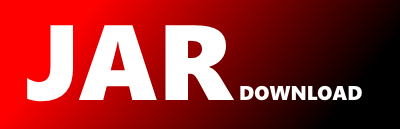
com.amazonaws.services.lexmodelbuilding.model.GetIntentResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetIntentResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the intent.
*
*/
private String name;
/**
*
* A description of the intent.
*
*/
private String description;
/**
*
* An array of intent slots configured for the intent.
*
*/
private java.util.List slots;
/**
*
* An array of sample utterances configured for the intent.
*
*/
private java.util.List sampleUtterances;
/**
*
* If defined in the bot, Amazon Lex uses prompt to confirm the intent before fulfilling the user's request. For
* more information, see PutIntent.
*
*/
private Prompt confirmationPrompt;
/**
*
* If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*/
private Statement rejectionStatement;
/**
*
* If defined in the bot, Amazon Lex uses this prompt to solicit additional user activity after the intent is
* fulfilled. For more information, see PutIntent.
*
*/
private FollowUpPrompt followUpPrompt;
/**
*
* After the Lambda function specified in the fulfillmentActivity
element fulfills the intent, Amazon
* Lex conveys this statement to the user.
*
*/
private Statement conclusionStatement;
/**
*
* If defined in the bot, Amazon Amazon Lex invokes this Lambda function for each user input. For more information,
* see PutIntent.
*
*/
private CodeHook dialogCodeHook;
/**
*
* Describes how the intent is fulfilled. For more information, see PutIntent.
*
*/
private FulfillmentActivity fulfillmentActivity;
/**
*
* A unique identifier for a built-in intent.
*
*/
private String parentIntentSignature;
/**
*
* The date that the intent was updated. When you create a resource, the creation date and the last updated date are
* the same.
*
*/
private java.util.Date lastUpdatedDate;
/**
*
* The date that the intent was created.
*
*/
private java.util.Date createdDate;
/**
*
* The version of the intent.
*
*/
private String version;
/**
*
* Checksum of the intent.
*
*/
private String checksum;
/**
*
* Configuration information, if any, to connect to an Amazon Kendra index with the
* AMAZON.KendraSearchIntent
intent.
*
*/
private KendraConfiguration kendraConfiguration;
/**
*
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex to
* choose the intent in a conversation with the user.
*
*/
private java.util.List inputContexts;
/**
*
* An array of OutputContext
objects that lists the contexts that the intent activates when the intent
* is fulfilled.
*
*/
private java.util.List outputContexts;
/**
*
* The name of the intent.
*
*
* @param name
* The name of the intent.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the intent.
*
*
* @return The name of the intent.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the intent.
*
*
* @param name
* The name of the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the intent.
*
*
* @param description
* A description of the intent.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the intent.
*
*
* @return A description of the intent.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the intent.
*
*
* @param description
* A description of the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* An array of intent slots configured for the intent.
*
*
* @return An array of intent slots configured for the intent.
*/
public java.util.List getSlots() {
return slots;
}
/**
*
* An array of intent slots configured for the intent.
*
*
* @param slots
* An array of intent slots configured for the intent.
*/
public void setSlots(java.util.Collection slots) {
if (slots == null) {
this.slots = null;
return;
}
this.slots = new java.util.ArrayList(slots);
}
/**
*
* An array of intent slots configured for the intent.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSlots(java.util.Collection)} or {@link #withSlots(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param slots
* An array of intent slots configured for the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withSlots(Slot... slots) {
if (this.slots == null) {
setSlots(new java.util.ArrayList(slots.length));
}
for (Slot ele : slots) {
this.slots.add(ele);
}
return this;
}
/**
*
* An array of intent slots configured for the intent.
*
*
* @param slots
* An array of intent slots configured for the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withSlots(java.util.Collection slots) {
setSlots(slots);
return this;
}
/**
*
* An array of sample utterances configured for the intent.
*
*
* @return An array of sample utterances configured for the intent.
*/
public java.util.List getSampleUtterances() {
return sampleUtterances;
}
/**
*
* An array of sample utterances configured for the intent.
*
*
* @param sampleUtterances
* An array of sample utterances configured for the intent.
