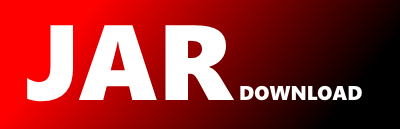
com.amazonaws.services.lexmodelbuilding.model.PutBotResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutBotResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the bot.
*
*/
private String name;
/**
*
* A description of the bot.
*
*/
private String description;
/**
*
* An array of Intent
objects. For more information, see PutBot.
*
*/
private java.util.List intents;
/**
*
* The prompts that Amazon Lex uses when it doesn't understand the user's intent. For more information, see
* PutBot.
*
*/
private Prompt clarificationPrompt;
/**
*
* The message that Amazon Lex uses to abort a conversation. For more information, see PutBot.
*
*/
private Statement abortStatement;
/**
*
* When you send a request to create a bot with processBehavior
set to BUILD
, Amazon Lex
* sets the status
response element to BUILDING
. After Amazon Lex builds the bot, it sets
* status
to READY
. If Amazon Lex can't build the bot, Amazon Lex sets status
* to FAILED
. Amazon Lex returns the reason for the failure in the failureReason
response
* element.
*
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
*
*/
private String status;
/**
*
* If status
is FAILED
, Amazon Lex provides the reason that it failed to build the bot.
*
*/
private String failureReason;
/**
*
* The date that the bot was updated. When you create a resource, the creation date and last updated date are the
* same.
*
*/
private java.util.Date lastUpdatedDate;
/**
*
* The date that the bot was created.
*
*/
private java.util.Date createdDate;
/**
*
* The maximum length of time that Amazon Lex retains the data gathered in a conversation. For more information, see
* PutBot.
*
*/
private Integer idleSessionTTLInSeconds;
/**
*
* The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information, see
* PutBot.
*
*/
private String voiceId;
/**
*
* Checksum of the bot that you created.
*
*/
private String checksum;
/**
*
* The version of the bot. For a new bot, the version is always $LATEST
.
*
*/
private String version;
/**
*
* The target locale for the bot.
*
*/
private String locale;
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*/
private Boolean childDirected;
/**
*
* The name of the bot.
*
*
* @param name
* The name of the bot.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the bot.
*
*
* @return The name of the bot.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the bot.
*
*
* @param name
* The name of the bot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the bot.
*
*
* @param description
* A description of the bot.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the bot.
*
*
* @return A description of the bot.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the bot.
*
*
* @param description
* A description of the bot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* An array of Intent
objects. For more information, see PutBot.
*
*
* @return An array of Intent
objects. For more information, see PutBot.
*/
public java.util.List getIntents() {
return intents;
}
/**
*
* An array of Intent
objects. For more information, see PutBot.
*
*
* @param intents
* An array of Intent
objects. For more information, see PutBot.
*/
public void setIntents(java.util.Collection intents) {
if (intents == null) {
this.intents = null;
return;
}
this.intents = new java.util.ArrayList(intents);
}
/**
*
* An array of Intent
objects. For more information, see PutBot.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIntents(java.util.Collection)} or {@link #withIntents(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param intents
* An array of Intent
objects. For more information, see PutBot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withIntents(Intent... intents) {
if (this.intents == null) {
setIntents(new java.util.ArrayList(intents.length));
}
for (Intent ele : intents) {
this.intents.add(ele);
}
return this;
}
/**
*
* An array of Intent
objects. For more information, see PutBot.
*
*
* @param intents
* An array of Intent
objects. For more information, see PutBot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withIntents(java.util.Collection intents) {
setIntents(intents);
return this;
}
/**
*
* The prompts that Amazon Lex uses when it doesn't understand the user's intent. For more information, see
* PutBot.
*
*
* @param clarificationPrompt
* The prompts that Amazon Lex uses when it doesn't understand the user's intent. For more information, see
* PutBot.
*/
public void setClarificationPrompt(Prompt clarificationPrompt) {
this.clarificationPrompt = clarificationPrompt;
}
/**
*
* The prompts that Amazon Lex uses when it doesn't understand the user's intent. For more information, see
* PutBot.
