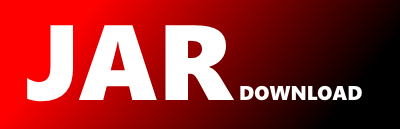
com.amazonaws.services.location.model.Place Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains details about addresses or points of interest that match the search criteria.
*
*
* Not all details are included with all responses. Some details may only be returned by specific data partners.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Place implements Serializable, Cloneable, StructuredPojo {
/**
*
* The full name and address of the point of interest such as a city, region, or country. For example,
* 123 Any Street, Any Town, USA
.
*
*/
private String label;
private PlaceGeometry geometry;
/**
*
* The numerical portion of an address, such as a building number.
*
*/
private String addressNumber;
/**
*
* The name for a street or a road to identify a location. For example, Main Street
.
*
*/
private String street;
/**
*
* The name of a community district. For example, Downtown
.
*
*/
private String neighborhood;
/**
*
* A name for a local area, such as a city or town name. For example, Toronto
.
*
*/
private String municipality;
/**
*
* A county, or an area that's part of a larger region. For example, Metro Vancouver
.
*
*/
private String subRegion;
/**
*
* A name for an area or geographical division, such as a province or state name. For example,
* British Columbia
.
*
*/
private String region;
/**
*
* A country/region specified using ISO 3166 3-digit
* country/region code. For example, CAN
.
*
*/
private String country;
/**
*
* A group of numbers and letters in a country-specific format, which accompanies the address for the purpose of
* identifying a location.
*
*/
private String postalCode;
/**
*
* True
if the result is interpolated from other known places.
*
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but returns
* True
if an address does not exist in the partner data and its location is calculated by
* interpolating between other known addresses.
*
*/
private Boolean interpolated;
/**
*
* The time zone in which the Place
is located. Returned only when using HERE or Grab as the selected
* partner.
*
*/
private TimeZone timeZone;
/**
*
* For addresses with a UnitNumber
, the type of unit. For example, Apartment
.
*
*
*
* Returned only for a place index that uses Esri as a data provider.
*
*
*/
private String unitType;
/**
*
* For addresses with multiple units, the unit identifier. Can include numbers and letters, for example
* 3B
or Unit 123
.
*
*
*
* Returned only for a place index that uses Esri or Grab as a data provider. Is not returned for
* SearchPlaceIndexForPosition
.
*
*
*/
private String unitNumber;
/**
*
* The Amazon Location categories that describe this Place.
*
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
*
*/
private java.util.List categories;
/**
*
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location categories.
*
*/
private java.util.List supplementalCategories;
/**
*
* An area that's part of a larger municipality. For example, Blissville
is a submunicipality in the
* Queen County in New York.
*
*
*
* This property supported by Esri and OpenData. The Esri property is district
, and the OpenData
* property is borough
.
*
*
*/
private String subMunicipality;
/**
*
* The full name and address of the point of interest such as a city, region, or country. For example,
* 123 Any Street, Any Town, USA
.
*
*
* @param label
* The full name and address of the point of interest such as a city, region, or country. For example,
* 123 Any Street, Any Town, USA
.
*/
public void setLabel(String label) {
this.label = label;
}
/**
*
* The full name and address of the point of interest such as a city, region, or country. For example,
* 123 Any Street, Any Town, USA
.
*
*
* @return The full name and address of the point of interest such as a city, region, or country. For example,
* 123 Any Street, Any Town, USA
.
*/
public String getLabel() {
return this.label;
}
/**
*
* The full name and address of the point of interest such as a city, region, or country. For example,
* 123 Any Street, Any Town, USA
.
