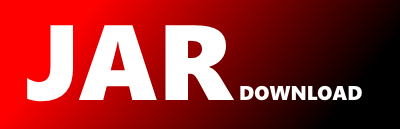
com.amazonaws.services.lexmodelbuilding.AmazonLexModelBuildingAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding;
import javax.annotation.Generated;
import com.amazonaws.services.lexmodelbuilding.model.*;
/**
* Interface for accessing Amazon Lex Model Building Service asynchronously. Each asynchronous method will return a Java
* Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.lexmodelbuilding.AbstractAmazonLexModelBuildingAsync} instead.
*
*
* Amazon Lex Build-Time Actions
*
* Amazon Lex is an AWS service for building conversational voice and text interfaces. Use these actions to create,
* update, and delete conversational bots for new and existing client applications.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonLexModelBuildingAsync extends AmazonLexModelBuilding {
/**
*
* Creates a new version of the bot based on the $LATEST
version. If the $LATEST
version
* of this resource hasn't changed since you created the last version, Amazon Lex doesn't create a new version. It
* returns the last created version.
*
*
*
* You can update only the $LATEST
version of the bot. You can't update the numbered versions that you
* create with the CreateBotVersion
operation.
*
*
*
* When you create the first version of a bot, Amazon Lex sets the version to 1. Subsequent versions increment by 1.
* For more information, see versioning-intro.
*
*
* This operation requires permission for the lex:CreateBotVersion
action.
*
*
* @param createBotVersionRequest
* @return A Java Future containing the result of the CreateBotVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsync.CreateBotVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createBotVersionAsync(CreateBotVersionRequest createBotVersionRequest);
/**
*
* Creates a new version of the bot based on the $LATEST
version. If the $LATEST
version
* of this resource hasn't changed since you created the last version, Amazon Lex doesn't create a new version. It
* returns the last created version.
*
*
*
* You can update only the $LATEST
version of the bot. You can't update the numbered versions that you
* create with the CreateBotVersion
operation.
*
*
*
* When you create the first version of a bot, Amazon Lex sets the version to 1. Subsequent versions increment by 1.
* For more information, see versioning-intro.
*
*
* This operation requires permission for the lex:CreateBotVersion
action.
*
*
* @param createBotVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBotVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.CreateBotVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createBotVersionAsync(CreateBotVersionRequest createBotVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new version of an intent based on the $LATEST
version of the intent. If the
* $LATEST
version of this intent hasn't changed since you last updated it, Amazon Lex doesn't create a
* new version. It returns the last version you created.
*
*
*
* You can update only the $LATEST
version of the intent. You can't update the numbered versions that
* you create with the CreateIntentVersion
operation.
*
*
*
* When you create a version of an intent, Amazon Lex sets the version to 1. Subsequent versions increment by 1. For
* more information, see versioning-intro.
*
*
* This operation requires permissions to perform the lex:CreateIntentVersion
action.
*
*
* @param createIntentVersionRequest
* @return A Java Future containing the result of the CreateIntentVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsync.CreateIntentVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createIntentVersionAsync(CreateIntentVersionRequest createIntentVersionRequest);
/**
*
* Creates a new version of an intent based on the $LATEST
version of the intent. If the
* $LATEST
version of this intent hasn't changed since you last updated it, Amazon Lex doesn't create a
* new version. It returns the last version you created.
*
*
*
* You can update only the $LATEST
version of the intent. You can't update the numbered versions that
* you create with the CreateIntentVersion
operation.
*
*
*
* When you create a version of an intent, Amazon Lex sets the version to 1. Subsequent versions increment by 1. For
* more information, see versioning-intro.
*
*
* This operation requires permissions to perform the lex:CreateIntentVersion
action.
*
*
* @param createIntentVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIntentVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.CreateIntentVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createIntentVersionAsync(CreateIntentVersionRequest createIntentVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new version of a slot type based on the $LATEST
version of the specified slot type. If the
* $LATEST
version of this resource has not changed since the last version that you created, Amazon Lex
* doesn't create a new version. It returns the last version that you created.
*
*
*
* You can update only the $LATEST
version of a slot type. You can't update the numbered versions that
* you create with the CreateSlotTypeVersion
operation.
*
*
*
* When you create a version of a slot type, Amazon Lex sets the version to 1. Subsequent versions increment by 1.
* For more information, see versioning-intro.
*
*
* This operation requires permissions for the lex:CreateSlotTypeVersion
action.
*
*
* @param createSlotTypeVersionRequest
* @return A Java Future containing the result of the CreateSlotTypeVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsync.CreateSlotTypeVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createSlotTypeVersionAsync(CreateSlotTypeVersionRequest createSlotTypeVersionRequest);
/**
*
* Creates a new version of a slot type based on the $LATEST
version of the specified slot type. If the
* $LATEST
version of this resource has not changed since the last version that you created, Amazon Lex
* doesn't create a new version. It returns the last version that you created.