*/
public void setSampleUtterances(java.util.Collection sampleUtterances) {
if (sampleUtterances == null) {
this.sampleUtterances = null;
return;
}
this.sampleUtterances = new java.util.ArrayList(sampleUtterances);
}
/**
*
* An array of sample utterances configured for the intent.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSampleUtterances(java.util.Collection)} or {@link #withSampleUtterances(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param sampleUtterances
* An array of sample utterances configured for the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withSampleUtterances(String... sampleUtterances) {
if (this.sampleUtterances == null) {
setSampleUtterances(new java.util.ArrayList(sampleUtterances.length));
}
for (String ele : sampleUtterances) {
this.sampleUtterances.add(ele);
}
return this;
}
/**
*
* An array of sample utterances configured for the intent.
*
*
* @param sampleUtterances
* An array of sample utterances configured for the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withSampleUtterances(java.util.Collection sampleUtterances) {
setSampleUtterances(sampleUtterances);
return this;
}
/**
*
* If defined in the bot, Amazon Lex uses prompt to confirm the intent before fulfilling the user's request. For
* more information, see PutIntent.
*
*
* @param confirmationPrompt
* If defined in the bot, Amazon Lex uses prompt to confirm the intent before fulfilling the user's request.
* For more information, see PutIntent.
*/
public void setConfirmationPrompt(Prompt confirmationPrompt) {
this.confirmationPrompt = confirmationPrompt;
}
/**
*
* If defined in the bot, Amazon Lex uses prompt to confirm the intent before fulfilling the user's request. For
* more information, see PutIntent.
*
*
* @return If defined in the bot, Amazon Lex uses prompt to confirm the intent before fulfilling the user's request.
* For more information, see PutIntent.
*/
public Prompt getConfirmationPrompt() {
return this.confirmationPrompt;
}
/**
*
* If defined in the bot, Amazon Lex uses prompt to confirm the intent before fulfilling the user's request. For
* more information, see PutIntent.
*
*
* @param confirmationPrompt
* If defined in the bot, Amazon Lex uses prompt to confirm the intent before fulfilling the user's request.
* For more information, see PutIntent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withConfirmationPrompt(Prompt confirmationPrompt) {
setConfirmationPrompt(confirmationPrompt);
return this;
}
/**
*
* If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
* @param rejectionStatement
* If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds
* with this statement to acknowledge that the intent was canceled.
*/
public void setRejectionStatement(Statement rejectionStatement) {
this.rejectionStatement = rejectionStatement;
}
/**
*
* If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
* @return If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds
* with this statement to acknowledge that the intent was canceled.
*/
public Statement getRejectionStatement() {
return this.rejectionStatement;
}
/**
*
* If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
* @param rejectionStatement
* If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds
* with this statement to acknowledge that the intent was canceled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withRejectionStatement(Statement rejectionStatement) {
setRejectionStatement(rejectionStatement);
return this;
}
/**
*
* If defined in the bot, Amazon Lex uses this prompt to solicit additional user activity after the intent is
* fulfilled. For more information, see PutIntent.
*
*
* @param followUpPrompt
* If defined in the bot, Amazon Lex uses this prompt to solicit additional user activity after the intent is
* fulfilled. For more information, see PutIntent.
*/
public void setFollowUpPrompt(FollowUpPrompt followUpPrompt) {
this.followUpPrompt = followUpPrompt;
}
/**
*
* If defined in the bot, Amazon Lex uses this prompt to solicit additional user activity after the intent is
* fulfilled. For more information, see PutIntent.
*
*
* @return If defined in the bot, Amazon Lex uses this prompt to solicit additional user activity after the intent
* is fulfilled. For more information, see PutIntent.
*/
public FollowUpPrompt getFollowUpPrompt() {
return this.followUpPrompt;
}
/**
*
* If defined in the bot, Amazon Lex uses this prompt to solicit additional user activity after the intent is
* fulfilled. For more information, see PutIntent.
*
*
* @param followUpPrompt
* If defined in the bot, Amazon Lex uses this prompt to solicit additional user activity after the intent is
* fulfilled. For more information, see PutIntent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withFollowUpPrompt(FollowUpPrompt followUpPrompt) {
setFollowUpPrompt(followUpPrompt);
return this;
}
/**
*
* After the Lambda function specified in the fulfillmentActivity
element fulfills the intent, Amazon
* Lex conveys this statement to the user.
