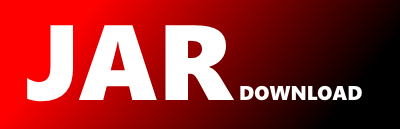
com.amazonaws.services.lexmodelbuilding.model.PutBotRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutBotRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the bot. The name is not case sensitive.
*
*/
private String name;
/**
*
* A description of the bot.
*
*/
private String description;
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*/
private java.util.List intents;
/**
*
* Set to true
to enable access to natural language understanding improvements.
*
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information, see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the parameter
* to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default. In
* these Regions setting the parameter to false
throws a ValidationException
exception.
*
*/
private Boolean enableModelImprovements;
/**
*
* Determines the threshold where Amazon Lex will insert the AMAZON.FallbackIntent
,
* AMAZON.KendraSearchIntent
, or both when returning alternative intents in a PostContent or PostText response.
* AMAZON.FallbackIntent
and AMAZON.KendraSearchIntent
are only inserted if they are
* configured for the bot.
*
*
* You must set the enableModelImprovements
parameter to true
to use confidence scores in
* the following regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default.
*
*
* For example, suppose a bot is configured with the confidence threshold of 0.80 and the
* AMAZON.FallbackIntent
. Amazon Lex returns three alternative intents with the following confidence
* scores: IntentA (0.70), IntentB (0.60), IntentC (0.50). The response from the PostText
operation
* would be:
*
*
* -
*
* AMAZON.FallbackIntent
*
*
* -
*
* IntentA
*
*
* -
*
* IntentB
*
*
* -
*
* IntentC
*
*
*
*/
private Double nluIntentConfidenceThreshold;
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeat the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* If you have defined a fallback intent, it will be invoked if the clarification prompt is repeated the number of
* times defined in the maxAttempts
field. For more information, see AMAZON.FallbackIntent.
*
*
* If you don't define a clarification prompt, at runtime Amazon Lex will return a 400 Bad Request exception in
* three cases:
*
*
* -
*
* Follow-up prompt - When the user responds to a follow-up prompt but does not provide an intent. For example, in
* response to a follow-up prompt that says "Would you like anything else today?" the user says "Yes." Amazon Lex
* will return a 400 Bad Request exception because it does not have a clarification prompt to send to the user to
* get an intent.
*
*
* -
*
* Lambda function - When using a Lambda function, you return an ElicitIntent
dialog type. Since Amazon
* Lex does not have a clarification prompt to get an intent from the user, it returns a 400 Bad Request exception.
*
*
* -
*
* PutSession operation - When using the PutSession
operation, you send an ElicitIntent
* dialog type. Since Amazon Lex does not have a clarification prompt to get an intent from the user, it returns a
* 400 Bad Request exception.
*
*
*
*/
private Prompt clarificationPrompt;
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then cancels the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*
* If you have defined a fallback intent the cancel statement will not be sent to the user, the fallback intent is
* used instead. For more information, see AMAZON.FallbackIntent.
*
*/
private Statement abortStatement;
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*/
private Integer idleSessionTTLInSeconds;
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Voices in Amazon Polly in the Amazon
* Polly Developer Guide.
*
*/
private String voiceId;
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*/
private String checksum;
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*/
private String processBehavior;
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*/
private String locale;
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*/
private Boolean childDirected;
/**
*
* When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If you don't
* specify detectSentiment
, the default is false
.
*
*/
private Boolean detectSentiment;
/**
*
* When set to true
a new numbered version of the bot is created. This is the same as calling the
* CreateBotVersion
operation. If you don't specify createVersion
, the default is
* false
.
*
*/
private Boolean createVersion;
/**
*
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the TagResource
* operation.
*
*/
private java.util.List tags;
/**
*
* The name of the bot. The name is not case sensitive.
*
*
* @param name
* The name of the bot. The name is not case sensitive.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the bot. The name is not case sensitive.
*
*
* @return The name of the bot. The name is not case sensitive.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the bot. The name is not case sensitive.
*
*
* @param name
* The name of the bot. The name is not case sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the bot.
*
*
* @param description
* A description of the bot.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the bot.
*
*
* @return A description of the bot.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the bot.
*
*
* @param description
* A description of the bot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* @return An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
*/
public java.util.List getIntents() {
return intents;
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* @param intents
* An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
*/
public void setIntents(java.util.Collection intents) {
if (intents == null) {
this.intents = null;
return;
}
this.intents = new java.util.ArrayList(intents);
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIntents(java.util.Collection)} or {@link #withIntents(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param intents
* An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withIntents(Intent... intents) {
if (this.intents == null) {
setIntents(new java.util.ArrayList(intents.length));
}
for (Intent ele : intents) {
this.intents.add(ele);
}
return this;
}
/**
*
* An array of Intent
objects. Each intent represents a command that a user can express. For example, a
* pizza ordering bot might support an OrderPizza intent. For more information, see how-it-works.
*
*
* @param intents
* An array of Intent
objects. Each intent represents a command that a user can express. For
* example, a pizza ordering bot might support an OrderPizza intent. For more information, see
* how-it-works.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withIntents(java.util.Collection intents) {
setIntents(intents);
return this;
}
/**
*
* Set to true
to enable access to natural language understanding improvements.
