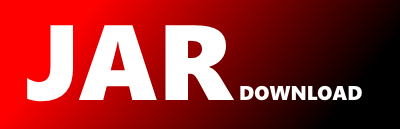
com.amazonaws.services.location.model.ApiKeyRestrictions Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* API Restrictions on the allowed actions, resources, and referers for an API key resource.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ApiKeyRestrictions implements Serializable, Cloneable, StructuredPojo {
/**
*
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least one
* action for each type of resource. For example, if you have a place resource, you must include at least one place
* action.
*
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action is
* geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use ["geo:SearchPlaceIndexFor*"]
- you
* must list each of the Place actions separately.
*
*
*/
private java.util.List allowActions;
/**
*
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the resource-id to
* match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
as the
* key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
, partition
,
* service
, region
, account-id
and resource-id
delimited by
* colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
*
*/
private java.util.List allowResources;
/**
*
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API key
* from other domains will not be allowed.
*
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL encoding
* purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
*
*/
private java.util.List allowReferers;
/**
*
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least one
* action for each type of resource. For example, if you have a place resource, you must include at least one place
* action.
*
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action is
* geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use ["geo:SearchPlaceIndexFor*"]
- you
* must list each of the Place actions separately.
*
*
*
* @return A list of allowed actions that an API key resource grants permissions to perform. You must have at least
* one action for each type of resource. For example, if you have a place resource, you must include at
* least one place action.
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid
* action is geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use
* ["geo:SearchPlaceIndexFor*"]
- you must list each of the Place actions separately.
*
*/
public java.util.List getAllowActions() {
return allowActions;
}
/**
*
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least one
* action for each type of resource. For example, if you have a place resource, you must include at least one place
* action.
*
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action is
* geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use ["geo:SearchPlaceIndexFor*"]
- you
* must list each of the Place actions separately.
*
*
*
* @param allowActions
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least
* one action for each type of resource. For example, if you have a place resource, you must include at least
* one place action.
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action
* is geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use
* ["geo:SearchPlaceIndexFor*"]
- you must list each of the Place actions separately.
*
*/
public void setAllowActions(java.util.Collection allowActions) {
if (allowActions == null) {
this.allowActions = null;
return;
}
this.allowActions = new java.util.ArrayList(allowActions);
}
/**
*
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least one
* action for each type of resource. For example, if you have a place resource, you must include at least one place
* action.
*
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action is
* geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use ["geo:SearchPlaceIndexFor*"]
- you
* must list each of the Place actions separately.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowActions(java.util.Collection)} or {@link #withAllowActions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param allowActions
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least
* one action for each type of resource. For example, if you have a place resource, you must include at least
* one place action.
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action
* is geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use
* ["geo:SearchPlaceIndexFor*"]
- you must list each of the Place actions separately.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApiKeyRestrictions withAllowActions(String... allowActions) {
if (this.allowActions == null) {
setAllowActions(new java.util.ArrayList(allowActions.length));
}
for (String ele : allowActions) {
this.allowActions.add(ele);
}
return this;
}
/**
*
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least one
* action for each type of resource. For example, if you have a place resource, you must include at least one place
* action.
*
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action is
* geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use ["geo:SearchPlaceIndexFor*"]
- you
* must list each of the Place actions separately.
*
*
*
* @param allowActions
* A list of allowed actions that an API key resource grants permissions to perform. You must have at least
* one action for each type of resource. For example, if you have a place resource, you must include at least
* one place action.
*
* The following are valid values for the actions.
*
*
* -
*
* Map actions
*
*
* -
*
* geo:GetMap*
- Allows all actions needed for map rendering.
*
*
*
*
* -
*
* Place actions
*
*
* -
*
* geo:SearchPlaceIndexForText
- Allows geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForPosition
- Allows reverse geocoding.
*
*
* -
*
* geo:SearchPlaceIndexForSuggestions
- Allows generating suggestions from text.
*
*
* -
*
* GetPlace
- Allows finding a place by place ID.
*
*
*
*
* -
*
* Route actions
*
*
* -
*
* geo:CalculateRoute
- Allows point to point routing.
*
*
* -
*
* geo:CalculateRouteMatrix
- Allows calculating a matrix of routes.
*
*
*
*
*
*
*
* You must use these strings exactly. For example, to provide access to map rendering, the only valid action
* is geo:GetMap*
as an input to the list. ["geo:GetMap*"]
is valid but
* ["geo:GetMapTile"]
is not. Similarly, you cannot use
* ["geo:SearchPlaceIndexFor*"]
- you must list each of the Place actions separately.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApiKeyRestrictions withAllowActions(java.util.Collection allowActions) {
setAllowActions(allowActions);
return this;
}
/**
*
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the resource-id to
* match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
as the
* key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
, partition
,
* service
, region
, account-id
and resource-id
delimited by
* colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
*
*
* @return A list of allowed resource ARNs that a API key bearer can perform actions on.
