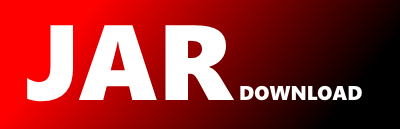
com.amazonaws.services.location.model.CalculateRouteMatrixRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CalculateRouteMatrixRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the route calculator resource that you want to use to calculate the route matrix.
*
*/
private String calculatorName;
/**
*
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*/
private java.util.List> departurePositions;
/**
*
* The list of destination positions for the route matrix. An array of points, each of which is itself a 2-value
* array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*/
private java.util.List> destinationPositions;
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data provider,
* and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
*
*/
private String travelMode;
/**
*
* Specifies the desired time of departure. Uses the given time to calculate the route matrix. You can't set both
* DepartureTime
and DepartNow
. If neither is set, the best time of day to travel with the
* best traffic conditions is used to calculate the route matrix.
*
*
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*
*/
private java.util.Date departureTime;
/**
*
* Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You can't
* set both DepartureTime
and DepartNow
. If neither is set, the best time of day to travel
* with the best traffic conditions is used to calculate the route matrix.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*/
private Boolean departNow;
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*/
private String distanceUnit;
/**
*
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries or
* tolls.
*
*
* Requirements: TravelMode
must be specified as Car
.
*
*/
private CalculateRouteCarModeOptions carModeOptions;
/**
*
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries or
* tolls, and truck specifications to consider when choosing an optimal road.
*
*
* Requirements: TravelMode
must be specified as Truck
.
*
*/
private CalculateRouteTruckModeOptions truckModeOptions;
/**
*
* The optional API key
* to authorize the request.
*
*/
private String key;
/**
*
* The name of the route calculator resource that you want to use to calculate the route matrix.
*
*
* @param calculatorName
* The name of the route calculator resource that you want to use to calculate the route matrix.
*/
public void setCalculatorName(String calculatorName) {
this.calculatorName = calculatorName;
}
/**
*
* The name of the route calculator resource that you want to use to calculate the route matrix.
*
*
* @return The name of the route calculator resource that you want to use to calculate the route matrix.
*/
public String getCalculatorName() {
return this.calculatorName;
}
/**
*
* The name of the route calculator resource that you want to use to calculate the route matrix.
*
*
* @param calculatorName
* The name of the route calculator resource that you want to use to calculate the route matrix.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withCalculatorName(String calculatorName) {
setCalculatorName(calculatorName);
return this;
}
/**
*
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @return The list of departure (origin) positions for the route matrix. An array of points, each of which is
* itself a 2-value array defined in WGS 84
* format: [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located
* on a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*/
public java.util.List> getDeparturePositions() {
return departurePositions;
}
/**
*
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param departurePositions
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself
* a 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on
* a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*/
public void setDeparturePositions(java.util.Collection> departurePositions) {
if (departurePositions == null) {
this.departurePositions = null;
return;
}
this.departurePositions = new java.util.ArrayList>(departurePositions);
}
/**
*
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDeparturePositions(java.util.Collection)} or {@link #withDeparturePositions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param departurePositions
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself
* a 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on
* a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withDeparturePositions(java.util.List... departurePositions) {
if (this.departurePositions == null) {
setDeparturePositions(new java.util.ArrayList>(departurePositions.length));
}
for (java.util.List ele : departurePositions) {
this.departurePositions.add(ele);
}
return this;
}
/**
*
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param departurePositions
* The list of departure (origin) positions for the route matrix. An array of points, each of which is itself
* a 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-123.115, 49.285]
.
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a departure that's not located on
* a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDeparturePositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withDeparturePositions(java.util.Collection> departurePositions) {
setDeparturePositions(departurePositions);
return this;
}
/**
*
* The list of destination positions for the route matrix. An array of points, each of which is itself a 2-value
* array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @return The list of destination positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located
* on a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*/
public java.util.List> getDestinationPositions() {
return destinationPositions;
}
/**
*
* The list of destination positions for the route matrix. An array of points, each of which is itself a 2-value
* array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param destinationPositions
* The list of destination positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located
* on a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*/
public void setDestinationPositions(java.util.Collection> destinationPositions) {
if (destinationPositions == null) {
this.destinationPositions = null;
return;
}
this.destinationPositions = new java.util.ArrayList>(destinationPositions);
}
/**
*
* The list of destination positions for the route matrix. An array of points, each of which is itself a 2-value
* array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDestinationPositions(java.util.Collection)} or {@link #withDestinationPositions(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param destinationPositions
* The list of destination positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located
* on a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withDestinationPositions(java.util.List... destinationPositions) {
if (this.destinationPositions == null) {
setDestinationPositions(new java.util.ArrayList>(destinationPositions.length));
}
for (java.util.List ele : destinationPositions) {
this.destinationPositions.add(ele);
}
return this;
}
/**
*
* The list of destination positions for the route matrix. An array of points, each of which is itself a 2-value
* array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
*
*
* Depending on the data provider selected in the route calculator resource there may be additional restrictions on
* the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located on a
* road, Amazon Location moves the position to
* the nearest road. The snapped value is available in the result in SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param destinationPositions
* The list of destination positions for the route matrix. An array of points, each of which is itself a
* 2-value array defined in WGS 84 format:
* [longitude, latitude]
. For example, [-122.339, 47.615]
*
* Depending on the data provider selected in the route calculator resource there may be additional
* restrictions on the inputs you can choose. See Position restrictions in the Amazon Location Service Developer Guide.