*
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information, see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the parameter
* to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default. In
* these Regions setting the parameter to false
throws a ValidationException
exception.
*
*
* @param enableModelImprovements
* Set to true
to enable access to natural language understanding improvements.
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information,
* see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the
* parameter to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by
* default. In these Regions setting the parameter to false
throws a
* ValidationException
exception.
*/
public void setEnableModelImprovements(Boolean enableModelImprovements) {
this.enableModelImprovements = enableModelImprovements;
}
/**
*
* Set to true
to enable access to natural language understanding improvements.
*
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information, see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the parameter
* to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default. In
* these Regions setting the parameter to false
throws a ValidationException
exception.
*
*
* @return Set to true
to enable access to natural language understanding improvements.
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information,
* see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the
* parameter to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
* are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by
* default. In these Regions setting the parameter to false
throws a
* ValidationException
exception.
*/
public Boolean getEnableModelImprovements() {
return this.enableModelImprovements;
}
/**
*
* Set to true
to enable access to natural language understanding improvements.
*
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information, see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the parameter
* to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default. In
* these Regions setting the parameter to false
throws a ValidationException
exception.
*
*
* @param enableModelImprovements
* Set to true
to enable access to natural language understanding improvements.
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information,
* see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the
* parameter to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by
* default. In these Regions setting the parameter to false
throws a
* ValidationException
exception.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withEnableModelImprovements(Boolean enableModelImprovements) {
setEnableModelImprovements(enableModelImprovements);
return this;
}
/**
*
* Set to true
to enable access to natural language understanding improvements.
*
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information, see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the parameter
* to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default. In
* these Regions setting the parameter to false
throws a ValidationException
exception.
*
*
* @return Set to true
to enable access to natural language understanding improvements.
*
* When you set the enableModelImprovements
parameter to true
you can use the
* nluIntentConfidenceThreshold
parameter to configure confidence scores. For more information,
* see Confidence Scores.
*
*
* You can only set the enableModelImprovements
parameter in certain Regions. If you set the
* parameter to true
, your bot has access to accuracy improvements.
*
*
* The Regions where you can set the enableModelImprovements
parameter to true
* are:
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by
* default. In these Regions setting the parameter to false
throws a
* ValidationException
exception.
*/
public Boolean isEnableModelImprovements() {
return this.enableModelImprovements;
}
/**
*
* Determines the threshold where Amazon Lex will insert the AMAZON.FallbackIntent
,
* AMAZON.KendraSearchIntent
, or both when returning alternative intents in a PostContent or PostText response.
* AMAZON.FallbackIntent
and AMAZON.KendraSearchIntent
are only inserted if they are
* configured for the bot.
*
*
* You must set the enableModelImprovements
parameter to true
to use confidence scores in
* the following regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default.
*
*
* For example, suppose a bot is configured with the confidence threshold of 0.80 and the
* AMAZON.FallbackIntent
. Amazon Lex returns three alternative intents with the following confidence
* scores: IntentA (0.70), IntentB (0.60), IntentC (0.50). The response from the PostText
operation
* would be:
*
*
* -
*
* AMAZON.FallbackIntent
*
*
* -
*
* IntentA
*
*
* -
*
* IntentB
*
*
* -
*
* IntentC
*
*
*
*
* @param nluIntentConfidenceThreshold
* Determines the threshold where Amazon Lex will insert the AMAZON.FallbackIntent
,
* AMAZON.KendraSearchIntent
, or both when returning alternative intents in a PostContent or PostText response.
* AMAZON.FallbackIntent
and AMAZON.KendraSearchIntent
are only inserted if they
* are configured for the bot.
*
* You must set the enableModelImprovements
parameter to true
to use confidence
* scores in the following regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by
* default.
*
*
* For example, suppose a bot is configured with the confidence threshold of 0.80 and the
* AMAZON.FallbackIntent
. Amazon Lex returns three alternative intents with the following
* confidence scores: IntentA (0.70), IntentB (0.60), IntentC (0.50). The response from the
* PostText
operation would be:
*
*
* -
*
* AMAZON.FallbackIntent
*
*
* -
*
* IntentA
*
*
* -
*
* IntentB
*
*
* -
*
* IntentC
*
*
*/
public void setNluIntentConfidenceThreshold(Double nluIntentConfidenceThreshold) {
this.nluIntentConfidenceThreshold = nluIntentConfidenceThreshold;
}
/**
*
* Determines the threshold where Amazon Lex will insert the AMAZON.FallbackIntent
,
* AMAZON.KendraSearchIntent
, or both when returning alternative intents in a PostContent or PostText response.
* AMAZON.FallbackIntent
and AMAZON.KendraSearchIntent
are only inserted if they are
* configured for the bot.
*
*
* You must set the enableModelImprovements
parameter to true
to use confidence scores in
* the following regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default.
