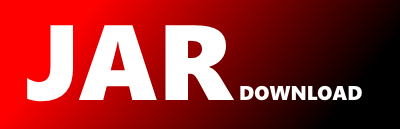
com.amazonaws.services.lexmodelbuilding.AmazonLexModelBuilding Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.lexmodelbuilding.model.*;
/**
* Interface for accessing Amazon Lex Model Building Service.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.lexmodelbuilding.AbstractAmazonLexModelBuilding} instead.
*
*
* Amazon Lex Build-Time Actions
*
* Amazon Lex is an AWS service for building conversational voice and text interfaces. Use these actions to create,
* update, and delete conversational bots for new and existing client applications.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonLexModelBuilding {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "models.lex";
/**
*
* Creates a new version of the bot based on the $LATEST
version. If the $LATEST
version
* of this resource hasn't changed since you created the last version, Amazon Lex doesn't create a new version. It
* returns the last created version.
*
*
*
* You can update only the $LATEST
version of the bot. You can't update the numbered versions that you
* create with the CreateBotVersion
operation.
*
*
*
* When you create the first version of a bot, Amazon Lex sets the version to 1. Subsequent versions increment by 1.
* For more information, see versioning-intro.
*
*
* This operation requires permission for the lex:CreateBotVersion
action.
*
*
* @param createBotVersionRequest
* @return Result of the CreateBotVersion operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws PreconditionFailedException
* The checksum of the resource that you are trying to change does not match the checksum in the request.
* Check the resource's checksum and try again.
* @sample AmazonLexModelBuilding.CreateBotVersion
* @see AWS
* API Documentation
*/
CreateBotVersionResult createBotVersion(CreateBotVersionRequest createBotVersionRequest);
/**
*
* Creates a new version of an intent based on the $LATEST
version of the intent. If the
* $LATEST
version of this intent hasn't changed since you last updated it, Amazon Lex doesn't create a
* new version. It returns the last version you created.
*
*
*
* You can update only the $LATEST
version of the intent. You can't update the numbered versions that
* you create with the CreateIntentVersion
operation.
*
*
*
* When you create a version of an intent, Amazon Lex sets the version to 1. Subsequent versions increment by 1. For
* more information, see versioning-intro.
*
*
* This operation requires permissions to perform the lex:CreateIntentVersion
action.
*
*
* @param createIntentVersionRequest
* @return Result of the CreateIntentVersion operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws PreconditionFailedException
* The checksum of the resource that you are trying to change does not match the checksum in the request.
* Check the resource's checksum and try again.
* @sample AmazonLexModelBuilding.CreateIntentVersion
* @see AWS
* API Documentation
*/
CreateIntentVersionResult createIntentVersion(CreateIntentVersionRequest createIntentVersionRequest);
/**
*
* Creates a new version of a slot type based on the $LATEST
version of the specified slot type. If the
* $LATEST
version of this resource has not changed since the last version that you created, Amazon Lex
* doesn't create a new version. It returns the last version that you created.
*
*
*
* You can update only the $LATEST
version of a slot type. You can't update the numbered versions that
* you create with the CreateSlotTypeVersion
operation.
*
*
*
* When you create a version of a slot type, Amazon Lex sets the version to 1. Subsequent versions increment by 1.
* For more information, see versioning-intro.
*
*
* This operation requires permissions for the lex:CreateSlotTypeVersion
action.
*
*
* @param createSlotTypeVersionRequest
* @return Result of the CreateSlotTypeVersion operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws PreconditionFailedException
* The checksum of the resource that you are trying to change does not match the checksum in the request.
* Check the resource's checksum and try again.
* @sample AmazonLexModelBuilding.CreateSlotTypeVersion
* @see AWS API Documentation
*/
CreateSlotTypeVersionResult createSlotTypeVersion(CreateSlotTypeVersionRequest createSlotTypeVersionRequest);
/**
*
* Deletes all versions of the bot, including the $LATEST
version. To delete a specific version of the
* bot, use the DeleteBotVersion operation. The DeleteBot
operation doesn't immediately remove
* the bot schema. Instead, it is marked for deletion and removed later.