*
*
* @return The prompts that Amazon Lex uses when it doesn't understand the user's intent. For more information, see
* PutBot.
*/
public Prompt getClarificationPrompt() {
return this.clarificationPrompt;
}
/**
*
* The prompts that Amazon Lex uses when it doesn't understand the user's intent. For more information, see
* PutBot.
*
*
* @param clarificationPrompt
* The prompts that Amazon Lex uses when it doesn't understand the user's intent. For more information, see
* PutBot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withClarificationPrompt(Prompt clarificationPrompt) {
setClarificationPrompt(clarificationPrompt);
return this;
}
/**
*
* The message that Amazon Lex uses to abort a conversation. For more information, see PutBot.
*
*
* @param abortStatement
* The message that Amazon Lex uses to abort a conversation. For more information, see PutBot.
*/
public void setAbortStatement(Statement abortStatement) {
this.abortStatement = abortStatement;
}
/**
*
* The message that Amazon Lex uses to abort a conversation. For more information, see PutBot.
*
*
* @return The message that Amazon Lex uses to abort a conversation. For more information, see PutBot.
*/
public Statement getAbortStatement() {
return this.abortStatement;
}
/**
*
* The message that Amazon Lex uses to abort a conversation. For more information, see PutBot.
*
*
* @param abortStatement
* The message that Amazon Lex uses to abort a conversation. For more information, see PutBot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withAbortStatement(Statement abortStatement) {
setAbortStatement(abortStatement);
return this;
}
/**
*
* When you send a request to create a bot with processBehavior
set to BUILD
, Amazon Lex
* sets the status
response element to BUILDING
. After Amazon Lex builds the bot, it sets
* status
to READY
. If Amazon Lex can't build the bot, Amazon Lex sets status
* to FAILED
. Amazon Lex returns the reason for the failure in the failureReason
response
* element.
*
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
*
*
* @param status
* When you send a request to create a bot with processBehavior
set to BUILD
,
* Amazon Lex sets the status
response element to BUILDING
. After Amazon Lex builds
* the bot, it sets status
to READY
. If Amazon Lex can't build the bot, Amazon Lex
* sets status
to FAILED
. Amazon Lex returns the reason for the failure in the
* failureReason
response element.
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
* @see Status
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* When you send a request to create a bot with processBehavior
set to BUILD
, Amazon Lex
* sets the status
response element to BUILDING
. After Amazon Lex builds the bot, it sets
* status
to READY
. If Amazon Lex can't build the bot, Amazon Lex sets status
* to FAILED
. Amazon Lex returns the reason for the failure in the failureReason
response
* element.
*
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
*
*
* @return When you send a request to create a bot with processBehavior
set to BUILD
,
* Amazon Lex sets the status
response element to BUILDING
. After Amazon Lex
* builds the bot, it sets status
to READY
. If Amazon Lex can't build the bot,
* Amazon Lex sets status
to FAILED
. Amazon Lex returns the reason for the failure
* in the failureReason
response element.
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
* @see Status
*/
public String getStatus() {
return this.status;
}
/**
*
* When you send a request to create a bot with processBehavior
set to BUILD
, Amazon Lex
* sets the status
response element to BUILDING
. After Amazon Lex builds the bot, it sets
* status
to READY
. If Amazon Lex can't build the bot, Amazon Lex sets status
* to FAILED
. Amazon Lex returns the reason for the failure in the failureReason
response
* element.
*
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
*
*
* @param status
* When you send a request to create a bot with processBehavior
set to BUILD
,
* Amazon Lex sets the status
response element to BUILDING
. After Amazon Lex builds
* the bot, it sets status
to READY
. If Amazon Lex can't build the bot, Amazon Lex
* sets status
to FAILED
. Amazon Lex returns the reason for the failure in the
* failureReason
response element.
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public PutBotResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* When you send a request to create a bot with processBehavior
set to BUILD
, Amazon Lex
* sets the status
response element to BUILDING
. After Amazon Lex builds the bot, it sets
* status
to READY
. If Amazon Lex can't build the bot, Amazon Lex sets status
* to FAILED
. Amazon Lex returns the reason for the failure in the failureReason
response
* element.