*
*
* @param label
* The full name and address of the point of interest such as a city, region, or country. For example,
* 123 Any Street, Any Town, USA
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withLabel(String label) {
setLabel(label);
return this;
}
/**
* @param geometry
*/
public void setGeometry(PlaceGeometry geometry) {
this.geometry = geometry;
}
/**
* @return
*/
public PlaceGeometry getGeometry() {
return this.geometry;
}
/**
* @param geometry
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withGeometry(PlaceGeometry geometry) {
setGeometry(geometry);
return this;
}
/**
*
* The numerical portion of an address, such as a building number.
*
*
* @param addressNumber
* The numerical portion of an address, such as a building number.
*/
public void setAddressNumber(String addressNumber) {
this.addressNumber = addressNumber;
}
/**
*
* The numerical portion of an address, such as a building number.
*
*
* @return The numerical portion of an address, such as a building number.
*/
public String getAddressNumber() {
return this.addressNumber;
}
/**
*
* The numerical portion of an address, such as a building number.
*
*
* @param addressNumber
* The numerical portion of an address, such as a building number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withAddressNumber(String addressNumber) {
setAddressNumber(addressNumber);
return this;
}
/**
*
* The name for a street or a road to identify a location. For example, Main Street
.
*
*
* @param street
* The name for a street or a road to identify a location. For example, Main Street
.
*/
public void setStreet(String street) {
this.street = street;
}
/**
*
* The name for a street or a road to identify a location. For example, Main Street
.
*
*
* @return The name for a street or a road to identify a location. For example, Main Street
.
*/
public String getStreet() {
return this.street;
}
/**
*
* The name for a street or a road to identify a location. For example, Main Street
.
*
*
* @param street
* The name for a street or a road to identify a location. For example, Main Street
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withStreet(String street) {
setStreet(street);
return this;
}
/**
*
* The name of a community district. For example, Downtown
.
*
*
* @param neighborhood
* The name of a community district. For example, Downtown
.
*/
public void setNeighborhood(String neighborhood) {
this.neighborhood = neighborhood;
}
/**
*
* The name of a community district. For example, Downtown
.
*
*
* @return The name of a community district. For example, Downtown
.
*/
public String getNeighborhood() {
return this.neighborhood;
}
/**
*
* The name of a community district. For example, Downtown
.
*
*
* @param neighborhood
* The name of a community district. For example, Downtown
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withNeighborhood(String neighborhood) {
setNeighborhood(neighborhood);
return this;
}
/**
*
* A name for a local area, such as a city or town name. For example, Toronto
.
*
*
* @param municipality
* A name for a local area, such as a city or town name. For example, Toronto
.
*/
public void setMunicipality(String municipality) {
this.municipality = municipality;
}
/**
*
* A name for a local area, such as a city or town name. For example, Toronto
.
*
*
* @return A name for a local area, such as a city or town name. For example, Toronto
.
*/
public String getMunicipality() {
return this.municipality;
}
/**
*
* A name for a local area, such as a city or town name. For example, Toronto
.
*
*
* @param municipality
* A name for a local area, such as a city or town name. For example, Toronto
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withMunicipality(String municipality) {
setMunicipality(municipality);
return this;
}
/**
*
* A county, or an area that's part of a larger region. For example, Metro Vancouver
.
*
*
* @param subRegion
* A county, or an area that's part of a larger region. For example, Metro Vancouver
.
*/
public void setSubRegion(String subRegion) {
this.subRegion = subRegion;
}
/**
*
* A county, or an area that's part of a larger region. For example, Metro Vancouver
.
*
*
* @return A county, or an area that's part of a larger region. For example, Metro Vancouver
.
*/
public String getSubRegion() {
return this.subRegion;
}
/**
*
* A county, or an area that's part of a larger region. For example, Metro Vancouver
.
*
*
* @param subRegion
* A county, or an area that's part of a larger region. For example, Metro Vancouver
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withSubRegion(String subRegion) {
setSubRegion(subRegion);
return this;
}
/**
*
* A name for an area or geographical division, such as a province or state name. For example,
* British Columbia
.