*
*
* @param conclusionStatement
* After the Lambda function specified in the fulfillmentActivity
element fulfills the intent,
* Amazon Lex conveys this statement to the user.
*/
public void setConclusionStatement(Statement conclusionStatement) {
this.conclusionStatement = conclusionStatement;
}
/**
*
* After the Lambda function specified in the fulfillmentActivity
element fulfills the intent, Amazon
* Lex conveys this statement to the user.
*
*
* @return After the Lambda function specified in the fulfillmentActivity
element fulfills the intent,
* Amazon Lex conveys this statement to the user.
*/
public Statement getConclusionStatement() {
return this.conclusionStatement;
}
/**
*
* After the Lambda function specified in the fulfillmentActivity
element fulfills the intent, Amazon
* Lex conveys this statement to the user.
*
*
* @param conclusionStatement
* After the Lambda function specified in the fulfillmentActivity
element fulfills the intent,
* Amazon Lex conveys this statement to the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withConclusionStatement(Statement conclusionStatement) {
setConclusionStatement(conclusionStatement);
return this;
}
/**
*
* If defined in the bot, Amazon Amazon Lex invokes this Lambda function for each user input. For more information,
* see PutIntent.
*
*
* @param dialogCodeHook
* If defined in the bot, Amazon Amazon Lex invokes this Lambda function for each user input. For more
* information, see PutIntent.
*/
public void setDialogCodeHook(CodeHook dialogCodeHook) {
this.dialogCodeHook = dialogCodeHook;
}
/**
*
* If defined in the bot, Amazon Amazon Lex invokes this Lambda function for each user input. For more information,
* see PutIntent.
*
*
* @return If defined in the bot, Amazon Amazon Lex invokes this Lambda function for each user input. For more
* information, see PutIntent.
*/
public CodeHook getDialogCodeHook() {
return this.dialogCodeHook;
}
/**
*
* If defined in the bot, Amazon Amazon Lex invokes this Lambda function for each user input. For more information,
* see PutIntent.
*
*
* @param dialogCodeHook
* If defined in the bot, Amazon Amazon Lex invokes this Lambda function for each user input. For more
* information, see PutIntent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withDialogCodeHook(CodeHook dialogCodeHook) {
setDialogCodeHook(dialogCodeHook);
return this;
}
/**
*
* Describes how the intent is fulfilled. For more information, see PutIntent.
*
*
* @param fulfillmentActivity
* Describes how the intent is fulfilled. For more information, see PutIntent.
*/
public void setFulfillmentActivity(FulfillmentActivity fulfillmentActivity) {
this.fulfillmentActivity = fulfillmentActivity;
}
/**
*
* Describes how the intent is fulfilled. For more information, see PutIntent.
*
*
* @return Describes how the intent is fulfilled. For more information, see PutIntent.
*/
public FulfillmentActivity getFulfillmentActivity() {
return this.fulfillmentActivity;
}
/**
*
* Describes how the intent is fulfilled. For more information, see PutIntent.
*
*
* @param fulfillmentActivity
* Describes how the intent is fulfilled. For more information, see PutIntent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withFulfillmentActivity(FulfillmentActivity fulfillmentActivity) {
setFulfillmentActivity(fulfillmentActivity);
return this;
}
/**
*
* A unique identifier for a built-in intent.
*
*
* @param parentIntentSignature
* A unique identifier for a built-in intent.
*/
public void setParentIntentSignature(String parentIntentSignature) {
this.parentIntentSignature = parentIntentSignature;
}
/**
*
* A unique identifier for a built-in intent.
*
*
* @return A unique identifier for a built-in intent.
*/
public String getParentIntentSignature() {
return this.parentIntentSignature;
}
/**
*
* A unique identifier for a built-in intent.
*
*
* @param parentIntentSignature
* A unique identifier for a built-in intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withParentIntentSignature(String parentIntentSignature) {
setParentIntentSignature(parentIntentSignature);
return this;
}
/**
*
* The date that the intent was updated. When you create a resource, the creation date and the last updated date are
* the same.
*
*
* @param lastUpdatedDate
* The date that the intent was updated. When you create a resource, the creation date and the last updated
* date are the same.