*
*
* Amazon Lex stores utterances indefinitely for improving the ability of your bot to respond to user inputs. These
* utterances are not removed when the bot is deleted. To remove the utterances, use the DeleteUtterances
* operation.
*
*
* If a bot has an alias, you can't delete it. Instead, the DeleteBot
operation returns a
* ResourceInUseException
exception that includes a reference to the alias that refers to the bot. To
* remove the reference to the bot, delete the alias. If you get the same exception again, delete the referring
* alias until the DeleteBot
operation is successful.
*
*
* This operation requires permissions for the lex:DeleteBot
action.
*
*
* @param deleteBotRequest
* @return Result of the DeleteBot operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ResourceInUseException
* The resource that you are attempting to delete is referred to by another resource. Use this information
* to remove references to the resource that you are trying to delete.
*
* The body of the exception contains a JSON object that describes the resource.
*
*
* { "resourceType": BOT | BOTALIAS | BOTCHANNEL | INTENT,
*
*
* "resourceReference": {
*
*
* "name": string, "version": string } }
* @sample AmazonLexModelBuilding.DeleteBot
* @see AWS API
* Documentation
*/
DeleteBotResult deleteBot(DeleteBotRequest deleteBotRequest);
/**
*
* Deletes an alias for the specified bot.
*
*
* You can't delete an alias that is used in the association between a bot and a messaging channel. If an alias is
* used in a channel association, the DeleteBot
operation returns a ResourceInUseException
* exception that includes a reference to the channel association that refers to the bot. You can remove the
* reference to the alias by deleting the channel association. If you get the same exception again, delete the
* referring association until the DeleteBotAlias
operation is successful.
*
*
* @param deleteBotAliasRequest
* @return Result of the DeleteBotAlias operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ResourceInUseException
* The resource that you are attempting to delete is referred to by another resource. Use this information
* to remove references to the resource that you are trying to delete.
*
* The body of the exception contains a JSON object that describes the resource.
*
*
* { "resourceType": BOT | BOTALIAS | BOTCHANNEL | INTENT,
*
*
* "resourceReference": {
*
*
* "name": string, "version": string } }
* @sample AmazonLexModelBuilding.DeleteBotAlias
* @see AWS API
* Documentation
*/
DeleteBotAliasResult deleteBotAlias(DeleteBotAliasRequest deleteBotAliasRequest);
/**
*
* Deletes the association between an Amazon Lex bot and a messaging platform.
*
*
* This operation requires permission for the lex:DeleteBotChannelAssociation
action.
*
*
* @param deleteBotChannelAssociationRequest
* @return Result of the DeleteBotChannelAssociation operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.DeleteBotChannelAssociation
* @see AWS API Documentation
*/
DeleteBotChannelAssociationResult deleteBotChannelAssociation(DeleteBotChannelAssociationRequest deleteBotChannelAssociationRequest);
/**
*
* Deletes a specific version of a bot. To delete all versions of a bot, use the DeleteBot operation.
*
*
* This operation requires permissions for the lex:DeleteBotVersion
action.
*
*
* @param deleteBotVersionRequest
* @return Result of the DeleteBotVersion operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ResourceInUseException
* The resource that you are attempting to delete is referred to by another resource. Use this information
* to remove references to the resource that you are trying to delete.
*
* The body of the exception contains a JSON object that describes the resource.
*
*
* { "resourceType": BOT | BOTALIAS | BOTCHANNEL | INTENT,
*
*
* "resourceReference": {
*
*
* "name": string, "version": string } }
* @sample AmazonLexModelBuilding.DeleteBotVersion
* @see AWS
* API Documentation
*/
DeleteBotVersionResult deleteBotVersion(DeleteBotVersionRequest deleteBotVersionRequest);
/**
*
* Deletes all versions of the intent, including the $LATEST
version. To delete a specific version of
* the intent, use the DeleteIntentVersion operation.