*
*
* For example, suppose a bot is configured with the confidence threshold of 0.80 and the
* AMAZON.FallbackIntent
. Amazon Lex returns three alternative intents with the following confidence
* scores: IntentA (0.70), IntentB (0.60), IntentC (0.50). The response from the PostText
operation
* would be:
*
*
* -
*
* AMAZON.FallbackIntent
*
*
* -
*
* IntentA
*
*
* -
*
* IntentB
*
*
* -
*
* IntentC
*
*
*
*
* @return Determines the threshold where Amazon Lex will insert the AMAZON.FallbackIntent
,
* AMAZON.KendraSearchIntent
, or both when returning alternative intents in a PostContent or PostText response.
* AMAZON.FallbackIntent
and AMAZON.KendraSearchIntent
are only inserted if they
* are configured for the bot.
*
* You must set the enableModelImprovements
parameter to true
to use confidence
* scores in the following regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by
* default.
*
*
* For example, suppose a bot is configured with the confidence threshold of 0.80 and the
* AMAZON.FallbackIntent
. Amazon Lex returns three alternative intents with the following
* confidence scores: IntentA (0.70), IntentB (0.60), IntentC (0.50). The response from the
* PostText
operation would be:
*
*
* -
*
* AMAZON.FallbackIntent
*
*
* -
*
* IntentA
*
*
* -
*
* IntentB
*
*
* -
*
* IntentC
*
*
*/
public Double getNluIntentConfidenceThreshold() {
return this.nluIntentConfidenceThreshold;
}
/**
*
* Determines the threshold where Amazon Lex will insert the AMAZON.FallbackIntent
,
* AMAZON.KendraSearchIntent
, or both when returning alternative intents in a PostContent or PostText response.
* AMAZON.FallbackIntent
and AMAZON.KendraSearchIntent
are only inserted if they are
* configured for the bot.
*
*
* You must set the enableModelImprovements
parameter to true
to use confidence scores in
* the following regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by default.
*
*
* For example, suppose a bot is configured with the confidence threshold of 0.80 and the
* AMAZON.FallbackIntent
. Amazon Lex returns three alternative intents with the following confidence
* scores: IntentA (0.70), IntentB (0.60), IntentC (0.50). The response from the PostText
operation
* would be:
*
*
* -
*
* AMAZON.FallbackIntent
*
*
* -
*
* IntentA
*
*
* -
*
* IntentB
*
*
* -
*
* IntentC
*
*
*
*
* @param nluIntentConfidenceThreshold
* Determines the threshold where Amazon Lex will insert the AMAZON.FallbackIntent
,
* AMAZON.KendraSearchIntent
, or both when returning alternative intents in a PostContent or PostText response.
* AMAZON.FallbackIntent
and AMAZON.KendraSearchIntent
are only inserted if they
* are configured for the bot.
*
* You must set the enableModelImprovements
parameter to true
to use confidence
* scores in the following regions.
*
*
* -
*
* US East (N. Virginia) (us-east-1)
*
*
* -
*
* US West (Oregon) (us-west-2)
*
*
* -
*
* Asia Pacific (Sydney) (ap-southeast-2)
*
*
* -
*
* EU (Ireland) (eu-west-1)
*
*
*
*
* In other Regions, the enableModelImprovements
parameter is set to true
by
* default.
*
*
* For example, suppose a bot is configured with the confidence threshold of 0.80 and the
* AMAZON.FallbackIntent
. Amazon Lex returns three alternative intents with the following
* confidence scores: IntentA (0.70), IntentB (0.60), IntentC (0.50). The response from the
* PostText
operation would be:
*
*
* -
*
* AMAZON.FallbackIntent
*
*
* -
*
* IntentA
*
*
* -
*
* IntentB
*
*
* -
*
* IntentC
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withNluIntentConfidenceThreshold(Double nluIntentConfidenceThreshold) {
setNluIntentConfidenceThreshold(nluIntentConfidenceThreshold);
return this;
}
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeat the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* If you have defined a fallback intent, it will be invoked if the clarification prompt is repeated the number of
* times defined in the maxAttempts
field. For more information, see AMAZON.FallbackIntent.
*
*
* If you don't define a clarification prompt, at runtime Amazon Lex will return a 400 Bad Request exception in
* three cases:
*
*
* -
*
* Follow-up prompt - When the user responds to a follow-up prompt but does not provide an intent. For example, in
* response to a follow-up prompt that says "Would you like anything else today?" the user says "Yes." Amazon Lex
* will return a 400 Bad Request exception because it does not have a clarification prompt to send to the user to
* get an intent.
*
*
* -
*
* Lambda function - When using a Lambda function, you return an ElicitIntent
dialog type. Since Amazon
* Lex does not have a clarification prompt to get an intent from the user, it returns a 400 Bad Request exception.
*
*
* -
*
* PutSession operation - When using the PutSession
operation, you send an ElicitIntent
* dialog type. Since Amazon Lex does not have a clarification prompt to get an intent from the user, it returns a
* 400 Bad Request exception.
*
*
*
*
* @param clarificationPrompt
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To
* specify how many times Amazon Lex should repeat the clarification prompt, use the maxAttempts
* field. If Amazon Lex still doesn't understand, it sends the message in the abortStatement
* field.