*
*
*
* You can update only the $LATEST
version of a slot type. You can't update the numbered versions that
* you create with the CreateSlotTypeVersion
operation.
*
*
*
* When you create a version of a slot type, Amazon Lex sets the version to 1. Subsequent versions increment by 1.
* For more information, see versioning-intro.
*
*
* This operation requires permissions for the lex:CreateSlotTypeVersion
action.
*
*
* @param createSlotTypeVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSlotTypeVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.CreateSlotTypeVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createSlotTypeVersionAsync(CreateSlotTypeVersionRequest createSlotTypeVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes all versions of the bot, including the $LATEST
version. To delete a specific version of the
* bot, use the DeleteBotVersion operation.
*
*
* If a bot has an alias, you can't delete it. Instead, the DeleteBot
operation returns a
* ResourceInUseException
exception that includes a reference to the alias that refers to the bot. To
* remove the reference to the bot, delete the alias. If you get the same exception again, delete the referring
* alias until the DeleteBot
operation is successful.
*
*
* This operation requires permissions for the lex:DeleteBot
action.
*
*
* @param deleteBotRequest
* @return A Java Future containing the result of the DeleteBot operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBotAsync(DeleteBotRequest deleteBotRequest);
/**
*
* Deletes all versions of the bot, including the $LATEST
version. To delete a specific version of the
* bot, use the DeleteBotVersion operation.
*
*
* If a bot has an alias, you can't delete it. Instead, the DeleteBot
operation returns a
* ResourceInUseException
exception that includes a reference to the alias that refers to the bot. To
* remove the reference to the bot, delete the alias. If you get the same exception again, delete the referring
* alias until the DeleteBot
operation is successful.
*
*
* This operation requires permissions for the lex:DeleteBot
action.
*
*
* @param deleteBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBot operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBotAsync(DeleteBotRequest deleteBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an alias for the specified bot.
*
*
* You can't delete an alias that is used in the association between a bot and a messaging channel. If an alias is
* used in a channel association, the DeleteBot
operation returns a ResourceInUseException
* exception that includes a reference to the channel association that refers to the bot. You can remove the
* reference to the alias by deleting the channel association. If you get the same exception again, delete the
* referring association until the DeleteBotAlias
operation is successful.
*
*
* @param deleteBotAliasRequest
* @return A Java Future containing the result of the DeleteBotAlias operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteBotAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBotAliasAsync(DeleteBotAliasRequest deleteBotAliasRequest);
/**
*
* Deletes an alias for the specified bot.
*
*
* You can't delete an alias that is used in the association between a bot and a messaging channel. If an alias is
* used in a channel association, the DeleteBot
operation returns a ResourceInUseException
* exception that includes a reference to the channel association that refers to the bot. You can remove the
* reference to the alias by deleting the channel association. If you get the same exception again, delete the
* referring association until the DeleteBotAlias
operation is successful.
*
*
* @param deleteBotAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBotAlias operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteBotAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBotAliasAsync(DeleteBotAliasRequest deleteBotAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the association between an Amazon Lex bot and a messaging platform.
*
*
* This operation requires permission for the lex:DeleteBotChannelAssociation
action.
*
*
* @param deleteBotChannelAssociationRequest
* @return A Java Future containing the result of the DeleteBotChannelAssociation operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteBotChannelAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBotChannelAssociationAsync(
DeleteBotChannelAssociationRequest deleteBotChannelAssociationRequest);
/**
*
* Deletes the association between an Amazon Lex bot and a messaging platform.
*
*
* This operation requires permission for the lex:DeleteBotChannelAssociation
action.
*
*
* @param deleteBotChannelAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBotChannelAssociation operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteBotChannelAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBotChannelAssociationAsync(
DeleteBotChannelAssociationRequest deleteBotChannelAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specific version of a bot. To delete all versions of a bot, use the DeleteBot operation.
*
*
* This operation requires permissions for the lex:DeleteBotVersion
action.
*
*
* @param deleteBotVersionRequest
* @return A Java Future containing the result of the DeleteBotVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteBotVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteBotVersionAsync(DeleteBotVersionRequest deleteBotVersionRequest);
/**
*
* Deletes a specific version of a bot. To delete all versions of a bot, use the DeleteBot operation.
*
*
* This operation requires permissions for the lex:DeleteBotVersion
action.
*
*
* @param deleteBotVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBotVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteBotVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteBotVersionAsync(DeleteBotVersionRequest deleteBotVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes all versions of the intent, including the $LATEST
version. To delete a specific version of
* the intent, use the DeleteIntentVersion operation.
*
*
* You can delete a version of an intent only if it is not referenced. To delete an intent that is referred to in
* one or more bots (see how-it-works), you must remove those references first.
*
*
*
* If you get the ResourceInUseException
exception, it provides an example reference that shows where
* the intent is referenced. To remove the reference to the intent, either update the bot or delete it. If you get
* the same exception when you attempt to delete the intent again, repeat until the intent has no references and the
* call to DeleteIntent
is successful.
