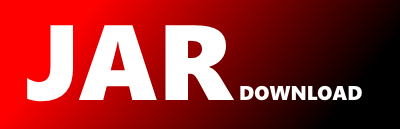
com.amazonaws.services.location.AmazonLocationAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location;
import javax.annotation.Generated;
import com.amazonaws.services.location.model.*;
/**
* Interface for accessing Amazon Location Service asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.location.AbstractAmazonLocationAsync} instead.
*
*
*
* "Suite of geospatial services including Maps, Places, Routes, Tracking, and Geofencing"
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonLocationAsync extends AmazonLocation {
/**
*
* Creates an association between a geofence collection and a tracker resource. This allows the tracker resource to
* communicate location data to the linked geofence collection.
*
*
* You can associate up to five geofence collections to each tracker resource.
*
*
*
* Currently not supported — Cross-account configurations, such as creating associations between a tracker resource
* in one account and a geofence collection in another account.
*
*
*
* @param associateTrackerConsumerRequest
* @return A Java Future containing the result of the AssociateTrackerConsumer operation returned by the service.
* @sample AmazonLocationAsync.AssociateTrackerConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTrackerConsumerAsync(AssociateTrackerConsumerRequest associateTrackerConsumerRequest);
/**
*
* Creates an association between a geofence collection and a tracker resource. This allows the tracker resource to
* communicate location data to the linked geofence collection.
*
*
* You can associate up to five geofence collections to each tracker resource.
*
*
*
* Currently not supported — Cross-account configurations, such as creating associations between a tracker resource
* in one account and a geofence collection in another account.
*
*
*
* @param associateTrackerConsumerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTrackerConsumer operation returned by the service.
* @sample AmazonLocationAsyncHandler.AssociateTrackerConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTrackerConsumerAsync(AssociateTrackerConsumerRequest associateTrackerConsumerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the position history of one or more devices from a tracker resource.
*
*
* @param batchDeleteDevicePositionHistoryRequest
* @return A Java Future containing the result of the BatchDeleteDevicePositionHistory operation returned by the
* service.
* @sample AmazonLocationAsync.BatchDeleteDevicePositionHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteDevicePositionHistoryAsync(
BatchDeleteDevicePositionHistoryRequest batchDeleteDevicePositionHistoryRequest);
/**
*
* Deletes the position history of one or more devices from a tracker resource.
*
*
* @param batchDeleteDevicePositionHistoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteDevicePositionHistory operation returned by the
* service.
* @sample AmazonLocationAsyncHandler.BatchDeleteDevicePositionHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteDevicePositionHistoryAsync(
BatchDeleteDevicePositionHistoryRequest batchDeleteDevicePositionHistoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a batch of geofences from a geofence collection.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param batchDeleteGeofenceRequest
* @return A Java Future containing the result of the BatchDeleteGeofence operation returned by the service.
* @sample AmazonLocationAsync.BatchDeleteGeofence
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDeleteGeofenceAsync(BatchDeleteGeofenceRequest batchDeleteGeofenceRequest);
/**
*
* Deletes a batch of geofences from a geofence collection.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param batchDeleteGeofenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteGeofence operation returned by the service.
* @sample AmazonLocationAsyncHandler.BatchDeleteGeofence
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDeleteGeofenceAsync(BatchDeleteGeofenceRequest batchDeleteGeofenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Evaluates device positions against the geofence geometries from a given geofence collection.
*
*
* This operation always returns an empty response because geofences are asynchronously evaluated. The evaluation
* determines if the device has entered or exited a geofenced area, and then publishes one of the following events
* to Amazon EventBridge:
*
*
* -
*
* ENTER
if Amazon Location determines that the tracked device has entered a geofenced area.
*
*
* -
*
* EXIT
if Amazon Location determines that the tracked device has exited a geofenced area.
*
*
*
*
*
* The last geofence that a device was observed within is tracked for 30 days after the most recent device position
* update.
*
*
*
* Geofence evaluation uses the given device position. It does not account for the optional Accuracy
of
* a DevicePositionUpdate
.
*
*
*
* The DeviceID
is used as a string to represent the device. You do not need to have a
* Tracker
associated with the DeviceID
.
*
*
*
* @param batchEvaluateGeofencesRequest
* @return A Java Future containing the result of the BatchEvaluateGeofences operation returned by the service.
* @sample AmazonLocationAsync.BatchEvaluateGeofences
* @see AWS API Documentation
*/
java.util.concurrent.Future batchEvaluateGeofencesAsync(BatchEvaluateGeofencesRequest batchEvaluateGeofencesRequest);
/**
*
* Evaluates device positions against the geofence geometries from a given geofence collection.
*
*
* This operation always returns an empty response because geofences are asynchronously evaluated. The evaluation
* determines if the device has entered or exited a geofenced area, and then publishes one of the following events
* to Amazon EventBridge:
*
*
* -
*
* ENTER
if Amazon Location determines that the tracked device has entered a geofenced area.
*
*
* -
*
* EXIT
if Amazon Location determines that the tracked device has exited a geofenced area.
*
*
*
*
*
* The last geofence that a device was observed within is tracked for 30 days after the most recent device position
* update.
*
*
*
* Geofence evaluation uses the given device position. It does not account for the optional Accuracy
of
* a DevicePositionUpdate
.
*
*
*
* The DeviceID
is used as a string to represent the device. You do not need to have a
* Tracker
associated with the DeviceID
.
*
*
*
* @param batchEvaluateGeofencesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchEvaluateGeofences operation returned by the service.
* @sample AmazonLocationAsyncHandler.BatchEvaluateGeofences
* @see AWS API Documentation
*/
java.util.concurrent.Future batchEvaluateGeofencesAsync(BatchEvaluateGeofencesRequest batchEvaluateGeofencesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the latest device positions for requested devices.
*
*
* @param batchGetDevicePositionRequest
* @return A Java Future containing the result of the BatchGetDevicePosition operation returned by the service.
* @sample AmazonLocationAsync.BatchGetDevicePosition
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetDevicePositionAsync(BatchGetDevicePositionRequest batchGetDevicePositionRequest);
/**
*
* Lists the latest device positions for requested devices.
*
*
* @param batchGetDevicePositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetDevicePosition operation returned by the service.
* @sample AmazonLocationAsyncHandler.BatchGetDevicePosition
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetDevicePositionAsync(BatchGetDevicePositionRequest batchGetDevicePositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A batch request for storing geofence geometries into a given geofence collection, or updates the geometry of an
* existing geofence if a geofence ID is included in the request.
*
*
* @param batchPutGeofenceRequest
* @return A Java Future containing the result of the BatchPutGeofence operation returned by the service.