*/
public void setLastUpdatedDate(java.util.Date lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
}
/**
*
* The date that the intent was updated. When you create a resource, the creation date and the last updated date are
* the same.
*
*
* @return The date that the intent was updated. When you create a resource, the creation date and the last updated
* date are the same.
*/
public java.util.Date getLastUpdatedDate() {
return this.lastUpdatedDate;
}
/**
*
* The date that the intent was updated. When you create a resource, the creation date and the last updated date are
* the same.
*
*
* @param lastUpdatedDate
* The date that the intent was updated. When you create a resource, the creation date and the last updated
* date are the same.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withLastUpdatedDate(java.util.Date lastUpdatedDate) {
setLastUpdatedDate(lastUpdatedDate);
return this;
}
/**
*
* The date that the intent was created.
*
*
* @param createdDate
* The date that the intent was created.
*/
public void setCreatedDate(java.util.Date createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date that the intent was created.
*
*
* @return The date that the intent was created.
*/
public java.util.Date getCreatedDate() {
return this.createdDate;
}
/**
*
* The date that the intent was created.
*
*
* @param createdDate
* The date that the intent was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withCreatedDate(java.util.Date createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
*
* The version of the intent.
*
*
* @param version
* The version of the intent.
*/
public void setVersion(String version) {
this.version = version;
}
/**
*
* The version of the intent.
*
*
* @return The version of the intent.
*/
public String getVersion() {
return this.version;
}
/**
*
* The version of the intent.
*
*
* @param version
* The version of the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withVersion(String version) {
setVersion(version);
return this;
}
/**
*
* Checksum of the intent.
*
*
* @param checksum
* Checksum of the intent.
*/
public void setChecksum(String checksum) {
this.checksum = checksum;
}
/**
*
* Checksum of the intent.
*
*
* @return Checksum of the intent.
*/
public String getChecksum() {
return this.checksum;
}
/**
*
* Checksum of the intent.
*
*
* @param checksum
* Checksum of the intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withChecksum(String checksum) {
setChecksum(checksum);
return this;
}
/**
*
* Configuration information, if any, to connect to an Amazon Kendra index with the
* AMAZON.KendraSearchIntent
intent.
*
*
* @param kendraConfiguration
* Configuration information, if any, to connect to an Amazon Kendra index with the
* AMAZON.KendraSearchIntent
intent.
*/
public void setKendraConfiguration(KendraConfiguration kendraConfiguration) {
this.kendraConfiguration = kendraConfiguration;
}
/**
*
* Configuration information, if any, to connect to an Amazon Kendra index with the
* AMAZON.KendraSearchIntent
intent.
*
*
* @return Configuration information, if any, to connect to an Amazon Kendra index with the
* AMAZON.KendraSearchIntent
intent.
*/
public KendraConfiguration getKendraConfiguration() {
return this.kendraConfiguration;
}
/**
*
* Configuration information, if any, to connect to an Amazon Kendra index with the
* AMAZON.KendraSearchIntent
intent.
*
*
* @param kendraConfiguration
* Configuration information, if any, to connect to an Amazon Kendra index with the
* AMAZON.KendraSearchIntent
intent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withKendraConfiguration(KendraConfiguration kendraConfiguration) {
setKendraConfiguration(kendraConfiguration);
return this;
}
/**
*
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex to
* choose the intent in a conversation with the user.
*
*
* @return An array of InputContext
objects that lists the contexts that must be active for Amazon Lex
* to choose the intent in a conversation with the user.
*/
public java.util.List getInputContexts() {
return inputContexts;
}
/**
*
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex to
* choose the intent in a conversation with the user.
*
*
* @param inputContexts
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex
* to choose the intent in a conversation with the user.
*/
public void setInputContexts(java.util.Collection inputContexts) {
if (inputContexts == null) {
this.inputContexts = null;
return;
}
this.inputContexts = new java.util.ArrayList(inputContexts);
}
/**
*
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex to
* choose the intent in a conversation with the user.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInputContexts(java.util.Collection)} or {@link #withInputContexts(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param inputContexts
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex
* to choose the intent in a conversation with the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withInputContexts(InputContext... inputContexts) {
if (this.inputContexts == null) {
setInputContexts(new java.util.ArrayList(inputContexts.length));
}
for (InputContext ele : inputContexts) {
this.inputContexts.add(ele);
}
return this;
}
/**
*
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex to
* choose the intent in a conversation with the user.
