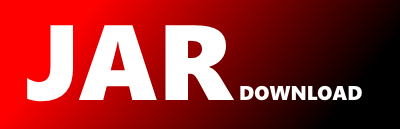
com.amazonaws.services.lexmodelbuilding.model.PutBotRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutBotRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the bot. The name is not case sensitive.
*
*/
private String name;
/**
*
* A description of the bot.
*
*/
private String description;
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*/
private java.util.List intents;
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeate the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*/
private Prompt clarificationPrompt;
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then aborts the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*/
private Statement abortStatement;
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*/
private Integer idleSessionTTLInSeconds;
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Available Voices in the Amazon Polly
* Developer Guide.
*
*/
private String voiceId;
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*/
private String checksum;
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*/
private String processBehavior;
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*/
private String locale;
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*/
private Boolean childDirected;
private Boolean createVersion;
/**
*
* The name of the bot. The name is not case sensitive.
*
*
* @param name
* The name of the bot. The name is not case sensitive.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the bot. The name is not case sensitive.
*
*
* @return The name of the bot. The name is not case sensitive.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the bot. The name is not case sensitive.
*
*
* @param name
* The name of the bot. The name is not case sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the bot.
*
*
* @param description
* A description of the bot.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the bot.
*
*
* @return A description of the bot.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the bot.
*
*
* @param description
* A description of the bot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* @return An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
*/
public java.util.List getIntents() {
return intents;
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* @param intents
* An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
*/
public void setIntents(java.util.Collection intents) {
if (intents == null) {
this.intents = null;
return;
}
this.intents = new java.util.ArrayList(intents);
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIntents(java.util.Collection)} or {@link #withIntents(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param intents
* An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withIntents(Intent... intents) {
if (this.intents == null) {
setIntents(new java.util.ArrayList(intents.length));
}
for (Intent ele : intents) {
this.intents.add(ele);
}
return this;
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* @param intents
* An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withIntents(java.util.Collection intents) {
setIntents(intents);
return this;
}
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeate the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* @param clarificationPrompt
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To
* specify how many times Amazon Lex should repeate the clarification prompt, use the
* maxAttempts
field. If Amazon Lex still doesn't understand, it sends the message in the
* abortStatement
field.
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*/
public void setClarificationPrompt(Prompt clarificationPrompt) {
this.clarificationPrompt = clarificationPrompt;
}
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeate the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* @return When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To
* specify how many times Amazon Lex should repeate the clarification prompt, use the
* maxAttempts
field. If Amazon Lex still doesn't understand, it sends the message in the
* abortStatement
field.
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user.
* for example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*/
public Prompt getClarificationPrompt() {
return this.clarificationPrompt;
}
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeate the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* @param clarificationPrompt
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To
* specify how many times Amazon Lex should repeate the clarification prompt, use the
* maxAttempts
field. If Amazon Lex still doesn't understand, it sends the message in the
* abortStatement
field.
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withClarificationPrompt(Prompt clarificationPrompt) {
setClarificationPrompt(clarificationPrompt);
return this;
}
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then aborts the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*
* @param abortStatement
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few
* times. After that, Amazon Lex sends the message defined in abortStatement
to the user, and
* then aborts the conversation. To set the number of retries, use the valueElicitationPrompt
* field for the slot type.
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If
* the user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.),
* Amazon Lex tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This
* intent might require the CrustType
slot. You specify the valueElicitationPrompt
* field when you create the CrustType
slot.
*/
public void setAbortStatement(Statement abortStatement) {
this.abortStatement = abortStatement;
}
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then aborts the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*
* @return When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few
* times. After that, Amazon Lex sends the message defined in abortStatement
to the user, and
* then aborts the conversation. To set the number of retries, use the valueElicitationPrompt
* field for the slot type.
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If
* the user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.),
* Amazon Lex tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This
* intent might require the CrustType
slot. You specify the valueElicitationPrompt
* field when you create the CrustType
slot.
*/
public Statement getAbortStatement() {
return this.abortStatement;
}
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then aborts the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*
* @param abortStatement
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few
* times. After that, Amazon Lex sends the message defined in abortStatement
to the user, and
* then aborts the conversation. To set the number of retries, use the valueElicitationPrompt
* field for the slot type.