*
*
* You can delete a version of an intent only if it is not referenced. To delete an intent that is referred to in
* one or more bots (see how-it-works), you must remove those references first.
*
*
*
* If you get the ResourceInUseException
exception, it provides an example reference that shows where
* the intent is referenced. To remove the reference to the intent, either update the bot or delete it. If you get
* the same exception when you attempt to delete the intent again, repeat until the intent has no references and the
* call to DeleteIntent
is successful.
*
*
*
* This operation requires permission for the lex:DeleteIntent
action.
*
*
* @param deleteIntentRequest
* @return Result of the DeleteIntent operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ResourceInUseException
* The resource that you are attempting to delete is referred to by another resource. Use this information
* to remove references to the resource that you are trying to delete.
*
* The body of the exception contains a JSON object that describes the resource.
*
*
* { "resourceType": BOT | BOTALIAS | BOTCHANNEL | INTENT,
*
*
* "resourceReference": {
*
*
* "name": string, "version": string } }
* @sample AmazonLexModelBuilding.DeleteIntent
* @see AWS API
* Documentation
*/
DeleteIntentResult deleteIntent(DeleteIntentRequest deleteIntentRequest);
/**
*
* Deletes a specific version of an intent. To delete all versions of a intent, use the DeleteIntent
* operation.
*
*
* This operation requires permissions for the lex:DeleteIntentVersion
action.
*
*
* @param deleteIntentVersionRequest
* @return Result of the DeleteIntentVersion operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ResourceInUseException
* The resource that you are attempting to delete is referred to by another resource. Use this information
* to remove references to the resource that you are trying to delete.
*
* The body of the exception contains a JSON object that describes the resource.
*
*
* { "resourceType": BOT | BOTALIAS | BOTCHANNEL | INTENT,
*
*
* "resourceReference": {
*
*
* "name": string, "version": string } }
* @sample AmazonLexModelBuilding.DeleteIntentVersion
* @see AWS
* API Documentation
*/
DeleteIntentVersionResult deleteIntentVersion(DeleteIntentVersionRequest deleteIntentVersionRequest);
/**
*
* Deletes all versions of the slot type, including the $LATEST
version. To delete a specific version
* of the slot type, use the DeleteSlotTypeVersion operation.
*
*
* You can delete a version of a slot type only if it is not referenced. To delete a slot type that is referred to
* in one or more intents, you must remove those references first.
*
*
*
* If you get the ResourceInUseException
exception, the exception provides an example reference that
* shows the intent where the slot type is referenced. To remove the reference to the slot type, either update the
* intent or delete it. If you get the same exception when you attempt to delete the slot type again, repeat until
* the slot type has no references and the DeleteSlotType
call is successful.
*
*
*
* This operation requires permission for the lex:DeleteSlotType
action.
*
*
* @param deleteSlotTypeRequest
* @return Result of the DeleteSlotType operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ResourceInUseException
* The resource that you are attempting to delete is referred to by another resource. Use this information
* to remove references to the resource that you are trying to delete.
*
* The body of the exception contains a JSON object that describes the resource.
*
*
* { "resourceType": BOT | BOTALIAS | BOTCHANNEL | INTENT,
*
*
* "resourceReference": {
*
*
* "name": string, "version": string } }
* @sample AmazonLexModelBuilding.DeleteSlotType
* @see AWS API
* Documentation
*/
DeleteSlotTypeResult deleteSlotType(DeleteSlotTypeRequest deleteSlotTypeRequest);
/**
*
* Deletes a specific version of a slot type. To delete all versions of a slot type, use the DeleteSlotType
* operation.
*
*
* This operation requires permissions for the lex:DeleteSlotTypeVersion
action.