* @sample AmazonLocationAsync.BatchPutGeofence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchPutGeofenceAsync(BatchPutGeofenceRequest batchPutGeofenceRequest);
/**
*
* A batch request for storing geofence geometries into a given geofence collection, or updates the geometry of an
* existing geofence if a geofence ID is included in the request.
*
*
* @param batchPutGeofenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchPutGeofence operation returned by the service.
* @sample AmazonLocationAsyncHandler.BatchPutGeofence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchPutGeofenceAsync(BatchPutGeofenceRequest batchPutGeofenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads position update data for one or more devices to a tracker resource (up to 10 devices per batch). Amazon
* Location uses the data when it reports the last known device position and position history. Amazon Location
* retains location data for 30 days.
*
*
*
* Position updates are handled based on the PositionFiltering
property of the tracker. When
* PositionFiltering
is set to TimeBased
, updates are evaluated against linked geofence
* collections, and location data is stored at a maximum of one position per 30 second interval. If your update
* frequency is more often than every 30 seconds, only one update per 30 seconds is stored for each unique device
* ID.
*
*
* When PositionFiltering
is set to DistanceBased
filtering, location data is stored and
* evaluated against linked geofence collections only if the device has moved more than 30 m (98.4 ft).
*
*
* When PositionFiltering
is set to AccuracyBased
filtering, location data is stored and
* evaluated against linked geofence collections only if the device has moved more than the measured accuracy. For
* example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the second update
* is neither stored or evaluated if the device has moved less than 15 m. If PositionFiltering
is set
* to AccuracyBased
filtering, Amazon Location uses the default value { "Horizontal": 0}
* when accuracy is not provided on a DevicePositionUpdate
.
*
*
*
* @param batchUpdateDevicePositionRequest
* @return A Java Future containing the result of the BatchUpdateDevicePosition operation returned by the service.
* @sample AmazonLocationAsync.BatchUpdateDevicePosition
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateDevicePositionAsync(
BatchUpdateDevicePositionRequest batchUpdateDevicePositionRequest);
/**
*
* Uploads position update data for one or more devices to a tracker resource (up to 10 devices per batch). Amazon
* Location uses the data when it reports the last known device position and position history. Amazon Location
* retains location data for 30 days.
*
*
*
* Position updates are handled based on the PositionFiltering
property of the tracker. When
* PositionFiltering
is set to TimeBased
, updates are evaluated against linked geofence
* collections, and location data is stored at a maximum of one position per 30 second interval. If your update
* frequency is more often than every 30 seconds, only one update per 30 seconds is stored for each unique device
* ID.
*
*
* When PositionFiltering
is set to DistanceBased
filtering, location data is stored and
* evaluated against linked geofence collections only if the device has moved more than 30 m (98.4 ft).
*
*
* When PositionFiltering
is set to AccuracyBased
filtering, location data is stored and
* evaluated against linked geofence collections only if the device has moved more than the measured accuracy. For
* example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the second update
* is neither stored or evaluated if the device has moved less than 15 m. If PositionFiltering
is set
* to AccuracyBased
filtering, Amazon Location uses the default value { "Horizontal": 0}
* when accuracy is not provided on a DevicePositionUpdate
.
*
*
*
* @param batchUpdateDevicePositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdateDevicePosition operation returned by the service.
* @sample AmazonLocationAsyncHandler.BatchUpdateDevicePosition
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateDevicePositionAsync(
BatchUpdateDevicePositionRequest batchUpdateDevicePositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Calculates a route
* given the following required parameters: DeparturePosition
and DestinationPosition
.
* Requires that you first create a
* route calculator resource.
*
*
* By default, a request that doesn't specify a departure time uses the best time of day to travel with the best
* traffic conditions when calculating the route.
*
*
* Additional options include:
*
*
* -
*
* Specifying a departure
* time using either DepartureTime
or DepartNow
. This calculates a route based on
* predictive traffic data at the given time.
*
*
*
* You can't specify both DepartureTime
and DepartNow
in a single request. Specifying both
* parameters returns a validation error.
*
*
* -
*
* Specifying a travel
* mode using TravelMode sets the transportation mode used to calculate the routes. This also lets you specify
* additional route preferences in CarModeOptions
if traveling by Car
, or
* TruckModeOptions
if traveling by Truck
.
*
*
*
* If you specify walking
for the travel mode and your data provider is Esri, the start and destination
* must be within 40km.
*
*
*
*
* @param calculateRouteRequest
* @return A Java Future containing the result of the CalculateRoute operation returned by the service.
* @sample AmazonLocationAsync.CalculateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future calculateRouteAsync(CalculateRouteRequest calculateRouteRequest);
/**
*
* Calculates a route
* given the following required parameters: DeparturePosition
and DestinationPosition
.
* Requires that you first create a
* route calculator resource.
*
*
* By default, a request that doesn't specify a departure time uses the best time of day to travel with the best
* traffic conditions when calculating the route.
*
*
* Additional options include:
*
*
* -
*
* Specifying a departure
* time using either DepartureTime
or DepartNow
. This calculates a route based on
* predictive traffic data at the given time.
*
*
*
* You can't specify both DepartureTime
and DepartNow
in a single request. Specifying both
* parameters returns a validation error.
*
*
* -
*
* Specifying a travel
* mode using TravelMode sets the transportation mode used to calculate the routes. This also lets you specify
* additional route preferences in CarModeOptions
if traveling by Car
, or
* TruckModeOptions
if traveling by Truck
.
*
*
*
* If you specify walking
for the travel mode and your data provider is Esri, the start and destination
* must be within 40km.
*
*
*
*
* @param calculateRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CalculateRoute operation returned by the service.
* @sample AmazonLocationAsyncHandler.CalculateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future calculateRouteAsync(CalculateRouteRequest calculateRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Calculates a
* route matrix given the following required parameters: DeparturePositions
and
* DestinationPositions
. CalculateRouteMatrix
calculates routes and returns the travel
* time and travel distance from each departure position to each destination position in the request. For example,
* given departure positions A and B, and destination positions X and Y, CalculateRouteMatrix
will
* return time and distance for routes from A to X, A to Y, B to X, and B to Y (in that order). The number of
* results returned (and routes calculated) will be the number of DeparturePositions
times the number
* of DestinationPositions
.
*
*
*
* Your account is charged for each route calculated, not the number of requests.
*
*
*
* Requires that you first create a
* route calculator resource.
*
*
* By default, a request that doesn't specify a departure time uses the best time of day to travel with the best
* traffic conditions when calculating routes.