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* If you have defined a fallback intent, it will be invoked if the clarification prompt is repeated the
* number of times defined in the maxAttempts
field. For more information, see AMAZON.FallbackIntent.
*
*
* If you don't define a clarification prompt, at runtime Amazon Lex will return a 400 Bad Request exception
* in three cases:
*
*
* -
*
* Follow-up prompt - When the user responds to a follow-up prompt but does not provide an intent. For
* example, in response to a follow-up prompt that says "Would you like anything else today?" the user says
* "Yes." Amazon Lex will return a 400 Bad Request exception because it does not have a clarification prompt
* to send to the user to get an intent.
*
*
* -
*
* Lambda function - When using a Lambda function, you return an ElicitIntent
dialog type. Since
* Amazon Lex does not have a clarification prompt to get an intent from the user, it returns a 400 Bad
* Request exception.
*
*
* -
*
* PutSession operation - When using the PutSession
operation, you send an
* ElicitIntent
dialog type. Since Amazon Lex does not have a clarification prompt to get an
* intent from the user, it returns a 400 Bad Request exception.
*
*
*/
public void setClarificationPrompt(Prompt clarificationPrompt) {
this.clarificationPrompt = clarificationPrompt;
}
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeat the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* If you have defined a fallback intent, it will be invoked if the clarification prompt is repeated the number of
* times defined in the maxAttempts
field. For more information, see AMAZON.FallbackIntent.
*
*
* If you don't define a clarification prompt, at runtime Amazon Lex will return a 400 Bad Request exception in
* three cases:
*
*
* -
*
* Follow-up prompt - When the user responds to a follow-up prompt but does not provide an intent. For example, in
* response to a follow-up prompt that says "Would you like anything else today?" the user says "Yes." Amazon Lex
* will return a 400 Bad Request exception because it does not have a clarification prompt to send to the user to
* get an intent.
*
*
* -
*
* Lambda function - When using a Lambda function, you return an ElicitIntent
dialog type. Since Amazon
* Lex does not have a clarification prompt to get an intent from the user, it returns a 400 Bad Request exception.
*
*
* -
*
* PutSession operation - When using the PutSession
operation, you send an ElicitIntent
* dialog type. Since Amazon Lex does not have a clarification prompt to get an intent from the user, it returns a
* 400 Bad Request exception.
*
*
*
*
* @return When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To
* specify how many times Amazon Lex should repeat the clarification prompt, use the
* maxAttempts
field. If Amazon Lex still doesn't understand, it sends the message in the
* abortStatement
field.
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user.
* for example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* If you have defined a fallback intent, it will be invoked if the clarification prompt is repeated the
* number of times defined in the maxAttempts
field. For more information, see
* AMAZON.FallbackIntent.
*
*
* If you don't define a clarification prompt, at runtime Amazon Lex will return a 400 Bad Request exception
* in three cases:
*
*
* -
*
* Follow-up prompt - When the user responds to a follow-up prompt but does not provide an intent. For
* example, in response to a follow-up prompt that says "Would you like anything else today?" the user says
* "Yes." Amazon Lex will return a 400 Bad Request exception because it does not have a clarification prompt
* to send to the user to get an intent.
*
*
* -
*
* Lambda function - When using a Lambda function, you return an ElicitIntent
dialog type.
* Since Amazon Lex does not have a clarification prompt to get an intent from the user, it returns a 400
* Bad Request exception.
*
*
* -
*
* PutSession operation - When using the PutSession
operation, you send an
* ElicitIntent
dialog type. Since Amazon Lex does not have a clarification prompt to get an
* intent from the user, it returns a 400 Bad Request exception.
*
*
*/
public Prompt getClarificationPrompt() {
return this.clarificationPrompt;
}
/**
*
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To specify how
* many times Amazon Lex should repeat the clarification prompt, use the maxAttempts
field. If Amazon
* Lex still doesn't understand, it sends the message in the abortStatement
field.
*
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* If you have defined a fallback intent, it will be invoked if the clarification prompt is repeated the number of
* times defined in the maxAttempts
field. For more information, see AMAZON.FallbackIntent.
*
*
* If you don't define a clarification prompt, at runtime Amazon Lex will return a 400 Bad Request exception in
* three cases:
*
*
* -
*
* Follow-up prompt - When the user responds to a follow-up prompt but does not provide an intent. For example, in
* response to a follow-up prompt that says "Would you like anything else today?" the user says "Yes." Amazon Lex
* will return a 400 Bad Request exception because it does not have a clarification prompt to send to the user to
* get an intent.
*
*
* -
*
* Lambda function - When using a Lambda function, you return an ElicitIntent
dialog type. Since Amazon
* Lex does not have a clarification prompt to get an intent from the user, it returns a 400 Bad Request exception.
*
*
* -
*
* PutSession operation - When using the PutSession
operation, you send an ElicitIntent
* dialog type. Since Amazon Lex does not have a clarification prompt to get an intent from the user, it returns a
* 400 Bad Request exception.
