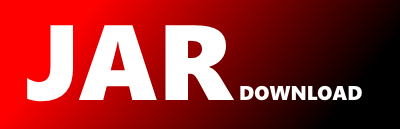
com.amazonaws.services.lexmodelbuilding.model.StartImportRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartImportRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A zip archive in binary format. The archive should contain one file, a JSON file containing the resource to
* import. The resource should match the type specified in the resourceType
field.
*
*/
private java.nio.ByteBuffer payload;
/**
*
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
*
*/
private String resourceType;
/**
*
* Specifies the action that the StartImport
operation should take when there is an existing resource
* with the same name.
*
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import file
* and an existing resource. The name of the resource causing the conflict is in the failureReason
* field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource. The
* $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
*
*/
private String mergeStrategy;
/**
*
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags to an
* intent or slot type.
*
*/
private java.util.List tags;
/**
*
* A zip archive in binary format. The archive should contain one file, a JSON file containing the resource to
* import. The resource should match the type specified in the resourceType
field.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param payload
* A zip archive in binary format. The archive should contain one file, a JSON file containing the resource
* to import. The resource should match the type specified in the resourceType
field.
*/
public void setPayload(java.nio.ByteBuffer payload) {
this.payload = payload;
}
/**
*
* A zip archive in binary format. The archive should contain one file, a JSON file containing the resource to
* import. The resource should match the type specified in the resourceType
field.
*
*
* {@code ByteBuffer}s are stateful. Calling their {@code get} methods changes their {@code position}. We recommend
* using {@link java.nio.ByteBuffer#asReadOnlyBuffer()} to create a read-only view of the buffer with an independent
* {@code position}, and calling {@code get} methods on this rather than directly on the returned {@code ByteBuffer}.
* Doing so will ensure that anyone else using the {@code ByteBuffer} will not be affected by changes to the
* {@code position}.
*
*
* @return A zip archive in binary format. The archive should contain one file, a JSON file containing the resource
* to import. The resource should match the type specified in the resourceType
field.
*/
public java.nio.ByteBuffer getPayload() {
return this.payload;
}
/**
*
* A zip archive in binary format. The archive should contain one file, a JSON file containing the resource to
* import. The resource should match the type specified in the resourceType
field.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param payload
* A zip archive in binary format. The archive should contain one file, a JSON file containing the resource
* to import. The resource should match the type specified in the resourceType
field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartImportRequest withPayload(java.nio.ByteBuffer payload) {
setPayload(payload);
return this;
}
/**
*
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
*
*
* @param resourceType
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
* @see ResourceType
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
*
*
* @return Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
* @see ResourceType
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
*
*
* @param resourceType
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceType
*/
public StartImportRequest withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
*
*
* @param resourceType
* Specifies the type of resource to export. Each resource also exports any resources that it depends on.
*
*
* -
*
* A bot exports dependent intents.
*
*
* -
*
* An intent exports dependent slot types.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceType
*/
public StartImportRequest withResourceType(ResourceType resourceType) {
this.resourceType = resourceType.toString();
return this;
}
/**
*
* Specifies the action that the StartImport
operation should take when there is an existing resource
* with the same name.
*
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import file
* and an existing resource. The name of the resource causing the conflict is in the failureReason
* field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource. The
* $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
*
*
* @param mergeStrategy
* Specifies the action that the StartImport
operation should take when there is an existing
* resource with the same name.
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import
* file and an existing resource. The name of the resource causing the conflict is in the
* failureReason
field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource.
* The $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
* @see MergeStrategy
*/
public void setMergeStrategy(String mergeStrategy) {
this.mergeStrategy = mergeStrategy;
}
/**
*
* Specifies the action that the StartImport
operation should take when there is an existing resource
* with the same name.
*
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import file
* and an existing resource. The name of the resource causing the conflict is in the failureReason
* field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource. The
* $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
*
*
* @return Specifies the action that the StartImport
operation should take when there is an existing
* resource with the same name.
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import
* file and an existing resource. The name of the resource causing the conflict is in the
* failureReason
field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource.
* The $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
* @see MergeStrategy
*/
public String getMergeStrategy() {
return this.mergeStrategy;
}
/**
*
* Specifies the action that the StartImport
operation should take when there is an existing resource
* with the same name.
*
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import file
* and an existing resource. The name of the resource causing the conflict is in the failureReason
* field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource. The
* $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
*
*
* @param mergeStrategy
* Specifies the action that the StartImport
operation should take when there is an existing
* resource with the same name.
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import
* file and an existing resource. The name of the resource causing the conflict is in the
* failureReason
field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource.
* The $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MergeStrategy
*/
public StartImportRequest withMergeStrategy(String mergeStrategy) {
setMergeStrategy(mergeStrategy);
return this;
}
/**
*
* Specifies the action that the StartImport
operation should take when there is an existing resource
* with the same name.
*
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import file
* and an existing resource. The name of the resource causing the conflict is in the failureReason
* field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource. The
* $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
*
*
* @param mergeStrategy
* Specifies the action that the StartImport
operation should take when there is an existing
* resource with the same name.
*
* -
*
* FAIL_ON_CONFLICT - The import operation is stopped on the first conflict between a resource in the import
* file and an existing resource. The name of the resource causing the conflict is in the
* failureReason
field of the response to the GetImport
operation.
*
*
* OVERWRITE_LATEST - The import operation proceeds even if there is a conflict with an existing resource.
* The $LASTEST version of the existing resource is overwritten with the data from the import file.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MergeStrategy
*/
public StartImportRequest withMergeStrategy(MergeStrategy mergeStrategy) {
this.mergeStrategy = mergeStrategy.toString();
return this;
}
/**
*
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags to an
* intent or slot type.
*
*
* @return A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add
* tags to an intent or slot type.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags to an
* intent or slot type.
*
*
* @param tags
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags
* to an intent or slot type.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags to an
* intent or slot type.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags
* to an intent or slot type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartImportRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags to an
* intent or slot type.
*
*
* @param tags
* A list of tags to add to the imported bot. You can only add tags when you import a bot, you can't add tags
* to an intent or slot type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartImportRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPayload() != null)
sb.append("Payload: ").append(getPayload()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getMergeStrategy() != null)
sb.append("MergeStrategy: ").append(getMergeStrategy()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartImportRequest == false)
return false;
StartImportRequest other = (StartImportRequest) obj;
if (other.getPayload() == null ^ this.getPayload() == null)
return false;
if (other.getPayload() != null && other.getPayload().equals(this.getPayload()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getMergeStrategy() == null ^ this.getMergeStrategy() == null)
return false;
if (other.getMergeStrategy() != null && other.getMergeStrategy().equals(this.getMergeStrategy()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPayload() == null) ? 0 : getPayload().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getMergeStrategy() == null) ? 0 : getMergeStrategy().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public StartImportRequest clone() {
return (StartImportRequest) super.clone();
}
}