*
*
* @param inputContexts
* An array of InputContext
objects that lists the contexts that must be active for Amazon Lex
* to choose the intent in a conversation with the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withInputContexts(java.util.Collection inputContexts) {
setInputContexts(inputContexts);
return this;
}
/**
*
* An array of OutputContext
objects that lists the contexts that the intent activates when the intent
* is fulfilled.
*
*
* @return An array of OutputContext
objects that lists the contexts that the intent activates when the
* intent is fulfilled.
*/
public java.util.List getOutputContexts() {
return outputContexts;
}
/**
*
* An array of OutputContext
objects that lists the contexts that the intent activates when the intent
* is fulfilled.
*
*
* @param outputContexts
* An array of OutputContext
objects that lists the contexts that the intent activates when the
* intent is fulfilled.
*/
public void setOutputContexts(java.util.Collection outputContexts) {
if (outputContexts == null) {
this.outputContexts = null;
return;
}
this.outputContexts = new java.util.ArrayList(outputContexts);
}
/**
*
* An array of OutputContext
objects that lists the contexts that the intent activates when the intent
* is fulfilled.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOutputContexts(java.util.Collection)} or {@link #withOutputContexts(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param outputContexts
* An array of OutputContext
objects that lists the contexts that the intent activates when the
* intent is fulfilled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withOutputContexts(OutputContext... outputContexts) {
if (this.outputContexts == null) {
setOutputContexts(new java.util.ArrayList(outputContexts.length));
}
for (OutputContext ele : outputContexts) {
this.outputContexts.add(ele);
}
return this;
}
/**
*
* An array of OutputContext
objects that lists the contexts that the intent activates when the intent
* is fulfilled.
*
*
* @param outputContexts
* An array of OutputContext
objects that lists the contexts that the intent activates when the
* intent is fulfilled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetIntentResult withOutputContexts(java.util.Collection outputContexts) {
setOutputContexts(outputContexts);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getSlots() != null)
sb.append("Slots: ").append(getSlots()).append(",");
if (getSampleUtterances() != null)
sb.append("SampleUtterances: ").append(getSampleUtterances()).append(",");
if (getConfirmationPrompt() != null)
sb.append("ConfirmationPrompt: ").append(getConfirmationPrompt()).append(",");
if (getRejectionStatement() != null)
sb.append("RejectionStatement: ").append(getRejectionStatement()).append(",");
if (getFollowUpPrompt() != null)
sb.append("FollowUpPrompt: ").append(getFollowUpPrompt()).append(",");
if (getConclusionStatement() != null)
sb.append("ConclusionStatement: ").append(getConclusionStatement()).append(",");
if (getDialogCodeHook() != null)
sb.append("DialogCodeHook: ").append(getDialogCodeHook()).append(",");
if (getFulfillmentActivity() != null)
sb.append("FulfillmentActivity: ").append(getFulfillmentActivity()).append(",");
if (getParentIntentSignature() != null)
sb.append("ParentIntentSignature: ").append(getParentIntentSignature()).append(",");
if (getLastUpdatedDate() != null)
sb.append("LastUpdatedDate: ").append(getLastUpdatedDate()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion()).append(",");
if (getChecksum() != null)
sb.append("Checksum: ").append(getChecksum()).append(",");
if (getKendraConfiguration() != null)
sb.append("KendraConfiguration: ").append(getKendraConfiguration()).append(",");
if (getInputContexts() != null)
sb.append("InputContexts: ").append(getInputContexts()).append(",");
if (getOutputContexts() != null)
sb.append("OutputContexts: ").append(getOutputContexts());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetIntentResult == false)
return false;
GetIntentResult other = (GetIntentResult) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getSlots() == null ^ this.getSlots() == null)
return false;
if (other.getSlots() != null && other.getSlots().equals(this.getSlots()) == false)
return false;
if (other.getSampleUtterances() == null ^ this.getSampleUtterances() == null)
return false;