*
*
*
* This operation requires permission for the lex:DeleteIntent
action.
*
*
* @param deleteIntentRequest
* @return A Java Future containing the result of the DeleteIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteIntent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIntentAsync(DeleteIntentRequest deleteIntentRequest);
/**
*
* Deletes all versions of the intent, including the $LATEST
version. To delete a specific version of
* the intent, use the DeleteIntentVersion operation.
*
*
* You can delete a version of an intent only if it is not referenced. To delete an intent that is referred to in
* one or more bots (see how-it-works), you must remove those references first.
*
*
*
* If you get the ResourceInUseException
exception, it provides an example reference that shows where
* the intent is referenced. To remove the reference to the intent, either update the bot or delete it. If you get
* the same exception when you attempt to delete the intent again, repeat until the intent has no references and the
* call to DeleteIntent
is successful.
*
*
*
* This operation requires permission for the lex:DeleteIntent
action.
*
*
* @param deleteIntentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteIntent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteIntentAsync(DeleteIntentRequest deleteIntentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specific version of an intent. To delete all versions of a intent, use the DeleteIntent
* operation.
*
*
* This operation requires permissions for the lex:DeleteIntentVersion
action.
*
*
* @param deleteIntentVersionRequest
* @return A Java Future containing the result of the DeleteIntentVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteIntentVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteIntentVersionAsync(DeleteIntentVersionRequest deleteIntentVersionRequest);
/**
*
* Deletes a specific version of an intent. To delete all versions of a intent, use the DeleteIntent
* operation.
*
*
* This operation requires permissions for the lex:DeleteIntentVersion
action.
*
*
* @param deleteIntentVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIntentVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteIntentVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteIntentVersionAsync(DeleteIntentVersionRequest deleteIntentVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes all versions of the slot type, including the $LATEST
version. To delete a specific version
* of the slot type, use the DeleteSlotTypeVersion operation.
*
*
* You can delete a version of a slot type only if it is not referenced. To delete a slot type that is referred to
* in one or more intents, you must remove those references first.
*
*
*
* If you get the ResourceInUseException
exception, the exception provides an example reference that
* shows the intent where the slot type is referenced. To remove the reference to the slot type, either update the
* intent or delete it. If you get the same exception when you attempt to delete the slot type again, repeat until
* the slot type has no references and the DeleteSlotType
call is successful.
*
*
*
* This operation requires permission for the lex:DeleteSlotType
action.
*
*
* @param deleteSlotTypeRequest
* @return A Java Future containing the result of the DeleteSlotType operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteSlotType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSlotTypeAsync(DeleteSlotTypeRequest deleteSlotTypeRequest);
/**
*
* Deletes all versions of the slot type, including the $LATEST
version. To delete a specific version
* of the slot type, use the DeleteSlotTypeVersion operation.
*
*
* You can delete a version of a slot type only if it is not referenced. To delete a slot type that is referred to
* in one or more intents, you must remove those references first.
*
*
*
* If you get the ResourceInUseException
exception, the exception provides an example reference that
* shows the intent where the slot type is referenced. To remove the reference to the slot type, either update the
* intent or delete it. If you get the same exception when you attempt to delete the slot type again, repeat until
* the slot type has no references and the DeleteSlotType
call is successful.
*
*
*
* This operation requires permission for the lex:DeleteSlotType
action.
*
*
* @param deleteSlotTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSlotType operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteSlotType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSlotTypeAsync(DeleteSlotTypeRequest deleteSlotTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specific version of a slot type. To delete all versions of a slot type, use the DeleteSlotType
* operation.
*
*
* This operation requires permissions for the lex:DeleteSlotTypeVersion
action.
*
*
* @param deleteSlotTypeVersionRequest
* @return A Java Future containing the result of the DeleteSlotTypeVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteSlotTypeVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlotTypeVersionAsync(DeleteSlotTypeVersionRequest deleteSlotTypeVersionRequest);
/**
*
* Deletes a specific version of a slot type. To delete all versions of a slot type, use the DeleteSlotType
* operation.
*
*
* This operation requires permissions for the lex:DeleteSlotTypeVersion
action.
*
*
* @param deleteSlotTypeVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSlotTypeVersion operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteSlotTypeVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlotTypeVersionAsync(DeleteSlotTypeVersionRequest deleteSlotTypeVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes stored utterances.
*
*
* Amazon Lex stores the utterances that users send to your bot unless the childDirected
field in the
* bot is set to true
. Utterances are stored for 15 days for use with the GetUtterancesView
* operation, and then stored indefinately for use in improving the ability of your bot to respond to user input.
*
*
* Use the DeleteStoredUtterances
operation to manually delete stored utterances for a specific user.
*
*
* This operation requires permissions for the lex:DeleteUtterances
action.
*
*
* @param deleteUtterancesRequest
* @return A Java Future containing the result of the DeleteUtterances operation returned by the service.