*
*
*
*
* @param clarificationPrompt
* When Amazon Lex doesn't understand the user's intent, it uses this message to get clarification. To
* specify how many times Amazon Lex should repeat the clarification prompt, use the maxAttempts
* field. If Amazon Lex still doesn't understand, it sends the message in the abortStatement
* field.
*
* When you create a clarification prompt, make sure that it suggests the correct response from the user. for
* example, for a bot that orders pizza and drinks, you might create this clarification prompt:
* "What would you like to do? You can say 'Order a pizza' or 'Order a drink.'"
*
*
* If you have defined a fallback intent, it will be invoked if the clarification prompt is repeated the
* number of times defined in the maxAttempts
field. For more information, see AMAZON.FallbackIntent.
*
*
* If you don't define a clarification prompt, at runtime Amazon Lex will return a 400 Bad Request exception
* in three cases:
*
*
* -
*
* Follow-up prompt - When the user responds to a follow-up prompt but does not provide an intent. For
* example, in response to a follow-up prompt that says "Would you like anything else today?" the user says
* "Yes." Amazon Lex will return a 400 Bad Request exception because it does not have a clarification prompt
* to send to the user to get an intent.
*
*
* -
*
* Lambda function - When using a Lambda function, you return an ElicitIntent
dialog type. Since
* Amazon Lex does not have a clarification prompt to get an intent from the user, it returns a 400 Bad
* Request exception.
*
*
* -
*
* PutSession operation - When using the PutSession
operation, you send an
* ElicitIntent
dialog type. Since Amazon Lex does not have a clarification prompt to get an
* intent from the user, it returns a 400 Bad Request exception.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withClarificationPrompt(Prompt clarificationPrompt) {
setClarificationPrompt(clarificationPrompt);
return this;
}
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then cancels the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*
* If you have defined a fallback intent the cancel statement will not be sent to the user, the fallback intent is
* used instead. For more information, see AMAZON.FallbackIntent.
*
*
* @param abortStatement
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few
* times. After that, Amazon Lex sends the message defined in abortStatement
to the user, and
* then cancels the conversation. To set the number of retries, use the valueElicitationPrompt
* field for the slot type.
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If
* the user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.),
* Amazon Lex tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This
* intent might require the CrustType
slot. You specify the valueElicitationPrompt
* field when you create the CrustType
slot.
*
*
* If you have defined a fallback intent the cancel statement will not be sent to the user, the fallback
* intent is used instead. For more information, see AMAZON.FallbackIntent.
*/
public void setAbortStatement(Statement abortStatement) {
this.abortStatement = abortStatement;
}
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then cancels the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*
* If you have defined a fallback intent the cancel statement will not be sent to the user, the fallback intent is
* used instead. For more information, see AMAZON.FallbackIntent.
*
*
* @return When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few
* times. After that, Amazon Lex sends the message defined in abortStatement
to the user, and
* then cancels the conversation. To set the number of retries, use the valueElicitationPrompt
* field for the slot type.
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If
* the user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.),
* Amazon Lex tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This
* intent might require the CrustType
slot. You specify the valueElicitationPrompt
* field when you create the CrustType
slot.
*
*
* If you have defined a fallback intent the cancel statement will not be sent to the user, the fallback
* intent is used instead. For more information, see
* AMAZON.FallbackIntent.
*/
public Statement getAbortStatement() {
return this.abortStatement;
}
/**
*
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few times.
* After that, Amazon Lex sends the message defined in abortStatement
to the user, and then cancels the
* conversation. To set the number of retries, use the valueElicitationPrompt
field for the slot type.
*
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If the
* user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.), Amazon Lex
* tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This intent
* might require the CrustType
slot. You specify the valueElicitationPrompt
field when you
* create the CrustType
slot.
*
*
* If you have defined a fallback intent the cancel statement will not be sent to the user, the fallback intent is
* used instead. For more information, see AMAZON.FallbackIntent.
*
*
* @param abortStatement
* When Amazon Lex can't understand the user's input in context, it tries to elicit the information a few
* times. After that, Amazon Lex sends the message defined in abortStatement
to the user, and
* then cancels the conversation. To set the number of retries, use the valueElicitationPrompt
* field for the slot type.
*
* For example, in a pizza ordering bot, Amazon Lex might ask a user "What type of crust would you like?" If
* the user's response is not one of the expected responses (for example, "thin crust, "deep dish," etc.),
* Amazon Lex tries to elicit a correct response a few more times.
*
*
* For example, in a pizza ordering application, OrderPizza
might be one of the intents. This
* intent might require the CrustType
slot. You specify the valueElicitationPrompt
* field when you create the CrustType
slot.
*
*
* If you have defined a fallback intent the cancel statement will not be sent to the user, the fallback
* intent is used instead. For more information, see AMAZON.FallbackIntent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withAbortStatement(Statement abortStatement) {
setAbortStatement(abortStatement);
return this;
}
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*
* @param idleSessionTTLInSeconds
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs
* during this time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through
* placing an order. If the user doesn't complete the order within the specified time, Amazon Lex discards
* the slot information that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation
* request, Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*/
public void setIdleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
this.idleSessionTTLInSeconds = idleSessionTTLInSeconds;
}
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*
* @return The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs
* during this time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through
* placing an order. If the user doesn't complete the order within the specified time, Amazon Lex discards
* the slot information that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation
* request, Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*/
public Integer getIdleSessionTTLInSeconds() {
return this.idleSessionTTLInSeconds;
}
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs during this
* time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through placing an
* order. If the user doesn't complete the order within the specified time, Amazon Lex discards the slot information
* that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation request,
* Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
*
*
* @param idleSessionTTLInSeconds
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation.