*
*
*
* For route calculators that use Esri as the data provider, if you specify a destination that's not located
* on a road, Amazon Location moves the
* position to the nearest road. The snapped value is available in the result in
* SnappedDestinationPositions
.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withDestinationPositions(java.util.Collection> destinationPositions) {
setDestinationPositions(destinationPositions);
return this;
}
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data provider,
* and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
*
*
* @param travelMode
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data
* provider, and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
* @see TravelMode
*/
public void setTravelMode(String travelMode) {
this.travelMode = travelMode;
}
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data provider,
* and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
*
*
* @return Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data
* provider, and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
* @see TravelMode
*/
public String getTravelMode() {
return this.travelMode;
}
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data provider,
* and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
*
*
* @param travelMode
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data
* provider, and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
* @return Returns a reference to this object so that method calls can be chained together.
* @see TravelMode
*/
public CalculateRouteMatrixRequest withTravelMode(String travelMode) {
setTravelMode(travelMode);
return this;
}
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data provider,
* and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
*
*
* @param travelMode
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
*
* Bicycle
or Motorcycle
are only valid when using Grab
as a data
* provider, and only within Southeast Asia.
*
*
* Truck
is not available for Grab.
*
*
* For more information about using Grab as a data provider, see GrabMaps in the Amazon
* Location Service Developer Guide.
*
*
*
* Default Value: Car
* @return Returns a reference to this object so that method calls can be chained together.
* @see TravelMode
*/
public CalculateRouteMatrixRequest withTravelMode(TravelMode travelMode) {
this.travelMode = travelMode.toString();
return this;
}
/**
*
* Specifies the desired time of departure. Uses the given time to calculate the route matrix. You can't set both
* DepartureTime
and DepartNow
. If neither is set, the best time of day to travel with the
* best traffic conditions is used to calculate the route matrix.
*
*
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*
*
* @param departureTime
* Specifies the desired time of departure. Uses the given time to calculate the route matrix. You can't set
* both DepartureTime
and DepartNow
. If neither is set, the best time of day to
* travel with the best traffic conditions is used to calculate the route matrix.
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*/
public void setDepartureTime(java.util.Date departureTime) {
this.departureTime = departureTime;
}
/**
*
* Specifies the desired time of departure. Uses the given time to calculate the route matrix. You can't set both
* DepartureTime
and DepartNow
. If neither is set, the best time of day to travel with the
* best traffic conditions is used to calculate the route matrix.
*
*
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*
*
* @return Specifies the desired time of departure. Uses the given time to calculate the route matrix. You can't set
* both DepartureTime
and DepartNow
. If neither is set, the best time of day to
* travel with the best traffic conditions is used to calculate the route matrix.
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*/
public java.util.Date getDepartureTime() {
return this.departureTime;
}
/**
*
* Specifies the desired time of departure. Uses the given time to calculate the route matrix. You can't set both
* DepartureTime
and DepartNow
. If neither is set, the best time of day to travel with the
* best traffic conditions is used to calculate the route matrix.
*
*
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*
*
* @param departureTime
* Specifies the desired time of departure. Uses the given time to calculate the route matrix. You can't set
* both DepartureTime
and DepartNow
. If neither is set, the best time of day to
* travel with the best traffic conditions is used to calculate the route matrix.
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withDepartureTime(java.util.Date departureTime) {
setDepartureTime(departureTime);
return this;
}
/**
*
* Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You can't
* set both DepartureTime
and DepartNow
. If neither is set, the best time of day to travel
* with the best traffic conditions is used to calculate the route matrix.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @param departNow
* Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You
* can't set both DepartureTime
and DepartNow
. If neither is set, the best time of
* day to travel with the best traffic conditions is used to calculate the route matrix.
*
* Default Value: false
*
*
* Valid Values: false
| true
*/
public void setDepartNow(Boolean departNow) {
this.departNow = departNow;
}
/**
*
* Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You can't
* set both DepartureTime
and DepartNow
. If neither is set, the best time of day to travel
* with the best traffic conditions is used to calculate the route matrix.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @return Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You
* can't set both DepartureTime
and DepartNow
. If neither is set, the best time of
* day to travel with the best traffic conditions is used to calculate the route matrix.