*
*
* Additional options include:
*
*
* -
*
* Specifying a departure
* time using either DepartureTime
or DepartNow
. This calculates routes based on
* predictive traffic data at the given time.
*
*
*
* You can't specify both DepartureTime
and DepartNow
in a single request. Specifying both
* parameters returns a validation error.
*
*
* -
*
* Specifying a travel
* mode using TravelMode sets the transportation mode used to calculate the routes. This also lets you specify
* additional route preferences in CarModeOptions
if traveling by Car
, or
* TruckModeOptions
if traveling by Truck
.
*
*
*
*
* @param calculateRouteMatrixRequest
* @return A Java Future containing the result of the CalculateRouteMatrix operation returned by the service.
* @sample AmazonLocationAsync.CalculateRouteMatrix
* @see AWS
* API Documentation
*/
java.util.concurrent.Future calculateRouteMatrixAsync(CalculateRouteMatrixRequest calculateRouteMatrixRequest);
/**
*
* Calculates a
* route matrix given the following required parameters: DeparturePositions
and
* DestinationPositions
. CalculateRouteMatrix
calculates routes and returns the travel
* time and travel distance from each departure position to each destination position in the request. For example,
* given departure positions A and B, and destination positions X and Y, CalculateRouteMatrix
will
* return time and distance for routes from A to X, A to Y, B to X, and B to Y (in that order). The number of
* results returned (and routes calculated) will be the number of DeparturePositions
times the number
* of DestinationPositions
.
*
*
*
* Your account is charged for each route calculated, not the number of requests.
*
*
*
* Requires that you first create a
* route calculator resource.
*
*
* By default, a request that doesn't specify a departure time uses the best time of day to travel with the best
* traffic conditions when calculating routes.
*
*
* Additional options include:
*
*
* -
*
* Specifying a departure
* time using either DepartureTime
or DepartNow
. This calculates routes based on
* predictive traffic data at the given time.
*
*
*
* You can't specify both DepartureTime
and DepartNow
in a single request. Specifying both
* parameters returns a validation error.
*
*
* -
*
* Specifying a travel
* mode using TravelMode sets the transportation mode used to calculate the routes. This also lets you specify
* additional route preferences in CarModeOptions
if traveling by Car
, or
* TruckModeOptions
if traveling by Truck
.
*
*
*
*
* @param calculateRouteMatrixRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CalculateRouteMatrix operation returned by the service.
* @sample AmazonLocationAsyncHandler.CalculateRouteMatrix
* @see AWS
* API Documentation
*/
java.util.concurrent.Future calculateRouteMatrixAsync(CalculateRouteMatrixRequest calculateRouteMatrixRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a geofence collection, which manages and stores geofences.
*
*
* @param createGeofenceCollectionRequest
* @return A Java Future containing the result of the CreateGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsync.CreateGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future createGeofenceCollectionAsync(CreateGeofenceCollectionRequest createGeofenceCollectionRequest);
/**
*
* Creates a geofence collection, which manages and stores geofences.
*
*
* @param createGeofenceCollectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsyncHandler.CreateGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future createGeofenceCollectionAsync(CreateGeofenceCollectionRequest createGeofenceCollectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an API key resource in your Amazon Web Services account, which lets you grant actions for Amazon Location
* resources to the API key bearer.
*
*
*
* For more information, see Using API keys.
*
*
*
* @param createKeyRequest
* @return A Java Future containing the result of the CreateKey operation returned by the service.
* @sample AmazonLocationAsync.CreateKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyAsync(CreateKeyRequest createKeyRequest);
/**
*
* Creates an API key resource in your Amazon Web Services account, which lets you grant actions for Amazon Location
* resources to the API key bearer.
*
*
*
* For more information, see Using API keys.
*
*
*
* @param createKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateKey operation returned by the service.
* @sample AmazonLocationAsyncHandler.CreateKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyAsync(CreateKeyRequest createKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a map resource in your Amazon Web Services account, which provides map tiles of different styles sourced
* from global location data providers.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you must not use Esri as your geolocation provider. See section 82 of the Amazon Web Services service terms for more details.
*
*
*
* @param createMapRequest
* @return A Java Future containing the result of the CreateMap operation returned by the service.
* @sample AmazonLocationAsync.CreateMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMapAsync(CreateMapRequest createMapRequest);
/**
*
* Creates a map resource in your Amazon Web Services account, which provides map tiles of different styles sourced
* from global location data providers.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you must not use Esri as your geolocation provider. See section 82 of the Amazon Web Services service terms for more details.
*
*
*
* @param createMapRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMap operation returned by the service.
* @sample AmazonLocationAsyncHandler.CreateMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMapAsync(CreateMapRequest createMapRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a place index resource in your Amazon Web Services account. Use a place index resource to geocode
* addresses and other text queries by using the SearchPlaceIndexForText
operation, and reverse geocode
* coordinates by using the SearchPlaceIndexForPosition
operation, and enable autosuggestions by using
* the SearchPlaceIndexForSuggestions
operation.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you must not use Esri as your geolocation provider. See section 82 of the Amazon Web Services service terms for more details.
*
*
*
* @param createPlaceIndexRequest
* @return A Java Future containing the result of the CreatePlaceIndex operation returned by the service.
* @sample AmazonLocationAsync.CreatePlaceIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPlaceIndexAsync(CreatePlaceIndexRequest createPlaceIndexRequest);
/**
*
* Creates a place index resource in your Amazon Web Services account. Use a place index resource to geocode
* addresses and other text queries by using the SearchPlaceIndexForText
operation, and reverse geocode
* coordinates by using the SearchPlaceIndexForPosition
operation, and enable autosuggestions by using
* the SearchPlaceIndexForSuggestions
operation.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you must not use Esri as your geolocation provider. See section 82 of the Amazon Web Services service terms for more details.
*
*
*
* @param createPlaceIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePlaceIndex operation returned by the service.
* @sample AmazonLocationAsyncHandler.CreatePlaceIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPlaceIndexAsync(CreatePlaceIndexRequest createPlaceIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a route calculator resource in your Amazon Web Services account.
*
*
* You can send requests to a route calculator resource to estimate travel time, distance, and get directions. A
* route calculator sources traffic and road network data from your chosen data provider.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you must not use Esri as your geolocation provider. See section 82 of the Amazon Web Services service terms for more details.
*
*
*
* @param createRouteCalculatorRequest
* @return A Java Future containing the result of the CreateRouteCalculator operation returned by the service.
* @sample AmazonLocationAsync.CreateRouteCalculator
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createRouteCalculatorAsync(CreateRouteCalculatorRequest createRouteCalculatorRequest);
/**
*
* Creates a route calculator resource in your Amazon Web Services account.
