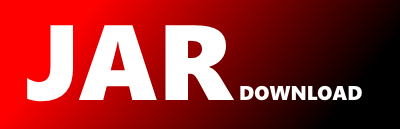
com.amazonaws.services.lexmodelbuilding.model.PutIntentRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutIntentRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the intent. The name is not case sensitive.
*
*
* The name can't match a built-in intent name, or a built-in intent name with "AMAZON." removed. For example,
* because there is a built-in intent called AMAZON.HelpIntent
, you can't create a custom intent called
* HelpIntent
.
*
*
* For a list of built-in intents, see Standard Built-in Intents in the Alexa Skills Kit.
*
*/
private String name;
/**
*
* A description of the intent.
*
*/
private String description;
/**
*
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts defined
* in the slots. For more information, see how-it-works.
*
*/
private java.util.List slots;
/**
*
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
*
* In each utterance, a slot name is enclosed in curly braces.
*
*/
private java.util.List sampleUtterances;
/**
*
* Prompts the user to confirm the intent. This question should have a yes or no answer.
*
*
* Amazon Lex uses this prompt to ensure that the user acknowledges that the intent is ready for fulfillment. For
* example, with the OrderPizza
intent, you might want to confirm that the order is correct before
* placing it. For other intents, such as intents that simply respond to user questions, you might not need to ask
* the user for confirmation before providing the information.
*
*
*
* You you must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*
*/
private Prompt confirmationPrompt;
/**
*
* When the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
*
* You must provide both the rejectionStatement
and the confirmationPrompt
, or neither.
*
*
*/
private Statement rejectionStatement;
/**
*
* Amazon Lex uses this prompt to solicit additional activity after fulfilling an intent. For example, after the
* OrderPizza
intent is fulfilled, you might prompt the user to order a drink.
*
*
* The action that Amazon Lex takes depends on the user's response, as follows:
*
*
* -
*
* If the user says "Yes" it responds with the clarification prompt that is configured for the bot.
*
*
* -
*
* if the user says "Yes" and continues with an utterance that triggers an intent it starts a conversation for the
* intent.
*
*
* -
*
* If the user says "No" it responds with the rejection statement configured for the the follow-up prompt.
*
*
* -
*
* If it doesn't recognize the utterance it repeats the follow-up prompt again.
*
*
*
*
* The followUpPrompt
field and the conclusionStatement
field are mutually exclusive. You
* can specify only one.
*
*/
private FollowUpPrompt followUpPrompt;
/**
*
* The statement that you want Amazon Lex to convey to the user after the intent is successfully fulfilled by the
* Lambda function.
*
*
* This element is relevant only if you provide a Lambda function in the fulfillmentActivity
. If you
* return the intent to the client application, you can't specify this element.
*
*
*
* The followUpPrompt
and conclusionStatement
are mutually exclusive. You can specify only
* one.
*
*
*/
private Statement conclusionStatement;
/**
*
* Specifies a Lambda function to invoke for each user input. You can invoke this Lambda function to personalize
* user interaction.
*
*
* For example, suppose your bot determines that the user is John. Your Lambda function might retrieve John's
* information from a backend database and prepopulate some of the values. For example, if you find that John is
* gluten intolerant, you might set the corresponding intent slot, GlutenIntolerant
, to true. You might
* find John's phone number and set the corresponding session attribute.
*
*/
private CodeHook dialogCodeHook;
/**
*
* Required. Describes how the intent is fulfilled. For example, after a user provides all of the information for a
* pizza order, fulfillmentActivity
defines how the bot places an order with a local pizza store.
*
*
* You might configure Amazon Lex to return all of the intent information to the client application, or direct it to
* invoke a Lambda function that can process the intent (for example, place an order with a pizzeria).
*
*/
private FulfillmentActivity fulfillmentActivity;
/**
*
* A unique identifier for the built-in intent to base this intent on. To find the signature for an intent, see Standard Built-in Intents in the Alexa Skills Kit.
*
*/
private String parentIntentSignature;
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new intent, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a intent, set the checksum
field to the checksum of the most recent revision
* of the $LATEST
version. If you don't specify the checksum
field, or if the checksum
* does not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*/
private String checksum;
/**
*
* The name of the intent. The name is not case sensitive.