*
*
* @param region
* A name for an area or geographical division, such as a province or state name. For example,
* British Columbia
.
*/
public void setRegion(String region) {
this.region = region;
}
/**
*
* A name for an area or geographical division, such as a province or state name. For example,
* British Columbia
.
*
*
* @return A name for an area or geographical division, such as a province or state name. For example,
* British Columbia
.
*/
public String getRegion() {
return this.region;
}
/**
*
* A name for an area or geographical division, such as a province or state name. For example,
* British Columbia
.
*
*
* @param region
* A name for an area or geographical division, such as a province or state name. For example,
* British Columbia
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withRegion(String region) {
setRegion(region);
return this;
}
/**
*
* A country/region specified using ISO 3166 3-digit
* country/region code. For example, CAN
.
*
*
* @param country
* A country/region specified using ISO 3166
* 3-digit country/region code. For example, CAN
.
*/
public void setCountry(String country) {
this.country = country;
}
/**
*
* A country/region specified using ISO 3166 3-digit
* country/region code. For example, CAN
.
*
*
* @return A country/region specified using ISO 3166
* 3-digit country/region code. For example, CAN
.
*/
public String getCountry() {
return this.country;
}
/**
*
* A country/region specified using ISO 3166 3-digit
* country/region code. For example, CAN
.
*
*
* @param country
* A country/region specified using ISO 3166
* 3-digit country/region code. For example, CAN
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withCountry(String country) {
setCountry(country);
return this;
}
/**
*
* A group of numbers and letters in a country-specific format, which accompanies the address for the purpose of
* identifying a location.
*
*
* @param postalCode
* A group of numbers and letters in a country-specific format, which accompanies the address for the purpose
* of identifying a location.
*/
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
/**
*
* A group of numbers and letters in a country-specific format, which accompanies the address for the purpose of
* identifying a location.
*
*
* @return A group of numbers and letters in a country-specific format, which accompanies the address for the
* purpose of identifying a location.
*/
public String getPostalCode() {
return this.postalCode;
}
/**
*
* A group of numbers and letters in a country-specific format, which accompanies the address for the purpose of
* identifying a location.
*
*
* @param postalCode
* A group of numbers and letters in a country-specific format, which accompanies the address for the purpose
* of identifying a location.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withPostalCode(String postalCode) {
setPostalCode(postalCode);
return this;
}
/**
*
* True
if the result is interpolated from other known places.
*
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but returns
* True
if an address does not exist in the partner data and its location is calculated by
* interpolating between other known addresses.
*
*
* @param interpolated
* True
if the result is interpolated from other known places.
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but
* returns True
if an address does not exist in the partner data and its location is calculated
* by interpolating between other known addresses.
*/
public void setInterpolated(Boolean interpolated) {
this.interpolated = interpolated;
}
/**
*
* True
if the result is interpolated from other known places.
*
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but returns
* True
if an address does not exist in the partner data and its location is calculated by
* interpolating between other known addresses.
*
*
* @return True
if the result is interpolated from other known places.
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but
* returns True
if an address does not exist in the partner data and its location is calculated
* by interpolating between other known addresses.
*/
public Boolean getInterpolated() {
return this.interpolated;
}
/**
*
* True
if the result is interpolated from other known places.
*
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but returns
* True
if an address does not exist in the partner data and its location is calculated by
* interpolating between other known addresses.
*
*
* @param interpolated
* True
if the result is interpolated from other known places.
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but
* returns True
if an address does not exist in the partner data and its location is calculated
* by interpolating between other known addresses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withInterpolated(Boolean interpolated) {
setInterpolated(interpolated);
return this;
}
/**
*
* True
if the result is interpolated from other known places.
*
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but returns
* True
if an address does not exist in the partner data and its location is calculated by
* interpolating between other known addresses.
*
*
* @return True
if the result is interpolated from other known places.
*
* False
if the Place is a known place.
*
*
* Not returned when the partner does not provide the information.