*
*
* You can send requests to a route calculator resource to estimate travel time, distance, and get directions. A
* route calculator sources traffic and road network data from your chosen data provider.
*
*
*
* If your application is tracking or routing assets you use in your business, such as delivery vehicles or
* employees, you must not use Esri as your geolocation provider. See section 82 of the Amazon Web Services service terms for more details.
*
*
*
* @param createRouteCalculatorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRouteCalculator operation returned by the service.
* @sample AmazonLocationAsyncHandler.CreateRouteCalculator
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createRouteCalculatorAsync(CreateRouteCalculatorRequest createRouteCalculatorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a tracker resource in your Amazon Web Services account, which lets you retrieve current and historical
* location of devices.
*
*
* @param createTrackerRequest
* @return A Java Future containing the result of the CreateTracker operation returned by the service.
* @sample AmazonLocationAsync.CreateTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTrackerAsync(CreateTrackerRequest createTrackerRequest);
/**
*
* Creates a tracker resource in your Amazon Web Services account, which lets you retrieve current and historical
* location of devices.
*
*
* @param createTrackerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTracker operation returned by the service.
* @sample AmazonLocationAsyncHandler.CreateTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTrackerAsync(CreateTrackerRequest createTrackerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a geofence collection from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently. If the geofence collection is the target of a tracker resource,
* the devices will no longer be monitored.
*
*
*
* @param deleteGeofenceCollectionRequest
* @return A Java Future containing the result of the DeleteGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsync.DeleteGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteGeofenceCollectionAsync(DeleteGeofenceCollectionRequest deleteGeofenceCollectionRequest);
/**
*
* Deletes a geofence collection from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently. If the geofence collection is the target of a tracker resource,
* the devices will no longer be monitored.
*
*
*
* @param deleteGeofenceCollectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsyncHandler.DeleteGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteGeofenceCollectionAsync(DeleteGeofenceCollectionRequest deleteGeofenceCollectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified API key. The API key must have been deactivated more than 90 days previously.
*
*
* @param deleteKeyRequest
* @return A Java Future containing the result of the DeleteKey operation returned by the service.
* @sample AmazonLocationAsync.DeleteKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteKeyAsync(DeleteKeyRequest deleteKeyRequest);
/**
*
* Deletes the specified API key. The API key must have been deactivated more than 90 days previously.
*
*
* @param deleteKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteKey operation returned by the service.
* @sample AmazonLocationAsyncHandler.DeleteKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteKeyAsync(DeleteKeyRequest deleteKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a map resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently. If the map is being used in an application, the map may not
* render.
*
*
*
* @param deleteMapRequest
* @return A Java Future containing the result of the DeleteMap operation returned by the service.
* @sample AmazonLocationAsync.DeleteMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMapAsync(DeleteMapRequest deleteMapRequest);
/**
*
* Deletes a map resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently. If the map is being used in an application, the map may not
* render.
*
*
*
* @param deleteMapRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMap operation returned by the service.
* @sample AmazonLocationAsyncHandler.DeleteMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMapAsync(DeleteMapRequest deleteMapRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a place index resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param deletePlaceIndexRequest
* @return A Java Future containing the result of the DeletePlaceIndex operation returned by the service.
* @sample AmazonLocationAsync.DeletePlaceIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePlaceIndexAsync(DeletePlaceIndexRequest deletePlaceIndexRequest);
/**
*
* Deletes a place index resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param deletePlaceIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePlaceIndex operation returned by the service.
* @sample AmazonLocationAsyncHandler.DeletePlaceIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePlaceIndexAsync(DeletePlaceIndexRequest deletePlaceIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a route calculator resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param deleteRouteCalculatorRequest
* @return A Java Future containing the result of the DeleteRouteCalculator operation returned by the service.
* @sample AmazonLocationAsync.DeleteRouteCalculator
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteRouteCalculatorAsync(DeleteRouteCalculatorRequest deleteRouteCalculatorRequest);
/**
*
* Deletes a route calculator resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently.
*
*
*
* @param deleteRouteCalculatorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRouteCalculator operation returned by the service.
* @sample AmazonLocationAsyncHandler.DeleteRouteCalculator
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteRouteCalculatorAsync(DeleteRouteCalculatorRequest deleteRouteCalculatorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a tracker resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently. If the tracker resource is in use, you may encounter an error.
* Make sure that the target resource isn't a dependency for your applications.
*
*
*
* @param deleteTrackerRequest
* @return A Java Future containing the result of the DeleteTracker operation returned by the service.
* @sample AmazonLocationAsync.DeleteTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTrackerAsync(DeleteTrackerRequest deleteTrackerRequest);
/**
*
* Deletes a tracker resource from your Amazon Web Services account.
*
*
*
* This operation deletes the resource permanently. If the tracker resource is in use, you may encounter an error.
* Make sure that the target resource isn't a dependency for your applications.
*
*
*
* @param deleteTrackerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTracker operation returned by the service.
* @sample AmazonLocationAsyncHandler.DeleteTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTrackerAsync(DeleteTrackerRequest deleteTrackerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the geofence collection details.
*
*
* @param describeGeofenceCollectionRequest
* @return A Java Future containing the result of the DescribeGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsync.DescribeGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGeofenceCollectionAsync(
DescribeGeofenceCollectionRequest describeGeofenceCollectionRequest);
/**
*
* Retrieves the geofence collection details.
*
*
* @param describeGeofenceCollectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsyncHandler.DescribeGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGeofenceCollectionAsync(
DescribeGeofenceCollectionRequest describeGeofenceCollectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the API key resource details.
*
*
* @param describeKeyRequest
* @return A Java Future containing the result of the DescribeKey operation returned by the service.
* @sample AmazonLocationAsync.DescribeKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeKeyAsync(DescribeKeyRequest describeKeyRequest);
/**
*
* Retrieves the API key resource details.
*
*
* @param describeKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeKey operation returned by the service.
* @sample AmazonLocationAsyncHandler.DescribeKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeKeyAsync(DescribeKeyRequest describeKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the map resource details.
*
*
* @param describeMapRequest
* @return A Java Future containing the result of the DescribeMap operation returned by the service.
* @sample AmazonLocationAsync.DescribeMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeMapAsync(DescribeMapRequest describeMapRequest);
/**
*
* Retrieves the map resource details.
*
*
* @param describeMapRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMap operation returned by the service.
* @sample AmazonLocationAsyncHandler.DescribeMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeMapAsync(DescribeMapRequest describeMapRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the place index resource details.
*
*
* @param describePlaceIndexRequest
* @return A Java Future containing the result of the DescribePlaceIndex operation returned by the service.