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If
* the user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.),
* Amazon Lex tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This
* intent might require the CrustType
slot. You specify the valueElicitationPrompt
* field when you create the CrustType
slot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withAbortStatement(Statement abortStatement) {
setAbortStatement(abortStatement);
return this;
}
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*
* @param idleSessionTTLInSeconds
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs
* during this time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through
* placing an order. If the user doesn't complete the order within the specified time, Amazon Lex discards
* the slot information that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation
* request, Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*/
public void setIdleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
this.idleSessionTTLInSeconds = idleSessionTTLInSeconds;
}
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*
* @return The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs
* during this time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through
* placing an order. If the user doesn't complete the order within the specified time, Amazon Lex discards
* the slot information that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation
* request, Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*/
public Integer getIdleSessionTTLInSeconds() {
return this.idleSessionTTLInSeconds;
}
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*
* @param idleSessionTTLInSeconds
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs
* during this time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through
* placing an order. If the user doesn't complete the order within the specified time, Amazon Lex discards
* the slot information that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation
* request, Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withIdleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
setIdleSessionTTLInSeconds(idleSessionTTLInSeconds);
return this;
}
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Available Voices in the Amazon Polly
* Developer Guide.
*
*
* @param voiceId
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Available Voices in the Amazon
* Polly Developer Guide.
*/
public void setVoiceId(String voiceId) {
this.voiceId = voiceId;
}
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Available Voices in the Amazon Polly
* Developer Guide.
*
*
* @return The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The
* locale configured for the voice must match the locale of the bot. For more information, see Available Voices in the Amazon
* Polly Developer Guide.
*/
public String getVoiceId() {
return this.voiceId;
}
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Available Voices in the Amazon Polly
* Developer Guide.
*
*
* @param voiceId
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Available Voices in the Amazon
* Polly Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withVoiceId(String voiceId) {
setVoiceId(voiceId);
return this;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @param checksum
* Identifies a specific revision of the $LATEST
version.
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get
* a BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or if
* the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
*/
public void setChecksum(String checksum) {
this.checksum = checksum;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @return Identifies a specific revision of the $LATEST
version.
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get
* a BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or
* if the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
*/
public String getChecksum() {
return this.checksum;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @param checksum
* Identifies a specific revision of the $LATEST
version.
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get
* a BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or if
* the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withChecksum(String checksum) {
setChecksum(checksum);
return this;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @see ProcessBehavior
*/
public void setProcessBehavior(String processBehavior) {
this.processBehavior = processBehavior;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @return If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @see ProcessBehavior
*/
public String getProcessBehavior() {
return this.processBehavior;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessBehavior
*/
public PutBotRequest withProcessBehavior(String processBehavior) {
setProcessBehavior(processBehavior);
return this;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @see ProcessBehavior
*/
public void setProcessBehavior(ProcessBehavior processBehavior) {
withProcessBehavior(processBehavior);
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessBehavior
*/
public PutBotRequest withProcessBehavior(ProcessBehavior processBehavior) {
this.processBehavior = processBehavior.toString();
return this;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @see Locale
*/
public void setLocale(String locale) {
this.locale = locale;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @return Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @see Locale
*/
public String getLocale() {
return this.locale;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Locale
*/
public PutBotRequest withLocale(String locale) {
setLocale(locale);
return this;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @see Locale
*/
public void setLocale(Locale locale) {
withLocale(locale);
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Locale
*/
public PutBotRequest withLocale(Locale locale) {
this.locale = locale.toString();
return this;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @param childDirected
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program, or
* other application that is directed or targeted, in whole or in part, to children under age 13 and subject
* to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or
* in part, to children under age 13, you must obtain any required verifiable parental consent under COPPA.
* For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public void setChildDirected(Boolean childDirected) {
this.childDirected = childDirected;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @return For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program,
* or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole
* or in part, to children under age 13, you must obtain any required verifiable parental consent under
* COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public Boolean getChildDirected() {
return this.childDirected;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @param childDirected
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program, or
* other application that is directed or targeted, in whole or in part, to children under age 13 and subject
* to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or
* in part, to children under age 13, you must obtain any required verifiable parental consent under COPPA.