* @sample AmazonLexModelBuildingAsync.DeleteUtterances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteUtterancesAsync(DeleteUtterancesRequest deleteUtterancesRequest);
/**
*
* Deletes stored utterances.
*
*
* Amazon Lex stores the utterances that users send to your bot unless the childDirected
field in the
* bot is set to true
. Utterances are stored for 15 days for use with the GetUtterancesView
* operation, and then stored indefinately for use in improving the ability of your bot to respond to user input.
*
*
* Use the DeleteStoredUtterances
operation to manually delete stored utterances for a specific user.
*
*
* This operation requires permissions for the lex:DeleteUtterances
action.
*
*
* @param deleteUtterancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUtterances operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.DeleteUtterances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteUtterancesAsync(DeleteUtterancesRequest deleteUtterancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata information for a specific bot. You must provide the bot name and the bot version or alias.
*
*
* The GetBot operation requires permissions for the lex:GetBot
action.
*
*
* @param getBotRequest
* @return A Java Future containing the result of the GetBot operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAsync(GetBotRequest getBotRequest);
/**
*
* Returns metadata information for a specific bot. You must provide the bot name and the bot version or alias.
*
*
* The GetBot operation requires permissions for the lex:GetBot
action.
*
*
* @param getBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBot operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAsync(GetBotRequest getBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about an Amazon Lex bot alias. For more information about aliases, see
* versioning-aliases.
*
*
* This operation requires permissions for the lex:GetBotAlias
action.
*
*
* @param getBotAliasRequest
* @return A Java Future containing the result of the GetBotAlias operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBotAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAliasAsync(GetBotAliasRequest getBotAliasRequest);
/**
*
* Returns information about an Amazon Lex bot alias. For more information about aliases, see
* versioning-aliases.
*
*
* This operation requires permissions for the lex:GetBotAlias
action.
*
*
* @param getBotAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBotAlias operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBotAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAliasAsync(GetBotAliasRequest getBotAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of aliases for a specified Amazon Lex bot.
*
*
* This operation requires permissions for the lex:GetBotAliases
action.
*
*
* @param getBotAliasesRequest
* @return A Java Future containing the result of the GetBotAliases operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBotAliases
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAliasesAsync(GetBotAliasesRequest getBotAliasesRequest);
/**
*
* Returns a list of aliases for a specified Amazon Lex bot.
*
*
* This operation requires permissions for the lex:GetBotAliases
action.
*
*
* @param getBotAliasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBotAliases operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBotAliases
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAliasesAsync(GetBotAliasesRequest getBotAliasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the association between an Amazon Lex bot and a messaging platform.
*
*
* This operation requires permissions for the lex:GetBotChannelAssociation
action.
*
*
* @param getBotChannelAssociationRequest
* @return A Java Future containing the result of the GetBotChannelAssociation operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBotChannelAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getBotChannelAssociationAsync(GetBotChannelAssociationRequest getBotChannelAssociationRequest);
/**
*
* Returns information about the association between an Amazon Lex bot and a messaging platform.
*
*
* This operation requires permissions for the lex:GetBotChannelAssociation
action.
*
*
* @param getBotChannelAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBotChannelAssociation operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBotChannelAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getBotChannelAssociationAsync(GetBotChannelAssociationRequest getBotChannelAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all of the channels associated with the specified bot.
*
*
* The GetBotChannelAssociations
operation requires permissions for the
* lex:GetBotChannelAssociations
action.
*
*
* @param getBotChannelAssociationsRequest
* @return A Java Future containing the result of the GetBotChannelAssociations operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBotChannelAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future getBotChannelAssociationsAsync(
GetBotChannelAssociationsRequest getBotChannelAssociationsRequest);
/**
*
* Returns a list of all of the channels associated with the specified bot.
*
*
* The GetBotChannelAssociations
operation requires permissions for the
* lex:GetBotChannelAssociations
action.
*
*
* @param getBotChannelAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBotChannelAssociations operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBotChannelAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future getBotChannelAssociationsAsync(
GetBotChannelAssociationsRequest getBotChannelAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about all of the versions of a bot.
*
*
* The GetBotVersions
operation returns a BotMetadata
object for each version of a bot.
* For example, if a bot has three numbered versions, the GetBotVersions
operation returns four
* BotMetadata
objects in the response, one for each numbered version and one for the
* $LATEST
version.
*
*
* The GetBotVersions
operation always returns at least one version, the $LATEST
version.
*
*
* This operation requires permissions for the lex:GetBotVersions
action.
*
*
* @param getBotVersionsRequest
* @return A Java Future containing the result of the GetBotVersions operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBotVersions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotVersionsAsync(GetBotVersionsRequest getBotVersionsRequest);
/**
*
* Gets information about all of the versions of a bot.
*
*
* The GetBotVersions
operation returns a BotMetadata
object for each version of a bot.
* For example, if a bot has three numbered versions, the GetBotVersions
operation returns four
* BotMetadata
objects in the response, one for each numbered version and one for the
* $LATEST
version.