*
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
*
*
* @param status
* When you send a request to create a bot with processBehavior
set to BUILD
,
* Amazon Lex sets the status
response element to BUILDING
. After Amazon Lex builds
* the bot, it sets status
to READY
. If Amazon Lex can't build the bot, Amazon Lex
* sets status
to FAILED
. Amazon Lex returns the reason for the failure in the
* failureReason
response element.
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
* @see Status
*/
public void setStatus(Status status) {
withStatus(status);
}
/**
*
* When you send a request to create a bot with processBehavior
set to BUILD
, Amazon Lex
* sets the status
response element to BUILDING
. After Amazon Lex builds the bot, it sets
* status
to READY
. If Amazon Lex can't build the bot, Amazon Lex sets status
* to FAILED
. Amazon Lex returns the reason for the failure in the failureReason
response
* element.
*
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
*
*
* @param status
* When you send a request to create a bot with processBehavior
set to BUILD
,
* Amazon Lex sets the status
response element to BUILDING
. After Amazon Lex builds
* the bot, it sets status
to READY
. If Amazon Lex can't build the bot, Amazon Lex
* sets status
to FAILED
. Amazon Lex returns the reason for the failure in the
* failureReason
response element.
*
* When you set processBehavior
to SAVE
, Amazon Lex sets the status code to
* NOT BUILT
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public PutBotResult withStatus(Status status) {
this.status = status.toString();
return this;
}
/**
*
* If status
is FAILED
, Amazon Lex provides the reason that it failed to build the bot.
*
*
* @param failureReason
* If status
is FAILED
, Amazon Lex provides the reason that it failed to build the
* bot.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* If status
is FAILED
, Amazon Lex provides the reason that it failed to build the bot.
*
*
* @return If status
is FAILED
, Amazon Lex provides the reason that it failed to build the
* bot.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* If status
is FAILED
, Amazon Lex provides the reason that it failed to build the bot.
*
*
* @param failureReason
* If status
is FAILED
, Amazon Lex provides the reason that it failed to build the
* bot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
*
* The date that the bot was updated. When you create a resource, the creation date and last updated date are the
* same.
*
*
* @param lastUpdatedDate
* The date that the bot was updated. When you create a resource, the creation date and last updated date are
* the same.
*/
public void setLastUpdatedDate(java.util.Date lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
}
/**
*
* The date that the bot was updated. When you create a resource, the creation date and last updated date are the
* same.
*
*
* @return The date that the bot was updated. When you create a resource, the creation date and last updated date
* are the same.
*/
public java.util.Date getLastUpdatedDate() {
return this.lastUpdatedDate;
}
/**
*
* The date that the bot was updated. When you create a resource, the creation date and last updated date are the
* same.
*
*
* @param lastUpdatedDate
* The date that the bot was updated. When you create a resource, the creation date and last updated date are
* the same.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withLastUpdatedDate(java.util.Date lastUpdatedDate) {
setLastUpdatedDate(lastUpdatedDate);
return this;
}
/**
*
* The date that the bot was created.
*
*
* @param createdDate
* The date that the bot was created.
*/
public void setCreatedDate(java.util.Date createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date that the bot was created.
*
*
* @return The date that the bot was created.
*/
public java.util.Date getCreatedDate() {
return this.createdDate;
}
/**
*
* The date that the bot was created.
*
*
* @param createdDate
* The date that the bot was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withCreatedDate(java.util.Date createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
*
* The maximum length of time that Amazon Lex retains the data gathered in a conversation. For more information, see
* PutBot.
*
*
* @param idleSessionTTLInSeconds
* The maximum length of time that Amazon Lex retains the data gathered in a conversation. For more
* information, see PutBot.
*/
public void setIdleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
this.idleSessionTTLInSeconds = idleSessionTTLInSeconds;
}
/**
*
* The maximum length of time that Amazon Lex retains the data gathered in a conversation. For more information, see
* PutBot.