* For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withChildDirected(Boolean childDirected) {
setChildDirected(childDirected);
return this;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @return For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program,
* or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole
* or in part, to children under age 13, you must obtain any required verifiable parental consent under
* COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public Boolean isChildDirected() {
return this.childDirected;
}
/**
* @param createVersion
*/
public void setCreateVersion(Boolean createVersion) {
this.createVersion = createVersion;
}
/**
* @return
*/
public Boolean getCreateVersion() {
return this.createVersion;
}
/**
* @param createVersion
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withCreateVersion(Boolean createVersion) {
setCreateVersion(createVersion);
return this;
}
/**
* @return
*/
public Boolean isCreateVersion() {
return this.createVersion;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getIntents() != null)
sb.append("Intents: ").append(getIntents()).append(",");
if (getClarificationPrompt() != null)
sb.append("ClarificationPrompt: ").append(getClarificationPrompt()).append(",");
if (getAbortStatement() != null)
sb.append("AbortStatement: ").append(getAbortStatement()).append(",");
if (getIdleSessionTTLInSeconds() != null)
sb.append("IdleSessionTTLInSeconds: ").append(getIdleSessionTTLInSeconds()).append(",");
if (getVoiceId() != null)
sb.append("VoiceId: ").append(getVoiceId()).append(",");
if (getChecksum() != null)
sb.append("Checksum: ").append(getChecksum()).append(",");
if (getProcessBehavior() != null)
sb.append("ProcessBehavior: ").append(getProcessBehavior()).append(",");
if (getLocale() != null)
sb.append("Locale: ").append(getLocale()).append(",");
if (getChildDirected() != null)
sb.append("ChildDirected: ").append(getChildDirected()).append(",");
if (getCreateVersion() != null)
sb.append("CreateVersion: ").append(getCreateVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutBotRequest == false)
return false;
PutBotRequest other = (PutBotRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getIntents() == null ^ this.getIntents() == null)
return false;
if (other.getIntents() != null && other.getIntents().equals(this.getIntents()) == false)
return false;
if (other.getClarificationPrompt() == null ^ this.getClarificationPrompt() == null)
return false;
if (other.getClarificationPrompt() != null && other.getClarificationPrompt().equals(this.getClarificationPrompt()) == false)
return false;
if (other.getAbortStatement() == null ^ this.getAbortStatement() == null)
return false;
if (other.getAbortStatement() != null && other.getAbortStatement().equals(this.getAbortStatement()) == false)
return false;
if (other.getIdleSessionTTLInSeconds() == null ^ this.getIdleSessionTTLInSeconds() == null)
return false;
if (other.getIdleSessionTTLInSeconds() != null && other.getIdleSessionTTLInSeconds().equals(this.getIdleSessionTTLInSeconds()) == false)
return false;
if (other.getVoiceId() == null ^ this.getVoiceId() == null)
return false;
if (other.getVoiceId() != null && other.getVoiceId().equals(this.getVoiceId()) == false)
return false;
if (other.getChecksum() == null ^ this.getChecksum() == null)
return false;
if (other.getChecksum() != null && other.getChecksum().equals(this.getChecksum()) == false)
return false;
if (other.getProcessBehavior() == null ^ this.getProcessBehavior() == null)
return false;
if (other.getProcessBehavior() != null && other.getProcessBehavior().equals(this.getProcessBehavior()) == false)
return false;
if (other.getLocale() == null ^ this.getLocale() == null)
return false;
if (other.getLocale() != null && other.getLocale().equals(this.getLocale()) == false)
return false;
if (other.getChildDirected() == null ^ this.getChildDirected() == null)
return false;
if (other.getChildDirected() != null && other.getChildDirected().equals(this.getChildDirected()) == false)
return false;
if (other.getCreateVersion() == null ^ this.getCreateVersion() == null)
return false;
if (other.getCreateVersion() != null && other.getCreateVersion().equals(this.getCreateVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getIntents() == null) ? 0 : getIntents().hashCode());
hashCode = prime * hashCode + ((getClarificationPrompt() == null) ? 0 : getClarificationPrompt().hashCode());
hashCode = prime * hashCode + ((getAbortStatement() == null) ? 0 : getAbortStatement().hashCode());
hashCode = prime * hashCode + ((getIdleSessionTTLInSeconds() == null) ? 0 : getIdleSessionTTLInSeconds().hashCode());
hashCode = prime * hashCode + ((getVoiceId() == null) ? 0 : getVoiceId().hashCode());
hashCode = prime * hashCode + ((getChecksum() == null) ? 0 : getChecksum().hashCode());
hashCode = prime * hashCode + ((getProcessBehavior() == null) ? 0 : getProcessBehavior().hashCode());
hashCode = prime * hashCode + ((getLocale() == null) ? 0 : getLocale().hashCode());
hashCode = prime * hashCode + ((getChildDirected() == null) ? 0 : getChildDirected().hashCode());
hashCode = prime * hashCode + ((getCreateVersion() == null) ? 0 : getCreateVersion().hashCode());
return hashCode;
}
@Override
public PutBotRequest clone() {
return (PutBotRequest) super.clone();
}
}