*
*
* The name can't match a built-in intent name, or a built-in intent name with "AMAZON." removed. For example,
* because there is a built-in intent called AMAZON.HelpIntent
, you can't create a custom intent called
* HelpIntent
.
*
*
* For a list of built-in intents, see Standard Built-in Intents in the Alexa Skills Kit.
*
*
* @param name
* The name of the intent. The name is not case sensitive.
*
* The name can't match a built-in intent name, or a built-in intent name with "AMAZON." removed. For
* example, because there is a built-in intent called AMAZON.HelpIntent
, you can't create a
* custom intent called HelpIntent
.
*
*
* For a list of built-in intents, see Standard Built-in Intents in the Alexa Skills Kit.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the intent. The name is not case sensitive.
*
*
* The name can't match a built-in intent name, or a built-in intent name with "AMAZON." removed. For example,
* because there is a built-in intent called AMAZON.HelpIntent
, you can't create a custom intent called
* HelpIntent
.
*
*
* For a list of built-in intents, see Standard Built-in Intents in the Alexa Skills Kit.
*
*
* @return The name of the intent. The name is not case sensitive.
*
* The name can't match a built-in intent name, or a built-in intent name with "AMAZON." removed. For
* example, because there is a built-in intent called AMAZON.HelpIntent
, you can't create a
* custom intent called HelpIntent
.
*
*
* For a list of built-in intents, see Standard Built-in Intents in the Alexa Skills Kit.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the intent. The name is not case sensitive.
*
*
* The name can't match a built-in intent name, or a built-in intent name with "AMAZON." removed. For example,
* because there is a built-in intent called AMAZON.HelpIntent
, you can't create a custom intent called
* HelpIntent
.
*
*
* For a list of built-in intents, see Standard Built-in Intents in the Alexa Skills Kit.
*
*
* @param name
* The name of the intent. The name is not case sensitive.
*
* The name can't match a built-in intent name, or a built-in intent name with "AMAZON." removed. For
* example, because there is a built-in intent called AMAZON.HelpIntent
, you can't create a
* custom intent called HelpIntent
.
*
*
* For a list of built-in intents, see Standard Built-in Intents in the Alexa Skills Kit.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the intent.
*
*
* @param description
* A description of the intent.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the intent.
*
*
* @return A description of the intent.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the intent.
*
*
* @param description
* A description of the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts defined
* in the slots. For more information, see how-it-works.
*
*
* @return An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts
* defined in the slots. For more information, see how-it-works.
*/
public java.util.List getSlots() {
return slots;
}
/**
*
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts defined
* in the slots. For more information, see how-it-works.
*
*
* @param slots
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts
* defined in the slots. For more information, see how-it-works.
*/
public void setSlots(java.util.Collection slots) {
if (slots == null) {
this.slots = null;
return;
}
this.slots = new java.util.ArrayList(slots);
}
/**
*
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts defined
* in the slots. For more information, see how-it-works.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSlots(java.util.Collection)} or {@link #withSlots(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param slots
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts
* defined in the slots. For more information, see how-it-works.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withSlots(Slot... slots) {
if (this.slots == null) {
setSlots(new java.util.ArrayList(slots.length));
}
for (Slot ele : slots) {
this.slots.add(ele);
}
return this;
}
/**
*
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts defined
* in the slots. For more information, see how-it-works.
*
*
* @param slots
* An array of intent slots. At runtime, Amazon Lex elicits required slot values from the user using prompts
* defined in the slots. For more information, see how-it-works.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withSlots(java.util.Collection slots) {
setSlots(slots);
return this;
}
/**
*
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
*
* In each utterance, a slot name is enclosed in curly braces.
*
*
* @return An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
* In each utterance, a slot name is enclosed in curly braces.
*/
public java.util.List getSampleUtterances() {
return sampleUtterances;
}
/**
*
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
*
* In each utterance, a slot name is enclosed in curly braces.
*
*
* @param sampleUtterances
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
* In each utterance, a slot name is enclosed in curly braces.