*
*
* @param deleteSlotTypeVersionRequest
* @return Result of the DeleteSlotTypeVersion operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ResourceInUseException
* The resource that you are attempting to delete is referred to by another resource. Use this information
* to remove references to the resource that you are trying to delete.
*
* The body of the exception contains a JSON object that describes the resource.
*
*
* { "resourceType": BOT | BOTALIAS | BOTCHANNEL | INTENT,
*
*
* "resourceReference": {
*
*
* "name": string, "version": string } }
* @sample AmazonLexModelBuilding.DeleteSlotTypeVersion
* @see AWS API Documentation
*/
DeleteSlotTypeVersionResult deleteSlotTypeVersion(DeleteSlotTypeVersionRequest deleteSlotTypeVersionRequest);
/**
*
* Deletes stored utterances.
*
*
* Amazon Lex stores the utterances that users send to your bot. Utterances are stored for 15 days for use with the
* GetUtterancesView operation, and then stored indefinitely for use in improving the ability of your bot to
* respond to user input.
*
*
* Use the DeleteUtterances
operation to manually delete stored utterances for a specific user. When
* you use the DeleteUtterances
operation, utterances stored for improving your bot's ability to
* respond to user input are deleted immediately. Utterances stored for use with the GetUtterancesView
* operation are deleted after 15 days.
*
*
* This operation requires permissions for the lex:DeleteUtterances
action.
*
*
* @param deleteUtterancesRequest
* @return Result of the DeleteUtterances operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.DeleteUtterances
* @see AWS
* API Documentation
*/
DeleteUtterancesResult deleteUtterances(DeleteUtterancesRequest deleteUtterancesRequest);
/**
*
* Returns metadata information for a specific bot. You must provide the bot name and the bot version or alias.
*
*
* This operation requires permissions for the lex:GetBot
action.
*
*
* @param getBotRequest
* @return Result of the GetBot operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBot
* @see AWS API
* Documentation
*/
GetBotResult getBot(GetBotRequest getBotRequest);
/**
*
* Returns information about an Amazon Lex bot alias. For more information about aliases, see
* versioning-aliases.
*
*
* This operation requires permissions for the lex:GetBotAlias
action.
*
*
* @param getBotAliasRequest
* @return Result of the GetBotAlias operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBotAlias
* @see AWS API
* Documentation
*/
GetBotAliasResult getBotAlias(GetBotAliasRequest getBotAliasRequest);
/**
*
* Returns a list of aliases for a specified Amazon Lex bot.
*
*
* This operation requires permissions for the lex:GetBotAliases
action.
*
*
* @param getBotAliasesRequest
* @return Result of the GetBotAliases operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBotAliases
* @see AWS API
* Documentation
*/
GetBotAliasesResult getBotAliases(GetBotAliasesRequest getBotAliasesRequest);
/**
*
* Returns information about the association between an Amazon Lex bot and a messaging platform.
*
*
* This operation requires permissions for the lex:GetBotChannelAssociation
action.
*
*
* @param getBotChannelAssociationRequest
* @return Result of the GetBotChannelAssociation operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBotChannelAssociation
* @see AWS API Documentation
*/
GetBotChannelAssociationResult getBotChannelAssociation(GetBotChannelAssociationRequest getBotChannelAssociationRequest);
/**
*
* Returns a list of all of the channels associated with the specified bot.
*
*
* The GetBotChannelAssociations
operation requires permissions for the
* lex:GetBotChannelAssociations
action.
*
*
* @param getBotChannelAssociationsRequest
* @return Result of the GetBotChannelAssociations operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBotChannelAssociations
* @see AWS API Documentation
*/
GetBotChannelAssociationsResult getBotChannelAssociations(GetBotChannelAssociationsRequest getBotChannelAssociationsRequest);
/**
*
* Gets information about all of the versions of a bot.
*
*
* The GetBotVersions
operation returns a BotMetadata
object for each version of a bot.
* For example, if a bot has three numbered versions, the GetBotVersions
operation returns four
* BotMetadata
objects in the response, one for each numbered version and one for the
* $LATEST
version.