* @sample AmazonLocationAsync.DescribePlaceIndex
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePlaceIndexAsync(DescribePlaceIndexRequest describePlaceIndexRequest);
/**
*
* Retrieves the place index resource details.
*
*
* @param describePlaceIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePlaceIndex operation returned by the service.
* @sample AmazonLocationAsyncHandler.DescribePlaceIndex
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describePlaceIndexAsync(DescribePlaceIndexRequest describePlaceIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the route calculator resource details.
*
*
* @param describeRouteCalculatorRequest
* @return A Java Future containing the result of the DescribeRouteCalculator operation returned by the service.
* @sample AmazonLocationAsync.DescribeRouteCalculator
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRouteCalculatorAsync(DescribeRouteCalculatorRequest describeRouteCalculatorRequest);
/**
*
* Retrieves the route calculator resource details.
*
*
* @param describeRouteCalculatorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRouteCalculator operation returned by the service.
* @sample AmazonLocationAsyncHandler.DescribeRouteCalculator
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRouteCalculatorAsync(DescribeRouteCalculatorRequest describeRouteCalculatorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the tracker resource details.
*
*
* @param describeTrackerRequest
* @return A Java Future containing the result of the DescribeTracker operation returned by the service.
* @sample AmazonLocationAsync.DescribeTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTrackerAsync(DescribeTrackerRequest describeTrackerRequest);
/**
*
* Retrieves the tracker resource details.
*
*
* @param describeTrackerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTracker operation returned by the service.
* @sample AmazonLocationAsyncHandler.DescribeTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeTrackerAsync(DescribeTrackerRequest describeTrackerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the association between a tracker resource and a geofence collection.
*
*
*
* Once you unlink a tracker resource from a geofence collection, the tracker positions will no longer be
* automatically evaluated against geofences.
*
*
*
* @param disassociateTrackerConsumerRequest
* @return A Java Future containing the result of the DisassociateTrackerConsumer operation returned by the service.
* @sample AmazonLocationAsync.DisassociateTrackerConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateTrackerConsumerAsync(
DisassociateTrackerConsumerRequest disassociateTrackerConsumerRequest);
/**
*
* Removes the association between a tracker resource and a geofence collection.
*
*
*
* Once you unlink a tracker resource from a geofence collection, the tracker positions will no longer be
* automatically evaluated against geofences.
*
*
*
* @param disassociateTrackerConsumerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateTrackerConsumer operation returned by the service.
* @sample AmazonLocationAsyncHandler.DisassociateTrackerConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateTrackerConsumerAsync(
DisassociateTrackerConsumerRequest disassociateTrackerConsumerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Evaluates device positions against geofence geometries from a given geofence collection. The event forecasts
* three states for which a device can be in relative to a geofence:
*
*
* ENTER
: If a device is outside of a geofence, but would breach the fence if the device is moving at
* its current speed within time horizon window.
*
*
* EXIT
: If a device is inside of a geofence, but would breach the fence if the device is moving at its
* current speed within time horizon window.
*
*
* IDLE
: If a device is inside of a geofence, and the device is not moving.
*
*
* @param forecastGeofenceEventsRequest
* @return A Java Future containing the result of the ForecastGeofenceEvents operation returned by the service.
* @sample AmazonLocationAsync.ForecastGeofenceEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future forecastGeofenceEventsAsync(ForecastGeofenceEventsRequest forecastGeofenceEventsRequest);
/**
*
* Evaluates device positions against geofence geometries from a given geofence collection. The event forecasts
* three states for which a device can be in relative to a geofence:
*
*
* ENTER
: If a device is outside of a geofence, but would breach the fence if the device is moving at
* its current speed within time horizon window.
*
*
* EXIT
: If a device is inside of a geofence, but would breach the fence if the device is moving at its
* current speed within time horizon window.
*
*
* IDLE
: If a device is inside of a geofence, and the device is not moving.
*
*
* @param forecastGeofenceEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ForecastGeofenceEvents operation returned by the service.
* @sample AmazonLocationAsyncHandler.ForecastGeofenceEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future forecastGeofenceEventsAsync(ForecastGeofenceEventsRequest forecastGeofenceEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a device's most recent position according to its sample time.
*
*
*
* Device positions are deleted after 30 days.
*
*
*
* @param getDevicePositionRequest
* @return A Java Future containing the result of the GetDevicePosition operation returned by the service.
* @sample AmazonLocationAsync.GetDevicePosition
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDevicePositionAsync(GetDevicePositionRequest getDevicePositionRequest);
/**
*
* Retrieves a device's most recent position according to its sample time.
*
*
*
* Device positions are deleted after 30 days.
*
*
*
* @param getDevicePositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDevicePosition operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetDevicePosition
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDevicePositionAsync(GetDevicePositionRequest getDevicePositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the device position history from a tracker resource within a specified range of time.
*
*
*
* Device positions are deleted after 30 days.
*
*
*
* @param getDevicePositionHistoryRequest
* @return A Java Future containing the result of the GetDevicePositionHistory operation returned by the service.
* @sample AmazonLocationAsync.GetDevicePositionHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future getDevicePositionHistoryAsync(GetDevicePositionHistoryRequest getDevicePositionHistoryRequest);
/**
*
* Retrieves the device position history from a tracker resource within a specified range of time.
*
*
*
* Device positions are deleted after 30 days.
*
*
*
* @param getDevicePositionHistoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDevicePositionHistory operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetDevicePositionHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future getDevicePositionHistoryAsync(GetDevicePositionHistoryRequest getDevicePositionHistoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the geofence details from a geofence collection.
*
*
*
* The returned geometry will always match the geometry format used when the geofence was created.
*
*
*
* @param getGeofenceRequest
* @return A Java Future containing the result of the GetGeofence operation returned by the service.
* @sample AmazonLocationAsync.GetGeofence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGeofenceAsync(GetGeofenceRequest getGeofenceRequest);
/**
*
* Retrieves the geofence details from a geofence collection.
*
*
*
* The returned geometry will always match the geometry format used when the geofence was created.
*
*
*
* @param getGeofenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGeofence operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetGeofence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGeofenceAsync(GetGeofenceRequest getGeofenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves glyphs used to display labels on a map.
*
*
* @param getMapGlyphsRequest
* @return A Java Future containing the result of the GetMapGlyphs operation returned by the service.
* @sample AmazonLocationAsync.GetMapGlyphs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMapGlyphsAsync(GetMapGlyphsRequest getMapGlyphsRequest);
/**
*
* Retrieves glyphs used to display labels on a map.