*/
public void setSampleUtterances(java.util.Collection sampleUtterances) {
if (sampleUtterances == null) {
this.sampleUtterances = null;
return;
}
this.sampleUtterances = new java.util.ArrayList(sampleUtterances);
}
/**
*
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
*
* In each utterance, a slot name is enclosed in curly braces.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSampleUtterances(java.util.Collection)} or {@link #withSampleUtterances(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param sampleUtterances
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
* In each utterance, a slot name is enclosed in curly braces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withSampleUtterances(String... sampleUtterances) {
if (this.sampleUtterances == null) {
setSampleUtterances(new java.util.ArrayList(sampleUtterances.length));
}
for (String ele : sampleUtterances) {
this.sampleUtterances.add(ele);
}
return this;
}
/**
*
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
*
* In each utterance, a slot name is enclosed in curly braces.
*
*
* @param sampleUtterances
* An array of utterances (strings) that a user might say to signal the intent. For example,
* "I want {PizzaSize} pizza", "Order {Quantity} {PizzaSize} pizzas".
*
* In each utterance, a slot name is enclosed in curly braces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withSampleUtterances(java.util.Collection sampleUtterances) {
setSampleUtterances(sampleUtterances);
return this;
}
/**
*
* Prompts the user to confirm the intent. This question should have a yes or no answer.
*
*
* Amazon Lex uses this prompt to ensure that the user acknowledges that the intent is ready for fulfillment. For
* example, with the OrderPizza
intent, you might want to confirm that the order is correct before
* placing it. For other intents, such as intents that simply respond to user questions, you might not need to ask
* the user for confirmation before providing the information.
*
*
*
* You you must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*
*
* @param confirmationPrompt
* Prompts the user to confirm the intent. This question should have a yes or no answer.
*
* Amazon Lex uses this prompt to ensure that the user acknowledges that the intent is ready for fulfillment.
* For example, with the OrderPizza
intent, you might want to confirm that the order is correct
* before placing it. For other intents, such as intents that simply respond to user questions, you might not
* need to ask the user for confirmation before providing the information.
*
*
*
* You you must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*/
public void setConfirmationPrompt(Prompt confirmationPrompt) {
this.confirmationPrompt = confirmationPrompt;
}
/**
*
* Prompts the user to confirm the intent. This question should have a yes or no answer.
*
*
* Amazon Lex uses this prompt to ensure that the user acknowledges that the intent is ready for fulfillment. For
* example, with the OrderPizza
intent, you might want to confirm that the order is correct before
* placing it. For other intents, such as intents that simply respond to user questions, you might not need to ask
* the user for confirmation before providing the information.
*
*
*
* You you must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*
*
* @return Prompts the user to confirm the intent. This question should have a yes or no answer.
*
* Amazon Lex uses this prompt to ensure that the user acknowledges that the intent is ready for
* fulfillment. For example, with the OrderPizza
intent, you might want to confirm that the
* order is correct before placing it. For other intents, such as intents that simply respond to user
* questions, you might not need to ask the user for confirmation before providing the information.
*
*
*
* You you must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*/
public Prompt getConfirmationPrompt() {
return this.confirmationPrompt;
}
/**
*
* Prompts the user to confirm the intent. This question should have a yes or no answer.
*
*
* Amazon Lex uses this prompt to ensure that the user acknowledges that the intent is ready for fulfillment. For
* example, with the OrderPizza
intent, you might want to confirm that the order is correct before
* placing it. For other intents, such as intents that simply respond to user questions, you might not need to ask
* the user for confirmation before providing the information.
*
*
*
* You you must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*
*
* @param confirmationPrompt
* Prompts the user to confirm the intent. This question should have a yes or no answer.
*
* Amazon Lex uses this prompt to ensure that the user acknowledges that the intent is ready for fulfillment.
* For example, with the OrderPizza
intent, you might want to confirm that the order is correct
* before placing it. For other intents, such as intents that simply respond to user questions, you might not
* need to ask the user for confirmation before providing the information.
*
*
*
* You you must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withConfirmationPrompt(Prompt confirmationPrompt) {
setConfirmationPrompt(confirmationPrompt);
return this;
}
/**
*
* When the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
*
* You must provide both the rejectionStatement
and the confirmationPrompt
, or neither.
*
*
*
* @param rejectionStatement
* When the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds
* with this statement to acknowledge that the intent was canceled.
*
* You must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*/
public void setRejectionStatement(Statement rejectionStatement) {
this.rejectionStatement = rejectionStatement;
}
/**
*
* When the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
*
* You must provide both the rejectionStatement
and the confirmationPrompt
, or neither.