*
*
* The GetBotVersions
operation always returns at least one version, the $LATEST
version.
*
*
* This operation requires permissions for the lex:GetBotVersions
action.
*
*
* @param getBotVersionsRequest
* @return Result of the GetBotVersions operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBotVersions
* @see AWS API
* Documentation
*/
GetBotVersionsResult getBotVersions(GetBotVersionsRequest getBotVersionsRequest);
/**
*
* Returns bot information as follows:
*
*
* -
*
* If you provide the nameContains
field, the response includes information for the
* $LATEST
version of all bots whose name contains the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, the operation returns information about the
* $LATEST
version of all of your bots.
*
*
*
*
* This operation requires permission for the lex:GetBots
action.
*
*
* @param getBotsRequest
* @return Result of the GetBots operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBots
* @see AWS API
* Documentation
*/
GetBotsResult getBots(GetBotsRequest getBotsRequest);
/**
*
* Returns information about a built-in intent.
*
*
* This operation requires permission for the lex:GetBuiltinIntent
action.
*
*
* @param getBuiltinIntentRequest
* @return Result of the GetBuiltinIntent operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBuiltinIntent
* @see AWS
* API Documentation
*/
GetBuiltinIntentResult getBuiltinIntent(GetBuiltinIntentRequest getBuiltinIntentRequest);
/**
*
* Gets a list of built-in intents that meet the specified criteria.
*
*
* This operation requires permission for the lex:GetBuiltinIntents
action.
*
*
* @param getBuiltinIntentsRequest
* @return Result of the GetBuiltinIntents operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBuiltinIntents
* @see AWS
* API Documentation
*/
GetBuiltinIntentsResult getBuiltinIntents(GetBuiltinIntentsRequest getBuiltinIntentsRequest);
/**
*
* Gets a list of built-in slot types that meet the specified criteria.
*
*
* For a list of built-in slot types, see Slot Type Reference in the Alexa Skills Kit.
*
*
* This operation requires permission for the lex:GetBuiltInSlotTypes
action.
*
*
* @param getBuiltinSlotTypesRequest
* @return Result of the GetBuiltinSlotTypes operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetBuiltinSlotTypes
* @see AWS
* API Documentation
*/
GetBuiltinSlotTypesResult getBuiltinSlotTypes(GetBuiltinSlotTypesRequest getBuiltinSlotTypesRequest);
/**
*
* Exports the contents of a Amazon Lex resource in a specified format.
*
*
* @param getExportRequest
* @return Result of the GetExport operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetExport
* @see AWS API
* Documentation
*/
GetExportResult getExport(GetExportRequest getExportRequest);
/**
*
* Gets information about an import job started with the StartImport
operation.
*
*
* @param getImportRequest
* @return Result of the GetImport operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetImport
* @see AWS API
* Documentation
*/
GetImportResult getImport(GetImportRequest getImportRequest);
/**
*
* Returns information about an intent. In addition to the intent name, you must specify the intent version.
*
*
* This operation requires permissions to perform the lex:GetIntent
action.
*
*
* @param getIntentRequest
* @return Result of the GetIntent operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetIntent
* @see AWS API
* Documentation
*/
GetIntentResult getIntent(GetIntentRequest getIntentRequest);
/**
*
* Gets information about all of the versions of an intent.
*
*
* The GetIntentVersions
operation returns an IntentMetadata
object for each version of an
* intent. For example, if an intent has three numbered versions, the GetIntentVersions
operation
* returns four IntentMetadata
objects in the response, one for each numbered version and one for the
* $LATEST
version.
*
*
* The GetIntentVersions
operation always returns at least one version, the $LATEST
* version.
*
*
* This operation requires permissions for the lex:GetIntentVersions
action.