*
* A user interaction session remains active for the amount of time specified. If no conversation occurs
* during this time, the session expires and Amazon Lex deletes any data provided before the timeout.
*
*
* For example, suppose that a user chooses the OrderPizza intent, but gets sidetracked halfway through
* placing an order. If the user doesn't complete the order within the specified time, Amazon Lex discards
* the slot information that it gathered, and the user must start over.
*
*
* If you don't include the idleSessionTTLInSeconds
element in a PutBot
operation
* request, Amazon Lex uses the default value. This is also true if the request replaces an existing bot.
*
*
* The default is 300 seconds (5 minutes).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withIdleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
setIdleSessionTTLInSeconds(idleSessionTTLInSeconds);
return this;
}
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Voices in Amazon Polly in the Amazon
* Polly Developer Guide.
*
*
* @param voiceId
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Voices in Amazon Polly in the
* Amazon Polly Developer Guide.
*/
public void setVoiceId(String voiceId) {
this.voiceId = voiceId;
}
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Voices in Amazon Polly in the Amazon
* Polly Developer Guide.
*
*
* @return The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The
* locale configured for the voice must match the locale of the bot. For more information, see Voices in Amazon Polly in the
* Amazon Polly Developer Guide.
*/
public String getVoiceId() {
return this.voiceId;
}
/**
*
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Voices in Amazon Polly in the Amazon
* Polly Developer Guide.
*
*
* @param voiceId
* The Amazon Polly voice ID that you want Amazon Lex to use for voice interactions with the user. The locale
* configured for the voice must match the locale of the bot. For more information, see Voices in Amazon Polly in the
* Amazon Polly Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withVoiceId(String voiceId) {
setVoiceId(voiceId);
return this;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @param checksum
* Identifies a specific revision of the $LATEST
version.
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get
* a BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or if
* the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
*/
public void setChecksum(String checksum) {
this.checksum = checksum;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @return Identifies a specific revision of the $LATEST
version.
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get
* a BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or
* if the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
*/
public String getChecksum() {
return this.checksum;
}
/**
*
* Identifies a specific revision of the $LATEST
version.
*
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get a
* BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent revision of
* the $LATEST
version. If you don't specify the checksum
field, or if the checksum does
* not match the $LATEST
version, you get a PreconditionFailedException
exception.
*
*
* @param checksum
* Identifies a specific revision of the $LATEST
version.
*
* When you create a new bot, leave the checksum
field blank. If you specify a checksum you get
* a BadRequestException
exception.
*
*
* When you want to update a bot, set the checksum
field to the checksum of the most recent
* revision of the $LATEST
version. If you don't specify the checksum
field, or if
* the checksum does not match the $LATEST
version, you get a
* PreconditionFailedException
exception.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withChecksum(String checksum) {
setChecksum(checksum);
return this;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @see ProcessBehavior
*/
public void setProcessBehavior(String processBehavior) {
this.processBehavior = processBehavior;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @return If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @see ProcessBehavior
*/
public String getProcessBehavior() {
return this.processBehavior;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessBehavior
*/
public PutBotRequest withProcessBehavior(String processBehavior) {
setProcessBehavior(processBehavior);
return this;
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @see ProcessBehavior
*/
public void setProcessBehavior(ProcessBehavior processBehavior) {
withProcessBehavior(processBehavior);
}
/**
*
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so that it
* can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't build it.
*
*
* If you don't specify this value, the default value is BUILD
.
*
*
* @param processBehavior
* If you set the processBehavior
element to BUILD
, Amazon Lex builds the bot so
* that it can be run. If you set the element to SAVE
Amazon Lex saves the bot, but doesn't
* build it.
*
* If you don't specify this value, the default value is BUILD
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessBehavior
*/
public PutBotRequest withProcessBehavior(ProcessBehavior processBehavior) {
this.processBehavior = processBehavior.toString();
return this;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @see Locale
*/
public void setLocale(String locale) {
this.locale = locale;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @return Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @see Locale
*/
public String getLocale() {
return this.locale;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Locale
*/
public PutBotRequest withLocale(String locale) {
setLocale(locale);
return this;
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @see Locale
*/
public void setLocale(Locale locale) {
withLocale(locale);
}
/**
*
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of the
* bot.
*
*
* The default is en-US
.
*
*
* @param locale
* Specifies the target locale for the bot. Any intent used in the bot must be compatible with the locale of
* the bot.
*
* The default is en-US
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Locale
*/
public PutBotRequest withLocale(Locale locale) {
this.locale = locale.toString();
return this;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @param childDirected
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program, or
* other application that is directed or targeted, in whole or in part, to children under age 13 and subject
* to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or
* in part, to children under age 13, you must obtain any required verifiable parental consent under COPPA.