if (other.getSampleUtterances() != null && other.getSampleUtterances().equals(this.getSampleUtterances()) == false)
return false;
if (other.getConfirmationPrompt() == null ^ this.getConfirmationPrompt() == null)
return false;
if (other.getConfirmationPrompt() != null && other.getConfirmationPrompt().equals(this.getConfirmationPrompt()) == false)
return false;
if (other.getRejectionStatement() == null ^ this.getRejectionStatement() == null)
return false;
if (other.getRejectionStatement() != null && other.getRejectionStatement().equals(this.getRejectionStatement()) == false)
return false;
if (other.getFollowUpPrompt() == null ^ this.getFollowUpPrompt() == null)
return false;
if (other.getFollowUpPrompt() != null && other.getFollowUpPrompt().equals(this.getFollowUpPrompt()) == false)
return false;
if (other.getConclusionStatement() == null ^ this.getConclusionStatement() == null)
return false;
if (other.getConclusionStatement() != null && other.getConclusionStatement().equals(this.getConclusionStatement()) == false)
return false;
if (other.getDialogCodeHook() == null ^ this.getDialogCodeHook() == null)
return false;
if (other.getDialogCodeHook() != null && other.getDialogCodeHook().equals(this.getDialogCodeHook()) == false)
return false;
if (other.getFulfillmentActivity() == null ^ this.getFulfillmentActivity() == null)
return false;
if (other.getFulfillmentActivity() != null && other.getFulfillmentActivity().equals(this.getFulfillmentActivity()) == false)
return false;
if (other.getParentIntentSignature() == null ^ this.getParentIntentSignature() == null)
return false;
if (other.getParentIntentSignature() != null && other.getParentIntentSignature().equals(this.getParentIntentSignature()) == false)
return false;
if (other.getLastUpdatedDate() == null ^ this.getLastUpdatedDate() == null)
return false;
if (other.getLastUpdatedDate() != null && other.getLastUpdatedDate().equals(this.getLastUpdatedDate()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getChecksum() == null ^ this.getChecksum() == null)
return false;
if (other.getChecksum() != null && other.getChecksum().equals(this.getChecksum()) == false)
return false;
if (other.getKendraConfiguration() == null ^ this.getKendraConfiguration() == null)
return false;
if (other.getKendraConfiguration() != null && other.getKendraConfiguration().equals(this.getKendraConfiguration()) == false)
return false;
if (other.getInputContexts() == null ^ this.getInputContexts() == null)
return false;
if (other.getInputContexts() != null && other.getInputContexts().equals(this.getInputContexts()) == false)
return false;
if (other.getOutputContexts() == null ^ this.getOutputContexts() == null)
return false;
if (other.getOutputContexts() != null && other.getOutputContexts().equals(this.getOutputContexts()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getSlots() == null) ? 0 : getSlots().hashCode());
hashCode = prime * hashCode + ((getSampleUtterances() == null) ? 0 : getSampleUtterances().hashCode());
hashCode = prime * hashCode + ((getConfirmationPrompt() == null) ? 0 : getConfirmationPrompt().hashCode());
hashCode = prime * hashCode + ((getRejectionStatement() == null) ? 0 : getRejectionStatement().hashCode());
hashCode = prime * hashCode + ((getFollowUpPrompt() == null) ? 0 : getFollowUpPrompt().hashCode());
hashCode = prime * hashCode + ((getConclusionStatement() == null) ? 0 : getConclusionStatement().hashCode());
hashCode = prime * hashCode + ((getDialogCodeHook() == null) ? 0 : getDialogCodeHook().hashCode());
hashCode = prime * hashCode + ((getFulfillmentActivity() == null) ? 0 : getFulfillmentActivity().hashCode());
hashCode = prime * hashCode + ((getParentIntentSignature() == null) ? 0 : getParentIntentSignature().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedDate() == null) ? 0 : getLastUpdatedDate().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode + ((getChecksum() == null) ? 0 : getChecksum().hashCode());
hashCode = prime * hashCode + ((getKendraConfiguration() == null) ? 0 : getKendraConfiguration().hashCode());
hashCode = prime * hashCode + ((getInputContexts() == null) ? 0 : getInputContexts().hashCode());
hashCode = prime * hashCode + ((getOutputContexts() == null) ? 0 : getOutputContexts().hashCode());
return hashCode;
}
@Override
public GetIntentResult clone() {
try {
return (GetIntentResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}