*
*
* The GetBotVersions
operation always returns at least one version, the $LATEST
version.
*
*
* This operation requires permissions for the lex:GetBotVersions
action.
*
*
* @param getBotVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBotVersions operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBotVersions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotVersionsAsync(GetBotVersionsRequest getBotVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns bot information as follows:
*
*
* -
*
* If you provide the nameContains
field, the response includes information for the
* $LATEST
version of all bots whose name contains the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, the operation returns information about the
* $LATEST
version of all of your bots.
*
*
*
*
* This operation requires permission for the lex:GetBots
action.
*
*
* @param getBotsRequest
* @return A Java Future containing the result of the GetBots operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotsAsync(GetBotsRequest getBotsRequest);
/**
*
* Returns bot information as follows:
*
*
* -
*
* If you provide the nameContains
field, the response includes information for the
* $LATEST
version of all bots whose name contains the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, the operation returns information about the
* $LATEST
version of all of your bots.
*
*
*
*
* This operation requires permission for the lex:GetBots
action.
*
*
* @param getBotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBots operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotsAsync(GetBotsRequest getBotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a built-in intent.
*
*
* This operation requires permission for the lex:GetBuiltinIntent
action.
*
*
* @param getBuiltinIntentRequest
* @return A Java Future containing the result of the GetBuiltinIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBuiltinIntent
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBuiltinIntentAsync(GetBuiltinIntentRequest getBuiltinIntentRequest);
/**
*
* Returns information about a built-in intent.
*
*
* This operation requires permission for the lex:GetBuiltinIntent
action.
*
*
* @param getBuiltinIntentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBuiltinIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBuiltinIntent
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBuiltinIntentAsync(GetBuiltinIntentRequest getBuiltinIntentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of built-in intents that meet the specified criteria.
*
*
* This operation requires permission for the lex:GetBuiltinIntents
action.
*
*
* @param getBuiltinIntentsRequest
* @return A Java Future containing the result of the GetBuiltinIntents operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBuiltinIntents
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBuiltinIntentsAsync(GetBuiltinIntentsRequest getBuiltinIntentsRequest);
/**
*
* Gets a list of built-in intents that meet the specified criteria.
*
*
* This operation requires permission for the lex:GetBuiltinIntents
action.
*
*
* @param getBuiltinIntentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBuiltinIntents operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBuiltinIntents
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBuiltinIntentsAsync(GetBuiltinIntentsRequest getBuiltinIntentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of built-in slot types that meet the specified criteria.
*
*
* For a list of built-in slot types, see Slot Type Reference in the Alexa Skills Kit.
*
*
* This operation requires permission for the lex:GetBuiltInSlotTypes
action.
*
*
* @param getBuiltinSlotTypesRequest
* @return A Java Future containing the result of the GetBuiltinSlotTypes operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetBuiltinSlotTypes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBuiltinSlotTypesAsync(GetBuiltinSlotTypesRequest getBuiltinSlotTypesRequest);
/**
*
* Gets a list of built-in slot types that meet the specified criteria.
*
*
* For a list of built-in slot types, see Slot Type Reference in the Alexa Skills Kit.
*
*
* This operation requires permission for the lex:GetBuiltInSlotTypes
action.
*
*
* @param getBuiltinSlotTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBuiltinSlotTypes operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetBuiltinSlotTypes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getBuiltinSlotTypesAsync(GetBuiltinSlotTypesRequest getBuiltinSlotTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about an intent. In addition to the intent name, you must specify the intent version.
*
*
* This operation requires permissions to perform the lex:GetIntent
action.
*
*
* @param getIntentRequest
* @return A Java Future containing the result of the GetIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetIntent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getIntentAsync(GetIntentRequest getIntentRequest);
/**
*
* Returns information about an intent. In addition to the intent name, you must specify the intent version.
*
*
* This operation requires permissions to perform the lex:GetIntent
action.
*
*
* @param getIntentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetIntent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getIntentAsync(GetIntentRequest getIntentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about all of the versions of an intent.
*
*
* The GetIntentVersions
operation returns an IntentMetadata
object for each version of an
* intent. For example, if an intent has three numbered versions, the GetIntentVersions
operation
* returns four IntentMetadata
objects in the response, one for each numbered version and one for the
* $LATEST
version.
*
*
* The GetIntentVersions
operation always returns at least one version, the $LATEST
* version.
*
*
* This operation requires permissions for the lex:GetIntentVersions
action.
*
*
* @param getIntentVersionsRequest
* @return A Java Future containing the result of the GetIntentVersions operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetIntentVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIntentVersionsAsync(GetIntentVersionsRequest getIntentVersionsRequest);
/**
*
* Gets information about all of the versions of an intent.
*
*
* The GetIntentVersions
operation returns an IntentMetadata
object for each version of an
* intent. For example, if an intent has three numbered versions, the GetIntentVersions
operation
* returns four IntentMetadata
objects in the response, one for each numbered version and one for the
* $LATEST
version.
