambda function as input.
*
*
* @param payload
* JSON that you want to provide to your Lambda function as input.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public InvokeRequest withPayload(java.nio.ByteBuffer payload) {
setPayload(payload);
return this;
}
/**
*
* JSON that you want to provide to your Lambda function as input.
*
*
* AWS SDK for Java performs a Base64 encoding on this field before sending
* this request to AWS service by default. Users of the SDK should not
* perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the
* content or position of the byte buffer will be seen by all objects that
* have a reference to this object. It is recommended to call
* ByteBuffer.duplicate() or ByteBuffer.asReadOnlyBuffer() before using or
* reading from the buffer. This behavior will be changed in a future major
* version of the SDK.
*
*
* @param payload
* JSON that you want to provide to your Lambda function as input.
*/
public void setPayload(String payload) {
setPayload(new com.amazonaws.adapters.types.StringToByteBufferAdapter()
.adapt(payload));
}
/**
*
* JSON that you want to provide to your Lambda function as input.
*
*
* @param payload
* JSON that you want to provide to your Lambda function as input.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public InvokeRequest withPayload(String payload) {
setPayload(new com.amazonaws.adapters.types.StringToByteBufferAdapter()
.adapt(payload));
return this;
}
/**
*
* You can use this optional parameter to specify a Lambda function version
* or alias name. If you specify a function version, the API uses the
* qualified function ARN to invoke a specific Lambda function. If you
* specify an alias name, the API uses the alias ARN to invoke the Lambda
* function version to which the alias points.
*
*
* If you don't provide this parameter, then the API uses unqualified
* function ARN which results in invocation of the $LATEST
* version.
*
*
* @param qualifier
* You can use this optional parameter to specify a Lambda function
* version or alias name. If you specify a function version, the API
* uses the qualified function ARN to invoke a specific Lambda
* function. If you specify an alias name, the API uses the alias ARN
* to invoke the Lambda function version to which the alias
* points.
*
* If you don't provide this parameter, then the API uses unqualified
* function ARN which results in invocation of the
* $LATEST
version.
*/
public void setQualifier(String qualifier) {
this.qualifier = qualifier;
}
/**
*
* You can use this optional parameter to specify a Lambda function version
* or alias name. If you specify a function version, the API uses the
* qualified function ARN to invoke a specific Lambda function. If you
* specify an alias name, the API uses the alias ARN to invoke the Lambda
* function version to which the alias points.
*
*
* If you don't provide this parameter, then the API uses unqualified
* function ARN which results in invocation of the $LATEST
* version.
*
*
* @return You can use this optional parameter to specify a Lambda function
* version or alias name. If you specify a function version, the API
* uses the qualified function ARN to invoke a specific Lambda
* function. If you specify an alias name, the API uses the alias
* ARN to invoke the Lambda function version to which the alias
* points.
*
* If you don't provide this parameter, then the API uses
* unqualified function ARN which results in invocation of the
* $LATEST
version.
*/
public String getQualifier() {
return this.qualifier;
}
/**
*
* You can use this optional parameter to specify a Lambda function version
* or alias name. If you specify a function version, the API uses the
* qualified function ARN to invoke a specific Lambda function. If you
* specify an alias name, the API uses the alias ARN to invoke the Lambda
* function version to which the alias points.
*
*
* If you don't provide this parameter, then the API uses unqualified
* function ARN which results in invocation of the $LATEST
* version.
*
*
* @param qualifier
* You can use this optional parameter to specify a Lambda function
* version or alias name. If you specify a function version, the API
* uses the qualified function ARN to invoke a specific Lambda
* function. If you specify an alias name, the API uses the alias ARN
* to invoke the Lambda function version to which the alias
* points.
*
* If you don't provide this parameter, then the API uses unqualified
* function ARN which results in invocation of the
* $LATEST
version.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public InvokeRequest withQualifier(String qualifier) {
setQualifier(qualifier);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFunctionName() != null)
sb.append("FunctionName: " + getFunctionName() + ",");
if (getInvocationType() != null)
sb.append("InvocationType: " + getInvocationType() + ",");
if (getLogType() != null)
sb.append("LogType: " + getLogType() + ",");
if (getClientContext() != null)
sb.append("ClientContext: " + getClientContext() + ",");
if (getPayload() != null)
sb.append("Payload: " + getPayload() + ",");
if (getQualifier() != null)
sb.append("Qualifier: " + getQualifier());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InvokeRequest == false)
return false;
InvokeRequest other = (InvokeRequest) obj;
if (other.getFunctionName() == null ^ this.getFunctionName() == null)
return false;
if (other.getFunctionName() != null
&& other.getFunctionName().equals(this.getFunctionName()) == false)
return false;
if (other.getInvocationType() == null
^ this.getInvocationType() == null)
return false;
if (other.getInvocationType() != null
&& other.getInvocationType().equals(this.getInvocationType()) == false)
return false;
if (other.getLogType() == null ^ this.getLogType() == null)
return false;
if (other.getLogType() != null
&& other.getLogType().equals(this.getLogType()) == false)
return false;
if (other.getClientContext() == null ^ this.getClientContext() == null)
return false;
if (other.getClientContext() != null
&& other.getClientContext().equals(this.getClientContext()) == false)
return false;
if (other.getPayload() == null ^ this.getPayload() == null)
return false;
if (other.getPayload() != null
&& other.getPayload().equals(this.getPayload()) == false)
return false;
if (other.getQualifier() == null ^ this.getQualifier() == null)
return false;
if (other.getQualifier() != null
&& other.getQualifier().equals(this.getQualifier()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getFunctionName() == null) ? 0 : getFunctionName()
.hashCode());
hashCode = prime
* hashCode
+ ((getInvocationType() == null) ? 0 : getInvocationType()
.hashCode());
hashCode = prime * hashCode
+ ((getLogType() == null) ? 0 : getLogType().hashCode());
hashCode = prime
* hashCode
+ ((getClientContext() == null) ? 0 : getClientContext()
.hashCode());
hashCode = prime * hashCode
+ ((getPayload() == null) ? 0 : getPayload().hashCode());
hashCode = prime * hashCode
+ ((getQualifier() == null) ? 0 : getQualifier().hashCode());
return hashCode;
}
@Override
public InvokeRequest clone() {
return (InvokeRequest) super.clone();
}
}