*
*
* @param getIntentVersionsRequest
* @return Result of the GetIntentVersions operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetIntentVersions
* @see AWS
* API Documentation
*/
GetIntentVersionsResult getIntentVersions(GetIntentVersionsRequest getIntentVersionsRequest);
/**
*
* Returns intent information as follows:
*
*
* -
*
* If you specify the nameContains
field, returns the $LATEST
version of all intents that
* contain the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, returns information about the $LATEST
* version of all intents.
*
*
*
*
* The operation requires permission for the lex:GetIntents
action.
*
*
* @param getIntentsRequest
* @return Result of the GetIntents operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetIntents
* @see AWS API
* Documentation
*/
GetIntentsResult getIntents(GetIntentsRequest getIntentsRequest);
/**
*
* Provides details about an ongoing or complete migration from an Amazon Lex V1 bot to an Amazon Lex V2 bot. Use
* this operation to view the migration alerts and warnings related to the migration.
*
*
* @param getMigrationRequest
* @return Result of the GetMigration operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @sample AmazonLexModelBuilding.GetMigration
* @see AWS API
* Documentation
*/
GetMigrationResult getMigration(GetMigrationRequest getMigrationRequest);
/**
*
* Gets a list of migrations between Amazon Lex V1 and Amazon Lex V2.
*
*
* @param getMigrationsRequest
* @return Result of the GetMigrations operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetMigrations
* @see AWS API
* Documentation
*/
GetMigrationsResult getMigrations(GetMigrationsRequest getMigrationsRequest);
/**
*
* Returns information about a specific version of a slot type. In addition to specifying the slot type name, you
* must specify the slot type version.
*
*
* This operation requires permissions for the lex:GetSlotType
action.
*
*
* @param getSlotTypeRequest
* @return Result of the GetSlotType operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetSlotType
* @see AWS API
* Documentation
*/
GetSlotTypeResult getSlotType(GetSlotTypeRequest getSlotTypeRequest);
/**
*
* Gets information about all versions of a slot type.
*
*
* The GetSlotTypeVersions
operation returns a SlotTypeMetadata
object for each version of
* a slot type. For example, if a slot type has three numbered versions, the GetSlotTypeVersions
* operation returns four SlotTypeMetadata
objects in the response, one for each numbered version and
* one for the $LATEST
version.
*
*
* The GetSlotTypeVersions
operation always returns at least one version, the $LATEST
* version.
*
*
* This operation requires permissions for the lex:GetSlotTypeVersions
action.
*
*
* @param getSlotTypeVersionsRequest
* @return Result of the GetSlotTypeVersions operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetSlotTypeVersions
* @see AWS
* API Documentation
*/
GetSlotTypeVersionsResult getSlotTypeVersions(GetSlotTypeVersionsRequest getSlotTypeVersionsRequest);
/**
*
* Returns slot type information as follows:
*
*
* -
*
* If you specify the nameContains
field, returns the $LATEST
version of all slot types
* that contain the specified string.
*
*
* -
*
* If you don't specify the nameContains
field, returns information about the $LATEST
* version of all slot types.
*
*
*
*
* The operation requires permission for the lex:GetSlotTypes
action.
*
*
* @param getSlotTypesRequest
* @return Result of the GetSlotTypes operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetSlotTypes
* @see AWS API
* Documentation
*/
GetSlotTypesResult getSlotTypes(GetSlotTypesRequest getSlotTypesRequest);
/**
*
* Use the GetUtterancesView
operation to get information about the utterances that your users have
* made to your bot. You can use this list to tune the utterances that your bot responds to.
*
*
* For example, say that you have created a bot to order flowers. After your users have used your bot for a while,
* use the GetUtterancesView
operation to see the requests that they have made and whether they have
* been successful. You might find that the utterance "I want flowers" is not being recognized. You could add this
* utterance to the OrderFlowers
intent so that your bot recognizes that utterance.
*
*
* After you publish a new version of a bot, you can get information about the old version and the new so that you
* can compare the performance across the two versions.