*
*
*
* @return When the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex
* responds with this statement to acknowledge that the intent was canceled.
*
* You must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
*/
public Statement getRejectionStatement() {
return this.rejectionStatement;
}
/**
*
* When the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
*
* You must provide both the rejectionStatement
and the confirmationPrompt
, or neither.
*
*
*
* @param rejectionStatement
* When the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds
* with this statement to acknowledge that the intent was canceled.
*
* You must provide both the rejectionStatement
and the confirmationPrompt
, or
* neither.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withRejectionStatement(Statement rejectionStatement) {
setRejectionStatement(rejectionStatement);
return this;
}
/**
*
* Amazon Lex uses this prompt to solicit additional activity after fulfilling an intent. For example, after the
* OrderPizza
intent is fulfilled, you might prompt the user to order a drink.
*
*
* The action that Amazon Lex takes depends on the user's response, as follows:
*
*
* -
*
* If the user says "Yes" it responds with the clarification prompt that is configured for the bot.
*
*
* -
*
* if the user says "Yes" and continues with an utterance that triggers an intent it starts a conversation for the
* intent.
*
*
* -
*
* If the user says "No" it responds with the rejection statement configured for the the follow-up prompt.
*
*
* -
*
* If it doesn't recognize the utterance it repeats the follow-up prompt again.
*
*
*
*
* The followUpPrompt
field and the conclusionStatement
field are mutually exclusive. You
* can specify only one.
*
*
* @param followUpPrompt
* Amazon Lex uses this prompt to solicit additional activity after fulfilling an intent. For example, after
* the OrderPizza
intent is fulfilled, you might prompt the user to order a drink.
*
* The action that Amazon Lex takes depends on the user's response, as follows:
*
*
* -
*
* If the user says "Yes" it responds with the clarification prompt that is configured for the bot.
*
*
* -
*
* if the user says "Yes" and continues with an utterance that triggers an intent it starts a conversation
* for the intent.
*
*
* -
*
* If the user says "No" it responds with the rejection statement configured for the the follow-up prompt.
*
*
* -
*
* If it doesn't recognize the utterance it repeats the follow-up prompt again.
*
*
*
*
* The followUpPrompt
field and the conclusionStatement
field are mutually
* exclusive. You can specify only one.
*/
public void setFollowUpPrompt(FollowUpPrompt followUpPrompt) {
this.followUpPrompt = followUpPrompt;
}
/**
*
* Amazon Lex uses this prompt to solicit additional activity after fulfilling an intent. For example, after the
* OrderPizza
intent is fulfilled, you might prompt the user to order a drink.
*
*
* The action that Amazon Lex takes depends on the user's response, as follows:
*
*
* -
*
* If the user says "Yes" it responds with the clarification prompt that is configured for the bot.
*
*
* -
*
* if the user says "Yes" and continues with an utterance that triggers an intent it starts a conversation for the
* intent.
*
*
* -
*
* If the user says "No" it responds with the rejection statement configured for the the follow-up prompt.
*
*
* -
*
* If it doesn't recognize the utterance it repeats the follow-up prompt again.
*
*
*
*
* The followUpPrompt
field and the conclusionStatement
field are mutually exclusive. You
* can specify only one.
*
*
* @return Amazon Lex uses this prompt to solicit additional activity after fulfilling an intent. For example, after
* the OrderPizza
intent is fulfilled, you might prompt the user to order a drink.
*
* The action that Amazon Lex takes depends on the user's response, as follows:
*
*
* -
*
* If the user says "Yes" it responds with the clarification prompt that is configured for the bot.
*
*
* -
*
* if the user says "Yes" and continues with an utterance that triggers an intent it starts a conversation
* for the intent.
*
*
* -
*
* If the user says "No" it responds with the rejection statement configured for the the follow-up prompt.
*
*
* -
*
* If it doesn't recognize the utterance it repeats the follow-up prompt again.
*
*
*
*
* The followUpPrompt
field and the conclusionStatement
field are mutually
* exclusive. You can specify only one.
*/
public FollowUpPrompt getFollowUpPrompt() {
return this.followUpPrompt;
}
/**
*
* Amazon Lex uses this prompt to solicit additional activity after fulfilling an intent. For example, after the
* OrderPizza
intent is fulfilled, you might prompt the user to order a drink.