* For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public void setChildDirected(Boolean childDirected) {
this.childDirected = childDirected;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @return For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program,
* or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole
* or in part, to children under age 13, you must obtain any required verifiable parental consent under
* COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public Boolean getChildDirected() {
return this.childDirected;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @param childDirected
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program, or
* other application that is directed or targeted, in whole or in part, to children under age 13 and subject
* to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or
* in part, to children under age 13, you must obtain any required verifiable parental consent under COPPA.
* For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withChildDirected(Boolean childDirected) {
setChildDirected(childDirected);
return this;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @return For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program,
* or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole
* or in part, to children under age 13, you must obtain any required verifiable parental consent under
* COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public Boolean isChildDirected() {
return this.childDirected;
}
/**
*
* When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If you don't
* specify detectSentiment
, the default is false
.
*
*
* @param detectSentiment
* When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If you
* don't specify detectSentiment
, the default is false
.
*/
public void setDetectSentiment(Boolean detectSentiment) {
this.detectSentiment = detectSentiment;
}
/**
*
* When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If you don't
* specify detectSentiment
, the default is false
.
*
*
* @return When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If
* you don't specify detectSentiment
, the default is false
.
*/
public Boolean getDetectSentiment() {
return this.detectSentiment;
}
/**
*
* When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If you don't
* specify detectSentiment
, the default is false
.
*
*
* @param detectSentiment
* When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If you
* don't specify detectSentiment
, the default is false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withDetectSentiment(Boolean detectSentiment) {
setDetectSentiment(detectSentiment);
return this;
}
/**
*
* When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If you don't
* specify detectSentiment
, the default is false
.
*
*
* @return When set to true
user utterances are sent to Amazon Comprehend for sentiment analysis. If
* you don't specify detectSentiment
, the default is false
.
*/
public Boolean isDetectSentiment() {
return this.detectSentiment;
}
/**
*
* When set to true
a new numbered version of the bot is created. This is the same as calling the
* CreateBotVersion
operation. If you don't specify createVersion
, the default is
* false
.
*
*
* @param createVersion
* When set to true
a new numbered version of the bot is created. This is the same as calling
* the CreateBotVersion
operation. If you don't specify createVersion
, the default
* is false
.
*/
public void setCreateVersion(Boolean createVersion) {
this.createVersion = createVersion;
}
/**
*
* When set to true
a new numbered version of the bot is created. This is the same as calling the
* CreateBotVersion
operation. If you don't specify createVersion
, the default is
* false
.
*
*
* @return When set to true
a new numbered version of the bot is created. This is the same as calling
* the CreateBotVersion
operation. If you don't specify createVersion
, the default
* is false
.
*/
public Boolean getCreateVersion() {
return this.createVersion;
}
/**
*
* When set to true
a new numbered version of the bot is created. This is the same as calling the
* CreateBotVersion
operation. If you don't specify createVersion
, the default is
* false
.
*
*
* @param createVersion
* When set to true
a new numbered version of the bot is created. This is the same as calling
* the CreateBotVersion
operation. If you don't specify createVersion
, the default
* is false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withCreateVersion(Boolean createVersion) {
setCreateVersion(createVersion);
return this;
}
/**
*
* When set to true
a new numbered version of the bot is created. This is the same as calling the
* CreateBotVersion
operation. If you don't specify createVersion
, the default is
* false
.
*
*
* @return When set to true
a new numbered version of the bot is created. This is the same as calling
* the CreateBotVersion
operation. If you don't specify createVersion
, the default
* is false
.
*/
public Boolean isCreateVersion() {
return this.createVersion;
}
/**
*
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the TagResource
* operation.
*
*
* @return A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the
* TagResource
operation.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the TagResource
* operation.
*
*
* @param tags
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the
* TagResource
operation.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the TagResource
* operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the
* TagResource
operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the TagResource
* operation.
