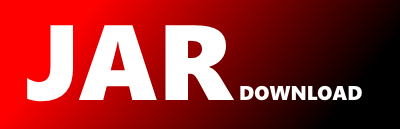
com.amazonaws.services.lexmodelbuilding.model.Slot Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Identifies the version of a specific slot.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Slot implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the slot.
*
*/
private String name;
/**
*
* A description of the slot.
*
*/
private String description;
/**
*
* Specifies whether the slot is required or optional.
*
*/
private String slotConstraint;
/**
*
* The type of the slot, either a custom slot type that you defined or one of the built-in slot types.
*
*/
private String slotType;
/**
*
* The version of the slot type.
*
*/
private String slotTypeVersion;
/**
*
* The prompt that Amazon Lex uses to elicit the slot value from the user.
*
*/
private Prompt valueElicitationPrompt;
/**
*
* Directs Amazon Lex the order in which to elicit this slot value from the user. For example, if the intent has two
* slots with priorities 1 and 2, AWS Amazon Lex first elicits a value for the slot with priority 1.
*
*
* If multiple slots share the same priority, the order in which Amazon Lex elicits values is arbitrary.
*
*/
private Integer priority;
/**
*
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value, you can
* provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is capable of
* understanding user utterances.
*
*/
private java.util.List sampleUtterances;
/**
*
* A set of possible responses for the slot type used by text-based clients. A user chooses an option from the
* response card, instead of using text to reply.
*
*/
private String responseCard;
/**
*
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a slot,
* the value is replaced by the slot name in curly braces ({}). For example, if the slot name is "full_name",
* obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
*
*/
private String obfuscationSetting;
/**
*
* A list of default values for the slot. Default values are used when Amazon Lex hasn't determined a value for a
* slot. You can specify default values from context variables, session attributes, and defined values.
*
*/
private SlotDefaultValueSpec defaultValueSpec;
/**
*
* The name of the slot.
*
*
* @param name
* The name of the slot.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the slot.
*
*
* @return The name of the slot.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the slot.
*
*
* @param name
* The name of the slot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withName(String name) {
setName(name);
return this;
}
/**
*
* A description of the slot.
*
*
* @param description
* A description of the slot.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the slot.
*
*
* @return A description of the slot.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the slot.
*
*
* @param description
* A description of the slot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Specifies whether the slot is required or optional.
*
*
* @param slotConstraint
* Specifies whether the slot is required or optional.
* @see SlotConstraint
*/
public void setSlotConstraint(String slotConstraint) {
this.slotConstraint = slotConstraint;
}
/**
*
* Specifies whether the slot is required or optional.
*
*
* @return Specifies whether the slot is required or optional.
* @see SlotConstraint
*/
public String getSlotConstraint() {
return this.slotConstraint;
}
/**
*
* Specifies whether the slot is required or optional.
*
*
* @param slotConstraint
* Specifies whether the slot is required or optional.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SlotConstraint
*/
public Slot withSlotConstraint(String slotConstraint) {
setSlotConstraint(slotConstraint);
return this;
}
/**
*
* Specifies whether the slot is required or optional.
*
*
* @param slotConstraint
* Specifies whether the slot is required or optional.
* @see SlotConstraint
*/
public void setSlotConstraint(SlotConstraint slotConstraint) {
withSlotConstraint(slotConstraint);
}
/**
*
* Specifies whether the slot is required or optional.
*
*
* @param slotConstraint
* Specifies whether the slot is required or optional.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SlotConstraint
*/
public Slot withSlotConstraint(SlotConstraint slotConstraint) {
this.slotConstraint = slotConstraint.toString();
return this;
}
/**
*
* The type of the slot, either a custom slot type that you defined or one of the built-in slot types.
*
*
* @param slotType
* The type of the slot, either a custom slot type that you defined or one of the built-in slot types.
*/
public void setSlotType(String slotType) {
this.slotType = slotType;
}
/**
*
* The type of the slot, either a custom slot type that you defined or one of the built-in slot types.
*
*
* @return The type of the slot, either a custom slot type that you defined or one of the built-in slot types.
*/
public String getSlotType() {
return this.slotType;
}
/**
*
* The type of the slot, either a custom slot type that you defined or one of the built-in slot types.
*
*
* @param slotType
* The type of the slot, either a custom slot type that you defined or one of the built-in slot types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withSlotType(String slotType) {
setSlotType(slotType);
return this;
}
/**
*
* The version of the slot type.
*
*
* @param slotTypeVersion
* The version of the slot type.