*
*
* The action that Amazon Lex takes depends on the user's response, as follows:
*
*
* -
*
* If the user says "Yes" it responds with the clarification prompt that is configured for the bot.
*
*
* -
*
* if the user says "Yes" and continues with an utterance that triggers an intent it starts a conversation for the
* intent.
*
*
* -
*
* If the user says "No" it responds with the rejection statement configured for the the follow-up prompt.
*
*
* -
*
* If it doesn't recognize the utterance it repeats the follow-up prompt again.
*
*
*
*
* The followUpPrompt
field and the conclusionStatement
field are mutually exclusive. You
* can specify only one.
*
*
* @param followUpPrompt
* Amazon Lex uses this prompt to solicit additional activity after fulfilling an intent. For example, after
* the OrderPizza
intent is fulfilled, you might prompt the user to order a drink.
*
* The action that Amazon Lex takes depends on the user's response, as follows:
*
*
* -
*
* If the user says "Yes" it responds with the clarification prompt that is configured for the bot.
*
*
* -
*
* if the user says "Yes" and continues with an utterance that triggers an intent it starts a conversation
* for the intent.
*
*
* -
*
* If the user says "No" it responds with the rejection statement configured for the the follow-up prompt.
*
*
* -
*
* If it doesn't recognize the utterance it repeats the follow-up prompt again.
*
*
*
*
* The followUpPrompt
field and the conclusionStatement
field are mutually
* exclusive. You can specify only one.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withFollowUpPrompt(FollowUpPrompt followUpPrompt) {
setFollowUpPrompt(followUpPrompt);
return this;
}
/**
*
* The statement that you want Amazon Lex to convey to the user after the intent is successfully fulfilled by the
* Lambda function.
*
*
* This element is relevant only if you provide a Lambda function in the fulfillmentActivity
. If you
* return the intent to the client application, you can't specify this element.
*
*
*
* The followUpPrompt
and conclusionStatement
are mutually exclusive. You can specify only
* one.
*
*
*
* @param conclusionStatement
* The statement that you want Amazon Lex to convey to the user after the intent is successfully fulfilled by
* the Lambda function.
*
* This element is relevant only if you provide a Lambda function in the fulfillmentActivity
. If
* you return the intent to the client application, you can't specify this element.
*
*
*
* The followUpPrompt
and conclusionStatement
are mutually exclusive. You can
* specify only one.
*
*/
public void setConclusionStatement(Statement conclusionStatement) {
this.conclusionStatement = conclusionStatement;
}
/**
*
* The statement that you want Amazon Lex to convey to the user after the intent is successfully fulfilled by the
* Lambda function.
*
*
* This element is relevant only if you provide a Lambda function in the fulfillmentActivity
. If you
* return the intent to the client application, you can't specify this element.
*
*
*
* The followUpPrompt
and conclusionStatement
are mutually exclusive. You can specify only
* one.
*
*
*
* @return The statement that you want Amazon Lex to convey to the user after the intent is successfully fulfilled
* by the Lambda function.
*
* This element is relevant only if you provide a Lambda function in the fulfillmentActivity
.
* If you return the intent to the client application, you can't specify this element.
*
*
*
* The followUpPrompt
and conclusionStatement
are mutually exclusive. You can
* specify only one.
*
*/
public Statement getConclusionStatement() {
return this.conclusionStatement;
}
/**
*
* The statement that you want Amazon Lex to convey to the user after the intent is successfully fulfilled by the
* Lambda function.
*
*
* This element is relevant only if you provide a Lambda function in the fulfillmentActivity
. If you
* return the intent to the client application, you can't specify this element.
*
*
*
* The followUpPrompt
and conclusionStatement
are mutually exclusive. You can specify only
* one.
*
*
*
* @param conclusionStatement
* The statement that you want Amazon Lex to convey to the user after the intent is successfully fulfilled by
* the Lambda function.
*
* This element is relevant only if you provide a Lambda function in the fulfillmentActivity
. If
* you return the intent to the client application, you can't specify this element.
*
*
*
* The followUpPrompt
and conclusionStatement
are mutually exclusive. You can
* specify only one.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withConclusionStatement(Statement conclusionStatement) {
setConclusionStatement(conclusionStatement);
return this;
}
/**
*
* Specifies a Lambda function to invoke for each user input. You can invoke this Lambda function to personalize
* user interaction.