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the
* resource-id to match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and
* account-id
as the key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
,
* partition
, service
, region
, account-id
and
* resource-id
delimited by colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
*/
public java.util.List getAllowResources() {
return allowResources;
}
/**
*
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the resource-id to
* match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
as the
* key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
, partition
,
* service
, region
, account-id
and resource-id
delimited by
* colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
*
*
* @param allowResources
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the
* resource-id to match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
* as the key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
,
* partition
, service
, region
, account-id
and
* resource-id
delimited by colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
*/
public void setAllowResources(java.util.Collection allowResources) {
if (allowResources == null) {
this.allowResources = null;
return;
}
this.allowResources = new java.util.ArrayList(allowResources);
}
/**
*
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the resource-id to
* match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
as the
* key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
, partition
,
* service
, region
, account-id
and resource-id
delimited by
* colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowResources(java.util.Collection)} or {@link #withAllowResources(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param allowResources
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the
* resource-id to match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
* as the key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
,
* partition
, service
, region
, account-id
and
* resource-id
delimited by colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApiKeyRestrictions withAllowResources(String... allowResources) {
if (this.allowResources == null) {
setAllowResources(new java.util.ArrayList(allowResources.length));
}
for (String ele : allowResources) {
this.allowResources.add(ele);
}
return this;
}
/**
*
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the resource-id to
* match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
as the
* key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
, partition
,
* service
, region
, account-id
and resource-id
delimited by
* colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
*
*
* @param allowResources
* A list of allowed resource ARNs that a API key bearer can perform actions on.
*
* -
*
* The ARN must be the correct ARN for a map, place, or route ARN. You may include wildcards in the
* resource-id to match multiple resources of the same type.
*
*
* -
*
* The resources must be in the same partition
, region
, and account-id
* as the key that is being created.
*
*
* -
*
* Other than wildcards, you must include the full ARN, including the arn
,
* partition
, service
, region
, account-id
and
* resource-id
delimited by colons (:).
*
*
* -
*
* No spaces allowed, even with wildcards. For example,
* arn:aws:geo:region:account-id:map/ExampleMap*
.
*
*
*
*
* For more information about ARN format, see Amazon Resource Names
* (ARNs).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApiKeyRestrictions withAllowResources(java.util.Collection allowResources) {
setAllowResources(allowResources);
return this;
}
/**
*
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API key
* from other domains will not be allowed.
*
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL encoding
* purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
*
*
* @return An optional list of allowed HTTP referers for which requests must originate from. Requests using this API
* key from other domains will not be allowed.
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL
* encoding purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
*/
public java.util.List getAllowReferers() {
return allowReferers;
}
/**
*
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API key
* from other domains will not be allowed.
*
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL encoding
* purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
*
*
* @param allowReferers
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API
* key from other domains will not be allowed.
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL
* encoding purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
*/
public void setAllowReferers(java.util.Collection allowReferers) {
if (allowReferers == null) {
this.allowReferers = null;
return;
}
this.allowReferers = new java.util.ArrayList(allowReferers);
}
/**
*
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API key
* from other domains will not be allowed.
*
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL encoding
* purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowReferers(java.util.Collection)} or {@link #withAllowReferers(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param allowReferers
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API
* key from other domains will not be allowed.
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL
* encoding purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApiKeyRestrictions withAllowReferers(String... allowReferers) {
if (this.allowReferers == null) {
setAllowReferers(new java.util.ArrayList(allowReferers.length));
}
for (String ele : allowReferers) {
this.allowReferers.add(ele);
}
return this;
}
/**
*
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API key
* from other domains will not be allowed.
*
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL encoding
* purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
*
*
* @param allowReferers
* An optional list of allowed HTTP referers for which requests must originate from. Requests using this API
* key from other domains will not be allowed.
*
* Requirements:
*
*
* -
*
* Contain only alphanumeric characters (A–Z, a–z, 0–9) or any symbols in this list
* $\-._+!*`(),;/?:@=&
*
*
* -
*
* May contain a percent (%) if followed by 2 hexadecimal digits (A-F, a-f, 0-9); this is used for URL
* encoding purposes.
*
*
* -
*
* May contain wildcard characters question mark (?) and asterisk (*).
*
*
* Question mark (?) will replace any single character (including hexadecimal digits).
*
*
* Asterisk (*) will replace any multiple characters (including multiple hexadecimal digits).
*
*
* -
*
* No spaces allowed. For example, https://example.com
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApiKeyRestrictions withAllowReferers(java.util.Collection allowReferers) {
setAllowReferers(allowReferers);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAllowActions() != null)
sb.append("AllowActions: ").append(getAllowActions()).append(",");
if (getAllowResources() != null)
sb.append("AllowResources: ").append(getAllowResources()).append(",");
if (getAllowReferers() != null)
sb.append("AllowReferers: ").append(getAllowReferers());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ApiKeyRestrictions == false)
return false;
ApiKeyRestrictions other = (ApiKeyRestrictions) obj;
if (other.getAllowActions() == null ^ this.getAllowActions() == null)
return false;
if (other.getAllowActions() != null && other.getAllowActions().equals(this.getAllowActions()) == false)
return false;
if (other.getAllowResources() == null ^ this.getAllowResources() == null)
return false;
if (other.getAllowResources() != null && other.getAllowResources().equals(this.getAllowResources()) == false)
return false;
if (other.getAllowReferers() == null ^ this.getAllowReferers() == null)
return false;
if (other.getAllowReferers() != null && other.getAllowReferers().equals(this.getAllowReferers()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAllowActions() == null) ? 0 : getAllowActions().hashCode());
hashCode = prime * hashCode + ((getAllowResources() == null) ? 0 : getAllowResources().hashCode());
hashCode = prime * hashCode + ((getAllowReferers() == null) ? 0 : getAllowReferers().hashCode());
return hashCode;
}
@Override
public ApiKeyRestrictions clone() {
try {
return (ApiKeyRestrictions) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.location.model.transform.ApiKeyRestrictionsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}