*/
public void setSlotTypeVersion(String slotTypeVersion) {
this.slotTypeVersion = slotTypeVersion;
}
/**
*
* The version of the slot type.
*
*
* @return The version of the slot type.
*/
public String getSlotTypeVersion() {
return this.slotTypeVersion;
}
/**
*
* The version of the slot type.
*
*
* @param slotTypeVersion
* The version of the slot type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withSlotTypeVersion(String slotTypeVersion) {
setSlotTypeVersion(slotTypeVersion);
return this;
}
/**
*
* The prompt that Amazon Lex uses to elicit the slot value from the user.
*
*
* @param valueElicitationPrompt
* The prompt that Amazon Lex uses to elicit the slot value from the user.
*/
public void setValueElicitationPrompt(Prompt valueElicitationPrompt) {
this.valueElicitationPrompt = valueElicitationPrompt;
}
/**
*
* The prompt that Amazon Lex uses to elicit the slot value from the user.
*
*
* @return The prompt that Amazon Lex uses to elicit the slot value from the user.
*/
public Prompt getValueElicitationPrompt() {
return this.valueElicitationPrompt;
}
/**
*
* The prompt that Amazon Lex uses to elicit the slot value from the user.
*
*
* @param valueElicitationPrompt
* The prompt that Amazon Lex uses to elicit the slot value from the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withValueElicitationPrompt(Prompt valueElicitationPrompt) {
setValueElicitationPrompt(valueElicitationPrompt);
return this;
}
/**
*
* Directs Amazon Lex the order in which to elicit this slot value from the user. For example, if the intent has two
* slots with priorities 1 and 2, AWS Amazon Lex first elicits a value for the slot with priority 1.
*
*
* If multiple slots share the same priority, the order in which Amazon Lex elicits values is arbitrary.
*
*
* @param priority
* Directs Amazon Lex the order in which to elicit this slot value from the user. For example, if the intent
* has two slots with priorities 1 and 2, AWS Amazon Lex first elicits a value for the slot with priority
* 1.
*
* If multiple slots share the same priority, the order in which Amazon Lex elicits values is arbitrary.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* Directs Amazon Lex the order in which to elicit this slot value from the user. For example, if the intent has two
* slots with priorities 1 and 2, AWS Amazon Lex first elicits a value for the slot with priority 1.
*
*
* If multiple slots share the same priority, the order in which Amazon Lex elicits values is arbitrary.
*
*
* @return Directs Amazon Lex the order in which to elicit this slot value from the user. For example, if the intent
* has two slots with priorities 1 and 2, AWS Amazon Lex first elicits a value for the slot with priority
* 1.
*
* If multiple slots share the same priority, the order in which Amazon Lex elicits values is arbitrary.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* Directs Amazon Lex the order in which to elicit this slot value from the user. For example, if the intent has two
* slots with priorities 1 and 2, AWS Amazon Lex first elicits a value for the slot with priority 1.
*
*
* If multiple slots share the same priority, the order in which Amazon Lex elicits values is arbitrary.
*
*
* @param priority
* Directs Amazon Lex the order in which to elicit this slot value from the user. For example, if the intent
* has two slots with priorities 1 and 2, AWS Amazon Lex first elicits a value for the slot with priority
* 1.
*
* If multiple slots share the same priority, the order in which Amazon Lex elicits values is arbitrary.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value, you can
* provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is capable of
* understanding user utterances.
*
*
* @return If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value,
* you can provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is
* capable of understanding user utterances.
*/
public java.util.List getSampleUtterances() {
return sampleUtterances;
}
/**
*
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value, you can
* provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is capable of
* understanding user utterances.
*
*
* @param sampleUtterances
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value,
* you can provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is
* capable of understanding user utterances.
*/
public void setSampleUtterances(java.util.Collection sampleUtterances) {
if (sampleUtterances == null) {
this.sampleUtterances = null;
return;
}
this.sampleUtterances = new java.util.ArrayList(sampleUtterances);
}
/**
*
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value, you can
* provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is capable of
* understanding user utterances.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSampleUtterances(java.util.Collection)} or {@link #withSampleUtterances(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param sampleUtterances
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value,
* you can provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is
* capable of understanding user utterances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withSampleUtterances(String... sampleUtterances) {
if (this.sampleUtterances == null) {
setSampleUtterances(new java.util.ArrayList(sampleUtterances.length));
}
for (String ele : sampleUtterances) {
this.sampleUtterances.add(ele);
}
return this;
}
/**
*
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value, you can
* provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is capable of
* understanding user utterances.