*
*
* For example, suppose your bot determines that the user is John. Your Lambda function might retrieve John's
* information from a backend database and prepopulate some of the values. For example, if you find that John is
* gluten intolerant, you might set the corresponding intent slot, GlutenIntolerant
, to true. You might
* find John's phone number and set the corresponding session attribute.
*
*
* @param dialogCodeHook
* Specifies a Lambda function to invoke for each user input. You can invoke this Lambda function to
* personalize user interaction.
*
* For example, suppose your bot determines that the user is John. Your Lambda function might retrieve John's
* information from a backend database and prepopulate some of the values. For example, if you find that John
* is gluten intolerant, you might set the corresponding intent slot, GlutenIntolerant
, to true.
* You might find John's phone number and set the corresponding session attribute.
*/
public void setDialogCodeHook(CodeHook dialogCodeHook) {
this.dialogCodeHook = dialogCodeHook;
}
/**
*
* Specifies a Lambda function to invoke for each user input. You can invoke this Lambda function to personalize
* user interaction.
*
*
* For example, suppose your bot determines that the user is John. Your Lambda function might retrieve John's
* information from a backend database and prepopulate some of the values. For example, if you find that John is
* gluten intolerant, you might set the corresponding intent slot, GlutenIntolerant
, to true. You might
* find John's phone number and set the corresponding session attribute.
*
*
* @return Specifies a Lambda function to invoke for each user input. You can invoke this Lambda function to
* personalize user interaction.
*
* For example, suppose your bot determines that the user is John. Your Lambda function might retrieve
* John's information from a backend database and prepopulate some of the values. For example, if you find
* that John is gluten intolerant, you might set the corresponding intent slot,
* GlutenIntolerant
, to true. You might find John's phone number and set the corresponding
* session attribute.
*/
public CodeHook getDialogCodeHook() {
return this.dialogCodeHook;
}
/**
*
* Specifies a Lambda function to invoke for each user input. You can invoke this Lambda function to personalize
* user interaction.
*
*
* For example, suppose your bot determines that the user is John. Your Lambda function might retrieve John's
* information from a backend database and prepopulate some of the values. For example, if you find that John is
* gluten intolerant, you might set the corresponding intent slot, GlutenIntolerant
, to true. You might
* find John's phone number and set the corresponding session attribute.
*
*
* @param dialogCodeHook
* Specifies a Lambda function to invoke for each user input. You can invoke this Lambda function to
* personalize user interaction.
*
* For example, suppose your bot determines that the user is John. Your Lambda function might retrieve John's
* information from a backend database and prepopulate some of the values. For example, if you find that John
* is gluten intolerant, you might set the corresponding intent slot, GlutenIntolerant
, to true.
* You might find John's phone number and set the corresponding session attribute.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withDialogCodeHook(CodeHook dialogCodeHook) {
setDialogCodeHook(dialogCodeHook);
return this;
}
/**
*
* Required. Describes how the intent is fulfilled. For example, after a user provides all of the information for a
* pizza order, fulfillmentActivity
defines how the bot places an order with a local pizza store.
*
*
* You might configure Amazon Lex to return all of the intent information to the client application, or direct it to
* invoke a Lambda function that can process the intent (for example, place an order with a pizzeria).
*
*
* @param fulfillmentActivity
* Required. Describes how the intent is fulfilled. For example, after a user provides all of the information
* for a pizza order, fulfillmentActivity
defines how the bot places an order with a local pizza
* store.
*
* You might configure Amazon Lex to return all of the intent information to the client application, or
* direct it to invoke a Lambda function that can process the intent (for example, place an order with a
* pizzeria).
*/
public void setFulfillmentActivity(FulfillmentActivity fulfillmentActivity) {
this.fulfillmentActivity = fulfillmentActivity;
}
/**
*
* Required. Describes how the intent is fulfilled. For example, after a user provides all of the information for a
* pizza order, fulfillmentActivity
defines how the bot places an order with a local pizza store.
*
*
* You might configure Amazon Lex to return all of the intent information to the client application, or direct it to
* invoke a Lambda function that can process the intent (for example, place an order with a pizzeria).
*
*
* @return Required. Describes how the intent is fulfilled. For example, after a user provides all of the
* information for a pizza order, fulfillmentActivity
defines how the bot places an order with
* a local pizza store.
