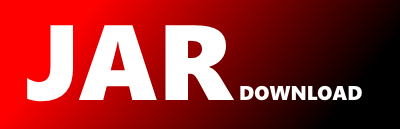
com.amazonaws.services.lexmodelbuilding.model.PutBotAliasResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutBotAliasResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the alias.
*
*/
private String name;
/**
*
* A description of the alias.
*
*/
private String description;
/**
*
* The version of the bot that the alias points to.
*
*/
private String botVersion;
/**
*
* The name of the bot that the alias points to.
*
*/
private String botName;
/**
*
* The date that the bot alias was updated. When you create a resource, the creation date and the last updated date
* are the same.
*
*/
private java.util.Date lastUpdatedDate;
/**
*
* The date that the bot alias was created.
*
*/
private java.util.Date createdDate;
/**
*
* The checksum for the current version of the alias.
*
*/
private String checksum;
/**
*
* The settings that determine how Amazon Lex uses conversation logs for the alias.
*
*/
private ConversationLogsResponse conversationLogs;
/**
*
* The name of the alias.
*
*
* @param name
* The name of the alias.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the alias.
*
*
* @return The name of the alias.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the alias.
*
*
* @param name
* The name of the alias.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the alias.
*
*
* @param description
* A description of the alias.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the alias.
*
*
* @return A description of the alias.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the alias.
*
*
* @param description
* A description of the alias.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The version of the bot that the alias points to.
*
*
* @param botVersion
* The version of the bot that the alias points to.
*/
public void setBotVersion(String botVersion) {
this.botVersion = botVersion;
}
/**
*
* The version of the bot that the alias points to.
*
*
* @return The version of the bot that the alias points to.
*/
public String getBotVersion() {
return this.botVersion;
}
/**
*
* The version of the bot that the alias points to.
*
*
* @param botVersion
* The version of the bot that the alias points to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withBotVersion(String botVersion) {
setBotVersion(botVersion);
return this;
}
/**
*
* The name of the bot that the alias points to.
*
*
* @param botName
* The name of the bot that the alias points to.
*/
public void setBotName(String botName) {
this.botName = botName;
}
/**
*
* The name of the bot that the alias points to.
*
*
* @return The name of the bot that the alias points to.
*/
public String getBotName() {
return this.botName;
}
/**
*
* The name of the bot that the alias points to.
*
*
* @param botName
* The name of the bot that the alias points to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withBotName(String botName) {
setBotName(botName);
return this;
}
/**
*
* The date that the bot alias was updated. When you create a resource, the creation date and the last updated date
* are the same.
*
*
* @param lastUpdatedDate
* The date that the bot alias was updated. When you create a resource, the creation date and the last
* updated date are the same.
*/
public void setLastUpdatedDate(java.util.Date lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
}
/**
*
* The date that the bot alias was updated. When you create a resource, the creation date and the last updated date
* are the same.
*
*
* @return The date that the bot alias was updated. When you create a resource, the creation date and the last
* updated date are the same.
*/
public java.util.Date getLastUpdatedDate() {
return this.lastUpdatedDate;
}
/**
*
* The date that the bot alias was updated. When you create a resource, the creation date and the last updated date
* are the same.
*
*
* @param lastUpdatedDate
* The date that the bot alias was updated. When you create a resource, the creation date and the last
* updated date are the same.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withLastUpdatedDate(java.util.Date lastUpdatedDate) {
setLastUpdatedDate(lastUpdatedDate);
return this;
}
/**
*
* The date that the bot alias was created.
*
*
* @param createdDate
* The date that the bot alias was created.
*/
public void setCreatedDate(java.util.Date createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date that the bot alias was created.
*
*
* @return The date that the bot alias was created.
*/
public java.util.Date getCreatedDate() {
return this.createdDate;
}
/**
*
* The date that the bot alias was created.
*
*
* @param createdDate
* The date that the bot alias was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withCreatedDate(java.util.Date createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
*
* The checksum for the current version of the alias.
*
*
* @param checksum
* The checksum for the current version of the alias.
*/
public void setChecksum(String checksum) {
this.checksum = checksum;
}
/**
*
* The checksum for the current version of the alias.
*
*
* @return The checksum for the current version of the alias.
*/
public String getChecksum() {
return this.checksum;
}
/**
*
* The checksum for the current version of the alias.
*
*
* @param checksum
* The checksum for the current version of the alias.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withChecksum(String checksum) {
setChecksum(checksum);
return this;
}
/**
*
* The settings that determine how Amazon Lex uses conversation logs for the alias.
*
*
* @param conversationLogs
* The settings that determine how Amazon Lex uses conversation logs for the alias.
*/
public void setConversationLogs(ConversationLogsResponse conversationLogs) {
this.conversationLogs = conversationLogs;
}
/**
*
* The settings that determine how Amazon Lex uses conversation logs for the alias.
*
*
* @return The settings that determine how Amazon Lex uses conversation logs for the alias.
*/
public ConversationLogsResponse getConversationLogs() {
return this.conversationLogs;
}
/**
*
* The settings that determine how Amazon Lex uses conversation logs for the alias.
*
*
* @param conversationLogs
* The settings that determine how Amazon Lex uses conversation logs for the alias.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotAliasResult withConversationLogs(ConversationLogsResponse conversationLogs) {
setConversationLogs(conversationLogs);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getBotVersion() != null)
sb.append("BotVersion: ").append(getBotVersion()).append(",");
if (getBotName() != null)
sb.append("BotName: ").append(getBotName()).append(",");
if (getLastUpdatedDate() != null)
sb.append("LastUpdatedDate: ").append(getLastUpdatedDate()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate()).append(",");
if (getChecksum() != null)
sb.append("Checksum: ").append(getChecksum()).append(",");
if (getConversationLogs() != null)
sb.append("ConversationLogs: ").append(getConversationLogs());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutBotAliasResult == false)
return false;
PutBotAliasResult other = (PutBotAliasResult) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getBotVersion() == null ^ this.getBotVersion() == null)
return false;
if (other.getBotVersion() != null && other.getBotVersion().equals(this.getBotVersion()) == false)
return false;
if (other.getBotName() == null ^ this.getBotName() == null)
return false;
if (other.getBotName() != null && other.getBotName().equals(this.getBotName()) == false)
return false;
if (other.getLastUpdatedDate() == null ^ this.getLastUpdatedDate() == null)
return false;
if (other.getLastUpdatedDate() != null && other.getLastUpdatedDate().equals(this.getLastUpdatedDate()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
if (other.getChecksum() == null ^ this.getChecksum() == null)
return false;
if (other.getChecksum() != null && other.getChecksum().equals(this.getChecksum()) == false)
return false;
if (other.getConversationLogs() == null ^ this.getConversationLogs() == null)
return false;
if (other.getConversationLogs() != null && other.getConversationLogs().equals(this.getConversationLogs()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getBotVersion() == null) ? 0 : getBotVersion().hashCode());
hashCode = prime * hashCode + ((getBotName() == null) ? 0 : getBotName().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedDate() == null) ? 0 : getLastUpdatedDate().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
hashCode = prime * hashCode + ((getChecksum() == null) ? 0 : getChecksum().hashCode());
hashCode = prime * hashCode + ((getConversationLogs() == null) ? 0 : getConversationLogs().hashCode());
return hashCode;
}
@Override
public PutBotAliasResult clone() {
try {
return (PutBotAliasResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}