*
*
* @return The maximum length of time that Amazon Lex retains the data gathered in a conversation. For more
* information, see PutBot.
*/
public Integer getIdleSessionTTLInSeconds() {
return this.idleSessionTTLInSeconds;
}
/**
*
* The maximum length of time that Amazon Lex retains the data gathered in a conversation. For more information, see
* PutBot.
*
*
* @param idleSessionTTLInSeconds
* The maximum length of time that Amazon Lex retains the data gathered in a conversation. For more
* information, see PutBot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withIdleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
setIdleSessionTTLInSeconds(idleSessionTTLInSeconds);
return this;
}
/**
*
* The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information, see
* PutBot.
*
*
* @param voiceId
* The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information,
* see PutBot.
*/
public void setVoiceId(String voiceId) {
this.voiceId = voiceId;
}
/**
*
* The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information, see
* PutBot.
*
*
* @return The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information,
* see PutBot.
*/
public String getVoiceId() {
return this.voiceId;
}
/**
*
* The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information, see
* PutBot.
*
*
* @param voiceId
* The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information,
* see PutBot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withVoiceId(String voiceId) {
setVoiceId(voiceId);
return this;
}
/**
*
* Checksum of the bot that you created.
*
*
* @param checksum
* Checksum of the bot that you created.
*/
public void setChecksum(String checksum) {
this.checksum = checksum;
}
/**
*
* Checksum of the bot that you created.
*
*
* @return Checksum of the bot that you created.
*/
public String getChecksum() {
return this.checksum;
}
/**
*
* Checksum of the bot that you created.
*
*
* @param checksum
* Checksum of the bot that you created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withChecksum(String checksum) {
setChecksum(checksum);
return this;
}
/**
*
* The version of the bot. For a new bot, the version is always $LATEST
.
*
*
* @param version
* The version of the bot. For a new bot, the version is always $LATEST
.
*/
public void setVersion(String version) {
this.version = version;
}
/**
*
* The version of the bot. For a new bot, the version is always $LATEST
.
*
*
* @return The version of the bot. For a new bot, the version is always $LATEST
.
*/
public String getVersion() {
return this.version;
}
/**
*
* The version of the bot. For a new bot, the version is always $LATEST
.
*
*
* @param version
* The version of the bot. For a new bot, the version is always $LATEST
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withVersion(String version) {
setVersion(version);
return this;
}
/**
*
* The target locale for the bot.
*
*
* @param locale
* The target locale for the bot.
* @see Locale
*/
public void setLocale(String locale) {
this.locale = locale;
}
/**
*
* The target locale for the bot.
*
*
* @return The target locale for the bot.
* @see Locale
*/
public String getLocale() {
return this.locale;
}
/**
*
* The target locale for the bot.
*
*
* @param locale
* The target locale for the bot.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Locale
*/
public PutBotResult withLocale(String locale) {
setLocale(locale);
return this;
}
/**
*
* The target locale for the bot.
*
*
* @param locale
* The target locale for the bot.
* @see Locale
*/
public void setLocale(Locale locale) {
withLocale(locale);
}
/**
*
* The target locale for the bot.
*
*
* @param locale
* The target locale for the bot.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Locale
*/
public PutBotResult withLocale(Locale locale) {
this.locale = locale.toString();
return this;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @param childDirected
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program, or
* other application that is directed or targeted, in whole or in part, to children under age 13 and subject
* to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or
* in part, to children under age 13, you must obtain any required verifiable parental consent under COPPA.
* For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public void setChildDirected(Boolean childDirected) {
this.childDirected = childDirected;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @return For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program,
* or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole
* or in part, to children under age 13, you must obtain any required verifiable parental consent under
* COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public Boolean getChildDirected() {
return this.childDirected;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @param childDirected
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program, or
* other application that is directed or targeted, in whole or in part, to children under age 13 and subject
* to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or
* in part, to children under age 13, you must obtain any required verifiable parental consent under COPPA.