*
* You might configure Amazon Lex to return all of the intent information to the client application, or
* direct it to invoke a Lambda function that can process the intent (for example, place an order with a
* pizzeria).
*/
public FulfillmentActivity getFulfillmentActivity() {
return this.fulfillmentActivity;
}
/**
*
* Required. Describes how the intent is fulfilled. For example, after a user provides all of the information for a
* pizza order, fulfillmentActivity
defines how the bot places an order with a local pizza store.
*
*
* You might configure Amazon Lex to return all of the intent information to the client application, or direct it to
* invoke a Lambda function that can process the intent (for example, place an order with a pizzeria).
*
*
* @param fulfillmentActivity
* Required. Describes how the intent is fulfilled. For example, after a user provides all of the information
* for a pizza order, fulfillmentActivity
defines how the bot places an order with a local pizza
* store.
*
* You might configure Amazon Lex to return all of the intent information to the client application, or
* direct it to invoke a Lambda function that can process the intent (for example, place an order with a
* pizzeria).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withFulfillmentActivity(FulfillmentActivity fulfillmentActivity) {
setFulfillmentActivity(fulfillmentActivity);
return this;
}
/**
*
* A unique identifier for the built-in intent to base this intent on. To find the signature for an intent, see Standard Built-in Intents in the Alexa Skills Kit.
*
*
* @param parentIntentSignature
* A unique identifier for the built-in intent to base this intent on. To find the signature for an intent,
* see Standard Built-in Intents in the Alexa Skills Kit.
*/
public void setParentIntentSignature(String parentIntentSignature) {
this.parentIntentSignature = parentIntentSignature;
}
/**
*
* A unique identifier for the built-in intent to base this intent on. To find the signature for an intent, see Standard Built-in Intents in the Alexa Skills Kit.
*
*
* @return A unique identifier for the built-in intent to base this intent on. To find the signature for an intent,
* see Standard Built-in Intents in the Alexa Skills Kit.
*/
public String getParentIntentSignature() {
return this.parentIntentSignature;
}
/**
*
* A unique identifier for the built-in intent to base this intent on. To find the signature for an intent, see Standard Built-in Intents in the Alexa Skills Kit.
*
*
* @param parentIntentSignature
* A unique identifier for the built-in intent to base this intent on. To find the signature for an intent,
* see Standard Built-in Intents in the Alexa Skills Kit.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withParentIntentSignature(String parentIntentSignature) {
setParentIntentSignature(parentIntentSignature);
return this;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new intent, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a intent, set the checksum
field to the checksum of the most recent revision
* of the $LATEST
version. If you don't specify the checksum
field, or if the checksum
* does not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @param checksum
* Identifies a specific revision of the $LATEST
version.
*
* When you create a new intent, leave the checksum
field blank. If you specify a checksum you
* get a BadRequestException
exception.
*
*
* When you want to update a intent, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or if
* the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
*/
public void setChecksum(String checksum) {
this.checksum = checksum;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new intent, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a intent, set the checksum
field to the checksum of the most recent revision
* of the $LATEST
version. If you don't specify the checksum
field, or if the checksum
* does not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @return Identifies a specific revision of the $LATEST
version.
*
* When you create a new intent, leave the checksum
field blank. If you specify a checksum you
* get a BadRequestException
exception.
*
*
* When you want to update a intent, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or
* if the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
*/
public String getChecksum() {
return this.checksum;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new intent, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a intent, set the checksum
field to the checksum of the most recent revision
* of the $LATEST
version. If you don't specify the checksum
field, or if the checksum
* does not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @param checksum
* Identifies a specific revision of the $LATEST
version.
*
* When you create a new intent, leave the checksum
field blank. If you specify a checksum you
* get a BadRequestException
exception.