*
*
* @param getMapGlyphsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMapGlyphs operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetMapGlyphs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMapGlyphsAsync(GetMapGlyphsRequest getMapGlyphsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the sprite sheet corresponding to a map resource. The sprite sheet is a PNG image paired with a JSON
* document describing the offsets of individual icons that will be displayed on a rendered map.
*
*
* @param getMapSpritesRequest
* @return A Java Future containing the result of the GetMapSprites operation returned by the service.
* @sample AmazonLocationAsync.GetMapSprites
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMapSpritesAsync(GetMapSpritesRequest getMapSpritesRequest);
/**
*
* Retrieves the sprite sheet corresponding to a map resource. The sprite sheet is a PNG image paired with a JSON
* document describing the offsets of individual icons that will be displayed on a rendered map.
*
*
* @param getMapSpritesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMapSprites operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetMapSprites
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMapSpritesAsync(GetMapSpritesRequest getMapSpritesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the map style descriptor from a map resource.
*
*
* The style descriptor contains specifications on how features render on a map. For example, what data to display,
* what order to display the data in, and the style for the data. Style descriptors follow the Mapbox Style
* Specification.
*
*
* @param getMapStyleDescriptorRequest
* @return A Java Future containing the result of the GetMapStyleDescriptor operation returned by the service.
* @sample AmazonLocationAsync.GetMapStyleDescriptor
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMapStyleDescriptorAsync(GetMapStyleDescriptorRequest getMapStyleDescriptorRequest);
/**
*
* Retrieves the map style descriptor from a map resource.
*
*
* The style descriptor contains specifications on how features render on a map. For example, what data to display,
* what order to display the data in, and the style for the data. Style descriptors follow the Mapbox Style
* Specification.
*
*
* @param getMapStyleDescriptorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMapStyleDescriptor operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetMapStyleDescriptor
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMapStyleDescriptorAsync(GetMapStyleDescriptorRequest getMapStyleDescriptorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a vector data tile from the map resource. Map tiles are used by clients to render a map. they're
* addressed using a grid arrangement with an X coordinate, Y coordinate, and Z (zoom) level.
*
*
* The origin (0, 0) is the top left of the map. Increasing the zoom level by 1 doubles both the X and Y dimensions,
* so a tile containing data for the entire world at (0/0/0) will be split into 4 tiles at zoom 1 (1/0/0, 1/0/1,
* 1/1/0, 1/1/1).
*
*
* @param getMapTileRequest
* @return A Java Future containing the result of the GetMapTile operation returned by the service.
* @sample AmazonLocationAsync.GetMapTile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMapTileAsync(GetMapTileRequest getMapTileRequest);
/**
*
* Retrieves a vector data tile from the map resource. Map tiles are used by clients to render a map. they're
* addressed using a grid arrangement with an X coordinate, Y coordinate, and Z (zoom) level.
*
*
* The origin (0, 0) is the top left of the map. Increasing the zoom level by 1 doubles both the X and Y dimensions,
* so a tile containing data for the entire world at (0/0/0) will be split into 4 tiles at zoom 1 (1/0/0, 1/0/1,
* 1/1/0, 1/1/1).
*
*
* @param getMapTileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMapTile operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetMapTile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMapTileAsync(GetMapTileRequest getMapTileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Finds a place by its unique ID. A PlaceId
is returned by other search operations.
*
*
*
* A PlaceId is valid only if all of the following are the same in the original search request and the call to
* GetPlace
.
*
*
* -
*
* Customer Amazon Web Services account
*
*
* -
*
* Amazon Web Services Region
*
*
* -
*
* Data provider specified in the place index resource
*
*
*
*
*
* @param getPlaceRequest
* @return A Java Future containing the result of the GetPlace operation returned by the service.
* @sample AmazonLocationAsync.GetPlace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPlaceAsync(GetPlaceRequest getPlaceRequest);
/**
*
* Finds a place by its unique ID. A PlaceId
is returned by other search operations.
*
*
*
* A PlaceId is valid only if all of the following are the same in the original search request and the call to
* GetPlace
.
*
*
* -
*
* Customer Amazon Web Services account
*
*
* -
*
* Amazon Web Services Region
*
*
* -
*
* Data provider specified in the place index resource
*
*
*
*
*
* @param getPlaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPlace operation returned by the service.
* @sample AmazonLocationAsyncHandler.GetPlace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPlaceAsync(GetPlaceRequest getPlaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A batch request to retrieve all device positions.
*
*
* @param listDevicePositionsRequest
* @return A Java Future containing the result of the ListDevicePositions operation returned by the service.
* @sample AmazonLocationAsync.ListDevicePositions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDevicePositionsAsync(ListDevicePositionsRequest listDevicePositionsRequest);
/**
*
* A batch request to retrieve all device positions.
*
*
* @param listDevicePositionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDevicePositions operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListDevicePositions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDevicePositionsAsync(ListDevicePositionsRequest listDevicePositionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists geofence collections in your Amazon Web Services account.
*
*
* @param listGeofenceCollectionsRequest
* @return A Java Future containing the result of the ListGeofenceCollections operation returned by the service.
* @sample AmazonLocationAsync.ListGeofenceCollections
* @see AWS API Documentation
*/
java.util.concurrent.Future listGeofenceCollectionsAsync(ListGeofenceCollectionsRequest listGeofenceCollectionsRequest);
/**
*
* Lists geofence collections in your Amazon Web Services account.
*
*
* @param listGeofenceCollectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGeofenceCollections operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListGeofenceCollections
* @see AWS API Documentation
*/
java.util.concurrent.Future listGeofenceCollectionsAsync(ListGeofenceCollectionsRequest listGeofenceCollectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists geofences stored in a given geofence collection.
*
*
* @param listGeofencesRequest
* @return A Java Future containing the result of the ListGeofences operation returned by the service.
* @sample AmazonLocationAsync.ListGeofences
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGeofencesAsync(ListGeofencesRequest listGeofencesRequest);
/**
*
* Lists geofences stored in a given geofence collection.
*
*
* @param listGeofencesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGeofences operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListGeofences
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGeofencesAsync(ListGeofencesRequest listGeofencesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists API key resources in your Amazon Web Services account.
*
*
* @param listKeysRequest
* @return A Java Future containing the result of the ListKeys operation returned by the service.
* @sample AmazonLocationAsync.ListKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listKeysAsync(ListKeysRequest listKeysRequest);
/**
*
* Lists API key resources in your Amazon Web Services account.
*
*
* @param listKeysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListKeys operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListKeys
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listKeysAsync(ListKeysRequest listKeysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists map resources in your Amazon Web Services account.
*
*
* @param listMapsRequest
* @return A Java Future containing the result of the ListMaps operation returned by the service.