*
* Default Value: false
*
*
* Valid Values: false
| true
*/
public Boolean getDepartNow() {
return this.departNow;
}
/**
*
* Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You can't
* set both DepartureTime
and DepartNow
. If neither is set, the best time of day to travel
* with the best traffic conditions is used to calculate the route matrix.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @param departNow
* Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You
* can't set both DepartureTime
and DepartNow
. If neither is set, the best time of
* day to travel with the best traffic conditions is used to calculate the route matrix.
*
* Default Value: false
*
*
* Valid Values: false
| true
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withDepartNow(Boolean departNow) {
setDepartNow(departNow);
return this;
}
/**
*
* Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You can't
* set both DepartureTime
and DepartNow
. If neither is set, the best time of day to travel
* with the best traffic conditions is used to calculate the route matrix.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @return Sets the time of departure as the current time. Uses the current time to calculate the route matrix. You
* can't set both DepartureTime
and DepartNow
. If neither is set, the best time of
* day to travel with the best traffic conditions is used to calculate the route matrix.
*
* Default Value: false
*
*
* Valid Values: false
| true
*/
public Boolean isDepartNow() {
return this.departNow;
}
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* @param distanceUnit
* Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @see DistanceUnit
*/
public void setDistanceUnit(String distanceUnit) {
this.distanceUnit = distanceUnit;
}
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* @return Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @see DistanceUnit
*/
public String getDistanceUnit() {
return this.distanceUnit;
}
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* @param distanceUnit
* Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @return Returns a reference to this object so that method calls can be chained together.
* @see DistanceUnit
*/
public CalculateRouteMatrixRequest withDistanceUnit(String distanceUnit) {
setDistanceUnit(distanceUnit);
return this;
}
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* @param distanceUnit
* Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @return Returns a reference to this object so that method calls can be chained together.
* @see DistanceUnit
*/
public CalculateRouteMatrixRequest withDistanceUnit(DistanceUnit distanceUnit) {
this.distanceUnit = distanceUnit.toString();
return this;
}
/**
*
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries or
* tolls.
*
*
* Requirements: TravelMode
must be specified as Car
.
*
*
* @param carModeOptions
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries
* or tolls.
*
* Requirements: TravelMode
must be specified as Car
.
*/
public void setCarModeOptions(CalculateRouteCarModeOptions carModeOptions) {
this.carModeOptions = carModeOptions;
}
/**
*
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries or
* tolls.
*
*
* Requirements: TravelMode
must be specified as Car
.
*
*
* @return Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries
* or tolls.
*
* Requirements: TravelMode
must be specified as Car
.
*/
public CalculateRouteCarModeOptions getCarModeOptions() {
return this.carModeOptions;
}
/**
*
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries or
* tolls.
*
*
* Requirements: TravelMode
must be specified as Car
.
*
*
* @param carModeOptions
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries
* or tolls.
*
* Requirements: TravelMode
must be specified as Car
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withCarModeOptions(CalculateRouteCarModeOptions carModeOptions) {
setCarModeOptions(carModeOptions);
return this;
}
/**
*
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries or
* tolls, and truck specifications to consider when choosing an optimal road.
*
*
* Requirements: TravelMode
must be specified as Truck
.
*
*
* @param truckModeOptions
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries
* or tolls, and truck specifications to consider when choosing an optimal road.
*
* Requirements: TravelMode
must be specified as Truck
.
*/
public void setTruckModeOptions(CalculateRouteTruckModeOptions truckModeOptions) {
this.truckModeOptions = truckModeOptions;
}
/**
*
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries or
* tolls, and truck specifications to consider when choosing an optimal road.
*
*
* Requirements: TravelMode
must be specified as Truck
.
*
*
* @return Specifies route preferences when traveling by Truck
, such as avoiding routes that use
* ferries or tolls, and truck specifications to consider when choosing an optimal road.
*
* Requirements: TravelMode
must be specified as Truck
.
*/
public CalculateRouteTruckModeOptions getTruckModeOptions() {
return this.truckModeOptions;
}
/**
*
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries or
* tolls, and truck specifications to consider when choosing an optimal road.
*
*
* Requirements: TravelMode
must be specified as Truck
.
*
*
* @param truckModeOptions
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries
* or tolls, and truck specifications to consider when choosing an optimal road.
*
* Requirements: TravelMode
must be specified as Truck
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withTruckModeOptions(CalculateRouteTruckModeOptions truckModeOptions) {
setTruckModeOptions(truckModeOptions);
return this;
}
/**
*
* The optional API key
* to authorize the request.
*
*
* @param key
* The optional API
* key to authorize the request.
*/
public void setKey(String key) {
this.key = key;
}
/**
*
* The optional API key
* to authorize the request.