*
*
* For example, returns False
for an address location that is found in the partner data, but
* returns True
if an address does not exist in the partner data and its location is calculated
* by interpolating between other known addresses.
*/
public Boolean isInterpolated() {
return this.interpolated;
}
/**
*
* The time zone in which the Place
is located. Returned only when using HERE or Grab as the selected
* partner.
*
*
* @param timeZone
* The time zone in which the Place
is located. Returned only when using HERE or Grab as the
* selected partner.
*/
public void setTimeZone(TimeZone timeZone) {
this.timeZone = timeZone;
}
/**
*
* The time zone in which the Place
is located. Returned only when using HERE or Grab as the selected
* partner.
*
*
* @return The time zone in which the Place
is located. Returned only when using HERE or Grab as the
* selected partner.
*/
public TimeZone getTimeZone() {
return this.timeZone;
}
/**
*
* The time zone in which the Place
is located. Returned only when using HERE or Grab as the selected
* partner.
*
*
* @param timeZone
* The time zone in which the Place
is located. Returned only when using HERE or Grab as the
* selected partner.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withTimeZone(TimeZone timeZone) {
setTimeZone(timeZone);
return this;
}
/**
*
* For addresses with a UnitNumber
, the type of unit. For example, Apartment
.
*
*
*
* Returned only for a place index that uses Esri as a data provider.
*
*
*
* @param unitType
* For addresses with a UnitNumber
, the type of unit. For example, Apartment
.
*
*
* Returned only for a place index that uses Esri as a data provider.
*
*/
public void setUnitType(String unitType) {
this.unitType = unitType;
}
/**
*
* For addresses with a UnitNumber
, the type of unit. For example, Apartment
.
*
*
*
* Returned only for a place index that uses Esri as a data provider.
*
*
*
* @return For addresses with a UnitNumber
, the type of unit. For example, Apartment
.
*
*
* Returned only for a place index that uses Esri as a data provider.
*
*/
public String getUnitType() {
return this.unitType;
}
/**
*
* For addresses with a UnitNumber
, the type of unit. For example, Apartment
.
*
*
*
* Returned only for a place index that uses Esri as a data provider.
*
*
*
* @param unitType
* For addresses with a UnitNumber
, the type of unit. For example, Apartment
.
*
*
* Returned only for a place index that uses Esri as a data provider.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withUnitType(String unitType) {
setUnitType(unitType);
return this;
}
/**
*
* For addresses with multiple units, the unit identifier. Can include numbers and letters, for example
* 3B
or Unit 123
.
*
*
*
* Returned only for a place index that uses Esri or Grab as a data provider. Is not returned for
* SearchPlaceIndexForPosition
.
*
*
*
* @param unitNumber
* For addresses with multiple units, the unit identifier. Can include numbers and letters, for example
* 3B
or Unit 123
.
*
* Returned only for a place index that uses Esri or Grab as a data provider. Is not returned for
* SearchPlaceIndexForPosition
.
*
*/
public void setUnitNumber(String unitNumber) {
this.unitNumber = unitNumber;
}
/**
*
* For addresses with multiple units, the unit identifier. Can include numbers and letters, for example
* 3B
or Unit 123
.
*
*
*
* Returned only for a place index that uses Esri or Grab as a data provider. Is not returned for
* SearchPlaceIndexForPosition
.
*
*
*
* @return For addresses with multiple units, the unit identifier. Can include numbers and letters, for example
* 3B
or Unit 123
.
*
* Returned only for a place index that uses Esri or Grab as a data provider. Is not returned for
* SearchPlaceIndexForPosition
.
*
*/
public String getUnitNumber() {
return this.unitNumber;
}
/**
*
* For addresses with multiple units, the unit identifier. Can include numbers and letters, for example
* 3B
or Unit 123
.