*
*
* @param sampleUtterances
* If you know a specific pattern with which users might respond to an Amazon Lex request for a slot value,
* you can provide those utterances to improve accuracy. This is optional. In most cases, Amazon Lex is
* capable of understanding user utterances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withSampleUtterances(java.util.Collection sampleUtterances) {
setSampleUtterances(sampleUtterances);
return this;
}
/**
*
* A set of possible responses for the slot type used by text-based clients. A user chooses an option from the
* response card, instead of using text to reply.
*
*
* @param responseCard
* A set of possible responses for the slot type used by text-based clients. A user chooses an option from
* the response card, instead of using text to reply.
*/
public void setResponseCard(String responseCard) {
this.responseCard = responseCard;
}
/**
*
* A set of possible responses for the slot type used by text-based clients. A user chooses an option from the
* response card, instead of using text to reply.
*
*
* @return A set of possible responses for the slot type used by text-based clients. A user chooses an option from
* the response card, instead of using text to reply.
*/
public String getResponseCard() {
return this.responseCard;
}
/**
*
* A set of possible responses for the slot type used by text-based clients. A user chooses an option from the
* response card, instead of using text to reply.
*
*
* @param responseCard
* A set of possible responses for the slot type used by text-based clients. A user chooses an option from
* the response card, instead of using text to reply.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withResponseCard(String responseCard) {
setResponseCard(responseCard);
return this;
}
/**
*
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a slot,
* the value is replaced by the slot name in curly braces ({}). For example, if the slot name is "full_name",
* obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
*
*
* @param obfuscationSetting
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a
* slot, the value is replaced by the slot name in curly braces ({}). For example, if the slot name is
* "full_name", obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
* @see ObfuscationSetting
*/
public void setObfuscationSetting(String obfuscationSetting) {
this.obfuscationSetting = obfuscationSetting;
}
/**
*
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a slot,
* the value is replaced by the slot name in curly braces ({}). For example, if the slot name is "full_name",
* obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
*
*
* @return Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a
* slot, the value is replaced by the slot name in curly braces ({}). For example, if the slot name is
* "full_name", obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
* @see ObfuscationSetting
*/
public String getObfuscationSetting() {
return this.obfuscationSetting;
}
/**
*
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a slot,
* the value is replaced by the slot name in curly braces ({}). For example, if the slot name is "full_name",
* obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
*
*
* @param obfuscationSetting
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a
* slot, the value is replaced by the slot name in curly braces ({}). For example, if the slot name is
* "full_name", obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObfuscationSetting
*/
public Slot withObfuscationSetting(String obfuscationSetting) {
setObfuscationSetting(obfuscationSetting);
return this;
}
/**
*
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a slot,
* the value is replaced by the slot name in curly braces ({}). For example, if the slot name is "full_name",
* obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
*
*
* @param obfuscationSetting
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a
* slot, the value is replaced by the slot name in curly braces ({}). For example, if the slot name is
* "full_name", obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
* @see ObfuscationSetting
*/
public void setObfuscationSetting(ObfuscationSetting obfuscationSetting) {
withObfuscationSetting(obfuscationSetting);
}
/**
*
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a slot,
* the value is replaced by the slot name in curly braces ({}). For example, if the slot name is "full_name",
* obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
*
*
* @param obfuscationSetting
* Determines whether a slot is obfuscated in conversation logs and stored utterances. When you obfuscate a
* slot, the value is replaced by the slot name in curly braces ({}). For example, if the slot name is
* "full_name", obfuscated values are replaced with "{full_name}". For more information, see Slot Obfuscation .
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObfuscationSetting
*/
public Slot withObfuscationSetting(ObfuscationSetting obfuscationSetting) {
this.obfuscationSetting = obfuscationSetting.toString();
return this;
}
/**
*
* A list of default values for the slot. Default values are used when Amazon Lex hasn't determined a value for a
* slot. You can specify default values from context variables, session attributes, and defined values.
*
*
* @param defaultValueSpec
* A list of default values for the slot. Default values are used when Amazon Lex hasn't determined a value
* for a slot. You can specify default values from context variables, session attributes, and defined values.
*/
public void setDefaultValueSpec(SlotDefaultValueSpec defaultValueSpec) {
this.defaultValueSpec = defaultValueSpec;
}
/**
*
* A list of default values for the slot. Default values are used when Amazon Lex hasn't determined a value for a
* slot. You can specify default values from context variables, session attributes, and defined values.
