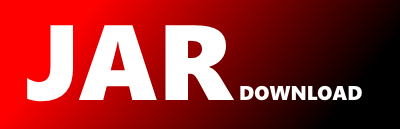
com.amazonaws.services.lexmodelbuilding.model.StartMigrationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lexmodelbuilding Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lexmodelbuilding.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartMigrationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*
*/
private String v1BotName;
/**
*
* The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as well as
* any numbered version.
*
*/
private String v1BotVersion;
/**
*
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to change the
* contents of the Amazon Lex V2 bot.
*
*
*
*/
private String v2BotName;
/**
*
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*
*/
private String v2BotRole;
/**
*
* The strategy used to conduct the migration.
*
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated.
* It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is
* created in the Amazon Lex V2 bot.
*
*
*
*/
private String migrationStrategy;
/**
*
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*
*
* @param v1BotName
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*/
public void setV1BotName(String v1BotName) {
this.v1BotName = v1BotName;
}
/**
*
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*
*
* @return The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*/
public String getV1BotName() {
return this.v1BotName;
}
/**
*
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*
*
* @param v1BotName
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMigrationRequest withV1BotName(String v1BotName) {
setV1BotName(v1BotName);
return this;
}
/**
*
* The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as well as
* any numbered version.
*
*
* @param v1BotVersion
* The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as
* well as any numbered version.
*/
public void setV1BotVersion(String v1BotVersion) {
this.v1BotVersion = v1BotVersion;
}
/**
*
* The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as well as
* any numbered version.
*
*
* @return The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as
* well as any numbered version.
*/
public String getV1BotVersion() {
return this.v1BotVersion;
}
/**
*
* The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as well as
* any numbered version.
*
*
* @param v1BotVersion
* The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as
* well as any numbered version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMigrationRequest withV1BotVersion(String v1BotVersion) {
setV1BotVersion(v1BotVersion);
return this;
}
/**
*
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to change the
* contents of the Amazon Lex V2 bot.
*
*
*
*
* @param v2BotName
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to
* change the contents of the Amazon Lex V2 bot.
*
*
*/
public void setV2BotName(String v2BotName) {
this.v2BotName = v2BotName;
}
/**
*
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to change the
* contents of the Amazon Lex V2 bot.
*
*
*
*
* @return The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to
* change the contents of the Amazon Lex V2 bot.
*
*
*/
public String getV2BotName() {
return this.v2BotName;
}
/**
*
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to change the
* contents of the Amazon Lex V2 bot.
*
*
*
*
* @param v2BotName
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to
* change the contents of the Amazon Lex V2 bot.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMigrationRequest withV2BotName(String v2BotName) {
setV2BotName(v2BotName);
return this;
}
/**
*
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*
*
* @param v2BotRole
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*/
public void setV2BotRole(String v2BotRole) {
this.v2BotRole = v2BotRole;
}
/**
*
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*
*
* @return The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*/
public String getV2BotRole() {
return this.v2BotRole;
}
/**
*
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*
*
* @param v2BotRole
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMigrationRequest withV2BotRole(String v2BotRole) {
setV2BotRole(v2BotRole);
return this;
}
/**
*
* The strategy used to conduct the migration.
*
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated.
* It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is
* created in the Amazon Lex V2 bot.
*
*
*
*
* @param migrationStrategy
* The strategy used to conduct the migration.
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new
* bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being
* migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new
* locale is created in the Amazon Lex V2 bot.
*
*
* @see MigrationStrategy
*/
public void setMigrationStrategy(String migrationStrategy) {
this.migrationStrategy = migrationStrategy;
}
/**
*
* The strategy used to conduct the migration.
*
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated.
* It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is
* created in the Amazon Lex V2 bot.
*
*
*
*
* @return The strategy used to conduct the migration.
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new
* bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being
* migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a
* new locale is created in the Amazon Lex V2 bot.
*
*
* @see MigrationStrategy
*/
public String getMigrationStrategy() {
return this.migrationStrategy;
}
/**
*
* The strategy used to conduct the migration.
*
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated.
* It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is
* created in the Amazon Lex V2 bot.
*
*
*
*
* @param migrationStrategy
* The strategy used to conduct the migration.
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new
* bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being
* migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new
* locale is created in the Amazon Lex V2 bot.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MigrationStrategy
*/
public StartMigrationRequest withMigrationStrategy(String migrationStrategy) {
setMigrationStrategy(migrationStrategy);
return this;
}
/**
*
* The strategy used to conduct the migration.
*
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated.
* It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is
* created in the Amazon Lex V2 bot.
*
*
*
*
* @param migrationStrategy
* The strategy used to conduct the migration.
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new
* bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being
* migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new
* locale is created in the Amazon Lex V2 bot.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MigrationStrategy
*/
public StartMigrationRequest withMigrationStrategy(MigrationStrategy migrationStrategy) {
this.migrationStrategy = migrationStrategy.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getV1BotName() != null)
sb.append("V1BotName: ").append(getV1BotName()).append(",");
if (getV1BotVersion() != null)
sb.append("V1BotVersion: ").append(getV1BotVersion()).append(",");
if (getV2BotName() != null)
sb.append("V2BotName: ").append(getV2BotName()).append(",");
if (getV2BotRole() != null)
sb.append("V2BotRole: ").append(getV2BotRole()).append(",");
if (getMigrationStrategy() != null)
sb.append("MigrationStrategy: ").append(getMigrationStrategy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartMigrationRequest == false)
return false;
StartMigrationRequest other = (StartMigrationRequest) obj;
if (other.getV1BotName() == null ^ this.getV1BotName() == null)
return false;
if (other.getV1BotName() != null && other.getV1BotName().equals(this.getV1BotName()) == false)
return false;
if (other.getV1BotVersion() == null ^ this.getV1BotVersion() == null)
return false;
if (other.getV1BotVersion() != null && other.getV1BotVersion().equals(this.getV1BotVersion()) == false)
return false;
if (other.getV2BotName() == null ^ this.getV2BotName() == null)
return false;
if (other.getV2BotName() != null && other.getV2BotName().equals(this.getV2BotName()) == false)
return false;
if (other.getV2BotRole() == null ^ this.getV2BotRole() == null)
return false;
if (other.getV2BotRole() != null && other.getV2BotRole().equals(this.getV2BotRole()) == false)
return false;
if (other.getMigrationStrategy() == null ^ this.getMigrationStrategy() == null)
return false;
if (other.getMigrationStrategy() != null && other.getMigrationStrategy().equals(this.getMigrationStrategy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getV1BotName() == null) ? 0 : getV1BotName().hashCode());
hashCode = prime * hashCode + ((getV1BotVersion() == null) ? 0 : getV1BotVersion().hashCode());
hashCode = prime * hashCode + ((getV2BotName() == null) ? 0 : getV2BotName().hashCode());
hashCode = prime * hashCode + ((getV2BotRole() == null) ? 0 : getV2BotRole().hashCode());
hashCode = prime * hashCode + ((getMigrationStrategy() == null) ? 0 : getMigrationStrategy().hashCode());
return hashCode;
}
@Override
public StartMigrationRequest clone() {
return (StartMigrationRequest) super.clone();
}
}