* @sample AmazonLocationAsync.ListMaps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMapsAsync(ListMapsRequest listMapsRequest);
/**
*
* Lists map resources in your Amazon Web Services account.
*
*
* @param listMapsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMaps operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListMaps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMapsAsync(ListMapsRequest listMapsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists place index resources in your Amazon Web Services account.
*
*
* @param listPlaceIndexesRequest
* @return A Java Future containing the result of the ListPlaceIndexes operation returned by the service.
* @sample AmazonLocationAsync.ListPlaceIndexes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPlaceIndexesAsync(ListPlaceIndexesRequest listPlaceIndexesRequest);
/**
*
* Lists place index resources in your Amazon Web Services account.
*
*
* @param listPlaceIndexesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPlaceIndexes operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListPlaceIndexes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPlaceIndexesAsync(ListPlaceIndexesRequest listPlaceIndexesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists route calculator resources in your Amazon Web Services account.
*
*
* @param listRouteCalculatorsRequest
* @return A Java Future containing the result of the ListRouteCalculators operation returned by the service.
* @sample AmazonLocationAsync.ListRouteCalculators
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRouteCalculatorsAsync(ListRouteCalculatorsRequest listRouteCalculatorsRequest);
/**
*
* Lists route calculator resources in your Amazon Web Services account.
*
*
* @param listRouteCalculatorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRouteCalculators operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListRouteCalculators
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listRouteCalculatorsAsync(ListRouteCalculatorsRequest listRouteCalculatorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tags that are applied to the specified Amazon Location resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonLocationAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of tags that are applied to the specified Amazon Location resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists geofence collections currently associated to the given tracker resource.
*
*
* @param listTrackerConsumersRequest
* @return A Java Future containing the result of the ListTrackerConsumers operation returned by the service.
* @sample AmazonLocationAsync.ListTrackerConsumers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTrackerConsumersAsync(ListTrackerConsumersRequest listTrackerConsumersRequest);
/**
*
* Lists geofence collections currently associated to the given tracker resource.
*
*
* @param listTrackerConsumersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTrackerConsumers operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListTrackerConsumers
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTrackerConsumersAsync(ListTrackerConsumersRequest listTrackerConsumersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists tracker resources in your Amazon Web Services account.
*
*
* @param listTrackersRequest
* @return A Java Future containing the result of the ListTrackers operation returned by the service.
* @sample AmazonLocationAsync.ListTrackers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTrackersAsync(ListTrackersRequest listTrackersRequest);
/**
*
* Lists tracker resources in your Amazon Web Services account.
*
*
* @param listTrackersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTrackers operation returned by the service.
* @sample AmazonLocationAsyncHandler.ListTrackers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTrackersAsync(ListTrackersRequest listTrackersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stores a geofence geometry in a given geofence collection, or updates the geometry of an existing geofence if a
* geofence ID is included in the request.
*
*
* @param putGeofenceRequest
* @return A Java Future containing the result of the PutGeofence operation returned by the service.
* @sample AmazonLocationAsync.PutGeofence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putGeofenceAsync(PutGeofenceRequest putGeofenceRequest);
/**
*
* Stores a geofence geometry in a given geofence collection, or updates the geometry of an existing geofence if a
* geofence ID is included in the request.
*
*
* @param putGeofenceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutGeofence operation returned by the service.
* @sample AmazonLocationAsyncHandler.PutGeofence
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putGeofenceAsync(PutGeofenceRequest putGeofenceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reverse geocodes a given coordinate and returns a legible address. Allows you to search for Places or points of
* interest near a given position.
*
*
* @param searchPlaceIndexForPositionRequest
* @return A Java Future containing the result of the SearchPlaceIndexForPosition operation returned by the service.
* @sample AmazonLocationAsync.SearchPlaceIndexForPosition
* @see AWS API Documentation
*/
java.util.concurrent.Future searchPlaceIndexForPositionAsync(
SearchPlaceIndexForPositionRequest searchPlaceIndexForPositionRequest);
/**
*
* Reverse geocodes a given coordinate and returns a legible address. Allows you to search for Places or points of
* interest near a given position.
*
*
* @param searchPlaceIndexForPositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchPlaceIndexForPosition operation returned by the service.
* @sample AmazonLocationAsyncHandler.SearchPlaceIndexForPosition
* @see AWS API Documentation
*/
java.util.concurrent.Future searchPlaceIndexForPositionAsync(
SearchPlaceIndexForPositionRequest searchPlaceIndexForPositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates suggestions for addresses and points of interest based on partial or misspelled free-form text. This
* operation is also known as autocomplete, autosuggest, or fuzzy matching.
*
*
* Optional parameters let you narrow your search results by bounding box or country, or bias your search toward a
* specific position on the globe.
*
*
*
* You can search for suggested place names near a specified position by using BiasPosition
, or filter
* results within a bounding box by using FilterBBox
. These parameters are mutually exclusive; using
* both BiasPosition
and FilterBBox
in the same command returns an error.
*
*
*
* @param searchPlaceIndexForSuggestionsRequest
* @return A Java Future containing the result of the SearchPlaceIndexForSuggestions operation returned by the
* service.
* @sample AmazonLocationAsync.SearchPlaceIndexForSuggestions
* @see AWS API Documentation
*/
java.util.concurrent.Future searchPlaceIndexForSuggestionsAsync(
SearchPlaceIndexForSuggestionsRequest searchPlaceIndexForSuggestionsRequest);
/**
*
* Generates suggestions for addresses and points of interest based on partial or misspelled free-form text. This
* operation is also known as autocomplete, autosuggest, or fuzzy matching.
*
*
* Optional parameters let you narrow your search results by bounding box or country, or bias your search toward a
* specific position on the globe.
*
*
*
* You can search for suggested place names near a specified position by using BiasPosition
, or filter
* results within a bounding box by using FilterBBox
. These parameters are mutually exclusive; using
* both BiasPosition
and FilterBBox
in the same command returns an error.
*
*
*
* @param searchPlaceIndexForSuggestionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchPlaceIndexForSuggestions operation returned by the
* service.
* @sample AmazonLocationAsyncHandler.SearchPlaceIndexForSuggestions
* @see AWS API Documentation
*/
java.util.concurrent.Future searchPlaceIndexForSuggestionsAsync(
SearchPlaceIndexForSuggestionsRequest searchPlaceIndexForSuggestionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Geocodes free-form text, such as an address, name, city, or region to allow you to search for Places or points of
* interest.
*
*
* Optional parameters let you narrow your search results by bounding box or country, or bias your search toward a
* specific position on the globe.