*
*
* @return A list of default values for the slot. Default values are used when Amazon Lex hasn't determined a value
* for a slot. You can specify default values from context variables, session attributes, and defined
* values.
*/
public SlotDefaultValueSpec getDefaultValueSpec() {
return this.defaultValueSpec;
}
/**
*
* A list of default values for the slot. Default values are used when Amazon Lex hasn't determined a value for a
* slot. You can specify default values from context variables, session attributes, and defined values.
*
*
* @param defaultValueSpec
* A list of default values for the slot. Default values are used when Amazon Lex hasn't determined a value
* for a slot. You can specify default values from context variables, session attributes, and defined values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Slot withDefaultValueSpec(SlotDefaultValueSpec defaultValueSpec) {
setDefaultValueSpec(defaultValueSpec);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getSlotConstraint() != null)
sb.append("SlotConstraint: ").append(getSlotConstraint()).append(",");
if (getSlotType() != null)
sb.append("SlotType: ").append(getSlotType()).append(",");
if (getSlotTypeVersion() != null)
sb.append("SlotTypeVersion: ").append(getSlotTypeVersion()).append(",");
if (getValueElicitationPrompt() != null)
sb.append("ValueElicitationPrompt: ").append(getValueElicitationPrompt()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getSampleUtterances() != null)
sb.append("SampleUtterances: ").append(getSampleUtterances()).append(",");
if (getResponseCard() != null)
sb.append("ResponseCard: ").append(getResponseCard()).append(",");
if (getObfuscationSetting() != null)
sb.append("ObfuscationSetting: ").append(getObfuscationSetting()).append(",");
if (getDefaultValueSpec() != null)
sb.append("DefaultValueSpec: ").append(getDefaultValueSpec());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Slot == false)
return false;
Slot other = (Slot) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getSlotConstraint() == null ^ this.getSlotConstraint() == null)
return false;
if (other.getSlotConstraint() != null && other.getSlotConstraint().equals(this.getSlotConstraint()) == false)
return false;
if (other.getSlotType() == null ^ this.getSlotType() == null)
return false;
if (other.getSlotType() != null && other.getSlotType().equals(this.getSlotType()) == false)
return false;
if (other.getSlotTypeVersion() == null ^ this.getSlotTypeVersion() == null)
return false;
if (other.getSlotTypeVersion() != null && other.getSlotTypeVersion().equals(this.getSlotTypeVersion()) == false)
return false;
if (other.getValueElicitationPrompt() == null ^ this.getValueElicitationPrompt() == null)
return false;
if (other.getValueElicitationPrompt() != null && other.getValueElicitationPrompt().equals(this.getValueElicitationPrompt()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getSampleUtterances() == null ^ this.getSampleUtterances() == null)
return false;
if (other.getSampleUtterances() != null && other.getSampleUtterances().equals(this.getSampleUtterances()) == false)
return false;
if (other.getResponseCard() == null ^ this.getResponseCard() == null)
return false;
if (other.getResponseCard() != null && other.getResponseCard().equals(this.getResponseCard()) == false)
return false;
if (other.getObfuscationSetting() == null ^ this.getObfuscationSetting() == null)
return false;
if (other.getObfuscationSetting() != null && other.getObfuscationSetting().equals(this.getObfuscationSetting()) == false)
return false;
if (other.getDefaultValueSpec() == null ^ this.getDefaultValueSpec() == null)
return false;
if (other.getDefaultValueSpec() != null && other.getDefaultValueSpec().equals(this.getDefaultValueSpec()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getSlotConstraint() == null) ? 0 : getSlotConstraint().hashCode());
hashCode = prime * hashCode + ((getSlotType() == null) ? 0 : getSlotType().hashCode());
hashCode = prime * hashCode + ((getSlotTypeVersion() == null) ? 0 : getSlotTypeVersion().hashCode());
hashCode = prime * hashCode + ((getValueElicitationPrompt() == null) ? 0 : getValueElicitationPrompt().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getSampleUtterances() == null) ? 0 : getSampleUtterances().hashCode());
hashCode = prime * hashCode + ((getResponseCard() == null) ? 0 : getResponseCard().hashCode());
hashCode = prime * hashCode + ((getObfuscationSetting() == null) ? 0 : getObfuscationSetting().hashCode());
hashCode = prime * hashCode + ((getDefaultValueSpec() == null) ? 0 : getDefaultValueSpec().hashCode());
return hashCode;
}
@Override
public Slot clone() {
try {
return (Slot) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.lexmodelbuilding.model.transform.SlotMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}