*
*
* @return The optional API
* key to authorize the request.
*/
public String getKey() {
return this.key;
}
/**
*
* The optional API key
* to authorize the request.
*
*
* @param key
* The optional API
* key to authorize the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CalculateRouteMatrixRequest withKey(String key) {
setKey(key);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCalculatorName() != null)
sb.append("CalculatorName: ").append(getCalculatorName()).append(",");
if (getDeparturePositions() != null)
sb.append("DeparturePositions: ").append("***Sensitive Data Redacted***").append(",");
if (getDestinationPositions() != null)
sb.append("DestinationPositions: ").append("***Sensitive Data Redacted***").append(",");
if (getTravelMode() != null)
sb.append("TravelMode: ").append(getTravelMode()).append(",");
if (getDepartureTime() != null)
sb.append("DepartureTime: ").append(getDepartureTime()).append(",");
if (getDepartNow() != null)
sb.append("DepartNow: ").append(getDepartNow()).append(",");
if (getDistanceUnit() != null)
sb.append("DistanceUnit: ").append(getDistanceUnit()).append(",");
if (getCarModeOptions() != null)
sb.append("CarModeOptions: ").append(getCarModeOptions()).append(",");
if (getTruckModeOptions() != null)
sb.append("TruckModeOptions: ").append(getTruckModeOptions()).append(",");
if (getKey() != null)
sb.append("Key: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CalculateRouteMatrixRequest == false)
return false;
CalculateRouteMatrixRequest other = (CalculateRouteMatrixRequest) obj;
if (other.getCalculatorName() == null ^ this.getCalculatorName() == null)
return false;
if (other.getCalculatorName() != null && other.getCalculatorName().equals(this.getCalculatorName()) == false)
return false;
if (other.getDeparturePositions() == null ^ this.getDeparturePositions() == null)
return false;
if (other.getDeparturePositions() != null && other.getDeparturePositions().equals(this.getDeparturePositions()) == false)
return false;
if (other.getDestinationPositions() == null ^ this.getDestinationPositions() == null)
return false;
if (other.getDestinationPositions() != null && other.getDestinationPositions().equals(this.getDestinationPositions()) == false)
return false;
if (other.getTravelMode() == null ^ this.getTravelMode() == null)
return false;
if (other.getTravelMode() != null && other.getTravelMode().equals(this.getTravelMode()) == false)
return false;
if (other.getDepartureTime() == null ^ this.getDepartureTime() == null)
return false;
if (other.getDepartureTime() != null && other.getDepartureTime().equals(this.getDepartureTime()) == false)
return false;
if (other.getDepartNow() == null ^ this.getDepartNow() == null)
return false;
if (other.getDepartNow() != null && other.getDepartNow().equals(this.getDepartNow()) == false)
return false;
if (other.getDistanceUnit() == null ^ this.getDistanceUnit() == null)
return false;
if (other.getDistanceUnit() != null && other.getDistanceUnit().equals(this.getDistanceUnit()) == false)
return false;
if (other.getCarModeOptions() == null ^ this.getCarModeOptions() == null)
return false;
if (other.getCarModeOptions() != null && other.getCarModeOptions().equals(this.getCarModeOptions()) == false)
return false;
if (other.getTruckModeOptions() == null ^ this.getTruckModeOptions() == null)
return false;
if (other.getTruckModeOptions() != null && other.getTruckModeOptions().equals(this.getTruckModeOptions()) == false)
return false;
if (other.getKey() == null ^ this.getKey() == null)
return false;
if (other.getKey() != null && other.getKey().equals(this.getKey()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCalculatorName() == null) ? 0 : getCalculatorName().hashCode());
hashCode = prime * hashCode + ((getDeparturePositions() == null) ? 0 : getDeparturePositions().hashCode());
hashCode = prime * hashCode + ((getDestinationPositions() == null) ? 0 : getDestinationPositions().hashCode());
hashCode = prime * hashCode + ((getTravelMode() == null) ? 0 : getTravelMode().hashCode());
hashCode = prime * hashCode + ((getDepartureTime() == null) ? 0 : getDepartureTime().hashCode());
hashCode = prime * hashCode + ((getDepartNow() == null) ? 0 : getDepartNow().hashCode());
hashCode = prime * hashCode + ((getDistanceUnit() == null) ? 0 : getDistanceUnit().hashCode());
hashCode = prime * hashCode + ((getCarModeOptions() == null) ? 0 : getCarModeOptions().hashCode());
hashCode = prime * hashCode + ((getTruckModeOptions() == null) ? 0 : getTruckModeOptions().hashCode());
hashCode = prime * hashCode + ((getKey() == null) ? 0 : getKey().hashCode());
return hashCode;
}
@Override
public CalculateRouteMatrixRequest clone() {
return (CalculateRouteMatrixRequest) super.clone();
}
}