*
*
*
* Returned only for a place index that uses Esri or Grab as a data provider. Is not returned for
* SearchPlaceIndexForPosition
.
*
*
*
* @param unitNumber
* For addresses with multiple units, the unit identifier. Can include numbers and letters, for example
* 3B
or Unit 123
.
*
* Returned only for a place index that uses Esri or Grab as a data provider. Is not returned for
* SearchPlaceIndexForPosition
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withUnitNumber(String unitNumber) {
setUnitNumber(unitNumber);
return this;
}
/**
*
* The Amazon Location categories that describe this Place.
*
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
*
*
* @return The Amazon Location categories that describe this Place.
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
*/
public java.util.List getCategories() {
return categories;
}
/**
*
* The Amazon Location categories that describe this Place.
*
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
*
*
* @param categories
* The Amazon Location categories that describe this Place.
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
*/
public void setCategories(java.util.Collection categories) {
if (categories == null) {
this.categories = null;
return;
}
this.categories = new java.util.ArrayList(categories);
}
/**
*
* The Amazon Location categories that describe this Place.
*
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCategories(java.util.Collection)} or {@link #withCategories(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param categories
* The Amazon Location categories that describe this Place.
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withCategories(String... categories) {
if (this.categories == null) {
setCategories(new java.util.ArrayList(categories.length));
}
for (String ele : categories) {
this.categories.add(ele);
}
return this;
}
/**
*
* The Amazon Location categories that describe this Place.
*
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
*
*
* @param categories
* The Amazon Location categories that describe this Place.
*
* For more information about using categories, including a list of Amazon Location categories, see Categories and
* filtering, in the Amazon Location Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withCategories(java.util.Collection categories) {
setCategories(categories);
return this;
}
/**
*
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location categories.
*
*
* @return Categories from the data provider that describe the Place that are not mapped to any Amazon Location
* categories.
*/
public java.util.List getSupplementalCategories() {
return supplementalCategories;
}
/**
*
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location categories.
*
*
* @param supplementalCategories
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location
* categories.
*/
public void setSupplementalCategories(java.util.Collection supplementalCategories) {
if (supplementalCategories == null) {
this.supplementalCategories = null;
return;
}
this.supplementalCategories = new java.util.ArrayList(supplementalCategories);
}
/**
*
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location categories.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupplementalCategories(java.util.Collection)} or
* {@link #withSupplementalCategories(java.util.Collection)} if you want to override the existing values.
*
*
* @param supplementalCategories
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location
* categories.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withSupplementalCategories(String... supplementalCategories) {
if (this.supplementalCategories == null) {
setSupplementalCategories(new java.util.ArrayList(supplementalCategories.length));
}
for (String ele : supplementalCategories) {
this.supplementalCategories.add(ele);
}
return this;
}
/**
*
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location categories.
*
*
* @param supplementalCategories
* Categories from the data provider that describe the Place that are not mapped to any Amazon Location
* categories.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withSupplementalCategories(java.util.Collection supplementalCategories) {
setSupplementalCategories(supplementalCategories);
return this;
}
/**
*
* An area that's part of a larger municipality. For example, Blissville
is a submunicipality in the
* Queen County in New York.
*
*
*
* This property supported by Esri and OpenData. The Esri property is district
, and the OpenData
* property is borough
.
*
*
*
* @param subMunicipality
* An area that's part of a larger municipality. For example, Blissville
is a submunicipality
* in the Queen County in New York.
*
* This property supported by Esri and OpenData. The Esri property is district
, and the OpenData
* property is borough
.
*
*/
public void setSubMunicipality(String subMunicipality) {
this.subMunicipality = subMunicipality;
}
/**
*
* An area that's part of a larger municipality. For example, Blissville
is a submunicipality in the
* Queen County in New York.
*
*
*
* This property supported by Esri and OpenData. The Esri property is district
, and the OpenData
* property is borough
.