*
*
* The GetIntentVersions
operation always returns at least one version, the $LATEST
* version.
*
*
* This operation requires permissions for the lex:GetIntentVersions
action.
*
*
* @param getIntentVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIntentVersions operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetIntentVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIntentVersionsAsync(GetIntentVersionsRequest getIntentVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns intent information as follows:
*
*
* -
*
* If you specify the nameContains
field, returns the $LATEST
version of all intents that
* contain the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, returns information about the $LATEST
* version of all intents.
*
*
*
*
* The operation requires permission for the lex:GetIntents
action.
*
*
* @param getIntentsRequest
* @return A Java Future containing the result of the GetIntents operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetIntents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getIntentsAsync(GetIntentsRequest getIntentsRequest);
/**
*
* Returns intent information as follows:
*
*
* -
*
* If you specify the nameContains
field, returns the $LATEST
version of all intents that
* contain the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, returns information about the $LATEST
* version of all intents.
*
*
*
*
* The operation requires permission for the lex:GetIntents
action.
*
*
* @param getIntentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIntents operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetIntents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getIntentsAsync(GetIntentsRequest getIntentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a specific version of a slot type. In addition to specifying the slot type name, you
* must specify the slot type version.
*
*
* This operation requires permissions for the lex:GetSlotType
action.
*
*
* @param getSlotTypeRequest
* @return A Java Future containing the result of the GetSlotType operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetSlotType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSlotTypeAsync(GetSlotTypeRequest getSlotTypeRequest);
/**
*
* Returns information about a specific version of a slot type. In addition to specifying the slot type name, you
* must specify the slot type version.
*
*
* This operation requires permissions for the lex:GetSlotType
action.
*
*
* @param getSlotTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSlotType operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetSlotType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSlotTypeAsync(GetSlotTypeRequest getSlotTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about all versions of a slot type.
*
*
* The GetSlotTypeVersions
operation returns a SlotTypeMetadata
object for each version of
* a slot type. For example, if a slot type has three numbered versions, the GetSlotTypeVersions
* operation returns four SlotTypeMetadata
objects in the response, one for each numbered version and
* one for the $LATEST
version.
*
*
* The GetSlotTypeVersions
operation always returns at least one version, the $LATEST
* version.
*
*
* This operation requires permissions for the lex:GetSlotTypeVersions
action.
*
*
* @param getSlotTypeVersionsRequest
* @return A Java Future containing the result of the GetSlotTypeVersions operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetSlotTypeVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSlotTypeVersionsAsync(GetSlotTypeVersionsRequest getSlotTypeVersionsRequest);
/**
*
* Gets information about all versions of a slot type.
*
*
* The GetSlotTypeVersions
operation returns a SlotTypeMetadata
object for each version of
* a slot type. For example, if a slot type has three numbered versions, the GetSlotTypeVersions
* operation returns four SlotTypeMetadata
objects in the response, one for each numbered version and
* one for the $LATEST
version.
*
*
* The GetSlotTypeVersions
operation always returns at least one version, the $LATEST
* version.
*
*
* This operation requires permissions for the lex:GetSlotTypeVersions
action.
*
*
* @param getSlotTypeVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSlotTypeVersions operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetSlotTypeVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSlotTypeVersionsAsync(GetSlotTypeVersionsRequest getSlotTypeVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns slot type information as follows:
*
*
* -
*
* If you specify the nameContains
field, returns the $LATEST
version of all slot types
* that contain the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, returns information about the $LATEST
* version of all slot types.
*
*
*
*
* The operation requires permission for the lex:GetSlotTypes
action.
*
*
* @param getSlotTypesRequest
* @return A Java Future containing the result of the GetSlotTypes operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetSlotTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSlotTypesAsync(GetSlotTypesRequest getSlotTypesRequest);
/**
*
* Returns slot type information as follows:
*
*
* -
*
* If you specify the nameContains
field, returns the $LATEST
version of all slot types
* that contain the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, returns information about the $LATEST
* version of all slot types.
*
*
*
*
* The operation requires permission for the lex:GetSlotTypes
action.
*
*
* @param getSlotTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSlotTypes operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetSlotTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSlotTypesAsync(GetSlotTypesRequest getSlotTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use the GetUtterancesView
operation to get information about the utterances that your users have
* made to your bot. You can use this list to tune the utterances that your bot responds to.
*
*
* For example, say that you have created a bot to order flowers. After your users have used your bot for a while,
* use the GetUtterancesView
operation to see the requests that they have made and whether they have
* been successful. You might find that the utterance "I want flowers" is not being recognized. You could add this
* utterance to the OrderFlowers
intent so that your bot recognizes that utterance.
*
*
* After you publish a new version of a bot, you can get information about the old version and the new so that you
* can compare the performance across the two versions.
*
*
* Data is available for the last 15 days. You can request information for up to 5 versions in each request. The
* response contains information about a maximum of 100 utterances for each version.
