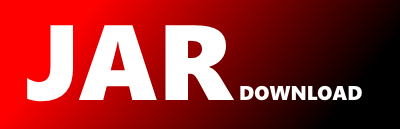
com.amazonaws.services.licensemanagerlinuxsubscriptions.AWSLicenseManagerLinuxSubscriptions Maven / Gradle / Ivy
Show all versions of aws-java-sdk-licensemanagerlinuxsubscriptions Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.licensemanagerlinuxsubscriptions;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.licensemanagerlinuxsubscriptions.model.*;
/**
* Interface for accessing AWS License Manager Linux Subscriptions.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.licensemanagerlinuxsubscriptions.AbstractAWSLicenseManagerLinuxSubscriptions} instead.
*
*
*
* With License Manager, you can discover and track your commercial Linux subscriptions on running Amazon EC2 instances.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSLicenseManagerLinuxSubscriptions {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "license-manager-linux-subscriptions";
/**
*
* Remove a third-party subscription provider from the Bring Your Own License (BYOL) subscriptions registered to
* your account.
*
*
* @param deregisterSubscriptionProviderRequest
* @return Result of the DeregisterSubscriptionProvider operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ResourceNotFoundException
* Unable to find the requested Amazon Web Services resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.DeregisterSubscriptionProvider
* @see AWS API Documentation
*/
DeregisterSubscriptionProviderResult deregisterSubscriptionProvider(DeregisterSubscriptionProviderRequest deregisterSubscriptionProviderRequest);
/**
*
* Get details for a Bring Your Own License (BYOL) subscription that's registered to your account.
*
*
* @param getRegisteredSubscriptionProviderRequest
* @return Result of the GetRegisteredSubscriptionProvider operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ResourceNotFoundException
* Unable to find the requested Amazon Web Services resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.GetRegisteredSubscriptionProvider
* @see AWS API Documentation
*/
GetRegisteredSubscriptionProviderResult getRegisteredSubscriptionProvider(GetRegisteredSubscriptionProviderRequest getRegisteredSubscriptionProviderRequest);
/**
*
* Lists the Linux subscriptions service settings for your account.
*
*
* @param getServiceSettingsRequest
* @return Result of the GetServiceSettings operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.GetServiceSettings
* @see AWS API Documentation
*/
GetServiceSettingsResult getServiceSettings(GetServiceSettingsRequest getServiceSettingsRequest);
/**
*
* Lists the running Amazon EC2 instances that were discovered with commercial Linux subscriptions.
*
*
* @param listLinuxSubscriptionInstancesRequest
* NextToken length limit is half of ddb accepted limit. Increase this limit if parameters in request
* increases.
* @return Result of the ListLinuxSubscriptionInstances operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.ListLinuxSubscriptionInstances
* @see AWS API Documentation
*/
ListLinuxSubscriptionInstancesResult listLinuxSubscriptionInstances(ListLinuxSubscriptionInstancesRequest listLinuxSubscriptionInstancesRequest);
/**
*
* Lists the Linux subscriptions that have been discovered. If you have linked your organization, the returned
* results will include data aggregated across your accounts in Organizations.
*
*
* @param listLinuxSubscriptionsRequest
* NextToken length limit is half of ddb accepted limit. Increase this limit if parameters in request
* increases.
* @return Result of the ListLinuxSubscriptions operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.ListLinuxSubscriptions
* @see AWS API Documentation
*/
ListLinuxSubscriptionsResult listLinuxSubscriptions(ListLinuxSubscriptionsRequest listLinuxSubscriptionsRequest);
/**
*
* List Bring Your Own License (BYOL) subscription registration resources for your account.
*
*
* @param listRegisteredSubscriptionProvidersRequest
* @return Result of the ListRegisteredSubscriptionProviders operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.ListRegisteredSubscriptionProviders
* @see AWS API Documentation
*/
ListRegisteredSubscriptionProvidersResult listRegisteredSubscriptionProviders(
ListRegisteredSubscriptionProvidersRequest listRegisteredSubscriptionProvidersRequest);
/**
*
* List the metadata tags that are assigned to the specified Amazon Web Services resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ResourceNotFoundException
* Unable to find the requested Amazon Web Services resource.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Register the supported third-party subscription provider for your Bring Your Own License (BYOL) subscription.
*
*
* @param registerSubscriptionProviderRequest
* @return Result of the RegisterSubscriptionProvider operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.RegisterSubscriptionProvider
* @see AWS API Documentation
*/
RegisterSubscriptionProviderResult registerSubscriptionProvider(RegisterSubscriptionProviderRequest registerSubscriptionProviderRequest);
/**
*
* Add metadata tags to the specified Amazon Web Services resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ResourceNotFoundException
* Unable to find the requested Amazon Web Services resource.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.TagResource
* @see AWS API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Remove one or more metadata tag from the specified Amazon Web Services resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ResourceNotFoundException
* Unable to find the requested Amazon Web Services resource.
* @sample AWSLicenseManagerLinuxSubscriptions.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the service settings for Linux subscriptions.
*
*
* @param updateServiceSettingsRequest
* @return Result of the UpdateServiceSettings operation returned by the service.
* @throws InternalServerException
* An exception occurred with the service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ValidationException
* The provided input is not valid. Try your request again.
* @sample AWSLicenseManagerLinuxSubscriptions.UpdateServiceSettings
* @see AWS API Documentation
*/
UpdateServiceSettingsResult updateServiceSettings(UpdateServiceSettingsRequest updateServiceSettingsRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}