*
*
*
* @return An area that's part of a larger municipality. For example, Blissville
is a submunicipality
* in the Queen County in New York.
*
* This property supported by Esri and OpenData. The Esri property is district
, and the
* OpenData property is borough
.
*
*/
public String getSubMunicipality() {
return this.subMunicipality;
}
/**
*
* An area that's part of a larger municipality. For example, Blissville
is a submunicipality in the
* Queen County in New York.
*
*
*
* This property supported by Esri and OpenData. The Esri property is district
, and the OpenData
* property is borough
.
*
*
*
* @param subMunicipality
* An area that's part of a larger municipality. For example, Blissville
is a submunicipality
* in the Queen County in New York.
*
* This property supported by Esri and OpenData. The Esri property is district
, and the OpenData
* property is borough
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Place withSubMunicipality(String subMunicipality) {
setSubMunicipality(subMunicipality);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getLabel() != null)
sb.append("Label: ").append(getLabel()).append(",");
if (getGeometry() != null)
sb.append("Geometry: ").append(getGeometry()).append(",");
if (getAddressNumber() != null)
sb.append("AddressNumber: ").append(getAddressNumber()).append(",");
if (getStreet() != null)
sb.append("Street: ").append(getStreet()).append(",");
if (getNeighborhood() != null)
sb.append("Neighborhood: ").append(getNeighborhood()).append(",");
if (getMunicipality() != null)
sb.append("Municipality: ").append(getMunicipality()).append(",");
if (getSubRegion() != null)
sb.append("SubRegion: ").append(getSubRegion()).append(",");
if (getRegion() != null)
sb.append("Region: ").append(getRegion()).append(",");
if (getCountry() != null)
sb.append("Country: ").append(getCountry()).append(",");
if (getPostalCode() != null)
sb.append("PostalCode: ").append(getPostalCode()).append(",");
if (getInterpolated() != null)
sb.append("Interpolated: ").append(getInterpolated()).append(",");
if (getTimeZone() != null)
sb.append("TimeZone: ").append(getTimeZone()).append(",");
if (getUnitType() != null)
sb.append("UnitType: ").append(getUnitType()).append(",");
if (getUnitNumber() != null)
sb.append("UnitNumber: ").append(getUnitNumber()).append(",");
if (getCategories() != null)
sb.append("Categories: ").append(getCategories()).append(",");
if (getSupplementalCategories() != null)
sb.append("SupplementalCategories: ").append(getSupplementalCategories()).append(",");
if (getSubMunicipality() != null)
sb.append("SubMunicipality: ").append(getSubMunicipality());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Place == false)
return false;
Place other = (Place) obj;
if (other.getLabel() == null ^ this.getLabel() == null)
return false;
if (other.getLabel() != null && other.getLabel().equals(this.getLabel()) == false)
return false;
if (other.getGeometry() == null ^ this.getGeometry() == null)
return false;
if (other.getGeometry() != null && other.getGeometry().equals(this.getGeometry()) == false)
return false;
if (other.getAddressNumber() == null ^ this.getAddressNumber() == null)
return false;
if (other.getAddressNumber() != null && other.getAddressNumber().equals(this.getAddressNumber()) == false)
return false;
if (other.getStreet() == null ^ this.getStreet() == null)
return false;
if (other.getStreet() != null && other.getStreet().equals(this.getStreet()) == false)
return false;
if (other.getNeighborhood() == null ^ this.getNeighborhood() == null)
return false;
if (other.getNeighborhood() != null && other.getNeighborhood().equals(this.getNeighborhood()) == false)
return false;
if (other.getMunicipality() == null ^ this.getMunicipality() == null)
return false;
if (other.getMunicipality() != null && other.getMunicipality().equals(this.getMunicipality()) == false)
return false;