* For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotResult withChildDirected(Boolean childDirected) {
setChildDirected(childDirected);
return this;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @return For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program,
* or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole
* or in part, to children under age 13, you must obtain any required verifiable parental consent under
* COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public Boolean isChildDirected() {
return this.childDirected;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getIntents() != null)
sb.append("Intents: ").append(getIntents()).append(",");
if (getClarificationPrompt() != null)
sb.append("ClarificationPrompt: ").append(getClarificationPrompt()).append(",");
if (getAbortStatement() != null)
sb.append("AbortStatement: ").append(getAbortStatement()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason()).append(",");
if (getLastUpdatedDate() != null)
sb.append("LastUpdatedDate: ").append(getLastUpdatedDate()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate()).append(",");
if (getIdleSessionTTLInSeconds() != null)
sb.append("IdleSessionTTLInSeconds: ").append(getIdleSessionTTLInSeconds()).append(",");
if (getVoiceId() != null)
sb.append("VoiceId: ").append(getVoiceId()).append(",");
if (getChecksum() != null)
sb.append("Checksum: ").append(getChecksum()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion()).append(",");
if (getLocale() != null)
sb.append("Locale: ").append(getLocale()).append(",");
if (getChildDirected() != null)
sb.append("ChildDirected: ").append(getChildDirected());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutBotResult == false)
return false;
PutBotResult other = (PutBotResult) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getIntents() == null ^ this.getIntents() == null)
return false;
if (other.getIntents() != null && other.getIntents().equals(this.getIntents()) == false)
return false;
if (other.getClarificationPrompt() == null ^ this.getClarificationPrompt() == null)
return false;
if (other.getClarificationPrompt() != null && other.getClarificationPrompt().equals(this.getClarificationPrompt()) == false)
return false;
if (other.getAbortStatement() == null ^ this.getAbortStatement() == null)
return false;
if (other.getAbortStatement() != null && other.getAbortStatement().equals(this.getAbortStatement()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
if (other.getLastUpdatedDate() == null ^ this.getLastUpdatedDate() == null)
return false;
if (other.getLastUpdatedDate() != null && other.getLastUpdatedDate().equals(this.getLastUpdatedDate()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
if (other.getIdleSessionTTLInSeconds() == null ^ this.getIdleSessionTTLInSeconds() == null)
return false;
if (other.getIdleSessionTTLInSeconds() != null && other.getIdleSessionTTLInSeconds().equals(this.getIdleSessionTTLInSeconds()) == false)
return false;
if (other.getVoiceId() == null ^ this.getVoiceId() == null)
return false;
if (other.getVoiceId() != null && other.getVoiceId().equals(this.getVoiceId()) == false)
return false;
if (other.getChecksum() == null ^ this.getChecksum() == null)
return false;
if (other.getChecksum() != null && other.getChecksum().equals(this.getChecksum()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getLocale() == null ^ this.getLocale() == null)
return false;
if (other.getLocale() != null && other.getLocale().equals(this.getLocale()) == false)
return false;
if (other.getChildDirected() == null ^ this.getChildDirected() == null)
return false;
if (other.getChildDirected() != null && other.getChildDirected().equals(this.getChildDirected()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getIntents() == null) ? 0 : getIntents().hashCode());
hashCode = prime * hashCode + ((getClarificationPrompt() == null) ? 0 : getClarificationPrompt().hashCode());
hashCode = prime * hashCode + ((getAbortStatement() == null) ? 0 : getAbortStatement().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedDate() == null) ? 0 : getLastUpdatedDate().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
hashCode = prime * hashCode + ((getIdleSessionTTLInSeconds() == null) ? 0 : getIdleSessionTTLInSeconds().hashCode());
hashCode = prime * hashCode + ((getVoiceId() == null) ? 0 : getVoiceId().hashCode());
hashCode = prime * hashCode + ((getChecksum() == null) ? 0 : getChecksum().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode + ((getLocale() == null) ? 0 : getLocale().hashCode());
hashCode = prime * hashCode + ((getChildDirected() == null) ? 0 : getChildDirected().hashCode());
return hashCode;
}
@Override
public PutBotResult clone() {
try {
return (PutBotResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}