*
*
*
* You can search for places near a given position using BiasPosition
, or filter results within a
* bounding box using FilterBBox
. Providing both parameters simultaneously returns an error.
*
*
*
* Search results are returned in order of highest to lowest relevance.
*
*
* @param searchPlaceIndexForTextRequest
* @return A Java Future containing the result of the SearchPlaceIndexForText operation returned by the service.
* @sample AmazonLocationAsync.SearchPlaceIndexForText
* @see AWS API Documentation
*/
java.util.concurrent.Future searchPlaceIndexForTextAsync(SearchPlaceIndexForTextRequest searchPlaceIndexForTextRequest);
/**
*
* Geocodes free-form text, such as an address, name, city, or region to allow you to search for Places or points of
* interest.
*
*
* Optional parameters let you narrow your search results by bounding box or country, or bias your search toward a
* specific position on the globe.
*
*
*
* You can search for places near a given position using BiasPosition
, or filter results within a
* bounding box using FilterBBox
. Providing both parameters simultaneously returns an error.
*
*
*
* Search results are returned in order of highest to lowest relevance.
*
*
* @param searchPlaceIndexForTextRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchPlaceIndexForText operation returned by the service.
* @sample AmazonLocationAsyncHandler.SearchPlaceIndexForText
* @see AWS API Documentation
*/
java.util.concurrent.Future searchPlaceIndexForTextAsync(SearchPlaceIndexForTextRequest searchPlaceIndexForTextRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Amazon Location Service resource.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only resources with certain tag values.
*
*
* You can use the TagResource
operation with an Amazon Location Service resource that already has
* tags. If you specify a new tag key for the resource, this tag is appended to the tags already associated with the
* resource. If you specify a tag key that's already associated with the resource, the new tag value that you
* specify replaces the previous value for that tag.
*
*
* You can associate up to 50 tags with a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonLocationAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Amazon Location Service resource.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only resources with certain tag values.
*
*
* You can use the TagResource
operation with an Amazon Location Service resource that already has
* tags. If you specify a new tag key for the resource, this tag is appended to the tags already associated with the
* resource. If you specify a tag key that's already associated with the resource, the new tag value that you
* specify replaces the previous value for that tag.
*
*
* You can associate up to 50 tags with a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonLocationAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified Amazon Location resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonLocationAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from the specified Amazon Location resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonLocationAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified properties of a given geofence collection.
*
*
* @param updateGeofenceCollectionRequest
* @return A Java Future containing the result of the UpdateGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsync.UpdateGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateGeofenceCollectionAsync(UpdateGeofenceCollectionRequest updateGeofenceCollectionRequest);
/**
*
* Updates the specified properties of a given geofence collection.
*
*
* @param updateGeofenceCollectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGeofenceCollection operation returned by the service.
* @sample AmazonLocationAsyncHandler.UpdateGeofenceCollection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateGeofenceCollectionAsync(UpdateGeofenceCollectionRequest updateGeofenceCollectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified properties of a given API key resource.
*
*
* @param updateKeyRequest
* @return A Java Future containing the result of the UpdateKey operation returned by the service.
* @sample AmazonLocationAsync.UpdateKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateKeyAsync(UpdateKeyRequest updateKeyRequest);
/**
*
* Updates the specified properties of a given API key resource.
*
*
* @param updateKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateKey operation returned by the service.
* @sample AmazonLocationAsyncHandler.UpdateKey
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateKeyAsync(UpdateKeyRequest updateKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified properties of a given map resource.
*
*
* @param updateMapRequest
* @return A Java Future containing the result of the UpdateMap operation returned by the service.
* @sample AmazonLocationAsync.UpdateMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMapAsync(UpdateMapRequest updateMapRequest);
/**
*
* Updates the specified properties of a given map resource.
*
*
* @param updateMapRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMap operation returned by the service.
* @sample AmazonLocationAsyncHandler.UpdateMap
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMapAsync(UpdateMapRequest updateMapRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified properties of a given place index resource.
*
*
* @param updatePlaceIndexRequest
* @return A Java Future containing the result of the UpdatePlaceIndex operation returned by the service.
* @sample AmazonLocationAsync.UpdatePlaceIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updatePlaceIndexAsync(UpdatePlaceIndexRequest updatePlaceIndexRequest);
/**
*
* Updates the specified properties of a given place index resource.
*
*
* @param updatePlaceIndexRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePlaceIndex operation returned by the service.
* @sample AmazonLocationAsyncHandler.UpdatePlaceIndex
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updatePlaceIndexAsync(UpdatePlaceIndexRequest updatePlaceIndexRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified properties for a given route calculator resource.
*
*
* @param updateRouteCalculatorRequest
* @return A Java Future containing the result of the UpdateRouteCalculator operation returned by the service.
* @sample AmazonLocationAsync.UpdateRouteCalculator
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateRouteCalculatorAsync(UpdateRouteCalculatorRequest updateRouteCalculatorRequest);
/**
*
* Updates the specified properties for a given route calculator resource.
*
*
* @param updateRouteCalculatorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRouteCalculator operation returned by the service.
* @sample AmazonLocationAsyncHandler.UpdateRouteCalculator
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateRouteCalculatorAsync(UpdateRouteCalculatorRequest updateRouteCalculatorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified properties of a given tracker resource.
*
*
* @param updateTrackerRequest
* @return A Java Future containing the result of the UpdateTracker operation returned by the service.
* @sample AmazonLocationAsync.UpdateTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTrackerAsync(UpdateTrackerRequest updateTrackerRequest);
/**
*
* Updates the specified properties of a given tracker resource.
*
*
* @param updateTrackerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTracker operation returned by the service.
* @sample AmazonLocationAsyncHandler.UpdateTracker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTrackerAsync(UpdateTrackerRequest updateTrackerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Verifies the integrity of the device's position by determining if it was reported behind a proxy, and by
* comparing it to an inferred position estimated based on the device's state.
*
*
* @param verifyDevicePositionRequest
* @return A Java Future containing the result of the VerifyDevicePosition operation returned by the service.
* @sample AmazonLocationAsync.VerifyDevicePosition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future verifyDevicePositionAsync(VerifyDevicePositionRequest verifyDevicePositionRequest);
/**
*
* Verifies the integrity of the device's position by determining if it was reported behind a proxy, and by
* comparing it to an inferred position estimated based on the device's state.
*
*
* @param verifyDevicePositionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the VerifyDevicePosition operation returned by the service.
* @sample AmazonLocationAsyncHandler.VerifyDevicePosition
* @see AWS
* API Documentation
*/
java.util.concurrent.Future verifyDevicePositionAsync(VerifyDevicePositionRequest verifyDevicePositionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}