if (other.getSubRegion() == null ^ this.getSubRegion() == null)
return false;
if (other.getSubRegion() != null && other.getSubRegion().equals(this.getSubRegion()) == false)
return false;
if (other.getRegion() == null ^ this.getRegion() == null)
return false;
if (other.getRegion() != null && other.getRegion().equals(this.getRegion()) == false)
return false;
if (other.getCountry() == null ^ this.getCountry() == null)
return false;
if (other.getCountry() != null && other.getCountry().equals(this.getCountry()) == false)
return false;
if (other.getPostalCode() == null ^ this.getPostalCode() == null)
return false;
if (other.getPostalCode() != null && other.getPostalCode().equals(this.getPostalCode()) == false)
return false;
if (other.getInterpolated() == null ^ this.getInterpolated() == null)
return false;
if (other.getInterpolated() != null && other.getInterpolated().equals(this.getInterpolated()) == false)
return false;
if (other.getTimeZone() == null ^ this.getTimeZone() == null)
return false;
if (other.getTimeZone() != null && other.getTimeZone().equals(this.getTimeZone()) == false)
return false;
if (other.getUnitType() == null ^ this.getUnitType() == null)
return false;
if (other.getUnitType() != null && other.getUnitType().equals(this.getUnitType()) == false)
return false;
if (other.getUnitNumber() == null ^ this.getUnitNumber() == null)
return false;
if (other.getUnitNumber() != null && other.getUnitNumber().equals(this.getUnitNumber()) == false)
return false;
if (other.getCategories() == null ^ this.getCategories() == null)
return false;
if (other.getCategories() != null && other.getCategories().equals(this.getCategories()) == false)
return false;
if (other.getSupplementalCategories() == null ^ this.getSupplementalCategories() == null)
return false;
if (other.getSupplementalCategories() != null && other.getSupplementalCategories().equals(this.getSupplementalCategories()) == false)
return false;
if (other.getSubMunicipality() == null ^ this.getSubMunicipality() == null)
return false;
if (other.getSubMunicipality() != null && other.getSubMunicipality().equals(this.getSubMunicipality()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getLabel() == null) ? 0 : getLabel().hashCode());
hashCode = prime * hashCode + ((getGeometry() == null) ? 0 : getGeometry().hashCode());
hashCode = prime * hashCode + ((getAddressNumber() == null) ? 0 : getAddressNumber().hashCode());
hashCode = prime * hashCode + ((getStreet() == null) ? 0 : getStreet().hashCode());
hashCode = prime * hashCode + ((getNeighborhood() == null) ? 0 : getNeighborhood().hashCode());
hashCode = prime * hashCode + ((getMunicipality() == null) ? 0 : getMunicipality().hashCode());
hashCode = prime * hashCode + ((getSubRegion() == null) ? 0 : getSubRegion().hashCode());
hashCode = prime * hashCode + ((getRegion() == null) ? 0 : getRegion().hashCode());
hashCode = prime * hashCode + ((getCountry() == null) ? 0 : getCountry().hashCode());
hashCode = prime * hashCode + ((getPostalCode() == null) ? 0 : getPostalCode().hashCode());
hashCode = prime * hashCode + ((getInterpolated() == null) ? 0 : getInterpolated().hashCode());
hashCode = prime * hashCode + ((getTimeZone() == null) ? 0 : getTimeZone().hashCode());
hashCode = prime * hashCode + ((getUnitType() == null) ? 0 : getUnitType().hashCode());
hashCode = prime * hashCode + ((getUnitNumber() == null) ? 0 : getUnitNumber().hashCode());
hashCode = prime * hashCode + ((getCategories() == null) ? 0 : getCategories().hashCode());
hashCode = prime * hashCode + ((getSupplementalCategories() == null) ? 0 : getSupplementalCategories().hashCode());
hashCode = prime * hashCode + ((getSubMunicipality() == null) ? 0 : getSubMunicipality().hashCode());
return hashCode;
}
@Override
public Place clone() {
try {
return (Place) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.location.model.transform.PlaceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}