*
*
* If the bot's childDirected
field is set to true
, utterances for the bot are not stored
* and cannot be retrieved with the GetUtterancesView
operation. For more information, see
* PutBot.
*
*
* This operation requires permissions for the lex:GetUtterancesView
action.
*
*
* @param getUtterancesViewRequest
* @return A Java Future containing the result of the GetUtterancesView operation returned by the service.
* @sample AmazonLexModelBuildingAsync.GetUtterancesView
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getUtterancesViewAsync(GetUtterancesViewRequest getUtterancesViewRequest);
/**
*
* Use the GetUtterancesView
operation to get information about the utterances that your users have
* made to your bot. You can use this list to tune the utterances that your bot responds to.
*
*
* For example, say that you have created a bot to order flowers. After your users have used your bot for a while,
* use the GetUtterancesView
operation to see the requests that they have made and whether they have
* been successful. You might find that the utterance "I want flowers" is not being recognized. You could add this
* utterance to the OrderFlowers
intent so that your bot recognizes that utterance.
*
*
* After you publish a new version of a bot, you can get information about the old version and the new so that you
* can compare the performance across the two versions.
*
*
* Data is available for the last 15 days. You can request information for up to 5 versions in each request. The
* response contains information about a maximum of 100 utterances for each version.
*
*
* If the bot's childDirected
field is set to true
, utterances for the bot are not stored
* and cannot be retrieved with the GetUtterancesView
operation. For more information, see
* PutBot.
*
*
* This operation requires permissions for the lex:GetUtterancesView
action.
*
*
* @param getUtterancesViewRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUtterancesView operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.GetUtterancesView
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getUtterancesViewAsync(GetUtterancesViewRequest getUtterancesViewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Lex conversational bot or replaces an existing bot. When you create or update a bot you are
* only required to specify a name. You can use this to add intents later, or to remove intents from an existing
* bot. When you create a bot with a name only, the bot is created or updated but Amazon Lex returns the
*
response FAILED
. You can build the bot after you add one or more intents. For more
* information about Amazon Lex bots, see how-it-works.
*
*
* If you specify the name of an existing bot, the fields in the request replace the existing values in the
* $LATEST
version of the bot. Amazon Lex removes any fields that you don't provide values for in the
* request, except for the idleTTLInSeconds
and privacySettings
fields, which are set to
* their default values. If you don't specify values for required fields, Amazon Lex throws an exception.
*
*
* This operation requires permissions for the lex:PutBot
action. For more information, see
* auth-and-access-control.
*
*
* @param putBotRequest
* @return A Java Future containing the result of the PutBot operation returned by the service.
* @sample AmazonLexModelBuildingAsync.PutBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putBotAsync(PutBotRequest putBotRequest);
/**
*
* Creates an Amazon Lex conversational bot or replaces an existing bot. When you create or update a bot you are
* only required to specify a name. You can use this to add intents later, or to remove intents from an existing
* bot. When you create a bot with a name only, the bot is created or updated but Amazon Lex returns the
*
response FAILED
. You can build the bot after you add one or more intents. For more
* information about Amazon Lex bots, see how-it-works.
*
*
* If you specify the name of an existing bot, the fields in the request replace the existing values in the
* $LATEST
version of the bot. Amazon Lex removes any fields that you don't provide values for in the
* request, except for the idleTTLInSeconds
and privacySettings
fields, which are set to
* their default values. If you don't specify values for required fields, Amazon Lex throws an exception.
*
*
* This operation requires permissions for the lex:PutBot
action. For more information, see
* auth-and-access-control.
*
*
* @param putBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutBot operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.PutBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putBotAsync(PutBotRequest putBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an alias for the specified version of the bot or replaces an alias for the specified bot. To change the
* version of the bot that the alias points to, replace the alias. For more information about aliases, see
* versioning-aliases.
*
*
* This operation requires permissions for the lex:PutBotAlias
action.
*
*
* @param putBotAliasRequest
* @return A Java Future containing the result of the PutBotAlias operation returned by the service.
* @sample AmazonLexModelBuildingAsync.PutBotAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putBotAliasAsync(PutBotAliasRequest putBotAliasRequest);
/**
*
* Creates an alias for the specified version of the bot or replaces an alias for the specified bot. To change the
* version of the bot that the alias points to, replace the alias. For more information about aliases, see
* versioning-aliases.
*
*
* This operation requires permissions for the lex:PutBotAlias
action.
*
*
* @param putBotAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutBotAlias operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.PutBotAlias
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putBotAliasAsync(PutBotAliasRequest putBotAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an intent or replaces an existing intent.
*
*
* To define the interaction between the user and your bot, you use one or more intents. For a pizza ordering bot,
* for example, you would create an OrderPizza
intent.
*
*
* To create an intent or replace an existing intent, you must provide the following:
*
*
* -
*
* Intent name. For example, OrderPizza
.