*
*
* When you want to update a intent, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or if
* the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutIntentRequest withChecksum(String checksum) {
setChecksum(checksum);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getSlots() != null)
sb.append("Slots: ").append(getSlots()).append(",");
if (getSampleUtterances() != null)
sb.append("SampleUtterances: ").append(getSampleUtterances()).append(",");
if (getConfirmationPrompt() != null)
sb.append("ConfirmationPrompt: ").append(getConfirmationPrompt()).append(",");
if (getRejectionStatement() != null)
sb.append("RejectionStatement: ").append(getRejectionStatement()).append(",");
if (getFollowUpPrompt() != null)
sb.append("FollowUpPrompt: ").append(getFollowUpPrompt()).append(",");
if (getConclusionStatement() != null)
sb.append("ConclusionStatement: ").append(getConclusionStatement()).append(",");
if (getDialogCodeHook() != null)
sb.append("DialogCodeHook: ").append(getDialogCodeHook()).append(",");
if (getFulfillmentActivity() != null)
sb.append("FulfillmentActivity: ").append(getFulfillmentActivity()).append(",");
if (getParentIntentSignature() != null)
sb.append("ParentIntentSignature: ").append(getParentIntentSignature()).append(",");
if (getChecksum() != null)
sb.append("Checksum: ").append(getChecksum());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutIntentRequest == false)
return false;
PutIntentRequest other = (PutIntentRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getSlots() == null ^ this.getSlots() == null)
return false;
if (other.getSlots() != null && other.getSlots().equals(this.getSlots()) == false)
return false;
if (other.getSampleUtterances() == null ^ this.getSampleUtterances() == null)
return false;
if (other.getSampleUtterances() != null && other.getSampleUtterances().equals(this.getSampleUtterances()) == false)
return false;
if (other.getConfirmationPrompt() == null ^ this.getConfirmationPrompt() == null)
return false;
if (other.getConfirmationPrompt() != null && other.getConfirmationPrompt().equals(this.getConfirmationPrompt()) == false)
return false;
if (other.getRejectionStatement() == null ^ this.getRejectionStatement() == null)
return false;
if (other.getRejectionStatement() != null && other.getRejectionStatement().equals(this.getRejectionStatement()) == false)
return false;
if (other.getFollowUpPrompt() == null ^ this.getFollowUpPrompt() == null)
return false;
if (other.getFollowUpPrompt() != null && other.getFollowUpPrompt().equals(this.getFollowUpPrompt()) == false)
return false;
if (other.getConclusionStatement() == null ^ this.getConclusionStatement() == null)
return false;
if (other.getConclusionStatement() != null && other.getConclusionStatement().equals(this.getConclusionStatement()) == false)
return false;
if (other.getDialogCodeHook() == null ^ this.getDialogCodeHook() == null)
return false;
if (other.getDialogCodeHook() != null && other.getDialogCodeHook().equals(this.getDialogCodeHook()) == false)
return false;
if (other.getFulfillmentActivity() == null ^ this.getFulfillmentActivity() == null)
return false;
if (other.getFulfillmentActivity() != null && other.getFulfillmentActivity().equals(this.getFulfillmentActivity()) == false)
return false;
if (other.getParentIntentSignature() == null ^ this.getParentIntentSignature() == null)
return false;
if (other.getParentIntentSignature() != null && other.getParentIntentSignature().equals(this.getParentIntentSignature()) == false)
return false;
if (other.getChecksum() == null ^ this.getChecksum() == null)
return false;
if (other.getChecksum() != null && other.getChecksum().equals(this.getChecksum()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getSlots() == null) ? 0 : getSlots().hashCode());
hashCode = prime * hashCode + ((getSampleUtterances() == null) ? 0 : getSampleUtterances().hashCode());
hashCode = prime * hashCode + ((getConfirmationPrompt() == null) ? 0 : getConfirmationPrompt().hashCode());
hashCode = prime * hashCode + ((getRejectionStatement() == null) ? 0 : getRejectionStatement().hashCode());
hashCode = prime * hashCode + ((getFollowUpPrompt() == null) ? 0 : getFollowUpPrompt().hashCode());
hashCode = prime * hashCode + ((getConclusionStatement() == null) ? 0 : getConclusionStatement().hashCode());
hashCode = prime * hashCode + ((getDialogCodeHook() == null) ? 0 : getDialogCodeHook().hashCode());
hashCode = prime * hashCode + ((getFulfillmentActivity() == null) ? 0 : getFulfillmentActivity().hashCode());
hashCode = prime * hashCode + ((getParentIntentSignature() == null) ? 0 : getParentIntentSignature().hashCode());
hashCode = prime * hashCode + ((getChecksum() == null) ? 0 : getChecksum().hashCode());
return hashCode;
}
@Override
public PutIntentRequest clone() {
return (PutIntentRequest) super.clone();
}
}