*
*
* @param tags
* A list of tags to add to the bot. You can only add tags when you create a bot, you can't use the
* PutBot
operation to update the tags on a bot. To update tags, use the
* TagResource
operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutBotRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getIntents() != null)
sb.append("Intents: ").append(getIntents()).append(",");
if (getEnableModelImprovements() != null)
sb.append("EnableModelImprovements: ").append(getEnableModelImprovements()).append(",");
if (getNluIntentConfidenceThreshold() != null)
sb.append("NluIntentConfidenceThreshold: ").append(getNluIntentConfidenceThreshold()).append(",");
if (getClarificationPrompt() != null)
sb.append("ClarificationPrompt: ").append(getClarificationPrompt()).append(",");
if (getAbortStatement() != null)
sb.append("AbortStatement: ").append(getAbortStatement()).append(",");
if (getIdleSessionTTLInSeconds() != null)
sb.append("IdleSessionTTLInSeconds: ").append(getIdleSessionTTLInSeconds()).append(",");
if (getVoiceId() != null)
sb.append("VoiceId: ").append(getVoiceId()).append(",");
if (getChecksum() != null)
sb.append("Checksum: ").append(getChecksum()).append(",");
if (getProcessBehavior() != null)
sb.append("ProcessBehavior: ").append(getProcessBehavior()).append(",");
if (getLocale() != null)
sb.append("Locale: ").append(getLocale()).append(",");
if (getChildDirected() != null)
sb.append("ChildDirected: ").append(getChildDirected()).append(",");
if (getDetectSentiment() != null)
sb.append("DetectSentiment: ").append(getDetectSentiment()).append(",");
if (getCreateVersion() != null)
sb.append("CreateVersion: ").append(getCreateVersion()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutBotRequest == false)
return false;
PutBotRequest other = (PutBotRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getIntents() == null ^ this.getIntents() == null)
return false;
if (other.getIntents() != null && other.getIntents().equals(this.getIntents()) == false)
return false;
if (other.getEnableModelImprovements() == null ^ this.getEnableModelImprovements() == null)
return false;
if (other.getEnableModelImprovements() != null && other.getEnableModelImprovements().equals(this.getEnableModelImprovements()) == false)
return false;
if (other.getNluIntentConfidenceThreshold() == null ^ this.getNluIntentConfidenceThreshold() == null)
return false;
if (other.getNluIntentConfidenceThreshold() != null && other.getNluIntentConfidenceThreshold().equals(this.getNluIntentConfidenceThreshold()) == false)
return false;
if (other.getClarificationPrompt() == null ^ this.getClarificationPrompt() == null)
return false;
if (other.getClarificationPrompt() != null && other.getClarificationPrompt().equals(this.getClarificationPrompt()) == false)
return false;
if (other.getAbortStatement() == null ^ this.getAbortStatement() == null)
return false;
if (other.getAbortStatement() != null && other.getAbortStatement().equals(this.getAbortStatement()) == false)
return false;
if (other.getIdleSessionTTLInSeconds() == null ^ this.getIdleSessionTTLInSeconds() == null)
return false;
if (other.getIdleSessionTTLInSeconds() != null && other.getIdleSessionTTLInSeconds().equals(this.getIdleSessionTTLInSeconds()) == false)
return false;
if (other.getVoiceId() == null ^ this.getVoiceId() == null)
return false;
if (other.getVoiceId() != null && other.getVoiceId().equals(this.getVoiceId()) == false)
return false;
if (other.getChecksum() == null ^ this.getChecksum() == null)
return false;
if (other.getChecksum() != null && other.getChecksum().equals(this.getChecksum()) == false)
return false;
if (other.getProcessBehavior() == null ^ this.getProcessBehavior() == null)
return false;
if (other.getProcessBehavior() != null && other.getProcessBehavior().equals(this.getProcessBehavior()) == false)
return false;
if (other.getLocale() == null ^ this.getLocale() == null)
return false;
if (other.getLocale() != null && other.getLocale().equals(this.getLocale()) == false)
return false;
if (other.getChildDirected() == null ^ this.getChildDirected() == null)
return false;
if (other.getChildDirected() != null && other.getChildDirected().equals(this.getChildDirected()) == false)
return false;
if (other.getDetectSentiment() == null ^ this.getDetectSentiment() == null)
return false;
if (other.getDetectSentiment() != null && other.getDetectSentiment().equals(this.getDetectSentiment()) == false)
return false;
if (other.getCreateVersion() == null ^ this.getCreateVersion() == null)
return false;
if (other.getCreateVersion() != null && other.getCreateVersion().equals(this.getCreateVersion()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getIntents() == null) ? 0 : getIntents().hashCode());
hashCode = prime * hashCode + ((getEnableModelImprovements() == null) ? 0 : getEnableModelImprovements().hashCode());
hashCode = prime * hashCode + ((getNluIntentConfidenceThreshold() == null) ? 0 : getNluIntentConfidenceThreshold().hashCode());
hashCode = prime * hashCode + ((getClarificationPrompt() == null) ? 0 : getClarificationPrompt().hashCode());
hashCode = prime * hashCode + ((getAbortStatement() == null) ? 0 : getAbortStatement().hashCode());
hashCode = prime * hashCode + ((getIdleSessionTTLInSeconds() == null) ? 0 : getIdleSessionTTLInSeconds().hashCode());
hashCode = prime * hashCode + ((getVoiceId() == null) ? 0 : getVoiceId().hashCode());
hashCode = prime * hashCode + ((getChecksum() == null) ? 0 : getChecksum().hashCode());
hashCode = prime * hashCode + ((getProcessBehavior() == null) ? 0 : getProcessBehavior().hashCode());
hashCode = prime * hashCode + ((getLocale() == null) ? 0 : getLocale().hashCode());
hashCode = prime * hashCode + ((getChildDirected() == null) ? 0 : getChildDirected().hashCode());
hashCode = prime * hashCode + ((getDetectSentiment() == null) ? 0 : getDetectSentiment().hashCode());
hashCode = prime * hashCode + ((getCreateVersion() == null) ? 0 : getCreateVersion().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public PutBotRequest clone() {
return (PutBotRequest) super.clone();
}
}