*
*
* -
*
* Sample utterances. For example, "Can I order a pizza, please." and "I want to order a pizza."
*
*
* -
*
* Information to be gathered. You specify slot types for the information that your bot will request from the user.
* You can specify standard slot types, such as a date or a time, or custom slot types such as the size and crust of
* a pizza.
*
*
* -
*
* How the intent will be fulfilled. You can provide a Lambda function or configure the intent to return the intent
* information to the client application. If you use a Lambda function, when all of the intent information is
* available, Amazon Lex invokes your Lambda function. If you configure your intent to return the intent information
* to the client application.
*
*
*
*
* You can specify other optional information in the request, such as:
*
*
* -
*
* A confirmation prompt to ask the user to confirm an intent. For example, "Shall I order your pizza?"
*
*
* -
*
* A conclusion statement to send to the user after the intent has been fulfilled. For example,
* "I placed your pizza order."
*
*
* -
*
* A follow-up prompt that asks the user for additional activity. For example, asking
* "Do you want to order a drink with your pizza?"
*
*
*
*
* If you specify an existing intent name to update the intent, Amazon Lex replaces the values in the
* $LATEST
version of the slot type with the values in the request. Amazon Lex removes fields that you
* don't provide in the request. If you don't specify the required fields, Amazon Lex throws an exception.
*
*
* For more information, see how-it-works.
*
*
* This operation requires permissions for the lex:PutIntent
action.
*
*
* @param putIntentRequest
* @return A Java Future containing the result of the PutIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsync.PutIntent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putIntentAsync(PutIntentRequest putIntentRequest);
/**
*
* Creates an intent or replaces an existing intent.
*
*
* To define the interaction between the user and your bot, you use one or more intents. For a pizza ordering bot,
* for example, you would create an OrderPizza
intent.
*
*
* To create an intent or replace an existing intent, you must provide the following:
*
*
* -
*
* Intent name. For example, OrderPizza
.
*
*
* -
*
* Sample utterances. For example, "Can I order a pizza, please." and "I want to order a pizza."
*
*
* -
*
* Information to be gathered. You specify slot types for the information that your bot will request from the user.
* You can specify standard slot types, such as a date or a time, or custom slot types such as the size and crust of
* a pizza.
*
*
* -
*
* How the intent will be fulfilled. You can provide a Lambda function or configure the intent to return the intent
* information to the client application. If you use a Lambda function, when all of the intent information is
* available, Amazon Lex invokes your Lambda function. If you configure your intent to return the intent information
* to the client application.
*
*
*
*
* You can specify other optional information in the request, such as:
*
*
* -
*
* A confirmation prompt to ask the user to confirm an intent. For example, "Shall I order your pizza?"
*
*
* -
*
* A conclusion statement to send to the user after the intent has been fulfilled. For example,
* "I placed your pizza order."
*
*
* -
*
* A follow-up prompt that asks the user for additional activity. For example, asking
* "Do you want to order a drink with your pizza?"
*
*
*
*
* If you specify an existing intent name to update the intent, Amazon Lex replaces the values in the
* $LATEST
version of the slot type with the values in the request. Amazon Lex removes fields that you
* don't provide in the request. If you don't specify the required fields, Amazon Lex throws an exception.
*
*
* For more information, see how-it-works.
*
*
* This operation requires permissions for the lex:PutIntent
action.
*
*
* @param putIntentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutIntent operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.PutIntent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putIntentAsync(PutIntentRequest putIntentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom slot type or replaces an existing custom slot type.
*
*
* To create a custom slot type, specify a name for the slot type and a set of enumeration values, which are the
* values that a slot of this type can assume. For more information, see how-it-works.
*
*
* If you specify the name of an existing slot type, the fields in the request replace the existing values in the
* $LATEST
version of the slot type. Amazon Lex removes the fields that you don't provide in the
* request. If you don't specify required fields, Amazon Lex throws an exception.
*
*
* This operation requires permissions for the lex:PutSlotType
action.
*
*
* @param putSlotTypeRequest
* @return A Java Future containing the result of the PutSlotType operation returned by the service.
* @sample AmazonLexModelBuildingAsync.PutSlotType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putSlotTypeAsync(PutSlotTypeRequest putSlotTypeRequest);
/**
*
* Creates a custom slot type or replaces an existing custom slot type.
*
*
* To create a custom slot type, specify a name for the slot type and a set of enumeration values, which are the
* values that a slot of this type can assume. For more information, see how-it-works.
*
*
* If you specify the name of an existing slot type, the fields in the request replace the existing values in the
* $LATEST
version of the slot type. Amazon Lex removes the fields that you don't provide in the
* request. If you don't specify required fields, Amazon Lex throws an exception.
*
*
* This operation requires permissions for the lex:PutSlotType
action.
*
*
* @param putSlotTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutSlotType operation returned by the service.
* @sample AmazonLexModelBuildingAsyncHandler.PutSlotType
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putSlotTypeAsync(PutSlotTypeRequest putSlotTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}