*
*
* Utterance statistics are generated once a day. Data is available for the last 15 days. You can request
* information for up to 5 versions of your bot in each request. Amazon Lex returns the most frequent utterances
* received by the bot in the last 15 days. The response contains information about a maximum of 100 utterances for
* each version.
*
*
* If you set childDirected
field to true when you created your bot, if you are using slot obfuscation
* with one or more slots, or if you opted out of participating in improving Amazon Lex, utterances are not
* available.
*
*
* This operation requires permissions for the lex:GetUtterancesView
action.
*
*
* @param getUtterancesViewRequest
* @return Result of the GetUtterancesView operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.GetUtterancesView
* @see AWS
* API Documentation
*/
GetUtterancesViewResult getUtterancesView(GetUtterancesViewRequest getUtterancesViewRequest);
/**
*
* Gets a list of tags associated with the specified resource. Only bots, bot aliases, and bot channels can have
* tags associated with them.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @sample AmazonLexModelBuilding.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates an Amazon Lex conversational bot or replaces an existing bot. When you create or update a bot you are
* only required to specify a name, a locale, and whether the bot is directed toward children under age 13. You can
* use this to add intents later, or to remove intents from an existing bot. When you create a bot with the minimum
* information, the bot is created or updated but Amazon Lex returns the
response FAILED
. You
* can build the bot after you add one or more intents. For more information about Amazon Lex bots, see
* how-it-works.
*
*
* If you specify the name of an existing bot, the fields in the request replace the existing values in the
* $LATEST
version of the bot. Amazon Lex removes any fields that you don't provide values for in the
* request, except for the idleTTLInSeconds
and privacySettings
fields, which are set to
* their default values. If you don't specify values for required fields, Amazon Lex throws an exception.
*
*
* This operation requires permissions for the lex:PutBot
action. For more information, see
* security-iam.
*
*
* @param putBotRequest
* @return Result of the PutBot operation returned by the service.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws PreconditionFailedException
* The checksum of the resource that you are trying to change does not match the checksum in the request.
* Check the resource's checksum and try again.
* @sample AmazonLexModelBuilding.PutBot
* @see AWS API
* Documentation
*/
PutBotResult putBot(PutBotRequest putBotRequest);
/**
*
* Creates an alias for the specified version of the bot or replaces an alias for the specified bot. To change the
* version of the bot that the alias points to, replace the alias. For more information about aliases, see
* versioning-aliases.
*
*
* This operation requires permissions for the lex:PutBotAlias
action.
*
*
* @param putBotAliasRequest
* @return Result of the PutBotAlias operation returned by the service.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws PreconditionFailedException
* The checksum of the resource that you are trying to change does not match the checksum in the request.
* Check the resource's checksum and try again.
* @sample AmazonLexModelBuilding.PutBotAlias
* @see AWS API
* Documentation
*/
PutBotAliasResult putBotAlias(PutBotAliasRequest putBotAliasRequest);
/**
*
* Creates an intent or replaces an existing intent.
*
*
* To define the interaction between the user and your bot, you use one or more intents. For a pizza ordering bot,
* for example, you would create an OrderPizza
intent.
*
*
* To create an intent or replace an existing intent, you must provide the following:
*
*
* -
*
* Intent name. For example, OrderPizza
.
*
*
* -
*
* Sample utterances. For example, "Can I order a pizza, please." and "I want to order a pizza."
*
*
* -
*
* Information to be gathered. You specify slot types for the information that your bot will request from the user.
* You can specify standard slot types, such as a date or a time, or custom slot types such as the size and crust of
* a pizza.
*
*
* -
*
* How the intent will be fulfilled. You can provide a Lambda function or configure the intent to return the intent
* information to the client application. If you use a Lambda function, when all of the intent information is
* available, Amazon Lex invokes your Lambda function. If you configure your intent to return the intent information
* to the client application.
*
*
*
*
* You can specify other optional information in the request, such as:
*
*
* -
*
* A confirmation prompt to ask the user to confirm an intent. For example, "Shall I order your pizza?"
*
*
* -
*
* A conclusion statement to send to the user after the intent has been fulfilled. For example,
* "I placed your pizza order."
*
*
* -
*
* A follow-up prompt that asks the user for additional activity. For example, asking
* "Do you want to order a drink with your pizza?"
*
*
*
*
* If you specify an existing intent name to update the intent, Amazon Lex replaces the values in the
* $LATEST
version of the intent with the values in the request. Amazon Lex removes fields that you
* don't provide in the request. If you don't specify the required fields, Amazon Lex throws an exception. When you
* update the $LATEST
version of an intent, the status
field of any bot that uses the
* $LATEST
version of the intent is set to NOT_BUILT
.
*
*
* For more information, see how-it-works.
*
*
* This operation requires permissions for the lex:PutIntent
action.
*
*
* @param putIntentRequest
* @return Result of the PutIntent operation returned by the service.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws PreconditionFailedException
* The checksum of the resource that you are trying to change does not match the checksum in the request.
* Check the resource's checksum and try again.
* @sample AmazonLexModelBuilding.PutIntent
* @see AWS API
* Documentation
*/
PutIntentResult putIntent(PutIntentRequest putIntentRequest);
/**
*
* Creates a custom slot type or replaces an existing custom slot type.
*
*
* To create a custom slot type, specify a name for the slot type and a set of enumeration values, which are the
* values that a slot of this type can assume. For more information, see how-it-works.
*
*
* If you specify the name of an existing slot type, the fields in the request replace the existing values in the
* $LATEST
version of the slot type. Amazon Lex removes the fields that you don't provide in the
* request. If you don't specify required fields, Amazon Lex throws an exception. When you update the
* $LATEST
version of a slot type, if a bot uses the $LATEST
version of an intent that
* contains the slot type, the bot's status
field is set to NOT_BUILT
.
*
*
* This operation requires permissions for the lex:PutSlotType
action.
*
*
* @param putSlotTypeRequest
* @return Result of the PutSlotType operation returned by the service.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws PreconditionFailedException
* The checksum of the resource that you are trying to change does not match the checksum in the request.
* Check the resource's checksum and try again.
* @sample AmazonLexModelBuilding.PutSlotType
* @see AWS API
* Documentation
*/
PutSlotTypeResult putSlotType(PutSlotTypeRequest putSlotTypeRequest);
/**
*
* Starts a job to import a resource to Amazon Lex.
*
*
* @param startImportRequest
* @return Result of the StartImport operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @sample AmazonLexModelBuilding.StartImport
* @see AWS API
* Documentation
*/
StartImportResult startImport(StartImportRequest startImportRequest);
/**
*
* Starts migrating a bot from Amazon Lex V1 to Amazon Lex V2. Migrate your bot when you want to take advantage of
* the new features of Amazon Lex V2.
*
*
* For more information, see Migrating a bot in
* the Amazon Lex developer guide.
*
*
* @param startMigrationRequest
* @return Result of the StartMigration operation returned by the service.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws AccessDeniedException
* Your IAM user or role does not have permission to call the Amazon Lex V2 APIs required to migrate your
* bot.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @sample AmazonLexModelBuilding.StartMigration
* @see AWS API
* Documentation
*/
StartMigrationResult startMigration(StartMigrationRequest startMigrationRequest);
/**
*
* Adds the specified tags to the specified resource. If a tag key already exists, the existing value is replaced
* with the new value.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @sample AmazonLexModelBuilding.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from a bot, bot alias or bot channel.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws NotFoundException
* The resource specified in the request was not found. Check the resource and try again.
* @throws BadRequestException
* The request is not well formed. For example, a value is invalid or a required field is missing. Check the
* field values, and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws InternalFailureException
* An internal Amazon Lex error occurred. Try your request again.
* @throws LimitExceededException
* The request exceeded a limit. Try your request again.
* @sample AmazonLexModelBuilding.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}